package com.example.myapplication;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.os.Message;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.Spinner;
import android.widget.TextView;
import com.google.gson.Gson;
import java.io.IOException;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private Spinner mSpinner; // 下拉框对象
private String[] mCities,mcitiesid; // 城市及对应id
private ArrayAdapter<String> mspAdapter; // 适配器组件 绑定下拉框控件
private TextView tempTv,cityTv,conditionTv,dateTv,windTv,tenpRangerTv,air_index,chuxing_index;
private TextView kouzhao_index,sport_index,kaichuang_index,kongtiao_index;
private ImageView dayTv;
private String air_index_info,sport_index_info,chuxing_index_info,kouzhao_index_info,kaichuang_index_info,kongtiao_index_info;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView_NSL();
}
//初始化
private void initView_NSL(){
mSpinner = findViewById(R.id.sp_city);
mCities = getResources().getStringArray(R.array.cities);
mcitiesid = getResources().getStringArray(R.array.citiesid);
mspAdapter = new ArrayAdapter<>(this,R.layout.sp_item_layout,mCities); // 数组适配器
mSpinner.setAdapter(mspAdapter);
mSpinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { // 给下拉框注册事件监听
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
String selectedCity = mCities[i]; // 获取下拉框中所选城市
String selectedCityid = mcitiesid[i]; // 获取下拉框中所选城市的id
Log.d("NSL","------weather----NSL---" + selectedCity);
Log.d("NSL","------weather----NSL---" + selectedCityid);
getWeatherOfCity(selectedCityid);
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
}
});
tempTv = findViewById(R.id.frag_tv_currenttemp);
cityTv = findViewById(R.id.frag_tv_city);
conditionTv = findViewById(R.id.frag_tv_condition);
dateTv = findViewById(R.id.frag_tv_date);
windTv = findViewById(R.id.frag_tv_wind);
tenpRangerTv = findViewById(R.id.frag_tv_temprange);
air_index = findViewById(R.id.frag_index_air);
air_index.setOnClickListener(this);
chuxing_index = findViewById(R.id.frag_index_chuxing);
chuxing_index.setOnClickListener(this);
kouzhao_index = findViewById(R.id.frag_index_kouzhao);
kouzhao_index.setOnClickListener(this);
sport_index = findViewById(R.id.frag_index_yundong);
sport_index.setOnClickListener(this);
kaichuang_index = findViewById(R.id.frag_index_kaichuang);
kaichuang_index.setOnClickListener(this);
kongtiao_index = findViewById(R.id.frag_index_kongtiao);
kongtiao_index.setOnClickListener(this);
dayTv = findViewById(R.id.frag_tv_today);
}
private void getWeatherOfCity(final String selectdCityid){
new Thread(new Runnable() {
String weatherOfCity = "";
@Override
public void run() {
try {
weatherOfCity = NetUtil_ChenXuan.getWeather(selectdCityid);
} catch (IOException e) {
e.printStackTrace();
}
//将数据传递给主线程
Message message = Message.obtain(); // 获取当前分线程的信息
message.what = 0; // 消息代码,便于区分
message.obj = weatherOfCity;
mHandler.sendMessage(message); // 发送信息
}
}).start();
}
//使用Handler来接受和发送信息
private Handler mHandler=new Handler(Looper.myLooper()){
@Override
public void handleMessage(@NonNull Message msg) {
super.handleMessage(msg);
if (msg.what == 0){
String weather=(String)msg.obj;
Log.d("NSL","---------主线程收到天气信息---------" + weather);
Gson gson = new Gson();
WeatherBean weatherBean = gson.fromJson(weather,WeatherBean.class);//将天气数据由JSON格式转换为WeatherBean对象
if (weatherBean!=null){
updateUiOfWeather(weatherBean);
}
}
}
};
//对UI界面数据进行更新
private void updateUiOfWeather(WeatherBean weatherBean) {
String tem = weatherBean.getTem();
String tem1= weatherBean.getTem1();
String tem2 = weatherBean.getTem2();
String city = weatherBean.getCity();
String wea = weatherBean.getWea();
String wea_img = weatherBean.getWea_img();
String date = weatherBean.getDate();
String win = weatherBean.getWin();
String win_speed= weatherBean.getWin_speed();
ApiBean aqi = weatherBean.getAqi();
air_index_info = aqi.getAir_tips();
chuxing_index_info = aqi.getWaichu();
kouzhao_index_info = aqi.getKouzhao();
sport_index_info = aqi.getYundong();
kaichuang_index_info = aqi.getKaichuang();
kongtiao_index_info = aqi.getJinghuaqi();
tempTv.setText(tem + "℃");
cityTv.setText(city);
conditionTv.setText(wea);
dateTv.setText(date);
windTv.setText(win + win_speed);
tenpRangerTv.setText(tem2 + "~" + tem1 + "℃");
dayTv.setImageResource(getImageOfWeather(wea_img));
}
private int getImageOfWeather(String wea_img){
int result=0;
//xue、lei、shachen、wu、bingbao、yun、yu、yin、qing
switch (wea_img){
case "qing":
result = R.mipmap.biz_plugin_weather_qing;
break;
case "yin":
result = R.mipmap.biz_plugin_weather_yin;
break;
case "wu":
result = R.mipmap.biz_plugin_weather_wu;
break;
case "yu":
result = R.mipmap.biz_plugin_weather_xiaoyu;
break;
case "yun":
result = R.mipmap.biz_plugin_weather_duoyun;
break;
case "bingbao":
result = R.mipmap.biz_plugin_weather_zhongxue;
break;
case "shachen":
result = R.mipmap.biz_plugin_weather_shachenbao;
break;
case "lei":
result = R.mipmap.biz_plugin_weather_leizhenyu;
break;
case "xue":
result = R.mipmap.biz_plugin_weather_xiaoxue;
break;
}
return result;
}
public void onClick(View view) {//点击事件处理器
Log.d("NSL","----------事件处理---------");
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
switch (view.getId()){
case R.id.frag_index_air:
builder.setTitle("空气指数");
builder.setMessage(air_index_info);
builder.setPositiveButton("确定",null);
break;
case R.id.frag_index_chuxing:
builder.setTitle("出行指数");
builder.setMessage(chuxing_index_info);
builder.setPositiveButton("确定",null);
break;
case R
没有合适的资源?快使用搜索试试~ 我知道了~
android开发期末大作业天气预报App项目源码(95分以上).zip
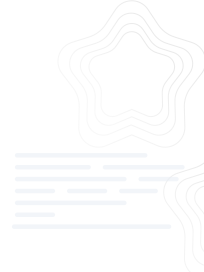
共77个文件
png:28个
xml:17个
webp:10个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
android开发期末大作业天气预报App项目源码(95分以上).zip 已获导师指导并通过的97分的高分期末大作业项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。 android开发期末大作业天气预报App项目源码(95分以上).zip 已获导师指导并通过的97分的高分期末大作业项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。android开发期末大作业天气预报App项目源码(95分以上).zip 已获导师指导并通过的97分的高分期末大作业项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。android开发期末大作业天气预报App项目源码(95分以上).zip 已获导师指导并通过的97分的高分期末大作业项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。android开发期末大作业天气预报App项目源码(95分以上).zip 已获导师指导并通过的97分的高分期末大作业项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。android开发期末大作业天气预报App项
资源推荐
资源详情
资源评论
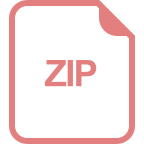
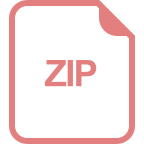
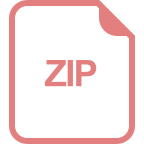
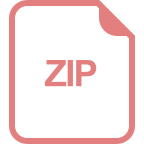
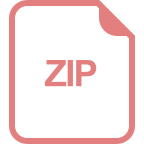
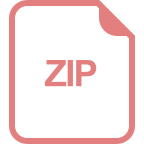
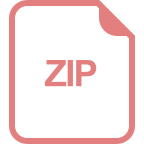
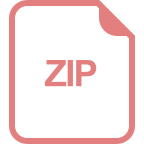
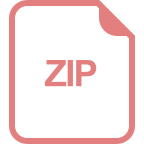
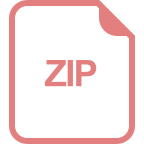
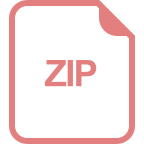
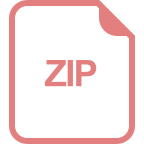
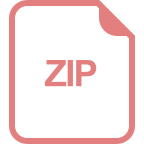
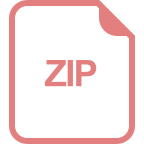
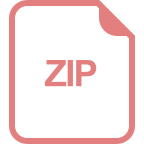
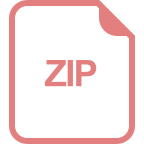
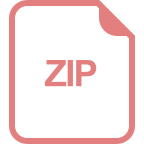
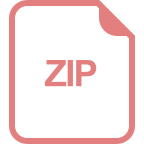
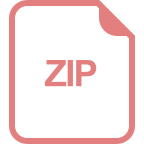
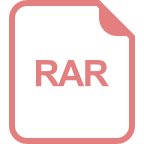
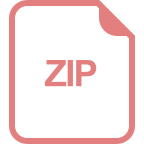
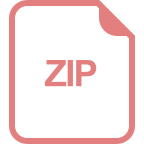
收起资源包目录


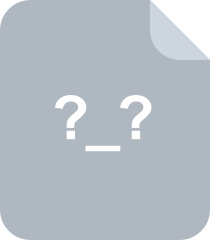


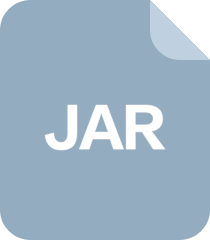
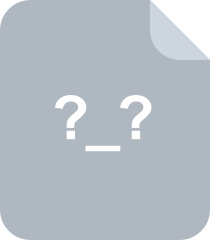

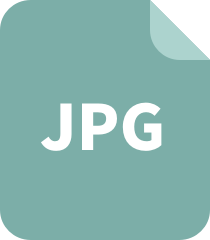
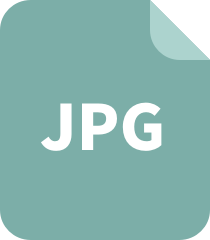







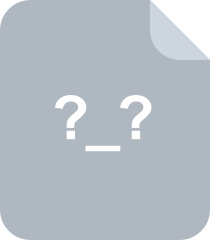





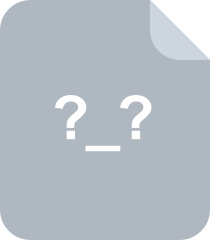





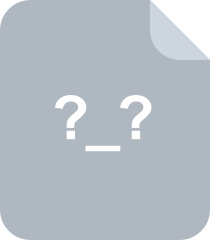
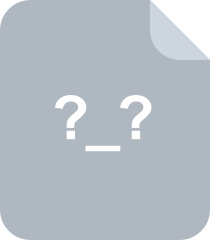
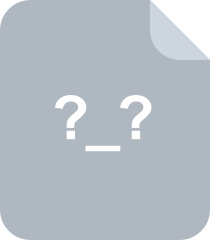
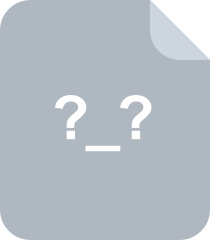


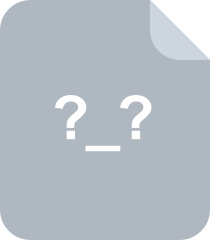
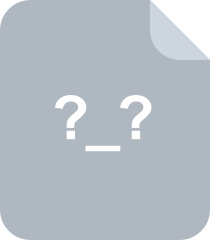

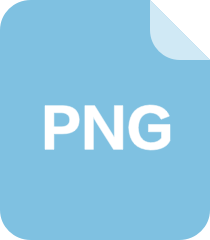
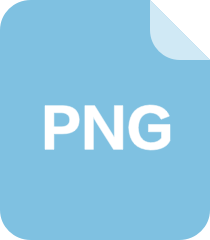
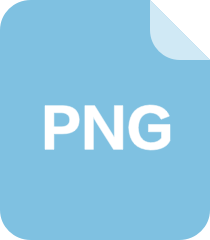
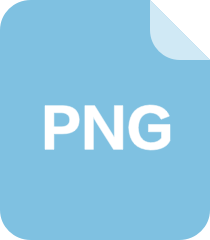
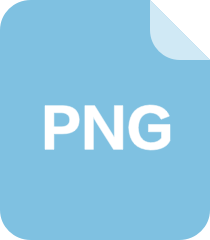
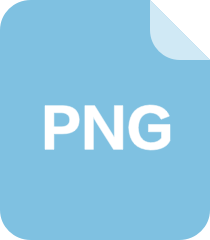
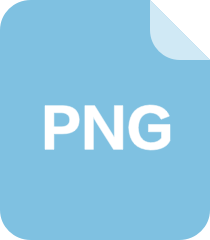
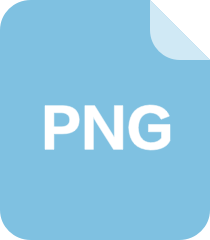
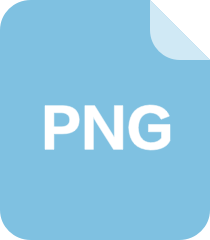
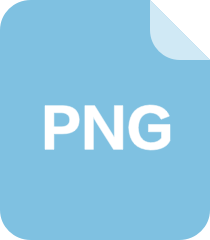
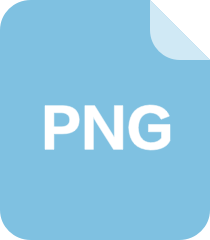
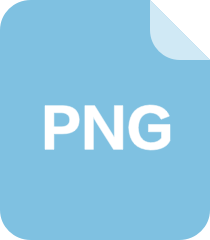
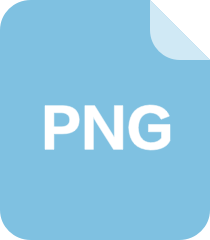
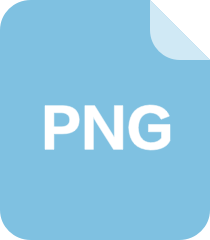
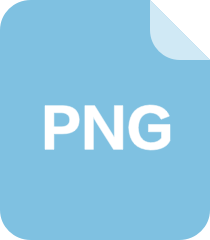
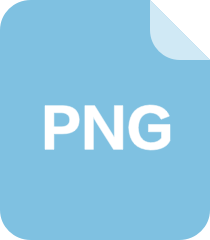
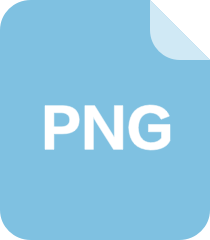
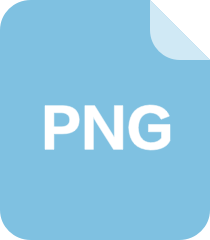
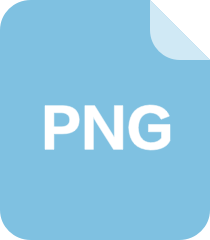
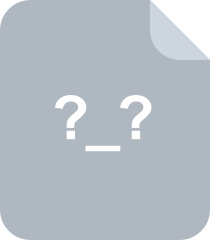
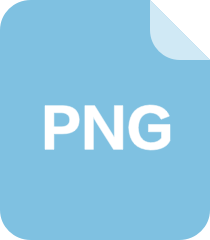
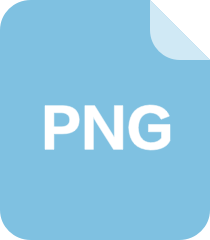
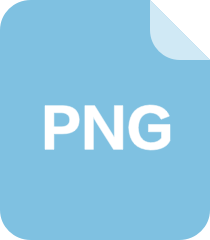
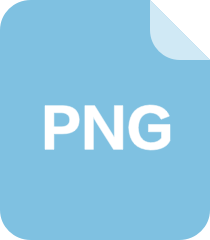
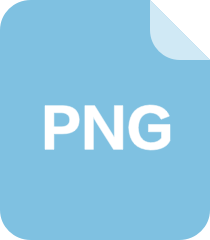
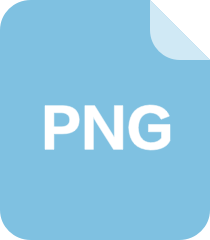
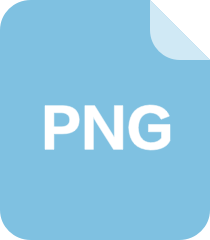
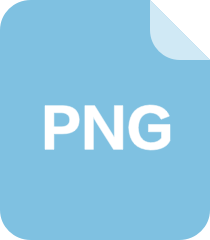
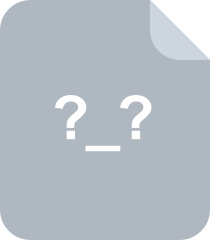
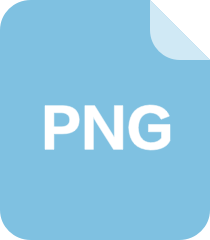

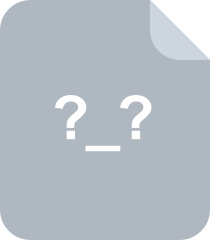

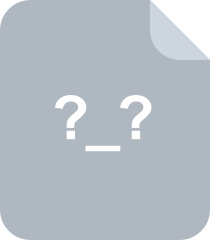
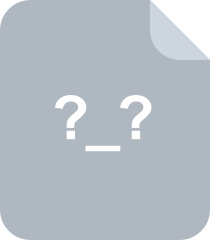

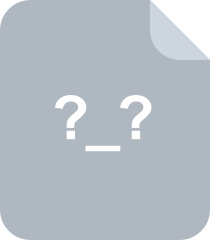

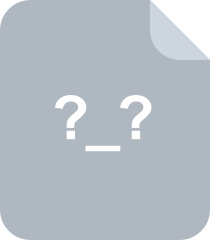
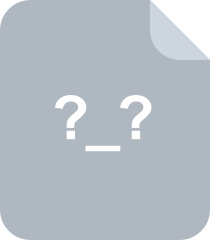

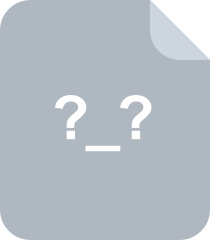
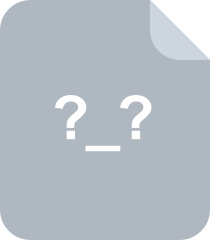

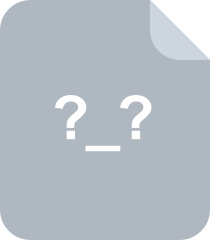
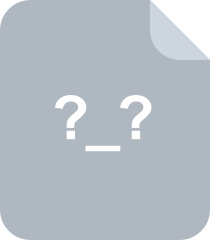

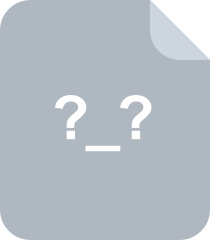
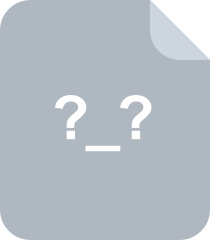

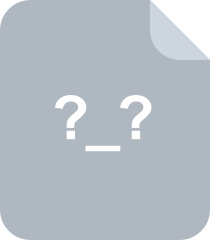
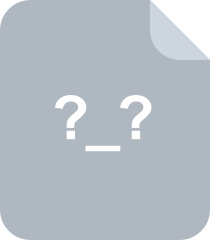
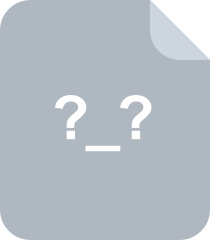

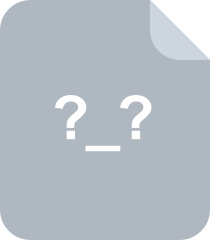
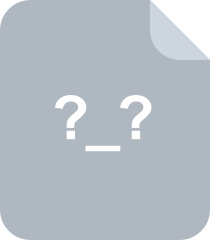

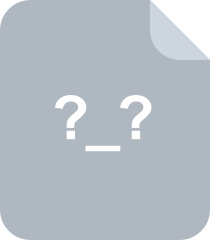
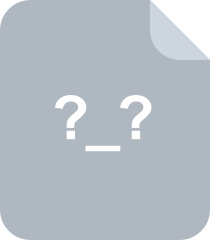
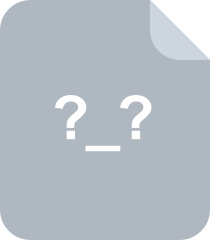

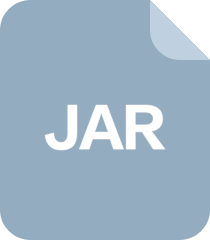
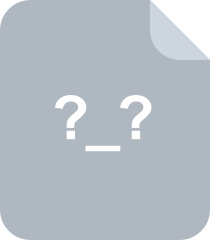
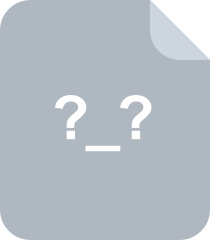
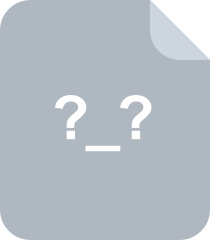
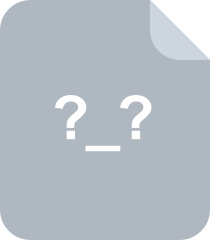

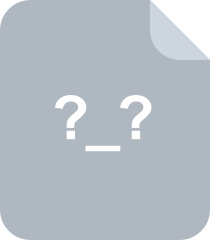
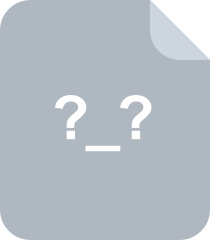
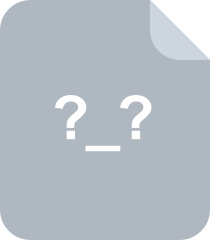
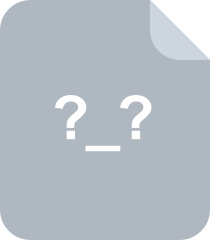
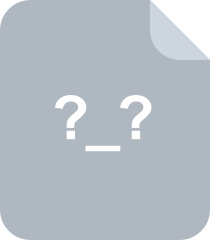
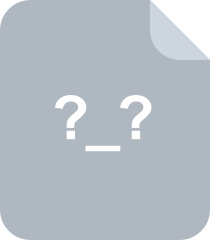
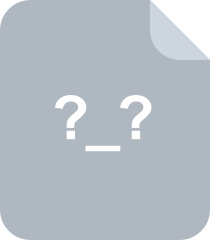
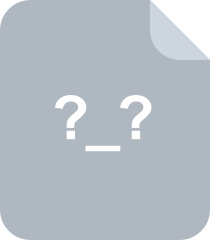
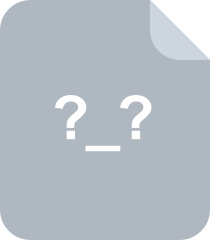
共 77 条
- 1

猰貐的新时代
- 粉丝: 1w+
- 资源: 2886

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

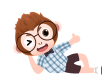
最新资源
- Power Quality Disturbance:基于MATLAB Simulink的各种电能质量扰动仿真模型,包括配电线路故障、感应电机启动、变压器励磁、单相 三相非线性负载等模型,可用于模拟各种
- 教务管理系统(jsp+servlet+mysql)130225.rar
- 教务管理系统(jsp+servlet+mysql).rar
- 酒店订单管理系统(Jsp+servlet+mysql)130224.rar
- 酒店订单管理系统(Jsp+servlet+mysql).rar
- 乐趣大型购物系统 v1.1(jsp+servlet+mysql).rar
- 聊天系统(java+applet)130227.rar
- 龙门物流管理系统(Ext+SSH).rar
- 乐趣大型购物系统 v1.1(jsp+servlet+mysql)130223.rar
- 基于动态窗口算法的AGV仿真避障 可设置起点目标点,设置地图,设置移动障碍物起始点目标点,未知静态障碍物 动态窗口方法(DynamicWindowApproach) 是一种可以实现实时避障的局部规划算
- 内容管理系统(hibernate3+struts2+spring2).rar
- 内容管理系统(hibernate3+struts2+spring2)130224.rar
- 企业费用管理系统(SSH+Oracle).rar
- 企业费用管理系统(SSH+Oracle)130222.rar
- 企业级新闻系统(SSH+MYSQL).rar
- 通用的在线考试系统(jsp+struts+hibernate+oracle).rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


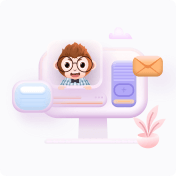
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页