在C#编程中,创建具有任意形状的按钮是一项高级且实用的技能,它不仅能够提升用户界面的美观度,还能增强应用程序的互动性和用户体验。本文将深入探讨如何利用C#中的GraphicsPath、Region以及Bitmap对象来实现这一功能,具体通过分析给定的代码片段进行详细解释。 ### 一、理解GraphicsPath与Region `GraphicsPath`类用于定义一个由直线和曲线组成的路径,它可以是闭合的或开放的,用于描绘任意形状。在图形绘制中,`GraphicsPath`常被用于复杂的形状设计,如圆角矩形、椭圆、多边形等。而`Region`类则代表了屏幕上的一个区域,通常用于剪裁或限制绘图操作的范围。当我们将`Region`与`GraphicsPath`结合使用时,可以为控件(如按钮)设定一个自定义形状,使其不局限于矩形边界。 ### 二、代码解析 #### 1. 初始化按钮形状 ```csharp public Form1() { InitializeComponent(); this.button1.Cursor = Cursors.Hand; Bitmap bmpBob = (Bitmap)this.button1.Image; GraphicsPath graphicsPath = CalculateControlGraphicsPath(bmpBob); this.button1.Region = new Region(graphicsPath); this.button2.Cursor = Cursors.Cross; GraphicsPath myPath = new GraphicsPath(); myPath.AddEllipse(5, 5, 97, 97); this.button2.Region = new Region(myPath); } ``` 这段代码展示了如何为两个按钮设置不同的形状。为`button1`设置了手型光标,并通过调用`CalculateControlGraphicsPath`方法,根据按钮图像(`bmpBob`)计算出一个`GraphicsPath`对象,然后使用该路径创建一个`Region`并应用到按钮上,使按钮的形状与图像轮廓一致。对于`button2`,则直接使用`AddEllipse`方法创建一个椭圆形的`GraphicsPath`,并同样将其转化为`Region`应用到按钮上,形成一个椭圆形按钮。 #### 2. 自定义形状计算 ```csharp private static GraphicsPath CalculateControlGraphicsPath(Bitmap bitmap) { GraphicsPath graphicsPath = new GraphicsPath(); Color colorTransparent = bitmap.GetPixel(0, 0); // ...代码省略... } ``` 此方法负责根据传入的`Bitmap`对象计算出其透明边缘的`GraphicsPath`。它通过遍历图像的每个像素,检查像素是否为透明色(此处假设透明色为图像左上角的第一个像素颜色),并使用非透明像素的坐标来构建矩形,最终这些矩形的集合形成了代表图像轮廓的`GraphicsPath`。 ### 三、扩展与优化 尽管上述代码实现了基本功能,但在实际开发中可能需要考虑更多因素,如性能优化、异常处理以及更复杂的形状定义。例如: - **性能优化**:在处理大尺寸图像时,逐像素的遍历可能会导致性能瓶颈,可以尝试采用图像缩放或双缓冲技术减少不必要的重绘。 - **异常处理**:应增加对空引用、无效图像尺寸等异常情况的处理,确保程序的健壮性。 - **复杂形状定义**:除了椭圆,可以通过组合多个`GraphicsPath`方法(如`AddArc`, `AddLine`, `AddCurve`等)创建更加复杂的形状。 通过`GraphicsPath`和`Region`,C#提供了强大的工具来定制控件的形状,从而实现更富创意和交互性的用户界面设计。开发者可以根据具体需求,灵活运用这些技术,为用户提供更加个性化和吸引人的视觉体验。
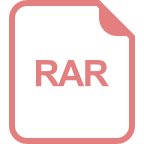
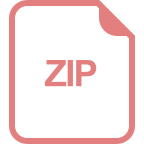
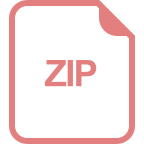
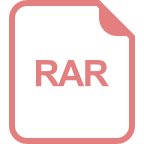
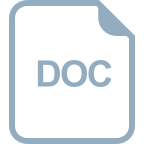
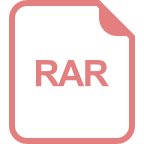
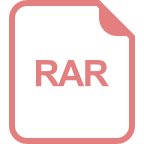
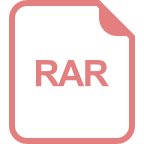
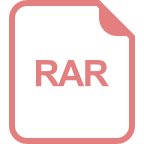
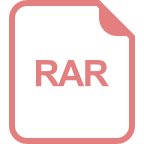
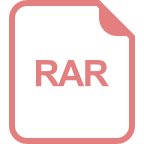
{
InitializeComponent();
this.button1.Cursor = Cursors.Hand;
Bitmap bmpBob = (Bitmap)this.button1.Image;
GraphicsPath graphicsPath = CalculateControlGraphicsPath(bmpBob);
this.button1.Region = new Region(graphicsPath);
this.button2.Cursor = Cursors.Cross;
GraphicsPath myPath = new GraphicsPath();
myPath.AddEllipse(5, 5, 97, 97);
//myPath.AddPie(0, 0, 150, 150, 190, 190);
//myPath.AddPolygon(new Point[] { new Point( 0, 0), new Point ( 50, 50 ), new Point (70, 70 ), new Point ( 80, 80 ), new Point ( 90, 90 ) });
this.button2.Region = new Region(myPath);
}
private static GraphicsPath CalculateControlGraphicsPath(Bitmap bitmap)
{
GraphicsPath graphicsPath = new GraphicsPath();
Color colorTransparent = bitmap.GetPixel(0, 0);
int colOpaquePixel = 0;
for (int row = 0; row < bitmap.Height; row++)
{
colOpaquePixel = 0;
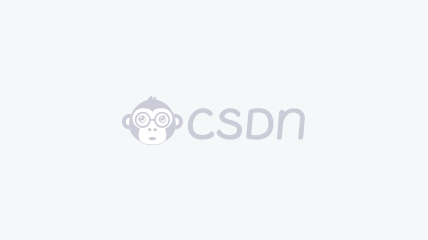
- u0109713682014-04-03。。。代码可以借鉴。。
- sanqianyuejia2015-11-20刚好可以用到我们的应用上,极好了……

- 粉丝: 3
- 资源: 5
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

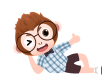
最新资源
- 冒泡排序算法详解及Java与Python实现
- 字幕网页文字检测20-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- FastAdmin后台框架开源且可以免费商用,一键生成CRUD, 一款基于ThinkPHP和Bootstrap的极速后台开发框架,基于Auth验证的权限管理系统,一键生成 CRUD,自动生成控制器等
- IMG_4525.jpg
- 基于 Spring Cloud 的一个分布式系统套件的整合 具备 JeeSite4 单机版的所有功能,统一身份认证,统一基础数据管理,弱化微服务开发难度
- GigaDevice.GD32F4xx-DFP.2.1.0 器件安装包
- 智慧校园数字孪生,三维可视化
- 多种土地使用类型图像分类数据集【已标注,约30,000张数据】
- 3.0(1).docx
- 国产文本编辑器:EverEdit用户手册 1.1.0

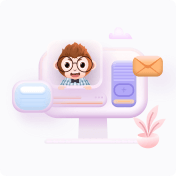
