 
[](https://github.com/avelino/awesome-go#machine-learning) [](https://godoc.org/github.com/owulveryck/onnx-go) [](https://goreportcard.com/report/github.com/owulveryck/onnx-go)
[](https://travis-ci.com/owulveryck/onnx-go)
[](https://codecov.io/gh/owulveryck/onnx-go)
This is a Go Interface to [Open Neural Network Exchange (ONNX)](https://onnx.ai/).
## Overview
onnx-go contains primitives to decode a onnx binary model into a computation backend, and use it like any other library in your go code.
for more information about onnx, please visit [onnx.ai](https://onnx.ai).
The implementation of the [the spec of ONNX](https://github.com/onnx/onnx/blob/master/docs/IR.md) is partial on the import, and non-existent for the export.
### Vision statement
> For the Go developer who needs to add a machine learning capability to his/her code,
> onnx-go is a package that facilitates the use of neural network models (software 2.0)
> and unlike any other computation library, this package does not require special skills in data-science.
**Warning** The API is experimental and may change.
### Disclaimer
[embedmd]:# (RELNOTES.md)
```md
This is a new version of the API.
The tweaked version of Gorgonia have been removed. It is now compatible with the master branch of Gorgonia.
Some operators are not yet available though.
A utility has been added in order to run models from the zoo.
check the `examples` subdirectory.
```
## Install
Install it via go get
```
go get github.com/owulveryck/onnx-go
```
onnx-go is compatible with [go modules](https://github.com/golang/go/wiki/Modules).
## Example
Those examples assumes that you have a pre-trained `model.onnx` file available.
You can download pre-trained modles from the [onnx model zoo](https://github.com/onnx/models).
### Very simple example
This example does nothing but decoding the graph into a simple backend.
Then you can do whatever you want with the generated graph.
[embedmd]:# (example_test.go /\/\/ Create/ /model.UnmarshalBinary.*/)
```go
// Create a backend receiver
backend := simple.NewSimpleGraph()
// Create a model and set the execution backend
model := onnx.NewModel(backend)
// read the onnx model
b, _ := ioutil.ReadFile("model.onnx")
// Decode it into the model
err := model.UnmarshalBinary(b)
```
### Simple example to run a pre-trained model
This example uses [Gorgonia](https://github.com/gorgonia/gorgonia) as a backend.
```go
import "github.com/owulveryck/onnx-go/backend/x/gorgonnx"
```
At the present time, Gorgonia does not implement all the operators of ONNX. Therefore, most of the model from the model zoo will not work.
Things will go better little by little by adding more operators to the backend.
You can find a list of tested examples and a coverage [here](https://github.com/owulveryck/onnx-go/blob/master/backend/x/gorgonnx/ONNX_COVERAGE.md).
[embedmd]:# (example_gorgonnx_test.go /func Ex/ /^}/)
```go
func Example_gorgonia() {
// Create a backend receiver
backend := gorgonnx.NewGraph()
// Create a model and set the execution backend
model := onnx.NewModel(backend)
// read the onnx model
b, _ := ioutil.ReadFile("model.onnx")
// Decode it into the model
err := model.UnmarshalBinary(b)
if err != nil {
log.Fatal(err)
}
// Set the first input, the number depends of the model
model.SetInput(0, input)
err = backend.Run()
if err != nil {
log.Fatal(err)
}
// Check error
output, _ := model.GetOutputTensors()
// write the first output to stdout
fmt.Println(output[0])
}
```
### Model zoo
In the `examples` subdirectory, you will find a utility to run a model from the zoo, as well as a sample utility to analyze a picture with [Tiny YOLO v2](https://pjreddie.com/darknet/yolov2/)
## Internal
### ONNX protobuf definition
The protobuf definition of onnx has is compiled into Go with the classic `protoc` tool. The definition can be found in the `internal` directory.
The definition is not exposed to avoid external dependencies to this repo. Indeed, the pb code can change to use a more efficient compiler such
as `gogo protobuf` and this change should be transparent to the user of this package.
### Execution backend
In order to execute the neural network, you need a backend able to execute a computation graph (_for more information on computation graphs, please read this [blog post](http://gopherdata.io/post/deeplearning_in_go_part_1/)_
This picture represents the mechanism:

onnx-go do not provide any executable backend, but for a reference, a simple backend that builds an information graph is provided as an example (see the `simple` subpackage).
Gorgonia is the main target backend of ONNX-Go.
#### Backend implementation
a backend is basically a Weighted directed graph that can apply on Operation on its nodes. It should fulfill this interface:
[embedmd]:# (backend.go /type Backend/ /}/)
```go
type Backend interface {
OperationCarrier
graph.DirectedWeightedBuilder
}
```
[embedmd]:# (backend.go /type OperationCarrier/ /}/)
```go
type OperationCarrier interface {
// ApplyOperation on the graph nodes
// graph.Node is an array because it allows to handle multiple output
// for example a split operation returns n nodes...
ApplyOperation(Operation, ...graph.Node) error
}
```
An Operation is represented by its `name` and a map of attributes. For example the Convolution operator as described in the [spec of onnx](https://github.com/onnx/onnx/blob/master/docs/Operators.md#Conv) will be represented like this:
[embedmd]:# (conv_example_test.go /convOperator/ /}$/)
```go
convOperator := Operation{
Name: "Conv",
Attributes: map[string]interface{}{
"auto_pad": "NOTSET",
"dilations": []int64{1, 1},
"group": 1,
"pads": []int64{1, 1},
"strides": []int64{1, 1},
},
}
```
Besides, operators, a node can carry a value. Values are described as [`tensor.Tensor`](https://godoc.org/gorgonia.org/tensor#Tensor)
To carry data, a *`Node`* of the graph should fulfill this interface:
[embedmd]:# (node.go /type DataCarrier/ /}/)
```go
type DataCarrier interface {
SetTensor(t tensor.Tensor) error
GetTensor() tensor.Tensor
}
```
#### Backend testing
onnx-go provides a some utilities to test a backend. Visit the [`testbackend` package](backend/testbackend) for more info.
## Contributing
Contributions are welcome. A contribution guide will be eventually written. Meanwhile, you can raise an issue or send a PR.
You can also contact me via Twitter or on the gophers' slack (I am @owulveryck on both)
This project is intended to be a safe, welcoming space for collaboration, and
contributors are expected to adhere to the [Contributor Covenant](http://contributor-covenant.org) code of conduct.
## Author
[Olivier Wulveryck](https://about.me/owulveryck/getstarted)
## License
MIT.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
This is a Go Interface to Open Neural Network Exchange (ONNX). 这是开放神经网络交换 (ONNX) 的 Go 接口。 onnx-go contains primitives to decode a onnx binary model into a computation backend, and use it like any other library in your go code. for more information about onnx, please visit onnx.ai. onnx-go 包含用于将 ONNX 二进制模型解码为计算后端的基元,并像 Go 代码中的任何其他库一样使用它。有关 ONNX 的更多信息,请访问 onnx.ai。 The implementation of the the spec of ONNX is partial on the import, and non-existent for the export. ONNX 规范的实现在导入时是部分的,而对于导出则不存在。
资源推荐
资源详情
资源评论
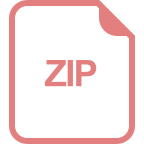
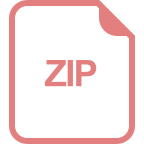
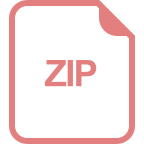
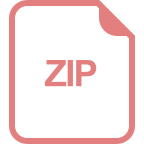
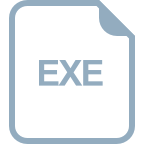
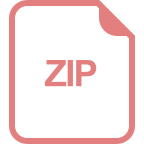
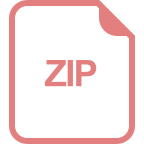
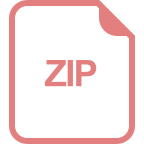
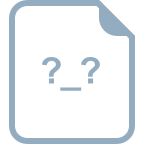
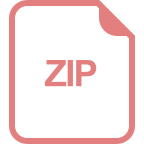
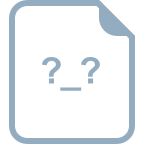
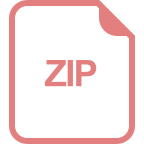
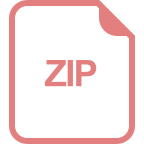
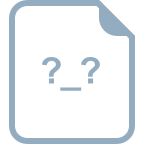
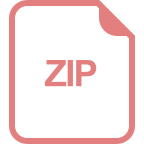
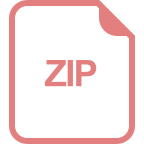
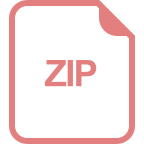
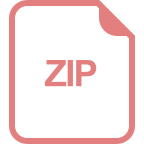
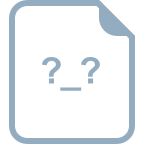
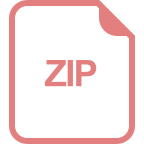
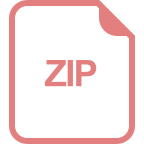
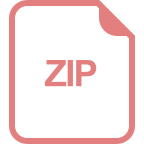
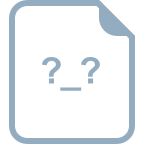
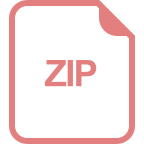
收起资源包目录

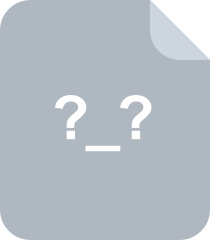
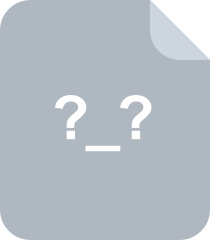
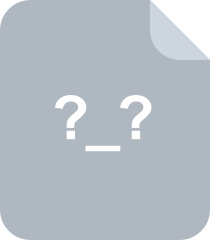
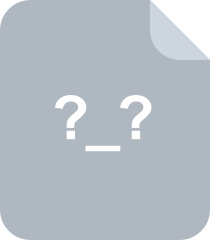
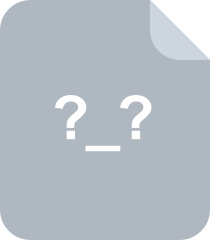
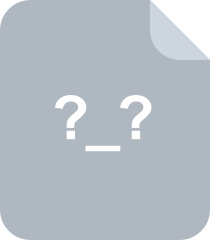
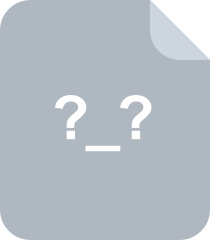
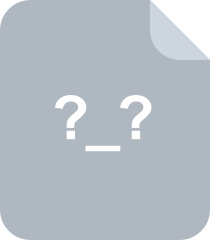
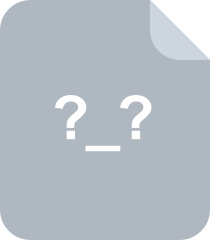
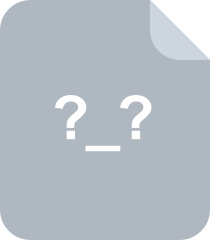
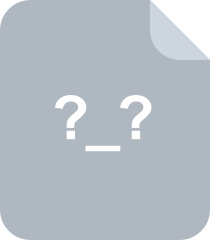
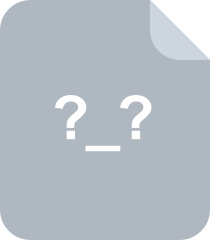
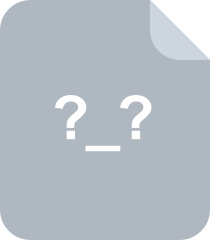
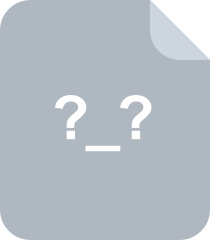
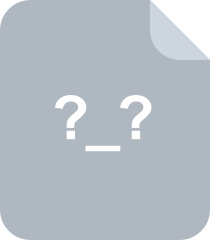
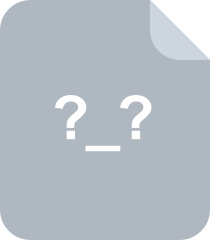
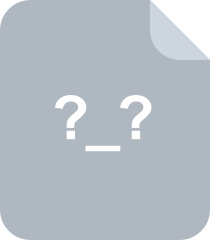
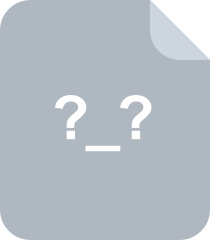
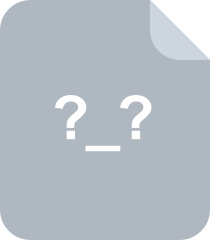
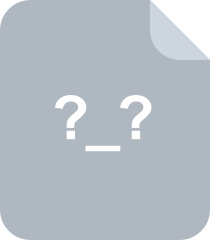
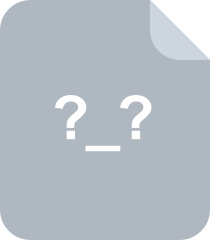
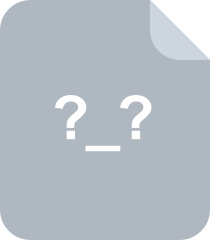
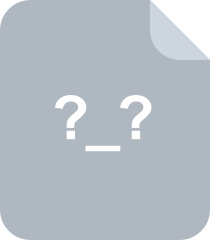
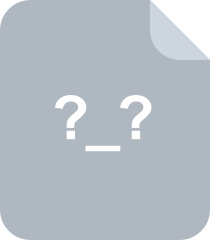
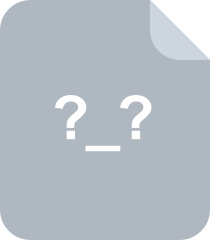
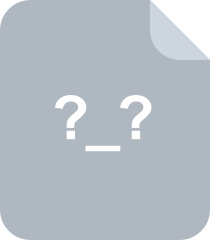
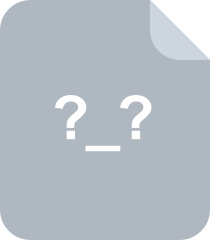
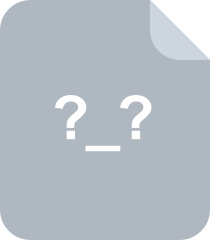
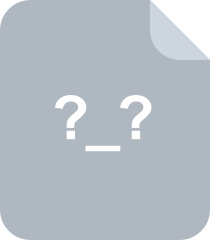
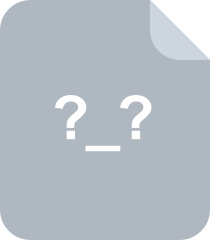
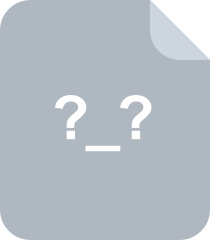
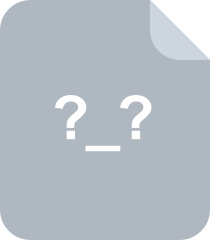
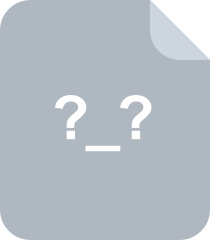
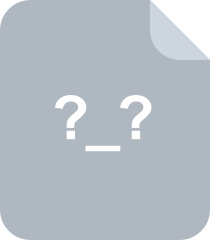
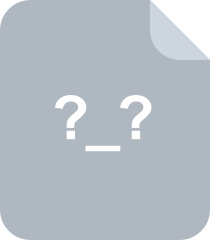
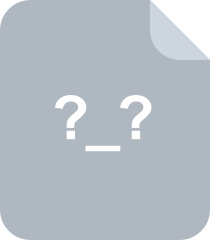
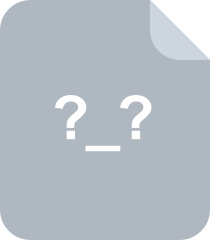
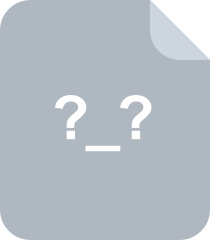
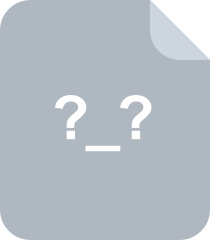
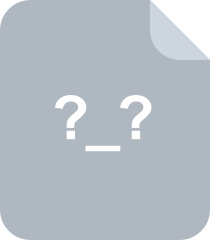
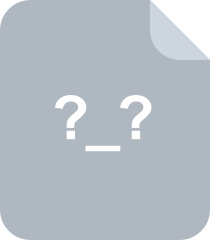
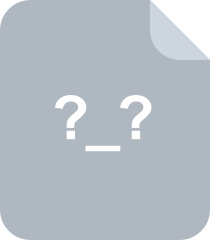
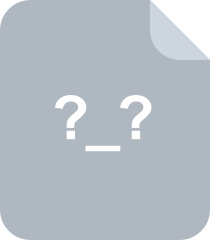
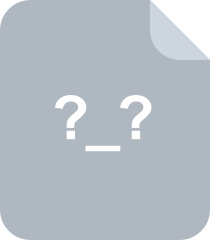
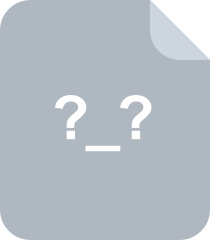
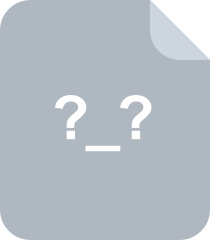
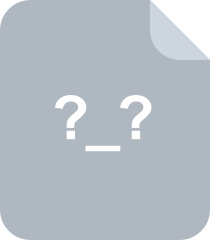
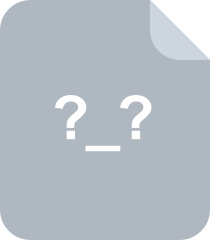
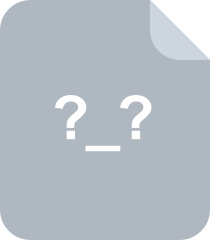
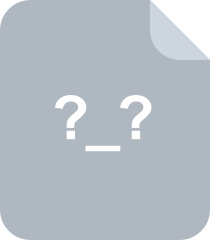
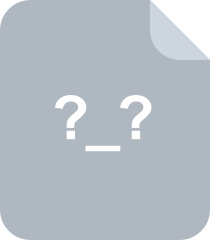
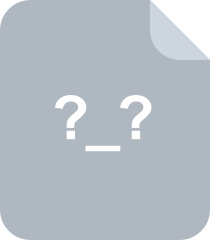
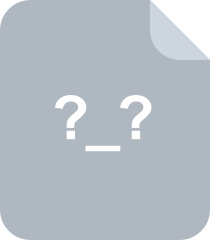
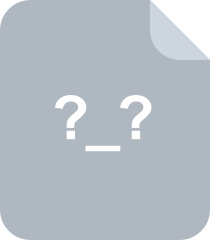
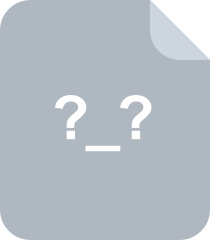
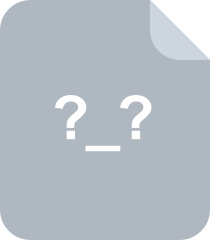
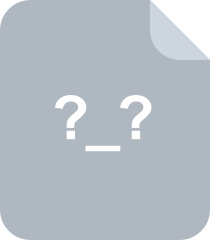
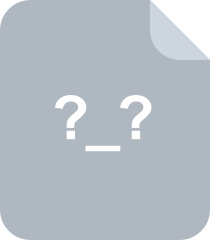
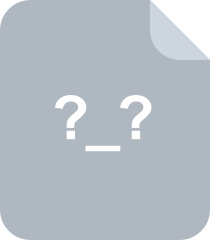
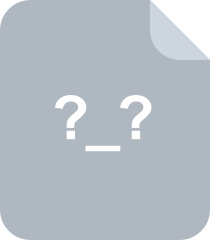
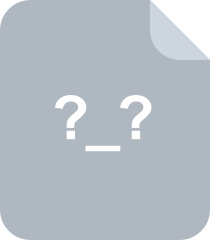
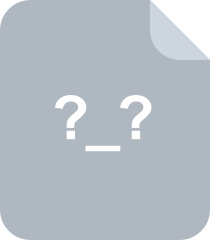
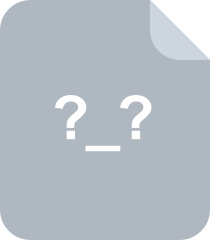
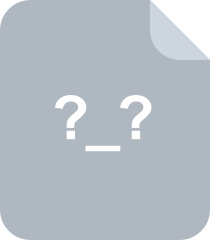
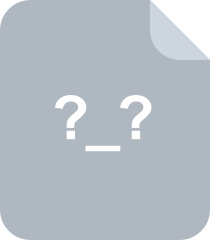
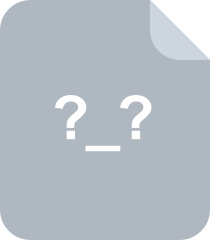
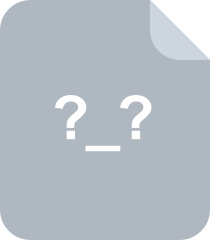
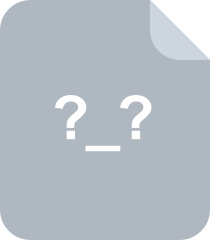
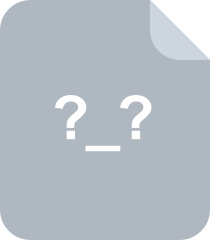
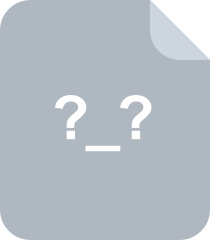
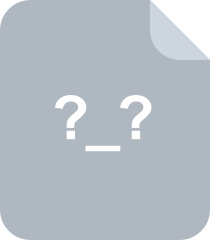
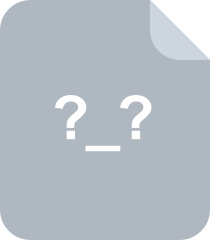
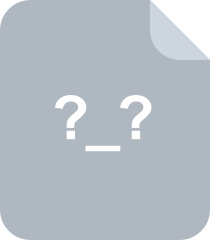
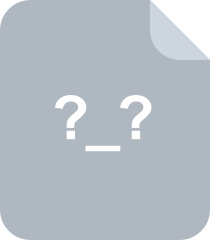
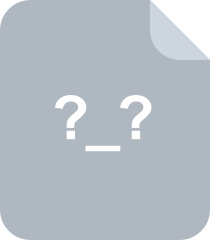
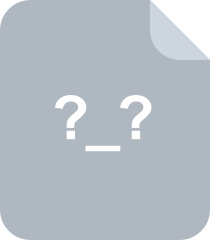
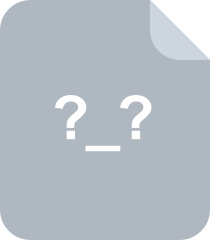
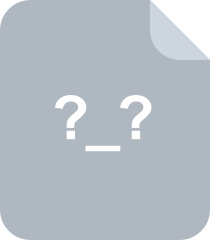
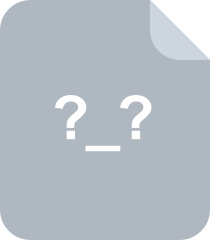
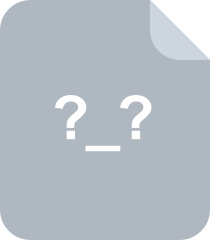
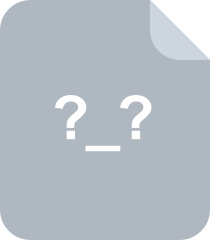
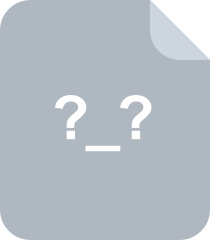
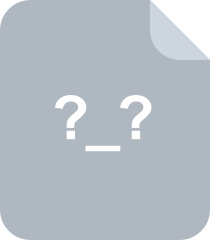
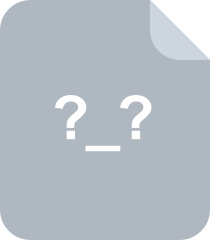
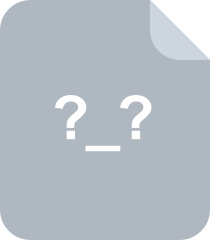
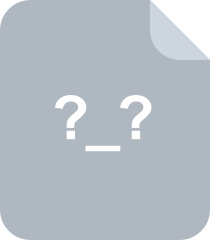
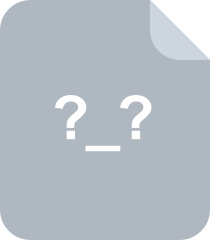
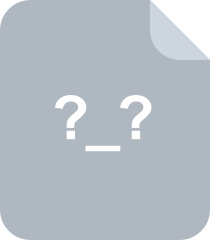
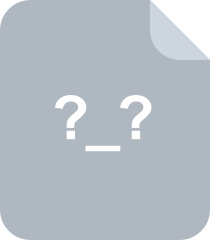
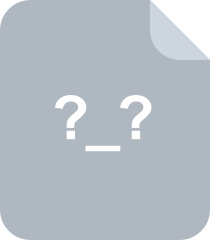
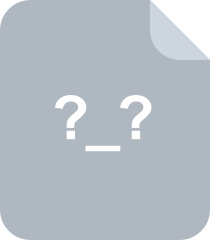
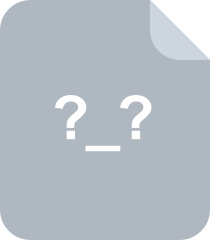
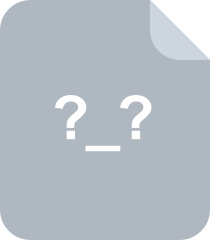
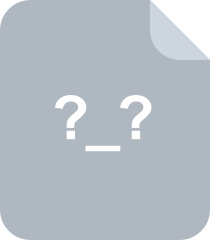
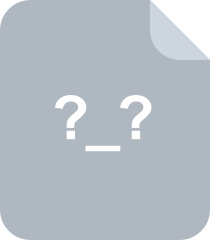
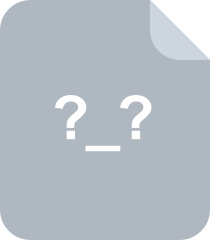
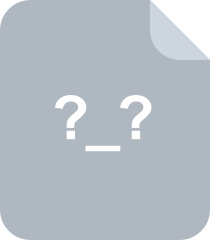
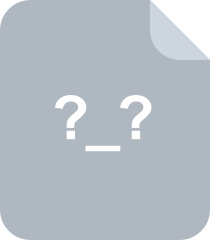
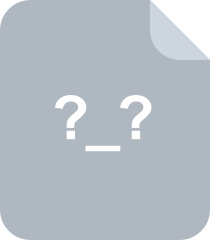
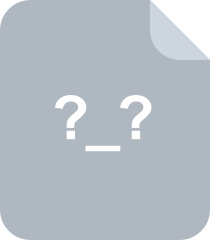
共 928 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
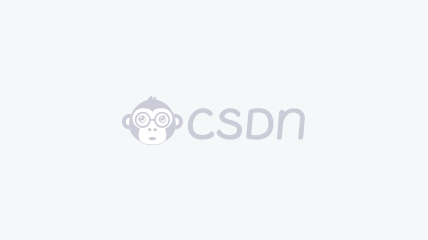

云上翔
- 粉丝: 222
- 资源: 13
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

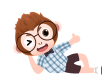
最新资源
- 毕业设计-基于python实现的爬取携程景点数据和评论数据源代码+文档说明
- 微网优化调度 机组组合 主题:基于YALMIP 的微网优化调度模型 内容简介:程序基于MATLAB yalmip 开发,做了一个简单的微网优化调度模型,模型中含有蓄电池储能、风电、光伏等发电单元,程
- DEEP LEARNING:A Comprehensive Guide.pdf
- 毕业设计基于python实现的爬取携程景点数据和评论数据源代码+文档说明
- 微网孤岛优化调度 matlab 编程语言:matlab 内容摘要:采用灰狼算法实现微网孤岛优化调度,考虑风光、微燃机、燃料电池和蓄电池等主体,考虑价格型和激励型需求响应,以经济成本和环境治理成本为目标
- FactoryIO堆垛机仿真 使用简单的梯形图与SCL语言编写,通俗易懂,写有详细注释,起到抛砖引玉的作用,比较适合有动手能力的入门初学者 软件环境: 1、西门子编程软件:TIA Portal V1
- Comsol激光仿真通孔,利用高斯热源脉冲激光对材料进行蚀除过程仿真,其中运用了变形几何和固体传热实现单脉冲通孔的加工
- 毕业设计Python+Django音乐推荐系统源码+文档说明(高分毕设)
- glibC自动升级脚本
- C语言编写一个简单的俄罗斯方块游戏.docx
- 3b083教师工作量计算系统_springboot+vue.zip
- 3b081火车订票系统_springboot+vue.zip
- 3b082健身房管理系统_springboot+vue.zip
- C#与松下PLC串口 以太网通讯,自己写的,注释包含了自己理解和整理的资料,公司项目中使用,通讯用的PLC型号为FP-XH C60ET,文件包含:dll封装,测试程序,通讯文档 有代码注释
- python求链表长度的递归方法
- 3b084教师考勤系统_springboot+vue0.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


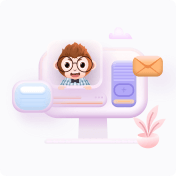
安全验证
文档复制为VIP权益,开通VIP直接复制
