package com.lamatek.tags.google;
import java.io.Serializable;
import java.util.Enumeration;
import java.util.Hashtable;
import java.util.Vector;
import javax.servlet.jsp.JspWriter;
import javax.servlet.jsp.tagext.BodyTagSupport;
import com.lamatek.tags.google.beans.TrafficEventBean;
import com.lamatek.tags.google.beans.XMLParser;
/**
* GoogleMapTag
*
* This class represents a <googlemaps;map> tag. Developers should not subclass or override this
* class or it's methods.
*
* @author Tom Cole
* @version 0.40
*/
public class GoogleMapTag extends BodyTagSupport implements Serializable, GoogleMapEventListener {
static final int SMALL_ZOOM_CONTROL = 0;
static final int LARGE_ZOOM_CONTROL = 1;
Hashtable markers = null;
Hashtable points = null;
Hashtable polylines = null;
Hashtable circles = null;
Hashtable polygons = null;
Hashtable boxes = null;
Hashtable traffic_tags = null;
Hashtable overlays = null;
Hashtable mapTypes = null;
Hashtable wms = null;
Vector events = null;
Vector labels = null;
Vector inserts = null;
Hashtable keys = null;
boolean showTypeControl = false;
boolean showZoomControl = false;
boolean showPanControl = true;
boolean showScaleControl = false;
boolean mouseWheelSupport = false;
boolean panEnabled = true;
String version = "2";
String id = null;
int zoomControlSize = SMALL_ZOOM_CONTROL;
String width = null;
String height = null;
String type = "map";
int zoom = -1;
String scope = "page";
double centerLatitude = -181.00d;
double centerLongitude = -181.00d;
double smallLon = 180.00d;
double smallLat = 180.00d;
double largeLon = -180.00d;
double largeLat = -180.00;
String body = "";
GoogleMapMessageTag message = null;
GoogleMapOverviewTag overviewControl = null;
GoogleMapClusterTag clusterer = null;
GoogleMapCoordinatesTag coords = null;
String xml = null;
boolean debug = false;
boolean headless = false;
int minZoom = 0;
int maxZoom = 17;
boolean bound = false;
String language = "en";
boolean showDaylight = false;
/**
* Overrides doStartTag from BodyTagSupport. Developers should not override this
* method.
*/
public int doStartTag() {
if (scope.equalsIgnoreCase("site")) {
if (pageContext.getSession().getAttribute(id) == null) {
if (xml != null) {
parseXML();
}
return EVAL_BODY_INCLUDE;
}
}
else {
if (pageContext.getAttribute(id) == null) {
if (xml != null) {
parseXML();
}
return EVAL_BODY_INCLUDE;
}
}
return SKIP_BODY;
}
/**
* Parses the defined XML file (or generator), if specified, and adds the resulting entities...
*
*/
private void parseXML() {
XMLParser.parseXML(xml, this);
}
/**
* Overrides doEndTag from BodyTagSupport. Developers should not override this
* method.
*/
public int doEndTag() {
if (scope.equalsIgnoreCase("site")) {
if (pageContext.getSession().getAttribute(id) == null)
pageContext.getSession().setAttribute(id, this);
}
else {
if (pageContext.getAttribute(id) == null)
pageContext.setAttribute(id, this);
}
return EVAL_PAGE;
}
/**
* This method adds a GoogleMapMarkerTag to the map.
*
* @param marker An initialized GoogleMapMarkerTag.
*/
public void addMarker(GoogleMapMarkerTag marker) {
if (markers == null)
markers = new Hashtable();
markers.put(marker.getId(), marker);
}
/**
* Removes a previously added GoogleMapMarkerTag from this map.
*
* @param marker The GoogleMapMarkerTag to remove.
*/
public void removeMarker(GoogleMapMarkerTag marker) {
if (markers != null && marker != null) {
markers.remove(marker.getId());
}
}
/**
* Retrieves a GoogleMapMarkerTag by it's id.
*
* @param name The id of the GoogleMapMarkerTag to retrieve.
* @return The GoogleMapMarkerTag with this id, or null.
*/
public GoogleMapMarkerTag getMarker(String name) {
if (markers != null) {
return (GoogleMapMarkerTag) markers.get(name);
}
else {
return null;
}
}
/**
* Adds a map type to the map storing it by id.
*
* @param mapType An initialized GoogleMapMapTypeTag
*/
public void addMapType(GoogleMapMapTypeTag mapType) {
if (mapTypes == null)
mapTypes = new Hashtable();
mapTypes.put(mapType.getId(), mapType);
}
/**
* Returns the GoogleMapMapTypeTag with the given id
* or null if it cannot be found.
*
* @return A GoogleMapMapTypeTag with the given id or null.
*/
public GoogleMapMapTypeTag getMapType(String id) {
if (mapTypes == null)
return null;
else
return (GoogleMapMapTypeTag) mapTypes.get(id);
}
/**
* Removes the map type with the given id.
*
* @param id The id of the map type to remove.
*/
public void removeMapType(String id) {
if (mapTypes != null)
mapTypes.remove(id);
}
/**
* Retrieves a GoogleMapBoxtag by it's id.
*
* @param name The id of the GoogleMapBoxTag to retrieve.
* @return A GoogleMapBoxTag with the given id or null.
*/
public GoogleMapBoxTag getBox(String name) {
if (boxes != null)
return (GoogleMapBoxTag) boxes.get(name);
else
return null;
}
/**
* Retrieves a GoogleMapCircletag by it's id.
*
* @param name The id of the GoogleMapCircleTag to retrieve.
* @return A GoogleMapCircleTag with the given id or null.
*/
public GoogleMapCircleTag getCircle(String name) {
if (circles != null)
return (GoogleMapCircleTag) circles.get(name);
else
return null;
}
/**
* Retrieves a GoogleMapImageOverlayTag by it's id.
*
* @param name the id of the GoogleMapImageOvelay to retrieve.
* @return A GoogleMapImageOverlayTag with the given id, or null.
*/
public GoogleMapImageOverlayTag getImageOverlay(String name) {
if (overlays == null)
return null;
else
return (GoogleMapImageOverlayTag) overlays.get(name);
}
/**
* Retrieves a GoogleMapPolygonTag by it's id.
*
* @param name The id of the GoogleMapPolygonTag to retrieve.
* @return A GoogleMapPolygonTag with the given id or null.
*/
public GoogleMapPolygonTag getPolygon(String name) {
if (polygons != null)
return (GoogleMapPolygonTag) polygons.get(name);
else
return null;
}
/**
* Retrieves a GoogleMapPolylineTag by it's id.
*
* @param name The id of the GoogleMapPolylineTag to retrieve.
* @return A GoogleMapPolylineTag with the given id or null.
*/
public GoogleMapPolylineTag getPolyline(String name) {
if (polylines != null)
return (GoogleMapPolylineTag) polylines.get(name);
else
return null;
}
/**
* Adds a new GoogleMapPointTag to this map. GoogleMapPointTags are used
* as location references for all overlays (markers, circles, boxes,
* polygons and polylines) in a map.
*
* @param point The initialized GoogleMapPointTag to add.
*/
p
没有合适的资源?快使用搜索试试~ 我知道了~
基于java的Google地图JSP标签库.zip
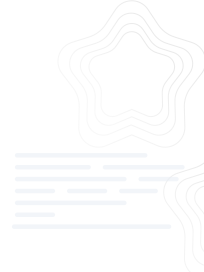
共53个文件
java:48个
html:4个
tld:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 37 浏览量
2023-05-27
10:23:53
上传
评论
收藏 97KB ZIP 举报
温馨提示
基于java的Google地图JSP标签库.zip
资源推荐
资源详情
资源评论
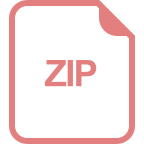
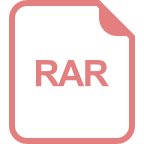
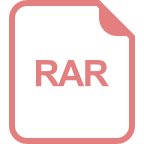
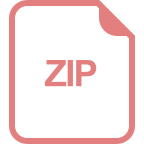
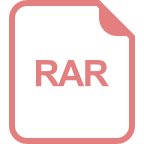
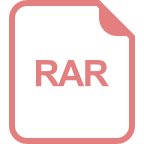
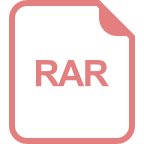
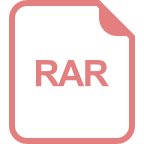
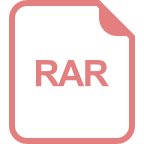
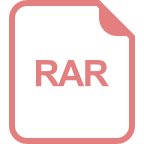
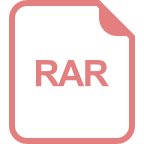
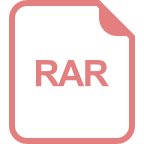
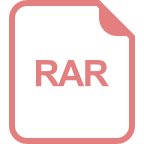
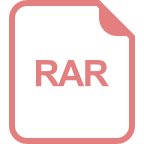
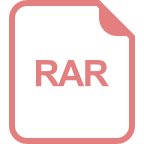
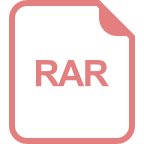
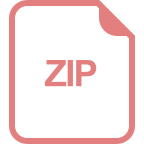
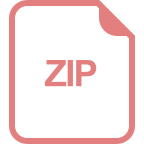
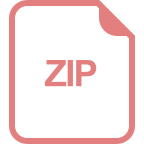
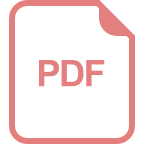
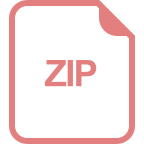
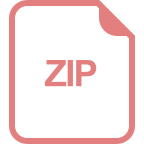
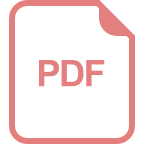
收起资源包目录






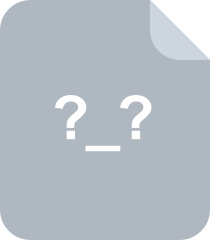




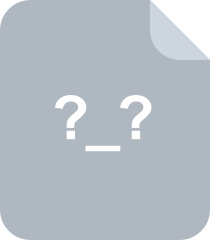
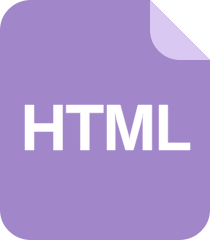


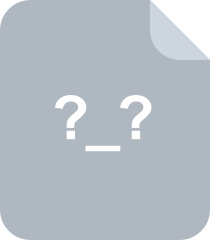
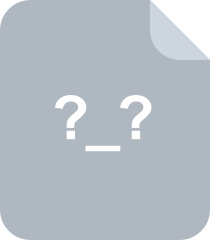
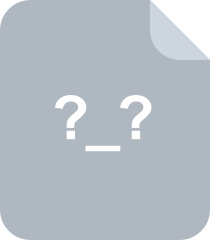
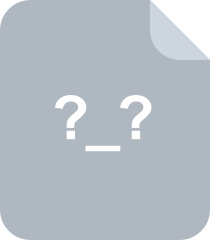
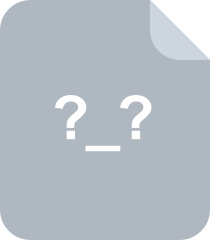
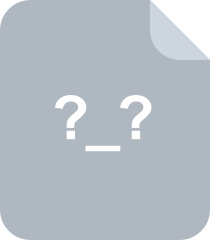
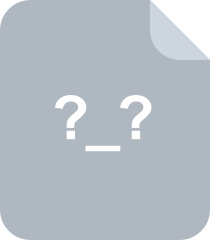
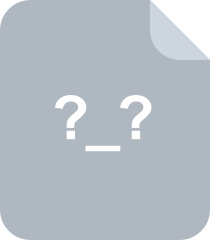
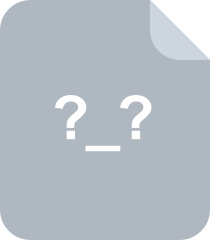
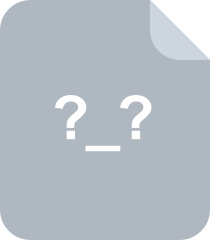
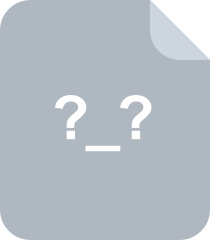
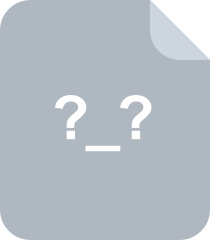
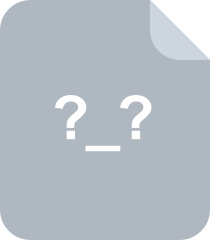
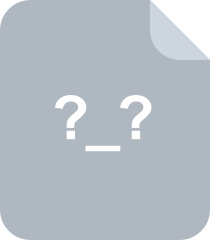
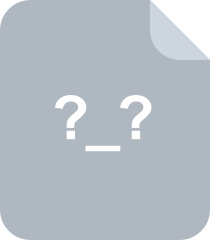
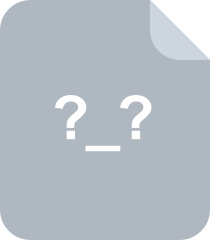

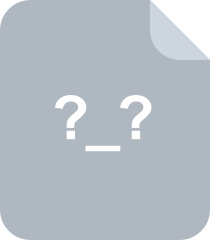
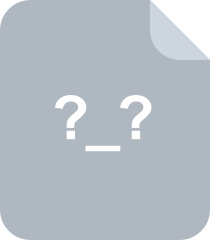
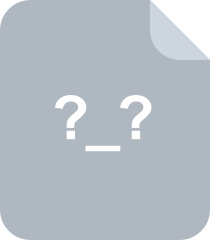
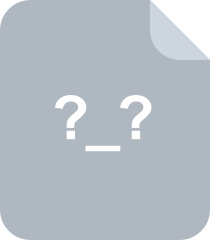
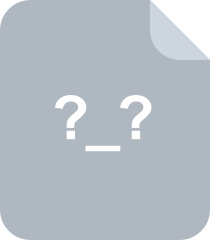
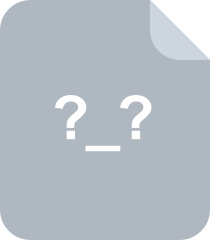
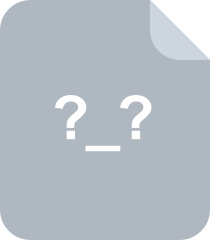
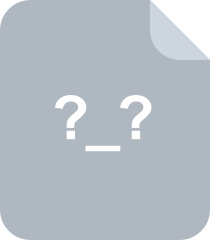
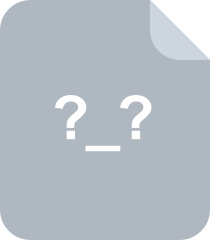
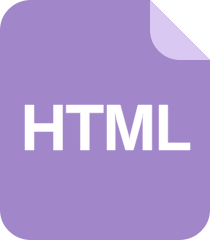
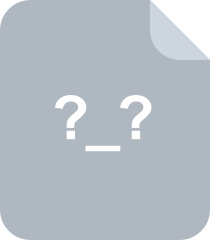
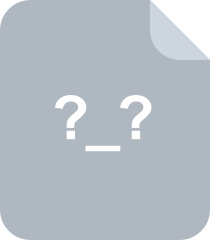
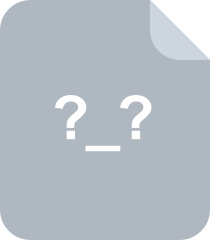
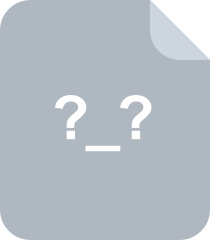
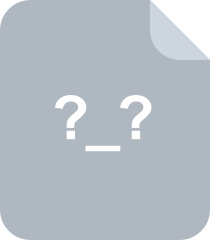
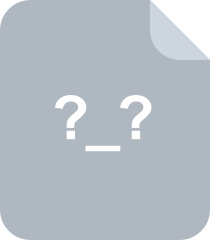
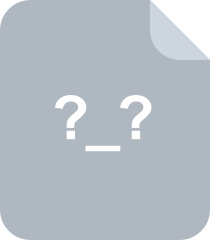
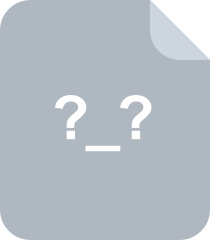
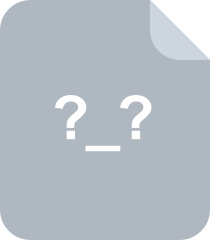
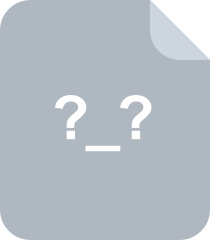
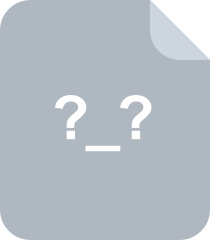
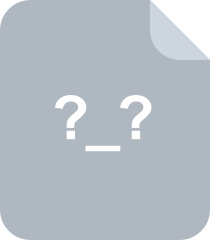
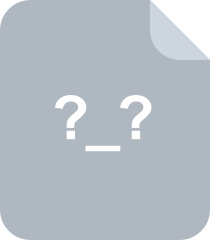
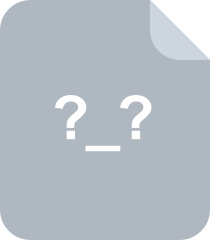
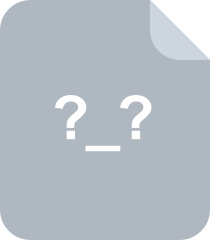
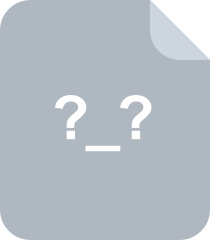
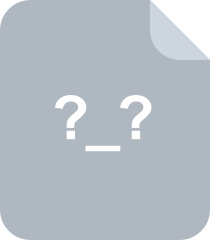
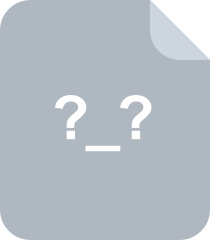
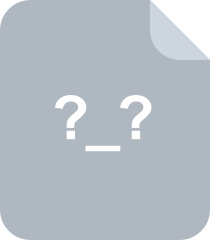
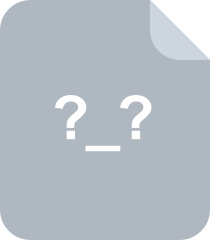
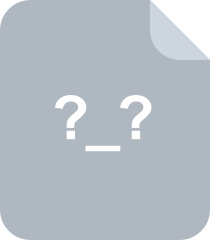
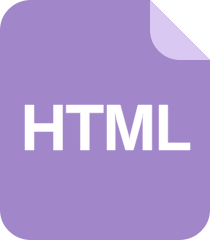


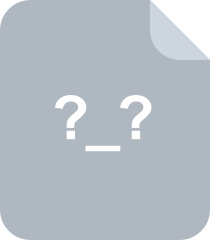
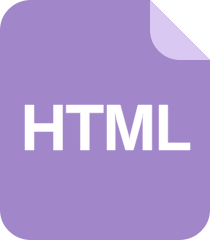
共 53 条
- 1
资源评论
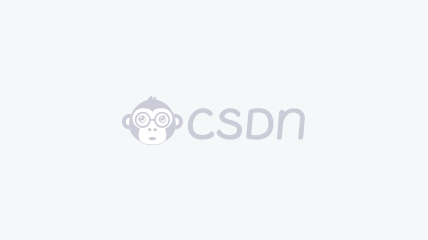


易小侠
- 粉丝: 6514
- 资源: 9万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

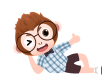
安全验证
文档复制为VIP权益,开通VIP直接复制
