/*
* Copyright (c) 2004-2007 The Trustees of Indiana University and Indiana
* University Research and Technology
* Corporation. All rights reserved.
* Copyright (c) 2004-2005 The University of Tennessee and The University
* of Tennessee Research Foundation. All rights
* reserved.
* Copyright (c) 2004-2005 High Performance Computing Center Stuttgart,
* University of Stuttgart. All rights reserved.
* Copyright (c) 2004-2005 The Regents of the University of California.
* All rights reserved.
* Copyright (c) 2015 Research Organization for Information Science
* and Technology (RIST). All rights reserved.
* Copyright (c) 2015 Los Alamos National Security, LLC. All rights
* reserved.
* Copyright (c) 2017-2018 FUJITSU LIMITED. All rights reserved.
* $COPYRIGHT$
*
* Additional copyrights may follow
*
* $HEADER$
*
*
* This file is almost a complete re-write for Open MPI compared to the
* original mpiJava package. Its license and copyright are listed below.
* See <path to ompi/mpi/java/README> for more information.
*
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*
* File : Comm.java
* Author : Sang Lim, Sung-Hoon Ko, Xinying Li, Bryan Carpenter
* Created : Thu Apr 9 12:22:15 1998
* Revision : $Revision: 1.20 $
* Updated : $Date: 2001/08/07 16:36:25 $
* Copyright: Northeast Parallel Architectures Center
* at Syracuse University 1998
*
*
*
* IMPLEMENTATION DETAILS
*
* All methods with buffers that can be direct or non direct have
* a companion argument 'db' which is true if the buffer is direct.
* For example, if the buffer argument is recvBuf, the companion
* argument will be 'rdb', meaning if the receive buffer is direct.
*
* Checking if a buffer is direct is faster in Java than C.
*/
package mpi;
import java.nio.*;
import static mpi.MPI.assertDirectBuffer;
/**
* The {@code Comm} class represents communicators.
*/
public class Comm implements Freeable, Cloneable
{
public final static int TYPE_SHARED = 0;
protected final static int SELF = 1;
protected final static int WORLD = 2;
protected long handle;
private Request request;
private static long nullHandle;
static
{
init();
}
private static native void init();
protected Comm()
{
}
protected Comm(long handle)
{
this.handle = handle;
}
protected Comm(long[] commRequest)
{
handle = commRequest[0];
request = new Request(commRequest[1]);
}
protected final void setType(int type)
{
getComm(type);
}
private native void getComm(int type);
/**
* Duplicates this communicator.
* <p>Java binding of {@code MPI_COMM_DUP}.
* <p>It is recommended to use {@link #dup} instead of {@link #clone}
* because the last can't throw an {@link mpi.MPIException}.
* @return copy of this communicator
*/
@Override public Comm clone()
{
try
{
return dup();
}
catch(MPIException e)
{
throw new RuntimeException(e.getMessage());
}
}
/**
* Duplicates this communicator.
* <p>Java binding of {@code MPI_COMM_DUP}.
* @return copy of this communicator
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
public Comm dup() throws MPIException
{
MPI.check();
return new Comm(dup(handle));
}
protected final native long dup(long comm) throws MPIException;
/**
* Duplicates this communicator.
* <p>Java binding of {@code MPI_COMM_IDUP}.
* <p>The new communicator can't be used before the operation completes.
* The request object must be obtained calling {@link #getRequest}.
* @return copy of this communicator
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
public Comm iDup() throws MPIException
{
MPI.check();
return new Comm(iDup(handle));
}
protected final native long[] iDup(long comm) throws MPIException;
/**
* Duplicates this communicator with the info object used in the call.
* <p>Java binding of {@code MPI_COMM_DUP_WITH_INFO}.
* @param info info object to associate with the new communicator
* @return copy of this communicator
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
public Comm dupWithInfo(Info info) throws MPIException
{
MPI.check();
return new Comm(dupWithInfo(handle, info.handle));
}
protected final native long dupWithInfo(long comm, long info) throws MPIException;
/**
* Returns the associated request to this communicator if it was
* created using {@link #iDup}.
* @return associated request if this communicator was created
* using {@link #iDup}, or null otherwise.
*/
public final Request getRequest()
{
return request;
}
/**
* Size of group of this communicator.
* <p>Java binding of the MPI operation {@code MPI_COMM_SIZE}.
* @return number of processors in the group of this communicator
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
public final int getSize() throws MPIException
{
MPI.check();
return getSize(handle);
}
private native int getSize(long comm) throws MPIException;
/**
* Rank of this process in group of this communicator.
* <p>Java binding of the MPI operation {@code MPI_COMM_RANK}.
* @return rank of the calling process in the group of this communicator
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
public final int getRank() throws MPIException
{
MPI.check();
return getRank(handle);
}
private native int getRank(long comm) throws MPIException;
/**
* Compare two communicators.
* <p>Java binding of the MPI operation {@code MPI_COMM_COMPARE}.
* @param comm1 first communicator
* @param comm2 second communicator
* @return
* {@code MPI.IDENT} results if the {@code comm1} and {@code comm2}
* are references to the same object (ie, if {@code comm1 == comm2}).<br>
* {@code MPI.CONGRUENT} results if the underlying groups are identical
* but the communicators differ by context.<br>
* {@code MPI.SIMILAR} results if the underlying groups are similar
* but the communicators differ by context.<br>
* {@code MPI.UNEQUAL} results otherwise.
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
public static int compare(Comm comm1, Comm comm2) throws MPIException
{
MPI.check();
return compare(comm1.handle, comm2.handle);
}
private static native int compare(long comm1, long comm2) throws MPIException;
/**
* Java binding of the MPI operation {@code MPI_COMM_FREE}.
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
@Override final public void free() throws MPIException
{
MPI.check();
handle = free(handle);
}
private native long free(long comm) throws MPIException;
/**
* Test if communicator object is null (has been freed).
* Java binding of {@code MPI_COMM_NULL}.
* @return true if the comm object is null, false otherwise
*/
public final boolean isNull()
{
return handle == nullHandle;
}
/**
* Java binding of {@code MPI_COMM_SET_INFO}.
* @param info info object
* @throws MPIException Signals that an MPI exception of some sort has occurred.
*/
public final void setInfo(Info info) throws MPIException
{
MPI.check();
setInfo(handle, info.ha
没有合适的资源?快使用搜索试试~ 我知道了~
ubuntu openmpi下载
需积分: 0 1 下载量 19 浏览量
2022-12-04
11:58:50
上传
评论
收藏 16.37MB GZ 举报
温馨提示
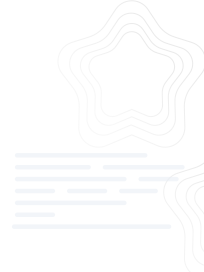
共7645个文件
c:3149个
h:1162个
f90:767个

ubuntu openmpi下载
资源推荐
资源详情
资源评论
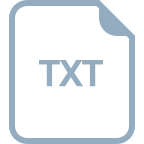
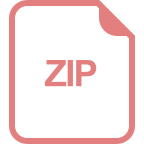
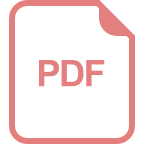
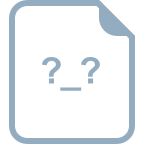
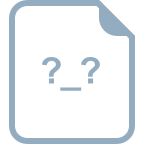
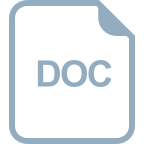
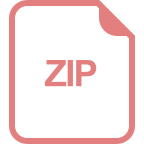
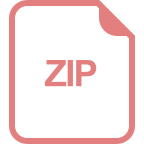
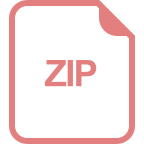
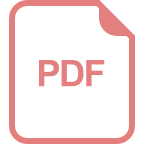
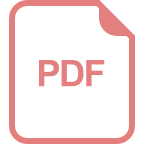
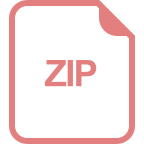
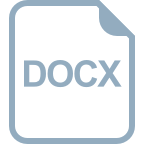
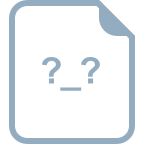
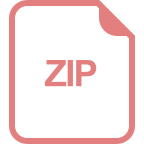
收起资源包目录

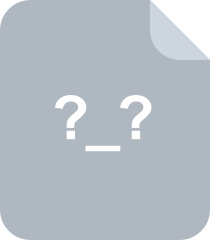
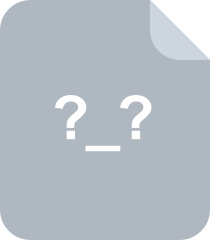
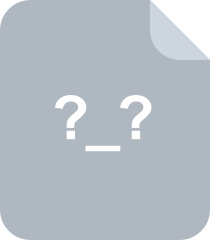
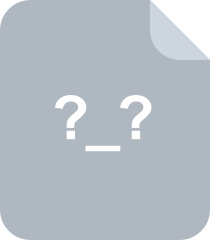
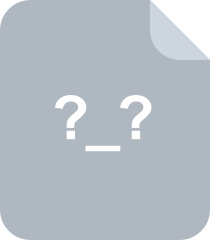
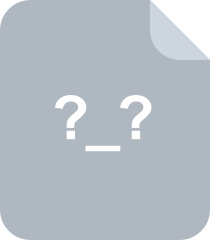
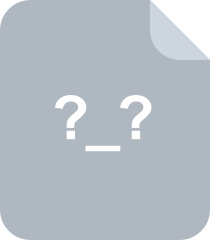
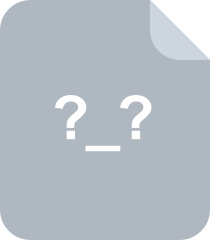
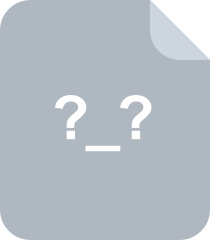
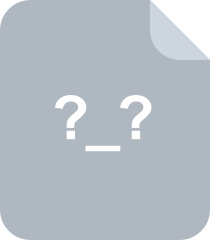
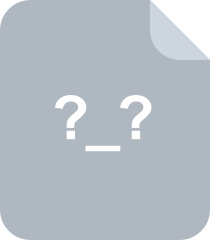
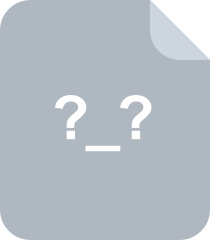
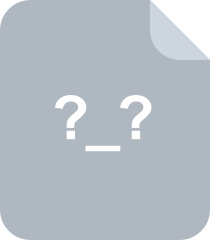
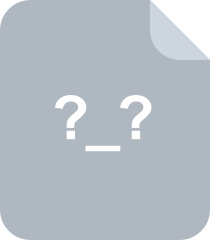
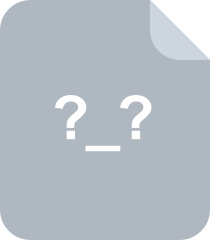
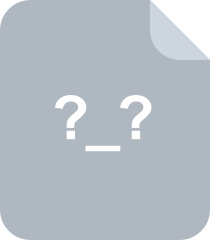
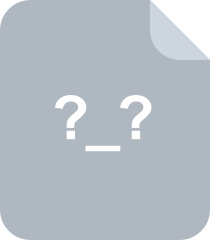
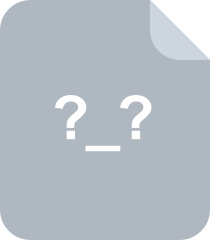
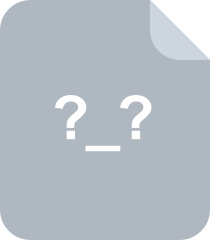
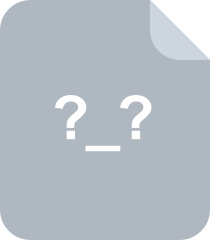
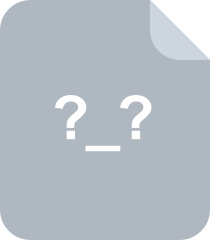
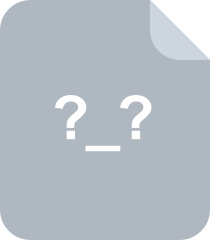
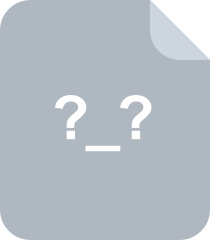
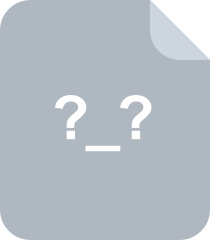
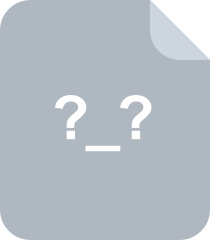
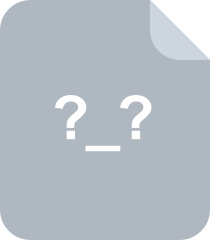
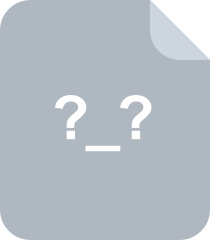
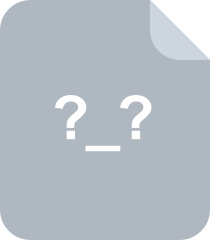
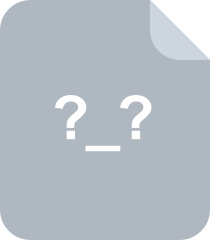
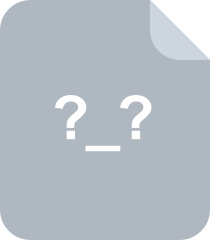
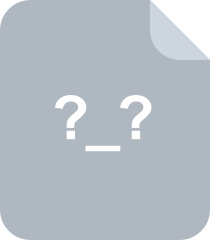
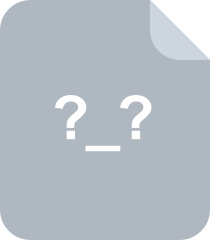
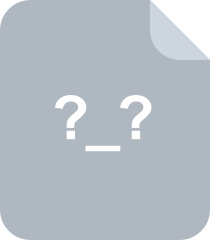
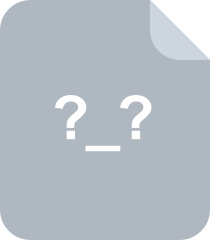
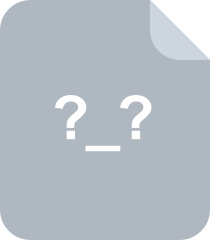
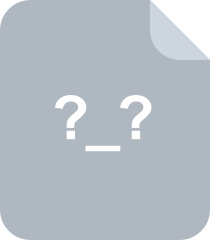
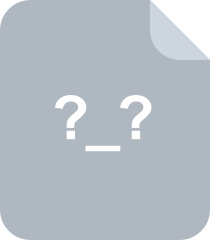
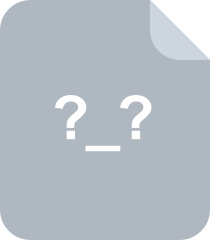
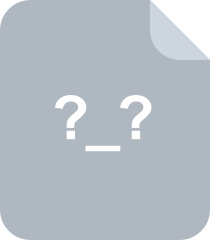
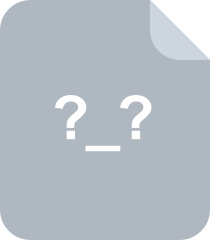
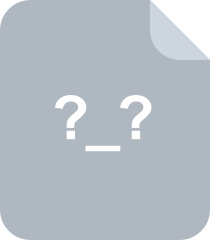
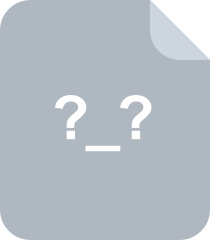
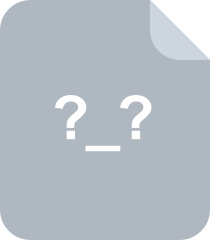
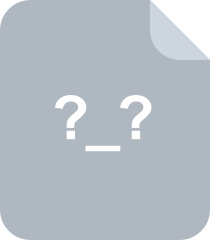
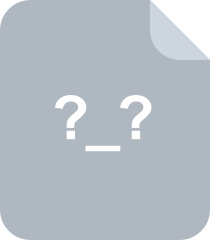
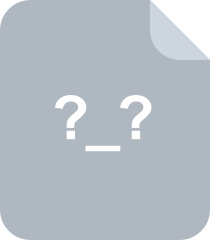
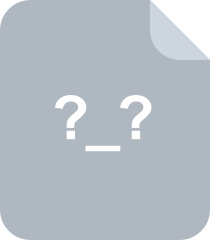
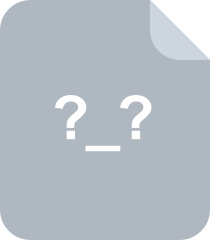
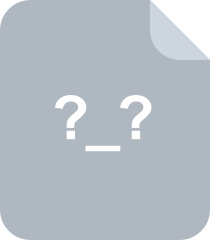
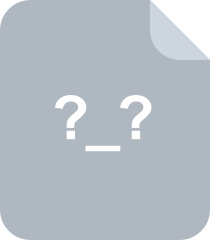
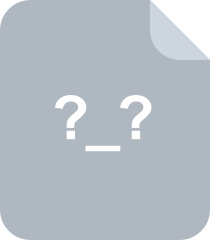
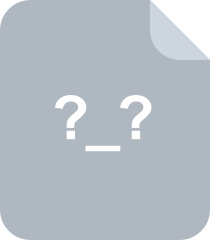
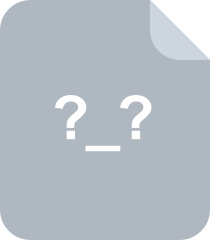
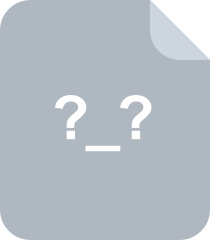
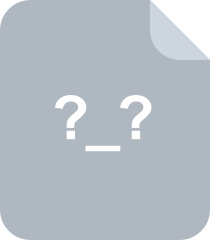
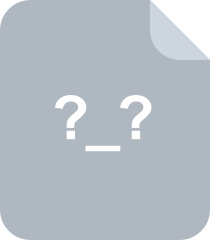
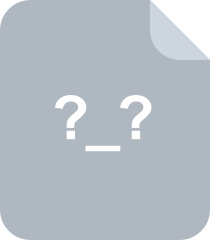
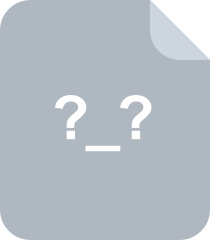
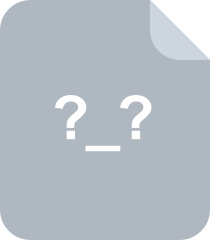
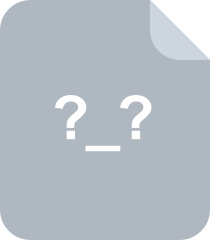
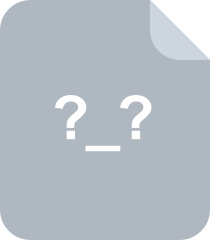
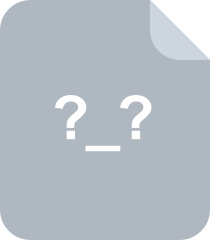
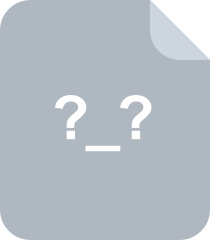
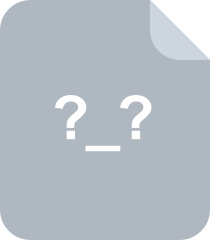
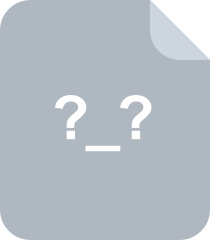
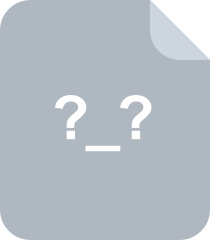
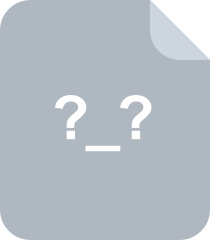
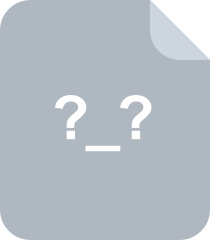
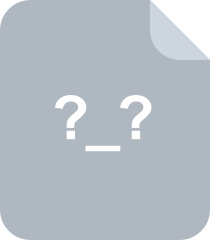
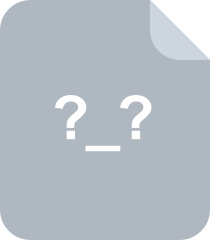
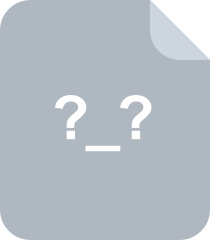
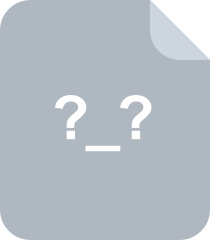
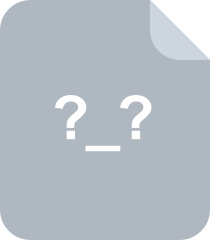
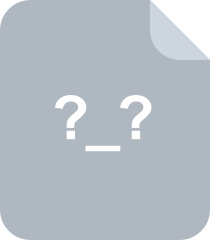
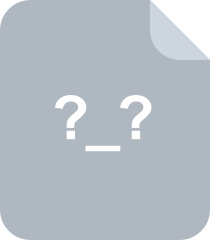
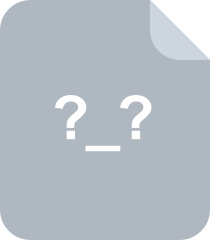
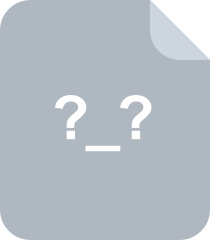
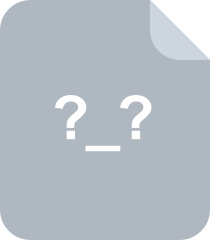
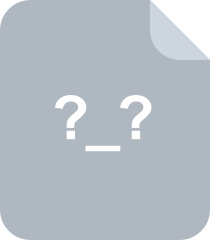
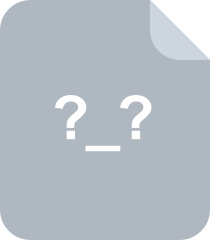
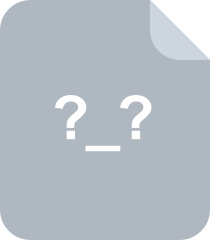
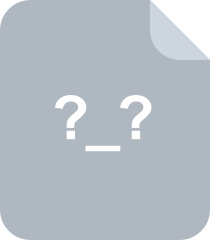
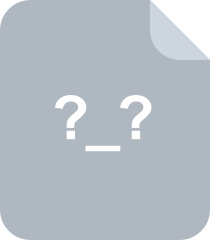
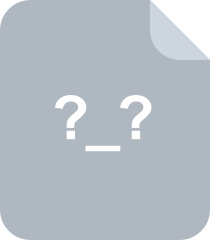
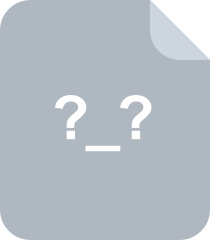
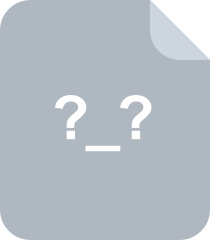
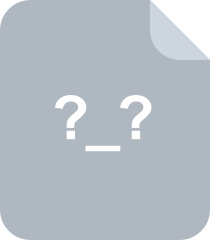
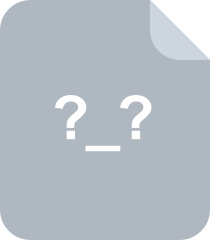
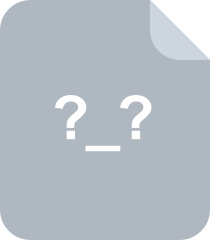
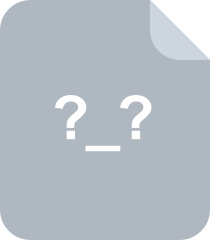
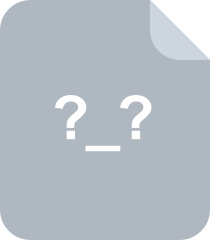
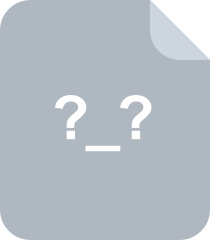
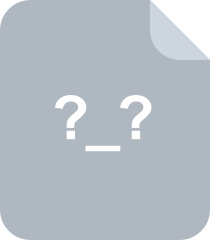
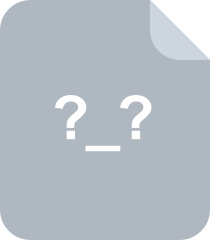
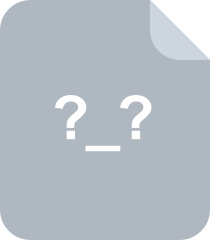
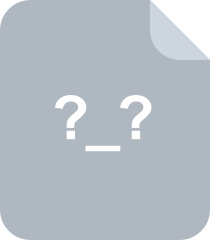
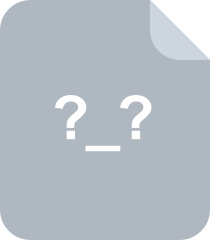
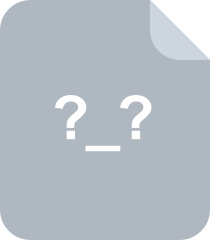
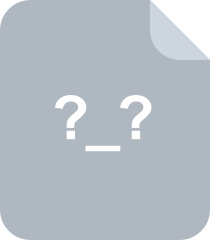
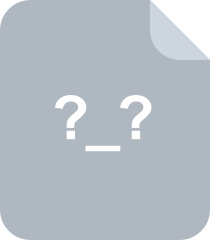
共 7645 条
- 1
- 2
- 3
- 4
- 5
- 6
- 77
资源评论
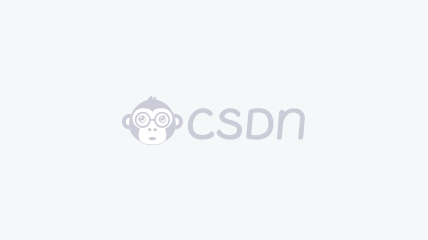

爱学习的广东仔
- 粉丝: 1w+
- 资源: 130
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

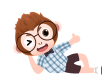
安全验证
文档复制为VIP权益,开通VIP直接复制
