/******************************************************************************
* Project: libspatialindex - A C++ library for spatial indexing
* Author: Marios Hadjieleftheriou, mhadji@gmail.com
******************************************************************************
* Copyright (c) 2004, Marios Hadjieleftheriou
*
* All rights reserved.
*
* Permission is hereby granted, free of charge, to any person obtaining a
* copy of this software and associated documentation files (the "Software"),
* to deal in the Software without restriction, including without limitation
* the rights to use, copy, modify, merge, publish, distribute, sublicense,
* and/or sell copies of the Software, and to permit persons to whom the
* Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included
* in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
* OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
* THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
* DEALINGS IN THE SOFTWARE.
******************************************************************************/
#pragma once
#if (defined _WIN32 || defined _WIN64 || defined WIN32 || defined WIN64) && !defined __GNUC__
typedef __int8 int8_t;
typedef __int16 int16_t;
typedef __int32 int32_t;
typedef __int64 int64_t;
typedef unsigned __int8 uint8_t;
typedef unsigned __int16 uint16_t;
typedef unsigned __int32 uint32_t;
typedef unsigned __int64 uint64_t;
// Nuke this annoying warning. See http://www.unknownroad.com/rtfm/VisualStudio/warningC4251.html
#pragma warning( disable: 4251 )
#else
#include <stdint.h>
#endif
#if (defined _WIN32 || defined _WIN64 || defined WIN32 || defined WIN64) && !defined __GNUC__
#ifdef SPATIALINDEX_CREATE_DLL
#define SIDX_DLL __declspec(dllexport)
#else
#define SIDX_DLL __declspec(dllimport)
#endif
#else
#define SIDX_DLL
#endif
#include <assert.h>
#include <iostream>
#include <iomanip>
#include <iterator>
#include <string>
#include <sstream>
#include <fstream>
#include <queue>
#include <vector>
#include <map>
#include <set>
#include <stack>
#include <list>
#include <algorithm>
#include <cwchar>
#if HAVE_PTHREAD_H
#include <pthread.h>
#endif
#include "SmartPointer.h"
#include "PointerPool.h"
#include "PoolPointer.h"
typedef uint8_t byte;
namespace Tools
{
SIDX_DLL enum IntervalType
{
IT_RIGHTOPEN = 0x0,
IT_LEFTOPEN,
IT_OPEN,
IT_CLOSED
};
SIDX_DLL enum VariantType
{
VT_LONG = 0x0,
VT_BYTE,
VT_SHORT,
VT_FLOAT,
VT_DOUBLE,
VT_CHAR,
VT_USHORT,
VT_ULONG,
VT_INT,
VT_UINT,
VT_BOOL,
VT_PCHAR,
VT_PVOID,
VT_EMPTY,
VT_LONGLONG,
VT_ULONGLONG,
VT_PWCHAR
};
SIDX_DLL enum FileMode
{
APPEND = 0x0,
CREATE
};
//
// Exceptions
//
class SIDX_DLL Exception
{
public:
virtual std::string what() = 0;
virtual ~Exception() {}
};
class SIDX_DLL IndexOutOfBoundsException : public Exception
{
public:
IndexOutOfBoundsException(size_t i);
virtual ~IndexOutOfBoundsException() {}
virtual std::string what();
private:
std::string m_error;
}; // IndexOutOfBoundsException
class SIDX_DLL IllegalArgumentException : public Exception
{
public:
IllegalArgumentException(std::string s);
virtual ~IllegalArgumentException() {}
virtual std::string what();
private:
std::string m_error;
}; // IllegalArgumentException
class SIDX_DLL IllegalStateException : public Exception
{
public:
IllegalStateException(std::string s);
virtual ~IllegalStateException() {}
virtual std::string what();
private:
std::string m_error;
}; // IllegalStateException
class SIDX_DLL EndOfStreamException : public Exception
{
public:
EndOfStreamException(std::string s);
virtual ~EndOfStreamException() {}
virtual std::string what();
private:
std::string m_error;
}; // EndOfStreamException
class SIDX_DLL ResourceLockedException : public Exception
{
public:
ResourceLockedException(std::string s);
virtual ~ResourceLockedException() {}
virtual std::string what();
private:
std::string m_error;
}; // ResourceLockedException
class SIDX_DLL NotSupportedException : public Exception
{
public:
NotSupportedException(std::string s);
virtual ~NotSupportedException() {}
virtual std::string what();
private:
std::string m_error;
}; // NotSupportedException
//
// Interfaces
//
class SIDX_DLL IInterval
{
public:
virtual ~IInterval() {}
virtual double getLowerBound() const = 0;
virtual double getUpperBound() const = 0;
virtual void setBounds(double, double) = 0;
virtual bool intersectsInterval(const IInterval&) const = 0;
virtual bool intersectsInterval(IntervalType type, const double start, const double end) const = 0;
virtual bool containsInterval(const IInterval&) const = 0;
virtual IntervalType getIntervalType() const = 0;
}; // IInterval
class SIDX_DLL IObject
{
public:
virtual ~IObject() {}
virtual IObject* clone() = 0;
// return a new object that is an exact copy of this one.
// IMPORTANT: do not return the this pointer!
}; // IObject
class SIDX_DLL ISerializable
{
public:
virtual ~ISerializable() {}
virtual uint32_t getByteArraySize() = 0;
// returns the size of the required byte array.
virtual void loadFromByteArray(const byte* data) = 0;
// load this object using the byte array.
virtual void storeToByteArray(byte** data, uint32_t& length) = 0;
// store this object in the byte array.
};
class SIDX_DLL IComparable
{
public:
virtual ~IComparable() {}
virtual bool operator<(const IComparable& o) const = 0;
virtual bool operator>(const IComparable& o) const = 0;
virtual bool operator==(const IComparable& o) const = 0;
}; //IComparable
class SIDX_DLL IObjectComparator
{
public:
virtual ~IObjectComparator() {}
virtual int compare(IObject* o1, IObject* o2) = 0;
}; // IObjectComparator
class SIDX_DLL IObjectStream
{
public:
virtual ~IObjectStream() {}
virtual IObject* getNext() = 0;
// returns a pointer to the next entry in the
// stream or 0 at the end of the stream.
virtual bool hasNext() = 0;
// returns true if there are more items in the stream.
virtual uint32_t size() = 0;
// returns the total number of entries available in the stream.
virtual void rewind() = 0;
// sets the stream pointer to the first entry, if possible.
}; // IObjectStream
//
// Classes & Functions
//
class SIDX_DLL Variant
{
public:
Variant();
VariantType m_varType;
union
{
int16_t iVal; // VT_SHORT
int32_t lVal; // VT_LONG
int64_t llVal; // VT_LONGLONG
byte bVal; // VT_BYTE
float fltVal; // VT_FLOAT
double dblVal; // VT_DOUBLE
char cVal; // VT_CHAR
uint16_t uiVal; // VT_USHORT
uint32_t ulVal; // VT_ULONG
uint64_t ullVal; // VT_ULONGLONG
bool blVal; // VT_BOOL
char* pcVal; // VT_PCHAR
void* pvVal; // VT_PVOID
wchar_t* pwcVal;
} m_val;
}; // Variant
class SIDX_DLL PropertySet;
SIDX_DLL std::ostream& operator<<(std::ostream& os, const Tools::PropertySet& p);
class SIDX_DLL PropertySet : public ISerializable
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
python 安装 Rtree 必不可少的库文件, libspatialindex改变从一个 LGPL 到一个 麻省理工学院 1.8.0 这个库的目的是提供: 一个可扩展的框架,将支持强大的空间索引 方法。 支持复杂的空间查询。 范围,点位置, 最近邻和再以及参数 查询(定义为空间约束)应该易于部署和运行。 易于使用的接口插入、删除和更新信息。 各种各样的定制功能。 基本的索引和存储 特征像页面大小、节点容量最低扇出, 分割算法等,应该很容易定制。 指数的持久性。 内存和外部内存结构 应该支持。 聚集和非聚集索引 很容易被持久化。
资源推荐
资源详情
资源评论
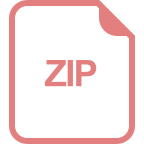
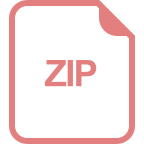
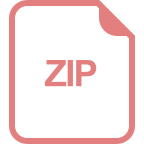
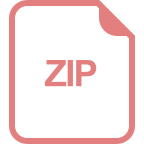
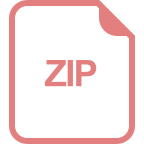
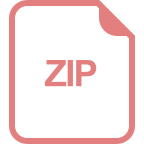
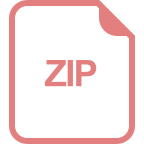
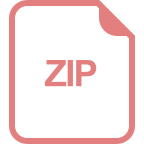
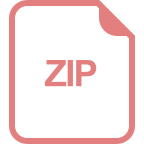
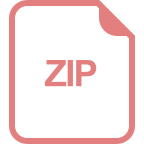
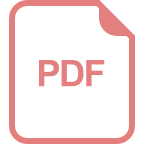
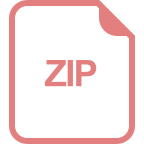
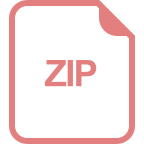
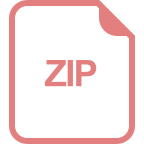
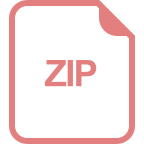
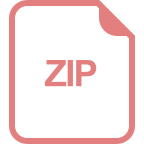
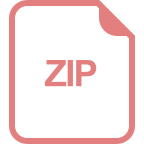
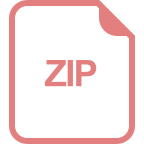
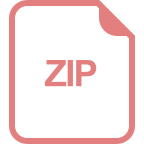
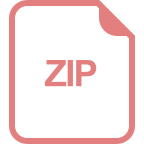
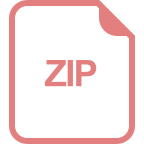
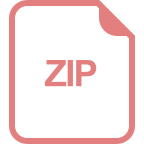
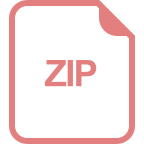
收起资源包目录



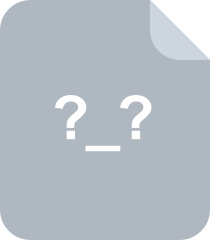
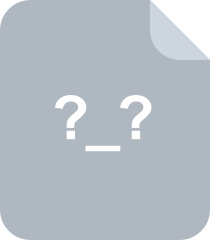


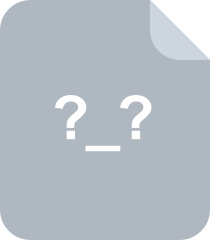
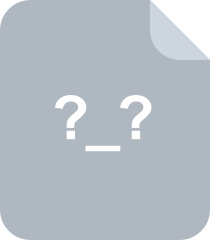
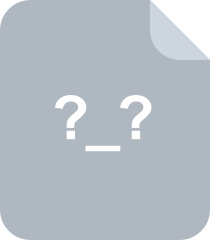
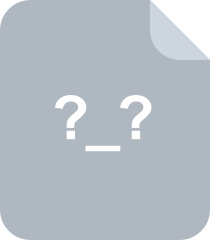
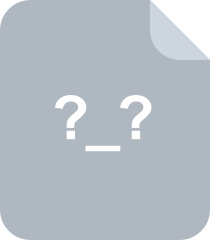

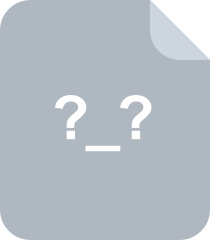
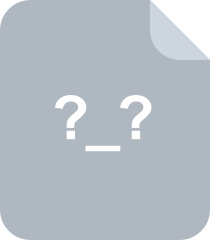
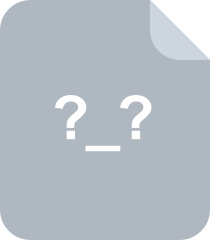
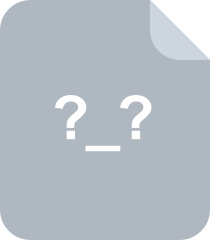
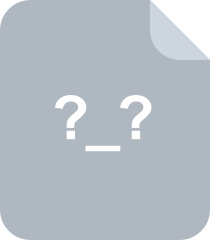

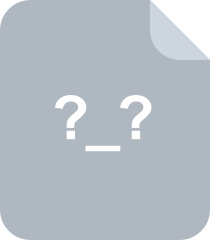
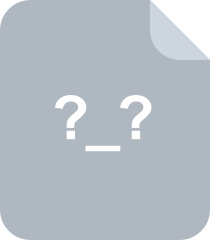
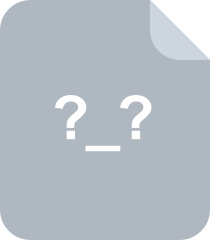
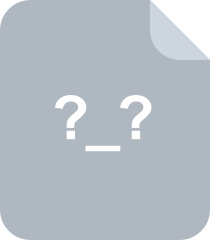
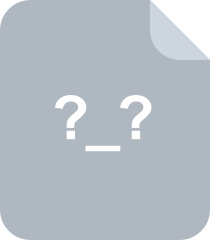
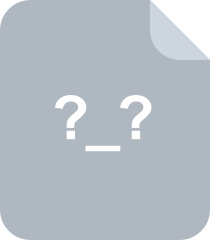
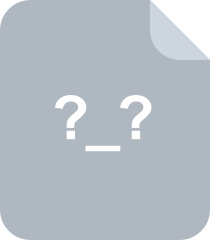
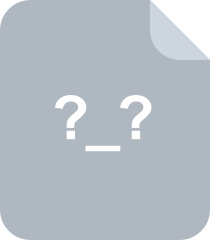
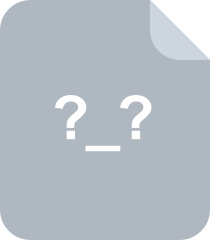
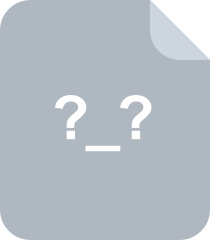
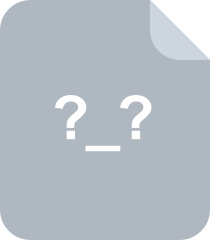
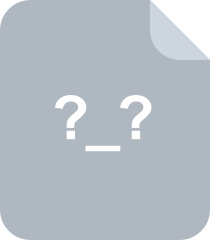
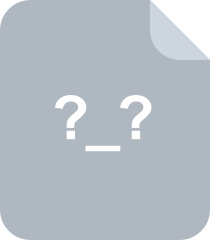
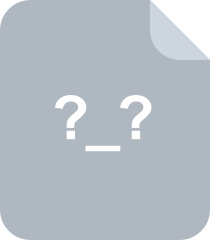
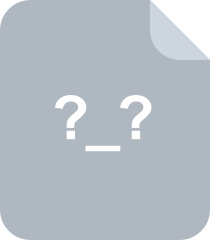
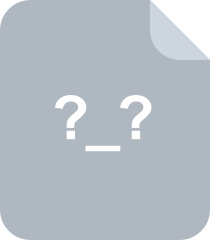
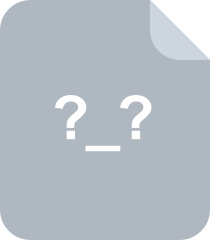
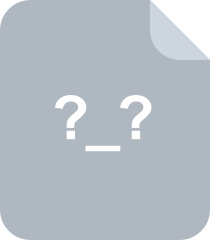
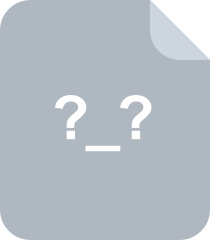
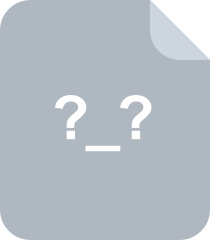
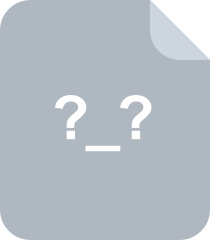
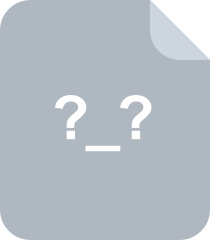

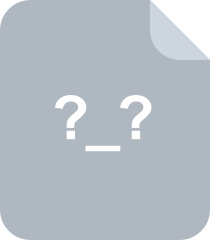
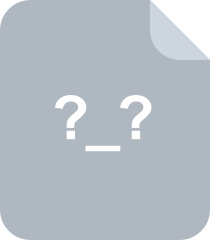
共 36 条
- 1
资源评论
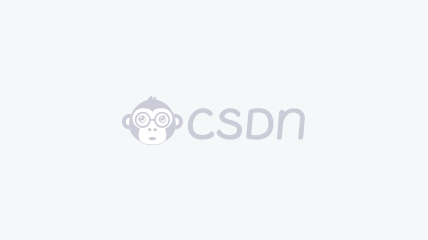

Mrchesian
- 粉丝: 139
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

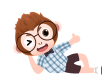
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


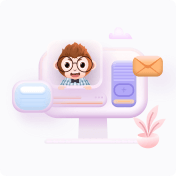
安全验证
文档复制为VIP权益,开通VIP直接复制
