#include <sys/types.h>
#include <stdio.h>
#include <sys/stat.h>
#include <unistd.h>
#include <sys/mman.h>
#include <fcntl.h>
#include <dirent.h>
#include <stdlib.h>
#include <errno.h>
#include <string.h>
#include <stdarg.h>
#include <linux/axfs_fs.h>
#include <zlib.h>
/* Library to parse the input XML file */
#include <libxml/xmlmemory.h>
#include <libxml/parser.h>
#include <libxml/tree.h>
#include <libxml/xpath.h>
/* Exit codes used by mkfs-type programs */
#define MKFS_OK 0 /* No errors */
#define MKFS_ERROR 8 /* Operational error */
#define MKFS_USAGE 16 /* Usage or syntax error */
#define PARSE_OK 1 /* No parse errors */
#define PARSE_ERROR 2 /* Parse error */
/* The kernel assumes PAGE_CACHE_SIZE as block size. */
#define PAGE_CACHE_SIZE (4096)
#define MAX_INPUT_NAMELEN 255
/*
* Maximum size fs you can create is roughly 256MB. (The last file's
* data must begin within 256MB boundary but can extend beyond that.)
*
* Note that if you want it to fit in a ROM then you're limited to what the
* hardware and kernel can support.
*/
#define MAXFSLEN ((((1 << AXFS_OFFSET_WIDTH) - 1) << 2) /* offset */ \
+ (1 << AXFS_SIZE_WIDTH) - 1 /* filesize */ \
+ (1 << AXFS_SIZE_WIDTH) * 8 / PAGE_CACHE_SIZE /* block pointers */)
#define DEBUG_MODE FALSE
#if DEBUG_MODE
#define DEBUG(x) printf(x)
#else
#define DEBUG(x)
#endif
/* Info of a chunk to be XIPed */
typedef struct xipchunk {
u32 size;
u32 offset;
} xipchunk, *xipchunkPtr;
/* A file with xip chunks */
typedef struct xipfile {
char *path;
u32 chunknb;
u32 index; /* index of the first chunk */
} xipfile, *xipfilePtr;
/* In-core version of inode / directory entry. */
struct entry {
u8 *name;
/* stats */
u32 mode;
u32 size;
u32 uid;
u32 gid;
/* these are only used for non-empty files */
char *path; /* always null except non-empty files */
int fd; /* temporarily open files while mmapped */
void *uncompressed; /* FS data */
u8 *bitmap; /* XIP info */
/* points to other identical file */
struct entry *same;
/* organization */
struct entry *child; /* null for non-directories and empty directories */
struct entry *next;
u32 total_entries; /* for dir: # of children; for file: # of node */
/* for non-empty file only, offset to the node table */
u32 array_offset;
};
/*
* Total # of files with XIP chunks
* and total # of chunks to be XIPed
*/
static u32 total_xipfiles, total_xipchunks;
static u32 next_xipchunk;
/*
* Array for all xip chunks
* and array for all valid xip files
*/
static xipchunkPtr xipchunkset = NULL;
static xipfilePtr xipfileset = NULL;
static const char *progname = "mkaxfs";
static unsigned int blksize = PAGE_CACHE_SIZE;
static int warn_dev, warn_gid, warn_namelen, warn_skip, warn_size, warn_uid;
/* Super block */
static struct axfs_super sb;
/* Total # of nodes and total # of inodes */
static u32 total_nodes; /* one node for one block */
static u32 total_inodes; /* one inode for one file/dir */
static u32 total_xipnodes;
/* Name size including '/0' */
static u32 total_namesize;
static u32 cur_xipoffset;
static u32 cur_baoffset;
/*****************************************************************************
*
* usage
*
* Description:
* Prints out the usage of this tool.
*
* Parameters:
*
* Returns:
*
* Assumptions:
*
****************************************************************************/
static void usage(int status)
{
FILE *stream = status ? stderr : stdout;
fprintf(stream, "usage: %s [-h] [-i infile] dirname outfile\n"
" -h print this help\n"
" -i infile input file of the XIP information\n"
" dirname root of the directory tree to be compressed\n"
" outfile output file\n", progname);
exit(status);
}
/*****************************************************************************
*
* die
*
* Description:
* Reports the error and exits.
*
* Parameters:
*
* Returns:
*
* Assumptions:
*
****************************************************************************/
static void die(int status, int syserr, const char *fmt, ...)
{
va_list arg_ptr;
int save = errno;
fflush(0);
va_start(arg_ptr, fmt);
fprintf(stderr, "%s: ", progname);
vfprintf(stderr, fmt, arg_ptr);
if (syserr) {
fprintf(stderr, ": %s", strerror(save));
}
fprintf(stderr, "\n");
va_end(arg_ptr);
exit(status);
}
/*****************************************************************************
*
* getnodenb
*
* Description:
* Get the total number of nodes with specific name.
*
* Parameters:
* (IN) doc - output of libxml2 for the XML file
*
* (IN) xpath - node name
*
* Returns:
* Number of nodes found in the XML file.
*
* Assumptions:
*
****************************************************************************/
static u32 getnodenb(xmlDocPtr doc, xmlChar *xpath)
{
xmlXPathContextPtr context;
xmlXPathObjectPtr result;
u32 nodenb;
context = xmlXPathNewContext(doc);
result = xmlXPathEvalExpression(xpath, context);
if(xmlXPathNodeSetIsEmpty(result->nodesetval)) {
return 0;
}
nodenb = result->nodesetval->nodeNr;
xmlXPathFreeContext(context);
xmlXPathFreeObject (result);
return nodenb;
}
/*****************************************************************************
*
* parseXipchunk
*
* Description:
* Parses the content of one <chunk> node.
*
* Parameters:
* (IN) doc - output of libxml2 for the XML file
*
* (IN) cur - current <chunk> node
*
* (OUT) chk - pointer to the xipchunk structure
*
* Returns:
* PARSE_OK
* PARSE_ERROR
*
* Assumptions:
*
****************************************************************************/
static int parseXipchunk(xmlDocPtr doc, xmlNodePtr cur, xipchunkPtr chk)
{
xmlChar *tmp;
char *ep; /* for strtoul */
chk->offset = 0;
chk->size = 0;
/* We don't care what the top level element name is */
cur = cur->xmlChildrenNode;
while (cur != NULL) {
if (!xmlStrcmp(cur->name, (const xmlChar *)"size")) {
errno = 0;
tmp = xmlNodeListGetString(doc, cur->xmlChildrenNode, 1);
chk->size = strtoul(tmp, &ep, 10);
if (errno || tmp[0] == '\0' || *ep != '\0' || chk->size == 0) {
fprintf(stderr, "input file error: invalid size\n");
return PARSE_ERROR;
}
}
if (!xmlStrcmp(cur->name, (const xmlChar *)"offset")) {
errno = 0;
tmp = xmlNodeListGetString(doc, cur->xmlChildrenNode, 1);
chk->offset = strtoul(tmp, &ep, 10);
if (errno || tmp[0] == '\0' || *ep != '\0') {
fprintf(stderr, "input file error: invalid offset\n");
return PARSE_ERROR;
}
}
cur = cur->next;
}
return PARSE_OK;
}
/*****************************************************************************
*
* parseXipfile
*
* Description:
* Parses the content of one <file> node.
*
* Parameters:
* (IN) doc - output of libxml2 for the XML file
*
* (IN) cur - current <file> node
*
* (OUT) fl - pointer to the xipfile structure
*
* (IN) dir - path of the root directory
*
* Returns:
* PARSE_OK
* PARSE_ERROR
*
* Assumptions:
*
****************************************************************************/
static int parseXipfile(xmlDocPtr doc, xmlNodePtr cur, xipfilePtr fl, char *dir)
{
struct stat st; /* for lstat */
u32 backup = next_xipchunk;
int ret;
char *path, *endpath, *namenode;
size_t len
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录



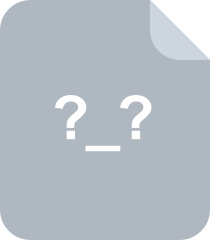
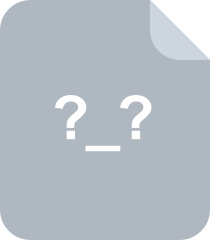
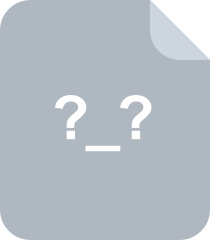
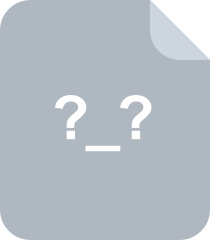


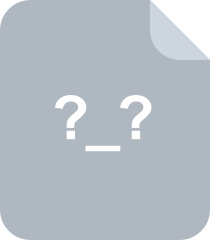
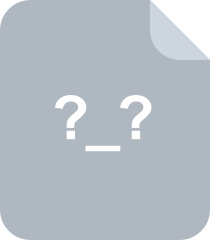
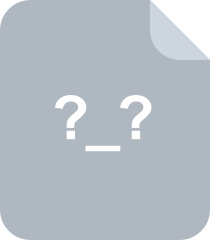
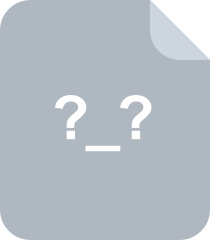
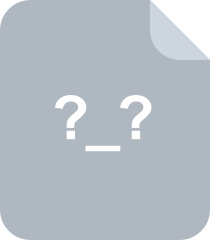


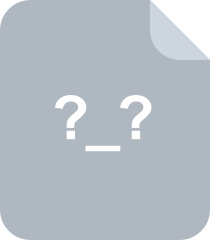
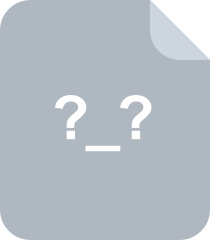



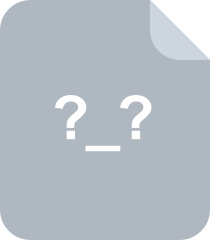

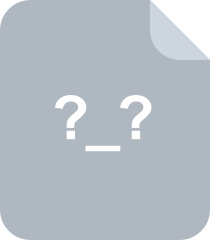
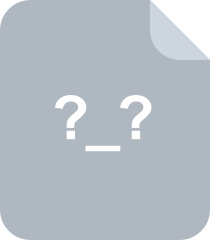
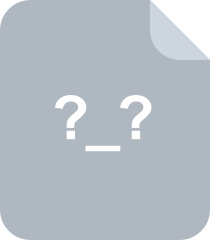


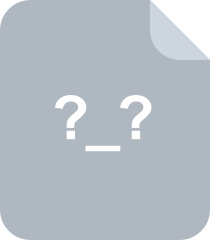
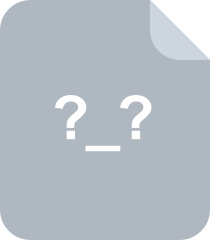
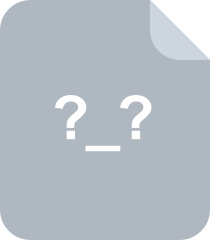
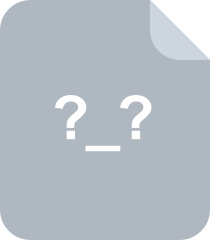
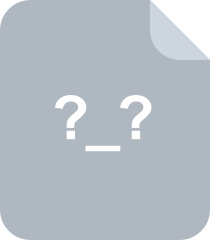


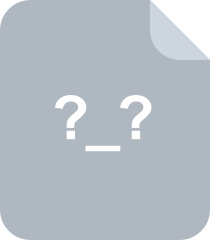
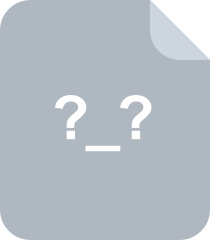
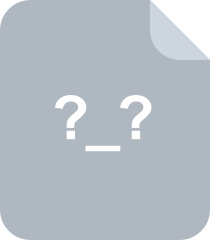
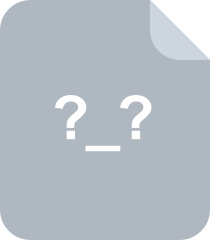

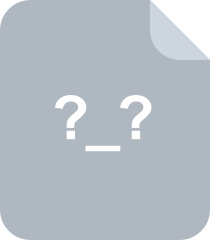
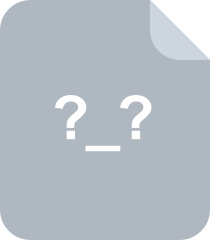
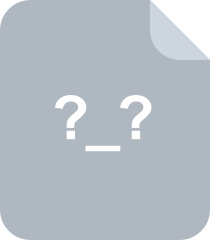
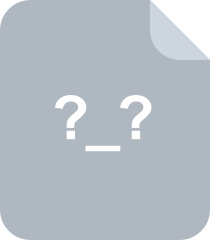
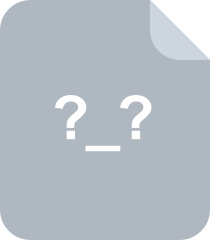
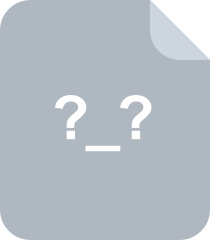
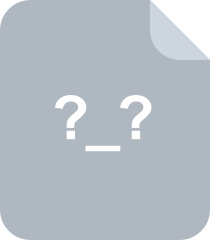


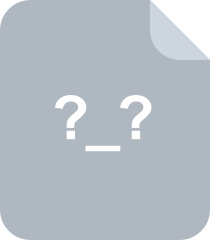


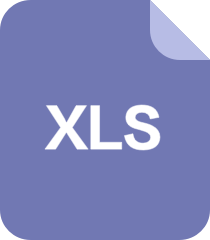

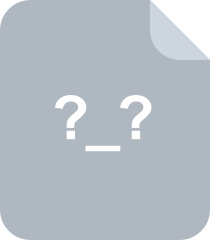
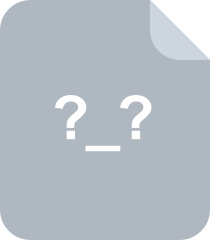
共 35 条
- 1
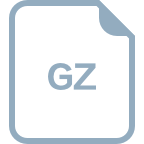
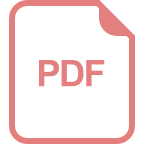
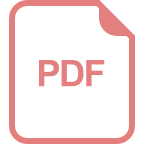
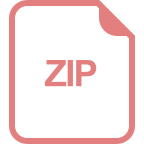
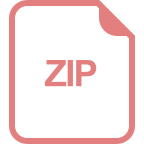
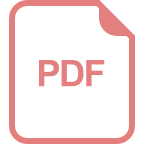
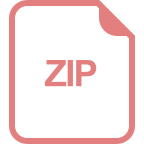
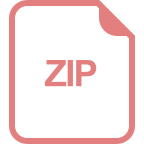
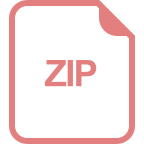
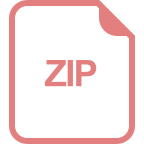
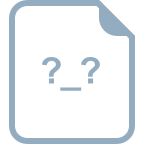
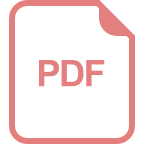
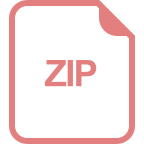
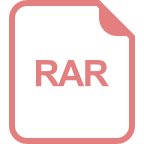

emmetyoung
- 粉丝: 5
- 资源: 16
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

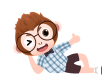
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


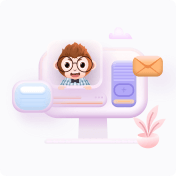
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0