vb.net 读取txt文件的几种方式
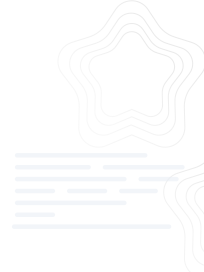


在VB.NET中,读取TXT文件是常见的任务,可以用于数据处理、日志分析等多种场景。下面我们将详细探讨几种不同的方法来实现这个功能。 1. **使用StreamReader** 使用`System.IO.StreamReader`类是最基本的读取文本文件的方式。以下是一个简单的示例: ```vb Dim filePath As String = "C:\path\to\file.txt" Using reader As New StreamReader(filePath) While Not reader.EndOfStream Dim line As String = reader.ReadLine() Console.WriteLine(line) End While End Using ``` 这个代码会逐行读取文件,并打印出每一行的内容。`Using`语句确保了资源在不再需要时被正确关闭和释放。 2. **使用File.ReadAllLines** 如果你需要一次性读取整个文件到一个字符串数组中,可以使用`File.ReadAllLines`函数: ```vb Dim filePath As String = "C:\path\to\file.txt" Dim lines() As String = File.ReadAllLines(filePath) For Each line In lines Console.WriteLine(line) Next ``` 这种方法适用于小文件,因为大文件可能会消耗大量内存。 3. **使用File.ReadAllText** 对于只需要读取整个文件内容的情况,可以使用`File.ReadAllText`,它将整个文件读入一个字符串: ```vb Dim filePath As String = "C:\path\to\file.txt" Dim content As String = File.ReadAllText(filePath) Console.WriteLine(content) ``` 这种方法适合文件较小,不需分多次读取的场景。 4. **使用FileStream和BinaryReader** `FileStream`和`BinaryReader`组合可以提供更多的控制,如读取特定字节或字符: ```vb Dim filePath As String = "C:\path\to\file.txt" Using stream As New FileStream(filePath, FileMode.Open, FileAccess.Read) Using reader As New BinaryReader(stream, Encoding.ASCII) While stream.Position < stream.Length Dim byteValue As Byte = reader.ReadByte() If byteValue <> &H0D Then ' 跳过回车符 Console.Write(Convert.ToChar(byteValue)) End If End While End Using End Using ``` 这个例子中,我们跳过了回车符,以避免在显示时产生额外的换行。 5. **异步读取** 在处理大文件或者需要非阻塞I/O操作时,可以使用异步方法,如`StreamReader.ReadLineAsync`: ```vb Dim filePath As String = "C:\path\to\file.txt" Using reader As New StreamReader(filePath) Do While True Dim line As String = Await reader.ReadLineAsync() If line Is Nothing Then Exit Do Console.WriteLine(line) Loop End Using ``` 异步读取允许其他任务在等待文件读取完成时继续执行,提高了程序的响应性。 6. **使用LINQ** 如果需要对文件内容进行复杂的查询操作,可以结合使用`File.ReadLines`和LINQ: ```vb Dim filePath As String = "C:\path\to\file.txt" Dim lines = File.ReadLines(filePath).Where(Function(l) l.StartsWith("Keyword")) For Each line In lines Console.WriteLine(line) Next ``` 这里,我们只显示以"Keyword"开头的行。 每种方法都有其适用的场景,选择哪种取决于你的具体需求,例如文件大小、是否需要逐行处理、是否需要进行异步操作等。在实际开发中,根据项目的性能要求和需求特性选择最合适的方法。
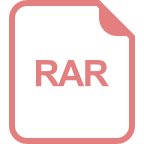
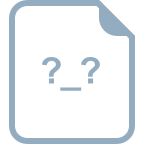
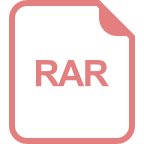
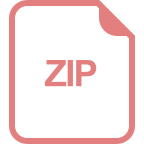
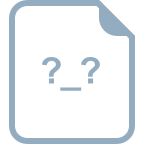
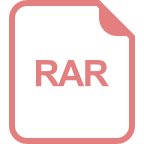
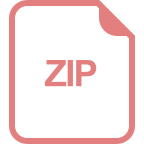
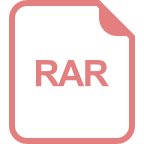
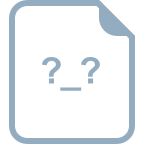



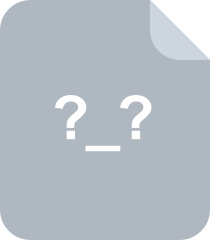


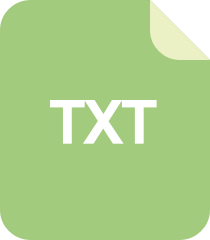
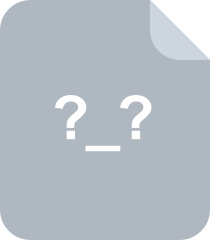
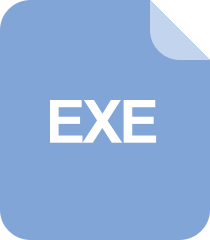
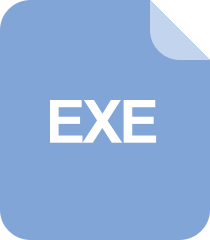
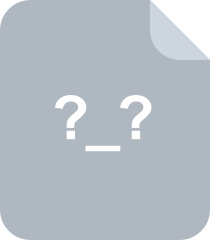
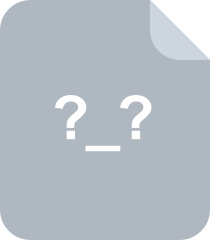


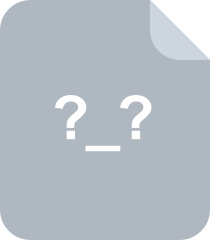
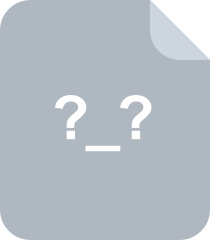
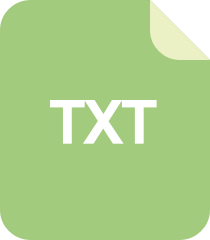

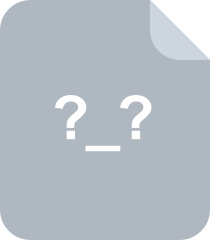
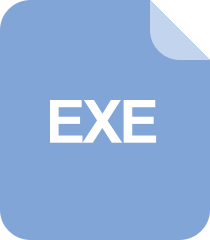
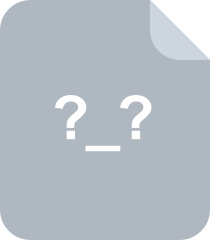
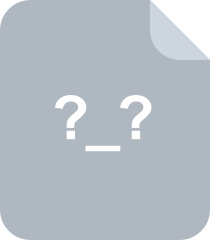

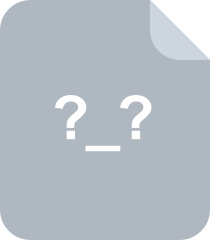
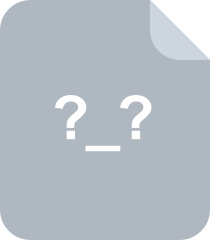
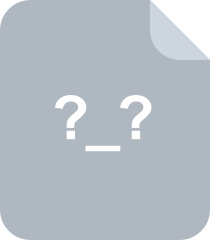
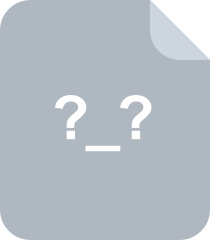
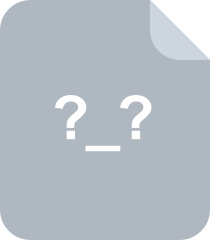
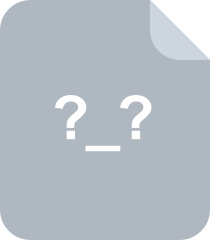
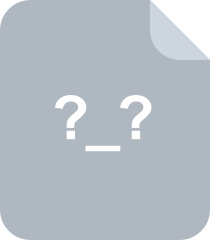
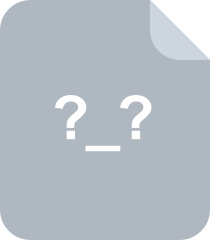
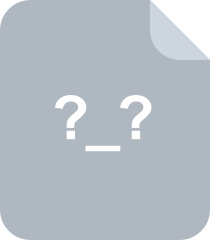
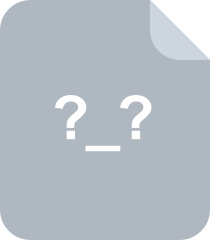
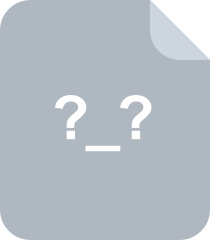
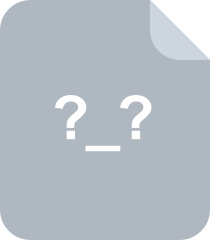
- 1
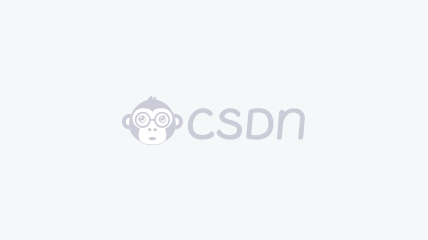
- yuanwen11962017-05-02能用,上面的那个可能是古人。我用的是VS2015我找了好多代码总还算找到这个能用的了~
- relax122242021-05-25可以用,感谢!
- qq_423757482019-10-26很好用,蟹蟹
- qq_395633052019-02-11有一点用处。
- lally3612017-03-29忽悠人呢吗!?根本打不开nimabi的

- 粉丝: 8
- 资源: 19
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

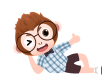
最新资源

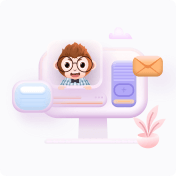
