/*
* Generated by MyEclipse Struts
* Template path: templates/java/JavaClass.vtl
*/
package com.aptech.jb.epet.web.action;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.action.ActionMessage;
import org.apache.struts.action.ActionMessages;
import org.apache.struts.actions.DispatchAction;
import com.aptech.jb.epet.biz.PetInfoBiz;
import com.aptech.jb.epet.biz.impl.PetInfoBizImpl;
import com.aptech.jb.epet.entity.PetInfo;
import com.aptech.jb.epet.web.form.PetForm;
/**
* MyEclipse Struts Creation date: 02-14-2011
*
* XDoclet definition:
*
* @struts.action validate="true"
*/
public class PetAction extends DispatchAction {
/*
* Generated Methods
*/
/**
* Method execute
*
* @param mapping
* @param form
* @param request
* @param response
* @return ActionForward
* @throws IOException
*/
public ActionForward doLogin(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException {
PetForm petForm = (PetForm) form;
String petId = String.valueOf(petForm.getItem().getPetId());
String petPassword = petForm.getItem().getPetPassword();
String vcode = request.getParameter("item.petVcode");
PetInfoBiz biz = new PetInfoBizImpl();
PetInfo petInfo = biz.login(petId, petPassword);
if (!vcode.equals(request.getSession().getAttribute("vcode"))) {
ActionMessages errors = new ActionMessages();
errors.add("login", new ActionMessage("error.login.wrong_vcode"));
super.saveErrors(request, errors);
return mapping.findForward("fail");
}
if (petInfo != null) {
request.getSession(true).setAttribute("CURRENT_PET", petInfo);
// this.showBaby(mapping, dynaForm, request, response);
response.sendRedirect("pet.do?id=" + petId+"&operate=showBaby");
return null;
} else {
ActionMessages errors = new ActionMessages();
errors.add("login",
new ActionMessage("error.login.wrong_id_or_pwd"));
super.saveErrors(request, errors);
return mapping.findForward("fail");
}
}
public ActionForward doFeed(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException {
String petId = request.getParameter("petId");
PetInfoBiz biz = new PetInfoBizImpl();
biz.doFeed(Integer.parseInt(petId));
response.sendRedirect("pet.do?id=" + petId+"&operate=showBaby");
return null;
}
public ActionForward doGame(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException {
String petId = request.getParameter("petId");
PetInfoBiz biz = new PetInfoBizImpl();
biz.doGame(Integer.parseInt(petId));
response.sendRedirect("pet.do?id=" + petId+"&operate=showBaby");
return null;
}
public ActionForward doStory(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException {
String petId = request.getParameter("petId");
PetInfoBiz biz = new PetInfoBizImpl();
biz.doStory(Integer.parseInt(petId));
response.sendRedirect("pet.do?id=" + petId+"&operate=showBaby");
return null;
}
public ActionForward showBaby(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
//PetDiaryBiz pdb=new PetDiaryBizImpl();
String id =request.getParameter("id");
String error = "";
if (null == id || "".equals(id)) {
request.setAttribute("error", "没有指定宠物编号");
return mapping.findForward("error");
}
Integer petId = Integer.valueOf(id);
//List diaryList=pdb.getListByPet(petId);
PetInfoBiz biz = new PetInfoBizImpl();
PetInfo petInfo = biz.load(petId);
if (null == petInfo) {
request.setAttribute("error", "没有指定编号的宠物记录");
return mapping.findForward("error");
}
request.setAttribute("item", petInfo);
//request.setAttribute("diaryList", diaryList);
return mapping.findForward("petInfo");
}
public ActionForward toEditPet(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
String id = request.getParameter("petId");
String error = "";
if (null == id || "".equals(id)) {
request.setAttribute("error", "没有指定宠物编号");
return mapping.findForward("error");
}
Integer petId = Integer.valueOf(id);
PetInfoBiz biz = new PetInfoBizImpl();
PetInfo petInfo = biz.load(petId);
if (null == petInfo) {
request.setAttribute("error", "没有指定编号的宠物记录");
return mapping.findForward("error");
}
request.setAttribute("item", petInfo);
return mapping.findForward("update");
}
public ActionForward doEditPet(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException {
PetForm petForm = (PetForm) form;
String name = petForm.getItem().getPetName();
String email = petForm.getItem().getPetOwnerEmail();
String intro = petForm.getItem().getPetIntro();
int petId = Integer.parseInt(request.getParameter("id"));
PetInfoBiz pib = new PetInfoBizImpl();
PetInfo pet = pib.load(Integer.valueOf(petId));
pet.setPetId(Integer.valueOf(petId));
pet.setPetName(name);
pet.setPetOwnerEmail(email);
pet.setPetIntro(intro);
int num = pib.updatePet(pet);
if (num > 0) {
// request.getRequestDispatcher("jsp/pet/petInfo.jsp").forward(request,
// response);
response.sendRedirect("pet.do?id=" + petId+"&operate=showBaby");
} else {
request.setAttribute("error", "更新失败");
return mapping.findForward("error");
}
return null;
}
public ActionForward toList(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
PetInfoBiz pib=new PetInfoBizImpl();
PetForm petForm=(PetForm) form;
List list=pib.search(petForm.getCondition(), petForm.getOrderBy());
if(list!=null &&list.size()>0){
request.setAttribute("list", list);
return mapping.findForward("list");
}else{
request.setAttribute("error", "没有宠物记录");
return mapping.findForward("error");
}
}
public ActionForward doAdopt(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
PetForm petForm = (PetForm) form;// TODO Auto-generated method stub
if(petForm.getItem().getPetName()==null){
return mapping.findForward("adopt");
}
PetInfo item=petForm.getItem();
PetInfoBiz pib=new PetInfoBizImpl();
pib.adopt(item);
try {
response.setContentType("text/html;charset=GBK");
PrintWriter out = response.getWriter();
out.print("<script>alert('宠物ID:"+item.getPetId()+"');location.href='pet.do?id="+item.getPetId()+"&operate=showBaby'</script>");
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public ActionForward doDel(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException {
int petId = Integer.parseInt(request.getParameter("id"));
PetInfoBiz pib = new PetInfoBizImpl();
int num = pib.del(petId);
if (num > 0) {
// request.getSession().setAttribute("CURRENT_PET",null);
response.sendRedirect("jsp/pet/index.jsp");
} else {
request.setAttribute("error", "删除失败");
return mapping.findForward("error");
}
return null;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架
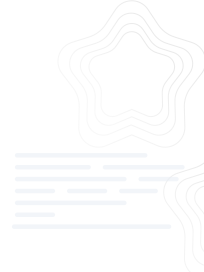
共121个文件
jar:31个
class:17个
java:17个


温馨提示
ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架 ssh 框架
资源推荐
资源详情
资源评论
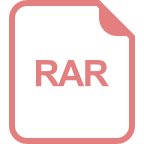
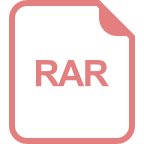
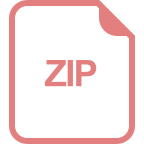
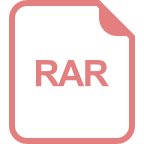
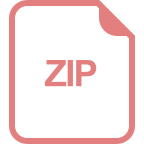
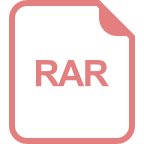
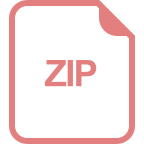
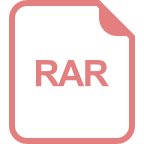
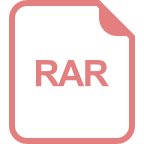
收起资源包目录

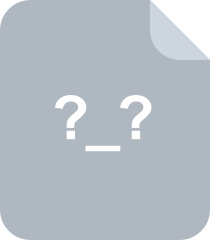
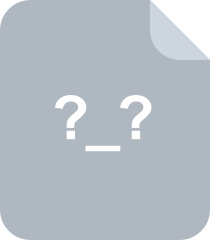
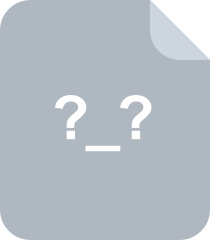
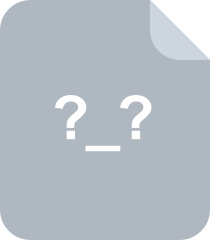
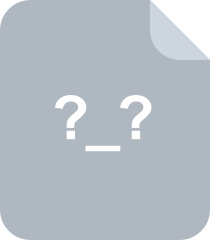
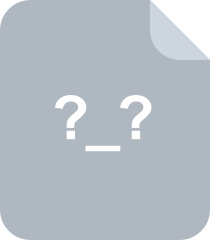
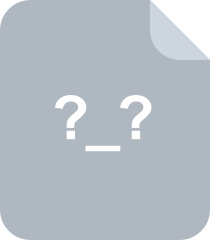
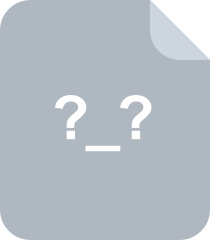
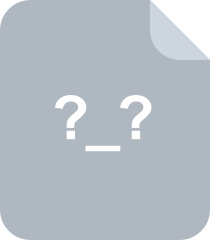
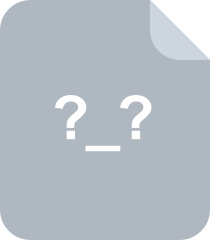
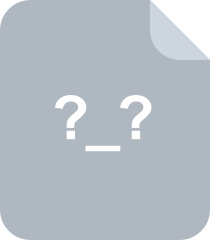
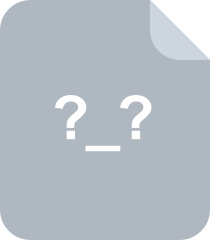
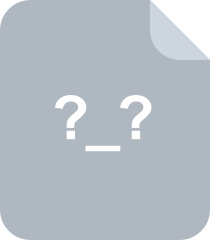
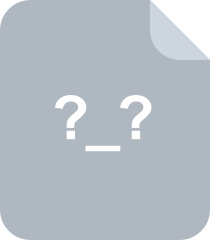
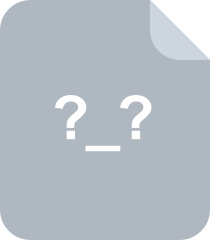
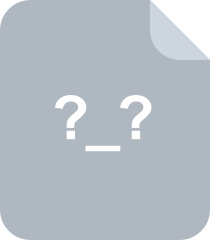
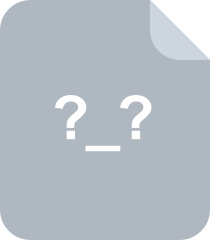
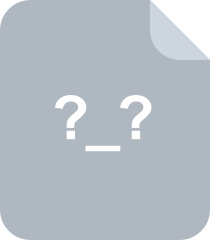
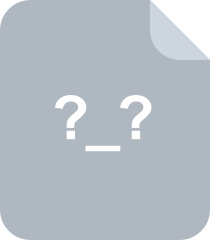
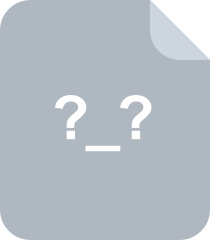
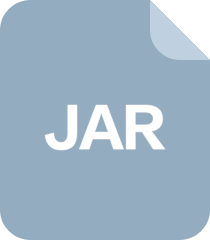
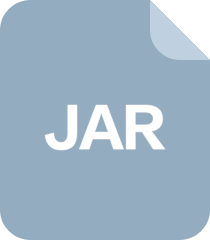
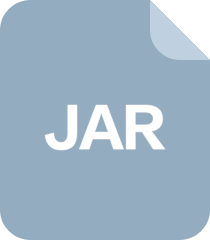
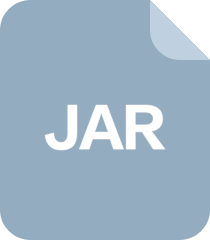
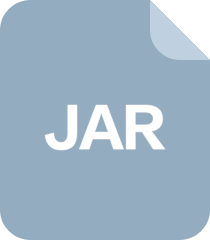
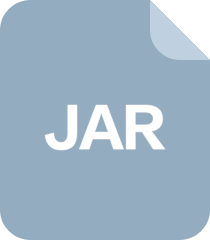
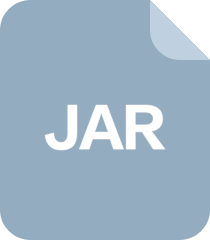
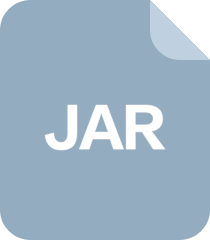
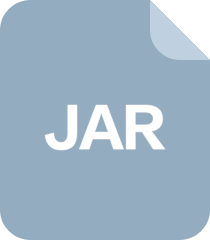
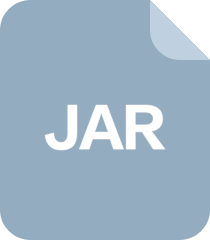
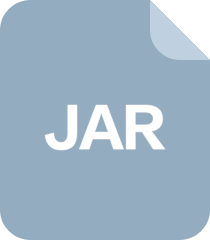
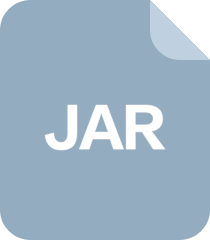
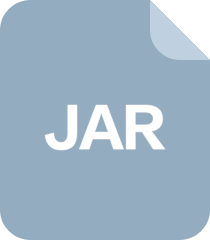
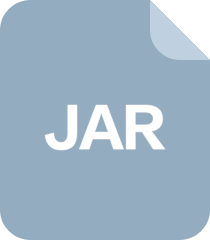
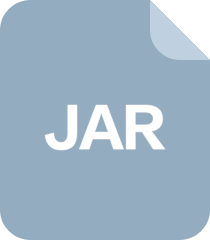
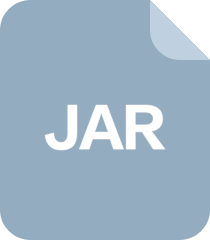
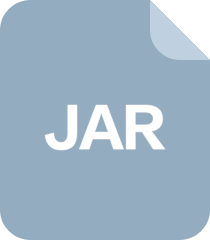
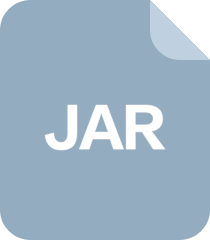
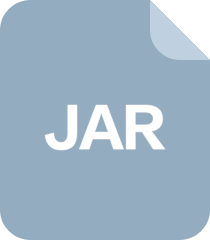
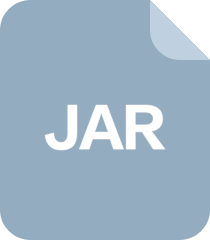
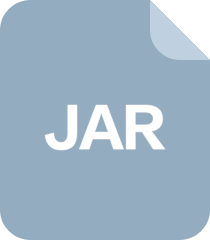
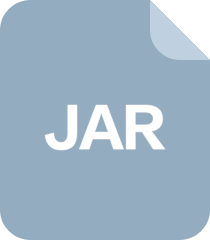
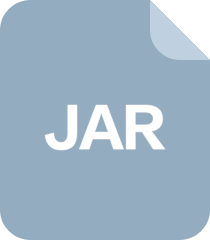
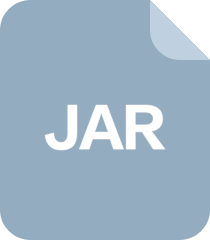
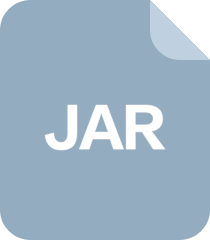
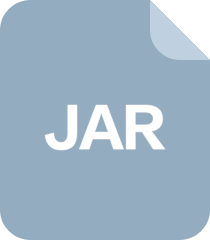
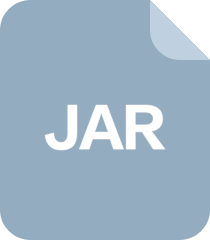
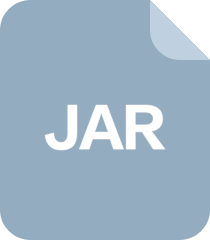
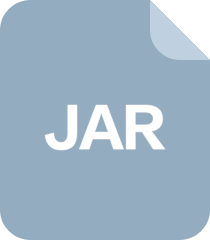
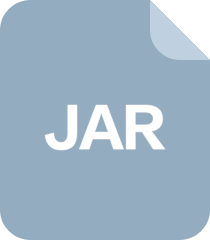
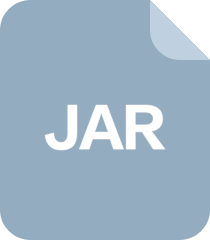
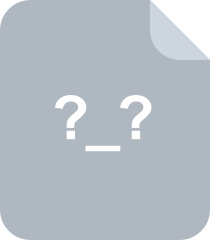
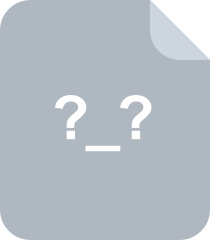
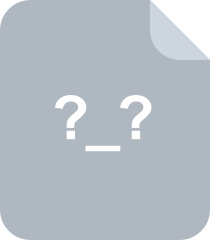
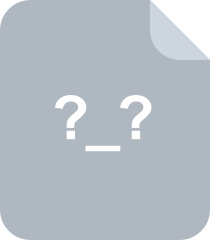
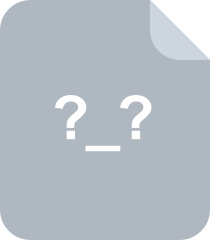
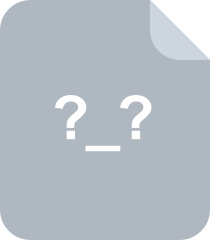
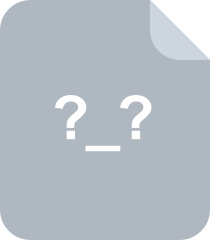
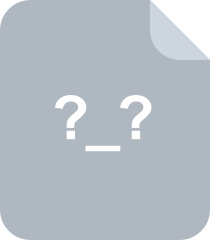
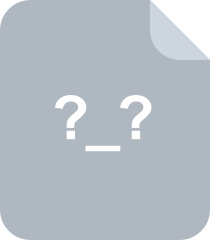
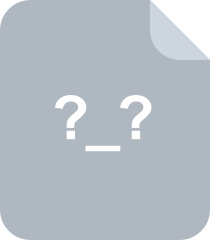
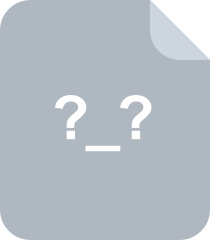
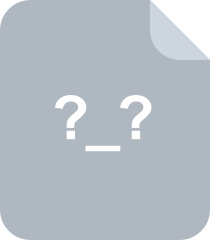
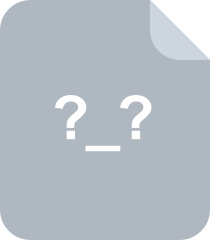
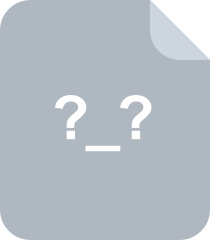
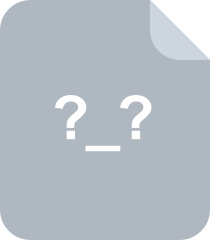
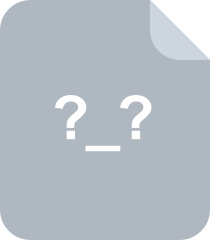
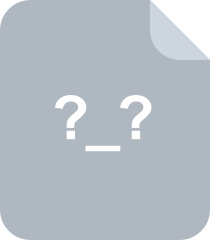
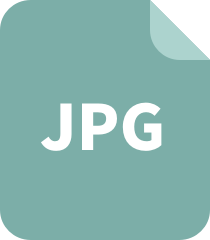
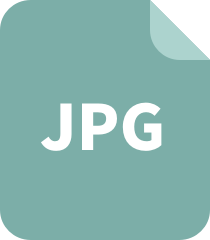
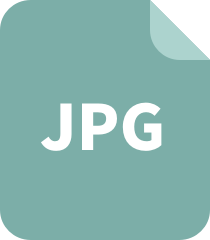
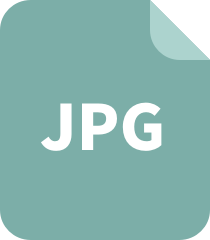
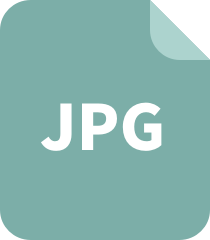
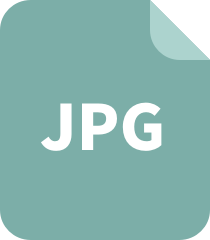
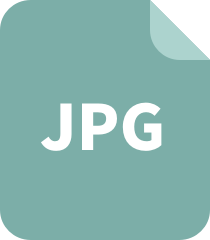
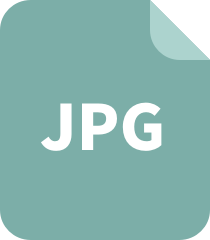
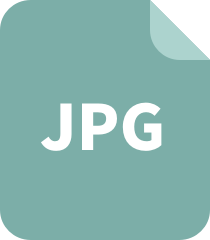
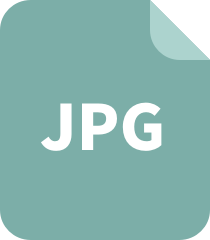
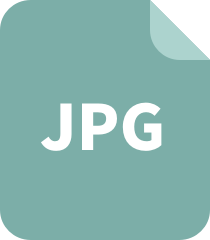
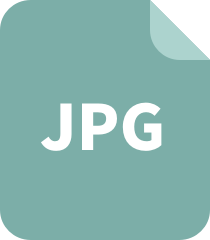
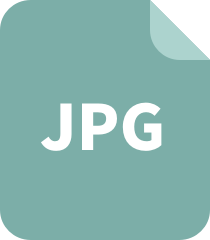
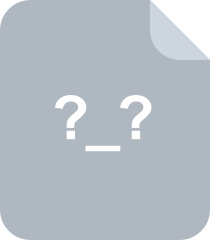
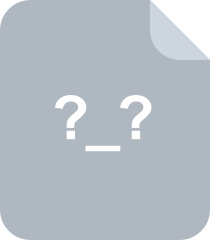
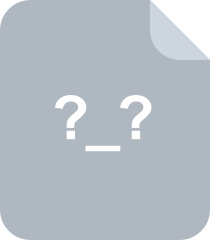
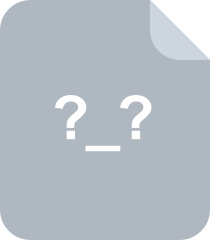
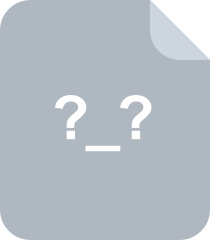
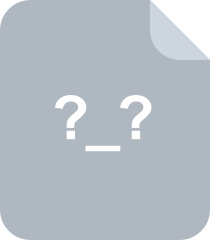
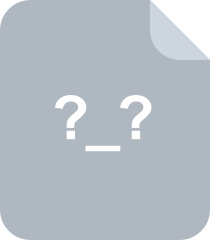
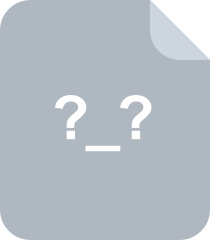
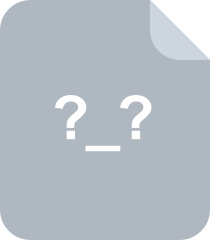
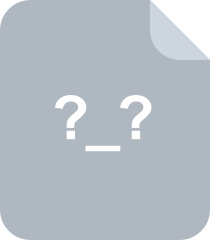
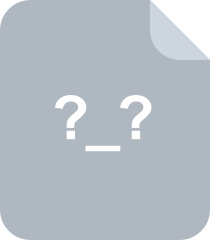
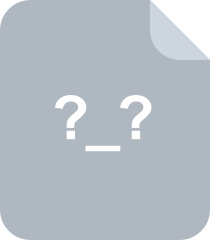
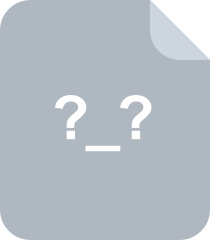
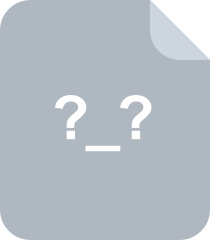
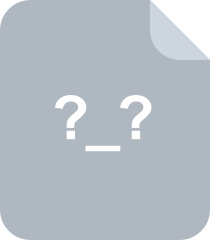
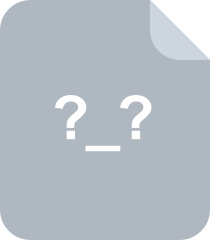
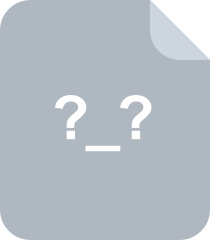
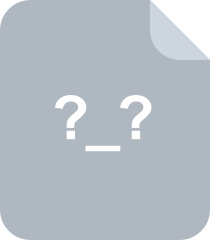
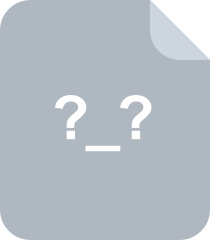
共 121 条
- 1
- 2
资源评论
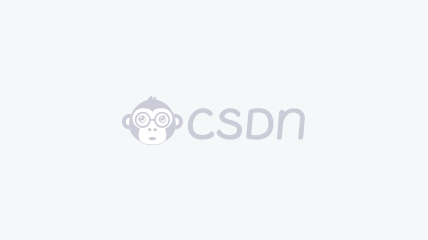
- imufengxianyong2012-06-24不是很完整
- cc陈(Skylar)2012-06-18啥,数据库导入不行,只用到2个框架,重要的sprig不见

chenglinjava
- 粉丝: 2
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

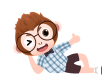
最新资源
- 西门子V90效率倍增-伺服驱动功能库详解-简易非循环功能库之绝对值编码器校准.mp4
- 六轴,scara机器人运动学分析,建模和运动控制 matlab,simulink,simscape.机器人工具箱,DH建模 Pd控制,滑模控制,模糊控制等等
- 车辆,汽车检测1-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 美国旧金山28R粗糙跑道数据
- mp3转换器小程序-音频20241222115740.mp3
- 车辆船只检测8-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- Questasim仿真脚本2
- Questasim仿真脚本
- Django开发中常见问题与解决方案的全面指南
- 西工大noj 116题及答案word版.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


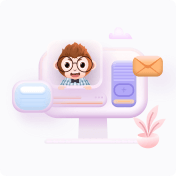
安全验证
文档复制为VIP权益,开通VIP直接复制
