<?xml version="1.0" encoding="utf-8"?><span>
<doc>
<assembly>
<name>System.Numerics.Vectors</name>
</assembly>
<members>
<member name="T:System.Numerics.Matrix3x2">
<summary>Represents a 3x2 matrix.</summary>
</member>
<member name="M:System.Numerics.Matrix3x2.#ctor(System.Single,System.Single,System.Single,System.Single,System.Single,System.Single)">
<summary>Creates a 3x2 matrix from the specified components.</summary>
<param name="m11">The value to assign to the first element in the first row.</param>
<param name="m12">The value to assign to the second element in the first row.</param>
<param name="m21">The value to assign to the first element in the second row.</param>
<param name="m22">The value to assign to the second element in the second row.</param>
<param name="m31">The value to assign to the first element in the third row.</param>
<param name="m32">The value to assign to the second element in the third row.</param>
</member>
<member name="M:System.Numerics.Matrix3x2.Add(System.Numerics.Matrix3x2,System.Numerics.Matrix3x2)">
<summary>Adds each element in one matrix with its corresponding element in a second matrix.</summary>
<param name="value1">The first matrix.</param>
<param name="value2">The second matrix.</param>
<returns>The matrix that contains the summed values of <paramref name="value1">value1</paramref> and <paramref name="value2">value2</paramref>.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateRotation(System.Single)">
<summary>Creates a rotation matrix using the given rotation in radians.</summary>
<param name="radians">The amount of rotation, in radians.</param>
<returns>The rotation matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateRotation(System.Single,System.Numerics.Vector2)">
<summary>Creates a rotation matrix using the specified rotation in radians and a center point.</summary>
<param name="radians">The amount of rotation, in radians.</param>
<param name="centerPoint">The center point.</param>
<returns>The rotation matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateScale(System.Single,System.Single)">
<summary>Creates a scaling matrix from the specified X and Y components.</summary>
<param name="xScale">The value to scale by on the X axis.</param>
<param name="yScale">The value to scale by on the Y axis.</param>
<returns>The scaling matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateScale(System.Single,System.Numerics.Vector2)">
<summary>Creates a scaling matrix that scales uniformly with the specified scale with an offset from the specified center.</summary>
<param name="scale">The uniform scale to use.</param>
<param name="centerPoint">The center offset.</param>
<returns>The scaling matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateScale(System.Single,System.Single,System.Numerics.Vector2)">
<summary>Creates a scaling matrix that is offset by a given center point.</summary>
<param name="xScale">The value to scale by on the X axis.</param>
<param name="yScale">The value to scale by on the Y axis.</param>
<param name="centerPoint">The center point.</param>
<returns>The scaling matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateScale(System.Single)">
<summary>Creates a scaling matrix that scales uniformly with the given scale.</summary>
<param name="scale">The uniform scale to use.</param>
<returns>The scaling matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateScale(System.Numerics.Vector2)">
<summary>Creates a scaling matrix from the specified vector scale.</summary>
<param name="scales">The scale to use.</param>
<returns>The scaling matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateScale(System.Numerics.Vector2,System.Numerics.Vector2)">
<summary>Creates a scaling matrix from the specified vector scale with an offset from the specified center point.</summary>
<param name="scales">The scale to use.</param>
<param name="centerPoint">The center offset.</param>
<returns>The scaling matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateSkew(System.Single,System.Single)">
<summary>Creates a skew matrix from the specified angles in radians.</summary>
<param name="radiansX">The X angle, in radians.</param>
<param name="radiansY">The Y angle, in radians.</param>
<returns>The skew matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateSkew(System.Single,System.Single,System.Numerics.Vector2)">
<summary>Creates a skew matrix from the specified angles in radians and a center point.</summary>
<param name="radiansX">The X angle, in radians.</param>
<param name="radiansY">The Y angle, in radians.</param>
<param name="centerPoint">The center point.</param>
<returns>The skew matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateTranslation(System.Numerics.Vector2)">
<summary>Creates a translation matrix from the specified 2-dimensional vector.</summary>
<param name="position">The translation position.</param>
<returns>The translation matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.CreateTranslation(System.Single,System.Single)">
<summary>Creates a translation matrix from the specified X and Y components.</summary>
<param name="xPosition">The X position.</param>
<param name="yPosition">The Y position.</param>
<returns>The translation matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.Equals(System.Numerics.Matrix3x2)">
<summary>Returns a value that indicates whether this instance and another 3x2 matrix are equal.</summary>
<param name="other">The other matrix.</param>
<returns>true if the two matrices are equal; otherwise, false.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.Equals(System.Object)">
<summary>Returns a value that indicates whether this instance and a specified object are equal.</summary>
<param name="obj">The object to compare with the current instance.</param>
<returns>true if the current instance and <paramref name="obj">obj</paramref> are equal; otherwise, false```. If <code data-dev-comment-type="paramref">obj</code> isnull, the method returnsfalse`.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.GetDeterminant">
<summary>Calculates the determinant for this matrix.</summary>
<returns>The determinant.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.GetHashCode">
<summary>Returns the hash code for this instance.</summary>
<returns>The hash code.</returns>
</member>
<member name="P:System.Numerics.Matrix3x2.Identity">
<summary>Gets the multiplicative identity matrix.</summary>
<returns>The multiplicative identify matrix.</returns>
</member>
<member name="M:System.Numerics.Matrix3x2.Invert(System.Numerics.Matrix3x2,System.Numerics.Matrix3x2@)">
<summary>Inverts the specified matrix. The return value indicates whether the operation succeeded.</summary>
<param name="matrix">The matrix to invert.</param>
<param name="result">When this method returns, contains the inverted matrix if the operation succeeded.</param>
<returns>true if <paramref name="matrix">matrix</paramref> was converted successfully; otherwise, false.</returns>
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
cocos creator的jsc解密程序,支持最新版。 请查看:https://blog.csdn.net/czysoft/article/details/105753711 有问题可以在底下留言,有时间我会改进的 这是最新版工具,需要.net framework4.7.2支持,支持1.9.3之前的版本的jsc解密 *修正有些情况下解出来还是PK开头的加密内容 *增加加密功能 *修正解密后中文为乱码的问题 *增加解密时显示解密方式(底下状态栏)
资源推荐
资源详情
资源评论
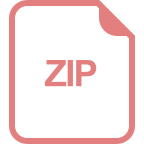
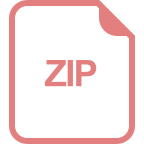
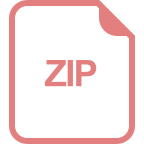
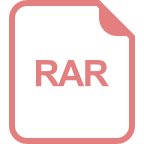
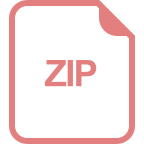
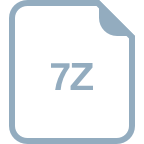
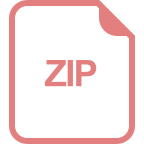
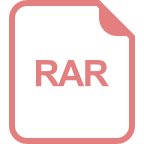
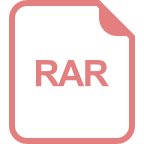
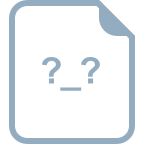
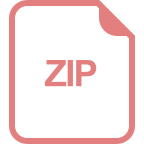
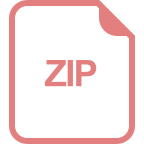
收起资源包目录

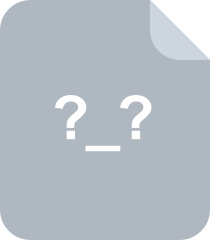
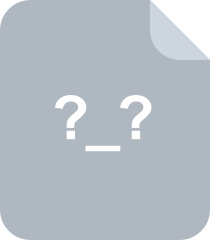
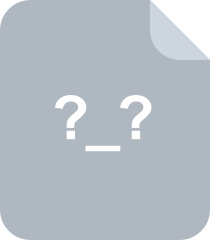
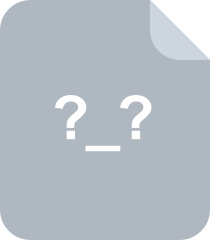
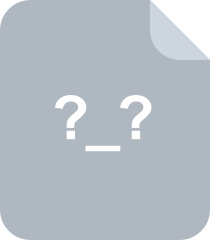
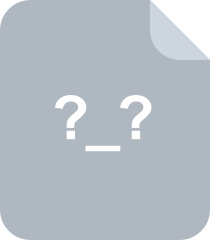
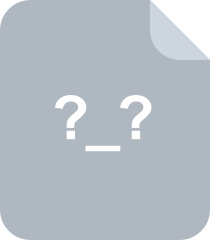
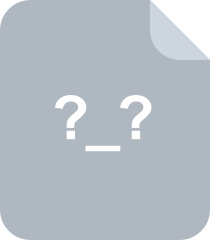
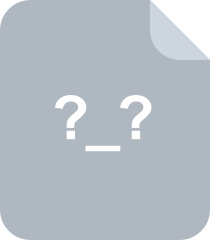
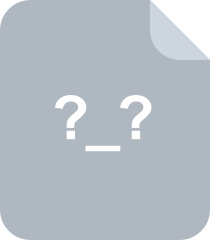
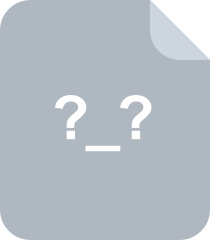
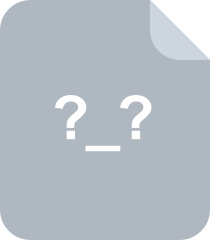
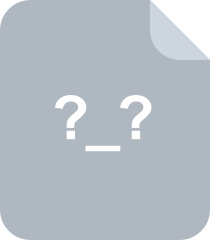
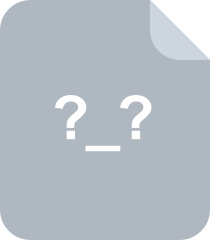
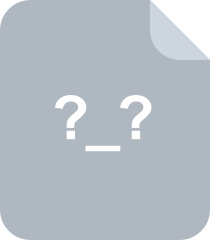
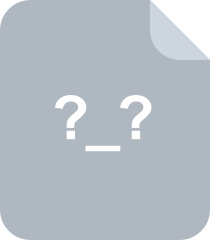
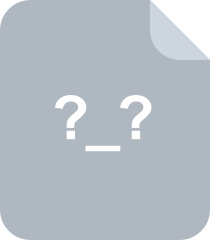
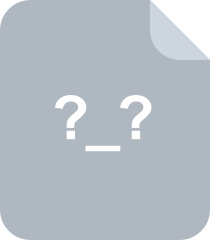
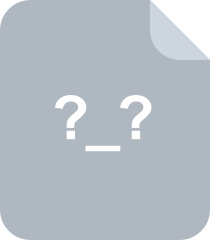
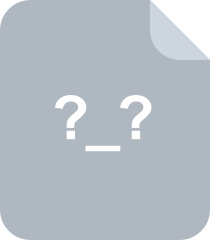
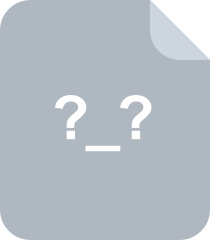
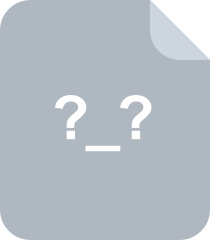
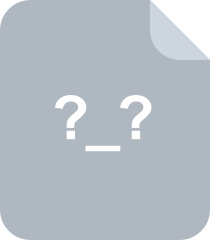
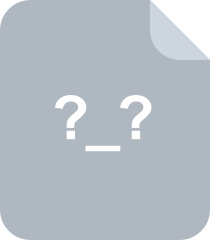
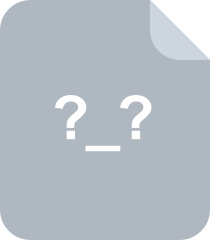
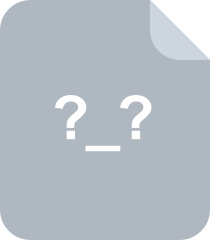
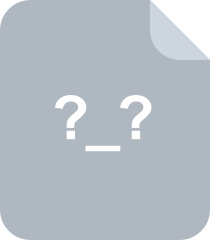
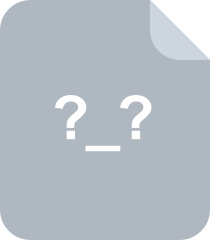
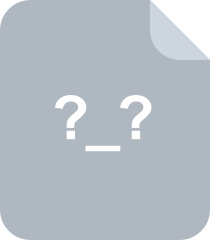
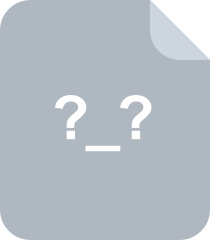
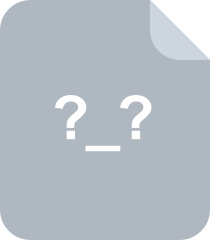
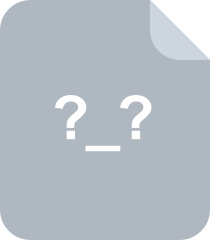
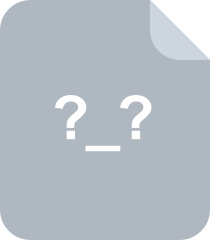
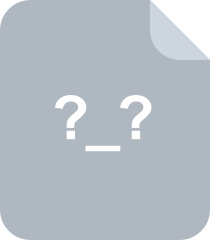
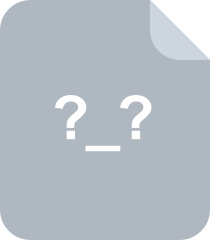
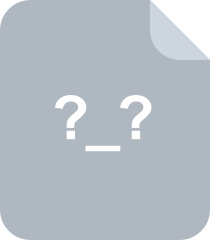
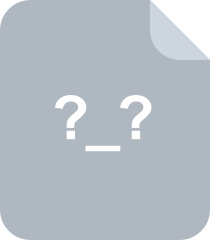
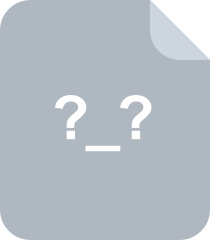
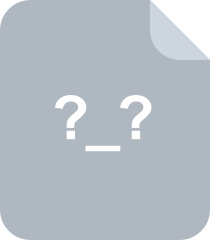
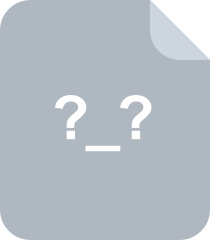
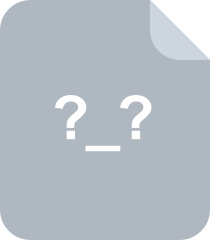
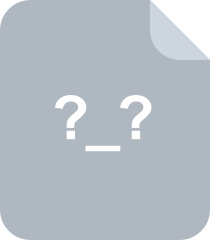
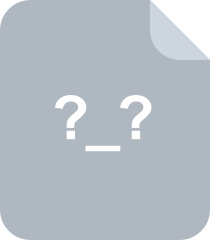
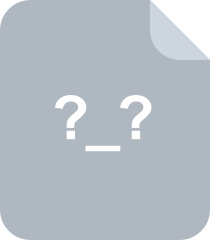
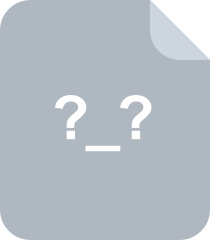
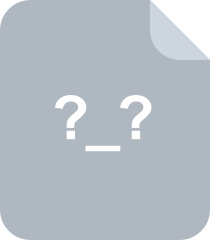
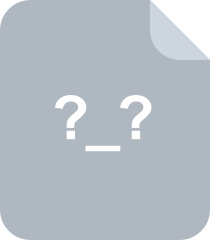
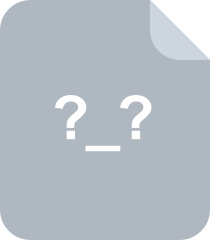
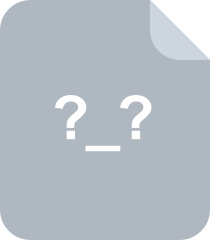
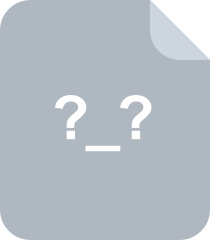
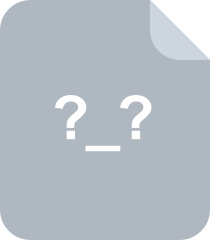
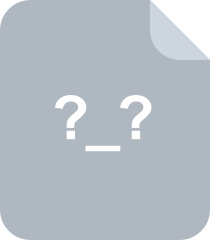
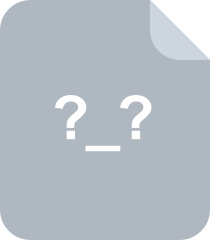
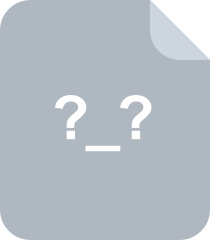
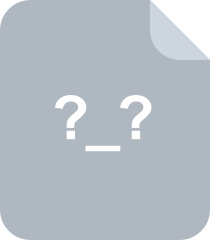
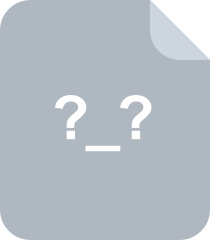
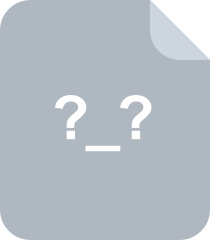
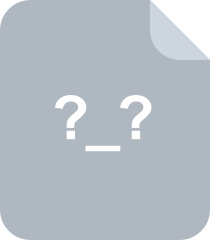
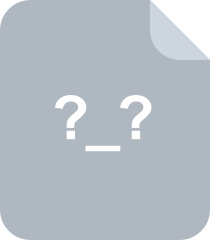
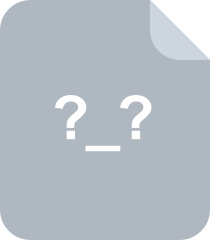
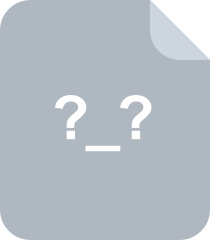
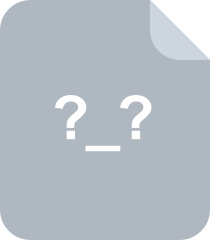
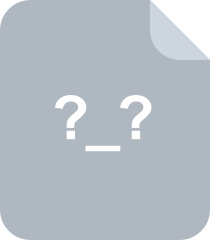
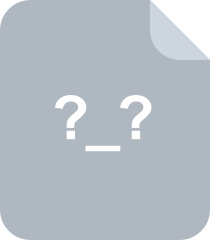
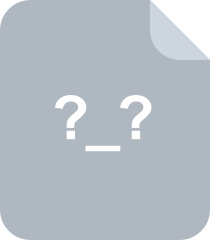
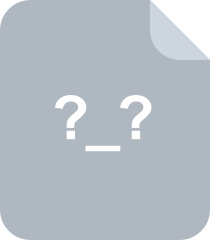
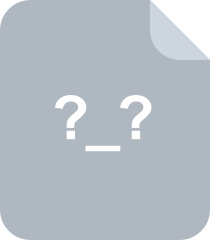
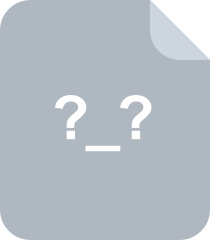
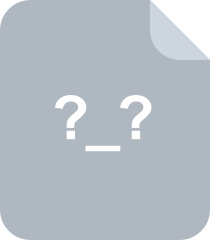
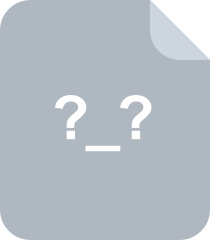
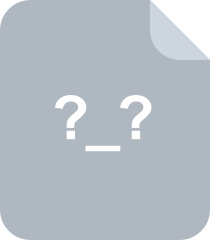
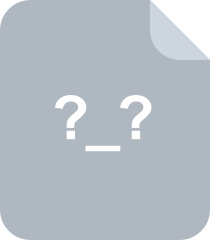
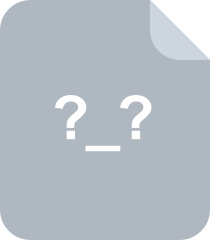
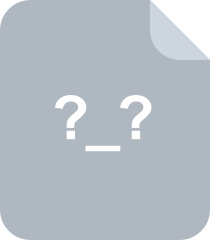
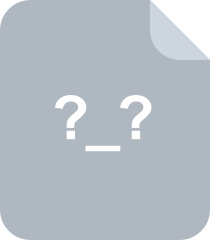
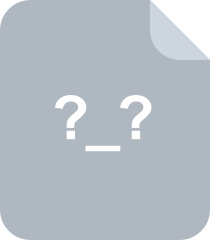
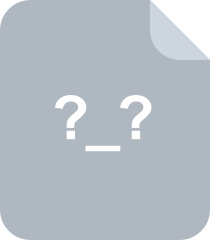
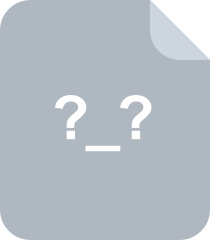
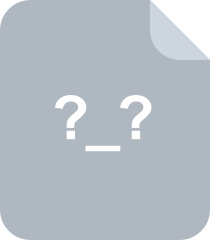
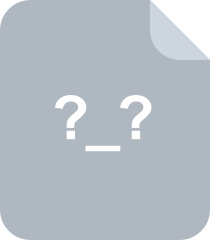
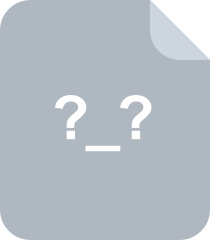
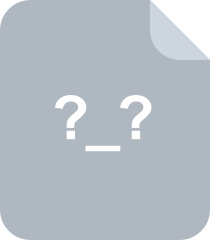
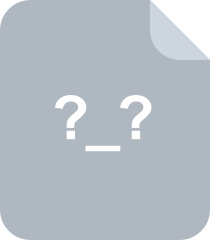
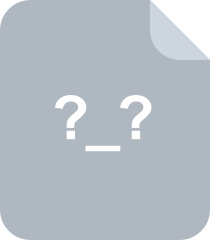
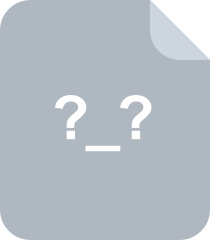
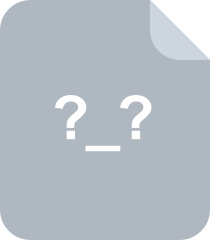
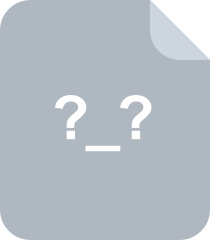
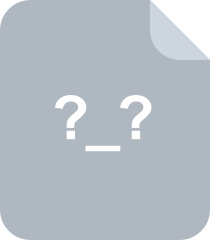
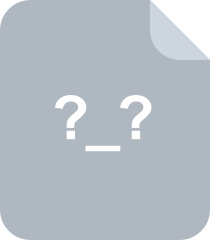
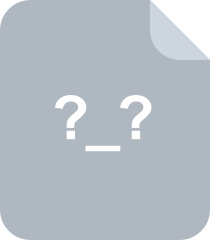
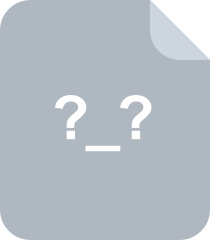
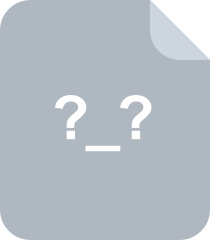
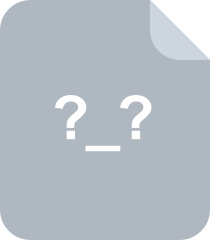
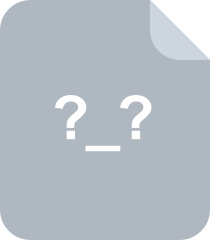
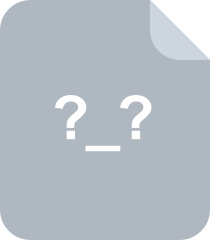
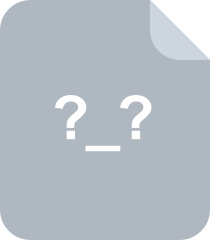
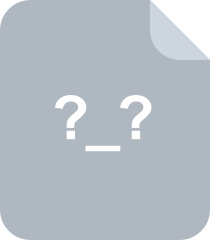
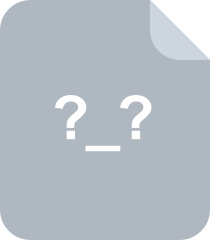
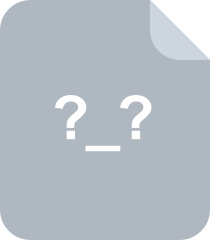
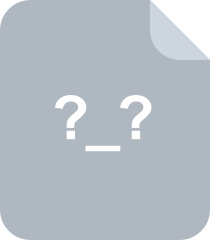
共 110 条
- 1
- 2
资源评论
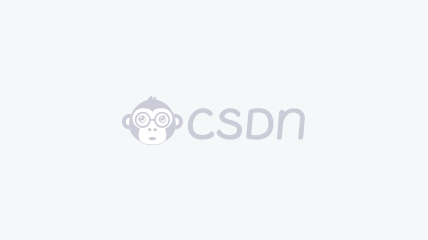
- 王清水2020-11-20这不是骗分???
- ttxyz55432021-04-05骗子,没有用压,需要密钥
- iosandAndroid2021-12-25应该还可以
- Ldkjfn2022-02-14都没有这个命令啊decrypt、有会的加我q2463536899.
- cexwovfpqq2021-07-30骗子,密钥正确的还乱码,别的地方不要分下载了一个一摸一样的,垃圾玩意

czysoft
- 粉丝: 21
- 资源: 19
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

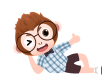
最新资源
- java毕设项目之ssm基于Vue.js的在线购物系统的设计与实现+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm汽车养护管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm简易版营业厅宽带系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm绿色农产品推广应用网站+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm人事管理信息系统+jsp(完整前后端+说明文档+mysql+lw).zip
- 自考04741《计算机网络原理》试题及答案2016-2018
- java毕设项目之ssm社区管理与服务的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm社区文化宣传网站+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm实验室耗材管理系统设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网络游戏公司官方平台设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm蜀都天香酒楼的网站设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网上医院预约挂号系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网上花店设计+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网上服装销售系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm小型企业办公自动化系统的设计和开发+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm物流管理系统设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


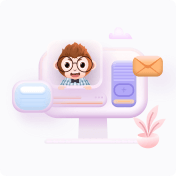
安全验证
文档复制为VIP权益,开通VIP直接复制
