验证码是网络应用中常用的一种安全机制,用于防止自动机器人或恶意软件进行非法操作。在C#编程语言中,我们可以利用GDI+库来创建复杂的验证码图像,包括添加噪点效果,增加识别难度。本篇文章将深入探讨如何使用C#来绘制包含噪点的验证码源码。 验证码的核心是生成随机字符串。在C#中,我们可以使用`System.Random`类生成随机数字,然后将其转换为字母或数字字符。通常,验证码长度为4到6位,包含字母和数字的组合,可以使用以下代码实现: ```csharp Random random = new Random(); string chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; int length = 5; StringBuilder captchaText = new StringBuilder(); for (int i = 0; i < length; i++) { captchaText.Append(chars[random.Next(chars.Length)]); } ``` 接下来,我们需要创建一个`Bitmap`对象作为验证码图像,并设置其大小和背景色。例如,我们可以创建一个200x50像素的图像,背景色为白色: ```csharp Bitmap bitmap = new Bitmap(200, 50); Graphics graphics = Graphics.FromImage(bitmap); graphics.Clear(Color.White); ``` 为了增加噪点效果,我们可以使用`Random`类生成随机点,并用黑色填充。这里我们使用`FillRectangle`方法在图像上画出这些点: ```csharp Random noiseRandom = new Random(); for (int i = 0; i < 200; i++) { int x = noiseRandom.Next(bitmap.Width); int y = noiseRandom.Next(bitmap.Height); SolidBrush brush = new SolidBrush(Color.Black); graphics.FillRectangle(brush, x, y, 1, 1); brush.Dispose(); } ``` 然后,我们将随机生成的字符串转化为图形。每个字符可以设置不同的字体、大小和旋转角度,增加识别难度: ```csharp FontFamily fontFamily = new FontFamily("Arial"); List<Font> fonts = new List<Font>(); for (int i = 0; i < length; i++) { float fontSize = 16 + random.Next(8); float angle = random.Next(-15, 15); fonts.Add(new Font(fontFamily, fontSize, FontStyle.Regular, GraphicsUnit.Pixel, 0, angle)); } foreach (char c in captchaText.ToString()) { SolidBrush textBrush = new SolidBrush(Color.Black); SizeF textSize = graphics.MeasureString(c.ToString(), fonts[i]); float x = (bitmap.Width - textSize.Width) / 2f + i * (textSize.Width + 5); float y = (bitmap.Height - textSize.Height) / 2f; graphics.DrawString(c.ToString(), fonts[i], textBrush, x, y); textBrush.Dispose(); } ``` 保存验证码图像到文件,例如JPG格式: ```csharp pictureBox1.Image = bitmap; pictureBox1.Invalidate(); MemoryStream stream = new MemoryStream(); bitmap.Save(stream, ImageFormat.Jpeg); byte[] imageBytes = stream.ToArray(); ``` 以上就是使用C#绘制验证码并添加噪点的基本步骤。当然,实际应用中可能还需要考虑其他安全措施,如添加扭曲、干扰线等,以进一步提高验证码的安全性。通过调整代码中的参数,可以实现不同风格和复杂度的验证码。这个过程展示了C#在图形处理方面的强大功能,以及如何通过GDI+库轻松地创建自定义的图形元素。
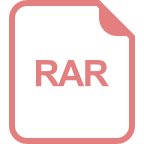
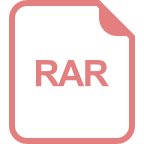
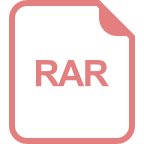
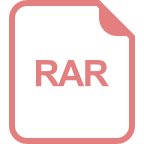
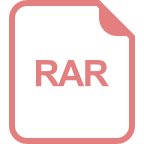
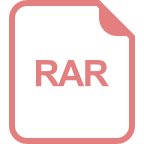
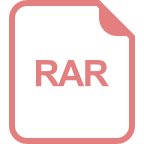
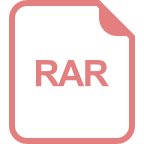
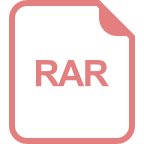
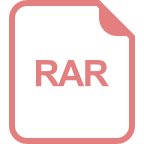
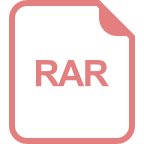
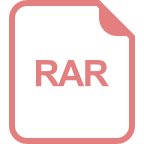
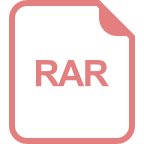
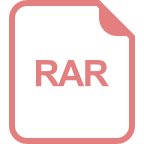
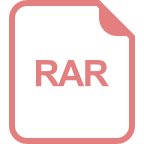
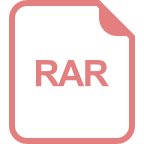

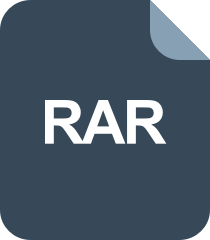
- 1
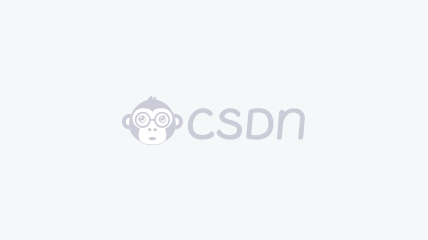

- 粉丝: 0
- 资源: 173
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

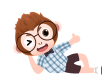
最新资源

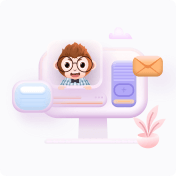
