没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
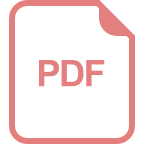
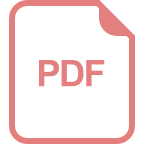
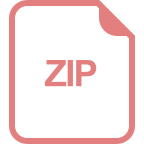
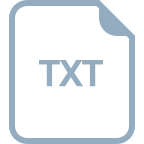
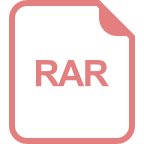
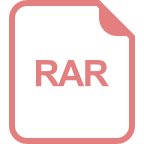
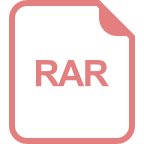
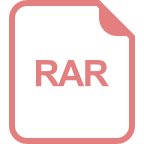
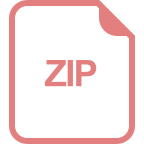
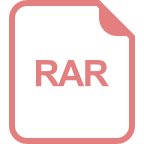
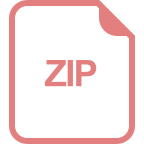
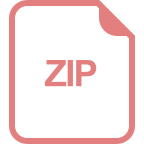
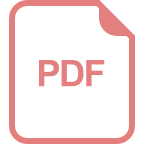
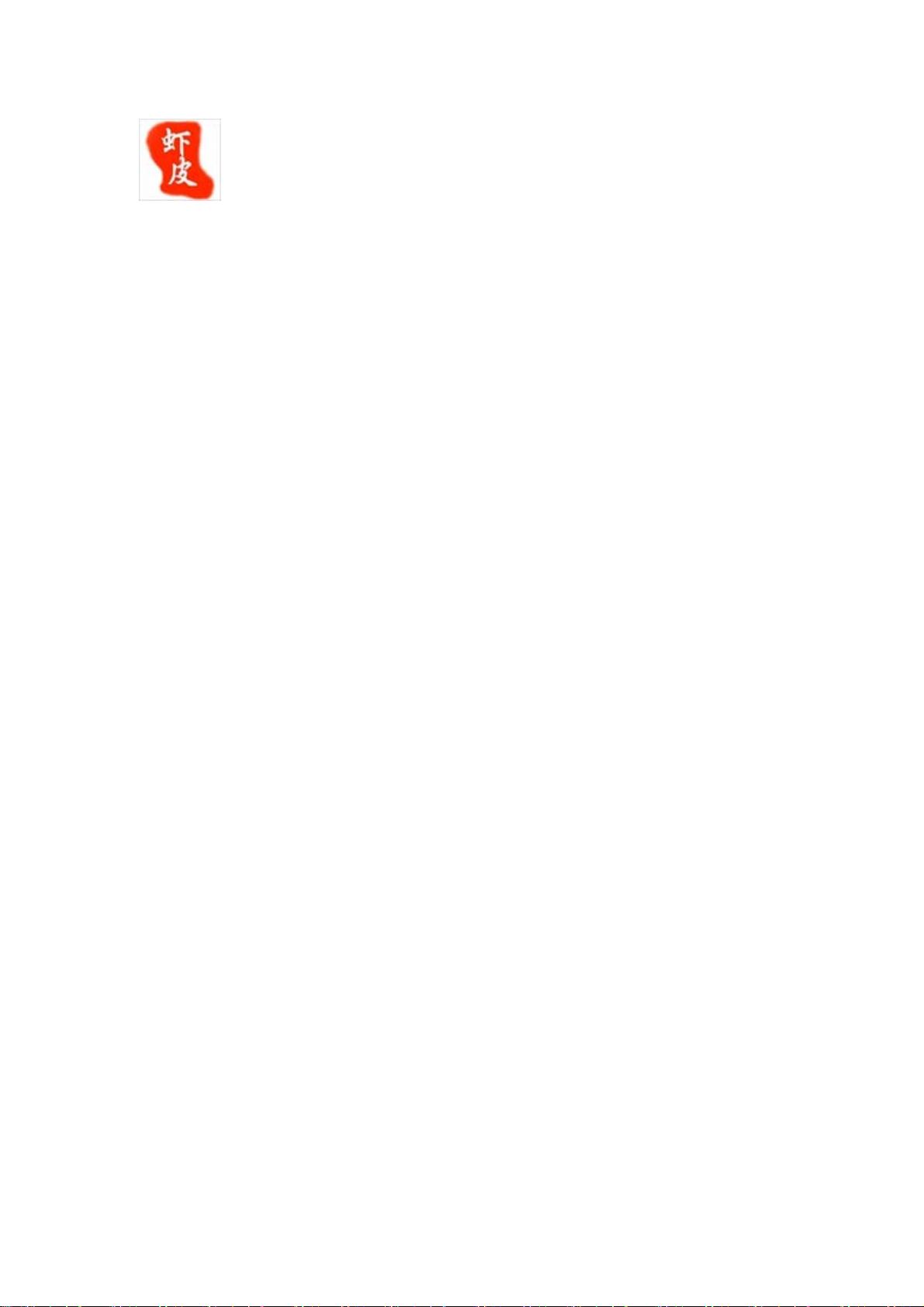
细细品味 C#
——抽象类、接口、委托、反射
精
华
集
锦
csAxp
虾皮工作室
http://www.cnblogs.com/xia520pi/
2011 年 7 月 29 日
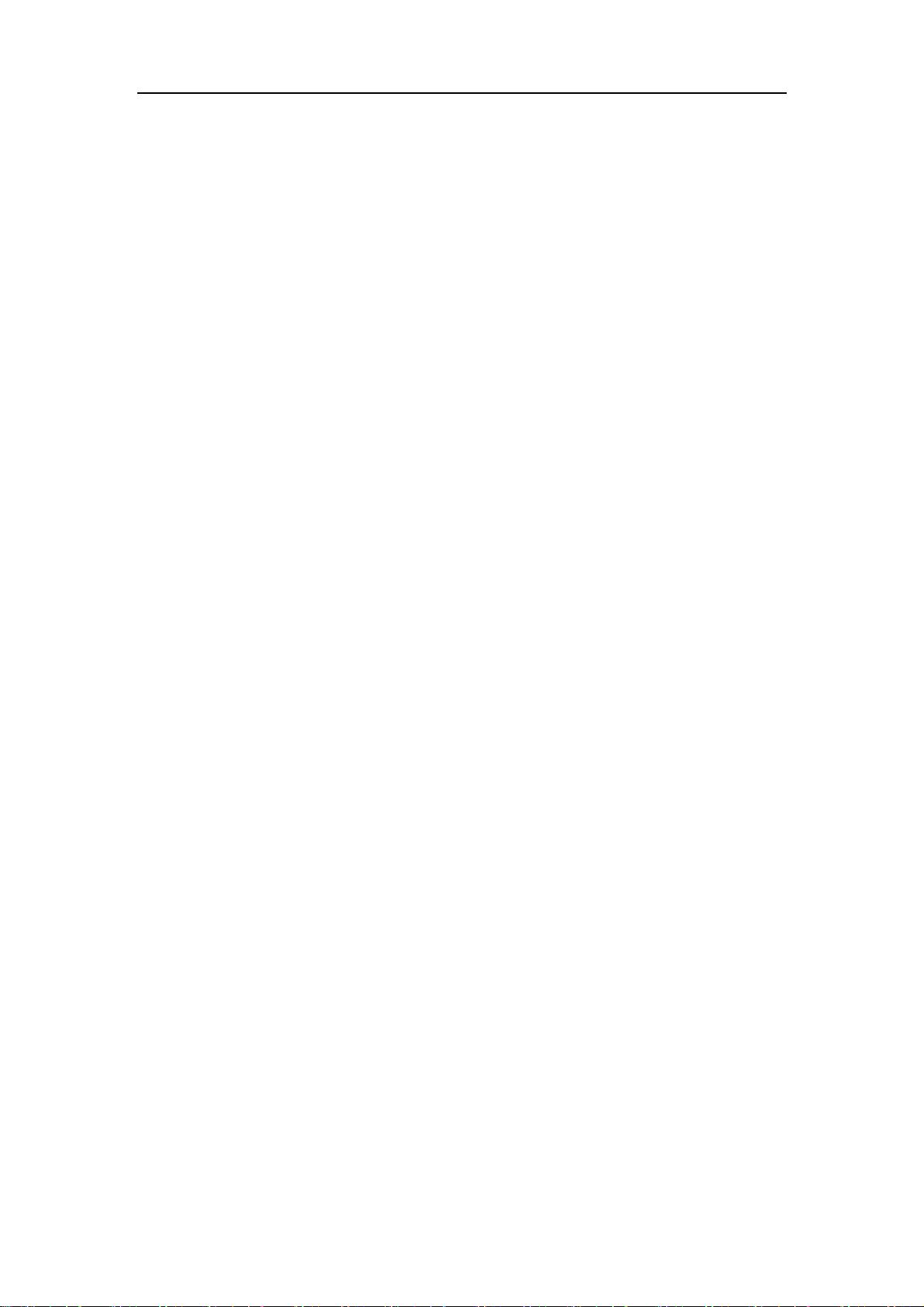
csAxp
河北工业大学——软件工程与理论 整理:虾皮
1
目录
1、抽象类与抽象方法..................................................................................................2
1.1、版权声明........................................................................................................2
1.2、内容详情........................................................................................................2
2、接口基础教程..........................................................................................................5
2.1、版权声明........................................................................................................5
2.2、内容详情........................................................................................................5
2.2.1、接口慨述..............................................................................................5
2.2.2、定义接口..............................................................................................7
2.2.3、定义接口成员....................................................................................10
2.2.4、访问接口............................................................................................12
2.2.5、实现接口............................................................................................16
2.2.6、接口转换............................................................................................28
2.2.7、覆盖虚接口........................................................................................34
3、抽象类与接口区别................................................................................................36
3.1、版权声明......................................................................................................36
3.2、内容详情......................................................................................................36
4、把委托说透............................................................................................................38
4.1、版权声明......................................................................................................38
4.2、内容详情......................................................................................................38
4.2.1、开始委托之旅 委托与接口..............................................................38
4.2.2、深入理解委托....................................................................................43
4.2.3、委托与事件........................................................................................51
4.2.4、委托与设计模式................................................................................57
5、反射........................................................................................................................63
5.1、版权声明......................................................................................................63
5.2、内容详情......................................................................................................63
5.2.1、序章....................................................................................................63
5.2.2、查看基本类型信息............................................................................75
5.2.3、反射特性............................................................................................89
5.2.4、动态创建类型实例............................................................................98
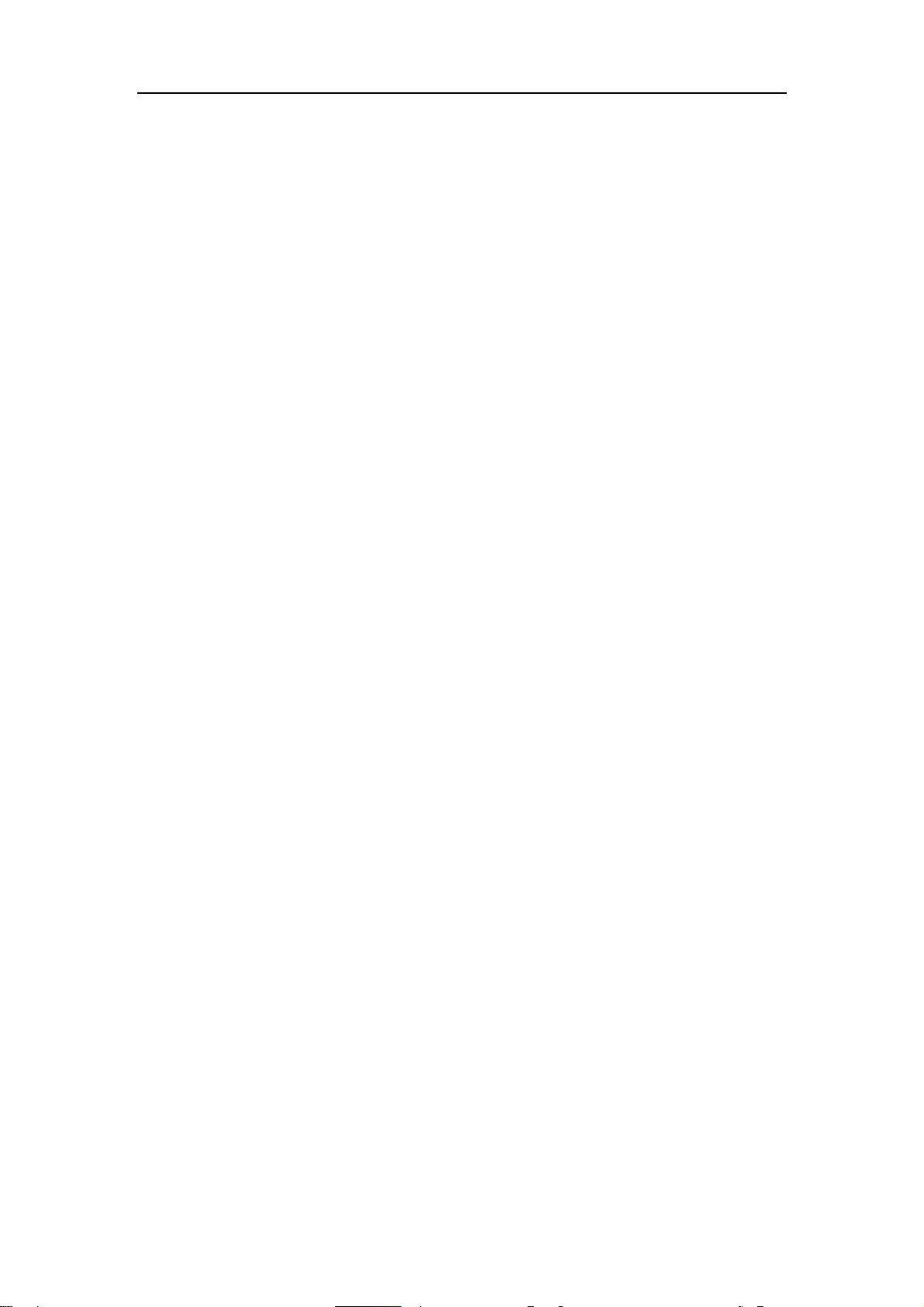
csAxp
河北工业大学——软件工程与理论 整理:虾皮
2
1、抽象类与抽象方法
1.1、版权声明
文章出处:http://www.cnblogs.com/wuhui369161243/archive/2009/03/29/1424677.html
文章作者:Me 丶紫龙
1.2、内容详情
朋友曾问我抽象类是否至少要有一个抽象方法,我查了很多资料,结果都是:“抽象类
允许(但不要求)抽象类包含抽象成员”。但是一个抽象类里不写抽象方法就没有意义了,
既然如此,还不如直接写个普通类? 在一个抽象类里可以不声明抽象方法,这在语法上是
没问题的,但实际来说,这样是没有任何意义的。也就是说,你为什么会选择写一个抽象类
呢?当然是为了想某个方法能够被 OVERRIDE,以实现多态。后来查找 MSDN 结果如下:
abstract 修饰符可以和类、方法、属性、索引器及事件一起使用。
在类声明中使用 abstract 修饰符以指示类只能是其他类的基类。
【抽象类】具有以下特性:
抽象类不能实例化。
抽象类可以包含抽象方法和抽象访问器。
不能用 sealed 修饰符修改抽象类,这意味着该类不能被继承。
从抽象类派生的非抽象类必须包括继承的所有抽象方法和抽象访问器的实实现。
在方法或属性声明中使用 abstract 修饰符以指示此方法或属性不包含实现。
【抽象方法】具有以下特性:
抽象方法是隐式的 virtual 方法。
只允许在抽象类中使用抽象方法声明。
因为抽象方法声明不提供实实现,所以没有方法体;方法声明只是以一个分号结束,
并且在签名后没有大括号 ({ })。例如:
public abstract void MyMethod();
实现由 overriding 方法提供,它是非抽象类的成员。
在抽象方法声明中使用 static 或 virtual 修饰符是错误的。
除了在声明和调用语法上不同外,抽象属性的行为与抽象方法一样。
在静态属性上使用 abstract 修饰符是错误的。
在派生类中,通过包括使用 ov
erride 修饰符的属性声明可以重写抽象的继承属性。
抽象类必须为所有接口成员提供实现。
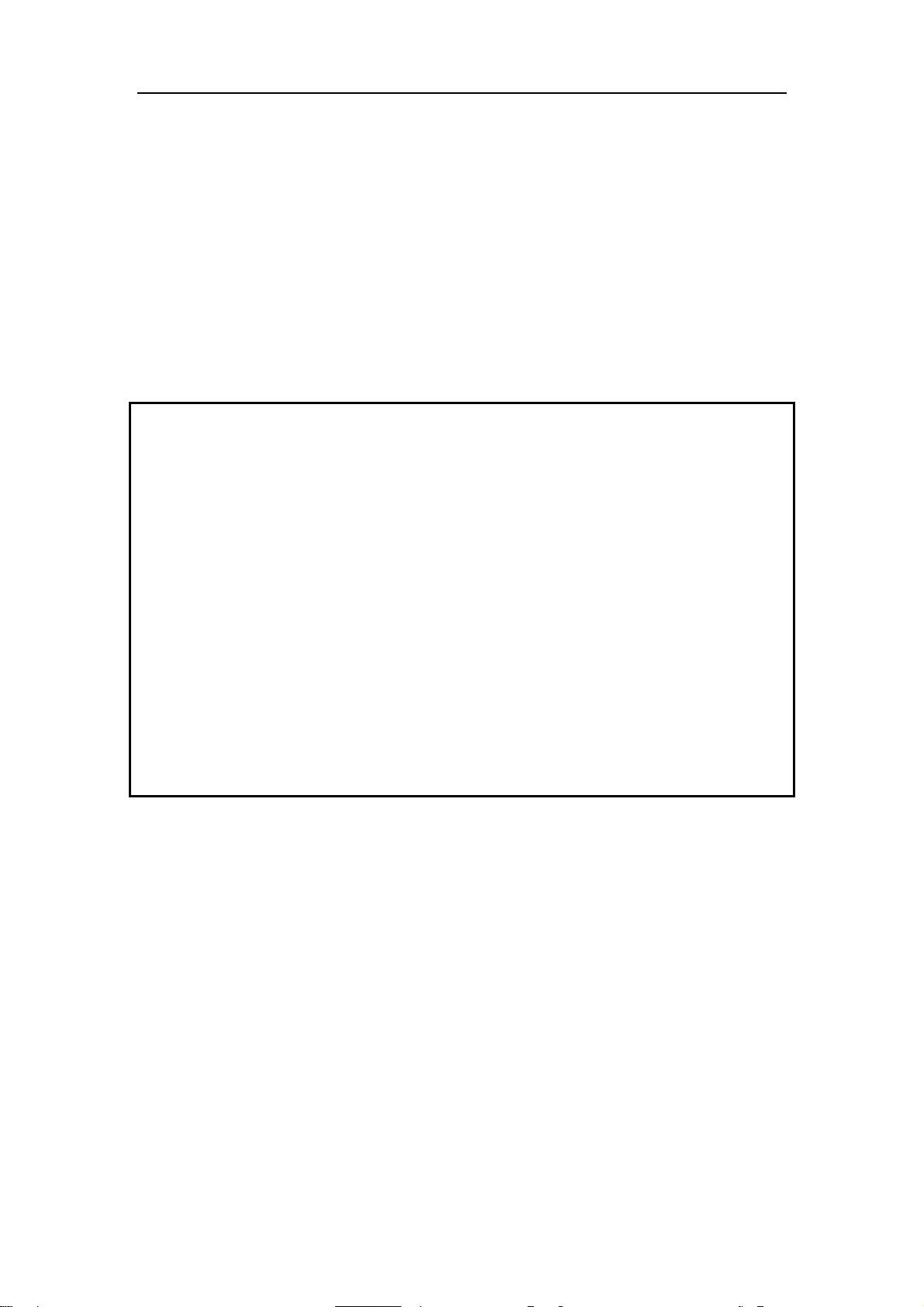
csAxp
河北工业大学——软件工程与理论 整理:虾皮
3
MSDN 中 C#语言规范的:10.1.1.1 抽象类。此文如下:
abstract 修饰符用于表示所修饰的类是不完整的,并且它只能用作基类。抽象类与非抽象类
在以下方面是不同的:
抽象类不能直接实例化,并且对抽象类使用 new 运算符是编译时错误。虽然一些
变量和值在编译时的类型可以是抽象的,但是这样的变量和值必须或者为 null,或
者含有对非抽象类的实例的引用(此非抽象类是从抽象类派生的)。
允许(但不要求)抽象类包含抽象成员。
抽象类不能被密封。
当从抽象类派生非抽象类时,这些非抽象类必须具体实现所继承的所有抽象成员,从而
重写那些抽象成员。在下面的示例中
abstract class A
{
public abstract void F();
}
abstract class B: A
{
public void G() {}
}
class C: B
{
public override void F() {
// actual implementation of F
}
}
抽象类 A 引入抽象方法 F。类 B 引入另一个方法 G,但由于它不提供 F 的实现,B 也
必须声明为抽象类。类 C 重写 F,并提供一个具体实现。由于 C 中没有了抽象成员,因
此可以(但并非必须)将 C 声明为非抽象类。
有关抽象类和抽象方法的问题,我个人也做了一些总结,如下:
abstract 关键字用于将类指定为抽象类,这些抽象类可以派生出其他类。
一个抽象类可以同时包含抽象方法和非抽象方法。
抽象方法的目的在于指定派生类必须实现与这一方法关联的行为。
抽象方法只在派生类中真正实现,这表明抽象方法只存放函数原型(方法的返回类
型,使用的名称及参数),而不涉及主体代码。
如果父类被声明为抽象类,并存在未实现的抽象方法,那么子类就必须实现父类中
所有的 abstract 成员,除非该类也是抽象的。
抽象类不能被实例化,使用 override 关键字可在派生类中实现抽象方法,经 override
声明重写的方法,其签名必须与 override 方法的签名一致。
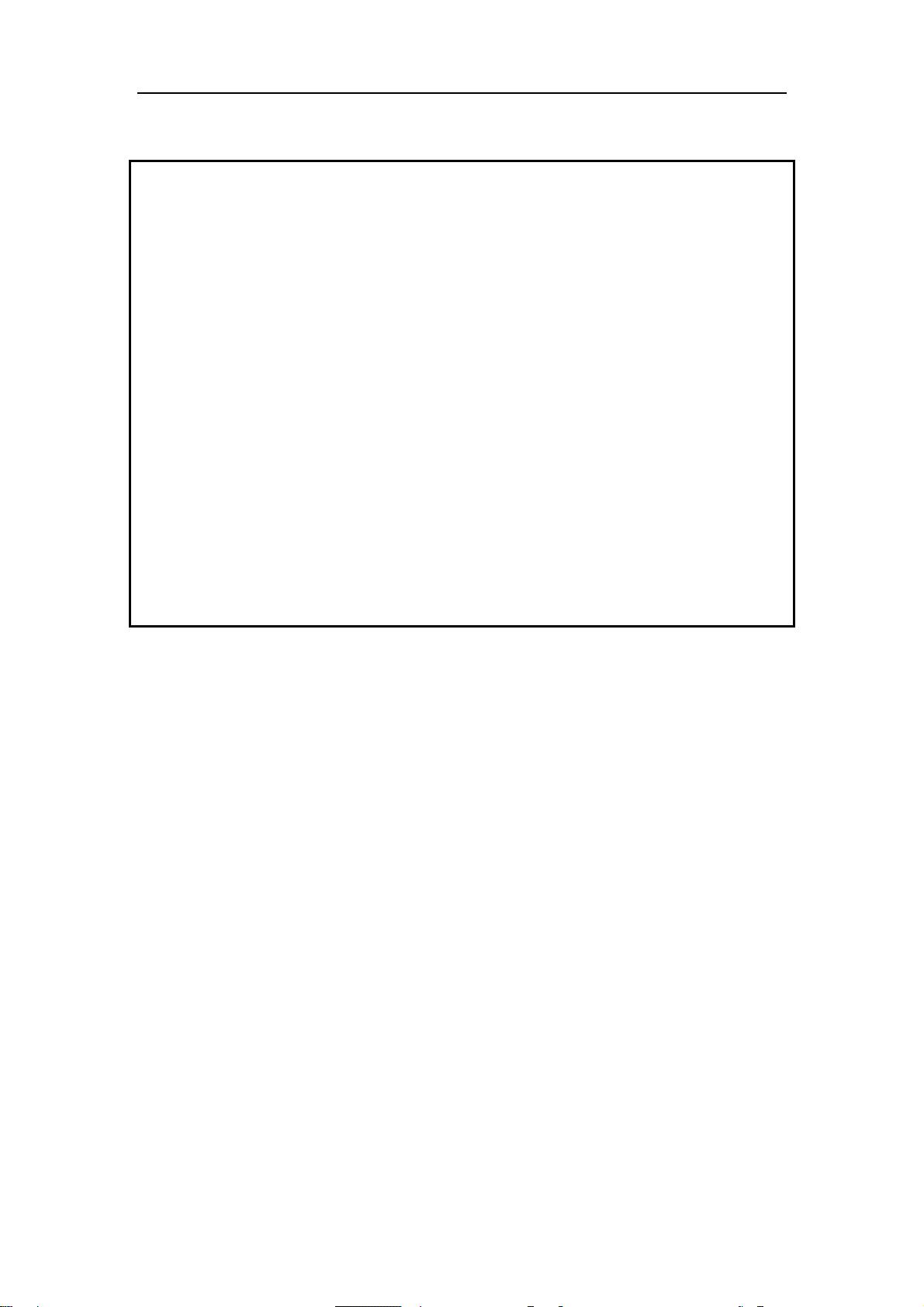
csAxp
河北工业大学——软件工程与理论 整理:虾皮
4
例如:
1abstract class A
2{
3 public abstract void F();
4}
5
6abstract class B: A
7{
8 public void G()
9 {}
10}
11
12class C: B
13{
14 public override void F()
15 {
16 // actual implementation of F
17 }
18}
19
说明: 抽象类 A 引入抽象方法 F。类 B 引入另一个方法 G,但由于它不提供 F 的
实现,B 也必须声明为抽象类。类 C 重写 F,并提供一个具体实现。由于 C 中没有了抽
象成员,因此可以(但并非必须)将 C 声明为非抽象类。
剩余108页未读,继续阅读
资源评论
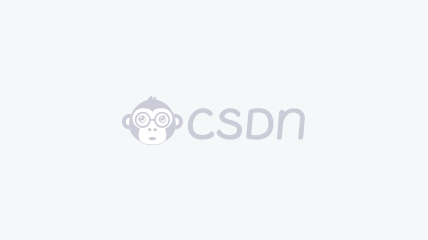
- sgfatboy2015-05-14作为参考还可以,不过分值偏高了。

eagle0618
- 粉丝: 0
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

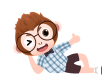
安全验证
文档复制为VIP权益,开通VIP直接复制
