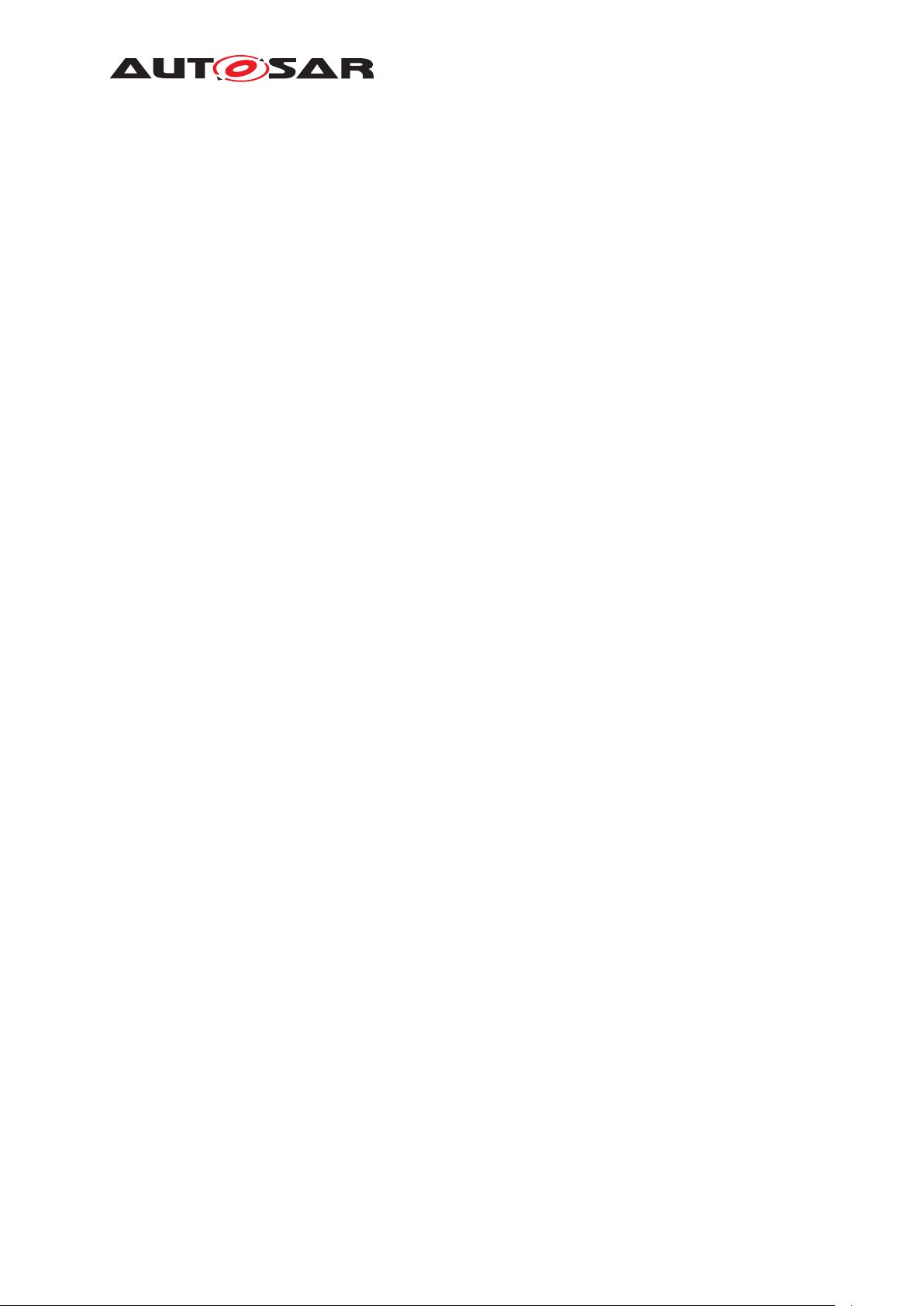
Guidelines for the use of the C++14 language in critical and safety-related systems
AUTOSAR AP Release 17-03
6.10.2 Member name lookup . . . . . . . . . . . . . . . . . . . . . . 132
6.10.3 Virtual functions . . . . . . . . . . . . . . . . . . . . . . . . . 133
6.11 Member access control . . . . . . . . . . . . . . . . . . . . . . . . . . . 139
6.11.0 General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 139
6.11.3 Friends . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 142
6.12 Special member functions . . . . . . . . . . . . . . . . . . . . . . . . . 142
6.12.0 General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 142
6.12.1 Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . 143
6.12.4 Destructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . 149
6.12.6 Initialization . . . . . . . . . . . . . . . . . . . . . . . . . . . . 151
6.12.7 Construction and destructions . . . . . . . . . . . . . . . . . 153
6.12.8 Copying and moving class objects . . . . . . . . . . . . . . . 155
6.13 Overloading . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 167
6.13.1 Overloadable declarations . . . . . . . . . . . . . . . . . . . 167
6.13.2 Declaration matching . . . . . . . . . . . . . . . . . . . . . . 170
6.13.3 Overload resolution . . . . . . . . . . . . . . . . . . . . . . . 173
6.13.5 Overloaded operators . . . . . . . . . . . . . . . . . . . . . . 174
6.13.6 Build-in operators . . . . . . . . . . . . . . . . . . . . . . . . 175
6.14 Templates . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 176
6.14.0 General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 176
6.14.1 Template parameters . . . . . . . . . . . . . . . . . . . . . . 176
6.14.5 Template declarations . . . . . . . . . . . . . . . . . . . . . . 179
6.14.6 Name resolution . . . . . . . . . . . . . . . . . . . . . . . . . 179
6.14.7 Template instantiation and specialization . . . . . . . . . . . 179
6.14.8 Function template specializations . . . . . . . . . . . . . . . 181
6.15 Exception handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183
6.15.0 General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 186
6.15.1 Throwing an exception . . . . . . . . . . . . . . . . . . . . . 201
6.15.2 Constructors and destructors . . . . . . . . . . . . . . . . . . 211
6.15.3 Handling an exception . . . . . . . . . . . . . . . . . . . . . . 215
6.15.4 Exception specifications . . . . . . . . . . . . . . . . . . . . . 225
6.15.5 Special functions . . . . . . . . . . . . . . . . . . . . . . . . . 234
6.16 Preprocessing directives . . . . . . . . . . . . . . . . . . . . . . . . . . 241
6.16.0 General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 241
6.16.1 Conditional inclusion . . . . . . . . . . . . . . . . . . . . . . . 244
6.16.2 Source file inclusion . . . . . . . . . . . . . . . . . . . . . . . 245
6.16.3 Macro replacement . . . . . . . . . . . . . . . . . . . . . . . 247
6.16.6 Error directive . . . . . . . . . . . . . . . . . . . . . . . . . . 248
6.16.7 Pragma directive . . . . . . . . . . . . . . . . . . . . . . . . . 249
6.17 Library introduction - partial . . . . . . . . . . . . . . . . . . . . . . . . 249
6.17.1 General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 249
6.17.2 The C standard library . . . . . . . . . . . . . . . . . . . . . . 251
6.17.3 Definitions . . . . . . . . . . . . . . . . . . . . . . . . . . . . 252
6.18 Language support library - partial . . . . . . . . . . . . . . . . . . . . . 253
6.18.0 General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 253
6.18.1 Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 254
5 of 371
— AUTOSAR CONFIDENTIAL —
Document ID 839: AUTOSAR_RS_CPP14Guidelines