/*
* Copyright (c) 1995, 2012, Oracle and/or its affiliates. All rights reserved.
* ORACLE PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.
*/
package java.awt;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.util.Vector;
import java.util.Locale;
import java.util.EventListener;
import java.util.Iterator;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.Collections;
import java.awt.peer.ComponentPeer;
import java.awt.peer.ContainerPeer;
import java.awt.peer.LightweightPeer;
import java.awt.image.BufferStrategy;
import java.awt.image.ImageObserver;
import java.awt.image.ImageProducer;
import java.awt.image.ColorModel;
import java.awt.image.VolatileImage;
import java.awt.event.*;
import java.awt.datatransfer.Transferable;
import java.awt.dnd.DnDConstants;
import java.awt.dnd.DragSource;
import java.awt.dnd.DragSourceContext;
import java.awt.dnd.DragSourceListener;
import java.awt.dnd.InvalidDnDOperationException;
import java.io.Serializable;
import java.io.ObjectOutputStream;
import java.io.ObjectInputStream;
import java.io.IOException;
import java.beans.PropertyChangeListener;
import java.beans.PropertyChangeSupport;
import java.awt.event.InputMethodListener;
import java.awt.event.InputMethodEvent;
import java.awt.im.InputContext;
import java.awt.im.InputMethodRequests;
import java.awt.dnd.DropTarget;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import javax.accessibility.*;
import java.awt.GraphicsConfiguration;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.security.AccessControlContext;
import javax.accessibility.*;
import java.lang.ref.*;
import java.util.logging.*;
import java.applet.Applet;
import sun.security.action.GetPropertyAction;
import sun.awt.AppContext;
import sun.awt.AWTAccessor;
import sun.awt.SunToolkit;
import sun.awt.ConstrainableGraphics;
import sun.awt.DebugHelper;
import sun.awt.SubRegionShowable;
import sun.awt.WindowClosingListener;
import sun.awt.WindowClosingSupport;
import sun.awt.GlobalCursorManager;
import sun.awt.CausedFocusEvent;
import sun.awt.EmbeddedFrame;
import sun.awt.dnd.SunDropTargetEvent;
import sun.awt.im.CompositionArea;
import sun.awt.image.VSyncedBSManager;
import sun.java2d.SunGraphics2D;
import sun.java2d.SunGraphicsEnvironment;
import sun.java2d.pipe.Region;
import sun.java2d.pipe.hw.ExtendedBufferCapabilities;
import static sun.java2d.pipe.hw.ExtendedBufferCapabilities.VSyncType.*;
import sun.awt.RequestFocusController;
/**
* A <em>component</em> is an object having a graphical representation
* that can be displayed on the screen and that can interact with the
* user. Examples of components are the buttons, checkboxes, and scrollbars
* of a typical graphical user interface. <p>
* The <code>Component</code> class is the abstract superclass of
* the nonmenu-related Abstract Window Toolkit components. Class
* <code>Component</code> can also be extended directly to create a
* lightweight component. A lightweight component is a component that is
* not associated with a native opaque window.
* <p>
* <h3>Serialization</h3>
* It is important to note that only AWT listeners which conform
* to the <code>Serializable</code> protocol will be saved when
* the object is stored. If an AWT object has listeners that
* aren't marked serializable, they will be dropped at
* <code>writeObject</code> time. Developers will need, as always,
* to consider the implications of making an object serializable.
* One situation to watch out for is this:
* <pre>
* import java.awt.*;
* import java.awt.event.*;
* import java.io.Serializable;
*
* class MyApp implements ActionListener, Serializable
* {
* BigObjectThatShouldNotBeSerializedWithAButton bigOne;
* Button aButton = new Button();
*
* MyApp()
* {
* // Oops, now aButton has a listener with a reference
* // to bigOne!
* aButton.addActionListener(this);
* }
*
* public void actionPerformed(ActionEvent e)
* {
* System.out.println("Hello There");
* }
* }
* </pre>
* In this example, serializing <code>aButton</code> by itself
* will cause <code>MyApp</code> and everything it refers to
* to be serialized as well. The problem is that the listener
* is serializable by coincidence, not by design. To separate
* the decisions about <code>MyApp</code> and the
* <code>ActionListener</code> being serializable one can use a
* nested class, as in the following example:
* <pre>
* import java.awt.*;
* import java.awt.event.*;
* import java.io.Serializable;
*
* class MyApp java.io.Serializable
* {
* BigObjectThatShouldNotBeSerializedWithAButton bigOne;
* Button aButton = new Button();
*
* static class MyActionListener implements ActionListener
* {
* public void actionPerformed(ActionEvent e)
* {
* System.out.println("Hello There");
* }
* }
*
* MyApp()
* {
* aButton.addActionListener(new MyActionListener());
* }
* }
* </pre>
* <p>
* <b>Note</b>: For more information on the paint mechanisms utilitized
* by AWT and Swing, including information on how to write the most
* efficient painting code, see
* <a href="http://java.sun.com/products/jfc/tsc/articles/painting/index.html">Painting in AWT and Swing</a>.
* <p>
* For details on the focus subsystem, see
* <a href="http://java.sun.com/docs/books/tutorial/uiswing/misc/focus.html">
* How to Use the Focus Subsystem</a>,
* a section in <em>The Java Tutorial</em>, and the
* <a href="../../java/awt/doc-files/FocusSpec.html">Focus Specification</a>
* for more information.
*
* @version %I%, %G%
* @author Arthur van Hoff
* @author Sami Shaio
*/
public abstract class Component implements ImageObserver, MenuContainer,
Serializable
{
private static final Logger focusLog = Logger.getLogger("java.awt.focus.Component");
private static final Logger log = Logger.getLogger("java.awt.Component");
private static final Logger mixingLog = Logger.getLogger("java.awt.mixing.Component");
/**
* The peer of the component. The peer implements the component's
* behavior. The peer is set when the <code>Component</code> is
* added to a container that also is a peer.
* @see #addNotify
* @see #removeNotify
*/
transient ComponentPeer peer;
/**
* The parent of the object. It may be <code>null</code>
* for top-level components.
* @see #getParent
*/
transient Container parent;
/**
* The <code>AppContext</code> of the component. Applets/Plugin may
* change the AppContext.
*/
transient AppContext appContext;
/**
* The x position of the component in the parent's coordinate system.
*
* @serial
* @see #getLocation
*/
int x;
/**
* The y position of the component in the parent's coordinate system.
*
* @serial
* @see #getLocation
*/
int y;
/**
* The width of the component.
*
* @serial
* @see #getSize
*/
int width;
/**
* The height of the component.
*
* @serial
* @see #getSize
*/
int height;
/**
* The foreground color for this component.
* <code>foreground</code> can be <code>null</code>.
*
* @serial
* @see #getForeground
* @see #setForeground
*/
Color foreground;
/**
* The background color for this component.
* <code>background</code> can be <code>null</code>.
*
* @serial
* @see #getBackground
* @see #setBackground
*/
Color background;
/**
* The font used by this component.

螃蟹变异了
- 粉丝: 12
- 资源: 4
最新资源
- 基于PyTorch的MOPSO算法:引导种群逼近Pareto前沿的粒子群优化方法程序研究与应用,基于PyTorch的多目标粒子群算法:MOPSO实现及逼近真实Pareto前沿的种群优化策略,基于pyt
- 车机(飞思卡尔芯片) 系统签名(app公签)
- 如何正确使用deepseek?99%的人都错了.zip
- 基于双边LCC移相控制的无线电能传输系统与PI及MPC模型预测控制实现输出电压恒定,双边LCC移相控制与无线电能传输技术的融合:实现恒定电压PI控制与MPC模型预测控制,双边LCC移相控制,pi控制输
- 零基础使用DeepSeek高效提问技巧.zip
- Multisim仿真工具在模拟电路设计中的首次应用:运算放大器电路构建与测试
- 车机公签,方易通9853 apk签名
- 1000个DeepSeek神级提示词,让你轻松驾驭AI赶紧收藏.zip
- MATLAB代码在线实现:基于最小二乘法的锂电池一阶RC模型参数快速辨识法,基于最小二乘法的锂电池一阶RC模型参数在线辨识MATLAB代码实现,采用最小二乘法在线辨识锂电池一阶RC模型参数的MATLA
- 3个DeepSeek隐藏玩法,99%的人都不知道!.zip
- 横向定标与逆合成孔径雷达ISAR成像的MATLAB仿真程序:精确两步交叉范围缩放法与散射点提取技术研究,**横纵探索:逆合成孔径雷达(ISAR)成像技术与信号处理的精准算法复现**,横向定标 地基逆合
- android安卓原生系统签名,app公签,车机公签
- SPSS workshop (data of construction)
- 全桥与半桥LLC谐振DC-DC变换器的设计与Simulink仿真,包括开环与电压闭环仿真及电路参数计算过程,全桥与半桥LLC谐振DC-DC变换器的设计与Simulink仿真,含开环与电压闭环仿真及电路
- 高速信号链设计中噪声源的影响及优化策略:噪声带宽与信噪比提高方法
- 基于FPGA的永磁同步伺服系统矢量控制设计:集成电流环、速度环与SVPWM模块,采用Verilog实现坐标变换与电机反馈接口,基于FPGA实现永磁同步伺服控制系统的矢量控制与电流环设计:Verilog
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


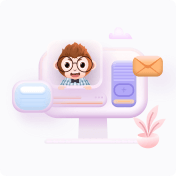
- 1
- 2
前往页