基于SOAP的一卡通充值查询系统(axis2)
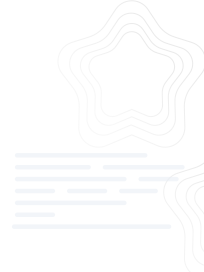


【基于SOAP的一卡通充值查询系统(axis2)】 一、SOAP(Simple Object Access Protocol)协议介绍 SOAP,简单对象访问协议,是一种轻量级的、基于XML的协议,用于在Web服务中交换结构化和类型化的信息。SOAP允许应用程序通过HTTP等传输协议进行通信,使得不同操作系统、编程语言和网络环境下的应用程序能够无缝地交换数据。SOAP消息通常包含三部分:Header(头)、Body(主体)和Fault(错误)。 二、Axis2框架解析 Axis2是Apache组织开发的一个开源Web服务引擎,它是基于SOAP协议的,用于构建和部署Web服务。Axis2提供了高性能、灵活且模块化的架构,支持多种编程模型,如Java代码、WSDL第一和第二样式。它包含了处理SOAP消息、编码和解码、事务管理以及安全性的组件,使得开发者可以更轻松地创建和消费Web服务。 三、一卡通充值查询系统设计 一卡通系统通常是指校园、企业或社区中用于支付小额消费的电子支付系统。在这个基于SOAP的系统中,用户可以通过Web服务接口进行充值和查询余额操作。系统可能包括以下几个关键组件: 1. 用户接口:用户可以通过网页、移动应用或其他客户端进行充值和查询操作。 2. Web服务接口:使用SOAP协议提供充值和查询功能的API,接受和响应XML格式的消息。 3. 数据库:存储用户账户信息、余额、交易记录等数据。 4. 安全机制:确保交易过程的安全性,如使用HTTPS加密传输、身份验证等。 5. 充值处理逻辑:接收充值请求,与银行或其他支付网关交互完成充值操作,并更新数据库。 6. 查询逻辑:根据用户请求,从数据库中检索余额信息并返回。 四、开发和学习价值 对于初学者来说,这个基于Axis2的一卡通充值查询系统具有以下学习价值: 1. 理解SOAP协议:通过实际项目,可以深入理解SOAP消息的结构和交互过程。 2. 掌握Axis2框架:学习如何利用Axis2创建和部署Web服务,以及如何处理SOAP消息。 3. Web服务开发:实践Web服务开发流程,包括编写WSDL文件、生成客户端和服务端代码。 4. 数据库设计与操作:了解如何设计数据库表结构,以及如何使用SQL语句进行数据操作。 5. 安全与测试:学习如何在Web服务中实现安全性措施,并进行功能和性能测试。 五、实际应用与扩展 基于SOAP的一卡通系统可以应用于多个场景,例如: 1. 校园生活:学生可以在食堂、图书馆、商店等地使用一卡通支付,方便快捷。 2. 企业环境:员工的门禁、食堂、内部消费等都可以通过一卡通实现。 3. 社区服务:居民的水电煤气缴费、停车费等可以通过一卡通支付。 系统还可以进一步扩展,例如集成更多支付方式、添加退款功能、实现多语言支持等,以满足不同用户的需求。 总结,基于SOAP的一卡通充值查询系统利用了Axis2框架实现了Web服务功能,对于学习和理解SOAP协议、Web服务开发以及数据库操作等方面具有很高的实践意义。同时,这样的系统在日常生活和工作中有着广泛的应用前景。





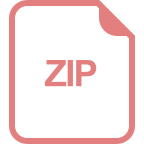









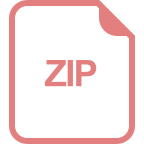








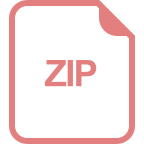



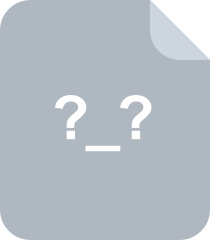
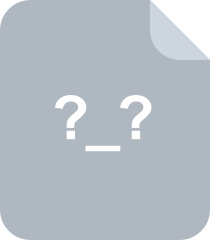
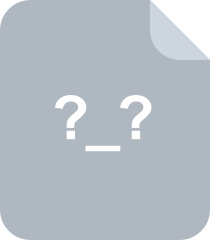
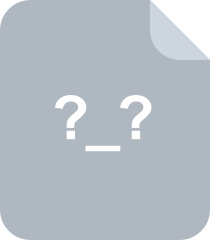
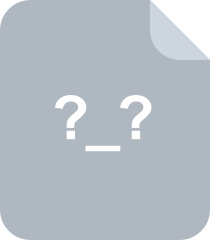
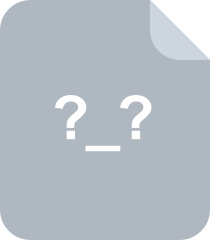
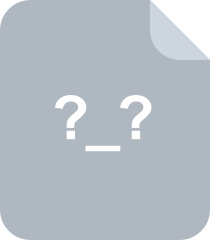
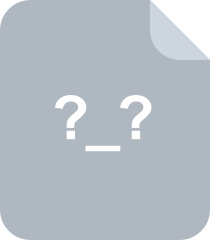
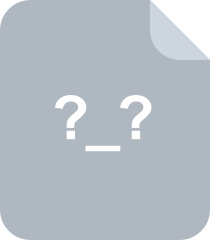
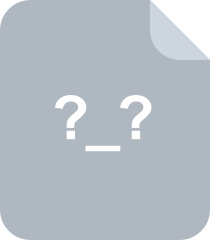
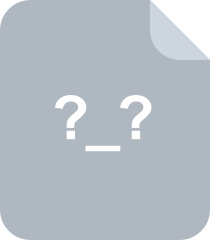
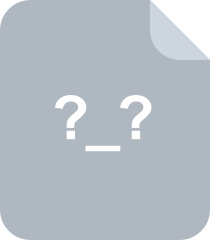
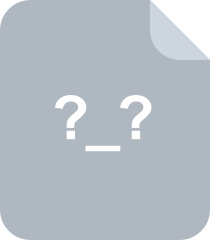
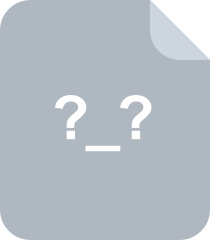
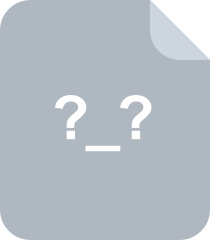
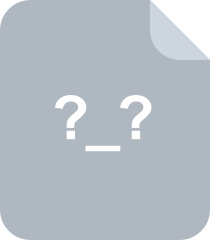
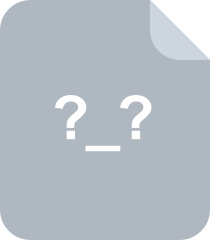
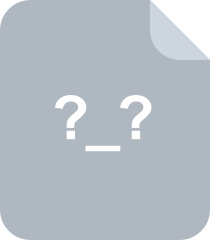
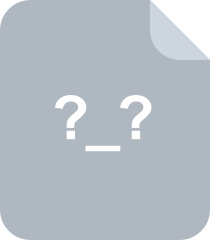
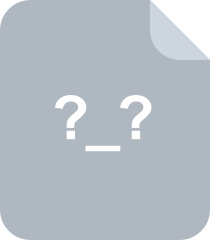
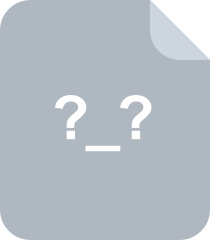
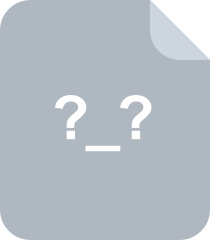
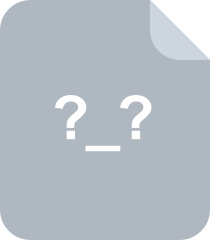
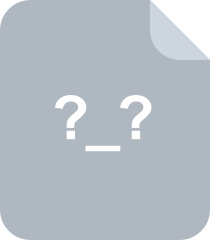
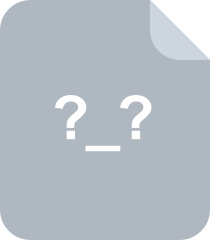
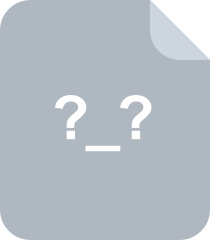
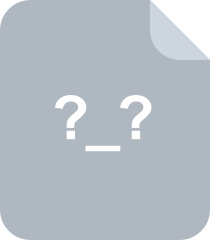
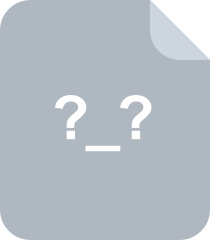
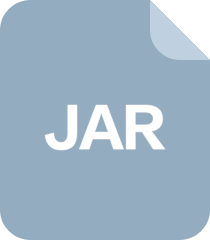
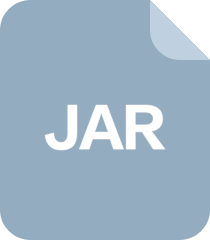
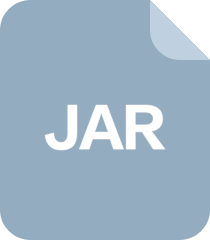
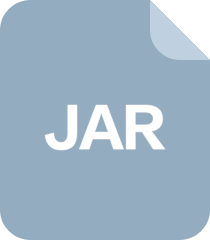
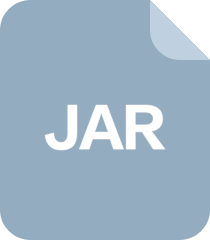
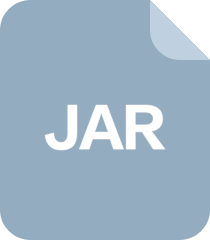
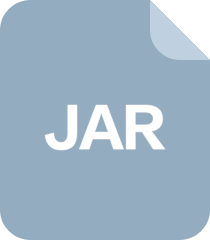
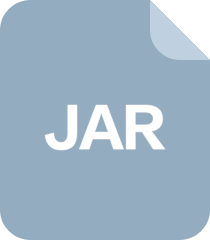
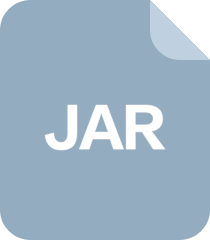
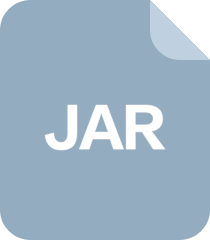
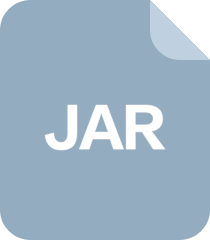
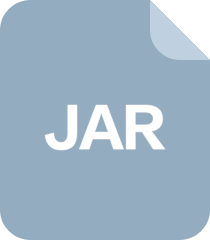
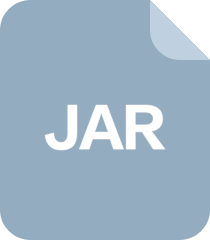
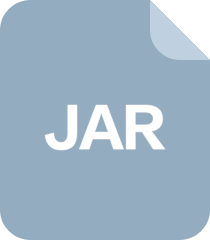
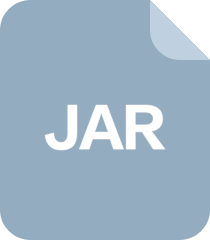
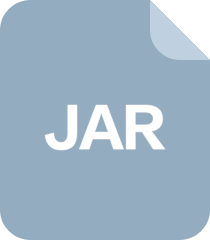
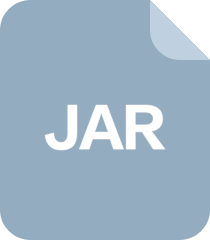
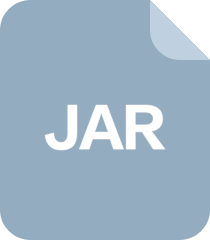
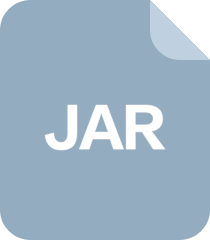
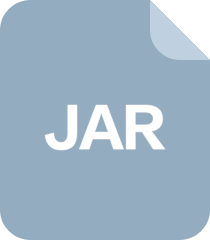
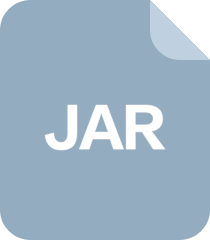
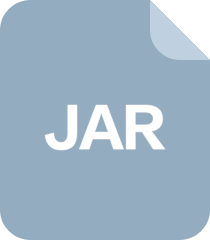
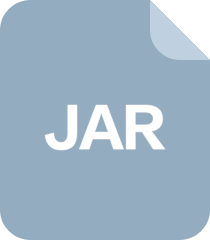
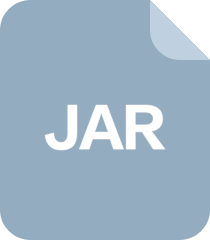
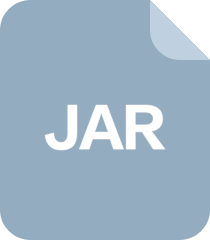
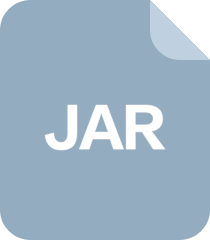
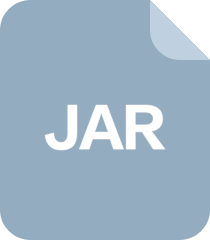
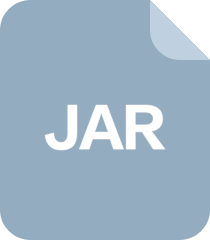
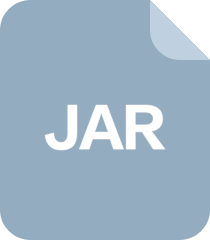
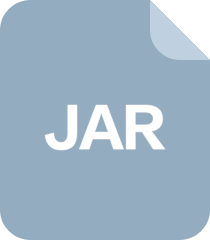
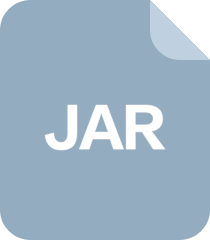
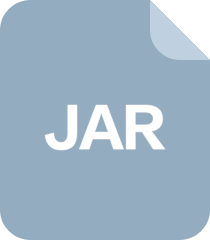
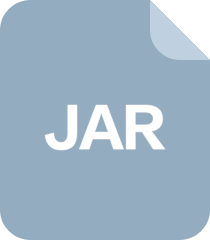
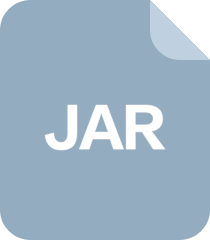
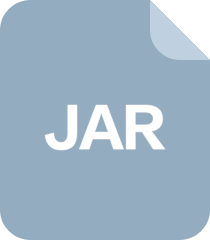
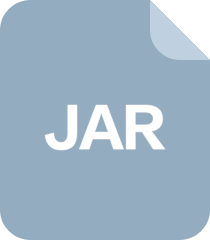
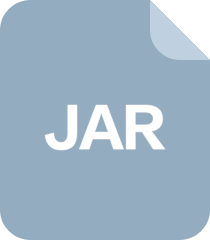
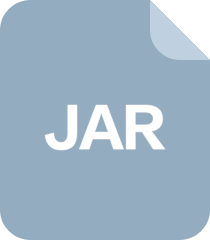
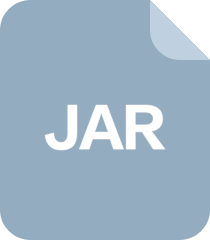
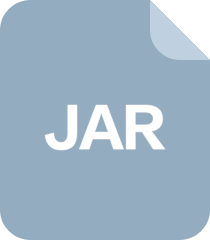
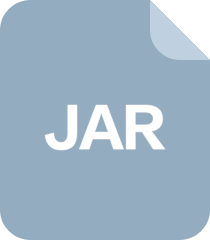
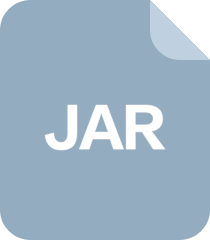
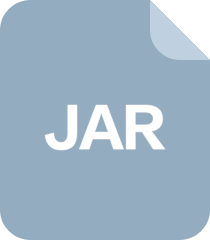
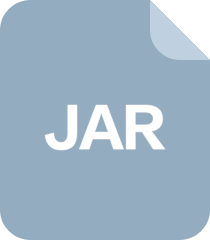
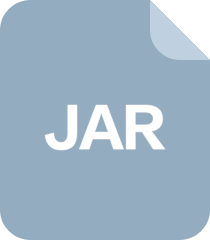
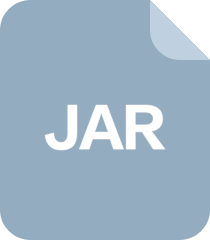
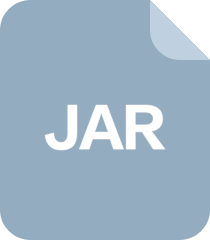
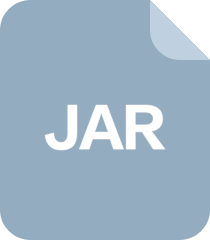
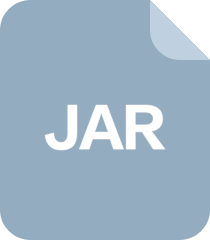
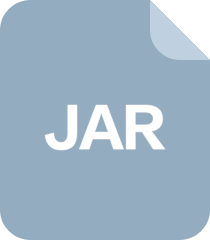
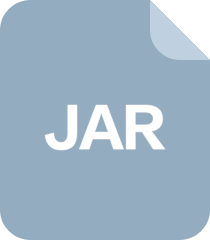
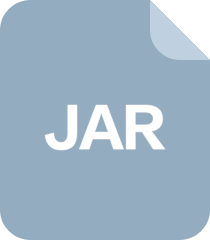
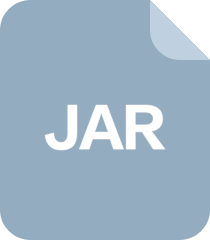
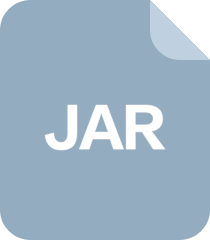
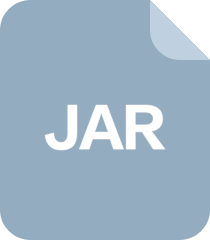
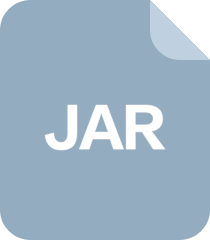
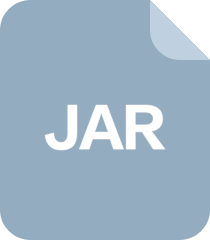
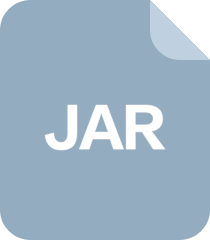
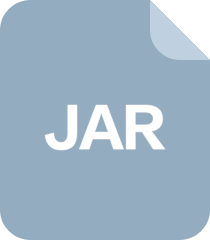
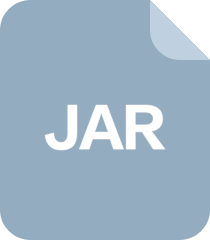
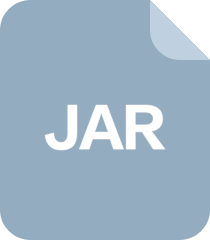
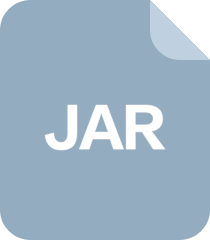
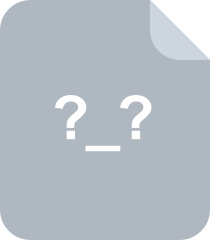
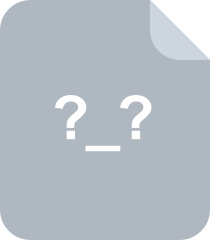
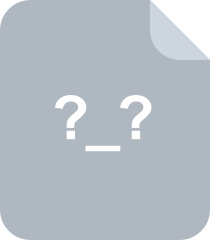
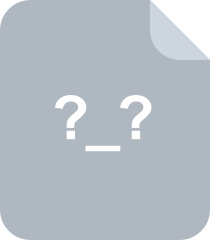
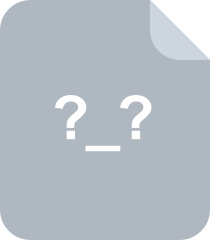
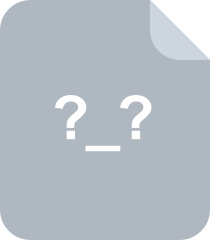
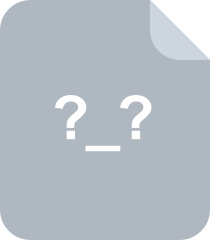
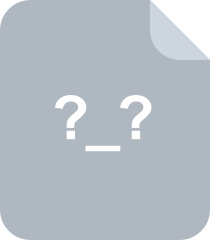
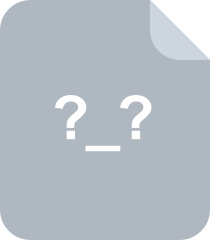
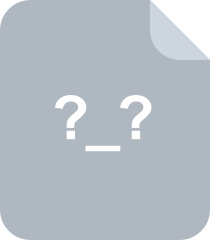
- 1
- 2
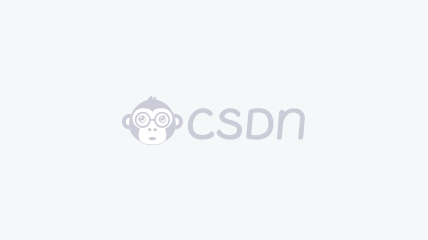
- zhongguorenmeiran2012-12-17都是基础性的,没有太过深入╮(╯▽╰)╭
- liuxsqq2014-02-18例子写的不错,就是axis里面那个4000多行的类是要自己写的吗,好难
- dmstudy2012-08-03还行了,就是还是不够详细

- 粉丝: 34
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

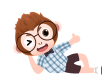
最新资源
- Matlab魔术轮胎公式:轮胎动力学仿真研究,涵盖制动、转弯及联合工况,解析纵向力与滑动率、侧向力与侧偏角关系,附参考文献及代码仿真复现 ,Matlab魔术轮胎公式:轮胎动力学仿真研究,涵盖制动、转弯
- 纯电动汽车Simulink整车仿真模型:构建高效动力系统,探索未来驾驶体验,纯电动汽车Simulink整车仿真模型构建与性能分析,纯电动汽车(含增程式)的 Simulink 整车仿真模型 ,纯电动;
- 贝叶斯优化算法在PID参数自动调参中的应用:一阶至高阶控制系统的自适应解决方案(附详细注释的M文件),贝叶斯优化算法在PID参数自动调整中的应用:一阶至高阶控制系统的自适应调参方法及详细注释说明,贝叶
- 基于Matlab的2PSK调制与解调系统仿真:原理、实现与源文件详解说明文档,基于MATLAB的2PSK调制与解调系统仿真及其详细说明文档与仿真源文件研究分析,基于matlab的2PSK调制与解调系统
- 基于BP神经网络的复杂故障诊断在三相逆变器仿真复现中的应用研究,基于BP神经网络的智能三相逆变器故障诊断技术研究与仿真复现:深度探索与验证,基于BP神经网络的三相逆变器故障诊断研究,仿真复现 ,基于B
- 四轮独立线控转向系统与Carsim-Simulink联合仿真的研究与应用,四轮转向线控转向系统的Carsim与Simulink联合仿真研究与实践,四轮转向线控转向系统的carsim与simulink联
- 基于Matlab与Simulink的六自由度水下机器人滑模控制运动模型:无差度轨迹跟踪效果与S-function及Matlab function互换性应用与说明文档,基于Matlab与Simulink
- C#与Halcon运动控制及视觉框架源码联合,可自定义连线式运动控制,开源可二次开发视觉处理利器 ,C#结合Halcon视觉框架的运动控制源代码分享与二次开发指南,C#联合Halcon运动控制源代码
- COMSOL MXene超材料吸收器的性能研究:探索高效能量转换与吸收机制,COMSOL MXene超材料吸收器的性能研究:探索高效能量转换与吸收机制,comsol MXene超材料吸收器 ,com
- 船用柴油机Simulink动态模型:四缸CI发动机设计与仿真(带PID控制)参考文档,船用柴油机Simulink动态模型构建:四缸CI发动机PID控制详解与文献参考,船用柴油机Simulink动态模
- 基于SOGI二阶广义积分器和高频注入的PMSM无速度传感器控制策略研究-MATLAB/Simulink仿真及传统滤波方法对比,基于pmsm永磁同步电机的高频注入技术与sogi二阶广义积分器传统滤波在
- 基于C++和C语言的农村污水处理系统设计源码
- 基于HTML和CSS技术的大学学业作业仓库设计源码
- 基于COMSOL的三相变压器仿真:振动噪声、温度及多场耦合计算的综合性研究,基于COMSOL的三相变压器仿真:振动噪声、温度场及多物理场耦合计算的研究,COMSOL三相变压器仿真振动噪声温度 变压器磁
- 基于JavaScript技术的自有家用源建设二分队设计源码
- 基于Vue与Electron的跨平台可视化页面与流程设计源码

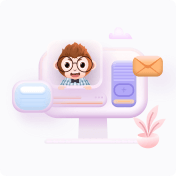
