#include "D3DClass.h"
D3DClass::D3DClass(void)
{
m_swapChain = 0;
m_device = 0;
m_deviceContext = 0;
m_renderTargetView = 0;
m_depthStencilBuffer = 0;
m_depthStencilState = 0;
m_depthStencilView = 0;
m_rasterState = 0;
}
D3DClass::D3DClass(const D3DClass& other)
{
}
D3DClass::~D3DClass(void)
{
}
//Initialize函数包含完成D3D设置的所有代码。
bool D3DClass::Initialize(int screenWidth, int screenHeight, bool vsync, HWND hwnd, bool fullscreen,
float screenDepth, float screenNear)
{
HRESULT result;
IDXGIFactory* factory;
IDXGIAdapter* adapter;
IDXGIOutput* adapterOutput;
unsigned int numModes, i, numerator=0, denominator=1, stringLength;
DXGI_MODE_DESC* displayModeList;
DXGI_ADAPTER_DESC adapterDesc;
int error;
DXGI_SWAP_CHAIN_DESC swapChainDesc;
D3D_FEATURE_LEVEL featureLevel;
ID3D11Texture2D* backBufferPtr;
D3D11_TEXTURE2D_DESC depthBufferDesc;
D3D11_DEPTH_STENCIL_DESC depthStencilDesc;
D3D11_DEPTH_STENCIL_VIEW_DESC depthStencilViewDesc;
D3D11_RASTERIZER_DESC rasterDesc;
D3D11_VIEWPORT viewport;
float fieldOfView, screenAspect;
// 保存垂直同步设置
m_vsync_enabled = vsync;
// 创建一个DirectX graphics interface factory.
result = CreateDXGIFactory(__uuidof(IDXGIFactory), (void**)&factory);
if(FAILED(result))
{
HR(result);
return false;
}
// 用接口工厂创建一个主显卡的适配
result = factory->EnumAdapters(0, &adapter);
if(FAILED(result))
{
HR(result);
return false;
}
// 得到主适配器的输出.
result = adapter->EnumOutputs(0, &adapterOutput);
if(FAILED(result))
{
HR(result);
return false;
}
//得到适合 DXGI_FORMAT_R8G8B8A8_UNORM 的显示模式.
result = adapterOutput->GetDisplayModeList(DXGI_FORMAT_R8G8B8A8_UNORM, DXGI_ENUM_MODES_INTERLACED, &numModes, NULL);
if(FAILED(result))
{
HR(result);
return false;
}
displayModeList = new DXGI_MODE_DESC[numModes];
if(!displayModeList)
{
HR(result);
return false;
}
// 保存显示模式到displayModeList中
result = adapterOutput->GetDisplayModeList(DXGI_FORMAT_R8G8B8A8_UNORM, DXGI_ENUM_MODES_INTERLACED, &numModes, displayModeList);
if(FAILED(result))
{
HR(result);
return false;
}
//遍历所有显示模式,得到刷新率两个参数值numerator 和 denominato
for(i=0; i<numModes; i++)
{
if(displayModeList[i].Width == (unsigned int)screenWidth)
{
if(displayModeList[i].Height == (unsigned int)screenHeight)
{
numerator = displayModeList[i].RefreshRate.Numerator;
denominator = displayModeList[i].RefreshRate.Denominator;
}
}
}
// 得到显卡描述
result = adapter->GetDesc(&adapterDesc);
if(FAILED(result))
{
HR(result);
return false;
}
// 保存显存大小.
m_videoCardMemory = (int)(adapterDesc.DedicatedVideoMemory / 1024 / 1024);
//保存显卡描述串
//wcstombs_s, wide char转化为char
error = wcstombs_s(&stringLength, m_videoCardDescription, 128, adapterDesc.Description, 128);
if(error != 0)
{
HR(result);
return false;
}
//// 释放显示模式列表
//delete [] displayModeList;
//displayModeList = 0;
////释放适配器输出.
//adapterOutput->Release();
//adapterOutput = 0;
////释放适配器
//adapter->Release();
//adapter = 0;
//// 释放接口工厂.
//factory->Release();
//factory = 0;
//如果屏幕高度或者宽度为0,则会创建深度缓冲失败,
//当窗口最小化时候,会出现窗口为0的情况。
if(screenWidth < 1)
screenWidth = 1;
if(screenHeight <1)
screenHeight = 1;
// 初始化交换链描述
ZeroMemory(&swapChainDesc, sizeof(swapChainDesc));
// 用1个后缓冲
swapChainDesc.BufferCount = 1;
//帧缓冲的大小和应用程序窗口大小相等.
swapChainDesc.BufferDesc.Width = screenWidth;
swapChainDesc.BufferDesc.Height = screenHeight;
// 后缓冲的surface的格式为DXGI_FORMAT_R8G8B8A8_UNORM.
// surface的每个像素用4个无符号的8bit[映射到0-1]来表示。NORM表示归一化。
swapChainDesc.BufferDesc.Format = DXGI_FORMAT_R8G8B8A8_UNORM;
// 如果使用垂直同步,设置后缓冲的刷新率。.
//刷新率就是一秒钟把后缓冲内容在屏幕上画出的次数。
//如果开启垂直同步,则锁定刷新率,则fps是固定的
if(m_vsync_enabled)
{
swapChainDesc.BufferDesc.RefreshRate.Numerator = numerator;
swapChainDesc.BufferDesc.RefreshRate.Denominator = denominator;
}
else
{
swapChainDesc.BufferDesc.RefreshRate.Numerator = 0;
swapChainDesc.BufferDesc.RefreshRate.Denominator = 1;
}
// 设置后缓冲的用途
// 我们的渲染目标缓冲为后缓冲。
swapChainDesc.BufferUsage = DXGI_USAGE_RENDER_TARGET_OUTPUT;
// 后缓冲输出的窗口句柄.
swapChainDesc.OutputWindow = hwnd;
// 不使用多重采样
swapChainDesc.SampleDesc.Count = 1;
swapChainDesc.SampleDesc.Quality = 0;
// 设置全屏或者窗口模式.
if(fullscreen)
{
swapChainDesc.Windowed = false;
}
else
{
swapChainDesc.Windowed = true;
}
// 设定扫描线ordering以及缩放为unspecified.
swapChainDesc.BufferDesc.ScanlineOrdering = DXGI_MODE_SCANLINE_ORDER_UNSPECIFIED;
swapChainDesc.BufferDesc.Scaling = DXGI_MODE_SCALING_UNSPECIFIED;
// 后缓冲内容呈现到屏幕后,放弃其内容
swapChainDesc.SwapEffect = DXGI_SWAP_EFFECT_DISCARD;
//不设置标志
swapChainDesc.Flags = DXGI_SWAP_CHAIN_FLAG_ALLOW_MODE_SWITCH ;
// 设置feature level为D3D11
// 如果显卡不支持D3D11,我们能够通过设置这个参数,使用D3D10,或者9.
featureLevel = D3D_FEATURE_LEVEL_11_0;
//在debug模式下,使得创建的设备支持debug.
UINT createDeviceFlags = 0;
#if defined(DEBUG) || defined(_DEBUG)
createDeviceFlags |= D3D11_CREATE_DEVICE_DEBUG;
#endif
// 创建交换链,设备以及设备上下文.
//result = D3D11CreateDeviceAndSwapChain(NULL, D3D_DRIVER_TYPE_HARDWARE, NULL,createDeviceFlags, &featureLevel, 1,
// D3D11_SDK_VERSION, &swapChainDesc, &m_swapChain, &m_device, NULL, &m_deviceContext);
//if(FAILED(result))
// {
// HR(result);
// return false;
// }
////禁止DXGI监视消息队列,捕捉ALT+ENTER,在全屏和窗口模式之间切换
//factory->MakeWindowAssociation(hwnd,DXGI_MWA_NO_WINDOW_CHANGES|DXGI_MWA_NO_ALT_ENTER );
//分开创建device和交换链,可以屏蔽dxgi自动的hook alt+enter
//注意的两点:如果用第一个参数用NULL,第二个参数为D3D_DRIVER_TYPE_HARDWARE,则后面创建swapchain
//会fail,如果直接用adapter,不改变第二个参数,则会提示创建设备失败,adapter无效。
result = D3D11CreateDevice(adapter,D3D_DRIVER_TYPE_UNKNOWN, NULL,createDeviceFlags, &featureLevel, 1,
D3D11_SDK_VERSION, &m_device, NULL, &m_deviceContext );
if(FAILED(result))
{
HR(result);
return false;
}
//创建交换链
result = factory->CreateSwapChain(m_device,&swapChainDesc, &m_swapChain);
if(FAILED(result))
{
HR(result);
return false;
}
//禁止DXGI监视消息队列,捕捉ALT+ENTER,在全屏和窗口模式之间切换
factory->MakeWindowAssociation(hwnd,DXGI_MWA_NO_WINDOW_CHANGES|DXGI_MWA_NO_ALT_ENTER );
// 释放显示模式列表
delete [] displayModeList;
displayModeList = 0;
//释放适配器输出.
adapterOutput->Release();
adapterOutput = 0;
//释放适配器
adapter->Release();
adapter = 0;
// 释放接口工厂.
factory->Release();
factory = 0;
// 得到交换链中的后缓冲指针.
result = m_swapChain->GetBuffer(0, __uuidof(ID3D11Texture2D), (LPVOID*)&backBufferPtr);
if(FAILED(result))
{
HR(result);
return false;
}
// 用后缓冲创建渲染目标视图.
result = m_device->CreateRenderTargetView(backBufferPtr, NULL, &m_renderTargetView);
if(FAILED(result))
{
HR(result);
return false;
}
//释放后缓冲.(引用计数减1)
backBufferPtr->Release();
backBufferPtr = 0;
// 初始化深度缓冲描述.
ZeroMemory(&depthBufferDesc, sizeof(depthBufferDesc));
//设置深度缓冲描述
depthBufferDesc.Width = screenWidth;
depthBufferDesc.Height = screenHeight;
depthBufferDesc.MipLevels = 1;//对于深度缓冲为1
depthBufferDesc.ArraySize = 1;//对于深度缓冲为1,对于纹理,这2个参数有更多用途
depthBufferDesc.Format = DXGI_FORMAT_D24_UNORM_S8_UINT;
depthBufferDesc.SampleDesc.Count = 1;
depthBufferDesc.SampleDesc.Quality = 0;
depth
没有合适的资源?快使用搜索试试~ 我知道了~
Directx11 非常全的教程 chm+源码
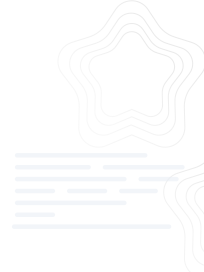
共814个文件
cpp:226个
h:217个
tlog:75个


温馨提示
首先感谢http://www.cnblogs.com/mikewolf2002/category/351011.html 这个博主,其实本教程就是他的博客, 我觉得写的很好,很有用,怕哪一天他博客像曾经很火的一个外国教程一样突然就消失了, 我把他的directx的文章一个一个下载下来,做成了chm文件,中间标题出现好多乱码,我也是一个一个改过来。现在终于能离线看了。由于找不到博主的联系方式,没有征求同意就 离线化了他的笔记,要是博主不愿意我这样做请联系我删除 email cq361106306@qq.com
资源推荐
资源详情
资源评论
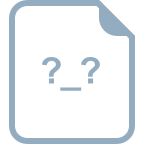
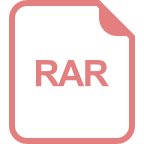
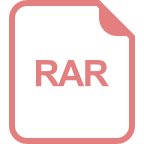
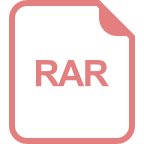
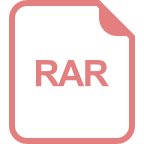
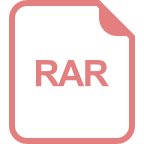
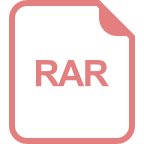
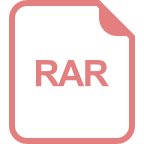
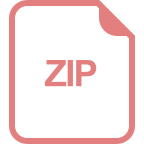
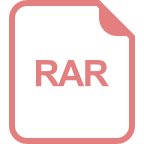
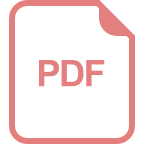
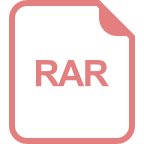
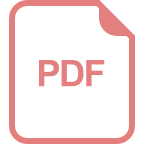
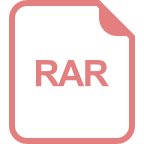
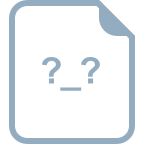
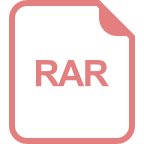
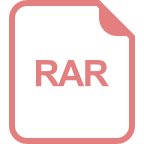
收起资源包目录

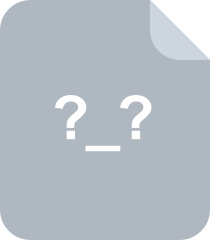
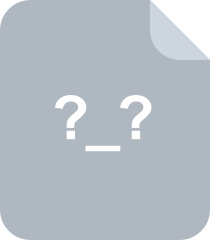
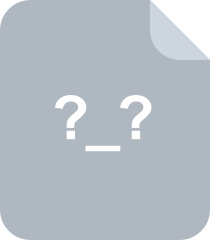
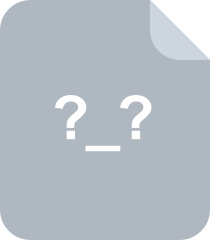
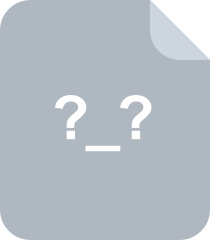
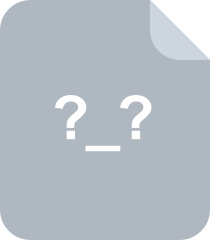
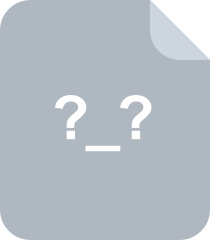
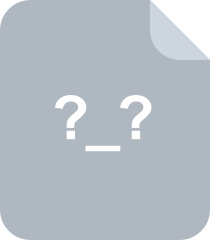
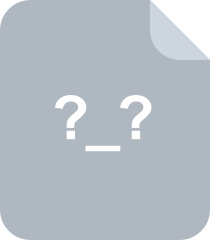
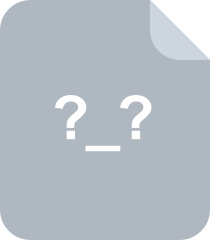
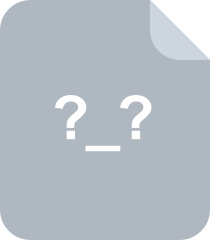
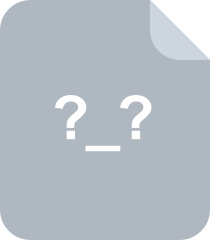
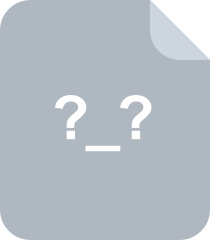
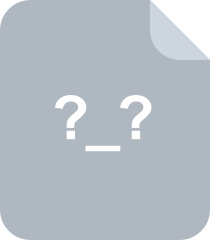
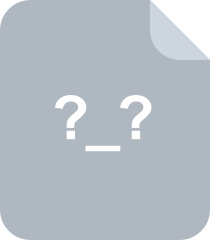
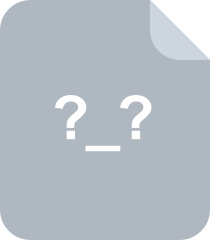
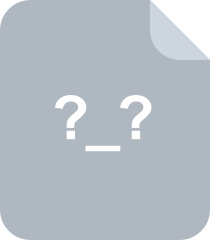
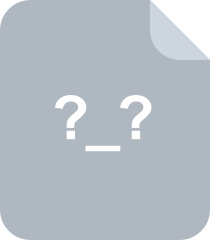
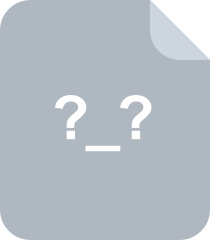
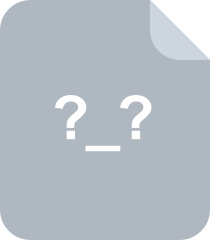
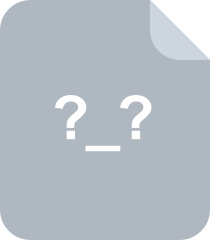
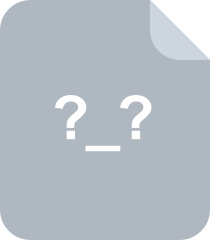
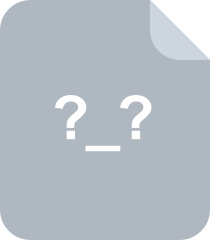
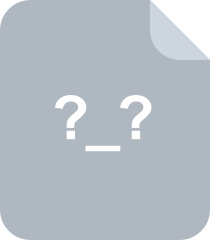
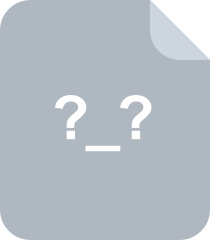
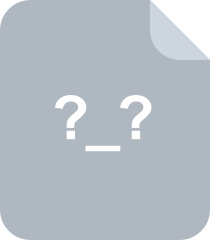
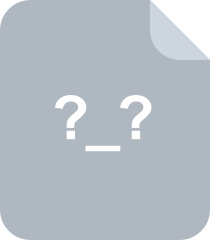
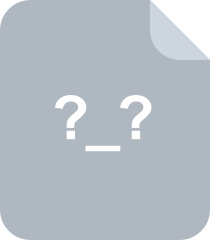
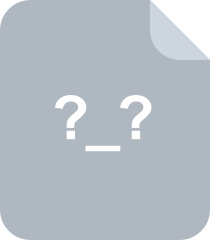
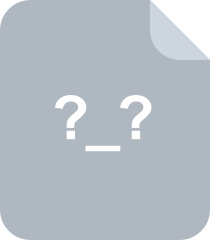
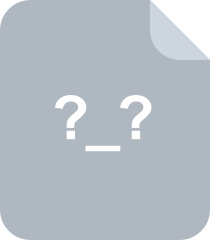
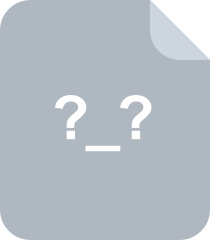
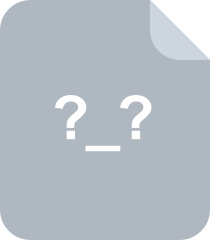
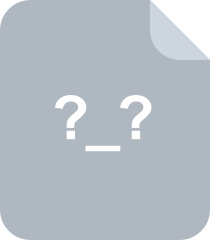
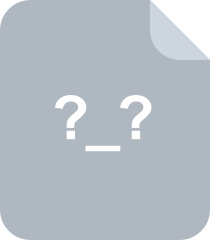
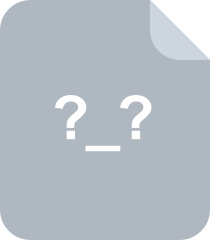
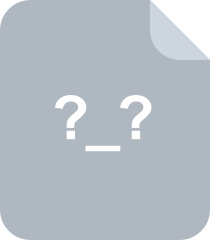
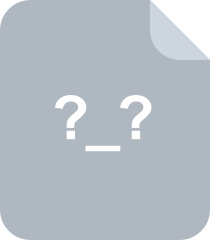
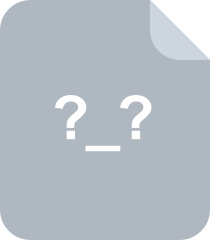
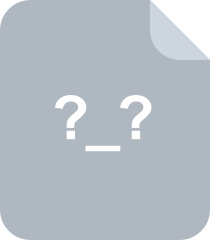
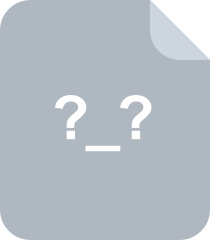
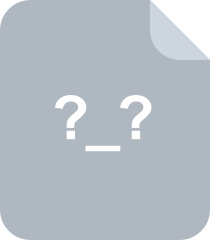
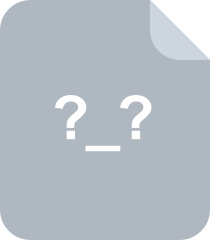
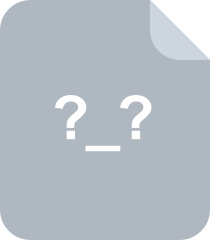
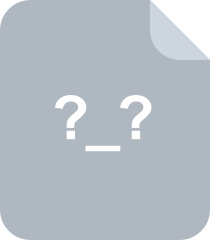
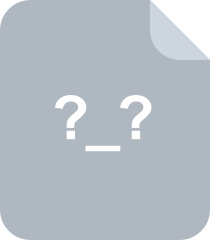
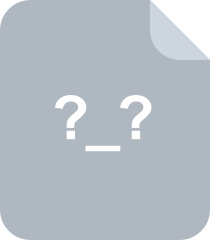
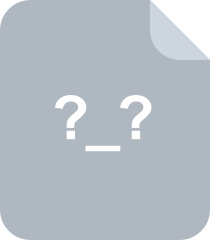
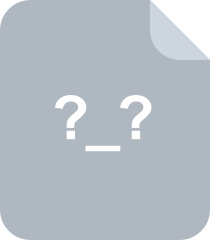
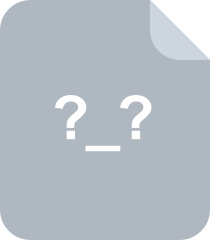
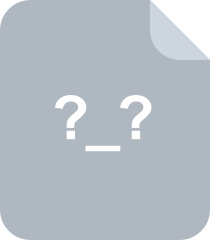
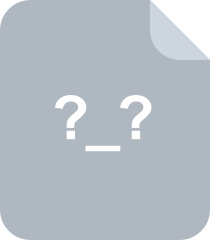
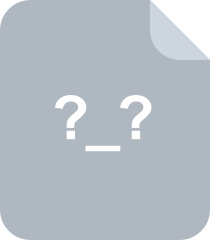
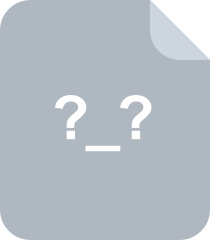
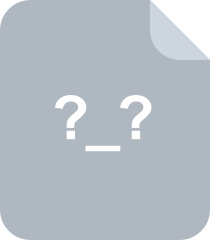
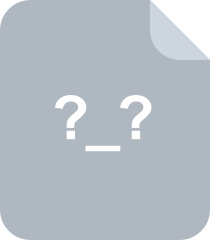
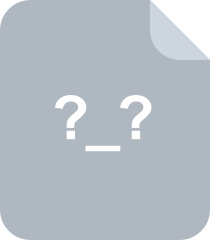
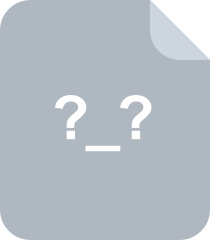
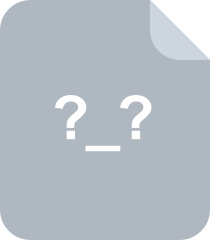
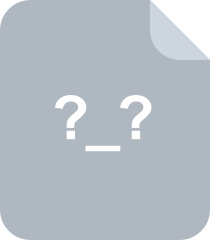
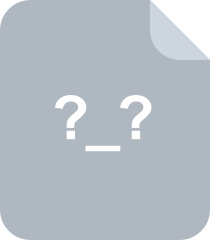
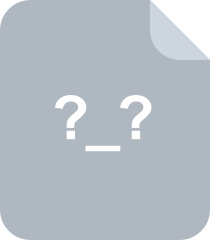
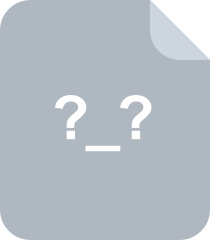
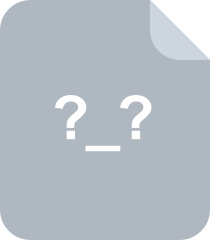
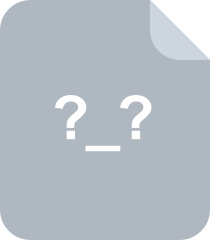
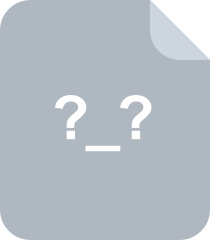
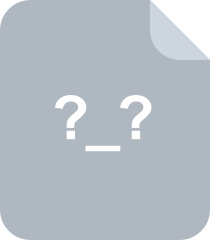
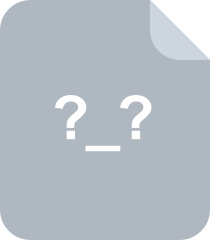
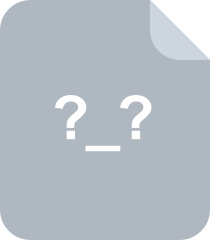
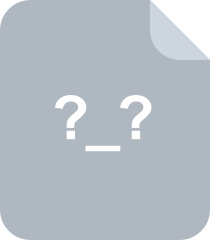
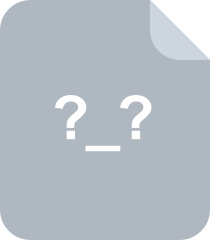
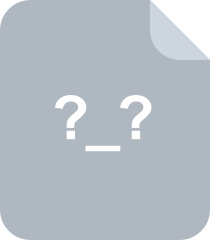
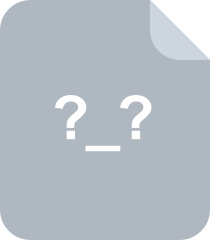
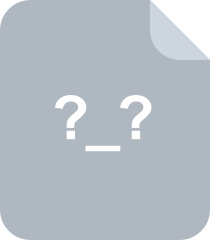
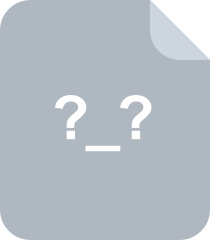
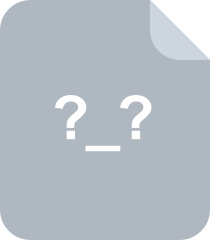
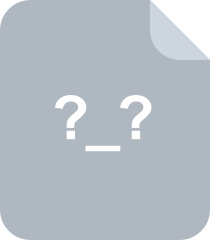
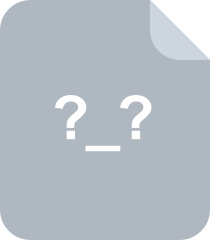
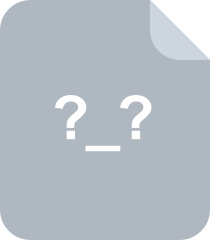
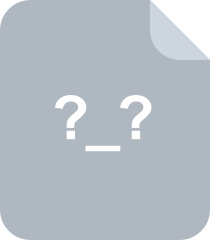
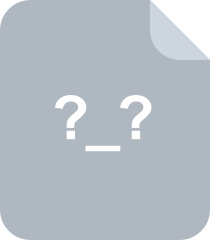
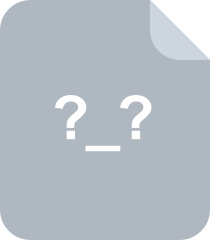
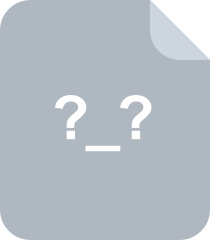
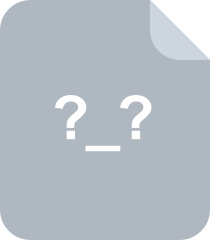
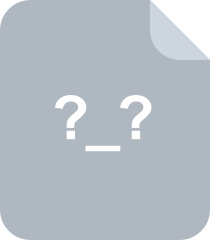
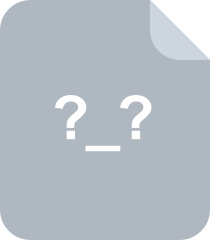
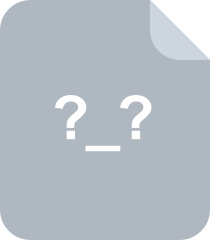
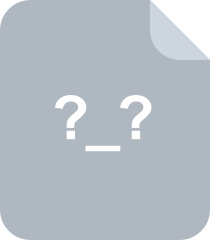
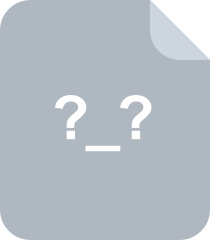
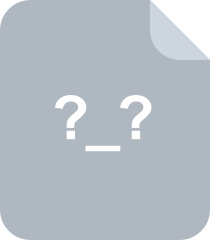
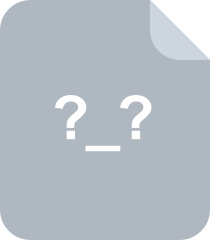
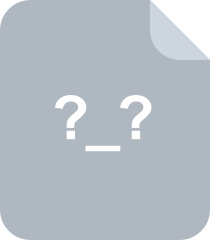
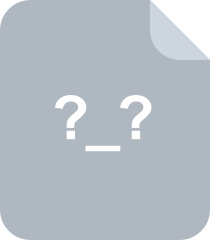
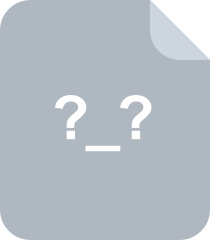
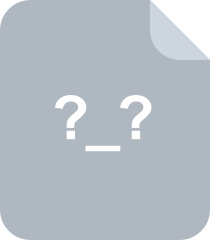
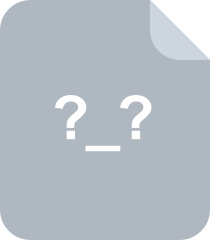
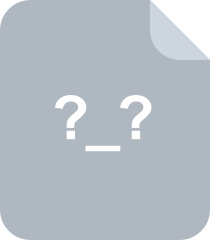
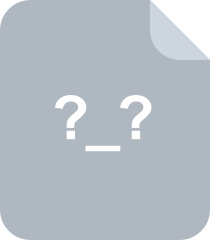
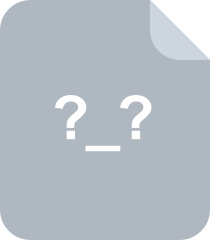
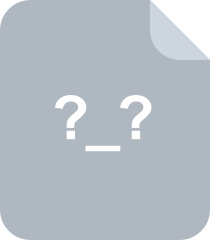
共 814 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9

Younfor
- 粉丝: 332
- 资源: 17
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

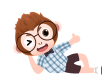
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页