函数名: abort 功 能: 异常终止一个进程 用 法: void abort(void); 程序例: #include <stdio.h> #include <stdlib.h> int main(void) { printf("Calling abort()\n"); abort(); return 0; /* This is never reached */ } 根据提供的文件信息,我们可以归纳出以下几个关键的C语言知识点: ### 1. `abort` 函数 **功能:** `abort` 函数用于异常终止一个进程。当调用该函数时,当前进程会立即终止,并返回一个错误信号给操作系统。 **语法:** ```c void abort(void); ``` **示例代码:** ```c #include <stdio.h> #include <stdlib.h> int main(void) { printf("Calling abort()\n"); abort(); return 0; /* 这一行永远不会执行 */ } ``` 在这个例子中,一旦`abort()`被调用,程序就会立即终止,因此`return 0`这一行将不会被执行。 ### 2. `abs` 函数 **功能:** `abs` 函数用于计算整型数值的绝对值。 **语法:** ```c int abs(int i); ``` **示例代码:** ```c #include <stdio.h> #include <math.h> int main(void) { int number = -1234; printf("number: %d absolute value: %d\n", number, abs(number)); return 0; } ``` 在这个示例中,`abs(number)` 计算了变量`number`的绝对值,并将其打印出来。 ### 3. `absread` 和 `abswrite` 函数 **功能:** `absread` 和 `abswrite` 函数用于直接读写磁盘扇区。 **语法:** ```c int absread(int drive, int nsects, int sectno, void *buffer); int abswrite(int drive, int nsects, int sectno, void *buffer); ``` **示例代码:** ```c /* absread 示例 */ #include <stdio.h> #include <conio.h> #include <process.h> #include <dos.h> int main(void) { int i, strt, ch_out, sector; char buf[512]; printf("Insert a diskette into drive A and press any key\n"); getch(); sector = 0; if (absread(0, 1, sector, &buf) != 0) { perror("Disk problem"); exit(1); } printf("Read OK\n"); strt = 3; for (i = 0; i < 80; i++) { ch_out = buf[strt + i]; putchar(ch_out); } printf("\n"); return 0; } ``` 这个示例演示了如何使用`absread`函数从磁盘读取数据到缓冲区`buf`中,并将部分内容打印出来。 ### 4. `access` 函数 **功能:** `access` 函数用于检查文件是否存在以及是否具有指定的访问权限。 **语法:** ```c int access(const char *filename, int amode); ``` **示例代码:** ```c #include <stdio.h> #include <io.h> int file_exists(char *filename); int main(void) { printf("Does NOTEXIST.FIL exist: %s\n", file_exists("NOTEXISTS.FIL") ? "YES" : "NO"); return 0; } int file_exists(char *filename) { return (access(filename, 0) == 0); } ``` 在此示例中,`access`函数被用来检测文件`NOTEXISTS.FIL`是否存在。如果文件存在,则返回0,否则返回非零值。 ### 5. `acos` 函数 **功能:** `acos` 函数用于计算一个数值的反余弦值。 **语法:** ```c double acos(double x); ``` **示例代码:** ```c #include <stdio.h> #include <math.h> int main(void) { double result; double x = 0.5; result = acos(x); printf("The arccosine of %lf is %lf\n", x, result); return 0; } ``` 在本示例中,我们计算了0.5的反余弦值,并将其打印出来。 ### 6. `allocmem` 函数 **功能:** `allocmem` 函数用于分配内存段。 **语法:** ```c int allocmem(unsigned size, unsigned *seg); ``` **示例代码:** ```c #include <dos.h> #include <alloc.h> #include <stdio.h> int main(void) { unsigned int size, segp; int stat; size = 64; /* (64x16) = 1024 bytes */ stat = allocmem(size, &segp); if (stat == -1) printf("Allocated memory at segment: %x\n", segp); else printf("Failed: maximum number of paragraphs available is %u\n", stat); return 0; } ``` 此示例演示了如何使用`allocmem`函数来分配内存段。如果成功,将打印出分配的内存段地址;如果失败,则打印可用的最大段数。 ### 7. `arc` 函数 **功能:** `arc` 函数用于绘制弧线。 **语法:** ```c void far arc(int x, int y, int stangle, int endangle, int radius); ``` **示例代码:** ```c #include <graphics.h> #include <stdlib.h> #include <stdio.h> #include <conio.h> int main(void) { /* request auto detection */ int gdriver = DETECT, gmode, errorcode; int midx, midy; int stangle = 45, endangle = 135; int radius = 100; /* initialize graphics and local variables */ initgraph(&gdriver, &gmode, "C:\\TC\\BGI"); /* get the coordinates of the center of the screen */ midx = getmaxx() / 2; midy = getmaxy() / 2; /* draw an arc centered at the middle of the screen */ arc(midx, midy, stangle, endangle, radius); /* wait for user to press any key before exiting */ getch(); closegraph(); return 0; } ``` 此示例展示了如何使用`arc`函数绘制一段弧线,其起始角度为45度,结束角度为135度,半径为100像素。 这些函数在C语言编程中是非常基础且实用的,它们能够帮助开发者完成各种各样的任务,从简单的数学运算到复杂的文件操作和图形绘制等。理解并熟练掌握这些函数对于成为一名合格的C语言程序员至关重要。
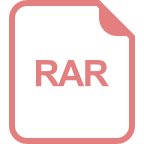
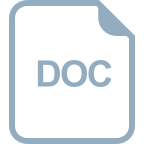
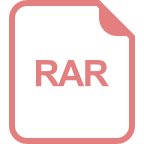
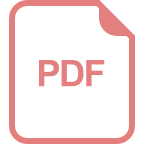
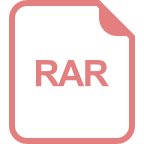
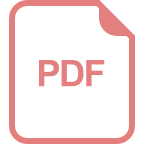
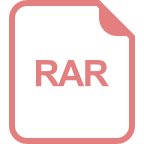
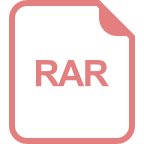
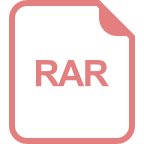
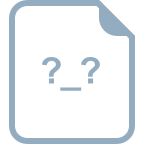
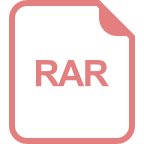
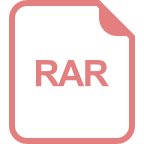
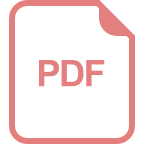
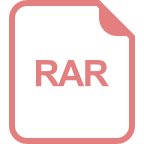
功 能: 异常终止一个进程
用 法: void abort(void);
程序例:
#include <stdio.h>
#include <stdlib.h>
int main(void)
{
printf("Calling abort()\n");
abort();
return 0; /* This is never reached */
}
函数名: abs
功 能: 求整数的绝对值
用 法: int abs(int i);
程序例:
#include <stdio.h>
#include <math.h>
int main(void)
{
int number = -1234;
printf("number: %d absolute value: %d\n", number, abs(number));
return 0;
}
函数名: absread, abswirte
功 能: 绝对磁盘扇区读、写数据
用 法: int absread(int drive, int nsects, int sectno, void *buffer);
int abswrite(int drive, int nsects, in tsectno, void *buffer);
程序例:
/* absread example */
#include <stdio.h>
#include <conio.h>
#include <process.h>
#include <dos.h>
int main(void)
{
int i, strt, ch_out, sector;
char buf[512];
printf("Insert a diskette into drive A and press any key\n");
getch();
sector = 0;
if (absread(0, 1, sector, &buf) != 0)
{
perror("Disk problem");
exit(1);
}
printf("Read OK\n");
strt = 3;
剩余13页未读,继续阅读
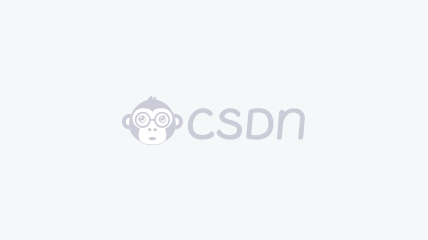

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

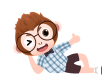
