package com.so206.po;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
public class SystemWebExample {
protected String orderByClause;
protected boolean distinct;
protected List<Criteria> oredCriteria;
public SystemWebExample() {
oredCriteria = new ArrayList<Criteria>();
}
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
public String getOrderByClause() {
return orderByClause;
}
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
public boolean isDistinct() {
return distinct;
}
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andIdIsNull() {
addCriterion("id is null");
return (Criteria) this;
}
public Criteria andIdIsNotNull() {
addCriterion("id is not null");
return (Criteria) this;
}
public Criteria andIdEqualTo(Integer value) {
addCriterion("id =", value, "id");
return (Criteria) this;
}
public Criteria andIdNotEqualTo(Integer value) {
addCriterion("id <>", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThan(Integer value) {
addCriterion("id >", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThanOrEqualTo(Integer value) {
addCriterion("id >=", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThan(Integer value) {
addCriterion("id <", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThanOrEqualTo(Integer value) {
addCriterion("id <=", value, "id");
return (Criteria) this;
}
public Criteria andIdIn(List<Integer> values) {
addCriterion("id in", values, "id");
return (Criteria) this;
}
public Criteria andIdNotIn(List<Integer> values) {
addCriterion("id not in", values, "id");
return (Criteria) this;
}
public Criteria andIdBetween(Integer value1, Integer value2) {
addCriterion("id between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andIdNotBetween(Integer value1, Integer value2) {
addCriterion("id not between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andPayStaff1IsNull() {
addCriterion("pay_staff1 is null");
return (Criteria) this;
}
public Criteria andPayStaff1IsNotNull() {
addCriterion("pay_staff1 is not null");
return (Criteria) this;
}
public Criteria andPayStaff1EqualTo(Double value) {
addCriterion("pay_staff1 =", value, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1NotEqualTo(Double value) {
addCriterion("pay_staff1 <>", value, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1GreaterThan(Double value) {
addCriterion("pay_staff1 >", value, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1GreaterThanOrEqualTo(Double value) {
addCriterion("pay_staff1 >=", value, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1LessThan(Double value) {
addCriterion("pay_staff1 <", value, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1LessThanOrEqualTo(Double value) {
addCriterion("pay_staff1 <=", value, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1In(List<Double> values) {
addCriterion("pay_staff1 in", values, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1NotIn(List<Double> values) {
addCriterion("pay_staff1 not in", values, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1Between(Double value1, Double value2) {
addCriterion("pay_staff1 between", value1, value2, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff1NotBetween(Double value1, Double value2) {
addCriterion("pay_staff1 not between", value1, value2, "payStaff1");
return (Criteria) this;
}
public Criteria andPayStaff2IsNull() {
addCriterion("pay_staff2 is null");
return (Criteria) this;
}
public Criteria andPayStaff2IsNotNull() {
addCriterion("pay_staff2 is not null");
return (Criteria) this;
}
public Criteria andPayStaff2EqualTo(Double value) {
addCriterion("pay_staff2 =", value, "payStaff2");
return (Criteria) this;
}
public Criteria andPayStaff2NotEqualTo(Double value) {
addCriterion("pay_staff2 <>", value, "payStaff2");
return (Criteria) this;
}
public Criteria andPayStaff2GreaterThan(Double value) {
addCriterion("pay_staff2 >", value, "payStaff2");
return (Criteria) this;
}
public Criteria andPayStaff2GreaterThanOrEqualTo(Double value) {
addCriterion("pay_staff2 >=", value, "payStaff2");
return (Criteria) this;
}
public Criteria andPayStaff2LessThan(Double value) {
addCriterion("pay_staff2 <", value, "payStaff2");
return (Criteria) this;
}
public Criteria andPayStaff2LessThanOrEqualT
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该开源易支付系统采用Java架构,完整源码包含872个文件,涵盖了206个Java源文件、136个JavaScript脚本、113个HTML文件、54个CSS样式表,以及各种图片和配置文件。系统以高效、易用性为核心,旨在为用户提供便捷的支付解决方案。
资源推荐
资源详情
资源评论
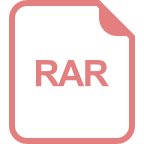
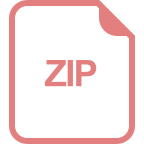
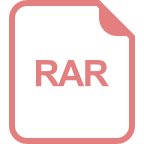
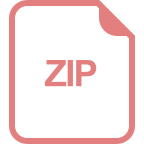
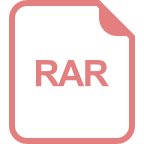
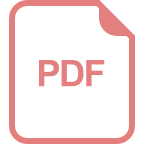
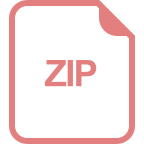
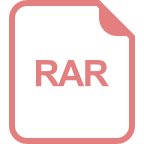
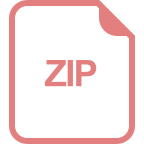
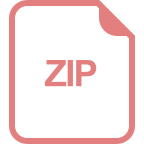
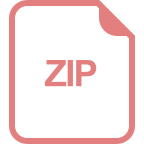
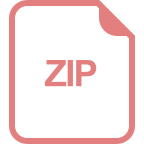
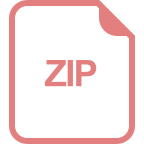
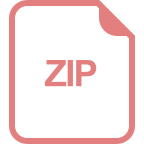
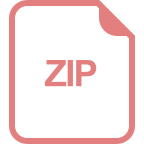
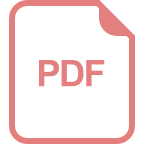
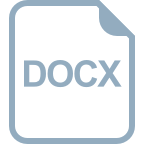
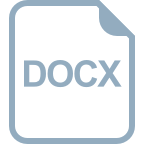
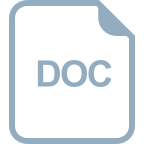
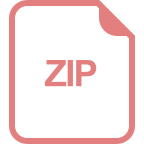
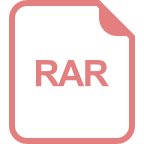
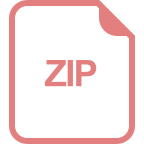
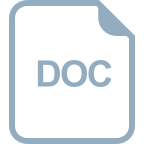
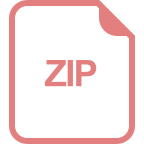
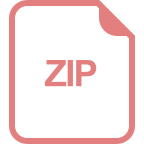
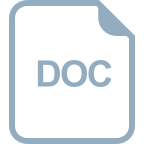
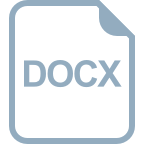
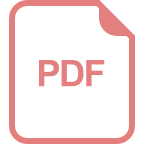
收起资源包目录

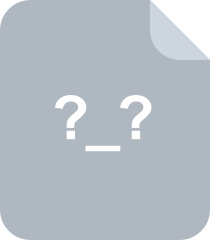
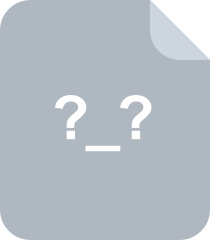
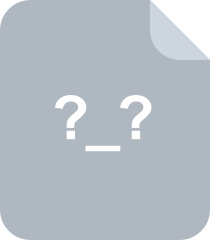
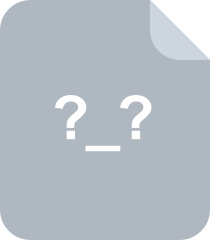
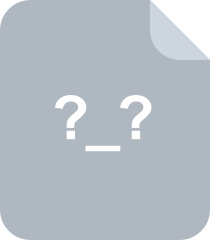
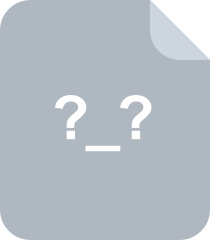
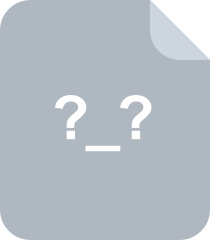
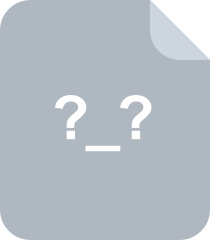
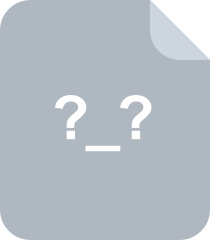
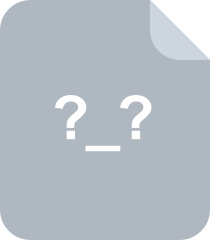
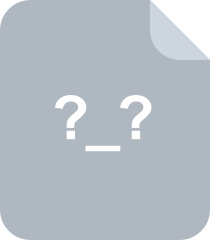
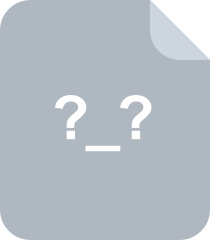
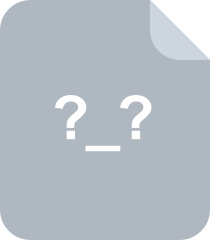
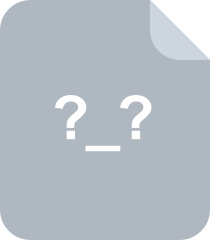
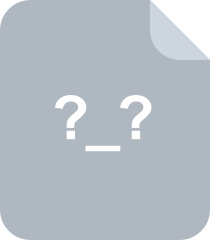
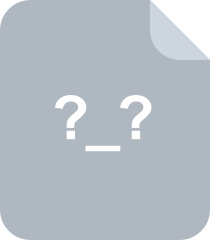
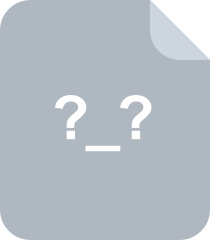
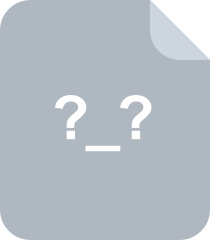
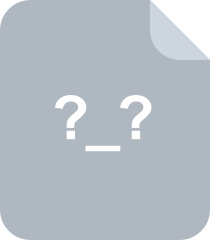
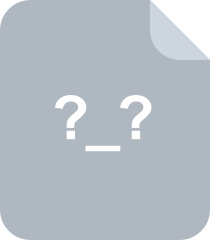
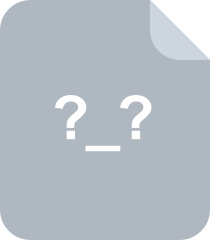
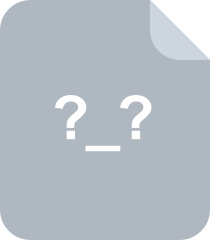
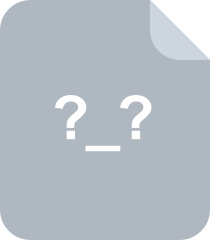
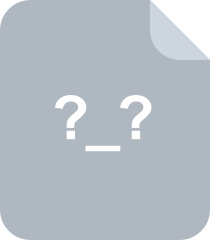
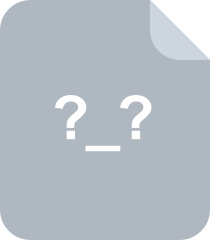
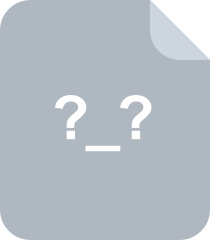
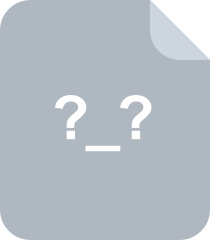
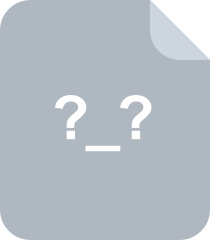
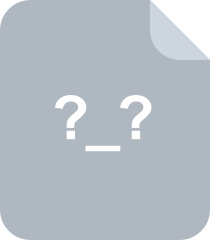
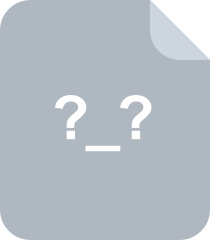
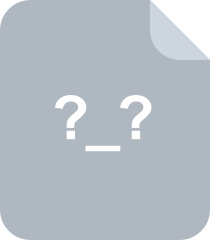
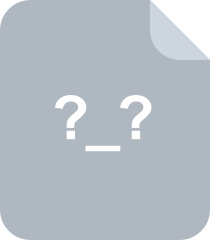
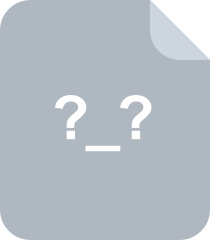
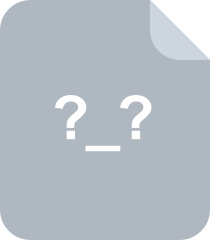
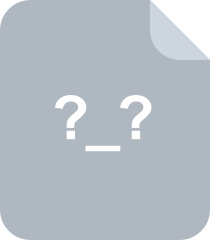
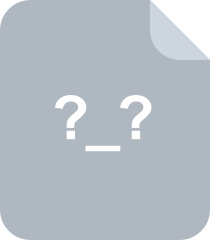
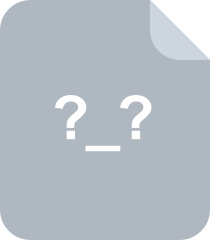
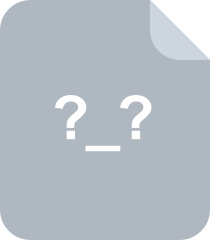
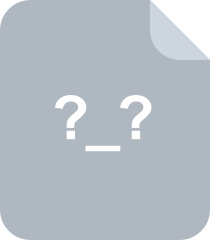
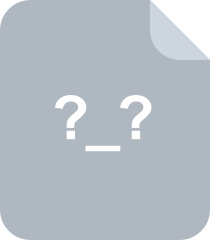
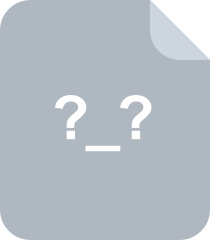
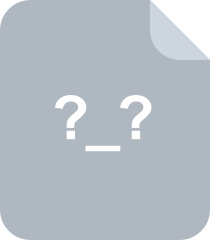
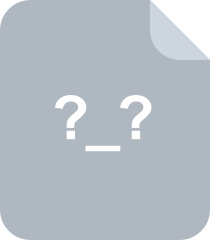
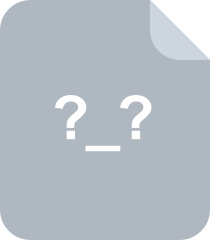
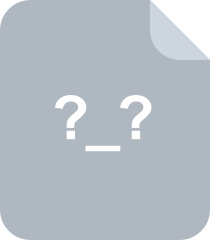
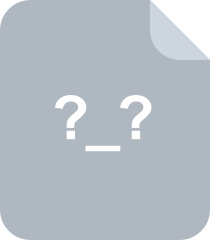
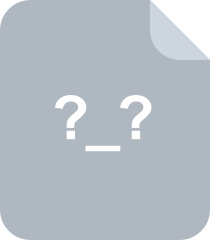
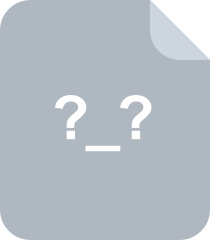
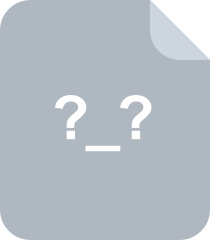
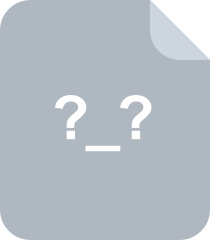
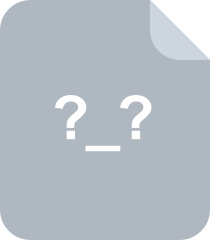
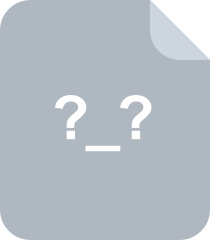
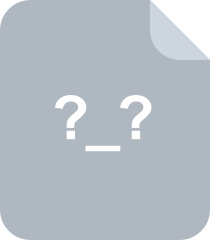
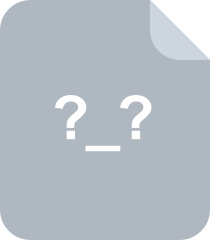
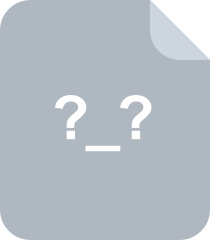
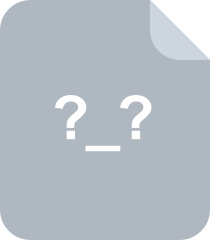
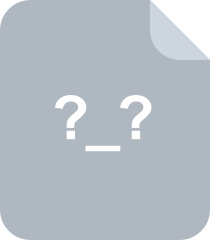
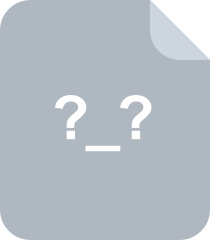
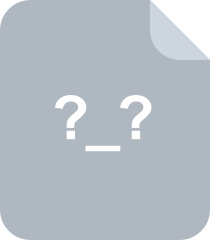
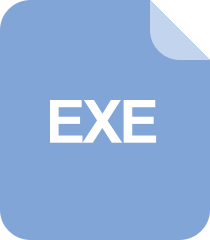
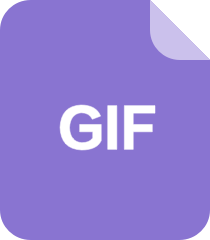
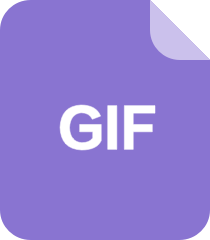
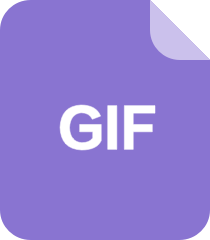
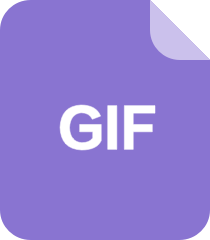
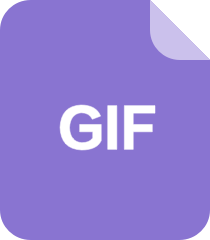
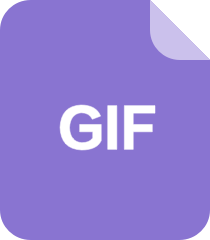
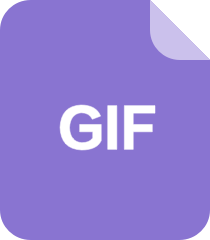
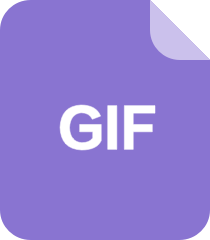
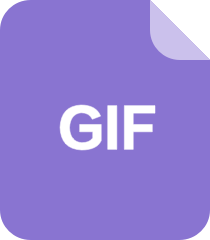
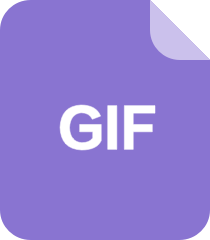
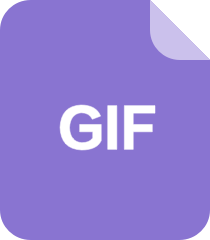
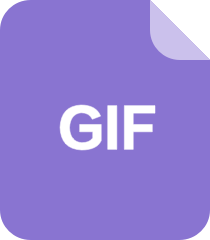
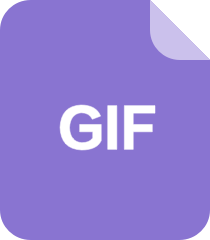
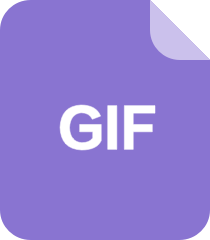
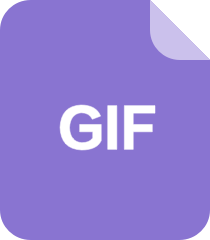
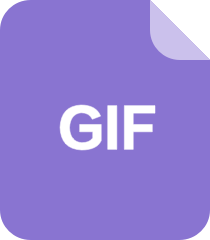
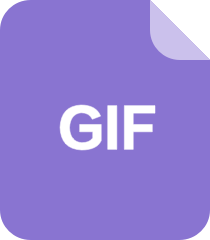
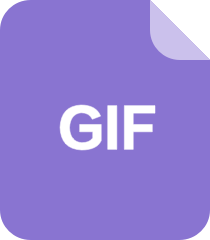
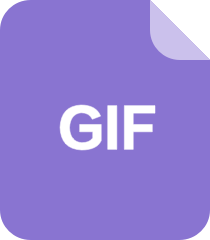
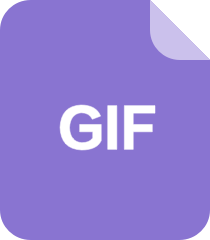
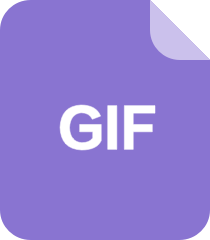
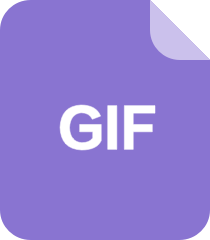
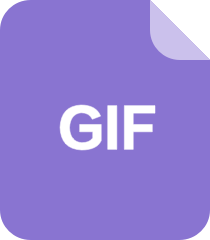
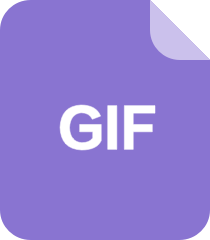
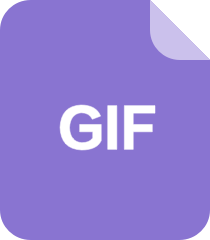
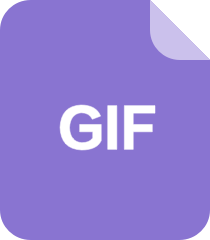
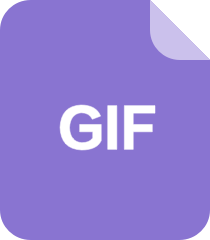
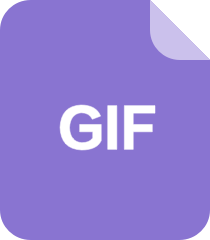
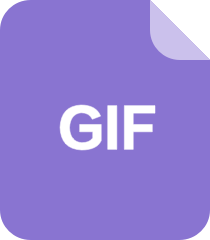
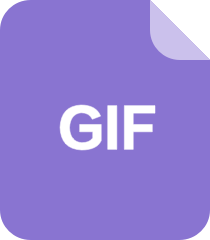
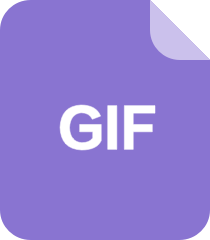
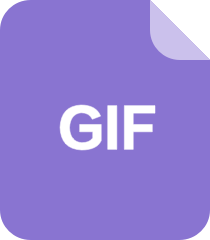
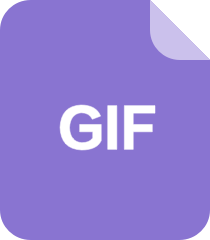
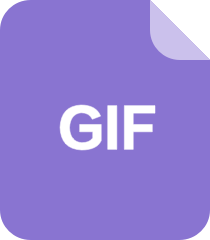
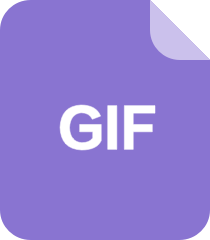
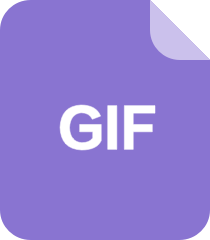
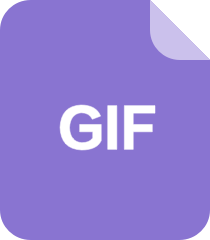
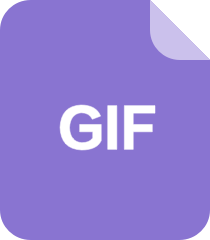
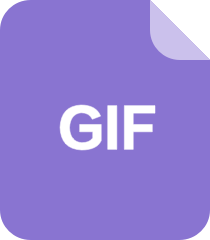
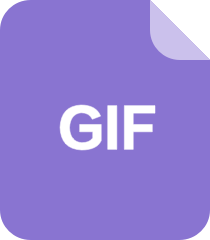
共 872 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
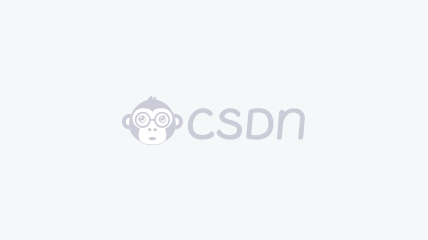

csbysj2020
- 粉丝: 2610
- 资源: 5500
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

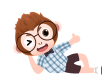
最新资源
- 一个由Java实现的游戏服务器端框架,可快速开发出易维护、高性能、高扩展能力的游戏服务器
- 生涯发展报告_编辑.pdf
- three.js开发的3D模型可视化编辑器 包含模型加载,模型文件导入导出,模型背景图,全景图,模型动画,模型灯光,模型定位,辅助线,模型辉光,模型拖拽,模型拆解, 模型材质等可视化操作编辑系统
- 全国330多个地级市一、二、三产业GDP和全国及各省土地流转和耕地面积数据-最新出炉.zip
- spring boot接口性能优化方案和spring cloud gateway网关限流实战
- 基于Netty实现的命令行斗地主游戏,新增癞子模式,德州扑克,增加超时机制,完美复现欢乐斗地主,欢迎体验在线版
- FIC7608-spec-brief-V1.1 - 20240419
- 惠普打印机(M233sdn)驱动下载
- 大飞哥本地离线AI智能抠图 1.0本地模型算法进行AI证件抠图支持单张和批量图片格式转换抠图软件
- 初学者Python入门指南:从安装到应用
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


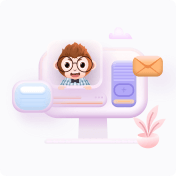
安全验证
文档复制为VIP权益,开通VIP直接复制
