package connection;
import java.io.Serializable;
import java.sql.*;
import java.util.*;
public class ConnPool implements java.io.Serializable{
private String driver = null; //数据库驱动程序对象
private String url = null; //数据源的位置
private int size = 0; //连接池的最大连接数目
private String username = ""; //数据源的用户名
private String password = ""; //数据源的密码
private DbConn dc=null;
private Vector pool = null; //连接池中的连接列表
public ConnPool(){}
//设置数据库驱动程序
public void setDriver(String driver){
if (driver!=null) this.driver=driver;
}
//获取数据库驱动程序
public String getDriver(){
return driver;
}
//设置数据源的位置
public void setURL(String url){
if (url!=null) this.url=url;
}
//获取数据源的位置
public String getURL(){
return url;
}
//设置最大连接数
public void setSize(int size){
if (size>1) this.size=size;
}
//获取最大连接数
public int getSize(){
return size;
}
//设置数据源的用户名
public void setUsername(String username){
if (username!=null) this.username=username;
}
//获取数据源的用户名
public String getUserName(){
return username;
}
//设置数据源的密码
public void setPassword(String password){
if (password!=null) this.password=password;
}
//获取数据源的密码
public String getPassword(){
return password;
}
//设置用于单个连接任务的DbConn对象
public void setConnBean(DbConn dc){
if (dc!=null) this.dc=dc;
}
//获取用于单个连接任务的DbConn对象
public DbConn getConnBean() throws Exception{
Connection conn = getConnection();
DbConn dc = new DbConn(conn); //实例化DbConn类
dc.setInuse(true); //设置此连接可用
return dc;
}
//创建到数据库的连接
private Connection createConnection() throws Exception{
Connection con = null;
con = DriverManager.getConnection(url,username,password);
return con;
}
//初始化连接池
public synchronized void initializePool() throws Exception{
if (driver==null) //如果没有加载驱动
throw new Exception("No Driver Provided!");
if (url==null) //如果没有设置数据源的位置
throw new Exception("No URL Proviced!");
if (size<1) //如果当前没有可用的连接
throw new Exception("Connection Pool Size is less than 1!");
try{
Class.forName(driver);
for (int i=0; i<size; i++){
//创建连接
Connection con = createConnection();
if (con!=null){
//将指定连接加入连接向量末尾
DbConn dc = new DbConn(con);
addConnection(dc);
}
}
}catch (Exception e){
System.err.println(e.getMessage());
throw new Exception(e.getMessage());
}
}
//将指定连接加入连接向量末尾
private void addConnection(DbConn conn){
if (pool==null) pool=new Vector(size);
pool.addElement(conn);
}
//释放指定连接的资源
public synchronized void releaseConnection(Connection con){
for (int i=0; i<pool.size(); i++){
DbConn connBean = (DbConn)pool.elementAt(i);
if (connBean.getConnection()==con){
//寻找到指定连接,将其置为未使用状态
connBean.setInuse(false);
break;
}
}
}
//从连接池得到一个连接
public synchronized Connection getConnection() throws Exception{
DbConn dc = null;
for (int i=0; i<pool.size(); i++){
dc = (DbConn)pool.elementAt(i); // 从连接列表中获得所有连接
if (dc.getInuse()==false){
//如果还有未使用的连接,则使用这个连接
dc.setInuse(true);
Connection con = dc.getConnection();
return con;
}
}
//如果连接都已使用,则新建一连接
try{
Connection con = createConnection();
dc = new DbConn(con);
dc.setInuse(true);
pool.addElement(dc);
}catch (Exception e){
System.err.println(e.getMessage());
throw new Exception(e.getMessage());
}
return dc.getConnection();
}
//清空连接池,释放资源
public synchronized void emptyPool(){
for (int i=0; i<pool.size(); i++){
DbConn connBean = (DbConn)pool.elementAt(i);
if (dc.getInuse()==false)
dc.close(); //释放连接资源
else{
try{
java.lang.Thread.sleep(20000);
dc.close();
}catch (InterruptedException ie){
System.err.println(ie.getMessage());
}
}
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
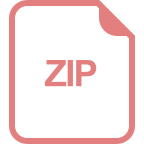
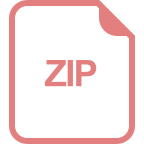
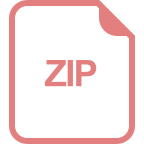
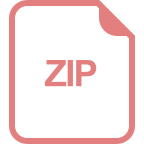
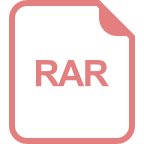
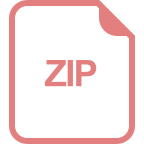
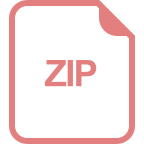
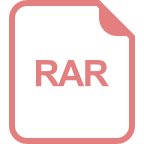
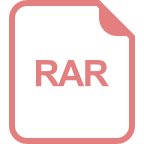
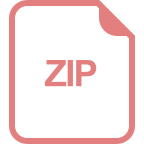
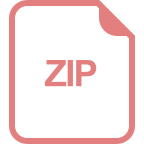
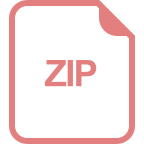
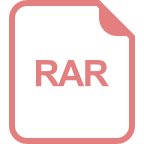
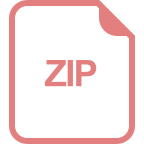
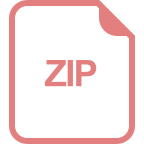
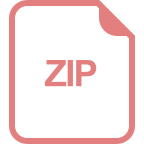
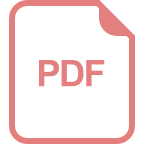
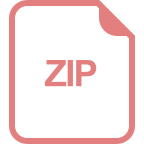
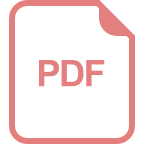
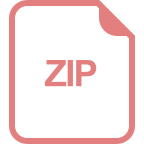
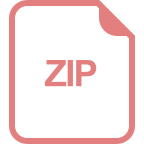
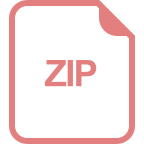
收起资源包目录

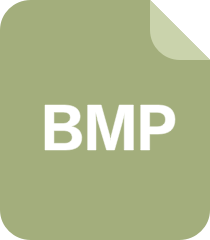
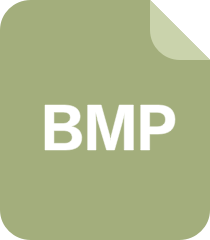
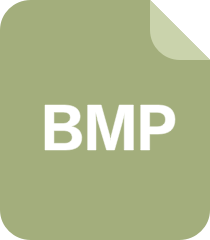
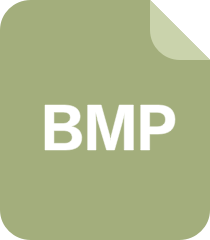
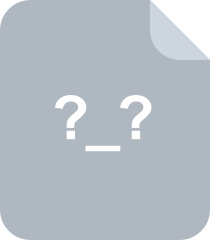
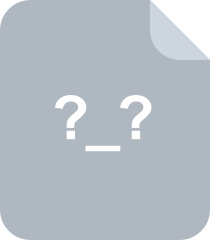
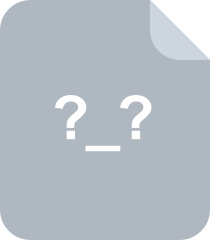
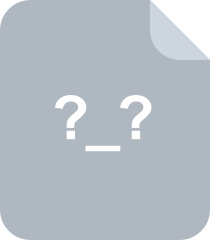
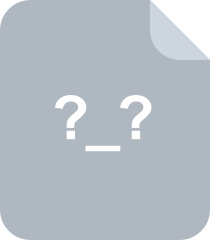
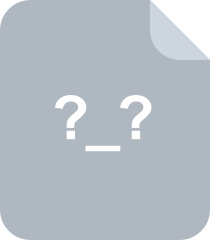
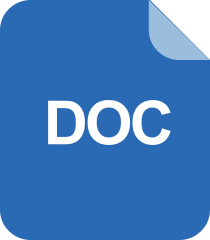
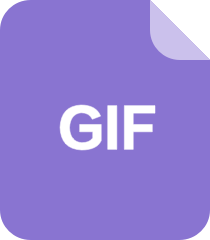
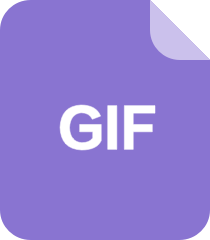
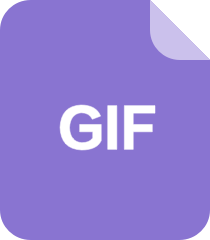
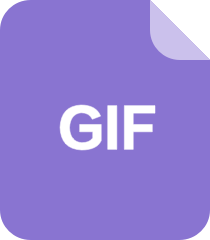
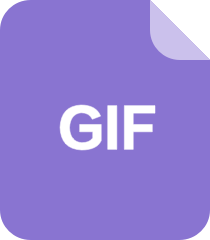
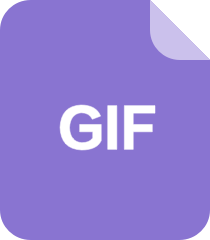
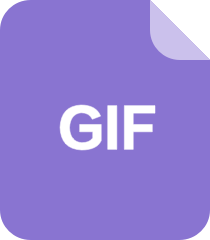
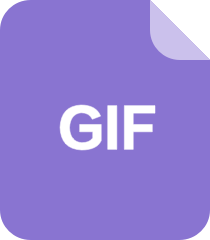
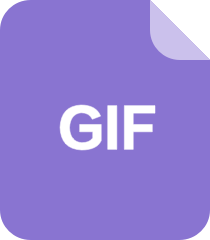
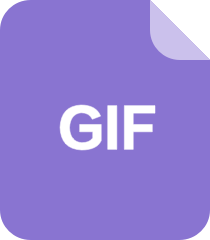
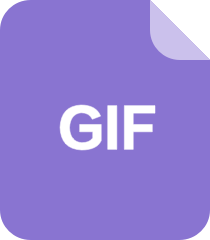
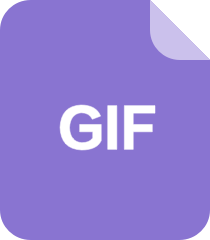
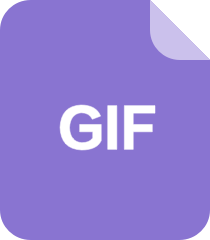
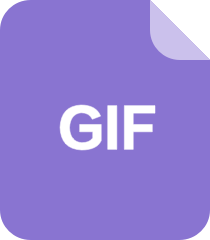
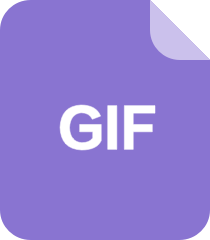
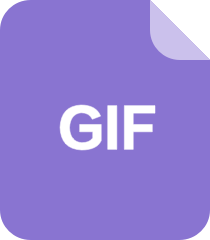
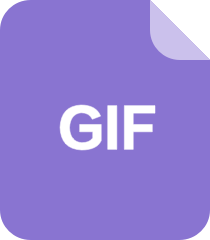
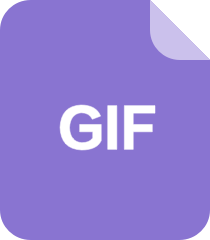
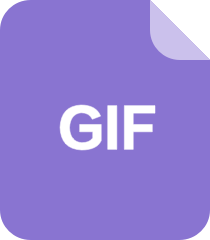
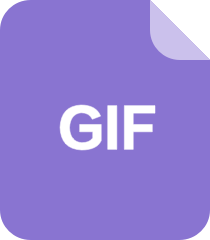
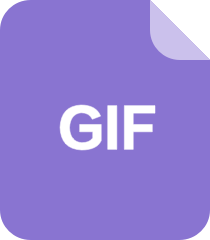
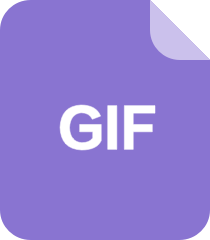
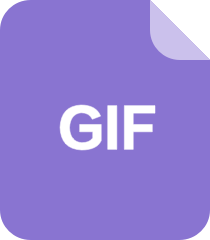
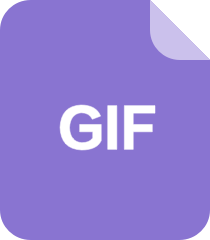
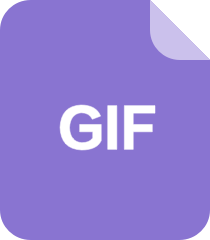
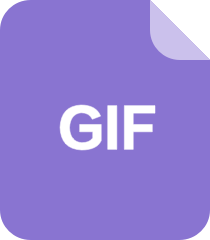
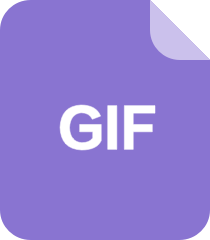
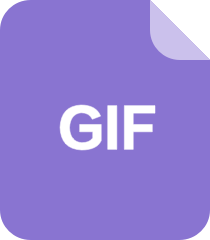
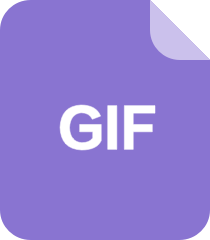
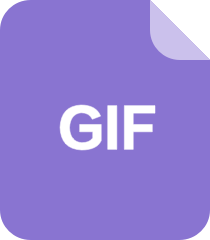
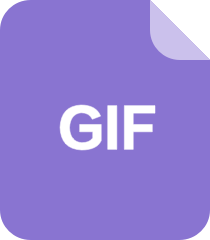
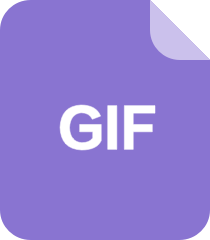
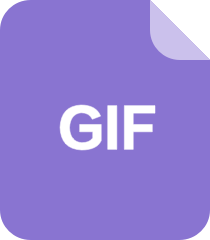
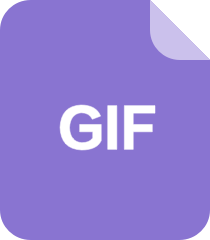
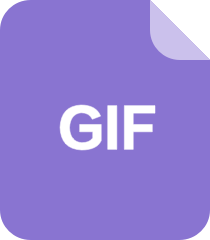
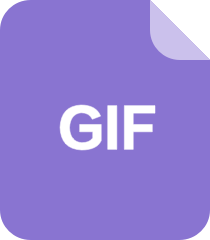
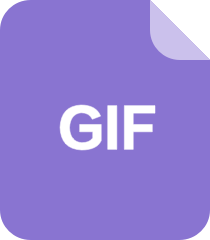
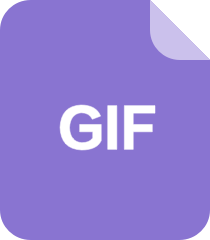
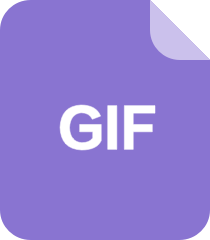
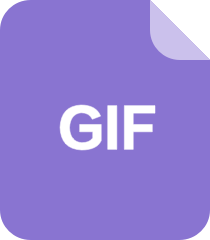
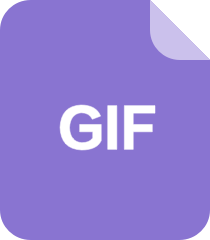
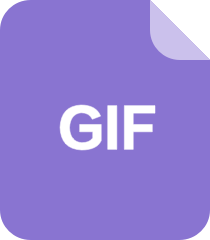
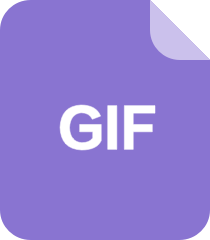
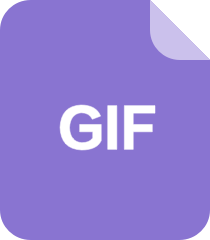
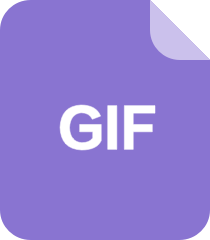
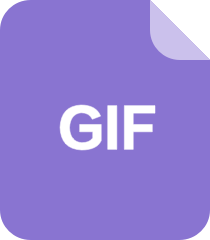
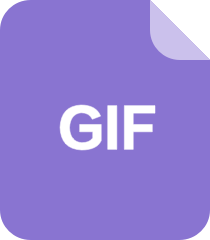
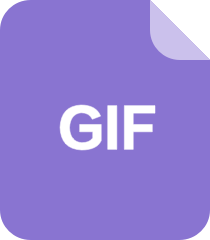
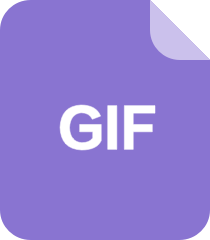
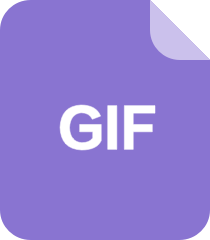
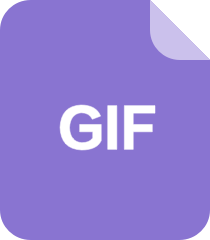
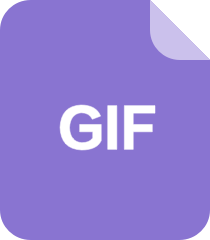
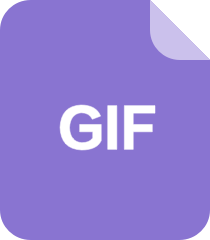
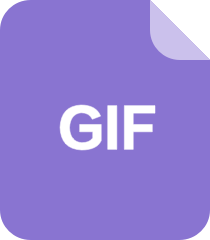
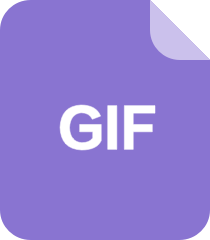
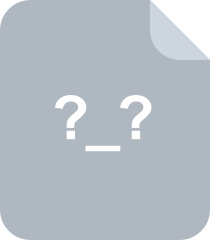
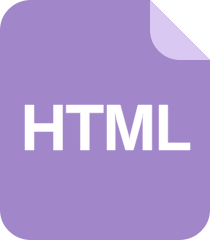
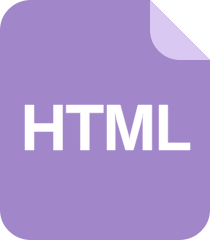
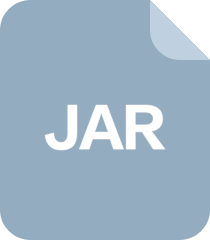
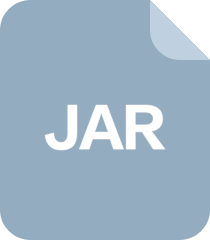
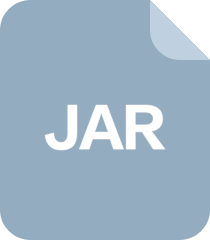
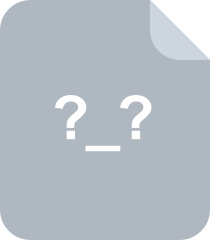
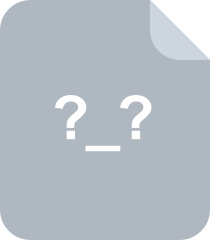
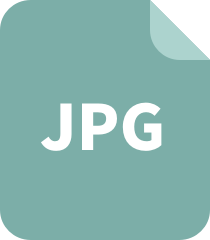
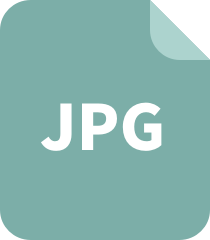
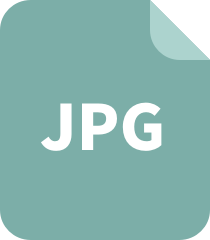
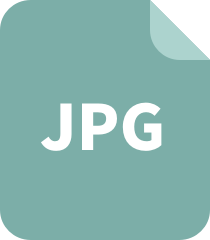
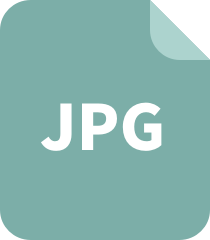
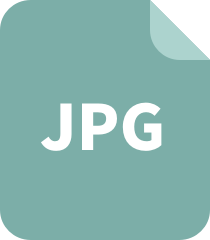
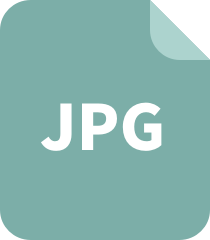
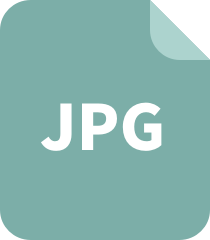
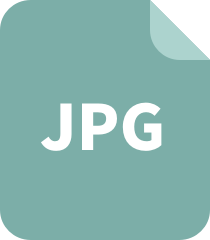
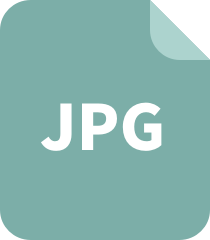
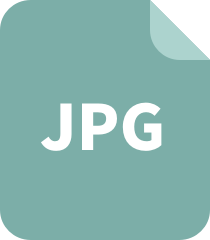
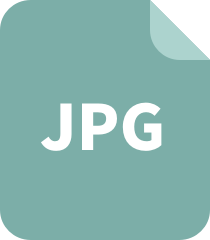
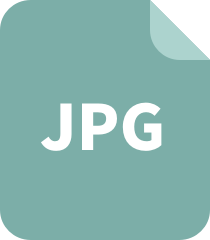
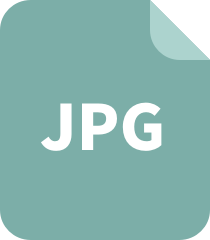
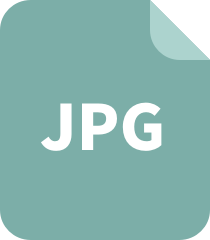
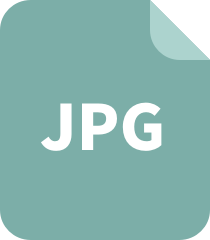
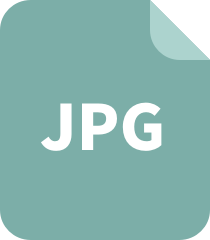
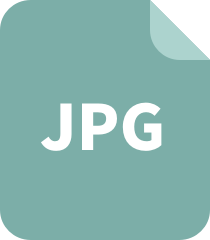
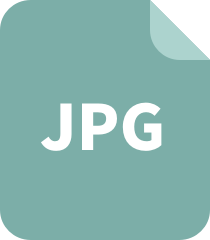
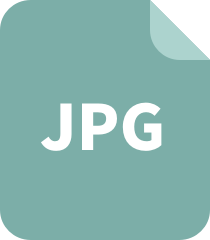
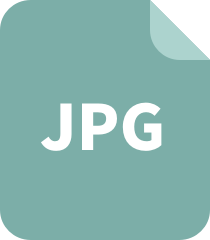
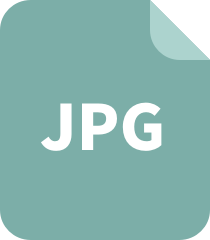
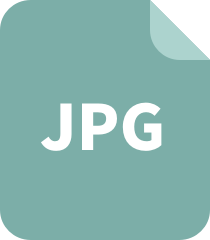
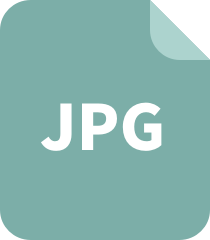
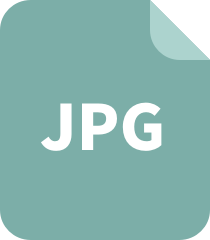
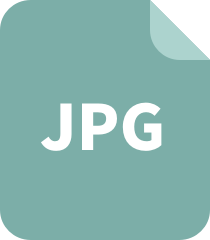
共 200 条
- 1
- 2
资源评论
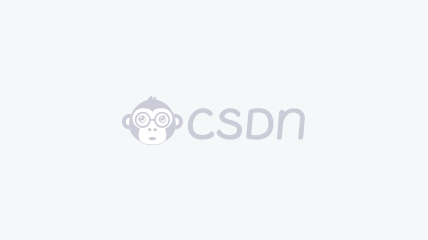


易小侠
- 粉丝: 6465
- 资源: 9万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

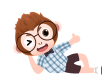
安全验证
文档复制为VIP权益,开通VIP直接复制
