C#中判断某软件是否已安装经典代码
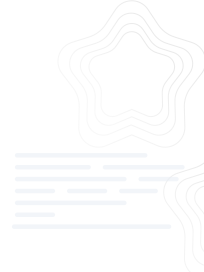


在C#编程中,有时我们需要检测用户的计算机上是否已经安装了特定的软件,这在开发安装程序、系统管理工具或依赖于特定应用的程序时尤为关键。本篇将详细讲解如何利用C#来实现这个功能,并提供经典代码示例。 我们可以利用Windows注册表来检查软件安装情况。大多数应用程序在安装时会在注册表中留下信息,包括安装路径、版本号等。C#提供了`Microsoft.Win32.Registry`命名空间,让我们能够方便地访问和操作注册表。以下是一种常见的通过注册表判断软件是否已安装的方法: ```csharp using Microsoft.Win32; public bool IsSoftwareInstalled(string softwareName) { RegistryKey key = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall"); if (key != null) { foreach (string subkeyName in key.GetSubKeyNames()) { using (RegistryKey subkey = key.OpenSubKey(subkeyName)) { if (subkey != null && (string)subkey.GetValue("DisplayName") == softwareName) { return true; } } } } return false; } ``` 这段代码会检查`HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall`下的所有子键,这是Windows卸载程序存储信息的地方。如果找到匹配的`DisplayName`值,即表示该软件已安装。 另外,对于64位操作系统,由于存在32位和64位程序的注册表隔离,我们还需要考虑`Wow6432Node`,这是一个32位应用程序在64位系统中注册表的映射。因此,为了全面检查,可以将上述代码稍作修改: ```csharp using Microsoft.Win32; public bool IsSoftwareInstalled(string softwareName) { // 检查64位程序注册表 if (IsSoftwareInstalledInRegistry(RegistryHive.LocalMachine, softwareName)) return true; // 检查32位程序注册表(在64位系统中) if (Environment.Is64BitOperatingSystem && Environment.Is64BitProcess) { if (IsSoftwareInstalledInRegistry(RegistryHive.LocalMachine, @"SOFTWARE\Wow6432Node\Microsoft\Windows\CurrentVersion\Uninstall", softwareName)) return true; } return false; } private bool IsSoftwareInstalledInRegistry(RegistryHive hive, string path, string softwareName = null) { using (RegistryKey key = RegistryKey.OpenBaseKey(hive, RegistryView.Default).OpenSubKey(path)) { if (key != null) { foreach (string subkeyName in key.GetSubKeyNames()) { using (RegistryKey subkey = key.OpenSubKey(subkeyName)) { if (subkey != null && (softwareName == null || (string)subkey.GetValue("DisplayName") == softwareName)) { return true; } } } } } return false; } ``` 这个增强版的`IsSoftwareInstalled`函数会同时检查64位和32位的注册表路径,确保在任何情况下都能准确判断。 需要注意的是,操作注册表可能会带来安全风险,因此在实际应用中应谨慎处理,避免引发权限问题或对用户数据造成意外修改。此外,某些软件可能不会遵循标准的卸载注册表键,此时可能需要采用其他方法,例如搜索进程、文件或文件夹是否存在,但这超出了本篇讨论的范围。 通过C#利用Windows注册表可以有效地判断软件是否已安装,而上述代码提供了一个简单且实用的解决方案。在实际开发中,可以根据具体需求进行调整和优化。
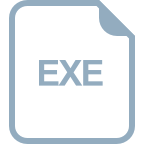
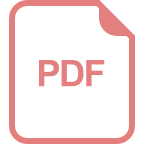
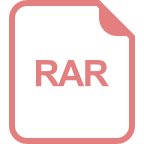
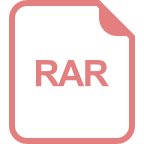
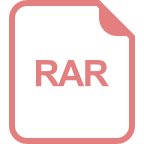
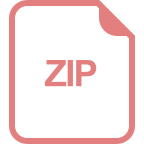
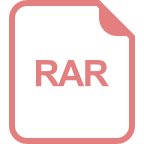
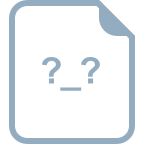
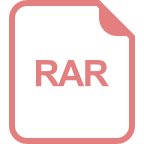
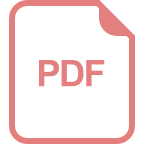
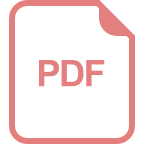
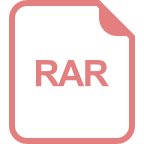
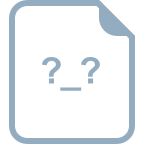
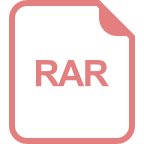
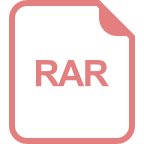
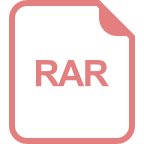
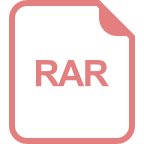
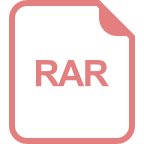
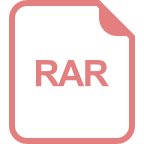
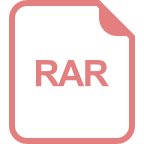

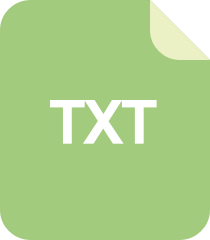
- 1

- 粉丝: 15
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

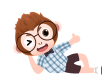
最新资源

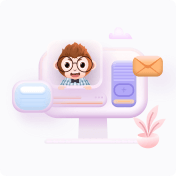

- 1
- 2
- 3
前往页