// Copyright 2014 PDFium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Original code copyright 2014 Foxit Software Inc. http://www.foxitsoftware.com
#ifndef PUBLIC_FPDF_FORMFILL_H_
#define PUBLIC_FPDF_FORMFILL_H_
// NOLINTNEXTLINE(build/include)
#include "fpdfview.h"
// These values are return values for a public API, so should not be changed
// other than the count when adding new values.
#define FORMTYPE_NONE 0 // Document contains no forms
#define FORMTYPE_ACRO_FORM 1 // Forms are specified using AcroForm spec
#define FORMTYPE_XFA_FULL 2 // Forms are specified using the entire XFA
// spec
#define FORMTYPE_XFA_FOREGROUND 3 // Forms are specified using the XFAF subset
// of XFA spec
#define FORMTYPE_COUNT 4 // The number of form types
#define JSPLATFORM_ALERT_BUTTON_OK 0 // OK button
#define JSPLATFORM_ALERT_BUTTON_OKCANCEL 1 // OK & Cancel buttons
#define JSPLATFORM_ALERT_BUTTON_YESNO 2 // Yes & No buttons
#define JSPLATFORM_ALERT_BUTTON_YESNOCANCEL 3 // Yes, No & Cancel buttons
#define JSPLATFORM_ALERT_BUTTON_DEFAULT JSPLATFORM_ALERT_BUTTON_OK
#define JSPLATFORM_ALERT_ICON_ERROR 0 // Error
#define JSPLATFORM_ALERT_ICON_WARNING 1 // Warning
#define JSPLATFORM_ALERT_ICON_QUESTION 2 // Question
#define JSPLATFORM_ALERT_ICON_STATUS 3 // Status
#define JSPLATFORM_ALERT_ICON_ASTERISK 4 // Asterisk
#define JSPLATFORM_ALERT_ICON_DEFAULT JSPLATFORM_ALERT_ICON_ERROR
#define JSPLATFORM_ALERT_RETURN_OK 1 // OK
#define JSPLATFORM_ALERT_RETURN_CANCEL 2 // Cancel
#define JSPLATFORM_ALERT_RETURN_NO 3 // No
#define JSPLATFORM_ALERT_RETURN_YES 4 // Yes
#define JSPLATFORM_BEEP_ERROR 0 // Error
#define JSPLATFORM_BEEP_WARNING 1 // Warning
#define JSPLATFORM_BEEP_QUESTION 2 // Question
#define JSPLATFORM_BEEP_STATUS 3 // Status
#define JSPLATFORM_BEEP_DEFAULT 4 // Default
// Exported Functions
#ifdef __cplusplus
extern "C" {
#endif
typedef struct _IPDF_JsPlatform {
/**
* Version number of the interface. Currently must be 2.
**/
int version;
/* Version 1. */
/**
* Method: app_alert
* pop up a dialog to show warning or hint.
* Interface Version:
* 1
* Implementation Required:
* yes
* Parameters:
* pThis - Pointer to the interface structure itself.
* Msg - A string containing the message to be displayed.
* Title - The title of the dialog.
* Type - The type of button group, see
* JSPLATFORM_ALERT_BUTTON_* above.
* nIcon - The icon type, see see
* JSPLATFORM_ALERT_ICON_* above .
*
* Return Value:
* Option selected by user in dialogue, see
* JSPLATFORM_ALERT_RETURN_* above.
*/
int (*app_alert)(struct _IPDF_JsPlatform* pThis,
FPDF_WIDESTRING Msg,
FPDF_WIDESTRING Title,
int Type,
int Icon);
/**
* Method: app_beep
* Causes the system to play a sound.
* Interface Version:
* 1
* Implementation Required:
* yes
* Parameters:
* pThis - Pointer to the interface structure itself
* nType - The sound type, see see JSPLATFORM_BEEP_TYPE_*
* above.
*
* Return Value:
* None
*/
void (*app_beep)(struct _IPDF_JsPlatform* pThis, int nType);
/**
* Method: app_response
* Displays a dialog box containing a question and an entry field for
* the user to reply to the question.
* Interface Version:
* 1
* Implementation Required:
* yes
* Parameters:
* pThis - Pointer to the interface structure itself
* Question - The question to be posed to the user.
* Title - The title of the dialog box.
* Default - A default value for the answer to the question. If
* not specified, no default value is presented.
* cLabel - A short string to appear in front of and on the
* same line as the edit text field.
* bPassword - If true, indicates that the user's response should
* show as asterisks (*) or bullets (?) to mask the response, which might be
* sensitive information. The default is false.
* response - A string buffer allocated by SDK, to receive the
* user's response.
* length - The length of the buffer, number of bytes.
* Currently, It's always be 2048.
* Return Value:
* Number of bytes the complete user input would actually require, not
* including trailing zeros, regardless of the value of the length
* parameter or the presence of the response buffer.
* Comments:
* No matter on what platform, the response buffer should be always
* written using UTF-16LE encoding. If a response buffer is
* present and the size of the user input exceeds the capacity of the
* buffer as specified by the length parameter, only the
* first "length" bytes of the user input are to be written to the
* buffer.
*/
int (*app_response)(struct _IPDF_JsPlatform* pThis,
FPDF_WIDESTRING Question,
FPDF_WIDESTRING Title,
FPDF_WIDESTRING Default,
FPDF_WIDESTRING cLabel,
FPDF_BOOL bPassword,
void* response,
int length);
/*
* Method: Doc_getFilePath
* Get the file path of the current document.
* Interface Version:
* 1
* Implementation Required:
* yes
* Parameters:
* pThis - Pointer to the interface structure itself
* filePath - The string buffer to receive the file path. Can be
* NULL.
* length - The length of the buffer, number of bytes. Can be
* 0.
* Return Value:
* Number of bytes the filePath consumes, including trailing zeros.
* Comments:
* The filePath should be always input in local encoding.
*
* The return value always indicated number of bytes required for the
* buffer , even when there is no buffer specified, or the buffer size is
* less than required. In this case, the buffer will not be modified.
*/
int (*Doc_getFilePath)(struct _IPDF_JsPlatform* pThis,
void* filePath,
int length);
/*
* Method: Doc_mail
* Mails the data buffer as an attachment to all recipients, with or
* without user interaction.
* Interface Version:
* 1
* Implementation Required:
* yes
* Parameters:
* pThis - Pointer to the interface structure itself
* mailData - Pointer to the data buffer to be sent.Can be NULL.
* length - The size,in bytes, of the buffer pointed by
* mailData parameter.Can be 0.
* bUI - If true, the rest of the parameters are used in a
* compose-new-message window that is displayed to the user. If false, the cTo
* parameter is required and all others are optional.
* To - A semicolon-delimited list of recipients for the
* message.
* Subject - The subject of the message. The length limit is 64
* KB.
* CC - A semicolon-delimited list of CC recipients for
* the message.
* BCC - A semicolon-delimited list of BCC recipients for
* the message.
* Msg - The content of the message. The length limit is 64
* KB.
* Return Value:
* None.
* Commen

dbyoung
- 粉丝: 220
- 资源: 31
最新资源
- 工作应聘数据,职位候选人数据,职位数据,近1万条数据(包含了结构化的信息,涵盖了职业目标、技能、教育背景、工作经验、认证以及其他相关细节)
- Boxy SVG for Mac v4.53.0
- 基于java的酒店管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的阿坝州旅游系统设计与实现.docx
- 基于java+springboot+vue+mysql的北部湾地区助农平台设计与实现.docx
- ThinkPHP6.0快速开发手册(案例版)中文PDF高清版最新版本
- 基于java+springboot+vue+mysql的个人财务系统设计与实现.docx
- 基于java+springboot+vue+mysql的宠物共享平台设计与实现.docx
- 基于java+springboot+vue+mysql的二手车交易系统设计与实现.docx
- 基于java+springboot+vue+mysql的花店销售系统设计与实现.docx
- 基于java+springboot+vue+mysql的海产品销售系统设计与实现.docx
- 基于java+springboot+vue+mysql的果树生长信息管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的旅游网站设计与实现.docx
- 基于java+springboot+vue+mysql的可追溯果蔬生产过程的管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的漫画天堂网设计与实现.docx
- 基于java+springboot+vue+mysql的体育商品推荐系统设计与实现.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


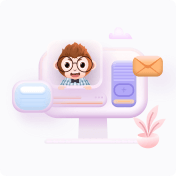