没有合适的资源?快使用搜索试试~ 我知道了~
Big.Nerd.Ranch.Guides.iOS.Programming.The.Big.Nerd.Ranch.Guide.6...

温馨提示
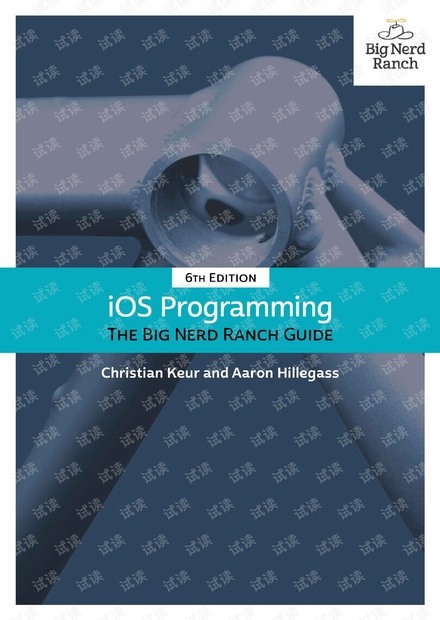

试读
480页
Big.Nerd.Ranch.Guides.iOS.Programming.The.Big.Nerd.Ranch.Guide.6th.Edition.2016
资源推荐
资源评论
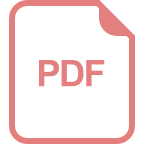
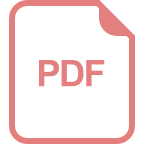
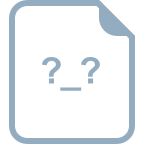
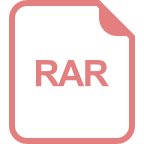
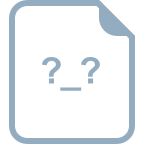
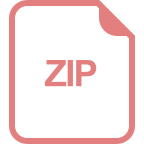
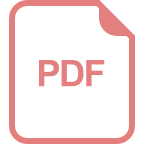
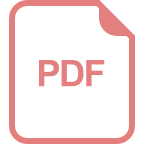
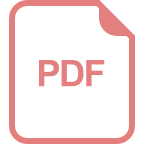
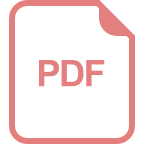
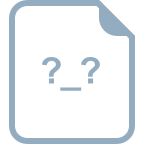
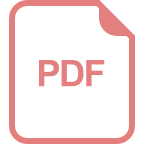
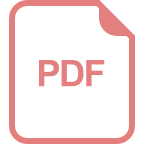
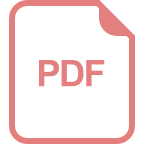
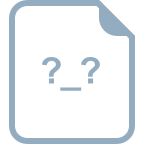
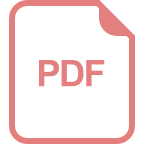
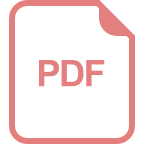
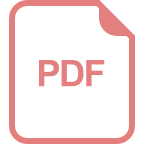
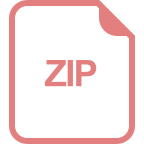
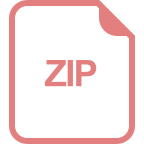
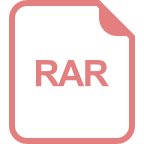
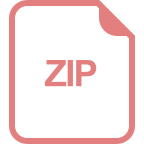
资源评论
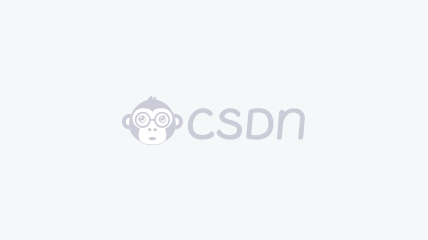
- qq_423384612018-07-12值得一看的好书,ios编程方面的经典。

DoomLord
- 粉丝: 114
- 资源: 1319
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

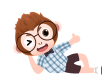
最新资源
- YOLOV4-TINY权重文件
- 以下是一个使用贪心算法解决多机调度问题的基本步骤0.txt
- 基于大数据的房产估价是近年来随着技术的发展而兴起的一种新型估价方法.txt
- 企业供应链管理系统v3.rar
- 富芮坤FR8016HA蓝牙开发板使用手册+硬件PCB图+封装库+DEMO演示软件源代码.zip
- 基于YOLOv7的芯片表面缺陷检测系统
- 京东物流 数字化供应链综合研究报告2018.rar
- 基于YOLOv7的植物虫害识别&防治系统
- 2000.1-2023.8中国经济政策不确定性指数月度数据.xlsx
- Screenshot_2024-04-21-20-42-15-443_com.tencent.mm.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


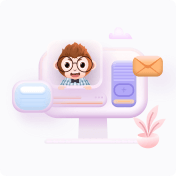
安全验证
文档复制为VIP权益,开通VIP直接复制
