#include "myappproxy.h"
#include "ipc_skeleton.h"
#include "system_ability_definition.h"
#include "iservice_registry.h"
#include "hitrace/trace.h"
#include "system_ability_load_callback_stub.h"
#include <iostream>
#include <future>
#include <utility>
using namespace std;
using namespace OHOS;
using namespace OHOS::myapp;
#undef LOG_TAG
#define LOG_TAG "MyAppClient"
static constexpr OHOS::HiviewDFX::HiLogLabel LABEL = { LOG_CORE, LOG_DOMAIN, LOG_TAG };
class CallBack : public SystemAbilityLoadCallbackStub
{
~CallBack() {
}
int32_t GetSystemAbilityId() const
{
return currSystemAbilityId;
}
sptr<IRemoteObject> GetRemoteObject() const
{
return currRemoteObject;
}
virtual void OnLoadSystemAbilitySuccess(int32_t systemAbilityId, const sptr<IRemoteObject>& remoteObject)
{
cout << "OnLoadSystemAbilitySuccess" << endl;
currSystemAbilityId = systemAbilityId;
currRemoteObject = remoteObject;
isload.set_value(true);
}
virtual void OnLoadSystemAbilityFail(int32_t systemAbilityId)
{
cout << "OnLoadSystemAbilityFail" << endl;
isload.set_value(false);
}
public:
bool getLoadResult(){
return futureToGet.get();
}
private:
int32_t currSystemAbilityId = 0;
sptr<IRemoteObject> currRemoteObject;
std::promise<bool> isload;
std::future<bool> futureToGet = isload.get_future();
};
sptr<IMyAppService> ConnectService()
{
auto saMgr = SystemAbilityManagerClient::GetInstance().GetSystemAbilityManager();
if (saMgr == nullptr) {
MYAPP_LOGE(LABEL, "get registry fail");
return nullptr;
}
// 加载动态库服务
sptr<CallBack> callback(new CallBack());
saMgr->LoadSystemAbility(MY_APP_SERVICE_ID, callback);
// 阻塞,等待isload传入值
bool laodResult = callback->getLoadResult();
(void)laodResult;
// 获取对象,后续可以进行业务数据交互
sptr<IRemoteObject> object = saMgr->GetSystemAbility(MY_APP_SERVICE_ID);
sptr<IMyAppService> client = nullptr;
if (object != nullptr) {
MYAPP_LOGE(LABEL, "Got MyApp Service object");
sptr<IRemoteObject::DeathRecipient> death(new MyAppDeathRecipient());
object->AddDeathRecipient(death.GetRefPtr());
// client 是一个proxy对象客户端类
client = iface_cast<IMyAppService>(object);
}
if (client == nullptr) {
MYAPP_LOGE(LABEL, "Could not find MyApp Service!");
return nullptr;
}
return client;
}
int main(int argc, char *argv[])
{
int result = 0;
auto client = ConnectService();
std::string name = client->GetName();
cout << "name: " << name << endl;
int32_t ret = client->SetBalance(1024);
cout << "after setbalance1: " << ret << endl;
ret = client->SetBalance(1024);
cout << "after setbalance2: " << ret << endl;
ret = client->GetBalance();
cout << "getbalance: " << ret << endl;
MYAPP_LOGE(LABEL, "get from service: %{public}d", result);
IPCSkeleton::JoinWorkThread();
return 0;
}
OpenHarmony IPC服务的动态加载(拉起)流程(L2)
需积分: 0 171 浏览量
2023-10-02
15:52:26
上传
评论
收藏 10KB RAR 举报

douluo998
- 粉丝: 2036
- 资源: 5356
最新资源
- 如何在C++中使用 vector 的引用语义
- 上市公司-环境绩效数据(EP)(2008-2022年)含dta数据
- QT中QSettings的使用系列之一:初步使用
- 最新版: PowerShell-7.4.3-win-x64.msi
- 小程序版python语言pytorch框架训练识别非机动车骑行有无佩戴安全帽-不含数据集图片-含逐行注释和说明文档.zip
- 上市公司-管理层治理能力(2000-2023年)数据合集,超全变量 权威详细
- 小程序版图像分类算法对墙体裂缝识别-不含数据集图片-含逐行注释和说明文档.zip
- QT中QSettings的使用系列之二:保存和恢复应用程序主窗口
- 小程序版深度学习CNN训练识别图片中是否含有行人-不含数据集图片-含逐行注释和说明文档.zip
- 小程序版python语言pytorch框架的图像分类玻璃是否破碎识别-不含数据集图片-含逐行注释和说明文档.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


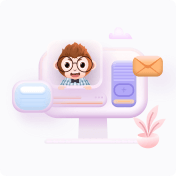