根据提供的文件信息,我们可以分析出该段代码主要涉及C#编程语言,并且是Windows Forms应用程序的一部分。下面将从几个方面来解析这段代码所涉及到的知识点。 ### 1. 命名空间(Namespaces) 在C#中,命名空间是用来组织类和其他类型的一种方式。在该段代码中,可以看到引入了多个命名空间: ```csharp using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; ``` 这些命名空间包含了创建Windows Forms应用程序所需的基本类库。例如`System.Windows.Forms`提供了用于创建GUI控件的类,如`Form`、`Button`等。 ### 2. 类定义(Class Definition) 代码中定义了一个名为`Form`的类,实际上这里可能是一个笔误,因为通常会自定义一个类名而不是使用`Form`。这个类继承自`System.Windows.Forms.Form`,这意味着它是一个窗口界面。 ```csharp public partial class Form { // ... } ``` ### 3. 构造函数(Constructor) 类中定义了一个构造函数,它接受两个参数:`string no` 和 `Boolean Exist`。这些参数分别表示某种编号和是否存在标志。 ```csharp public Form(string no, Boolean Exist) { InitializeComponent(); this.no = no; this.Exist = Exist; } ``` `InitializeComponent()`是一个由Visual Studio自动生成的方法,用于初始化窗体上的控件。 ### 4. 数据表操作(Data Table Operations) 代码中有两处涉及到了对数据表的操作,包括从数据库获取大类和小类的信息。 ```csharp private void GetTree() { DataTable BigClass = Program.DBOpertor.GetBigClass(); DataTable SmallClass; string SBigClass; int i, j; ProjectTreeView.BeginUpdate(); for (i = 0; i < BigClass.Rows.Count; i++) { SBigClass = BigClass.Rows[i]["ʳƷ"].ToString(); ProjectTreeView.Nodes.Add(SBigClass); SmallClass = Program.DBOpertor.GetSmallClass(SBigClass); for (j = 0; j < SmallClass.Rows.Count; j++) ProjectTreeView.Nodes[i].Nodes.Add(SmallClass.Rows[j]["Ŀ"].ToString() + "" + SmallClass.Rows[j][""].ToString()); } ProjectTreeView.EndUpdate(); } ``` 这里通过调用`DBOperatort.GetBigClass()`和`DBOperatort.GetSmallClass()`方法获取到数据表`BigClass`和`SmallClass`。然后使用`TreeNode`对象向`TreeView`控件添加节点。 ### 5. 窗体加载事件(Load Event) 当窗体加载时,触发`_Load`事件。在这个事件中填充数据表格并调用`GetTree()`方法。 ```csharp private void _Load(object sender, EventArgs e) { // TODO: 这里应该是填充数据集的操作 this.TableAdapter.Fill(this.dieteticManagementDataSet.); GetTree(); if (Exist) { // 根据存在标志加载数据 } } ``` `TableAdapter.Fill`方法用于从数据源填充数据集。 ### 6. 数据网格视图操作(DataGrid View Operations) 在`_Load`方法中还涉及到了`DataGridView`控件的使用,用于显示查询结果。 ```csharp string ColorClass; DataTable Dtable = Program.DBOpertor.Select("", Column, data).Tables[0]; for (int i = 0; i < Dtable.Rows.Count; i++) { // 填充DataGridView的行 sdata[0] = Dtable.Rows[i][""].ToString(); // ... dataGridView.Rows.Add(sdata); // 设置行的颜色 ColorClass = Dtable.Rows[i]["״̬"].ToString(); if (ColorClass == "Blue") dataGridView.Rows[i].DefaultCellStyle.ForeColor = Color.Blue; else if (ColorClass == "Red") dataGridView.Rows[i].DefaultCellStyle.ForeColor = Color.Red; else dataGridView.Rows[i].DefaultCellStyle.ForeColor = Color.Black; } ``` 这部分代码展示了如何根据查询结果填充`DataGridView`并设置行颜色。 ### 7. 事件处理(Event Handling) 我们看到了一个事件处理方法`BindingNavigatorSaveItem_Click`,但该方法的实现部分被截断了。 ```csharp private void BindingNavigatorSaveItem_Click(object sender, EventArgs e) { // ... } ``` 该方法应该是在用户点击保存按钮时触发,用于处理保存逻辑。 这段代码主要涉及到了Windows Forms应用程序的开发,包括控件的使用、数据表的操作以及事件处理等方面的知识点。
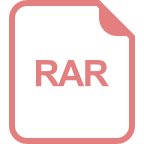
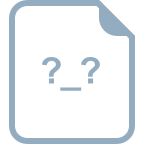
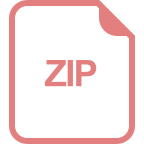
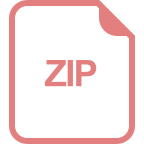
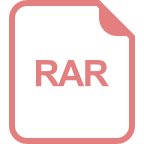
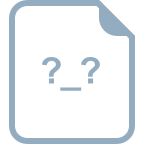
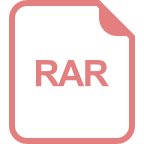
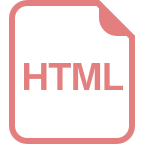
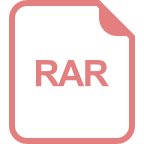
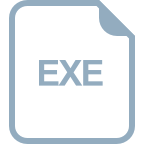
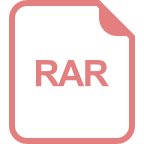
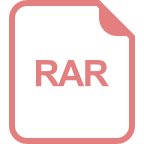
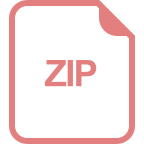
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace 餐饮管理系统
{
public partial class 增加消费 : Form
{
string CurrentAccount = Program.Admin;
float Discount = 1;
string no;
Boolean Exist;
public 增加消费(string no,Boolean Exist)
{
InitializeComponent();
this.no = no;
this.Exist = Exist;
}
private void GetTree()
{
DataTable BigClass = Program.DBOpertor.GetBigClass();
DataTable SmallClass;
string SBigClass;
int i,j;
for (i = 0; i < BigClass.Rows.Count; i++)
{
SBigClass=BigClass.Rows[i]["食品类别"].ToString();
ProjectTreeView.Nodes.Add(SBigClass);
SmallClass = Program.DBOpertor.GetSmallClass(SBigClass);
for (j = 0; j < SmallClass.Rows.Count; j++)
ProjectTreeView.Nodes[i].Nodes.Add(SmallClass.Rows[j]["项目名称"].ToString()+" ¥"+SmallClass.Rows[j]["单价"].ToString());
}
ProjectTreeView.EndUpdate();
}
private void 增加消费_Load(object sender, EventArgs e)
{
// TODO: 这行代码将数据加载到表“dieteticManagementDataSet.餐牌”中。您可以根据需要移动或移除它。
this.餐牌TableAdapter.Fill(this.dieteticManagementDataSet.餐牌);
GetTree();
if (Exist)
{
string[] Column ={ "餐台号" };
string[] data ={ this.no };
string[] sdata = new string[10];
string ColorClass;
DataTable Dtable = Program.DBOpertor.Select("开单", Column, data).Tables[0];
for (int i = 0; i < Dtable.Rows.Count; i++)
{
sdata[0] = Dtable.Rows[i]["编号"].ToString();
sdata[1] = Dtable.Rows[i]["餐台号"].ToString();
sdata[2] = Dtable.Rows[i]["项目名称"].ToString();
sdata[3] = Dtable.Rows[i]["单价"].ToString();
剩余7页未读,继续阅读
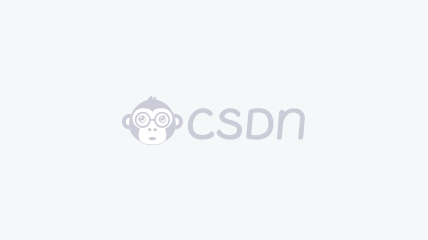

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

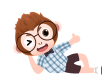
最新资源

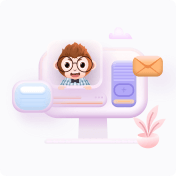
