package com.vaccination.controller;
import com.alibaba.fastjson.JSONObject;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.vaccination.entity.InoculationRecord;
import com.vaccination.entity.User;
import com.vaccination.service.InoculationRecordService;
import com.vaccination.service.UserService;
import com.vaccination.util.PageRequest;
import com.vaccination.util.PageResponse;
import com.vaccination.util.Result;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.List;
@RestController
public class InoculationController {
@Autowired
private InoculationRecordService inoculationRecordService;
@Autowired
private UserService userService;
@PostMapping("/listInoculations")
public PageResponse listInoculations(HttpServletRequest request, PageRequest page) {
String loginUser = (String) request.getSession().getAttribute("loginUser");
User user = JSONObject.parseObject(loginUser, User.class);
if (user == null) {
PageResponse pageResponse = new PageResponse();
pageResponse.setMsg("请登陆");
return pageResponse;
}
if (user.getRole() == 2) {
user.setId(-1L);
}
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
IPage<InoculationRecord> iPage = inoculationRecordService.listInoculations(new Page<>(page.getPage(), page.getLimit()), user.getId());
List<InoculationRecord> records = iPage.getRecords();
records.forEach(item -> {
if (StringUtils.isBlank(item.getUsername()) && item.getUserId() != null) {
User byId = userService.getById(item.getUserId());
if (byId != null) {
item.setUsername(byId.getName());
item.setUserIdentity(byId.getIdentityNum());
}
}
if (item.getInoculationTimeOne() != null) {
item.setInoculationTimeStrOne(dateFormat.format(item.getInoculationTimeOne()));
}
if (item.getInoculationTimeTwo() != null) {
item.setInoculationTimeStrTwo(dateFormat.format(item.getInoculationTimeTwo()));
}
if (item.getInoculationTimeThree() != null) {
item.setInoculationTimeStrThree(dateFormat.format(item.getInoculationTimeThree()));
}
});
return new PageResponse("0", "请求成功", iPage.getTotal(), records);
}
@GetMapping("/delInoculation")
public Result delInoculation(Long id) {
inoculationRecordService.removeById(id);
return Result.success("删除成功");
}
@PostMapping("/saveInoculation")
public Result saveInoculation(InoculationRecord record, HttpServletRequest request) throws ParseException {
String loginUser = (String) request.getSession().getAttribute("loginUser");
User user = JSONObject.parseObject(loginUser, User.class);
if (user == null) {
return Result.error("请登陆");
}
if(record.getStatusThree() == 1 && (record.getStatusTwo() == 2 || record.getStatusOne() == 2)) {
return Result.error("请先接种第一二针");
}
if(record.getStatusTwo() == 1 && record.getStatusTwo() == 2) {
return Result.error("请先接种第一针");
}
record.setUserId(user.getId());
if (StringUtils.isNoneBlank(record.getUsername())){
User byUsername = userService.getByUsername(record.getUsername());
if(byUsername == null) {
User newUser = new User();
newUser.setUsername(record.getUsername());
newUser.setName(record.getUsername());
newUser.setPassword("123456");
newUser.setRole(1);
newUser.setStatus(1);
userService.save(newUser);
byUsername = newUser;
}
record.setUserId(byUsername.getId());
}
if (StringUtils.isNoneBlank(record.getInoculationTimeStrOne())) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
record.setInoculationTimeOne(dateFormat.parse(record.getInoculationTimeStrOne()));
}
if (StringUtils.isNoneBlank(record.getInoculationTimeStrTwo())) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
record.setInoculationTimeTwo(dateFormat.parse(record.getInoculationTimeStrTwo()));
}
if (StringUtils.isNoneBlank(record.getInoculationTimeStrThree())) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
record.setInoculationTimeThree(dateFormat.parse(record.getInoculationTimeStrThree()));
}
inoculationRecordService.save(record);
return Result.success("添加成功");
}
@PostMapping("/updateInoculation")
public Result updateInoculation(InoculationRecord record) throws ParseException {
if (record.getId() == null) {
return Result.error("更新失败");
}
if(record.getStatusThree() == 1 && (record.getStatusTwo() == 2 || record.getStatusOne() == 2)) {
return Result.error("请先接种第一二针");
}
if(record.getStatusTwo() == 1 && record.getStatusTwo() == 2) {
return Result.error("请先接种第一针");
}
if (StringUtils.isNoneBlank(record.getInoculationTimeStrOne())) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
record.setInoculationTimeOne(dateFormat.parse(record.getInoculationTimeStrOne()));
}
if (StringUtils.isNoneBlank(record.getInoculationTimeStrTwo())) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
record.setInoculationTimeTwo(dateFormat.parse(record.getInoculationTimeStrTwo()));
}
if (StringUtils.isNoneBlank(record.getInoculationTimeStrThree())) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
record.setInoculationTimeThree(dateFormat.parse(record.getInoculationTimeStrThree()));
}
inoculationRecordService.updateById(record);
return Result.success("更新成功");
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于 springboot 的疫苗接种管理系统+数据库(95分以上大作业项目).zip 已获老师指导并通过的高分设计项目,可作为期末大作业和课程设计,纯手打高分项目,小白实战没难度。 基于 springboot 的疫苗接种管理系统+数据库(95分以上大作业项目).zip 已获老师指导并通过的高分设计项目,可作为期末大作业和课程设计,纯手打高分项目,小白实战没难度。 基于 springboot 的疫苗接种管理系统+数据库(95分以上大作业项目).zip 已获老师指导并通过的高分设计项目,可作为期末大作业和课程设计,纯手打高分项目,小白实战没难度。 基于 springboot 的疫苗接种管理系统+数据库(95分以上大作业项目).zip 已获老师指导并通过的高分设计项目,可作为期末大作业和课程设计,纯手打高分项目,小白实战没难度。 基于 springboot 的疫苗接种管理系统+数据库(95分以上大作业项目).zip 已获老师指导并通过的高分设计项目,可作为期末大作业和课程设计,纯手打高分项目,小白实战没难度。 基于 springboot 的疫苗接种管理系统+数据库(95
资源推荐
资源详情
资源评论
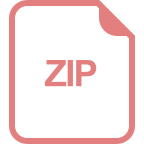
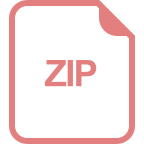
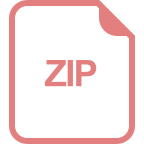
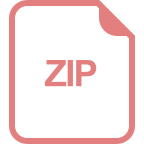
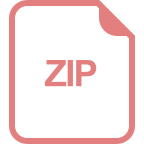
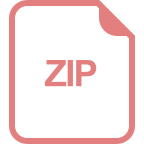
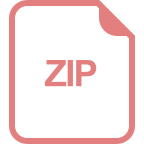
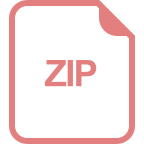
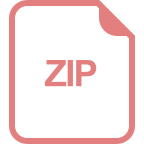
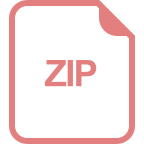
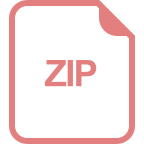
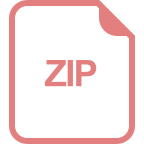
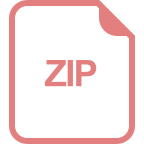
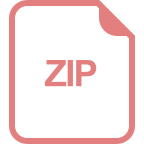
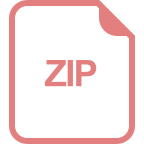
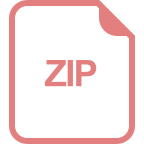
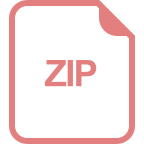
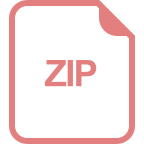
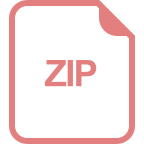
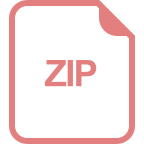
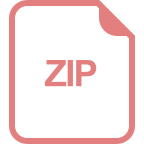
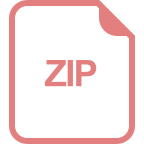
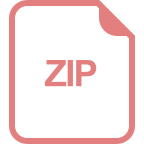
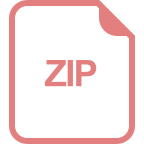
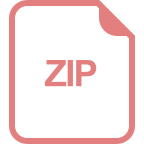
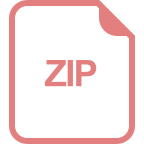
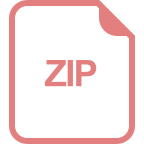
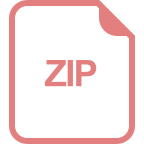
收起资源包目录


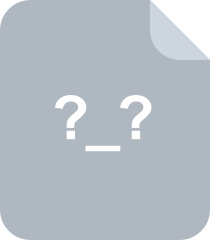
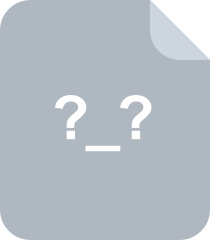
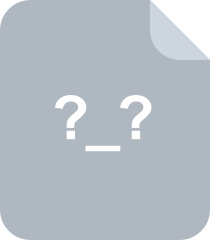





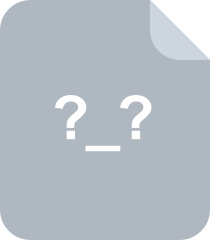



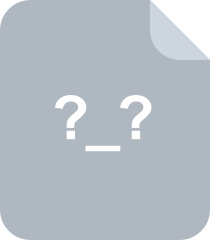
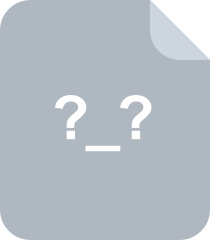
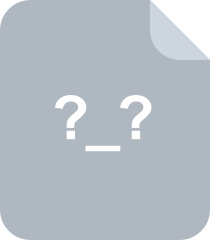
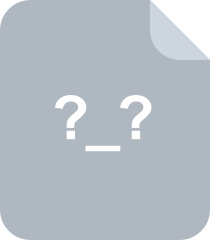
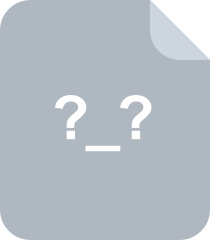
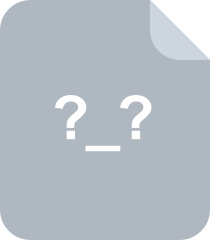
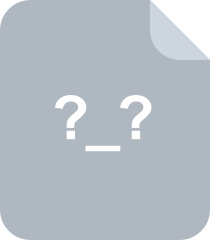

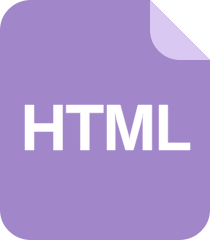
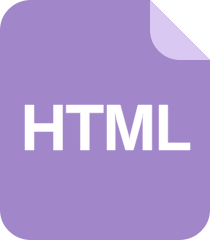
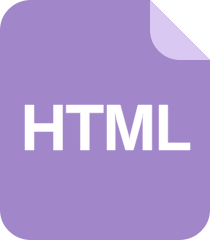
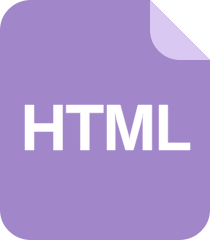
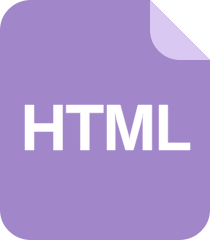
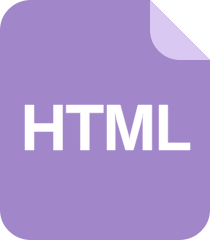
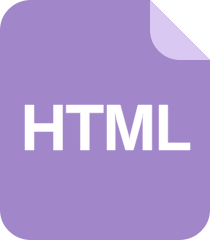
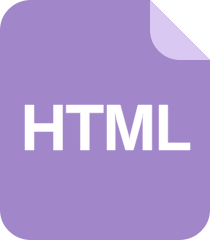
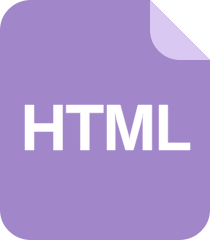
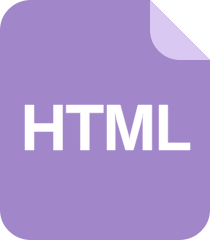
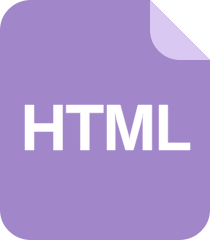
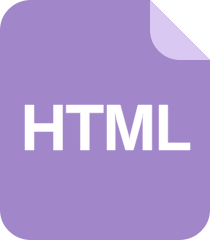
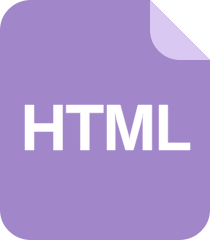
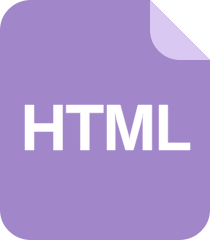
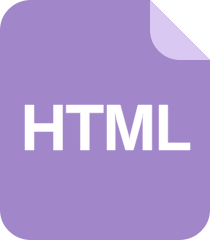
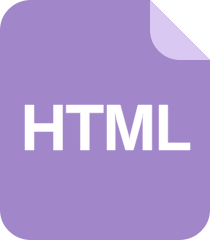
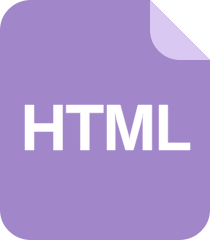
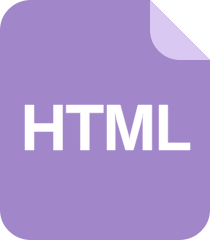
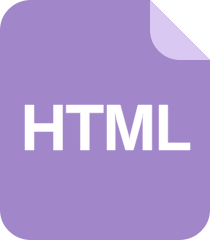

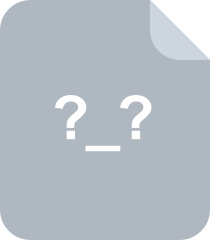

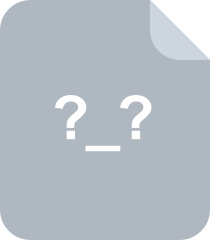
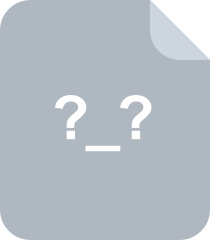
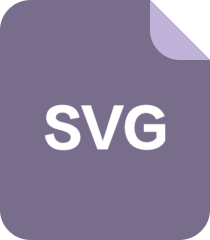
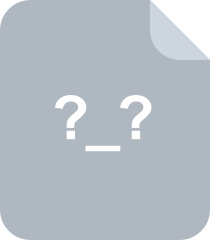
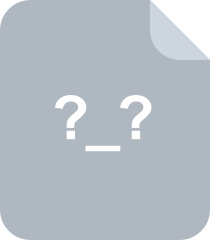

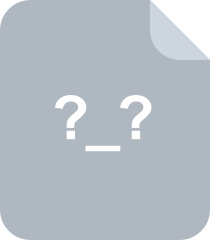

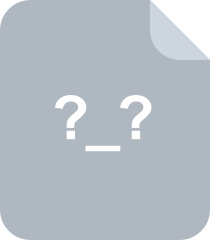



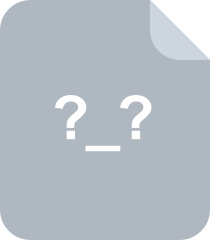
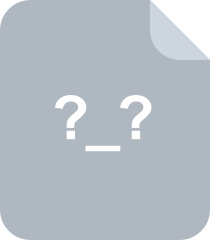


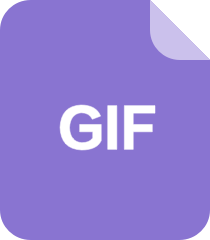
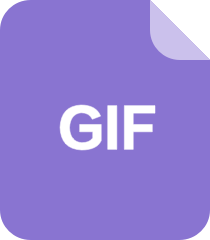
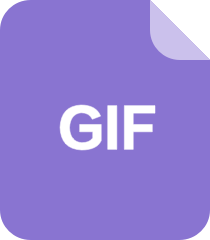
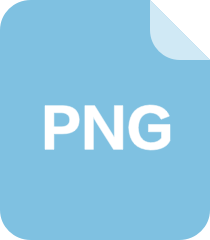
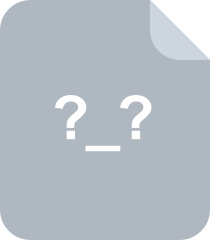
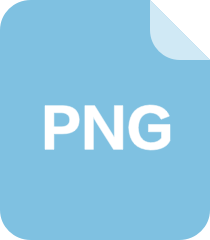
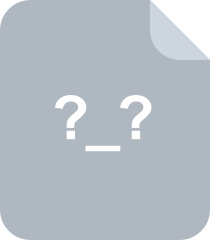




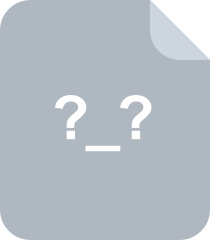
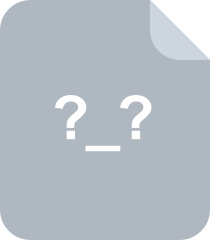
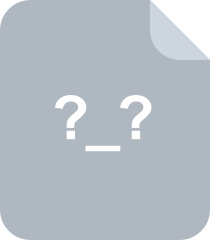
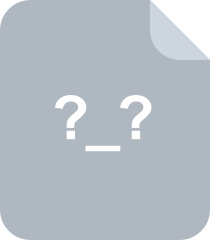
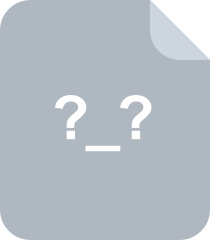
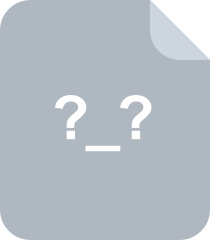
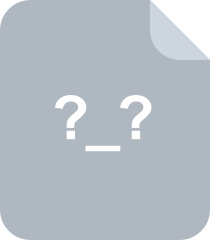

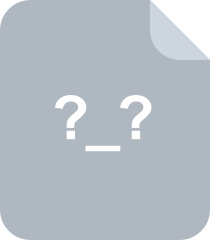
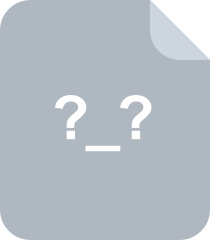
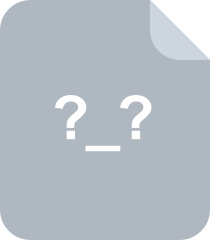
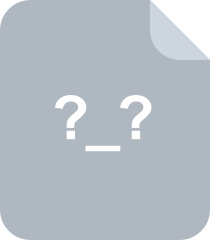
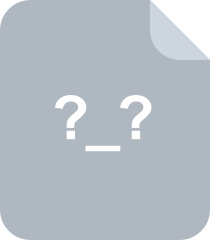
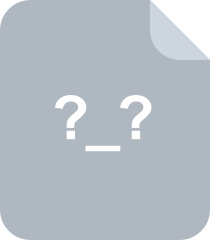
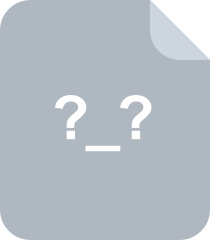
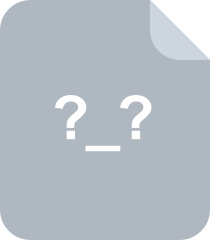

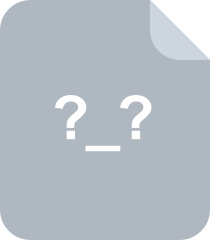
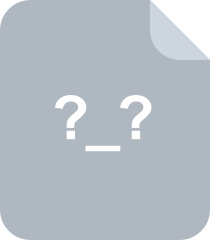
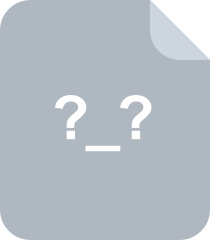
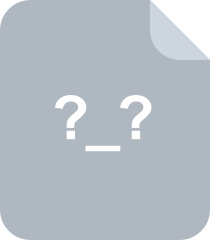
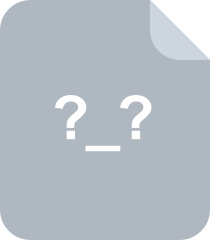

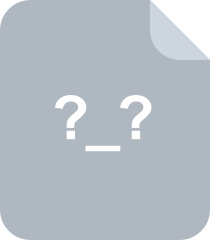
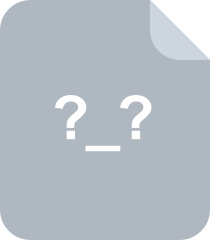
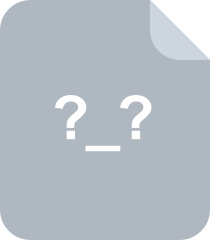
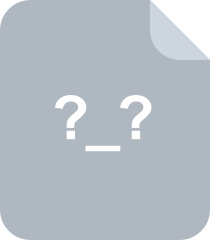
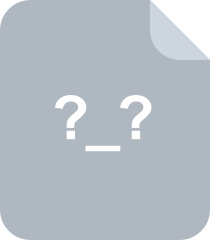
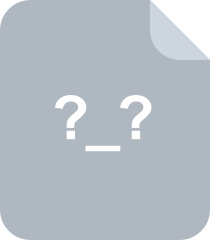
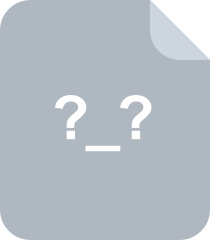
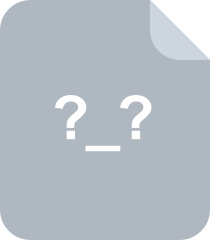
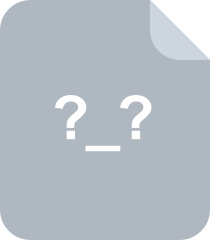

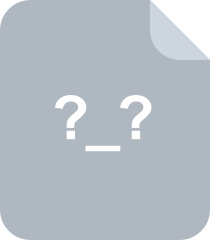
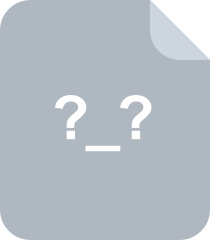
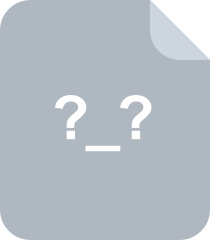
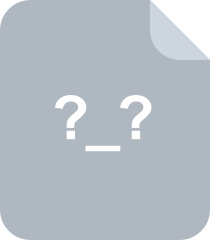
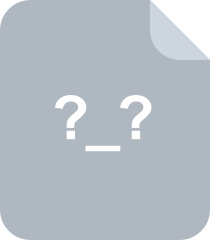
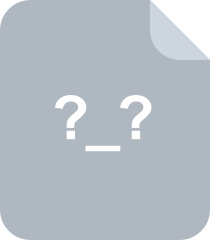
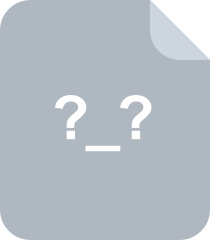

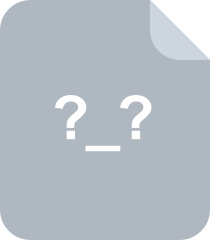
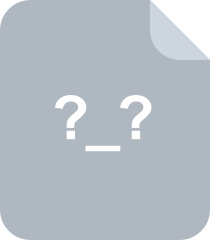
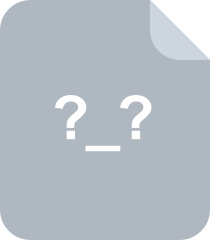
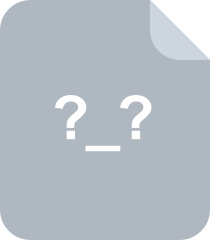

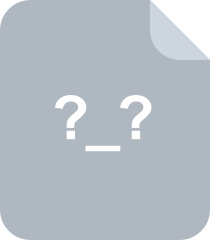
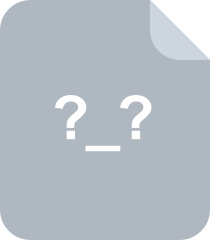
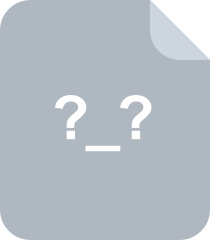
共 90 条
- 1
资源评论
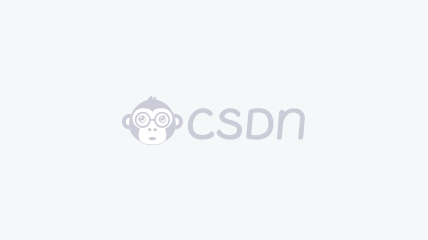
- 努力不掉队2024-03-28超级好的资源,很值得参考学习,对我启发很大,支持!

猰貐的新时代
- 粉丝: 1w+
- 资源: 2571

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

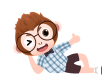
最新资源
- C语言-leetcode题解之70-climbing-stairs.c
- C语言-leetcode题解之68-text-justification.c
- C语言-leetcode题解之66-plus-one.c
- C语言-leetcode题解之64-minimum-path-sum.c
- C语言-leetcode题解之63-unique-paths-ii.c
- C语言-leetcode题解之62-unique-paths.c
- C语言-leetcode题解之61-rotate-list.c
- C语言-leetcode题解之59-spiral-matrix-ii.c
- C语言-leetcode题解之58-length-of-last-word.c
- 计算机编程课程设计基础教程
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


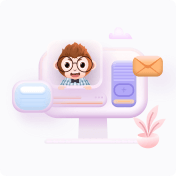
安全验证
文档复制为VIP权益,开通VIP直接复制
