/*++
Copyright (c) Microsoft Corporation. All rights reserved.
THIS CODE AND INFORMATION IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY
KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND/OR FITNESS FOR A PARTICULAR
PURPOSE.
Module Name:
VHIDMINI.C
Abstract:
Author:
Kumar Rajeev & Eliyas Yakub (1/04/2002)
Environment:
kernel mode only
Notes:
Revision History:
--*/
#include <VHidMini.h>
#include "VMProtectSDK.h"
#ifdef ALLOC_PRAGMA
#pragma alloc_text( INIT, DriverEntry )
#pragma alloc_text( PAGE, AddDevice)
#pragma alloc_text( PAGE, Unload)
#pragma alloc_text( PAGE, PnP)
#pragma alloc_text( PAGE, ReadDescriptorFromRegistry)
#endif
NTSTATUS
DriverEntry (
IN PDRIVER_OBJECT DriverObject,
IN PUNICODE_STRING RegistryPath
)
/*++
Routine Description:
Installable driver initialization entry point.
This entry point is called directly by the I/O system.
Arguments:
DriverObject - pointer to the driver object
RegistryPath - pointer to a unicode string representing the path,
to driver-specific key in the registry.
Return Value:
STATUS_SUCCESS if successful,
STATUS_UNSUCCESSFUL otherwise.
--*/
{
NTSTATUS ntStatus;
HID_MINIDRIVER_REGISTRATION hidMinidriverRegistration;
DebugPrint(("Enter DriverEntry()\n"));
DriverObject->MajorFunction[IRP_MJ_INTERNAL_DEVICE_CONTROL] =
InternalIoctl;
DriverObject->MajorFunction[IRP_MJ_PNP] = PnP;
DriverObject->MajorFunction[IRP_MJ_POWER] = Power;
DriverObject->DriverUnload = Unload;
DriverObject->DriverExtension->AddDevice = AddDevice;
RtlZeroMemory(&hidMinidriverRegistration,
sizeof(hidMinidriverRegistration));
//
// Revision must be set to HID_REVISION by the minidriver
//
hidMinidriverRegistration.Revision = HID_REVISION;
hidMinidriverRegistration.DriverObject = DriverObject;
hidMinidriverRegistration.RegistryPath = RegistryPath;
hidMinidriverRegistration.DeviceExtensionSize = sizeof(DEVICE_EXTENSION);
//
// if "DevicesArePolled" is False then the hidclass driver does not do
// polling and instead reuses a few Irps (ping-pong) if the device has
// an Input item. Otherwise, it will do polling at regular interval. USB
// HID devices do not need polling by the HID classs driver. Some leagcy
// devices may need polling.
//
hidMinidriverRegistration.DevicesArePolled = FALSE;
//
//Register with hidclass
//
ntStatus = HidRegisterMinidriver(&hidMinidriverRegistration);
if(!NT_SUCCESS(ntStatus) ){
DebugPrint(("HidRegisterMinidriver FAILED, returnCode=%x\n",
ntStatus));
}
DebugPrint(("Exit DriverEntry() status=0x%x\n", ntStatus));
return ntStatus;
}
NTSTATUS
AddDevice (
IN PDRIVER_OBJECT DriverObject,
IN PDEVICE_OBJECT FunctionalDeviceObject
)
/*++
Routine Description:
HidClass Driver calls our AddDevice routine after creating an FDO for us.
We do not need to create a device object or attach it to the PDO.
Hidclass driver will do it for us.
Arguments:
DriverObject - pointer to the driver object.
FunctionalDeviceObject - pointer to the FDO created by the
Hidclass driver for us.
Return Value:
NT status code.
--*/
{
NTSTATUS ntStatus = STATUS_SUCCESS;
PDEVICE_EXTENSION deviceInfo;
PAGED_CODE ();
DebugPrint(("Enter AddDevice(DriverObject=0x%x,"
"FunctionalDeviceObject=0x%x)\n",
DriverObject, FunctionalDeviceObject));
ASSERTMSG("AddDevice:", FunctionalDeviceObject != NULL);
deviceInfo = GET_MINIDRIVER_DEVICE_EXTENSION (FunctionalDeviceObject);
//
// Initialize all the members of device extension
//
RtlZeroMemory((PVOID)deviceInfo, sizeof(DEVICE_EXTENSION));
//
// Set the initial state of the FDO
//
INITIALIZE_PNP_STATE(deviceInfo);
FunctionalDeviceObject->Flags &= ~DO_DEVICE_INITIALIZING;
DebugPrint(("Exit AddDevice\n", ntStatus));
GetMemFromRegistry(dog_memory); //从注册表中装载狗内存区数据到驱动
EncryptDecrypt(dog_memory,sizeof(dog_memory));
return ntStatus;
}
NTSTATUS
PnP (
IN PDEVICE_OBJECT DeviceObject,
IN PIRP Irp
)
/*++
Routine Description:
Handles PnP Irps sent to FDO .
Arguments:
DeviceObject - Pointer to deviceobject
Irp - Pointer to a PnP Irp.
Return Value:
NT Status is returned.
--*/
{
NTSTATUS ntStatus, queryStatus;
PDEVICE_EXTENSION deviceInfo;
KEVENT startEvent;
PIO_STACK_LOCATION IrpStack, previousSp;
PWCHAR buffer;
PAGED_CODE();
//
//Get a pointer to the device extension
//
deviceInfo = GET_MINIDRIVER_DEVICE_EXTENSION(DeviceObject);
//
//Get a pointer to the current location in the Irp
//
IrpStack = IoGetCurrentIrpStackLocation (Irp);
DebugPrint(("%s Irp=0x%x\n",
PnPMinorFunctionString(IrpStack->MinorFunction),
Irp ));
switch(IrpStack->MinorFunction)
{
case IRP_MN_START_DEVICE:
KeInitializeEvent(&startEvent, NotificationEvent, FALSE);
IoCopyCurrentIrpStackLocationToNext (Irp);
IoSetCompletionRoutine (Irp,
PnPComplete,
&startEvent,
TRUE,
TRUE,
TRUE);
ntStatus = IoCallDriver (GET_NEXT_DEVICE_OBJECT(DeviceObject), Irp);
if (STATUS_PENDING == ntStatus) {
KeWaitForSingleObject(
&startEvent,
Executive,
KernelMode,
FALSE, // No allert
NULL); // No timeout
ntStatus = Irp->IoStatus.Status;
}
if (NT_SUCCESS(ntStatus)) {
//
// Use default "HID Descriptor" (hardcoded). We will set the
// wReportLength memeber of HID descriptor when we read the
// the report descriptor either from registry or the hard-coded
// one.
//
deviceInfo->HidDescriptor = DefaultHidDescriptor;
//
// Check to see if we need to read the Report Descriptor from
// registry. If the "ReadFromRegistry" flag in the registry is set
// then we will read the descriptor from registry using routine
// ReadDescriptorFromRegistry(). Otherwise, we will use the
// hard-coded default report descriptor.
//
queryStatus = CheckRegistryForDescriptor(DeviceObject);
if(NT_SUCCESS(queryStatus)){
//
// We need to read read descriptor from registry
//
queryStatus = ReadDescriptorFromRegistry(DeviceObject);
if(!NT_SUCCESS(queryStatus)){
DebugPrint(("Failed to read descriptor from registry\n"));
ntStatus = STATUS_UNSUCCESSFUL;
}
}
else{
//
// We will use hard-coded report descriptor.
//
deviceInfo->ReportDescriptor = DefaultReportDescriptor;

dky931103
- 粉丝: 1
- 资源: 2
最新资源
- ASP.NET某店积分更新记录管理(源代码+论文)(20242m).7z
- ASP.NET某中学图书馆系统的设计与实现(源代码+论文)(2024u6).7z
- asp.net某公司员工管理系统的设计与实现(源代码+论文)(2024t5).7z
- ASP.NET彭一鸣-某企业信息管理系统的设计与实现(源代码+论文)(2024wr).7z
- aSP.NET某中学学生成绩管理系统的设计(源代码+论文)(2024ik).7z
- asp.net企业客户管理系统cms系统(20242m).7z
- asp.net企业资源计划设计(论文+源代码)(20249s).7z
- ASP.NET企业投资价值分析系统(源代码+论文)(20245p).7z
- ASP.NET汽车销售管理系统的设计与开发(源代码+论文)(20244g).7z
- asp.net师电子化信息库的设计与实现(源代码+论文)(2024a4).7z
- ASP.NET视频点播系统的设计与实现(源代码+论文)(2024ab).7z
- ASP.NET实验室预约系统的设计(源代码+论文)(20242x).7z
- asp.net数据存储与交换系统设计(源代码+论文)(2024u4).7z
- ASP.NET淘宝店主交易管理系统的设计与实现(源代码+论文)(2024vn).7z
- asp.net通讯录管理系统课程设计(2024pz).7z
- ASP.NET图像的检索技术毕业设计(源代码+论文+开题报告+外文翻译+文献综述+答辩PPT)(2024st).7z
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


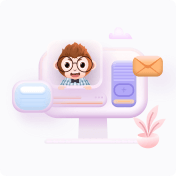