import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Point;
import java.awt.Toolkit;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.SocketException;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class ChessBoard extends JPanel implements Runnable {// 棋盘类
public static final short REDPLAYER = 1;
public static final short BLACKPLAYER = 0;
public Chess[] chess = new Chess[32]; // 所有棋子
public int[][] Map = new int[9 + 1][10 + 1]; //棋盘的棋子布局
public Image bufferImage; // 双缓存
private Chess firstChess2 = null; // 鼠标单击时选定的棋子
private Chess secondChess2 = null;
private boolean first = true; // 区分第一次跟第二次选中的棋子
private int x1, y1, x2, y2;
private int tempx, tempy;
private int r; // 棋子半径
private boolean IsMyTurn = true; // IsMyTurn判断是否该自己走了
public short LocalPlayer = REDPLAYER; // LocalPlayer记录自己是红方还是黑方
private String message = ""; // 提示信息
// 线程消亡的标识位
private boolean flag = false;
private int otherport; // 对方端口
private int receiveport; // 本机接收端口
public void startJoin(String ip, int otherport, int receiveport) // 开始
{
flag = true;
this.otherport = otherport;
this.receiveport = receiveport;
send("join|");
// 创建一个线程()
Thread th = new Thread(this);
// 启动线程
th.start();
message = "程序处于等待联机状态!";
}
public ChessBoard()// 构造方法
{
r = 20;
cls_map();
/**
* 鼠标事件监听
*/
addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
if (IsMyTurn == false) {
message = "该对方走棋";
repaint();
return;
}
int x = e.getX();
int y = e.getY();
selectChess(e);
System.out.println(x);
repaint();
}
private void selectChess(MouseEvent e) {
int idx, idx2; // 保存第一次和第二次被单击棋子的索引号
if (first) {
// 第一次棋子
firstChess2 = analyse(e.getX(), e.getY());
x1 = tempx;
y1 = tempy;
if (firstChess2 != null) {
if (firstChess2.player != LocalPlayer) {
message = "单击成对方棋子了!";
return;
}
first = false;
}
} else {
// 第2次棋子
secondChess2 = analyse(e.getX(), e.getY());
x2 = tempx;
y2 = tempy;
// 如果是自己的棋子,则换上次选择的棋子
if (secondChess2 != null) {
if (secondChess2.player == LocalPlayer) {
// 取消上次选择的棋子
firstChess2 = secondChess2;
x1 = tempx;
y1 = tempy;
secondChess2 = null;
return;
}
}
if (secondChess2 == null) // 目标处没棋子,移动棋子
{
if (IsAbleToPut(firstChess2, x2, y2)) {
// 在map取掉原CurSelect棋子
idx = Map[x1][y1];
Map[x1][y1] = -1;
Map[x2][y2] = idx;
chess[idx].SetPos(x2, y2);
// send
send("move" + "|" + idx + "|" + (10 - x2) + "|"
+ String.valueOf(11 - y2) + "|");
// CurSelect = 0;
first = true;
repaint();
SetMyTurn(false); // 该对方了
// toolStripStatusLabel1.Text = "";
} else {
// 错误走棋
message = "不符合走棋规则";
}
return;
}
if (secondChess2 != null
&& IsAbleToPut(firstChess2, x2, y2)) // 可以吃子
{
first = true;
// 在map取掉原CurSelect棋子
idx = Map[x1][y1];
idx2 = Map[x2][y2];
Map[x1][y1] = -1;
Map[x2][y2] = idx;
chess[idx].SetPos(x2, y2);
chess[idx2] = null;
repaint();
if (idx2 == 0) // 0---"将"
{
message = "红方赢了";
JOptionPane.showConfirmDialog(null, "红方赢了", "提示",
JOptionPane.DEFAULT_OPTION);
// send
send("move" + "|" + idx + "|" + (10 - x2) + "|"
+ String.valueOf(11 - y2) + "|");
send("succ" + "|" + "红方赢了" + "|");
// btnNew.setEnabled(true); //可以重新开始
return;
}
if (idx2 == 16) // 16--"帅"
{
message = "黑方赢了";
JOptionPane.showConfirmDialog(null, "黑方赢了", "提示",
JOptionPane.DEFAULT_OPTION);
send("move" + "|" + idx + "|" + (10 - x2) + "|"
+ String.valueOf(11 - y2) + "|");
send("succ" + "|" + "黑方赢了" + "|");
// btnNew.setEnabled(true); //可以重新开始
return;
}
// send
send("move" + "|" + idx + "|" + (10 - x2) + "|"
+ String.valueOf(11 - y2) + "|");
SetMyTurn(false); // 该对方了
// toolStripStatusLabel1.Text = "";
} else // 不能吃子
{
message = "不能吃子";
}
}
}
});
}
// 解析鼠标之下的棋子对象
private Chess analyse(int x, int y) {
tempx = (int) Math.floor((double) x / 40) + 1;
tempy = (int) Math.floor((double) (y - 20) / 40) + 1;
System.out.println(x + " , " + y);
System.out.println(tempx + " , " + tempy);
// 防止超出范围
if (tempx > 9 || tempy > 10 || tempx < 0 || tempy < 0) {
return null;
} else {
int idx = Map[tempx][tempy];
if (idx == -1) {
return null;
}
return chess[idx];
}
}
private boolean IsMyChess(int idx) {
boolean functionReturnValue = false;
if (idx >= 0 && idx < 16 && LocalPlayer == BLACKPLAYER) {
functionReturnValue = true;
}
if (idx >= 16 && idx < 32 && LocalPlayer == REDPLAYER) {
functionReturnValue = true;
}
return functionReturnValue;
}
// 设置是否该自己走的提示信息
private void SetMyTurn(boolean bolIsMyTurn) {
IsMyTurn = bolIsMyTurn;
if (bolIsMyTurn) {
message = "请您开始走棋";
} else {
message = "对方正在思考...";
}
/*
* //add 7-1 if(LocalPlayer==REDPLAYER) LocalPlayer=BLACKPLAYER; else
* LocalPlayer=REDPLAYER; //add 7-1
*/}
public void send(String str)// 发送信息
{
// message=str;
DatagramSocket s = null;
try {
s = new DatagramSocket();
byte[] buffer;
buffer = new String(str).getBytes();
InetAddress ia = InetAddress.getLocalHost();// 本机地址
// 目的主机地址
// InetAddress ia =
DatagramPacket dgp = new DatagramPacket(buffer, buffer.length, ia,
otherport);
s.send(dgp);
System.out.println("发送信息:" + str);
} catch (IOException e) {
System.out.println(e.toString());
} finally {
if (s != null)
s.close();
}
}
private void cls_map() {
int i, j;
for (i = 1; i <= 9; i++) {
for (j = 1; j <= 10; j++) {
Map[i][j] = -1;
}
}
}
public final void NewGame(short player) // 棋子初始布局
{
cls_map(); // 清空存储棋子信息数组
InitChess();//初始棋子布局
if (player == BLACKPLAYER) {
ReverseBoard();
}
repaint();
}
private void InitChess() {
// 布置黑方棋子
chess[0] = new Chess(BLACKPLAYER, "将", new Point(5, 1));
Map[5][1] = 0;
chess[1] = new Chess(BLACKPLAYER, "士", new Point(4, 1));
Map[4][1] = 1;
chess[2] = new Chess(BLACKPLAYER, "士", new Point(6, 1));
Map[6][1] = 2;
chess[3] = new Chess(BLACKPLAYER, "象", new Point(3, 1));
Map[3][1] = 3;
chess[4] = new Chess(BLACKPLAYER, "象", new Point(7, 1));
Map[7][1] = 4;
chess[5] = new Chess(BLACKPLAYER, "马", new Point(2, 1));
Map[2][1] = 5;
chess[6] = new Chess(BLACKPLAYER, "马", new Point(8, 1));
Map[8][1] = 6;
chess[7] = new Chess(BLACKPLAYER, "车", new Point(1, 1));
Map[1][1] = 7;
chess[8] = new Chess(BLACKPLAYER, "车", new Point(9, 1));
Map[9][1] = 8;
chess[9] = new Chess(BLACKPLAYER, "炮", new Point(2, 3));
Map[2][3] = 9;
chess[10] = new Chess(BLACKPLAYER, "炮", new Point(8, 3));
Map[8][3] = 10;
for (int i = 0; i <= 4; i++) {
chess[11 +

项目花园范德彪
- 粉丝: 7802
- 资源: 229
最新资源
- 【岗位说明】广告公司各职员职务说明书(精美版).doc
- 【岗位说明】广告公司各职员职务说明书.doc
- 【岗位说明】广告公司各岗位职责.docx
- 【岗位说明】XX培训机构岗位职责行政职责.doc
- 【岗位说明】风华教育培训中心岗位职责说明书.doc
- 【岗位说明】高校行政人员岗位职责.doc
- 【岗位说明】教师各岗位岗位职责.doc
- 【岗位说明】教学秘书岗位职责.doc
- 【岗位说明】培训机构助教老师岗位职责.doc
- 【岗位说明】培训机构老师岗位职责.doc
- 【岗位说明】培训学校人员岗位职责及任职要求.doc
- 【岗位说明】学校及培训机构岗位职责大全.doc
- 【岗位说明】幼儿园岗位责任制度.doc
- 【岗位说明】幼儿园岗位职责.doc
- 【岗位说明】幼儿园各类人员岗位职责.doc
- 【岗位说明】辅导机构各岗位职责.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


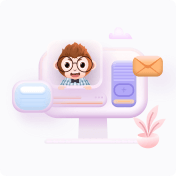