package ServletHandle;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.text.SimpleDateFormat;
import java.util.*;
public class UserHandle extends HttpServlet {
HttpServletRequest request;
HttpServletResponse response;
DAL.User user=new DAL.User();
//通过表单get方式传值 将进入doGet函数(method="get")
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.response=response;
this.request=request;
int handleType=Integer.parseInt(request.getParameter("type").toString());
switch (handleType) {
case 1://类型1代表删除表中的数据
deleteEntity();
break;
case 4://类型4代表获取表中信息
getEntity();
break;
case 5://类型5代表根据查询条件获取表中信息
getEntityByWhere();
break;
default:
break;
}
}
//通过表单post方式传值 将进入doPost函数(method="post")
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.request=request;
this.response=response;
int handleType=Integer.parseInt(request.getParameter("type").toString());//将前台页面传过来的type类型转化成整型
switch (handleType) {
case 2://类型2代表更新表中的数据
updateEntity();
break;
case 3://类型3代表向表中添加数据
insertEntity();
break;
case 6://类型6代表向更改密码
chagePwd();
break;
case 7://类型7代表用户更新个人数据
updateEntity();
break;
case 8://用户注册
register();
default:
break;
}
}
//更改密码
private void chagePwd() throws IOException
{
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=UTF-8");
PrintWriter out=response.getWriter();
HttpSession session=request.getSession();
String userId=session.getAttribute("user_id").toString();
String oldPwd=new String(request.getParameter("OldPwd").getBytes("ISO8859_1"),"UTF-8");
String newPwd=new String(request.getParameter("NewPwd").getBytes("ISO8859_1"),"UTF-8");
if(user.checkPwd(userId, oldPwd))
{
if(user.updataPwd(userId, newPwd))
{
out.write("<script>alert('密码更改成功~~~');location.href='/Parking/Common/UserInfo.jsp'</script>");
}
else {
out.write("<script>alert('密码更改失败~~~');location.href='/Parking/Common/ChagePwd.jsp'</script>");
}
}
else {
out.write("<script>alert('原始密码错误~~~');location.href='/Parking/Common/ChagePwd.jsp'</script>");
}
}
//用户注册
private void register() throws UnsupportedEncodingException, IOException
{
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=UTF-8");
PrintWriter out=response.getWriter();
String UserId=new String(request.getParameter("user_id").getBytes("ISO8859_1"),"UTF-8");
String RoleId=new String(request.getParameter("role_id").getBytes("ISO8859_1"),"UTF-8");
String UserName=new String(request.getParameter("user_name").getBytes("ISO8859_1"),"UTF-8");
String RealName=new String(request.getParameter("real_name").getBytes("ISO8859_1"),"UTF-8");
String UserPwd=new String(request.getParameter("user_pwd1").getBytes("ISO8859_1"),"UTF-8");
String UserPhone=new String(request.getParameter("user_phone").getBytes("ISO8859_1"),"UTF-8");
if(!user.checkExist(UserId))
{
if(user.insertEntity(UserId,RoleId,UserName,RealName,UserPwd,UserPhone)==1)
{
SimpleDateFormat dateFormat =new SimpleDateFormat("yyyyMMddHHmmss");
String AId=dateFormat.format(new Date());
//Account account=new Account();
//account.insertEntity(AId, UserId, "0","2015-12-30");
out.write("<script>alert('恭喜你,注册成功~'); location.href = '/Parking/Login.jsp';</script>");
}
}
else {
out.write("<script>alert('您注册的登陆账号已存在,请重新注册!'); location.href = '/Parking/Login.jsp';</script>");
}
}
//删除数据操作
private void deleteEntity() throws IOException
{
String user_id=request.getParameter("user_id");//获取前台通过get方式传过来的JId
user.deleteEntity(user_id);//执行删除操作
response.sendRedirect("/Parking/UserHandle?type=4");//删除成功后跳转至管理页面
}
//更新数据操作
private void updateEntity() throws UnsupportedEncodingException
{
String user_id=new String(request.getParameter("user_id").getBytes("ISO8859_1"),"UTF-8");
String role_id=new String(request.getParameter("role_id").getBytes("ISO8859_1"),"UTF-8");
String user_name=new String(request.getParameter("user_name").getBytes("ISO8859_1"),"UTF-8");
String real_name=new String(request.getParameter("real_name").getBytes("ISO8859_1"),"UTF-8");
String user_pwd=new String(request.getParameter("user_pwd").getBytes("ISO8859_1"),"UTF-8");
String user_phone=new String(request.getParameter("user_phone").getBytes("ISO8859_1"),"UTF-8");
if(user.updateEntity(user_id,role_id,user_name,real_name,user_pwd,user_phone)==1)
{
try {
if(request.getSession().getAttribute("role_id").toString().equals("r001"))
{
response.sendRedirect("/Parking/UserHandle?type=4");//成功更新数据后跳转至UserInfo.jsp页面
}
else {
response.sendRedirect("/Parking/Common/UserInfo.jsp");//成功更新数据后跳转至UserInfo.jsp页面
}
} catch (IOException e) {
e.printStackTrace();//异常处理
}
}
}
//插入数据操作
private void insertEntity() throws UnsupportedEncodingException, IOException
{
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=UTF-8");
PrintWriter out=response.getWriter();
String user_id=new String(request.getParameter("user_id").getBytes("ISO8859_1"),"UTF-8");
String role_id=new String(request.getParameter("role_id").getBytes("ISO8859_1"),"UTF-8");
String user_name=new String(request.getParameter("user_name").getBytes("ISO8859_1"),"UTF-8");
String real_name=new String(request.getParameter("real_name").getBytes("ISO8859_1"),"UTF-8");
String user_pwd=new String(request.getParameter("user_pwd").getBytes("ISO8859_1"),"UTF-8");
String user_phone=new String(request.getParameter("user_phone").getBytes("ISO8859_1"),"UTF-8");
if(!user.checkExist(user_id))
{
if(user.insertEntity(user_id,role_id,user_name,real_name,user_pwd,user_phone)==1)
{
out.write("<script>alert('数据添加成功!'); location.href = '/Parking/UserHandle?type=4';</script>");
}
else {
out.write("<script>alert('数据添失败!'); location.href = '/Parking/UserHandle?type=4';</script>");
}
}
else {
out.write("<script>alert('主键重复,数据添加失败!'); location.href = '/Parking/UserHandle?type=4';</script>");
}
}
//获取对象所有数据列表
private void getEntity() throws ServletException, IOException
{
request.setCharacterEncoding("UTF-8");
int page=request.getParameter("page")==null?1:Integer.parseInt(request.getParameter("page").toString());//获取跳转的页面号
int totalPage=Integer.parseInt(user.getPageCount().toString()) ;//获取分页
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于jsp的停车场管理系统的设计与实现--源代码--【课程设计】 SpringBoot知识范围-学习步骤--【思维导图知识范围】 https://blog.csdn.net/dearmite/article/details/131842655 下载资源请看同名的博客,学习整个过程 本系列校训 用免费公开视频,卷飞培训班哈人!打死不报班,赚钱靠狠干! 只要自己有电脑,前后项目都能搞!N年苦学无人问,一朝成名天下知! 黑马的JAVA学习路线--详解JAVA部分的学习 https://blog.csdn.net/dearmite/article/details/131609206
资源推荐
资源详情
资源评论























收起资源包目录

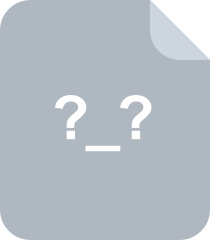


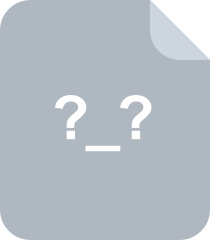
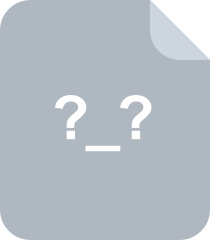
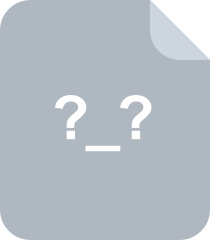
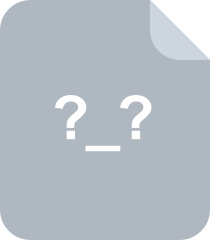

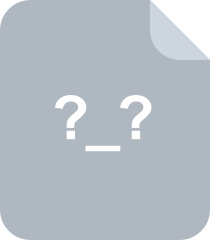
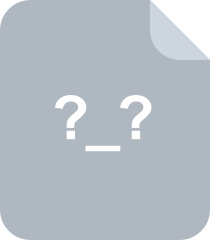
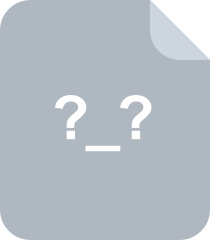
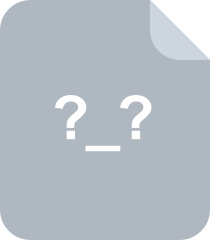
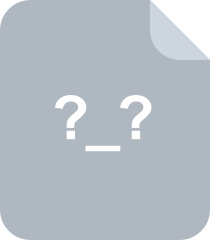
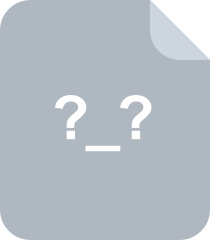
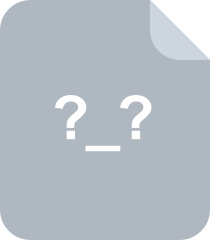

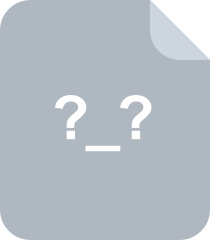
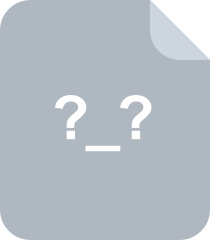
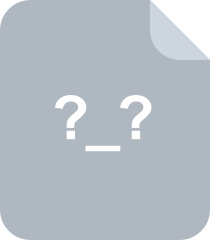
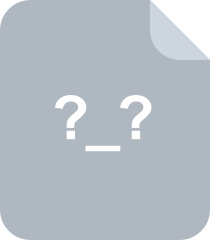
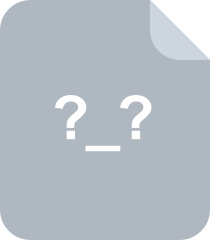
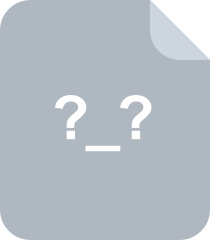
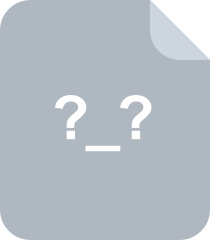
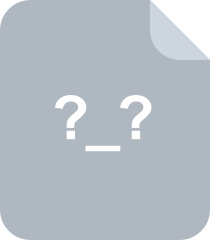


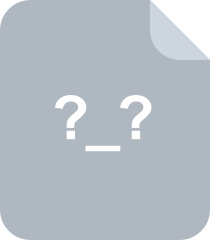

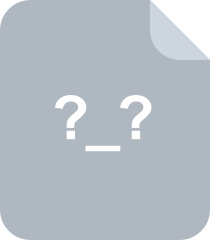
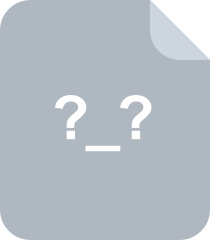
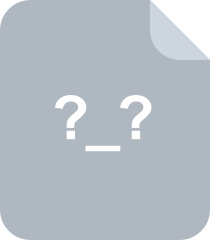
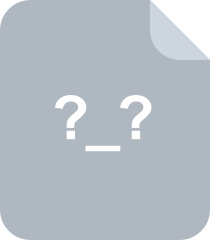


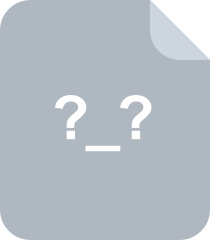
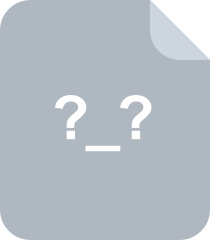
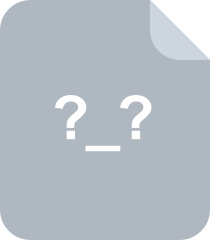
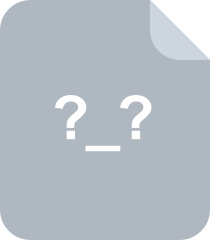
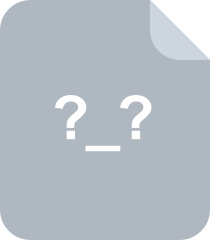



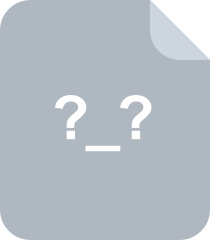
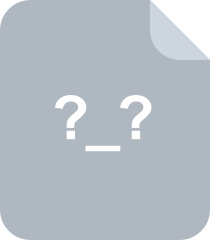
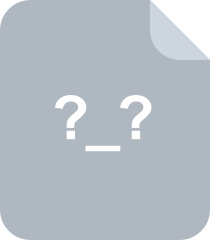
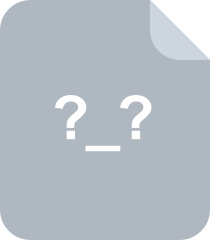

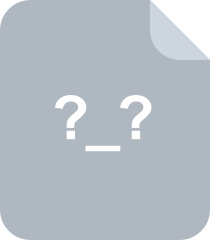
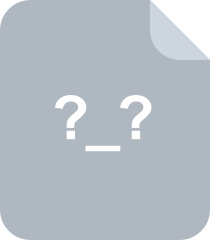
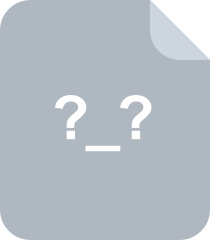
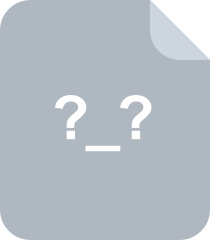
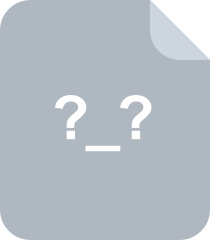
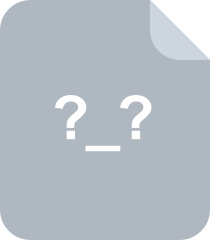
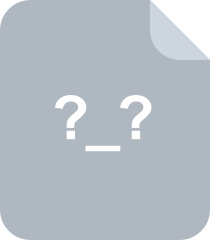

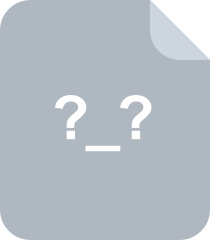
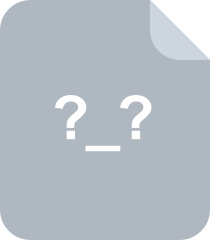
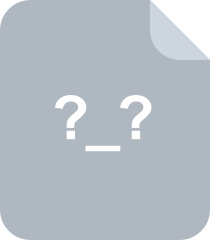
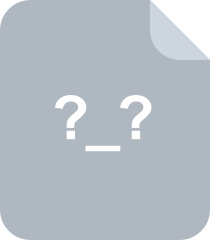
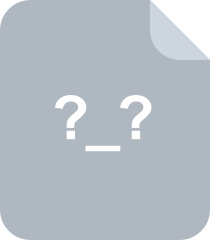
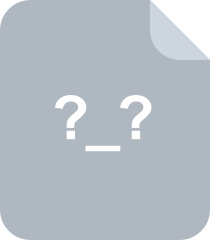
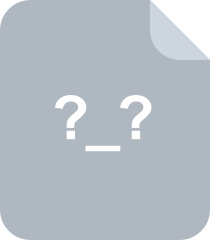

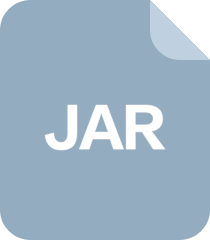
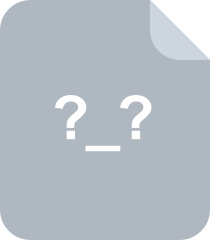

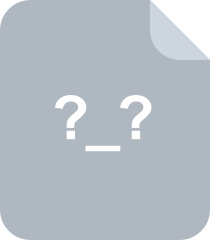
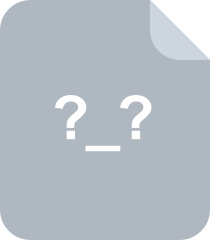
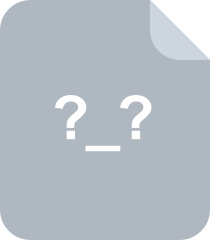
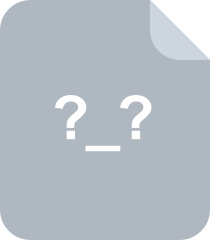
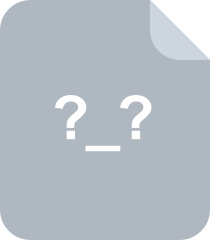
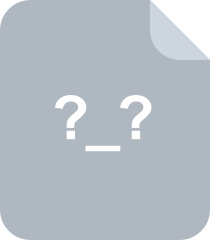
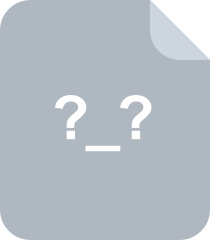
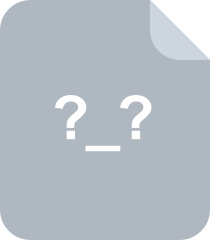
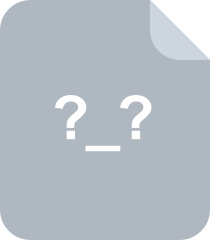
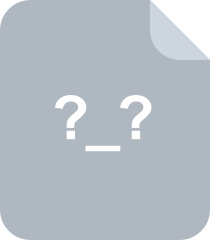
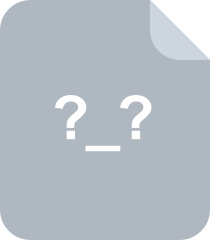
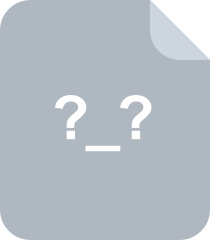
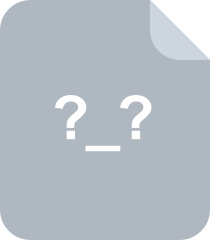
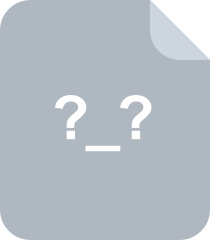
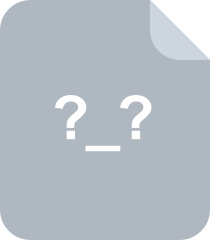
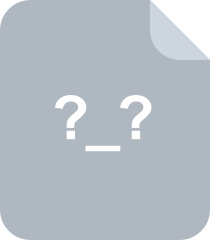
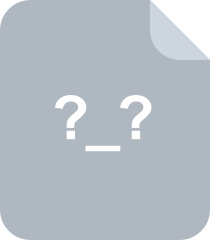
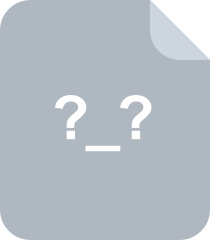
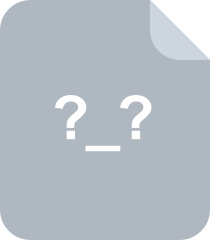
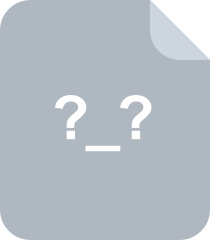

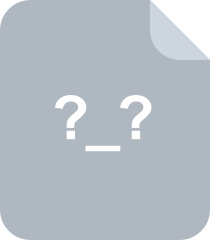
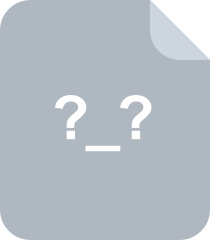

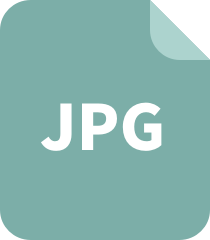
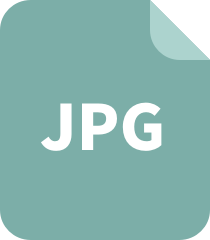
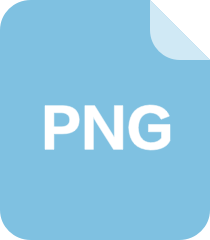
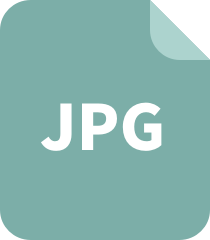
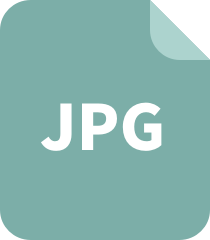
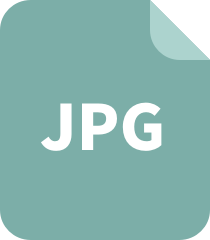
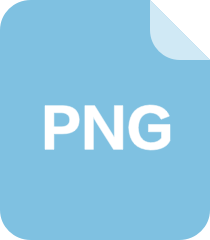
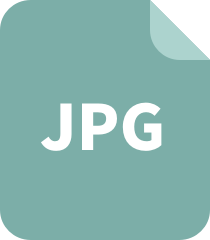
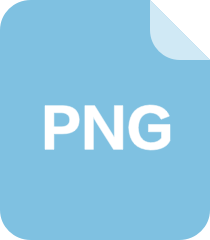
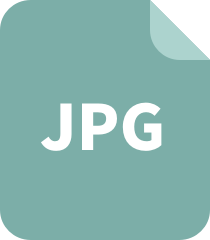
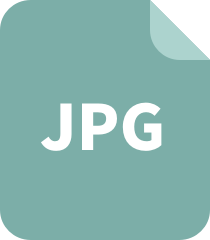
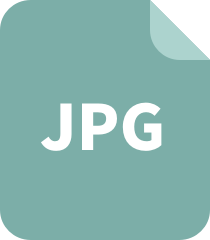
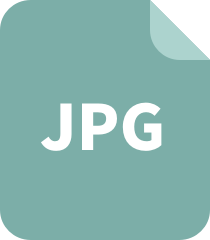
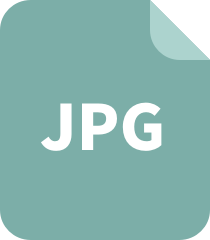
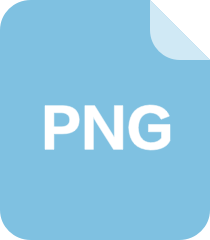
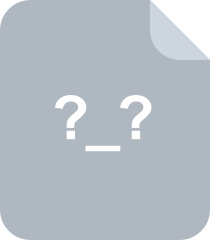

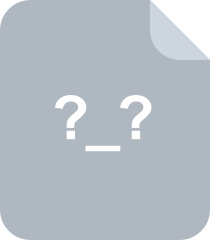
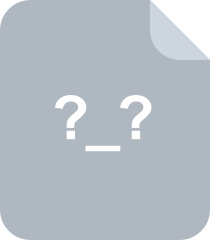
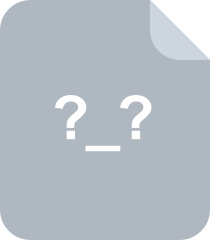

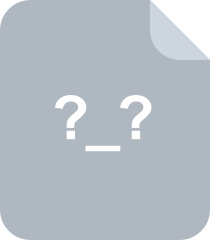
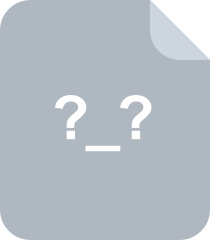
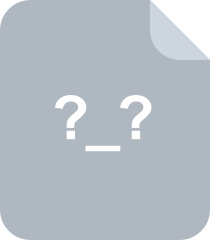
共 94 条
- 1
资源评论
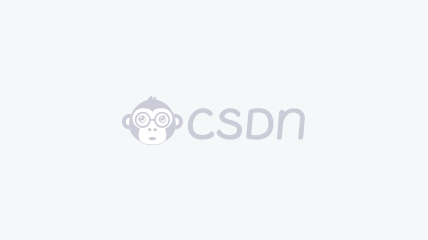

项目花园范德彪
- 粉丝: 8271
- 资源: 250
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

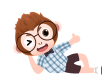
最新资源
- 基于Node.js的JavaScript、CSS、HTML个人博客项目设计源码
- (源码)基于C++和Qt框架的2048游戏.zip
- 基于CSS和JavaScript的HTML车位共享系统物业端设计源码
- 基于扰动观测器的永磁同步电机模型预测控制仿真研究:理想控制效果与通俗易通的模块程序设计,基于扰动观测器的永磁同步电机模型预测控制仿真研究:速度外环与电流内环的协同控制效果分析,基于扰动观测器的永磁同步
- 基于Vue框架的健身后台管理系统设计源码
- (源码)基于Arduino的电动门控制系统.zip
- (源码)基于ESP32和TTGO显示板的停车场传感器系统.zip
- 基于Java架构的电影管家后端与GPT接口设计源码
- (源码)基于Flutter框架的物联网应用.zip
- (源码)基于JavaScript的合成大西瓜游戏魔改版.zip
- 基于JavaScript的先锋积分档案管理系统源码设计
- (源码)基于模块化设计的RSA加密解密系统.zip
- (源码)基于ARM CMSIS DSP库的数字信号处理工具集.zip
- 基于5.2MW永磁风机与虚拟同步机控制的九节点并网系统:高效稳定与可扩展的风电仿真模型,基于双PWM环设计的5.2MW永磁风机一次调频并网三机九节点系统:融合虚拟惯性与下垂控制及虚拟同步机VSG,风电
- (源码)基于TensorRT的Yolo模型推理.zip
- 基于COMSOL多场耦合技术的水合物降压开采与地质能源应用案例分析,COMSOL多场耦合技术在地质能源开采与地质灾害防护中的应用分析,comsol多场耦合 水合物降压降压开采THMC,注气驱替甲烷TH
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


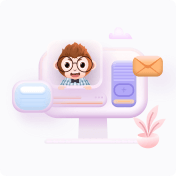
安全验证
文档复制为VIP权益,开通VIP直接复制
