<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN">
<!-- saved from url=(0074)http://home.student.utwente.nl/s.p.ekkebus/portfolio/resource/sms_pdu.html -->
<HTML><HEAD><TITLE>SMS and PDU format</TITLE>
<META http-equiv=Content-Type content="text/html; charset=windows-1252">
<SCRIPT language=javascript>
/* Script written by Swen-Peter Ekkebus, edited by Ing. Milan Chudik.
*
* Further fixes and functionality by Andrew Alexander
*
* ekkebus[at]cs.utwente.nl
* Feel free to use it, please don't forget to link to the source ;)
*
* Version 1.5 r2aja
*
* Official BPS develop tool
*
* (c) BPS & co, 2003
*/
//Array with "The 7 bit defaultalphabet"
sevenbitdefault = new Array('@', '£', '$', '¥', 'è', 'é', 'ù', 'ì', 'ò', 'Ç', '\n', 'Ø', 'ø', '\r','Å', 'å','\u0394', '_', '\u03a6', '\u0393', '\u039b', '\u03a9', '\u03a0','\u03a8', '\u03a3', '\u0398', '\u039e','', 'Æ', 'æ', 'ß', 'É', ' ', '!', '"', '#', '¤', '%', '&', '\'', '(', ')','*', '+', ',', '-', '.', '/', '0', '1', '2', '3', '4', '5', '6', '7','8', '9', ':', ';', '<', '=', '>', '?', '¡', 'A', 'B', 'C', 'D', 'E','F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S','T', 'U', 'V', 'W', 'X', 'Y', 'Z', 'Ä', 'Ö', 'Ñ', 'Ü', '§', '¿', 'a','b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o','p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'ä', 'ö', 'ñ','ü', 'à');
// Variable that stores the information to show the user the calculation of the translation
var calculation = "";
maxkeys = 160;
alerted = false;
// function te convert a bit string into a integer
function binToInt(x)//sp
{
var total = 0;
var power = parseInt(x.length)-1;
for(var i=0;i<x.length;i++)
{
if(x.charAt(i) == '1')
{
total = total +Math.pow(2,power);
}
power --;
}
return total;
}
// function to convert a integer into a bit String
function intToBin(x,size) //sp
{
var base = 2;
var num = parseInt(x);
var bin = num.toString(base);
for(var i=bin.length;i<size;i++)
{
bin = "0" + bin;
}
return bin;
}
// function to convert a Hexnumber into a 10base number
function HexToNum(numberS)
{
var tens = MakeNum(numberS.substring(0,1));
var ones = 0;
if(numberS.length > 1) // means two characters entered
ones=MakeNum(numberS.substring(1,2));
if(ones == 'X')
{
return "00";
}
return (tens * 16) + (ones * 1);
}
// helper function for HexToNum
function MakeNum(str)
{
if((str >= 0) && (str <= 9))
return str;
switch(str.toUpperCase())
{
case "A": return 10;
case "B": return 11;
case "C": return 12;
case "D": return 13;
case "E": return 14;
case "F": return 15;
default: alert('Only insert Hex values!!');
return 'X';
}
}
//function to convert integer to Hex
function intToHex(i) //sp
{
var sHex = "0123456789ABCDEF";
h = "";
i = parseInt(i);
for(j = 0; j <= 3; j++)
{
h += sHex.charAt((i >> (j * 8 + 4)) & 0x0F) +
sHex.charAt((i >> (j * 8)) & 0x0F);
}
return h.substring(0,2);
}
function ToHex(i)
{
var sHex = "0123456789ABCDEF";
var Out = "";
Out = sHex.charAt(i&0xf);
i>>=4;
Out = sHex.charAt(i&0xf) + Out;
return Out;
}
function getSevenBit(character) //sp
{
for(var i=0;i<sevenbitdefault.length;i++)
{
if(sevenbitdefault[i] == character)
{
return i;
}
}
alert("No 7 bit char!");
return 0;
}
function getEightBit(character)
{
return character;
}
function get16Bit(character)
{
return character;
}
// function to convert semioctets to a string
function semiOctetToString(inp) //sp
{
var out = "";
for(var i=0;i<inp.length;i=i+2)
{
var temp = inp.substring(i,i+2);
out = out + temp.charAt(1) + temp.charAt(0);
}
return out;
}
//Main function to translate the input to a "human readable" string
function getUserMessage(input,truelength) // Add truelength AJA
{
var byteString = "";
octetArray = new Array();
restArray = new Array();
septetsArray = new Array();
var s=1;
var count = 0;
var matchcount = 0; // AJA
var smsMessage = "";
var calculation1 = "<table border=1 ><tr><td align=center width=75><b>Hex</b></td>";
var calculation2 = "<tr><td align=center width=75> <b> Octets </b></td>";
var calculation3 = "<table border=1 ><tr><td align=center width=75><b>septets</b></td>";
var calculation4 = "<tr><td align=center width=75><b>Character</b></td>";
calculation = "";
//Cut the input string into pieces of2 (just get the hex octets)
for(var i=0;i<input.length;i=i+2)
{
var hex = input.substring(i,i+2);
byteString = byteString + intToBin(HexToNum(hex),8);
if((i%14 == 0 && i!=0))
{
calculation1 = calculation1 + "<td align=center width=75>+++++++</td>";
}
calculation1 = calculation1 + "<td align=center width=75>" + hex + "</td>";
}
calculation1 = calculation1 + "<td align=center width=75>+++++++</td>";
// make two array's these are nessesery to
for(var i=0;i<byteString.length;i=i+8)
{
octetArray[count] = byteString.substring(i,i+8);
restArray[count] = octetArray[count].substring(0,(s%8));
septetsArray[count] = octetArray[count].substring((s%8),8);
if((i%56 == 0 && i!=0))
{
calculation2 = calculation2 + "<td align=center width=75> </td>";
}
calculation2 = calculation2 + "<td align=center width=75><span style='background-color: #FFFF00'>" + restArray[count] + "</span>"+ septetsArray[count]+"</td>";
s++;
count++;
if(s == 8)
{
s = 1;
}
}
calculation2 = calculation2 + "<td align=center width=75> </td>";
// put the right parts of the array's together to make the sectets
for(var i=0;i<restArray.length;i++)
{
if(i%7 == 0)
{
if(i != 0)
{
smsMessage = smsMessage + sevenbitdefault[binToInt(restArray[i-1])];
calculation3 = calculation3 + "<td align=center width=75><span style='background-color: #FFFF00'> " + restArray[i-1] + "</span> </td>";
calculation4 = calculation4 + "<td align=center width=75> " + sevenbitdefault[binToInt(restArray[i-1])] + " </td>";
matchcount ++; // AJA
}
smsMessage = smsMessage + sevenbitdefault[binToInt(septetsArray[i])];
calculation3 = calculation3 + "<td align=center width=75> " +septetsArray[i] + " </td>";
calculation4 = calculation4 + "<td align=center width=75> " + sevenbitdefault[binToInt(septetsArray[i])] + " </td>";
matchcount ++; // AJA
}
else
{
smsMessage = smsMessage + sevenbitdefault[binToInt(septetsArray[i]+restArray[i-1])];
calculation3 = calculation3 + "<td align=center width=75> " +septetsArray[i]+ "<span style='background-color: #FFFF00'>" +restArray[i-1] + " </span>" + "</td>"
calculation4 = calculation4 + "<td align=center width=75> " + sevenbitdefault[binToInt(septetsArray[i]+restArray[i-1])] + " </td>";
matchcount ++; // AJA
}
}
if(matchcount != truelength) // Don't forget trailing characters!! AJA
{
smsMessage = smsMessage + sevenbitdefault[binToInt(restArray[i-1])];
calculation3 = calculation3 + "<td align=center width=75><span style='background-color: #FFFF00'> " + restArray[i-1] + "</span> </td>";
calculation4 = calculation4 + "<td align=center width=75> " + sevenbitdefault[binToInt(restArray[i-1])] + " </td>";
}
else // Blank Filler
{
calculation3 = calculation3 + "<td align=center width=75>+++++++</td>";
calculation4 = calculation4 + "<td align=center width=75> </td>";
}
//Put all the calculation info together
calculation = "Conversion of 8-bit octets to 7-bit default alphabet<br><br>"+calculation1 + "</tr>" + calculation2 + "</tr></table>" + calculation3 + "</tr>"+ calculation4 + "</tr></table>";
return smsMessage;
}
function getUserMessage16(input,truelength)
{
var sms
没有合适的资源?快使用搜索试试~ 我知道了~
PDU Converter(SMS)
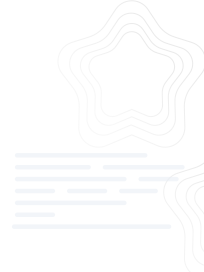
共15个文件
gif:3个
pas:2个
cfg:1个

需积分: 50 112 下载量 86 浏览量
2006-02-23
09:05:59
上传
评论
收藏 336KB RAR 举报
温馨提示
PDU string analyser with sourcecode(algorithm)PDU = Protocol Discription Unit;Some documentation to verify;
资源详情
资源评论
资源推荐
收起资源包目录

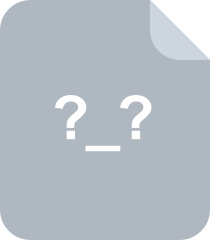

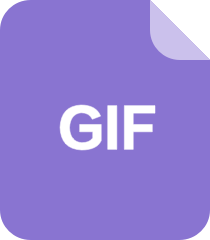
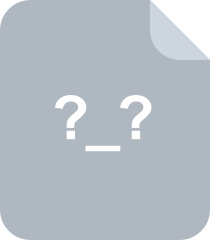
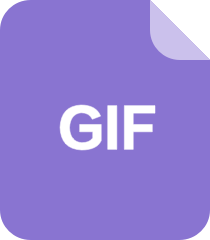
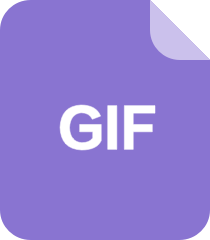
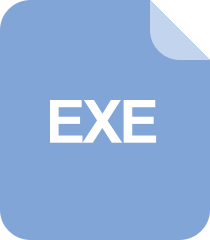
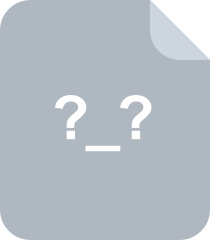
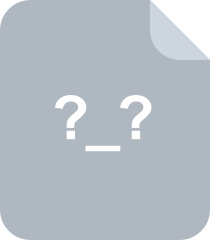
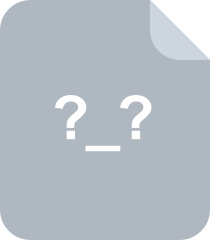
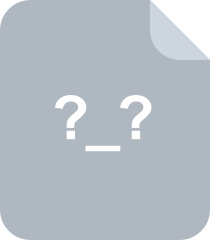
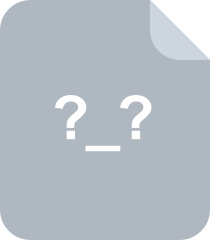
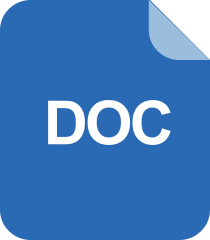
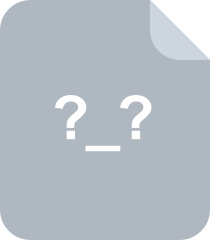
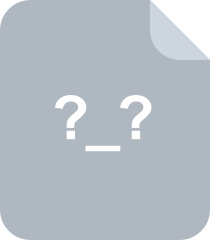
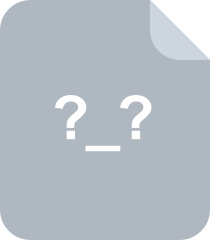
共 15 条
- 1
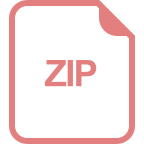
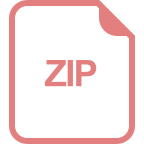
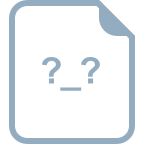
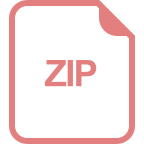
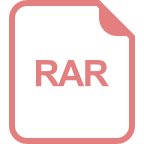
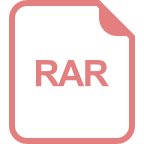
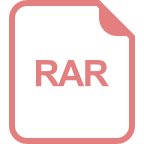
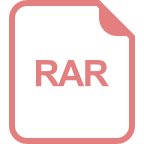
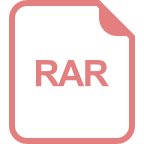
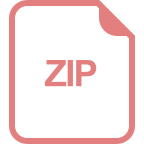
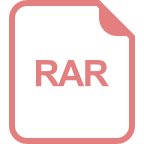
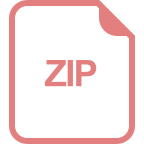
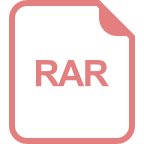
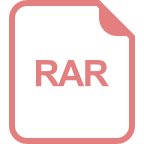
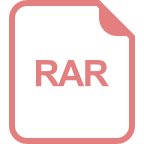
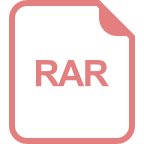
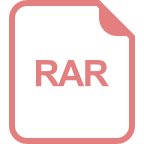
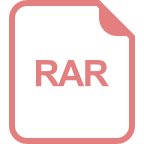
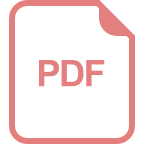
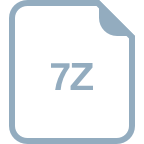
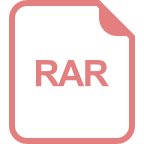
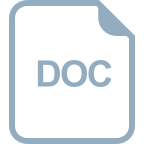
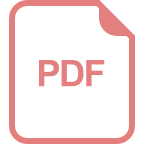
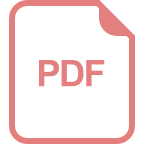
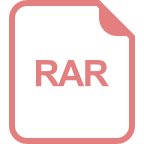

普通网友
- 粉丝: 882
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

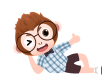
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


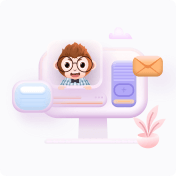
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0