<?php
/**
* additional_images module
*
* Prepares list of additional product images to be displayed in template
*
* @package templateSystem
* @copyright Copyright 2003-2007 Zen Cart Development Team
* @copyright Portions Copyright 2003 osCommerce
* @license http://www.zen-cart.com/license/2_0.txt GNU Public License V2.0
* @version $Id: additional_images.php 6132 2007-04-08 06:58:40Z drbyte $
*/
if (!defined('IS_ADMIN_FLAG')) {
die('Illegal Access');
}
if (!defined('IMAGE_ADDITIONAL_DISPLAY_LINK_EVEN_WHEN_NO_LARGE')) define('IMAGE_ADDITIONAL_DISPLAY_LINK_EVEN_WHEN_NO_LARGE','Yes');
$images_array = array();
if ($products_image != '') {
// prepare image name
$products_image_extension = substr($products_image, strrpos($products_image, '.'));
$products_image_base = str_replace($products_image_extension, '', $products_image);
// if in a subdirectory
if (strrpos($products_image, '/')) {
$products_image_match = substr($products_image, strrpos($products_image, '/')+1);
//echo 'TEST 1: I match ' . $products_image_match . ' - ' . $file . ' - base ' . $products_image_base . '<br>';
$products_image_match = str_replace($products_image_extension, '', $products_image_match) . '_';
$products_image_base = $products_image_match;
}
$products_image_directory = str_replace($products_image, '', substr($products_image, strrpos($products_image, '/')));
if ($products_image_directory != '') {
$products_image_directory = DIR_WS_IMAGES . str_replace($products_image_directory, '', $products_image) . "/";
} else {
$products_image_directory = DIR_WS_IMAGES;
}
// Check for additional matching images
$file_extension = $products_image_extension;
$products_image_match_array = array();
if ($dir = @dir($products_image_directory)) {
while ($file = $dir->read()) {
if (!is_dir($products_image_directory . $file)) {
if (substr($file, strrpos($file, '.')) == $file_extension) {
// if(preg_match("/" . $products_image_match . "/i", $file) == '1') {
if(preg_match("/" . $products_image_base . "/i", $file) == 1) {
if ($file != $products_image) {
if ($products_image_base . str_replace($products_image_base, '', $file) == $file) {
// echo 'I AM A MATCH ' . $file . '<br>';
$images_array[] = $file;
} else {
// echo 'I AM NOT A MATCH ' . $file . '<br>';
}
}
}
}
}
}
if (sizeof($images_array)) {
sort($images_array);
}
$dir->close();
}
}
// Build output based on images found
$num_images = sizeof($images_array);
$list_box_contents = '';
$title = '';
if ($num_images) {
$row = 0;
$col = 0;
if ($num_images < IMAGES_AUTO_ADDED || IMAGES_AUTO_ADDED == 0 ) {
$col_width = floor(100/$num_images);
} else {
$col_width = floor(100/IMAGES_AUTO_ADDED);
}
for ($i=0, $n=$num_images; $i<$n; $i++) {
$file = $images_array[$i];
$products_image_large = str_replace(DIR_WS_IMAGES, DIR_WS_IMAGES . 'large/', $products_image_directory) . str_replace($products_image_extension, '', $file) . IMAGE_SUFFIX_LARGE . $products_image_extension;
// bof Zen Lightbox 2008-12-11 aclarke
if (function_exists('handle_image')) {
$flag_has_large = true;
} else {
// eof Zen Lightbox 2008-12-11 aclarke
$flag_has_large = file_exists($products_image_large);
// bof Zen Lightbox 2008-12-11 aclarke
}
// eof Zen Lightbox 2008-12-11 aclarke
$products_image_large = ($flag_has_large ? $products_image_large : $products_image_directory . $file);
$flag_display_large = (IMAGE_ADDITIONAL_DISPLAY_LINK_EVEN_WHEN_NO_LARGE == 'Yes' || $flag_has_large);
$base_image = $products_image_directory . $file;
$thumb_slashes = zen_image($base_image, addslashes($products_name), SMALL_IMAGE_WIDTH, SMALL_IMAGE_HEIGHT);
// bof Zen Lightbox 2008-12-11 aclarke
if (function_exists('handle_image')) {
// remove additional single quotes from image attributes (important!)
$thumb_slashes = preg_replace("/([^\\\\])'/", '$1\\\'', $thumb_slashes);
}
// eof Zen Lightbox 2008-12-11 aclarke
$thumb_regular = zen_image($base_image, $products_name, SMALL_IMAGE_WIDTH, SMALL_IMAGE_HEIGHT);
$large_link = zen_href_link(FILENAME_POPUP_IMAGE_ADDITIONAL, 'pID=' . $_GET['products_id'] . '&pic=' . $i . '&products_image_large_additional=' . $products_image_large);
// Link Preparation:
// bof Zen Lightbox 2008-12-11 aclarke
if (ZEN_LIGHTBOX_STATUS == 'true') {
if (ZEN_LIGHTBOX_GALLERY_MODE == 'true') {
$rel = 'lightbox-g';
} else {
$rel = 'lightbox';
}
$script_link = '<script language="javascript" type="text/javascript"><!--' . "\n" . 'document.write(\'' . ($flag_display_large ? '<a href="' . zen_lightbox($products_image_large, addslashes($products_name), LARGE_IMAGE_WIDTH, LARGE_IMAGE_HEIGHT) . '" rel="' . $rel . '" title="' . addslashes($products_name) . '">' . $thumb_slashes . '<br />' . TEXT_CLICK_TO_ENLARGE . '</a>' : $thumb_slashes) . '\');' . "\n" . '//--></script>';
} else {
// eof Zen Lightbox 2008-12-11 aclarke
$script_link = '<script language="javascript" type="text/javascript"><!--' . "\n" . 'document.write(\'' . ($flag_display_large ? '<a href="javascript:popupWindow(\\\'' . $large_link . '\\\')">' . $thumb_slashes . '<br />' . TEXT_CLICK_TO_ENLARGE . '</a>' : $thumb_slashes) . '\');' . "\n" . '//--></script>';
// bof Zen Lightbox 2008-12-11 aclarke
}
// eof Zen Lightbox 2008-12-11 aclarke
$noscript_link = '<noscript>' . ($flag_display_large ? '<a href="' . zen_href_link(FILENAME_POPUP_IMAGE_ADDITIONAL, 'pID=' . $_GET['products_id'] . '&pic=' . $i . '&products_image_large_additional=' . $products_image_large) . '" target="_blank">' . $thumb_regular . '<br /><span class="imgLinkAdditional">' . TEXT_CLICK_TO_ENLARGE . '</span></a>' : $thumb_regular ) . '</noscript>';
// $alternate_link = '<a href="' . $products_image_large . '" onclick="javascript:popupWindow(\''. $large_link . '\') return false;" title="' . $products_name . '" target="_blank">' . $thumb_regular . '<br />' . TEXT_CLICK_TO_ENLARGE . '</a>';
$link = $script_link . "\n " . $noscript_link;
// $link = $alternate_link;
// List Box array generation:
$list_box_contents[$row][$col] = array('params' => 'class="additionalImages centeredContent back"' . ' ' . 'style="width:' . $col_width . '%;"',
'text' => "\n " . $link);
$col ++;
if ($col > (IMAGES_AUTO_ADDED -1)) {
$col = 0;
$row ++;
}
} // end for loop
} // endif
?>

chenjie3392593
- 粉丝: 65
- 资源: 29
最新资源
- ros中用话题通信的方式实现Robotiq 300-S力矩传感器的数据传输(2024年12月17日)
- 恶劣天气下道路上的行人、车辆,图像目标检测数据【已标注,约1000张数据,YOLO 标注格式】
- Multisim 11.0安装包
- GPA案例介绍之计算耕地保有量
- 设计学长素材 (5).psd
- Python+Vue开发各类Web应用系统的源码推荐
- 开源OCR文字识别,身份证识别
- 160) Novo - 摄影 WordPress 主题 v4.2.7.zip
- 161) Ronneby - 高性能 WordPress 主题 v3.5.62.zip
- winformc#调用onnx模型,需自备:onnx,yaml,机器运行环境:cuda11.6,cudnn8.5.0,Microsoft.ML.OnnxRuntime.Gpu1.14.0
- 159) Mirasat - 互联网提供商与卫星电视 WordPress 主题 v1.1.0.zip
- 156) Basel - 响应式电子商务主题 v5.8.0.zip
- 158) Norebro - 多用途创意作品集主题 v1.6.4.zip
- 17类 MATLAB神经网络智能算法 源码 解决日常问题.zip 语音特征信号分类、语音特征信号分类、上证开盘指数预测、患者癌症发病预测、柴油机故障诊断、人脸朝向识别、短时交通流量预测
- 157) Oshine - 多用途创意主题 v7.1.6.zip
- 155) Gillion - 多概念博客 - 杂志与商店 WordPress 主题 v4.11.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


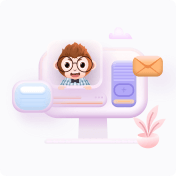