/*
* This file has been generated to support Visual Studio IntelliSense.
* You should not use this file at runtime inside the browser--it is only
* intended to be used only for design-time IntelliSense. Please use the
* standard jQuery library for all production use.
*
* Comment version: 1.5
*/
/*!
* jQuery JavaScript Library v1.5
* http://jquery.com/
*
* Distributed in whole under the terms of the MIT
*
* Copyright 2010, John Resig
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*
* Includes Sizzle.js
* http://sizzlejs.com/
* Copyright 2010, The Dojo Foundation
* Released under the MIT and BSD Licenses.
*/
(function (window, undefined) {
var jQuery = function (selector, context) {
/// <summary>
/// 1: Accepts a string containing a CSS selector which is then used to match a set of elements.
/// 1.1 - $(selector, context)
/// 1.2 - $(element)
/// 1.3 - $(elementArray)
/// 1.4 - $(jQuery object)
/// 1.5 - $()
/// 2: Creates DOM elements on the fly from the provided string of raw HTML.
/// 2.1 - $(html, ownerDocument)
/// 2.2 - $(html, props)
/// 3: Binds a function to be executed when the DOM has finished loading.
/// 3.1 - $(callback)
/// </summary>
/// <param name="selector" type="String">
/// A string containing a selector expression
/// </param>
/// <param name="context" type="jQuery">
/// A DOM Element, Document, or jQuery to use as context
/// </param>
/// <returns type="jQuery" />
// The jQuery object is actually just the init constructor 'enhanced'
return new jQuery.fn.init(selector, context, rootjQuery);
};
jQuery.Deferred = function (func) {
var deferred = jQuery._Deferred(),
failDeferred = jQuery._Deferred(),
promise;
// Add errorDeferred methods, then and promise
jQuery.extend(deferred, {
then: function (doneCallbacks, failCallbacks) {
deferred.done(doneCallbacks).fail(failCallbacks);
return this;
},
fail: failDeferred.done,
rejectWith: failDeferred.resolveWith,
reject: failDeferred.resolve,
isRejected: failDeferred.isResolved,
// Get a promise for this deferred
// If obj is provided, the promise aspect is added to the object
promise: function (obj, i /* internal */) {
if (obj == null) {
if (promise) {
return promise;
}
promise = obj = {};
}
i = promiseMethods.length;
while (i--) {
obj[promiseMethods[i]] = deferred[promiseMethods[i]];
}
return obj;
}
});
// Make sure only one callback list will be used
deferred.then(failDeferred.cancel, deferred.cancel);
// Unexpose cancel
delete deferred.cancel;
// Call given func if any
if (func) {
func.call(deferred, deferred);
}
return deferred;
};
jQuery.Event = function (src) {
// Allow instantiation without the 'new' keyword
if (!this.preventDefault) {
return new jQuery.Event(src);
}
// Event object
if (src && src.type) {
this.originalEvent = src;
this.type = src.type;
// Events bubbling up the document may have been marked as prevented
// by a handler lower down the tree; reflect the correct value.
this.isDefaultPrevented = (src.defaultPrevented || src.returnValue === false ||
src.getPreventDefault && src.getPreventDefault()) ? returnTrue : returnFalse;
// Event type
} else {
this.type = src;
}
// timeStamp is buggy for some events on Firefox(#3843)
// So we won't rely on the native value
this.timeStamp = jQuery.now();
// Mark it as fixed
this[jQuery.expando] = true;
};
jQuery._Deferred = function () {
var // callbacks list
callbacks = [],
// stored [ context , args ]
fired,
// to avoid firing when already doing so
firing,
// flag to know if the deferred has been cancelled
cancelled,
// the deferred itself
deferred = {
// done( f1, f2, ...)
done: function () {
if (!cancelled) {
var args = arguments,
i,
length,
elem,
type,
_fired;
if (fired) {
_fired = fired;
fired = 0;
}
for (i = 0, length = args.length; i < length; i++) {
elem = args[i];
type = jQuery.type(elem);
if (type === "array") {
deferred.done.apply(deferred, elem);
} else if (type === "function") {
callbacks.push(elem);
}
}
if (_fired) {
deferred.resolveWith(_fired[0], _fired[1]);
}
}
return this;
},
// resolve with given context and args
resolveWith: function (context, args) {
if (!cancelled && !fired && !firing) {
firing = 1;
try {
while (callbacks[0]) {
callbacks.shift().apply(context, args);
}
}
finally {
fired = [context, args];
firing = 0;
}
}
return this;
},
// resolve with this as context and given arguments
resolve: function () {
deferred.resolveWith(jQuery.isFunction(this.promise) ? this.promise() : this, arguments);
return this;
},
// Has this deferred been resolved?
isResolved: function () {
return !!(firing || fired);
},
// Cancel
cancel: function () {
cancelled = 1;
callbacks = [];
return this;
}
};
return deferred;
};
jQuery._data = function (elem, name, data) {
return jQuery.data(elem, name, data, true);
};
jQuery.acceptData = function (elem) {
if (elem.nodeName) {
var match = jQuery.noData[elem.nodeName.toLowerCase()];
if (match) {
return !(match === true || elem.getAttribute("cl
没有合适的资源?快使用搜索试试~ 我知道了~
全版本 jQuery.vsdoc.js 智能感应文档, For Visual Studio
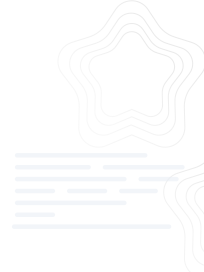
共5个文件
js:5个

需积分: 3 10 下载量 27 浏览量
2011-12-29
11:56:22
上传
评论 1
收藏 191KB RAR 举报
温馨提示
目前(2012.1)为止全版本的 jQuery.vsdoc 系列文档,满足您不同版本的需求。 在此下载包中,目前受支持的 jQuery 版本是: 1.3.2; 1.4.2; 1.5; 1.6; 1.7; 如果还需要其他小版本,如 1.5.1 或 1.6.3 等其他小版本的 jQuery.vsdoc 文件,请移步:http://1000eb.com/5w8n (1000eb.com 千易是完全免费、无需登录的清爽网盘,请放心下载)
资源推荐
资源详情
资源评论
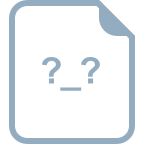
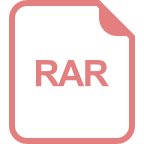
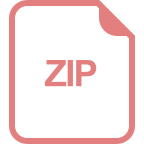
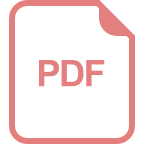
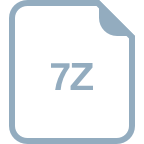
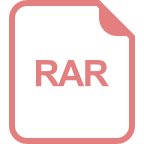
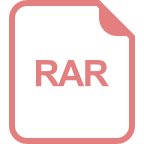
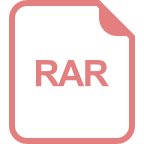
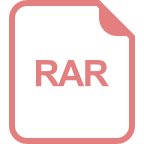
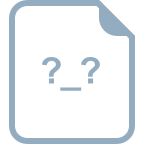
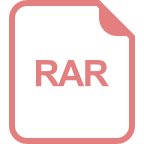
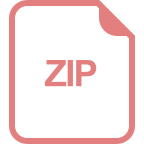
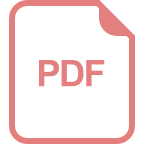
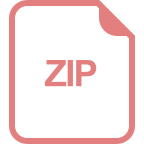
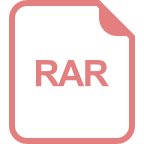
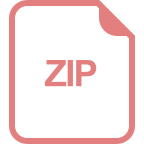
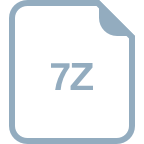
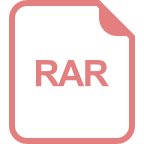
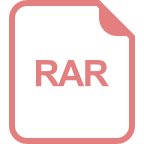
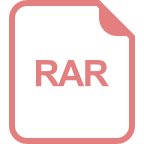
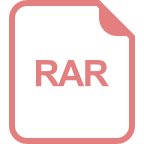
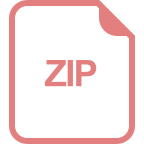
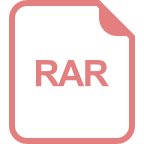
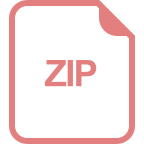
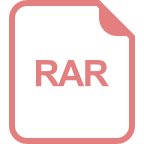
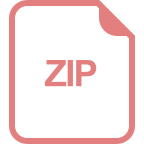
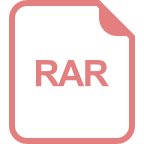
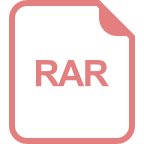
收起资源包目录

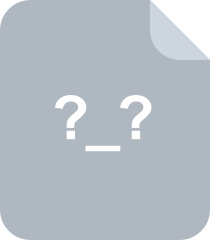
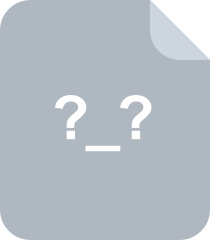
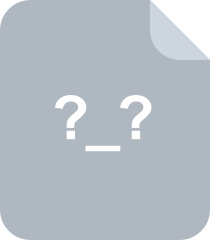
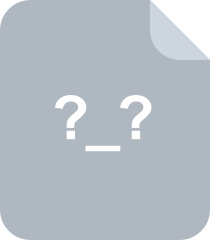
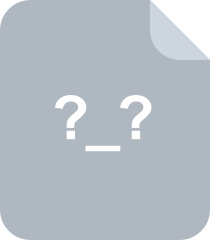
共 5 条
- 1
资源评论
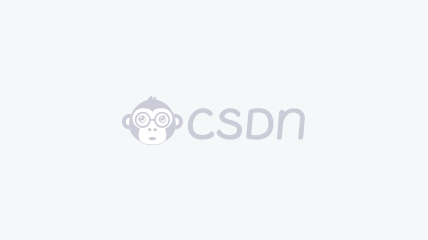

ciznx
- 粉丝: 35
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

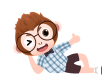
最新资源
- 包括用 Java 编写的程序 欢迎您在此做出贡献!.zip
- (源码)基于QT框架的学生管理系统.zip
- 功能齐全的 Java Socket.IO 客户端库,兼容 Socket.IO v1.0 及更高版本 .zip
- 功能性 javascript 研讨会 无需任何库(即无需下划线),只需 ES5 .zip
- 分享Java相关的东西 - Java安全漫谈笔记相关内容.zip
- 具有适合 Java 应用程序的顺序定义的 Cloud Native Buildpack.zip
- 网络建设运维资料库职业
- 关于 Java 的一切.zip
- 爬虫安装 XPath Helper 2.0
- 使用特定版本的 Java 设置 GitHub Actions 工作流程.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


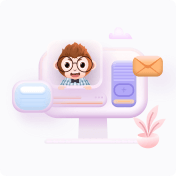
安全验证
文档复制为VIP权益,开通VIP直接复制
