/*
* | | | | \ \ / / | | | | / _______|
* | |____| | \ \/ / | |____| | / /
* | |____| | \ / | |____| | | | _____
* | | | | / \ | | | | | | |____ |
* | | | | / /\ \ | | | | \ \______| |
* | | | | /_/ \_\ | | | | \_________|
*
* Copyright (c) 2011 ~ 2017 Shenzhen HXHG. All rights reserved.
*/
#define JPUSH_VERSION_NUMBER 4.6.0
#import <Foundation/Foundation.h>
@class CLRegion;
@class UILocalNotification;
@class CLLocation;
@class UNNotificationCategory;
@class UNNotificationSettings;
@class UNNotificationRequest;
@class UNNotification;
@class UIView;
@protocol JPUSHRegisterDelegate;
@protocol JPUSHGeofenceDelegate;
@protocol JPUSHNotiInMessageDelegate;
typedef void (^JPUSHTagsOperationCompletion)(NSInteger iResCode, NSSet *iTags, NSInteger seq);
typedef void (^JPUSHTagValidOperationCompletion)(NSInteger iResCode, NSSet *iTags, NSInteger seq, BOOL isBind);
typedef void (^JPUSHAliasOperationCompletion)(NSInteger iResCode, NSString *iAlias, NSInteger seq);
extern NSString *const kJPFNetworkIsConnectingNotification; // 正在连接中
extern NSString *const kJPFNetworkDidSetupNotification; // 建立连接
extern NSString *const kJPFNetworkDidCloseNotification; // 关闭连接
extern NSString *const kJPFNetworkDidRegisterNotification; // 注册成功
extern NSString *const kJPFNetworkFailedRegisterNotification; //注册失败
extern NSString *const kJPFNetworkDidLoginNotification; // 登录成功
extern NSString *const kJPFNetworkDidReceiveMessageNotification; // 收到消息(非APNS)
extern NSString *const kJPFServiceErrorNotification; // 错误提示
typedef NS_OPTIONS(NSUInteger, JPAuthorizationOptions) {
JPAuthorizationOptionNone = 0, // the application may not present any UI upon a notification being received
JPAuthorizationOptionBadge = (1 << 0), // the application may badge its icon upon a notification being received
JPAuthorizationOptionSound = (1 << 1), // the application may play a sound upon a notification being received
JPAuthorizationOptionAlert = (1 << 2), // the application may display an alert upon a notification being received
JPAuthorizationOptionCarPlay = (1 << 3), // The ability to display notifications in a CarPlay environment.
JPAuthorizationOptionCriticalAlert NS_AVAILABLE_IOS(12.0) = (1 << 4) , //The ability to play sounds for critical alerts.
JPAuthorizationOptionProvidesAppNotificationSettings NS_AVAILABLE_IOS(12.0) = (1 << 5) , //An option indicating the system should display a button for in-app notification settings.
JPAuthorizationOptionProvisional NS_AVAILABLE_IOS(12.0) = (1 << 6) , //The ability to post noninterrupting notifications provisionally to the Notification Center.
JPAuthorizationOptionAnnouncement NS_AVAILABLE_IOS(13.0) = (1 << 7) , //The ability for Siri to automatically read out messages over AirPods.
};
typedef NS_ENUM(NSUInteger, JPAuthorizationStatus) {
JPAuthorizationNotDetermined = 0, // The user has not yet made a choice regarding whether the application may post user notifications.
JPAuthorizationStatusDenied, // The application is not authorized to post user notifications.
JPAuthorizationStatusAuthorized, // The application is authorized to post user notifications.
JPAuthorizationStatusProvisional NS_AVAILABLE_IOS(12.0), // The application is authorized to post non-interruptive user notifications.
};
/*!
* 通知注册实体类
*/
@interface JPUSHRegisterEntity : NSObject
/*!
* 支持的类型
* badge,sound,alert
*/
@property (nonatomic, assign) NSInteger types;
/*!
* 注入的类别
* iOS10 UNNotificationCategory
* iOS8-iOS9 UIUserNotificationCategory
*/
@property (nonatomic, strong) NSSet *categories;
@end
/*!
* 进行删除、查找推送实体类
*/
@interface JPushNotificationIdentifier : NSObject<NSCopying, NSCoding>
@property (nonatomic, copy) NSArray<NSString *> *identifiers; // 推送的标识数组
@property (nonatomic, copy) UILocalNotification *notificationObj NS_DEPRECATED_IOS(4_0, 10_0); // iOS10以下可以传UILocalNotification对象数据,iOS10以上无效
@property (nonatomic, assign) BOOL delivered NS_AVAILABLE_IOS(10_0); // 在通知中心显示的或待推送的标志,默认为NO,YES表示在通知中心显示的,NO表示待推送的
@property (nonatomic, copy) void (^findCompletionHandler)(NSArray *results); // 用于查询回调,调用[findNotification:]方法前必须设置,results为返回相应对象数组,iOS10以下返回UILocalNotification对象数组;iOS10以上根据delivered传入值返回UNNotification或UNNotificationRequest对象数组(delivered传入YES,则返回UNNotification对象数组,否则返回UNNotificationRequest对象数组)
@end
/*!
* 推送通知声音实体类
* iOS10以上有效
*/
@interface JPushNotificationSound : NSObject <NSCopying, NSCoding>
@property (nonatomic, copy) NSString *soundName; //普通通知铃声
@property (nonatomic, copy) NSString *criticalSoundName NS_AVAILABLE_IOS(12.0); //警告通知铃声
@property (nonatomic, assign) float criticalSoundVolume NS_AVAILABLE_IOS(12.0); //警告通知铃声音量,有效值在0~1之间,默认为1
@end
/*!
* 推送内容实体类
*/
@interface JPushNotificationContent : NSObject<NSCopying, NSCoding>
@property (nonatomic, copy) NSString *title; // 推送标题
@property (nonatomic, copy) NSString *subtitle; // 推送副标题
@property (nonatomic, copy) NSString *body; // 推送内容
@property (nonatomic, copy) NSNumber *badge; // 角标的数字。如果不需要改变角标传@(-1)
@property (nonatomic, copy) NSString *action NS_DEPRECATED_IOS(8_0, 10_0); // 弹框的按钮显示的内容(IOS 8默认为"打开", 其他默认为"启动",iOS10以上无效)
@property (nonatomic, copy) NSString *categoryIdentifier; // 行为分类标识
@property (nonatomic, copy) NSDictionary *userInfo; // 本地推送时可以设置userInfo来增加附加信息,远程推送时设置的payload推送内容作为此userInfo
@property (nonatomic, copy) NSString *sound; // 声音名称,不设置则为默认声音
@property (nonatomic, copy) JPushNotificationSound *soundSetting NS_AVAILABLE_IOS(10.0); //推送声音实体
@property (nonatomic, copy) NSArray *attachments NS_AVAILABLE_IOS(10_0); // 附件,iOS10以上有效,需要传入UNNotificationAttachment对象数组类型
@property (nonatomic, copy) NSString *threadIdentifier NS_AVAILABLE_IOS(10_0); // 线程或与推送请求相关对话的标识,iOS10以上有效,可用来对推送进行分组
@property (nonatomic, copy) NSString *launchImageName NS_AVAILABLE_IOS(10_0); // 启动图片名,iOS10以上有效,从推送启动时将会用到
@property (nonatomic, copy) NSString *summaryArgument NS_AVAILABLE_IOS(12.0); //插入到通知摘要中的部分参数。iOS12以上有效。
@property (nonatomic, assign) NSUInteger summaryArgumentCount NS_AVAILABLE_IOS(12.0); //插入到通知摘要中的项目数。iOS12以上有效。
@property (nonatomic, copy) NSString *targetContentIdentifier NS_AVAILABLE_IOS(13.0); // An identifier for the content of the notification used by the system to customize the scene to be activated when tapping on a notification.
//iOS15以上的新增属性 interruptionLevel为枚举UNNotificationInterruptionLevel
// The interruption level determines the degree of interruption associated with the notification
@property (nonatomic, assign) NSUInteger interruptionLevel NS_AVAILABLE_IOS(15.0);
// Relevance score determines the sorting for the notification across app notifications. The expected range is between 0.0f and 1.0f.
@property (nonatomic, assign) double relevanceScore NS_AVAILABLE_IOS(15.0);
@end
/*!
* 推送触发方式实体类
* 注:dateComponents、timeInterval�
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录



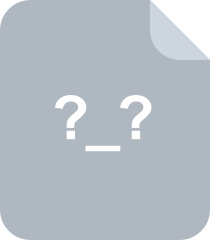
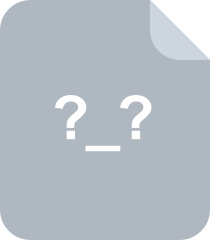

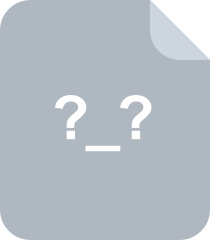

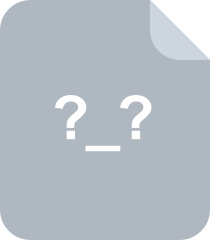
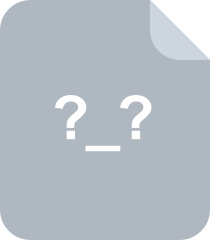
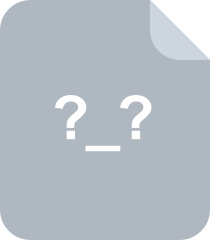
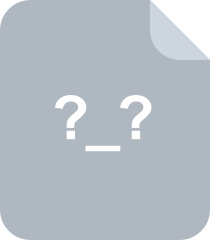
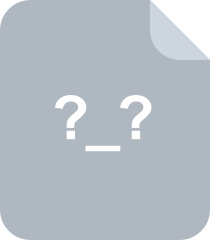
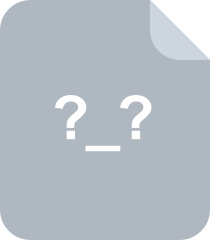

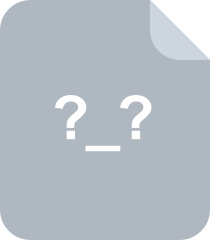
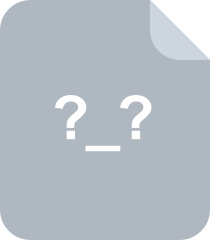
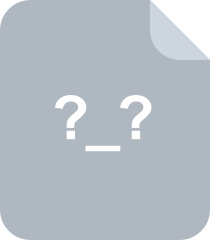
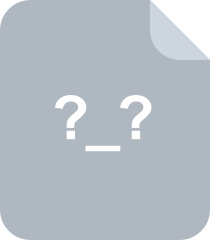
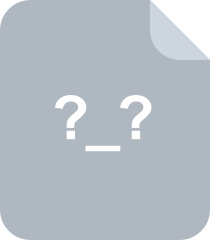
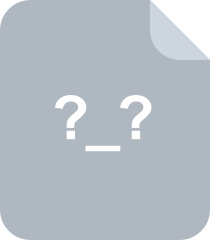
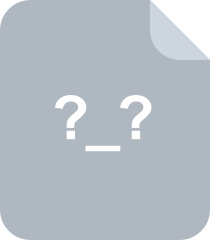
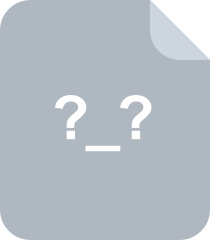
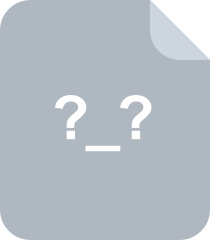
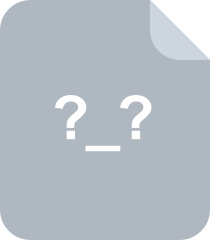
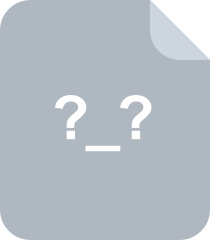
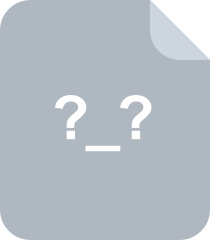

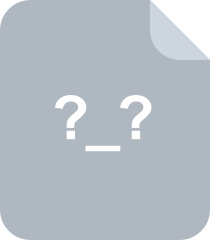
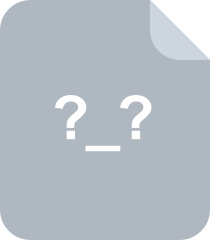
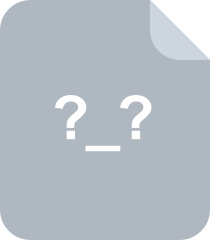


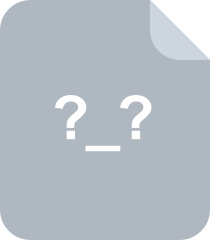

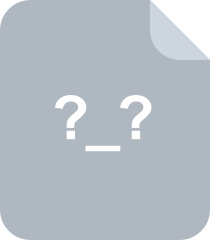
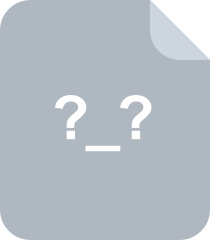



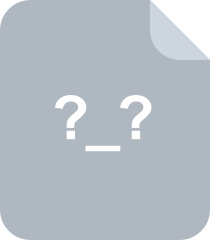
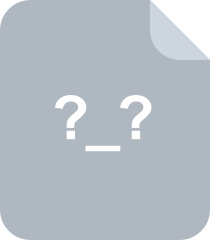


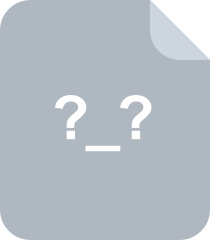
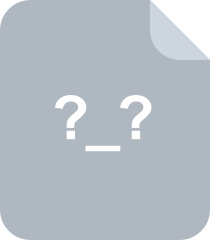



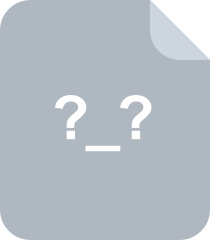
共 32 条
- 1
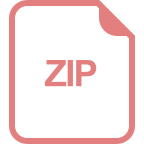
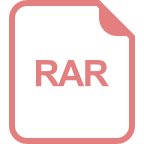
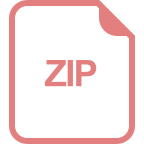
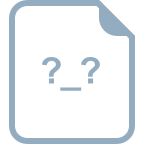
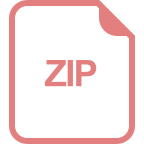
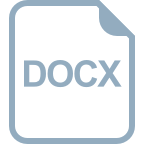
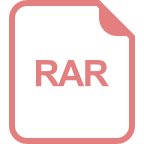
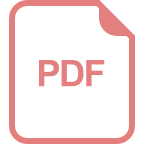
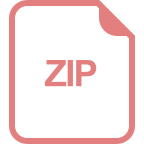
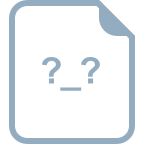
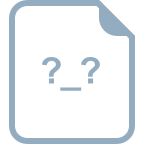
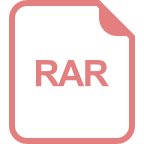
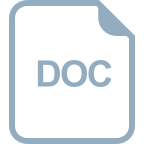
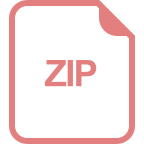
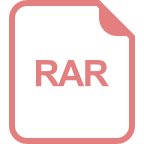
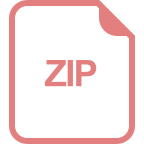
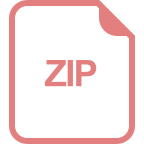
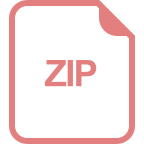
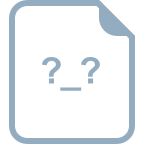

晒干的老咸鱼
- 粉丝: 9957
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

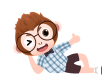
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0