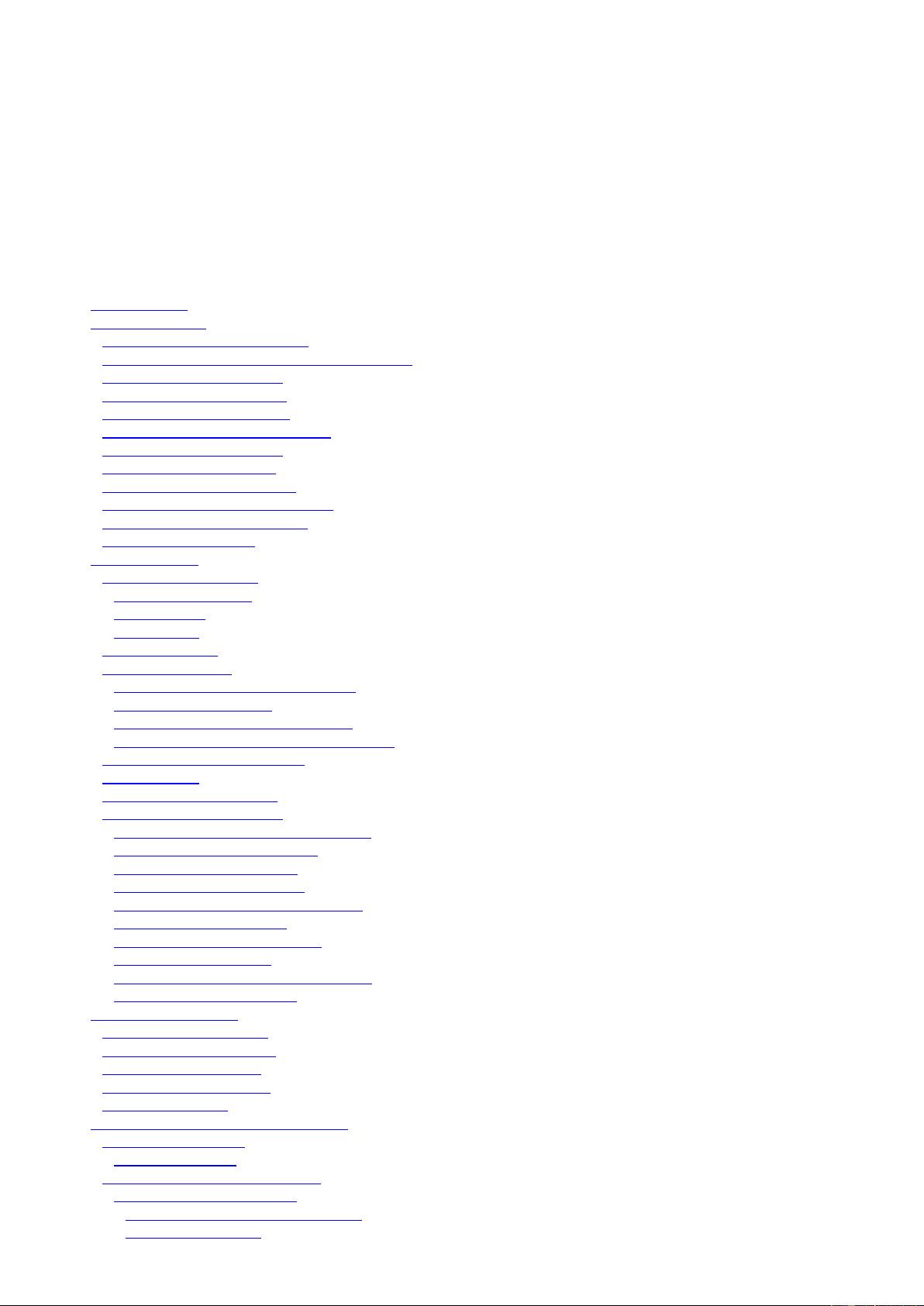
2
The Grails Framework - Reference Documentation
Authors: Graeme Rocher, Peter Ledbrook, Marc Palmer, Jeff Brown, Luke Daley, Burt Beckwith
Version: 1.3.7
Copies of this document may be made for your own use and for distribution to others, provided that you do not
charge any fee for such copies and further provided that each copy contains this Copyright Notice, whether
distributed in print or electronically.
Table of Contents
1. Introduction ................................................................................................................................................................ 6
2. Getting Started ............................................................................................................................................................ 7
2.1 Downloading and Installing .................................................................................................................................. 7
2.2 Upgrading from previous versions of Grails ......................................................................................................... 7
2.3 Creating an Application ....................................................................................................................................... 10
2.4 A Hello World Example ...................................................................................................................................... 11
2.5 Getting Set-up in an IDE ..................................................................................................................................... 11
2.6 Convention over Configuration ........................................................................................................................... 12
2.7 Running an Application ....................................................................................................................................... 12
2.8 Testing an Application ........................................................................................................................................ 13
2.9 Deploying an Application .................................................................................................................................... 13
2.10 Supported Java EE Containers .......................................................................................................................... 13
2.11 Generating an Application ................................................................................................................................. 14
2.12 Creating Artefacts .............................................................................................................................................. 14
3. Configuration ............................................................................................................................................................ 15
3.1 Basic Configuration ............................................................................................................................................. 15
3.1.1 Built in options .............................................................................................................................................. 15
3.1.2 Logging .......................................................................................................................................................... 16
3.1.3 GORM ........................................................................................................................................................... 23
3.2 Environments ....................................................................................................................................................... 23
3.3 The DataSource ................................................................................................................................................... 25
3.3.1 DataSources and Environments ..................................................................................................................... 26
3.3.2 JNDI DataSources ......................................................................................................................................... 26
3.3.3 Automatic Database Migration ...................................................................................................................... 27
3.3.4 Transaction-aware DataSource Proxy ........................................................................................................... 28
3.4 Externalized Configuration ................................................................................................................................. 28
3.5 Versioning ........................................................................................................................................................... 29
3.6 Project Documentation ........................................................................................................................................ 29
3.7 Dependency Resolution ....................................................................................................................................... 33
3.7.1 Configurations and Dependencies ................................................................................................................. 34
3.7.2 Dependency Repositories .............................................................................................................................. 35
3.7.3 Debugging Resolution ................................................................................................................................... 37
3.7.4 Inherited Dependencies ................................................................................................................................. 37
3.7.5 Providing Default Dependencies ................................................................................................................... 38
3.7.6 Dependency Reports ...................................................................................................................................... 38
3.7.7 Plugin JAR Dependencies ............................................................................................................................. 38
3.7.8 Maven Integration .......................................................................................................................................... 39
3.7.9 Deploying to a Maven Repository ................................................................................................................. 39
3.7.10 Plugin Dependencies ................................................................................................................................... 41
4. The Command Line .................................................................................................................................................. 43
4.1 Creating Gant Scripts .......................................................................................................................................... 44
4.2 Re-using Grails scripts ........................................................................................................................................ 44
4.3 Hooking into Events ............................................................................................................................................ 46
4.4 Customising the build .......................................................................................................................................... 48
4.5 Ant and Maven .................................................................................................................................................... 50
5. Object Relational Mapping (GORM) ....................................................................................................................... 55
5.1 Quick Start Guide ................................................................................................................................................ 55
5.1.1 Basic CRUD .................................................................................................................................................. 55
5.2 Domain Modelling in GORM ............................................................................................................................. 56
5.2.1 Association in GORM ................................................................................................................................... 57
5.2.1.1 Many-to-one and one-to-one ................................................................................................................... 57
5.2.1.2 One-to-many ............................................................................................................................................ 58
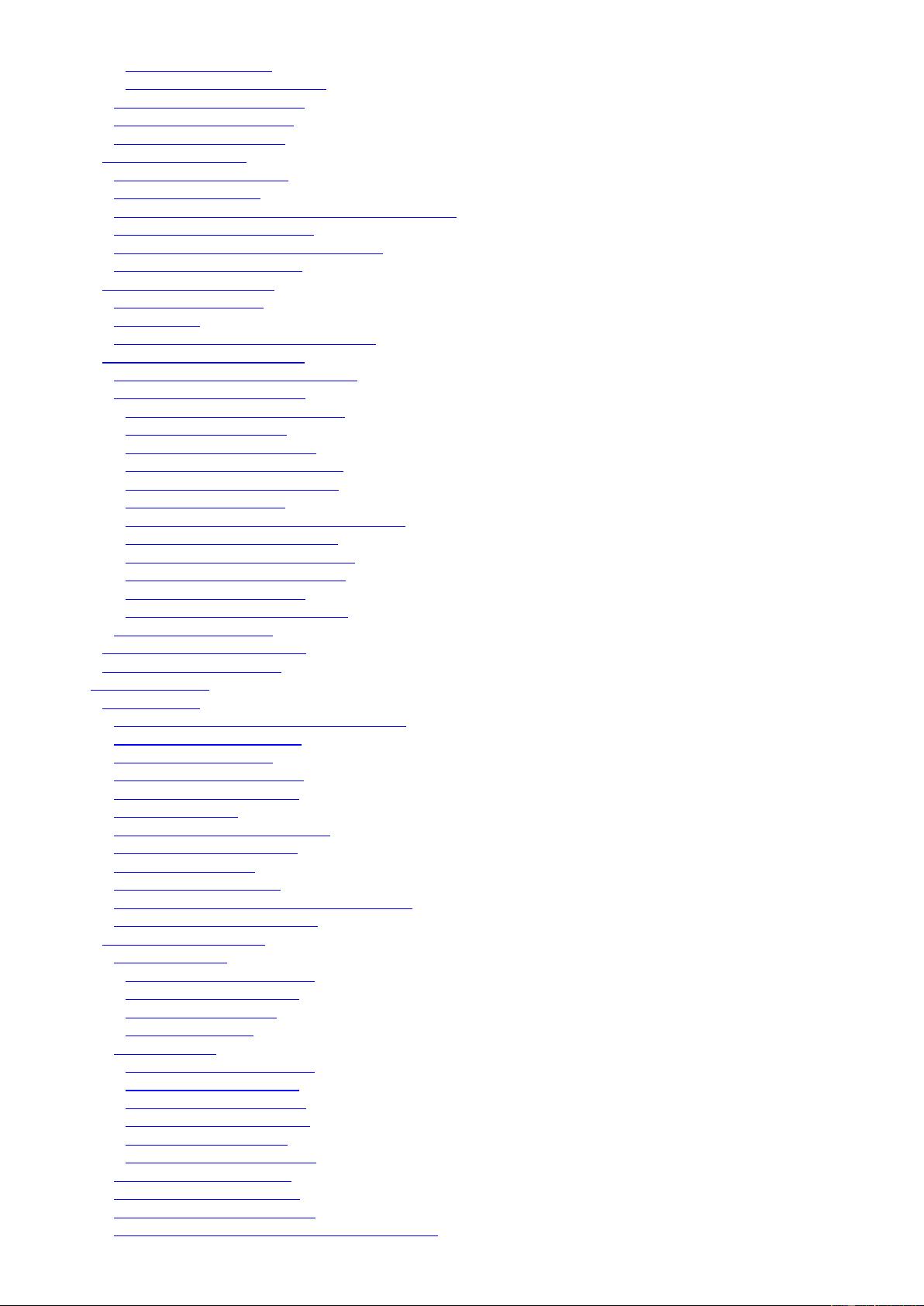
3
5.2.1.3 Many-to-many ......................................................................................................................................... 59
5.2.1.4 Basic Collection Types ............................................................................................................................ 60
5.2.2 Composition in GORM ................................................................................................................................. 61
5.2.3 Inheritance in GORM .................................................................................................................................... 61
5.2.4 Sets, Lists and Maps ...................................................................................................................................... 62
5.3 Persistence Basics ................................................................................................................................................ 64
5.3.1 Saving and Updating ..................................................................................................................................... 64
5.3.2 Deleting Objects ............................................................................................................................................ 65
5.3.3 Understanding Cascading Updates and Deletes ............................................................................................ 65
5.3.4 Eager and Lazy Fetching ............................................................................................................................... 67
5.3.5 Pessimistic and Optimistic Locking .............................................................................................................. 68
5.3.6 Modification Checking .................................................................................................................................. 69
5.4 Querying with GORM ......................................................................................................................................... 70
5.4.1 Dynamic Finders ............................................................................................................................................ 71
5.4.2 Criteria ........................................................................................................................................................... 73
5.4.3 Hibernate Query Language (HQL) ................................................................................................................ 76
5.5 Advanced GORM Features ................................................................................................................................. 77
5.5.1 Events and Auto Timestamping .................................................................................................................... 78
5.5.2 Custom ORM Mapping ................................................................................................................................. 82
5.5.2.1 Table and Column Names ........................................................................................................................ 83
5.5.2.2 Caching Strategy ...................................................................................................................................... 85
5.5.2.3 Inheritance Strategies ............................................................................................................................... 87
5.5.2.4 Custom Database Identity ........................................................................................................................ 87
5.5.2.5 Composite Primary Keys ......................................................................................................................... 88
5.5.2.6 Database Indices ...................................................................................................................................... 88
5.5.2.7 Optimistic Locking and Versioning ......................................................................................................... 88
5.5.2.8 Eager and Lazy Fetching ......................................................................................................................... 89
5.5.2.9 Custom Cascade Behaviour ..................................................................................................................... 91
5.5.2.10 Custom Hibernate Types ....................................................................................................................... 91
5.5.2.11 Derived Properties ................................................................................................................................. 93
5.5.2.12 Custom Naming Strategy ....................................................................................................................... 94
5.5.3 Default Sort Order ......................................................................................................................................... 94
5.6 Programmatic Transactions ................................................................................................................................. 95
5.7 GORM and Constraints ....................................................................................................................................... 96
6. The Web Layer ......................................................................................................................................................... 99
6.1 Controllers ........................................................................................................................................................... 99
6.1.1 Understanding Controllers and Actions ........................................................................................................ 99
6.1.2 Controllers and Scopes ................................................................................................................................ 100
6.1.3 Models and Views ....................................................................................................................................... 100
6.1.4 Redirects and Chaining ................................................................................................................................ 103
6.1.5 Controller Interceptors ................................................................................................................................. 105
6.1.6 Data Binding ................................................................................................................................................ 106
6.1.7 XML and JSON Responses ......................................................................................................................... 110
6.1.8 More on JSONBuilder ................................................................................................................................. 112
6.1.9 Uploading Files ............................................................................................................................................ 114
6.1.10 Command Objects ..................................................................................................................................... 115
6.1.11 Handling Duplicate Form Submissions ..................................................................................................... 116
6.1.12 Simple Type Converters ............................................................................................................................ 117
6.2 Groovy Server Pages ......................................................................................................................................... 117
6.2.1 GSP Basics .................................................................................................................................................. 118
6.2.1.1 Variables and Scopes ............................................................................................................................. 118
6.2.1.2 Logic and Iteration ................................................................................................................................. 118
6.2.1.3 Page Directives ...................................................................................................................................... 119
6.2.1.4 Expressions ............................................................................................................................................ 119
6.2.2 GSP Tags ..................................................................................................................................................... 120
6.2.2.1 Variables and Scopes ............................................................................................................................. 120
6.2.2.2 Logic and Iteration ................................................................................................................................. 121
6.2.2.3 Search and Filtering ............................................................................................................................... 121
6.2.2.4 Links and Resources .............................................................................................................................. 122
6.2.2.5 Forms and Fields .................................................................................................................................... 122
6.2.2.6 Tags as Method Calls ............................................................................................................................. 123
6.2.3 Views and Templates ................................................................................................................................... 123
6.2.4 Layouts with Sitemesh ................................................................................................................................. 125
6.2.5 Sitemesh Content Blocks ............................................................................................................................. 127
6.2.6 Making Changes to a Deployed Application ............................................................................................... 128
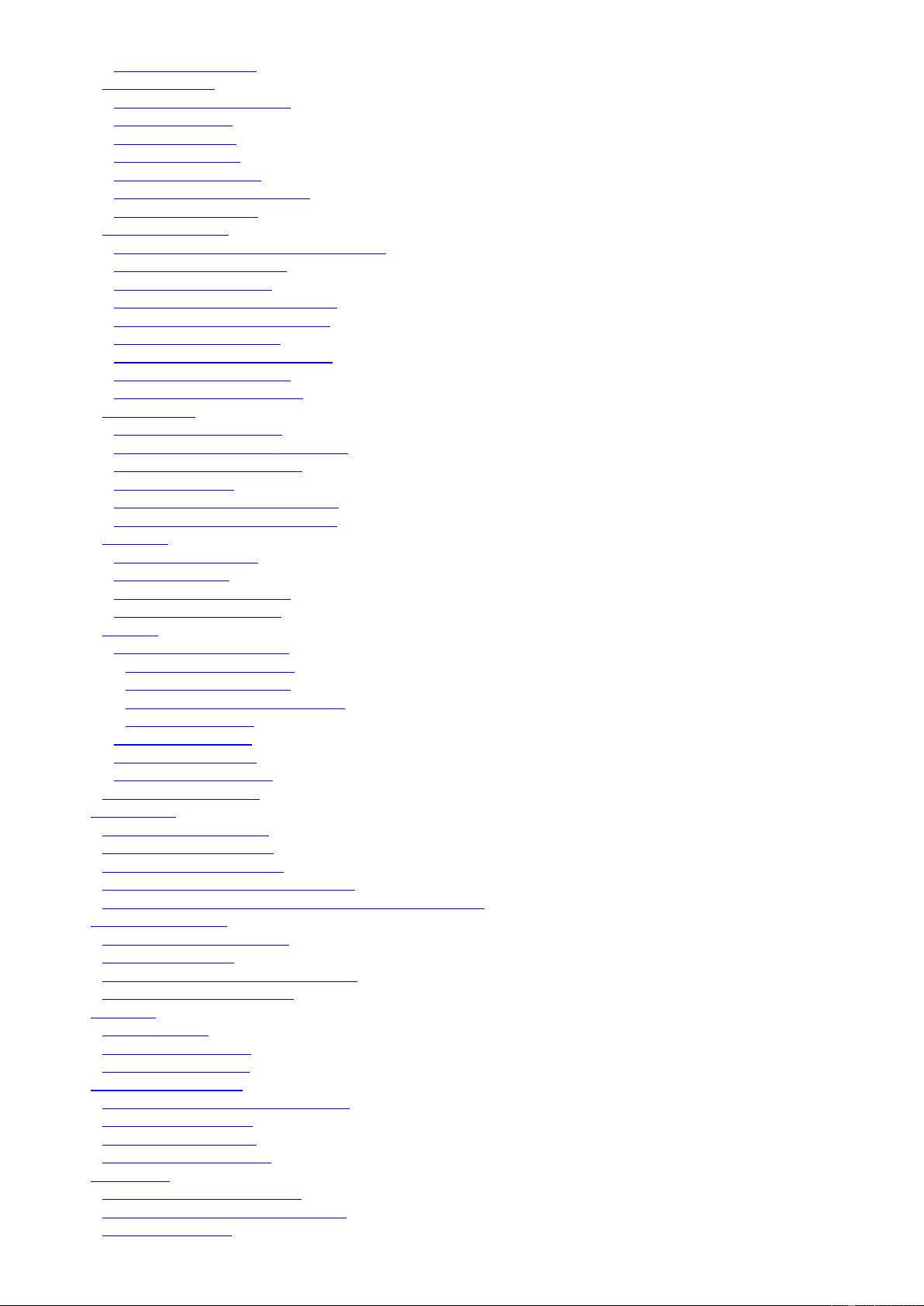
4
6.2.7 GSP Debugging ........................................................................................................................................... 129
6.3 Tag Libraries ..................................................................................................................................................... 129
6.3.1 Variables and Scopes ................................................................................................................................... 130
6.3.2 Simple Tags ................................................................................................................................................. 131
6.3.3 Logical Tags ................................................................................................................................................ 131
6.3.4 Iterative Tags ............................................................................................................................................... 132
6.3.5 Tag Namespaces .......................................................................................................................................... 133
6.3.6 Using JSP Tag Libraries .............................................................................................................................. 133
6.3.7 Tag return value ........................................................................................................................................... 133
6.4 URL Mappings .................................................................................................................................................. 134
6.4.1 Mapping to Controllers and Actions ........................................................................................................... 134
6.4.2 Embedded Variables .................................................................................................................................... 135
6.4.3 Mapping to Views ....................................................................................................................................... 136
6.4.4 Mapping to Response Codes ....................................................................................................................... 137
6.4.5 Mapping to HTTP methods ......................................................................................................................... 137
6.4.6 Mapping Wildcards ..................................................................................................................................... 138
6.4.7 Automatic Link Re-Writing ......................................................................................................................... 139
6.4.8 Applying Constraints ................................................................................................................................... 139
6.4.9 Named URL Mappings ................................................................................................................................ 140
6.5 Web Flow .......................................................................................................................................................... 141
6.5.1 Start and End States ..................................................................................................................................... 142
6.5.2 Action States and View States ..................................................................................................................... 142
6.5.3 Flow Execution Events ................................................................................................................................ 144
6.5.4 Flow Scopes ................................................................................................................................................. 145
6.5.5 Data Binding and Validation ....................................................................................................................... 146
6.5.6 Subflows and Conversations ....................................................................................................................... 147
6.6 Filters ................................................................................................................................................................. 148
6.6.1 Applying Filters ........................................................................................................................................... 149
6.6.2 Filter Types .................................................................................................................................................. 150
6.6.3 Variables and Scopes ................................................................................................................................... 150
6.6.4 Filter Dependencies ..................................................................................................................................... 151
6.7 Ajax ................................................................................................................................................................... 151
6.7.1 Ajax using Prototype ................................................................................................................................... 151
6.7.1.1 Remoting Linking .................................................................................................................................. 152
6.7.1.2 Updating Content ................................................................................................................................... 152
6.7.1.3 Remote Form Submission ...................................................................................................................... 153
6.7.1.4 Ajax Events ............................................................................................................................................ 153
6.7.2 Ajax with Dojo ............................................................................................................................................ 154
6.7.3 Ajax with GWT ........................................................................................................................................... 154
6.7.4 Ajax on the Server ....................................................................................................................................... 154
6.8 Content Negotiation ........................................................................................................................................... 156
7. Validation ............................................................................................................................................................... 159
7.1 Declaring Constraints ........................................................................................................................................ 159
7.2 Validating Constraints ....................................................................................................................................... 160
7.3 Validation on the Client ..................................................................................................................................... 161
7.4 Validation and Internationalization ................................................................................................................... 161
7.5 Validation Non Domain and Command Object Classes ................................................................................... 162
8. The Service Layer .................................................................................................................................................. 164
8.1 Declarative Transactions ................................................................................................................................... 164
8.2 Scoped Services ................................................................................................................................................. 165
8.3 Dependency Injection and Services ................................................................................................................... 165
8.4 Using Services from Java .................................................................................................................................. 166
9. Testing .................................................................................................................................................................... 168
9.1 Unit Testing ....................................................................................................................................................... 170
9.2 Integration Testing ............................................................................................................................................. 174
9.3 Functional Testing ............................................................................................................................................. 180
10. Internationalization ............................................................................................................................................... 182
10.1 Understanding Message Bundles .................................................................................................................... 182
10.2 Changing Locales ............................................................................................................................................ 182
10.3 Reading Messages ........................................................................................................................................... 182
10.4 Scaffolding and i18n ........................................................................................................................................ 183
11. Security ................................................................................................................................................................. 184
11.1 Securing Against Attacks ................................................................................................................................ 184
11.2 Encoding and Decoding Objects ..................................................................................................................... 185
11.3 Authentication ................................................................................................................................................. 187
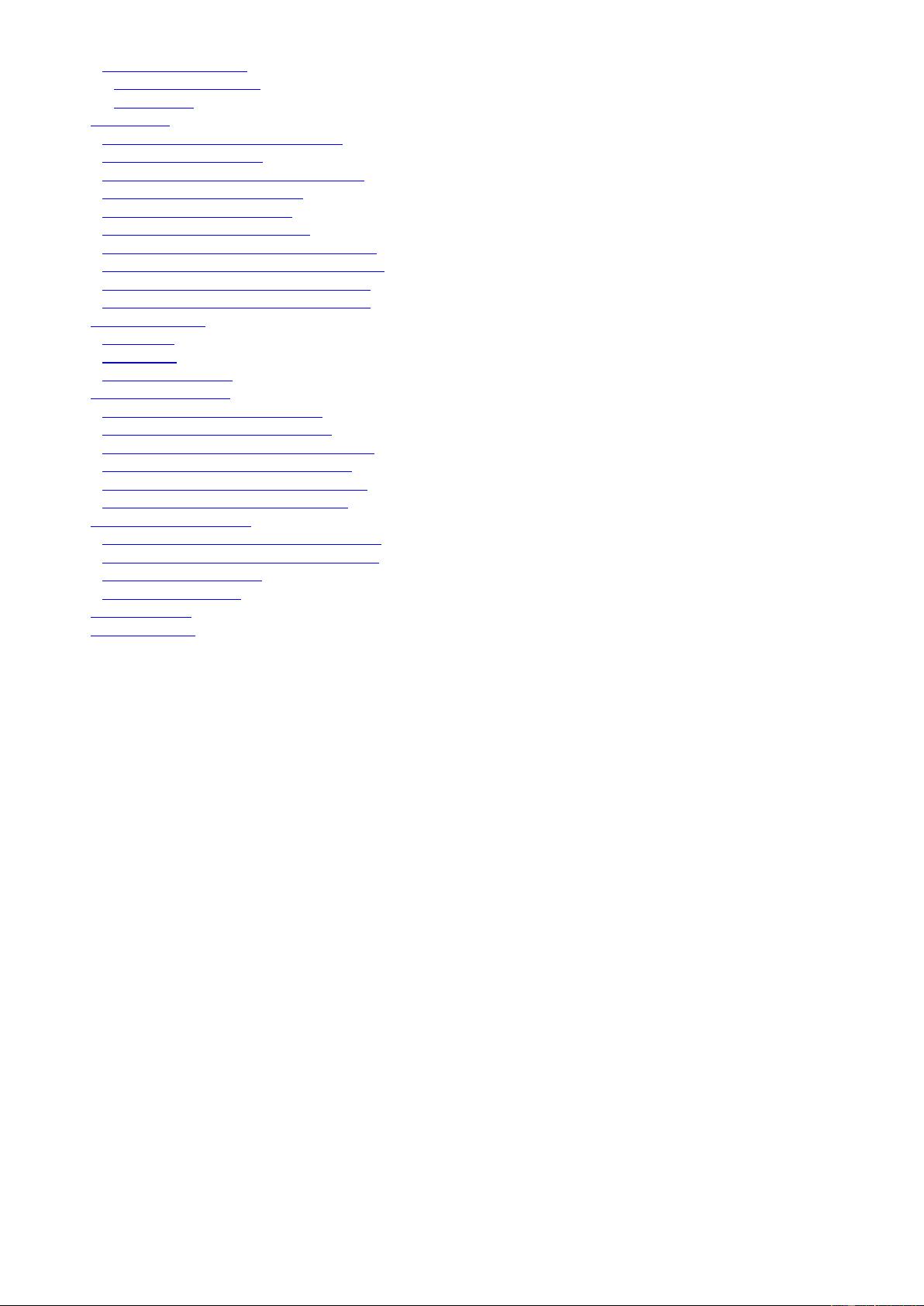
5
11.4 Security Plug-ins ............................................................................................................................................. 188
11.4.1 Spring Security .......................................................................................................................................... 188
11.4.2 Shiro ........................................................................................................................................................... 188
12. Plug-ins ................................................................................................................................................................. 190
12.1 Creating and Installing Plug-ins ...................................................................................................................... 190
12.2 Plugin Repositories .......................................................................................................................................... 191
12.3 Understanding a Plug-ins Structure ................................................................................................................. 193
12.4 Providing Basic Artefacts ................................................................................................................................ 194
12.5 Evaluating Conventions ................................................................................................................................... 195
12.6 Hooking into Build Events .............................................................................................................................. 196
12.7 Hooking into Runtime Configuration .............................................................................................................. 196
12.8 Adding Dynamic Methods at Runtime ............................................................................................................ 197
12.9 Participating in Auto Reload Events ............................................................................................................... 198
12.10 Understanding Plug-in Load Order ............................................................................................................... 200
13. Web Services ........................................................................................................................................................ 202
13.1 REST ............................................................................................................................................................... 202
13.2 SOAP ............................................................................................................................................................... 204
13.3 RSS and Atom ................................................................................................................................................. 204
14. Grails and Spring .................................................................................................................................................. 206
14.1 The Underpinnings of Grails ........................................................................................................................... 206
14.2 Configuring Additional Beans ......................................................................................................................... 206
14.3 Runtime Spring with the Beans DSL .............................................................................................................. 208
14.4 The BeanBuilder DSL Explained .................................................................................................................... 210
14.5 Property Placeholder Configuration ................................................................................................................ 215
14.6 Property Override Configuration ..................................................................................................................... 215
15. Grails and Hibernate ............................................................................................................................................. 216
15.1 Using Hibernate XML Mapping Files ............................................................................................................. 216
15.2 Mapping with Hibernate Annotations ............................................................................................................. 216
15.3 Adding Constraints .......................................................................................................................................... 217
15.4 Further Reading ............................................................................................................................................... 217
16. Scaffolding ........................................................................................................................................................... 219
17. Deployment .......................................................................................................................................................... 222
评论1