/*
*******************************************************************************
** Include files
*******************************************************************************
*/
#include <iom16.h>
#include <comp_a90.h>
#include <avr_macros.h>
#include <intrinsics.h>
#include "onewire.h"
//-----------------------------------------------------------------------------
//Generate a 1-wire reset, return 1 of no presence detect was found,
//return 0 otherwise.
//(NOTE: Does not handle alarm presence for DS2404/DS1994)
//
/*
**-----------------------------------------------------------------------------
**
** Abstract:
** This function is OneWire Reset.
**
** Parameters:
** None
**
** Returns:
** Return 1 of no presence detect was found, return 0 otherwise.
**
**-----------------------------------------------------------------------------
*/
unsigned char OWTouchReset(void)
{
unsigned char result = 0;
unsigned char i;
DQOut_Low(); //Drives DQ_T low
__delay_cycles(480*8);
DQOutData = High;
DQChangeInput(); //Releases the bus
__delay_cycles(40*8);
// result = DQ_T ^ 0x01; //Sample for presence pulse from slave
i = 250;
while(!DQ_T)
{
if(--i)
result = 1;
else
{
result = 0;
break;
}
_NOP();
}
__delay_cycles(300*8);
return result; //Return sample presence pulse result
}
/*
**-----------------------------------------------------------------------------
**
** Abstract:
** This function is Send a 1-wire bit.
**
** Parameters:
** provide 10us recovery time
**
** Returns:
** None
**
**-----------------------------------------------------------------------------
*/
void OWWriteBit(unsigned char bit)
{
//write '1' bit
DQOut_Low(); //Drives DQ_T low
// delay1us();
__delay_cycles(8);
DQOutData = bit;
__delay_cycles(90*8); //Complete the time slot and 10us recovery
DQChangeInput(); //Releases the bus
__delay_cycles(30*8);
}
/*
**-----------------------------------------------------------------------------
**
** Abstract:
** This function is Read a bit from the 1-wire bus return it.
** Provide 10us recovery time.
** Parameters:
** None
**
** Returns:
** Return a bit from the 1-wire bus.
**
**-----------------------------------------------------------------------------
*/
unsigned char OWReadBit(void)
{
unsigned char result;
DQOut_Low(); //Drives DQ_T low
// delay1us();
DQChangeInput(); //Releases the bus
__delay_cycles(10*8);
_NOP();_NOP();_NOP();
result = DQ_T & 0x01; //Sample the bit value from the slave
__delay_cycles(60*8); //Complete the time slot and 10us recovery
return result;
}
/*
*******************************************************************************
** This is all for bit-wise manipulation of the 1-Wire bus.The above routines
** can be built upon to create byte-wise manipulator functions as seen in
** Example 3.
** Example 3.Derived 1-Wire Functions
*******************************************************************************
*/
/*
**-----------------------------------------------------------------------------
**
** Abstract:
** This function is Wirte 1-Wire data byte.
**
** Parameters:
** None
**
** Returns:
** None
**
**-----------------------------------------------------------------------------
*/
void OWWriteByte(unsigned char data)
{
unsigned char loop;
//Loop to write each bit in the byte, LS-bit first
for(loop = 8; loop != 0 ; loop--)
{
OWWriteBit(data & 0x01);
data >>= 1;
}
}
/*
**-----------------------------------------------------------------------------
**
** Abstract:
** This function is Read 1-wire data byte and retrurn it.
**
** Parameters:
** None
**
** Returns:
** Return read 1-wire
**
**-----------------------------------------------------------------------------
*/
unsigned char OWReadByte(void)
{
unsigned char loop,result = 0;
for(loop = 8;loop != 0;loop --)
{
//Shift the result to get it ready for the nexit bit
result >>= 1;
//if result is one,then set MS bit
if(OWReadBit())
result |= 0x80;
}
return result;
}
/*
**-----------------------------------------------------------------------------
**
** Abstract:
** This function is Write a 1-wire data byte and return the
** sampled result.
**
** Parameters:
** Write value.
**
** Returns:
** return the sampled result.
**
**-----------------------------------------------------------------------------
*/
unsigned char OWTouchByte(unsigned char data)
{
unsigned char loop,result = 0;
for(loop = 8;loop != 0;loop --)
{
//Shift the result to get it ready for the next bit
result >>= 1;
//if sending a '1' then read a bit else write a '0'
if(data & 0x01)
{
if(OWReadBit())
result |= 0x80;
}
else
OWWriteBit(0);
//Sfhift the data byte for the next bit
data >>= 1;
}
return result;
}
/*
**-----------------------------------------------------------------------------
**
** Abstract:
** This function is Wirte a block 1-wire data bytes and return
** the sampled result in the same buffer.
**
** Parameters:
** None
**
** Returns:
** Return the sampled result.
**
**-----------------------------------------------------------------------------
*/
void OWOverdriveSkip(unsigned char *data,unsigned char data_len)
{
unsigned char loop;
for( loop = 8; loop != 0; loop --)
{
data[loop] = OWTouchByte(data[loop]);
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
1-wire+DS18B20+数码显示+Proteus+595温度测试C源码
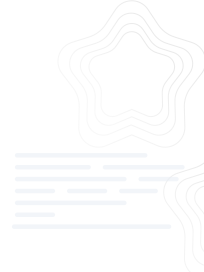
共31个文件
c:7个
r90:5个
h:4个


温馨提示
1-wire总线协议已经应用到各方各面,它具有节省硬件资源,使用方便。通过对DS18B20OneWire的学习与使用,一通百通,对单总线协议有更深的理解。本程序可以直接使用,改进提出更好建议。 本程序已经设计通过,特别是其结构化的设计结构,可以给学习C程序者有个非常好的借鉴。 DS18B20 数码显示 Proteus 74HC595温度测试 C源码。 内包含有Proteus仿真文件。
资源推荐
资源详情
资源评论
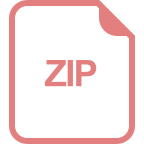
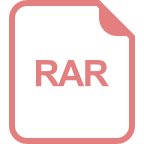
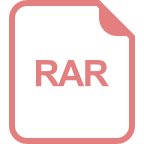
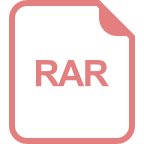
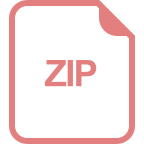
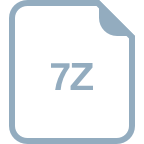
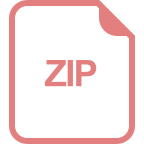
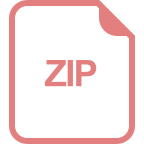
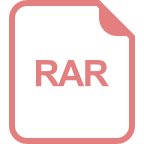
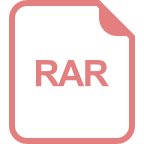
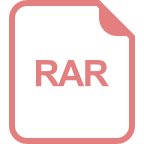
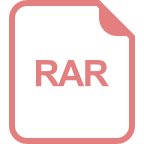
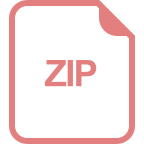
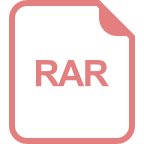
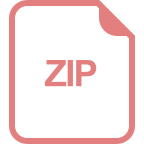
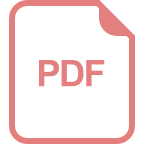
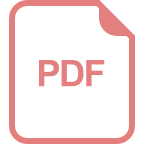
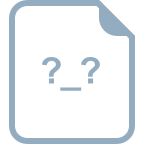
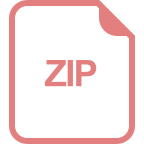
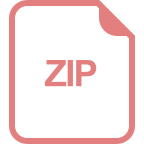
收起资源包目录



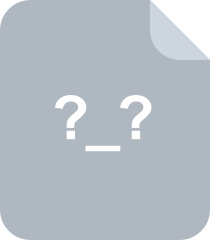

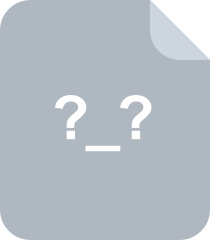
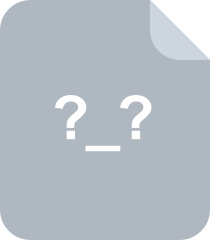
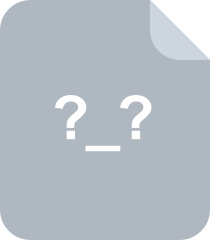
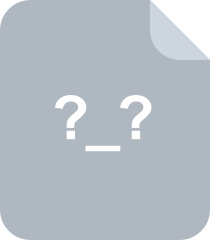
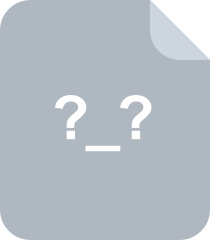
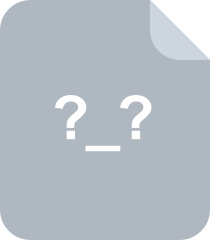


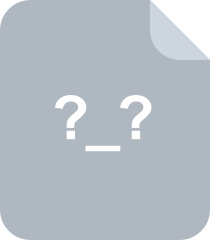
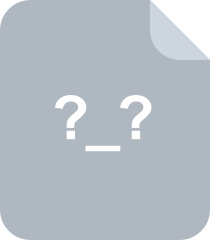
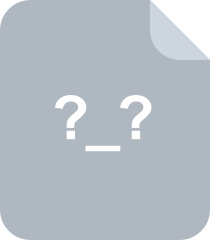
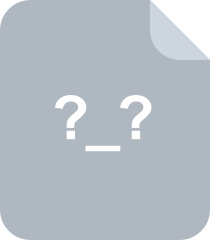
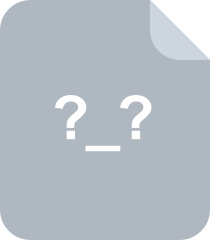
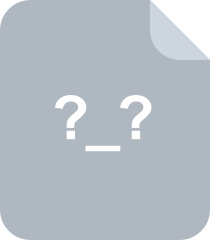


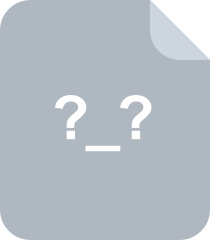
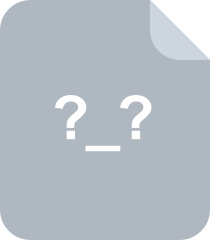
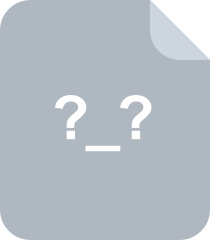

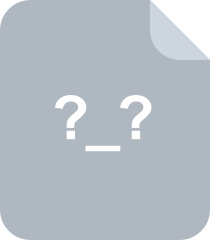
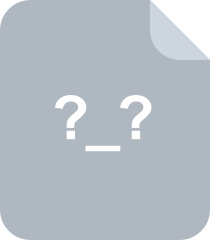
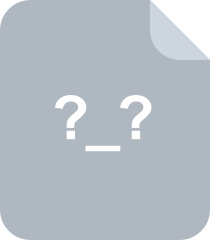
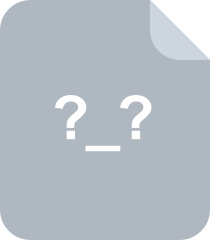
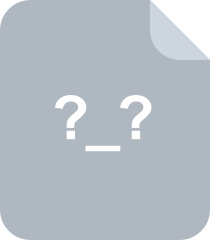
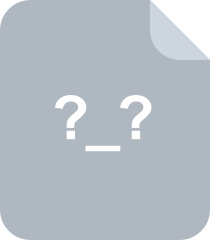
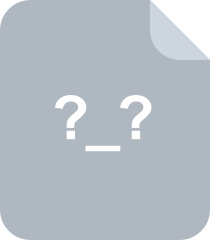
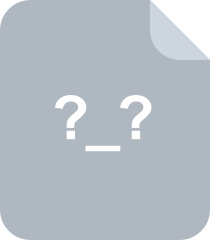
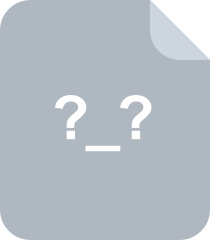
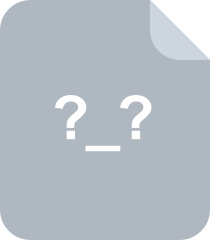
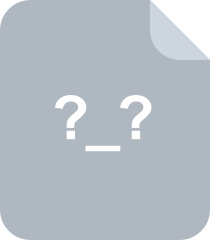
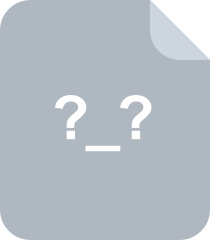
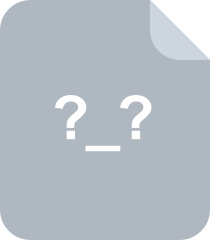
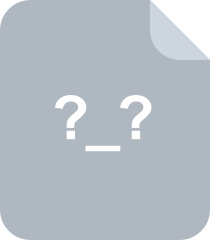
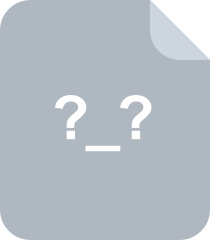
共 31 条
- 1
资源评论
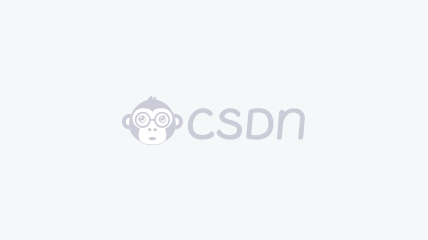
- cnBnuLee2013-04-06很好的资源,对我的帮助太大了,谢谢分享,学到了很多东西!!

熊已出没
- 粉丝: 60
- 资源: 29
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

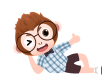
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


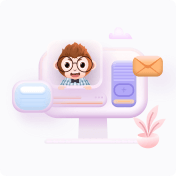
安全验证
文档复制为VIP权益,开通VIP直接复制
