#include "stdafx.h"
#include "cPic.h"
/**************************************************************************
* *
* " T H E _ F I L E _ C L A S S " *
* *
**************************************************************************/
/*
WHY THIS CLASS?
JUST TO MAKE THINGS EASIER WHEN READING THE IMAGE FILE
*/
//constructor
_FILE_::_FILE_()
{
m_File = NULL;
}
//destructor !
_FILE_::~_FILE_()
{
// this->Close();
}
//opens a file either for reading or writing
BOOL _FILE_::OpenA(char * FileName ,FILE_OPENMODE Open_Mode)
{
switch (Open_Mode)
{
case OFM_READ:
m_File = fopen(FileName,"rb");
break;
case OFM_WRITE:
m_File = fopen(FileName,"wb");
break;
}
if(!m_File) return FALSE;
return TRUE;
}
BOOL _FILE_::OpenW(WCHAR * FileName ,FILE_OPENMODE Open_Mode)
{
switch (Open_Mode)
{
case OFM_READ:
m_File =_wfsopen(FileName,L"rb",_SH_DENYNO);
break;
case OFM_WRITE:
m_File = _wfsopen(FileName,L"wb",_SH_DENYNO);
break;
}
if(!m_File) return FALSE;
return TRUE;
}
//Reads data from the file stream.
BOOL _FILE_::Read(VOID * zBuffer,DWORD cSize)
{
if(fread(zBuffer,sizeof(BYTE),cSize,m_File) < cSize)
return FALSE;
return TRUE;
}
//Writes data to the file stream.
BOOL _FILE_::Write(VOID * zBuffer,DWORD cSize)
{
if(fwrite(zBuffer,sizeof(BYTE),cSize,m_File) < cSize)
return FALSE;
return TRUE;
}
//Gets the File Size
LONG _FILE_::GetSize(VOID)
{
long t_location = ftell(m_File);
fseek(this->m_File,0,SEEK_END);
long f_size = ftell(this->m_File );
fseek(this->m_File ,t_location,SEEK_SET);
return f_size;
}
//Closes the File
VOID _FILE_::Close(VOID)
{
if(m_File)
{
fclose(this->m_File);
this->m_File = NULL;
}
return;
}
/**************************************************************************
* *
* " T H E _ P I C T U R E _ C L A S S " *
* *
**************************************************************************/
/*
A CLASS FOR READING IMAGE FILES LIKE JPG,BMP,WMF,GIF...LIKE THE ONE IN VISUAL BASIC
AND DRAWING THEM AS WELL
*/
//constructor
_PICTURE_::_PICTURE_()
{
m_pic = NULL;
hGlobal = NULL;
}
//destructor
_PICTURE_::~_PICTURE_()
{
this->FreePicture();
}
/*
OUR MAIN FUNCTION
*/
HBITMAP _PICTURE_::LoadPictureA(char *FileName)
{
_FILE_ cFile; //the image file
//if we couldn't open the image file then we should get out of here
if(!cFile.OpenA(FileName,OFM_READ)) return NULL;
//we must know exactly the buffer size to allocate
//in order to read the image in it
//so get the image file size
long nSize = cFile.GetSize();
//allocate enough memory to hold the image
//it must be allocated using GlobalAlloc
//otherwise it will fail...try using new or malloc and you'll see!!!
hGlobal = GlobalAlloc(GMEM_MOVEABLE,nSize);
//get pointer to first byte
void* pData = GlobalLock(hGlobal);
//read the file in the prev allocated memory
if(!cFile.Read(pData,nSize)) return NULL;;
//we don't need the file any more so close it
cFile.Close();
GlobalUnlock(hGlobal);
IStream* pStream = NULL;
//ole routines....
//creates a stream from our handle
//don't know why and how?
if (CreateStreamOnHGlobal(hGlobal, TRUE, &pStream) != S_OK)
return NULL;
HRESULT hr;
//aha..now let ole reads the image and load it for us
if ((hr = OleLoadPicture(pStream, nSize, FALSE, IID_IPicture, (LPVOID *)&this->m_pic)) != S_OK)
return NULL;
HBITMAP hbmp = NULL;
//return an HBITMAP to our image like the LoadImage function does
//we might need the handle
this->m_pic->get_Handle((OLE_HANDLE *) &hbmp);
return hbmp;
}
HBITMAP _PICTURE_::LoadPictureW(WCHAR *FileName)
{
_FILE_ cFile; //the image file
//if we couldn't open the image file then we should get out of here
if(!cFile.Open(FileName,OFM_READ)) return NULL;
//we must know exactly the buffer size to allocate
//in order to read the image in it
//so get the image file size
long nSize = cFile.GetSize();
//allocate enough memory to hold the image
//it must be allocated using GlobalAlloc
//otherwise it will fail...try using new or malloc and you'll see!!!
hGlobal = GlobalAlloc(GMEM_MOVEABLE,nSize);
//get pointer to first byte
void* pData = GlobalLock(hGlobal);
//read the file in the prev allocated memory
if(!cFile.Read(pData,nSize)) return NULL;;
//we don't need the file any more so close it
cFile.Close();
GlobalUnlock(hGlobal);
IStream* pStream = NULL;
//ole routines....
//creates a stream from our handle
//don't know why and how?
if (CreateStreamOnHGlobal(hGlobal, TRUE, &pStream) != S_OK)
return NULL;
HRESULT hr;
//aha..now let ole reads the image and load it for us
if ((hr = OleLoadPicture(pStream, nSize, FALSE, IID_IPicture, (LPVOID *)&this->m_pic)) != S_OK)
return NULL;
HBITMAP hbmp = NULL;
//return an HBITMAP to our image like the LoadImage function does
//we might need the handle
this->m_pic->get_Handle((OLE_HANDLE *) &hbmp);
return hbmp;
}
//an overloaded version of the above to draw the picture
//as well in a given DC
HBITMAP _PICTURE_::LoadPictureA(char *FileName,HDC hdc)
{
HBITMAP hbmp = this->LoadPictureA(FileName);
this->DrawPicture(hdc,0,0,this->_GetWidth(),_GetHeight());
return hbmp;
}
HBITMAP _PICTURE_::LoadPictureW(WCHAR *FileName,HDC hdc)
{
HBITMAP hbmp = this->LoadPicture(FileName);
this->DrawPicture(hdc,0,0,this->_GetWidth(),_GetHeight());
return hbmp;
}
//returns the width of the loaded image
DWORD _PICTURE_::_GetWidth(VOID)
{
if(! m_pic) return 0;
OLE_XSIZE_HIMETRIC pWidth;
m_pic->get_Width(&pWidth);
HDC tDC = CreateCompatibleDC(0);
int nWidth = MulDiv(pWidth,GetDeviceCaps(tDC,LOGPIXELSX), HIMETRIC_INCH);
DeleteDC(tDC);
return (DWORD) nWidth;
}
//returns the height of the loaded image
DWORD _PICTURE_::_GetHeight(VOID)
{
if(! m_pic) return 0;
OLE_YSIZE_HIMETRIC pHeight;
m_pic->get_Height(&pHeight);
HDC tDC = CreateCompatibleDC(0);
int nHeight = MulDiv(pHeight,GetDeviceCaps(tDC,LOGPIXELSY), HIMETRIC_INCH);
DeleteDC(tDC);
return (DWORD) nHeight;
}
//returns the current DC of the loaded image
HDC _PICTURE_::_GetDC(VOID)
{
if(! m_pic) return NULL;
HDC pDC ;
m_pic->get_CurDC(&pDC);
return pDC;
}
//returns the Handle of the loaded image(HBITMAP)
HBITMAP _PICTURE_::_GetHandle( VOID )
{
if(! m_pic) return NULL;
HBITMAP hbmp;
m_pic->get_Handle((OLE_HANDLE *) &hbmp);
return hbmp;
}
//Draws the image in a specified DC..with given dimensions
//specify -1 for width and height if you like to draw
//with original dimensions
BOOL _PICTURE_::DrawPicture(HDC hdc,long x,long y,long cx,long cy)
{
if(! m_pic) return FALSE;
LONG pHeight,pWidth;
if(cx == -1) cx = this->_GetWidth();
if(cy == -1) cy = this->_GetHeight();
m_pic->get_Width(&pWidth);
m_pic->get_Height(&pHeight);
if (m_pic->Render(hdc, x, y, cx, cy,0, pHeight, pWidth,-pHeight, NULL) != S_OK)
return FALSE ;
return TRUE;
}
//Cleans up
VOID _PICTURE_::FreePicture(VOID)
{
if (m_pic)
{
m_pic->Release();
m_pic = NULL;
}
if (hGlobal)
{
GlobalFree(hGlobal);
hGlobal = NULL;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
实现图片的预览,能自由在控件中实现图片预览,只需传递窗体句柄、控件ID和图片文件路径即可预览图片文件 改天上传一个调用的演示程序 调用代码: CFileDialog file(true); int Width,Higth; if (file.DoModal() == IDOK) { CShowImagePreview showImage; showImage.ShowImagePreview(m_hWnd,IDC_PIC,file.GetPathName().AllocSysString(),&Width,&Higth); }
资源推荐
资源详情
资源评论
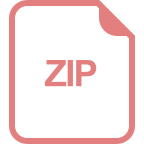
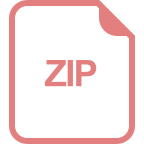
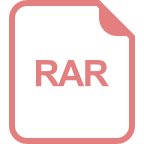
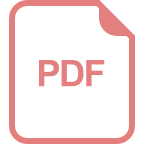
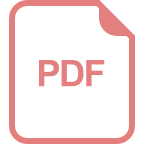
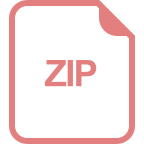
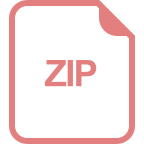
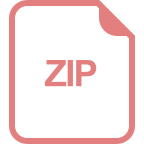
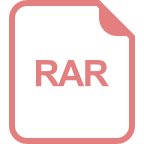
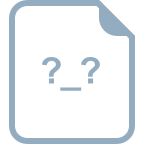
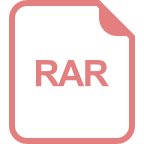
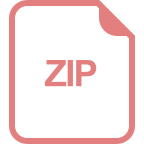
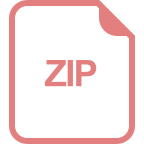
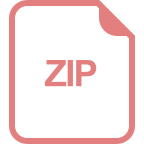
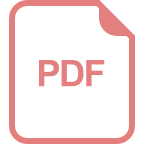
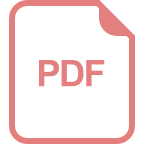
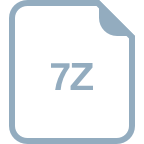
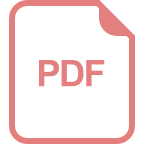
收起资源包目录

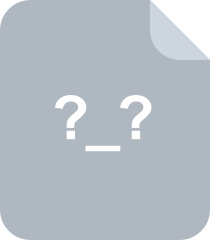
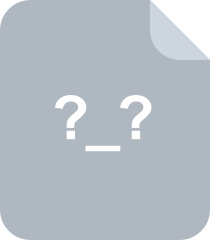
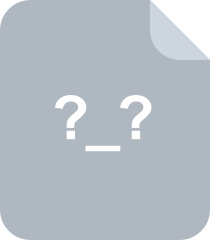
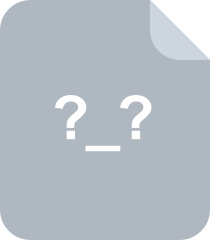
共 4 条
- 1
资源评论
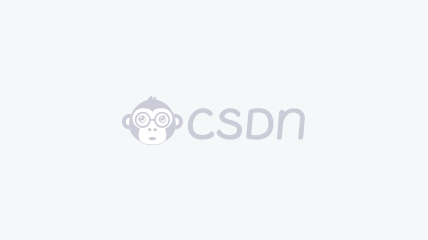
- C51ram2012-08-05可以用,下载学习,辛苦,谢谢

daiafei
- 粉丝: 83
- 资源: 54
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

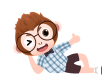
最新资源
- DirectX Overlay 的简单基础 .zip
- 1第一章Sympy介绍2(1).ipynb
- DirectX Math SIMD Pascal 数学库.zip
- 计算机网络第三章作业/
- DirectX API 的 Rust 包装器 .zip
- Spring Boot打造全方位家装服务管理平台:一站式解决方案的设计与实现
- 1. excel两张子表A列乱序对比-红色高亮显示不同之处 2. pdf转word 3. 合并多个pdf
- DirectX API 挂钩框架.zip
- DirectX 9 组件框架.zip
- 基于springboot+mybatis后台vue实现的音乐网站项目毕业设计源码+数据库(高分项目)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


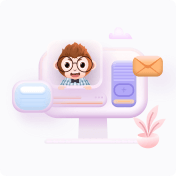
安全验证
文档复制为VIP权益,开通VIP直接复制
