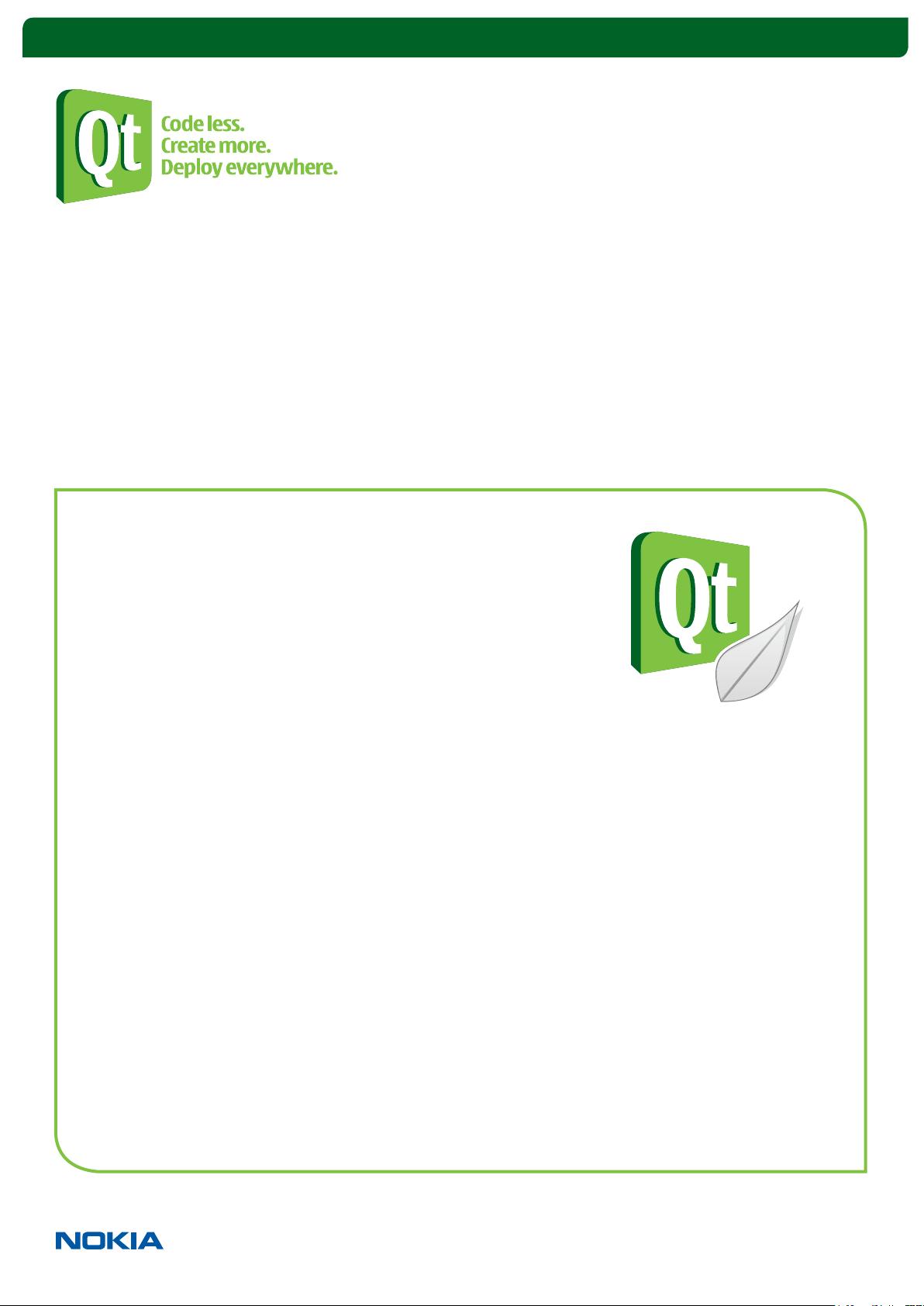
This whitepaper describes the Qt C++ framework. Qt supports the development of cross-
platform GUI applications with its “write once, compile anywhere” approach. Using a single
source tree and a simple recompilation, applications can be written for Windows 98 to XP and
Vista, Mac OS X, Linux, Solaris, HP-UX, and many other versions of Unix with X11. Qt applications
can also be compiled to run on embedded Linux and Windows CE platforms. Qt introduces a
unique inter-object communication mechanism called “signals and slots”. Qt has excellent
cross-platform support for multimedia and 3D graphics, internationalization, SQL, XML, unit
testing, as well as providing platform-specific extensions for specialized applications. Qt ap-
plications can be built visually using Qt Designer, a flexible user interface builder with support
for IDE integration.
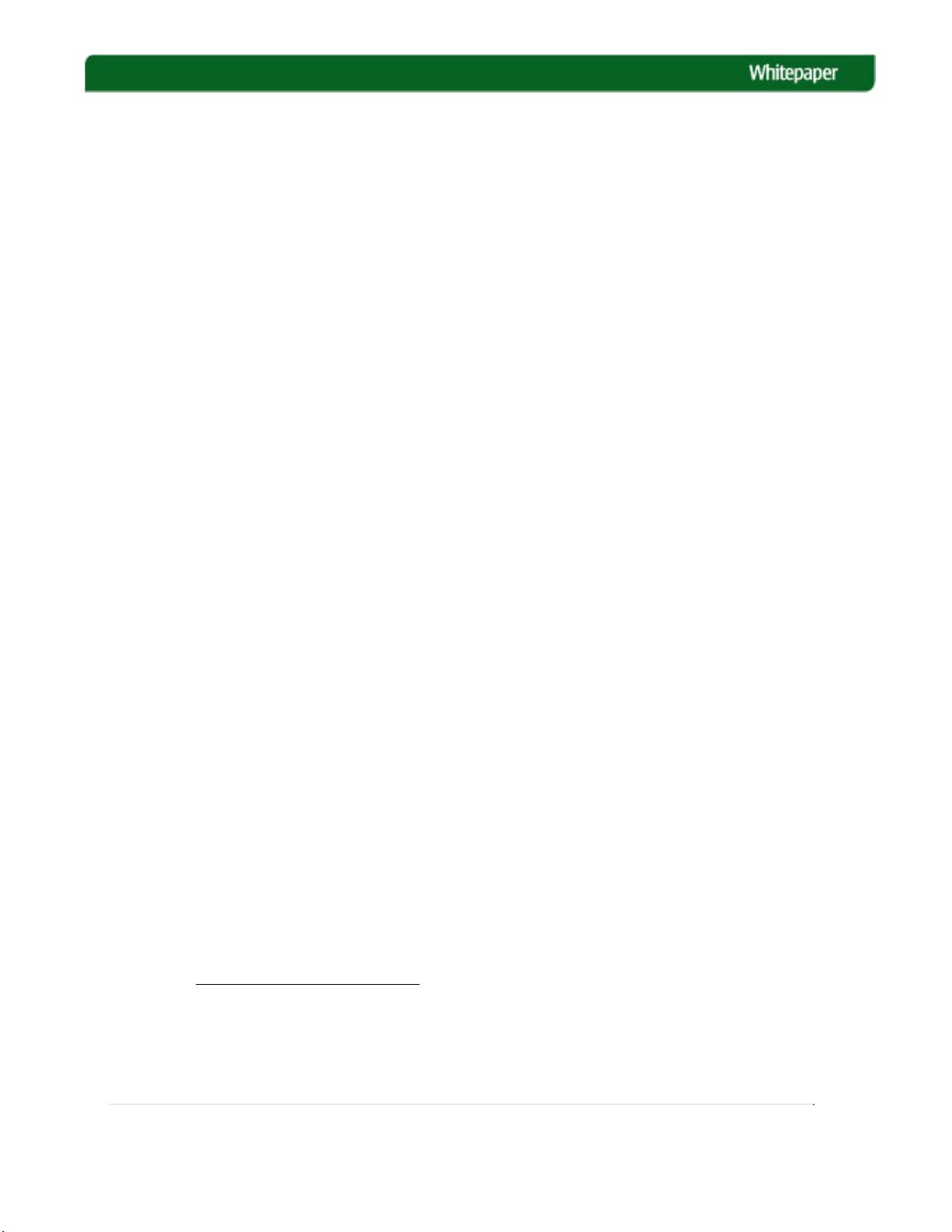
Contents
1. Introduction 3
1.1. Executive Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
2. Graphical User Interfaces 5
2.1. Widgets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2.2. Layouts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.3. Signals and Slots . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
3. Application Features 8
3.1. Main Window Features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
3.2. Dialogs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
3.3. Interactive Help . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
3.4. Wizards . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
3.5. Settings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
3.6. Multithreading and Concurrent Programming . . . . . . . . . . . . . . . . . . . . . 14
3.7. Desktop Integration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
4. Qt Designer 15
4.1. Working with Qt Designer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
4.2. Qt Assistant . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16
4.3. Extending Qt Designer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17
5. Graphics and Multimedia 18
5.1. Painting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
5.2. Images . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
5.3. Paint Devices and Printing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
5.4. Graphics View Framework . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
5.5. Scalable Vector Graphics (SVG) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
5.6. 3D Graphics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
5.7. Multimedia . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
6. Item Views 24
6.1. Standard Item Views . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
6.2. Qt’s Model/View Framework . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25
7. Text Handling 26
7.1. Rich Text Editing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 26
7.2. Customization, Printing and Document Export . . . . . . . . . . . . . . . . . . . . . 27
8. Web Integration with WebKit 28
8.1. Native Application Integration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 28
8.2. Netscape Plugin Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
9. Databases 30
9.1. Executing SQL Commands . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30
9.2. SQL Models . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30
9.3. Data-Aware Widgets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
10.Internationalization 33
10.1.Text Entry and Rendering . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
10.2.Translating Applications . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
10.3.Qt Linguist . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 35
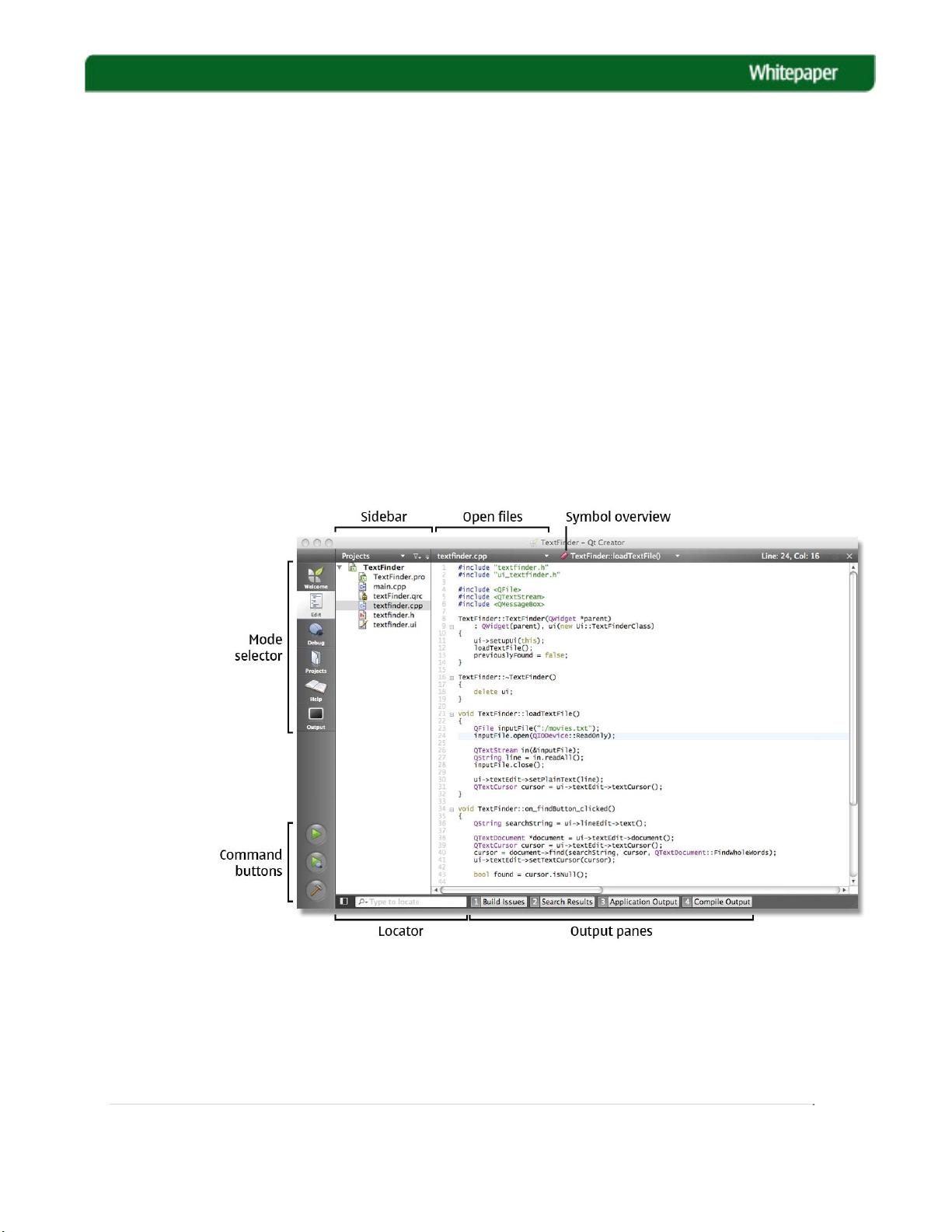
11.Qt Script 37
11.1.Scripting Architecture . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
11.2.Debugging . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38
11.3.Benefits and Uses . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 39
12.Styles and Themes 40
12.1.Built-in Styles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40
12.2.Widget Style Sheets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
12.3.Custom Styles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
13.Events 43
13.1.Event Creation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 43
13.2.Event Delivery . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 43
14.Input/Output and Networking 44
14.1.File Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
14.2.XML . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
14.3.Inter-Process Communication . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 45
14.4.Networking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 45
14.4.1.Encrypted Communications . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
15.Collection Classes 47
15.1.Containers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 47
15.2.Implicit Sharing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 47
16.Plugins and Dynamic Libraries 49
16.1.Plugins . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49
16.2.Dynamic Libraries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49
17.Building Qt Applications 50
17.1.Qt’s Build System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 50
17.2.Qt’s Resource System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
17.3.Testing and Benchmarking Qt Applications . . . . . . . . . . . . . . . . . . . . . . . 51
17.4.Qt Creator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52
18.Qt’s Architecture 53
18.1.X11 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
18.2.Microsoft Windows . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 54
18.3.Mac OS X . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 54
19.Platform Specific Extensions and Qt Solutions 55
19.1.ActiveX Interoperability . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 55
19.2.D-Bus Interoperability . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 55
19.3.Qt Solutions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56
20.The Qt Development Community 57
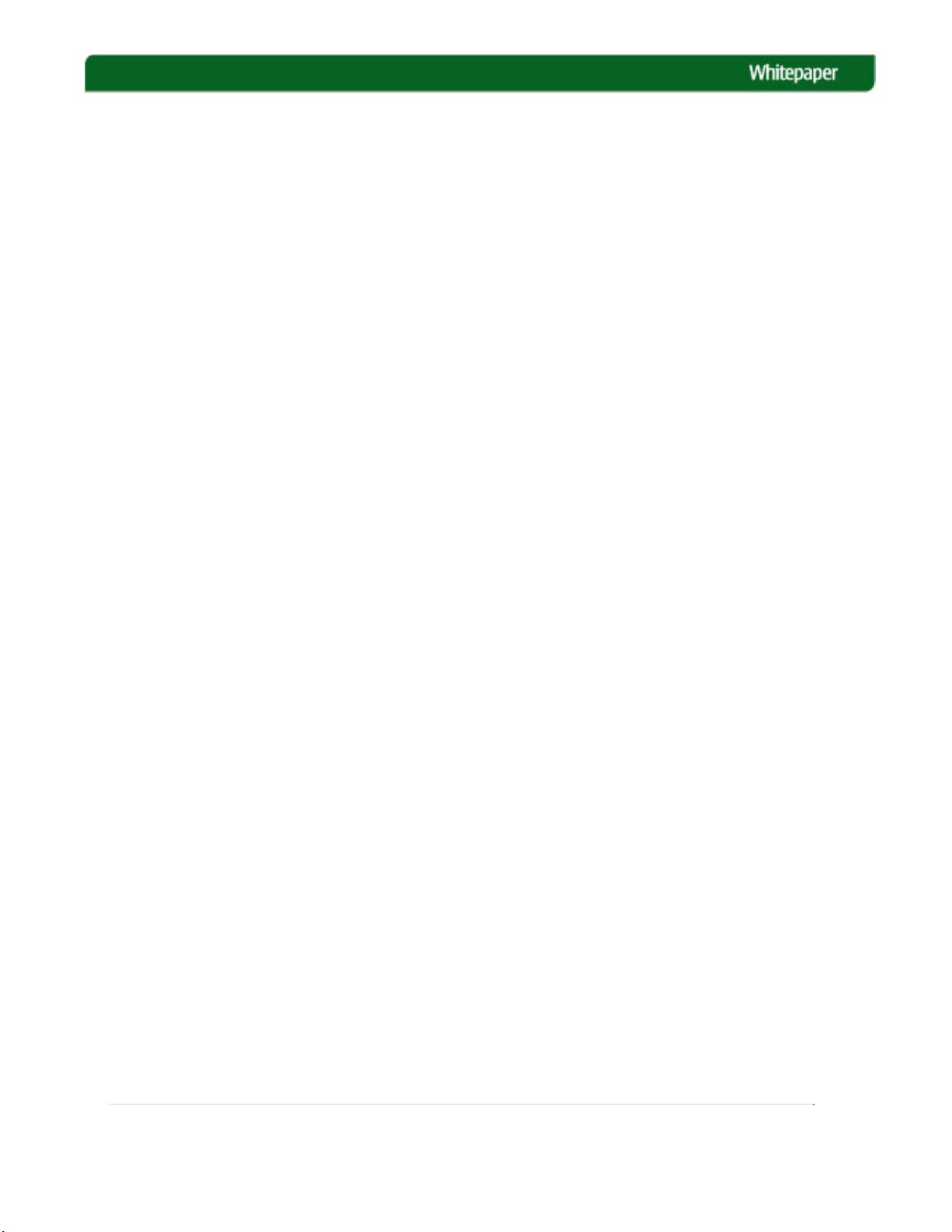
Qt 4.5 Whitepaper
©
2009 Nokia Corporation and/or its subsidiary(-ies)
1. Introduction
Qt is the de facto standard C++ framework for high performance cross-platform soft-
ware development. In addition to an extensive C++ class library, Qt includes tools to
make writing applications fast and straightforward. Qt’s cross-platform capabilities
and internationalization support ensure that Qt applications reach the widest possible
market.
The Qt C++ framework has been at the heart of commercial applications since 1995. Qt is used
by companies and organizations as diverse as Adobe
®
, Boeing
®
, Google
®
, IBM
®
, Motorola
®
,
NASA, Skype
®
, and by numerous smaller companies and organizations. Qt 4 is designed to be
easier to use than previous versions of Qt, while adding more powerful functionality. Qt’s classes
are fully featured and provide consistent interfaces to assist learning, reduce developer workload,
and increase programmer productivity. Qt is, and always has been, fully object-oriented.
This whitepaper gives an overview of Qt’s tools and functionality. Each section begins with a non-
technical introduction before providing a more detailed description of relevant features. Links
to online resources are also given for each subject area.
To evaluate Qt for 30 days, visit http://qt.nokia.com/.
1.1. Executive Summary
Qt includes a rich set of widgets (“controls” in Windows terminology) that provide standard GUI
functionality (see page 5). Qt introduces an innovative alternative for inter-object communica-
tion, called “signals and slots” (see page 6), that replaces the old and unsafe callback technique
used in many legacy frameworks. Qt also provides a conventional event model (page 43) for
handling mouse clicks, key presses, and other user input. Qt’s cross-platform GUI applications
(page 8) can support all the user interface functionality required by modern applications, such
as menus, context menus, drag and drop, and dockable toolbars. Desktop integration features
(page 14) provided by Qt can be used to extend applications into the surrounding desktop envi-
ronment, taking advantage of some of the services provided on each platform.
Qt also includes Qt Designer (page 15), a tool for graphically designing user interfaces. Qt Designer
supports Qt’s powerful layout features (page 6) in addition to absolute positioning. Qt Designer
can be used purely for GUI design, or to create entire applications with its support for integration
with popular integrated development environments (IDEs).
Qt has excellent support for multimedia and 3D graphics (page 18). Qt is the de facto standard
GUI framework for platform-independent OpenGL
®
programming. Qt’s painting system offers
high quality rendering across all supported platforms. A sophisticated canvas framework (page
21) enables developers to create interactive graphical applications that take advantage of Qt’s
advanced painting features.
Qt makes it possible to create platform-independent database applications using standard data-
bases (page 30). Qt includes native drivers for Oracle
®
, Microsoft
®
SQL Server, Sybase
®
Adap-
tive Server, IBM DB2
®
, PostgreSQL
, MySQL
®
, Borland
®
Interbase, SQLite, and ODBC-compliant
databases. Qt includes database-specific widgets, and any built-in or custom widget can be made
data-aware.
Qt programs have native look and feel on all supported platforms using Qt’s styles and themes
3
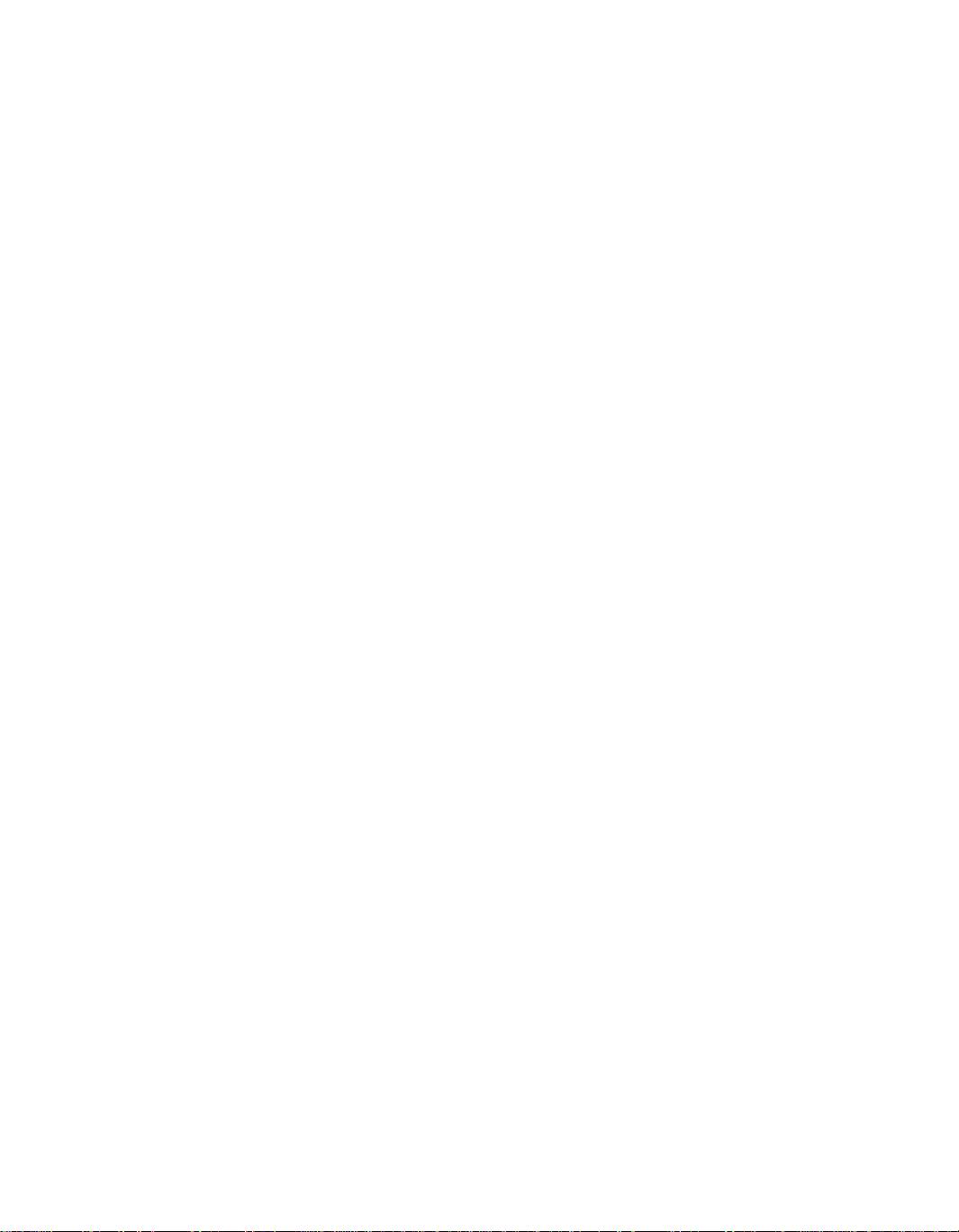
Qt 4.5 Whitepaper
©
2009 Nokia Corporation and/or its subsidiary(-ies)
support (page 40). From a single source tree, recompilation is all that is required to produce
applications for Windows
®
98 to XP
®
and Windows Vista
, Mac OS X
®
, Linux
®
, Solaris
, HP-
UX
, and many other versions of Unix
®
with X11
. Qt’s qmake build tool produces makefiles or
.dsp files appropriate to the target platform (page 50).
Since Qt’s architecture takes advantage of the underlying platform, many customers use Qt for
single-platform development on Windows, Mac OS X, and Unix because they prefer Qt’s approach.
Qt includes support for important platform-specific features, such as ActiveX
®
on Windows, and
Motif
on Unix. See the section on Qt’s Architecture (page 53) for more information.
Qt uses Unicode
throughout and has considerable support for internationalization (page 33).
Qt includes Qt Linguist and other tools to support translators. Applications can easily use and mix
text in Arabic, Chinese, English, Hebrew, Japanese, Russian, and other languages supported by
Unicode.
Qt includes a variety of domain-specific classes. For example, Qt has an XML module (page 44) that
includes SAX and DOM classes for reading and manipulating data stored in XML-based formats.
Objects can be stored in memory using Qt’s STL-compatible collection classes (page 47), and
handled using styles of iterators used in Java
®
and the C++ Standard Template Library (STL).
Local and remote file handling using standard protocols are provided by Qt’s input/output and
networking classes (page 44).
Qt applications can have their functionality extended by plugins and dynamic libraries (page 49).
Plugins provide additional codecs, database drivers, image formats, styles, and widgets. Plugins
and libraries can be sold as products in their own right.
The QtScript module (see page 37) enables applications to be scripted with Qt Script, an ECMAScript-
based language related to JavaScript. This technology allows developers to give users restricted
access to parts of their applications for scripting purposes.
Qt is a mature C++ framework that is widely used around the world. In addition to Qt’s many
commercial uses, the Open Source edition of Qt is the foundation of KDE, the Linux desktop en-
vironment. Qt makes application development a pleasure, with its cross-platform build system,
visual form design, and elegant API.
Online References
http://qt.nokia.com/qt-in-use
4