### Java文件读写详解 在Java编程中,文件读写是一项基本且重要的功能,它涉及到对数据的存储、检索和管理。以下将详细介绍Java中几种常见的文件读写方法及其应用场景。 #### 一、控制台输入读取 在Java中,通过`System.in`可以读取来自标准输入流的数据。下面的代码示例展示了如何读取控制台输入并将其转换为字符串: ```java public String getInputMessage() throws IOException { byte[] buffer = new byte[1024]; int count = System.in.read(buffer); char[] ch = new char[count - 2]; // 去除不必要的字符 for (int i = 0; i < count - 2; i++) { ch[i] = (char) buffer[i]; } String str = new String(ch); return str; } ``` 这段代码首先定义了一个字节数组用于接收输入流中的数据,然后通过`System.in.read(buffer)`读取数据到缓冲区,最后将字节转换为字符数组,并构建为字符串返回。 #### 二、文件复制 在进行文件操作时,文件复制是常见需求之一。以下代码演示了如何使用`FileInputStream`和`FileOutputStream`实现文件复制: ```java public void copyFile(String src, String dest) throws IOException { FileInputStream in = new FileInputStream(src); File file = new File(dest); if (!file.exists()) { file.createNewFile(); } FileOutputStream out = new FileOutputStream(file); byte[] buffer = new byte[1024]; int c; while ((c = in.read(buffer)) != -1) { out.write(buffer, 0, c); } in.close(); out.close(); } ``` 此代码段创建了源文件的输入流和目标文件的输出流,循环读取源文件数据直至读完,然后将读取的数据写入目标文件。 #### 三、文件写入 Java提供了多种方式来写入文件,包括使用`PrintStream`、`StringBuffer`等。 1. **使用PrintStream写入文件** ```java public void PrintStreamDemo() { try { FileOutputStream out = new FileOutputStream("D:/test.txt"); PrintStream p = new PrintStream(out); for (int i = 0; i < 10; i++) { p.println("This is line " + i); } } catch (FileNotFoundException e) { e.printStackTrace(); } } ``` 这段代码创建了一个指向文件的输出流,使用`PrintStream`对象将字符串写入文件。 2. **使用StringBuffer写入文件** ```java public void StringBufferDemo() throws IOException { File file = new File("/root/sms.log"); if (!file.exists()) { file.createNewFile(); } FileOutputStream out = new FileOutputStream(file, true); for (int i = 0; i < 10000; i++) { StringBuffer sb = new StringBuffer(); sb.append("Message " + i + ": Received your message, what is the value"); out.write(sb.toString().getBytes("utf-8")); } out.close(); } ``` 此代码使用`StringBuffer`构建字符串,然后将字符串转化为字节数组并写入文件。 #### 四、文件重命名 Java允许通过`File`类的方法对文件进行重命名。以下代码展示了如何实现文件重命名: ```java public void renameFile(String path, String oldname, String newname) { if (!oldname.equals(newname)) { File oldfile = new File(path + "/" + oldname); File newfile = new File(path + "/" + newname); if (newfile.exists()) { System.out.println(newname + " already exists."); } else { boolean success = oldfile.renameTo(newfile); if (success) { System.out.println("File renamed successfully."); } else { System.out.println("Failed to rename file."); } } } } ``` 这段代码检查新文件名是否已存在,如果不存在,则尝试将旧文件重命名为新文件名。 #### 五、文件移动与目录变更 除了基本的读写操作,Java还支持文件和目录的移动。以下代码示例演示了如何移动文件到新的目录: ```java public void changeDirectory(String filename, String oldpath, String newpath, boolean cover) { if (!oldpath.equals(newpath)) { File oldfile = new File(oldpath + "/" + filename); File newfile = new File(newpath + "/" + filename); if (newfile.exists()) { if (cover) { oldfile.renameTo(newfile); } else { System.out.println("Directory already contains " + filename); } } else { oldfile.renameTo(newfile); } } } ``` 此代码检查目标目录下是否已有同名文件,根据`cover`参数决定是否覆盖。 #### 六、文件输入流读取 使用`FileInputStream`读取文件是一种常见的文件读取方式。以下代码示例展示如何使用`FileInputStream`读取文件: ```java public String FileInputStreamDemo(String path) throws IOException { FileInputStream fis = new FileInputStream(path); StringBuilder sb = new StringBuilder(); int data; while ((data = fis.read()) != -1) { sb.append((char) data); } fis.close(); return sb.toString(); } ``` 这段代码通过`FileInputStream`逐字节读取文件内容,并将字节转换为字符,最终构建为字符串返回。 以上介绍了Java中文件读写的一些基础方法和示例,这些技术在日常开发中非常实用,能够帮助开发者高效地处理文件相关的任务。

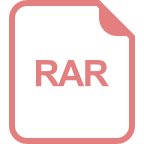

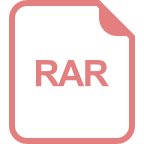



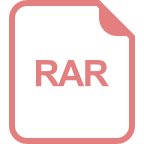






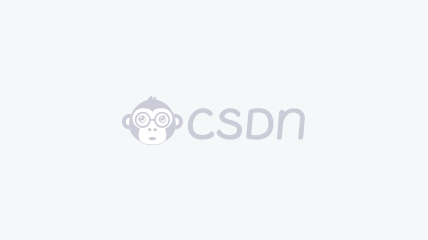

- 粉丝: 4
- 资源: 11
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

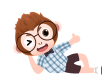
最新资源
- 基于C语言的第十九届全国大学生智能汽车竞赛越野信标组全国一等奖SUV设计源码
- NSM LSHADE CnEpSin算法-NSM-LSHADE-CnEpSin Algorithm-matlab
- 基于Vue与PHP的招商系统服务商管理系统设计源码
- 基于9月28号版本测试的C语言设计源码仓库
- 基于Java语言的九职消防演示系统后端设计源码
- 基于Python和Shell的树莓派学习项目设计源码
- 基于波传播曲率的N体重力模拟-N-body Gravity Simulation by Curvature of Wave Propagation-模拟太阳系、轨道进动、时间延迟、光偏转、黑洞阴影
- 基于Vue框架的白云机场机位分配前端设计源码
- 基于Python实现的photo-to-cartoon卡通化图像转换设计源码
- 基于Vue框架的ditan项目设计源码
- 基于Scala语言的毕设项目设计源码
- 基于Vue与TypeScript的vms自助办理入住Web端设计源码
- 基于JAVA的客房管理系统HTML前端设计源码
- 基于Java语言的个人运动健康饮食管理助手设计源码
- Simscape中具有路径规划的四足机器人-Quadruped Robot with Path Planning in Simscape-matlab
- 基于Go语言的成品项目仓库Project warehouse设计源码

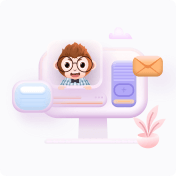
