/**
* Copyright (c) 2007, Gaudenz Alder
*/
package com.mxgraph.view;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.Shape;
import java.beans.PropertyChangeListener;
import java.beans.PropertyChangeSupport;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.w3c.dom.Element;
import com.mxgraph.canvas.mxGraphics2DCanvas;
import com.mxgraph.canvas.mxICanvas;
import com.mxgraph.canvas.mxImageCanvas;
import com.mxgraph.model.mxCell;
import com.mxgraph.model.mxGeometry;
import com.mxgraph.model.mxGraphModel;
import com.mxgraph.model.mxGraphModel.Filter;
import com.mxgraph.model.mxGraphModel.mxChildChange;
import com.mxgraph.model.mxGraphModel.mxCollapseChange;
import com.mxgraph.model.mxGraphModel.mxGeometryChange;
import com.mxgraph.model.mxGraphModel.mxRootChange;
import com.mxgraph.model.mxGraphModel.mxStyleChange;
import com.mxgraph.model.mxGraphModel.mxTerminalChange;
import com.mxgraph.model.mxGraphModel.mxValueChange;
import com.mxgraph.model.mxGraphModel.mxVisibleChange;
import com.mxgraph.model.mxICell;
import com.mxgraph.model.mxIGraphModel;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxEvent;
import com.mxgraph.util.mxEventObject;
import com.mxgraph.util.mxEventSource;
import com.mxgraph.util.mxImageBundle;
import com.mxgraph.util.mxPoint;
import com.mxgraph.util.mxRectangle;
import com.mxgraph.util.mxResources;
import com.mxgraph.util.mxStyleUtils;
import com.mxgraph.util.mxUndoableEdit;
import com.mxgraph.util.mxUndoableEdit.mxUndoableChange;
import com.mxgraph.util.mxUtils;
/**
* Implements a graph object that allows to create diagrams from a graph model
* and stylesheet.
*
* <h3>Images</h3>
* To create an image from a graph, use the following code for a given
* XML document (doc) and File (file):
*
* <code>
* Image img = mxCellRenderer.createBufferedImage(
* graph, null, 1, Color.WHITE, false, null);
* ImageIO.write(img, "png", file);
* </code>
*
* If the XML is given as a string rather than a document, the document can
* be obtained using mxUtils.parse.
*
* This class fires the following events:
*
* mxEvent.ROOT fires if the root in the model has changed. This event has no
* properties.
*
* mxEvent.ALIGN_CELLS fires between begin- and endUpdate in alignCells. The
* <code>cells</code> and <code>align</code> properties contain the respective
* arguments that were passed to alignCells.
*
* mxEvent.FLIP_EDGE fires between begin- and endUpdate in flipEdge. The
* <code>edge</code> property contains the edge passed to flipEdge.
*
* mxEvent.ORDER_CELLS fires between begin- and endUpdate in orderCells. The
* <code>cells</code> and <code>back</code> properties contain the respective
* arguments that were passed to orderCells.
*
* mxEvent.CELLS_ORDERED fires between begin- and endUpdate in cellsOrdered.
* The <code>cells</code> and <code>back</code> arguments contain the
* respective arguments that were passed to cellsOrdered.
*
* mxEvent.GROUP_CELLS fires between begin- and endUpdate in groupCells. The
* <code>group</code>, <code>cells</code> and <code>border</code> arguments
* contain the respective arguments that were passed to groupCells.
*
* mxEvent.UNGROUP_CELLS fires between begin- and endUpdate in ungroupCells.
* The <code>cells</code> property contains the array of cells that was passed
* to ungroupCells.
*
* mxEvent.REMOVE_CELLS_FROM_PARENT fires between begin- and endUpdate in
* removeCellsFromParent. The <code>cells</code> property contains the array of
* cells that was passed to removeCellsFromParent.
*
* mxEvent.ADD_CELLS fires between begin- and endUpdate in addCells. The
* <code>cells</code>, <code>parent</code>, <code>index</code>,
* <code>source</code> and <code>target</code> properties contain the
* respective arguments that were passed to addCells.
*
* mxEvent.CELLS_ADDED fires between begin- and endUpdate in cellsAdded. The
* <code>cells</code>, <code>parent</code>, <code>index</code>,
* <code>source</code>, <code>target</code> and <code>absolute</code>
* properties contain the respective arguments that were passed to cellsAdded.
*
* mxEvent.REMOVE_CELLS fires between begin- and endUpdate in removeCells. The
* <code>cells</code> and <code>includeEdges</code> arguments contain the
* respective arguments that were passed to removeCells.
*
* mxEvent.CELLS_REMOVED fires between begin- and endUpdate in cellsRemoved.
* The <code>cells</code> argument contains the array of cells that was
* removed.
*
* mxEvent.SPLIT_EDGE fires between begin- and endUpdate in splitEdge. The
* <code>edge</code> property contains the edge to be splitted, the
* <code>cells</code>, <code>newEdge</code>, <code>dx</code> and
* <code>dy</code> properties contain the respective arguments that were passed
* to splitEdge.
*
* mxEvent.TOGGLE_CELLS fires between begin- and endUpdate in toggleCells. The
* <code>show</code>, <code>cells</code> and <code>includeEdges</code>
* properties contain the respective arguments that were passed to toggleCells.
*
* mxEvent.FOLD_CELLS fires between begin- and endUpdate in foldCells. The
* <code>collapse</code>, <code>cells</code> and <code>recurse</code>
* properties contain the respective arguments that were passed to foldCells.
*
* mxEvent.CELLS_FOLDED fires between begin- and endUpdate in cellsFolded. The
* <code>collapse</code>, <code>cells</code> and <code>recurse</code>
* properties contain the respective arguments that were passed to cellsFolded.
*
* mxEvent.UPDATE_CELL_SIZE fires between begin- and endUpdate in
* updateCellSize. The <code>cell</code> and <code>ignoreChildren</code>
* properties contain the respective arguments that were passed to
* updateCellSize.
*
* mxEvent.RESIZE_CELLS fires between begin- and endUpdate in resizeCells. The
* <code>cells</code> and <code>bounds</code> properties contain the respective
* arguments that were passed to resizeCells.
*
* mxEvent.CELLS_RESIZED fires between begin- and endUpdate in cellsResized.
* The <code>cells</code> and <code>bounds</code> properties contain the
* respective arguments that were passed to cellsResized.
*
* mxEvent.MOVE_CELLS fires between begin- and endUpdate in moveCells. The
* <code>cells</code>, <code>dx</code>, <code>dy</code>, <code>clone</code>,
* <code>target</code> and <code>location</code> properties contain the
* respective arguments that were passed to moveCells.
*
* mxEvent.CELLS_MOVED fires between begin- and endUpdate in cellsMoved. The
* <code>cells</code>, <code>dx</code>, <code>dy</code> and
* <code>disconnect</code> properties contain the respective arguments that
* were passed to cellsMoved.
*
* mxEvent.CONNECT_CELL fires between begin- and endUpdate in connectCell. The
* <code>edge</code>, <code>terminal</code> and <code>source</code> properties
* contain the respective arguments that were passed to connectCell.
*
* mxEvent.CELL_CONNECTED fires between begin- and endUpdate in cellConnected.
* The <code>edge</code>, <code>terminal</code> and <code>source</code>
* properties contain the respective arguments that were passed to
* cellConnected.
*
* mxEvent.REPAINT fires if a repaint was requested by calling repaint. The
* <code>region</code> property contains the optional mxRectangle that was
* passed to repaint to define the dirty region.
*/
public class mxGraph extends mxEventSource
{
/**
* Adds required resources.
*/
static
{
try
{
mxResources.add("com.mxgraph.resources.graph");
}
catch (Exception e)
{
// ignore
}
}
/**
* Holds the version number of this release. Current version
* is 3.7.2.
*/
public static final String VERSION = "3.7.2";
/**
没有合适的资源?快使用搜索试试~ 我知道了~
jgraphx自动布局的demo
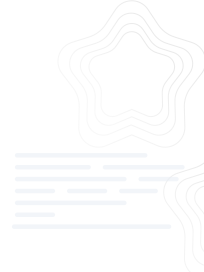
共1224个文件
class:637个
java:246个
gif:152个

需积分: 0 0 下载量 52 浏览量
2023-07-12
16:54:47
上传
评论
收藏 5.04MB ZIP 举报
温馨提示
jgraphx自动布局的demo
资源推荐
资源详情
资源评论
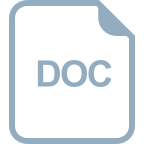
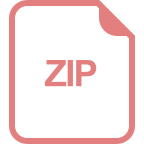
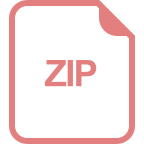
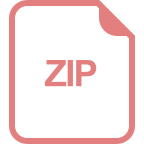
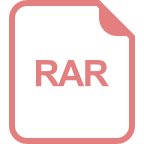
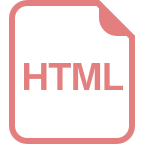
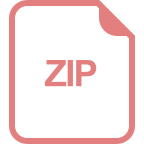
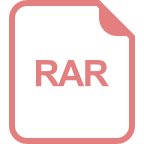
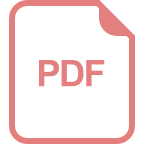
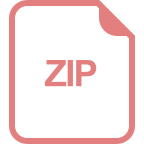
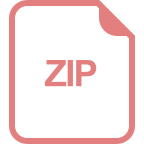
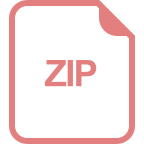
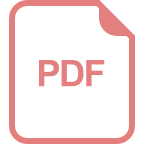
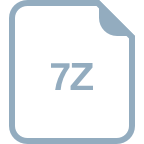
收起资源包目录

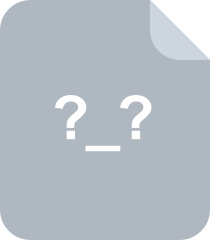
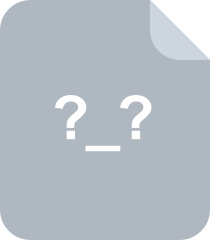
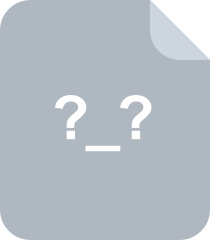
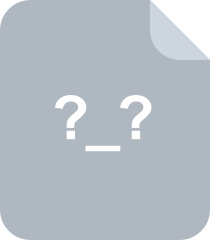
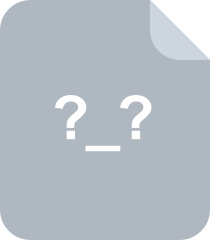
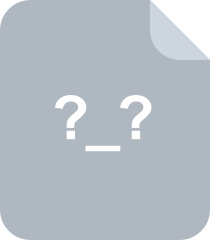
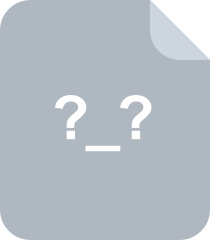
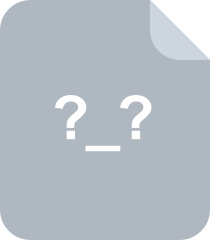
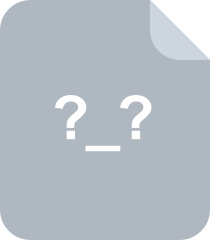
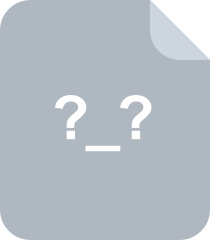
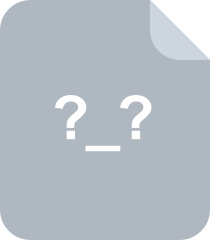
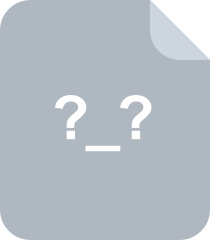
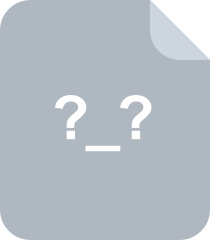
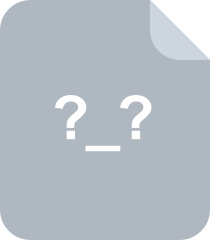
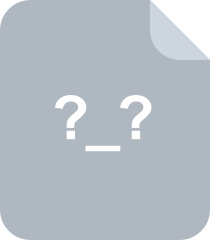
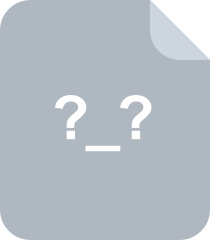
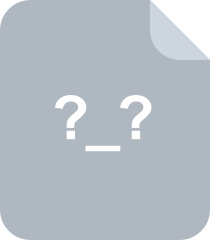
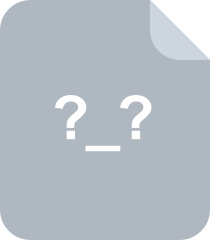
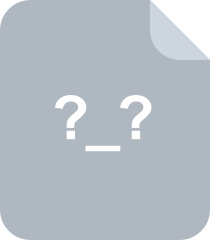
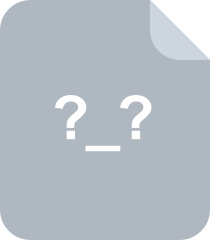
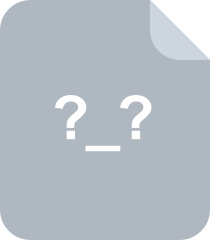
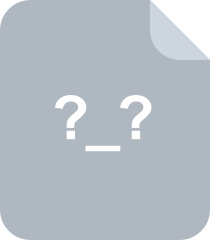
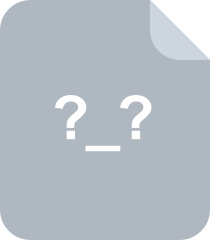
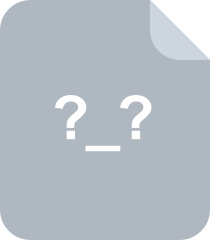
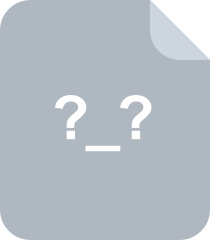
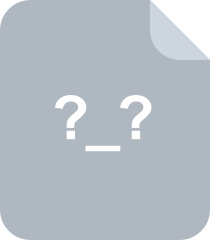
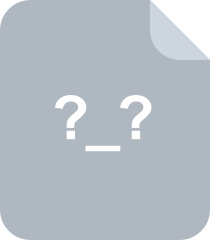
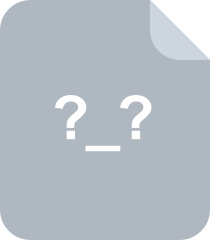
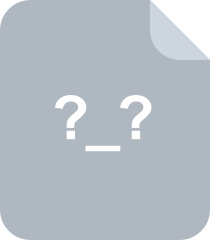
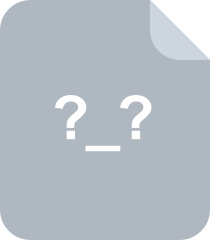
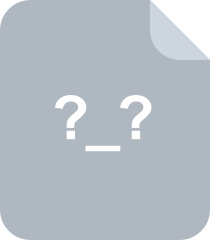
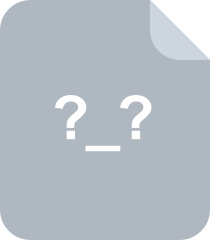
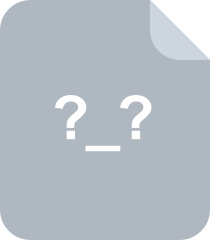
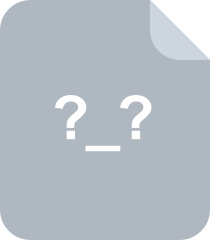
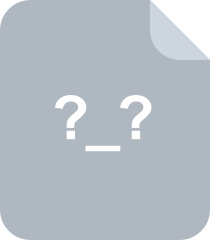
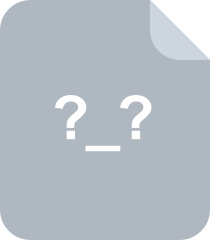
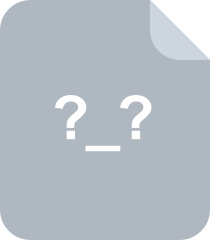
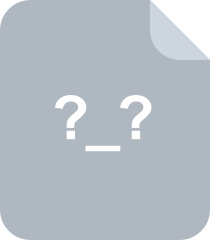
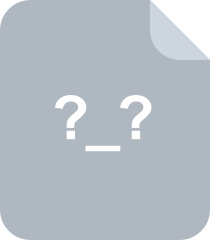
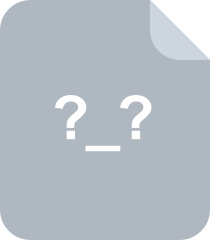
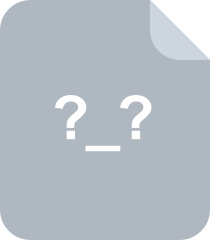
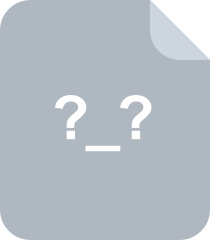
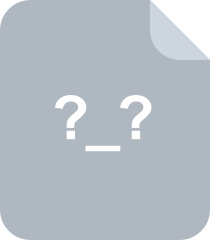
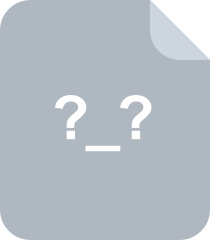
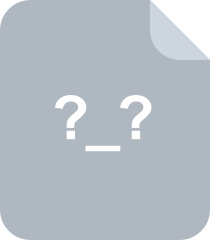
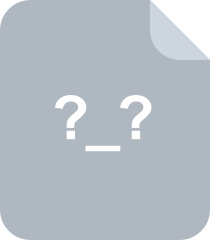
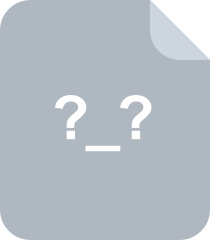
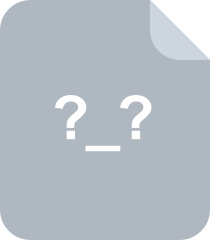
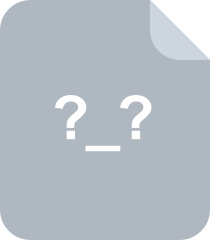
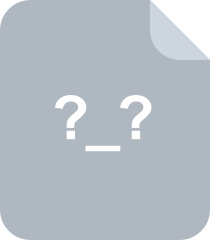
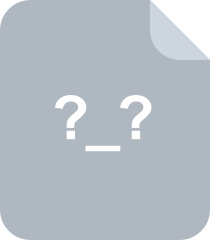
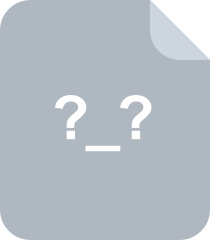
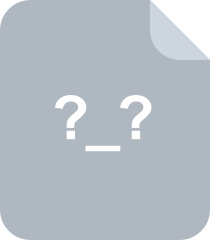
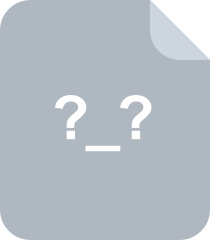
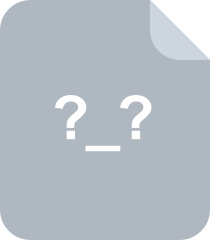
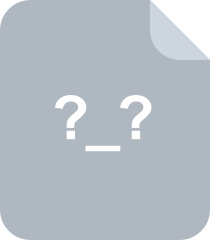
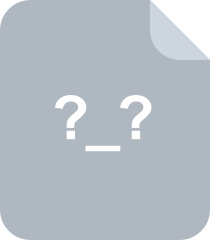
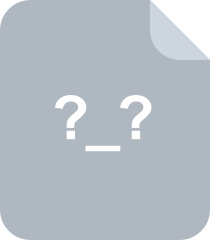
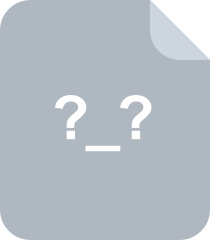
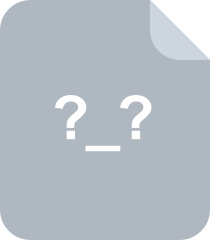
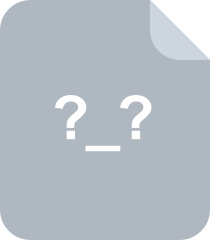
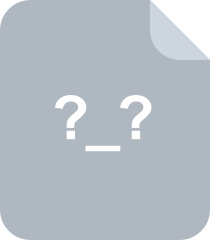
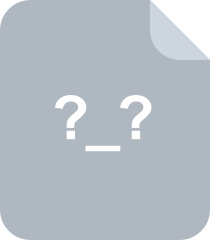
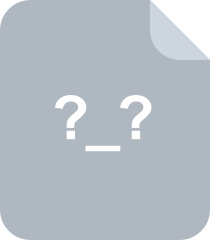
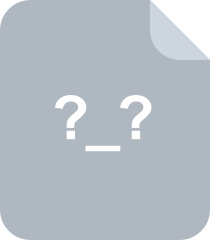
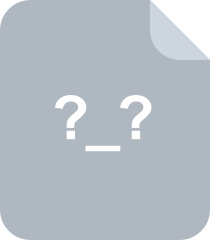
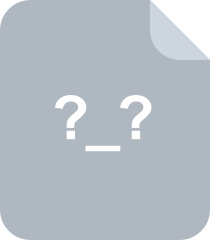
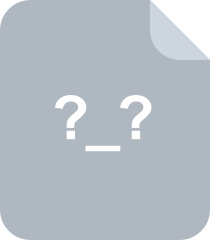
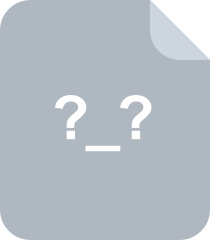
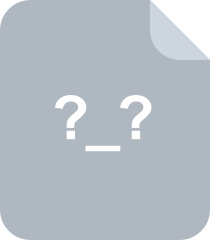
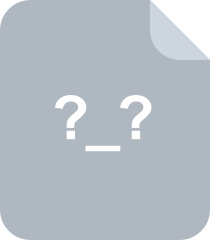
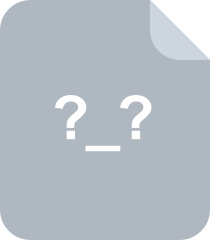
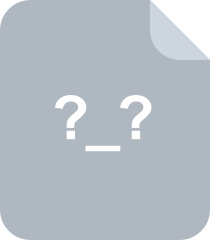
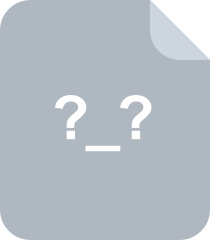
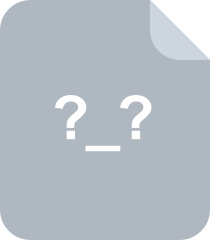
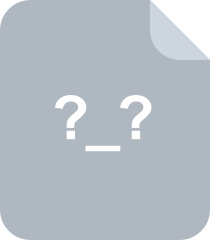
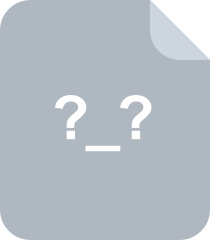
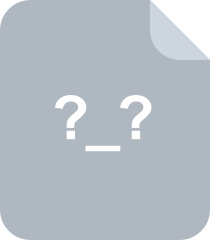
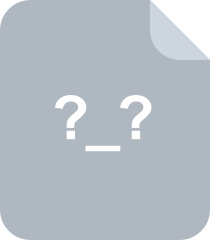
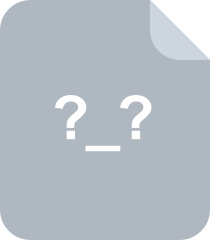
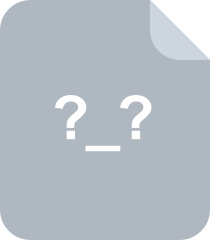
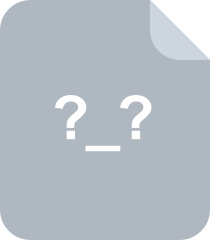
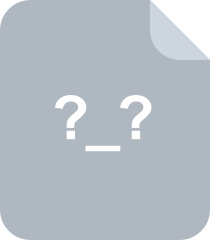
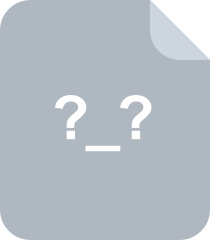
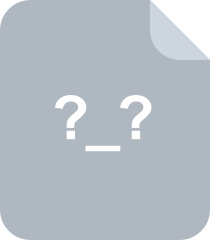
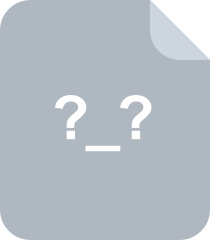
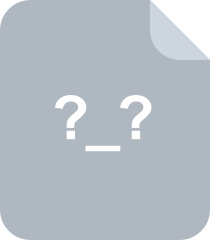
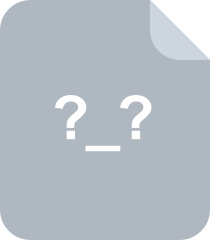
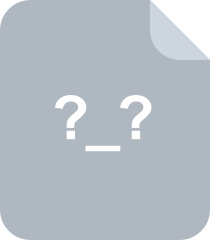
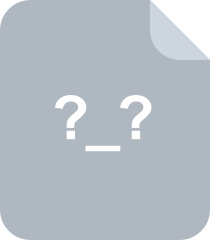
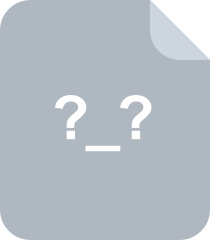
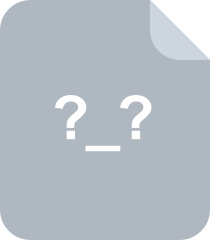
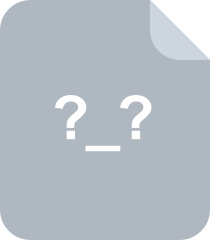
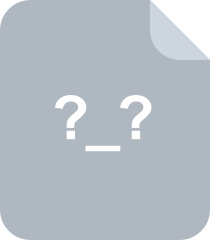
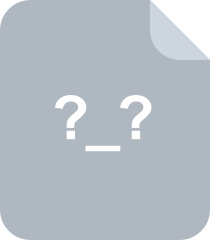
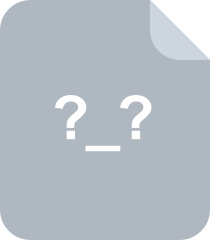
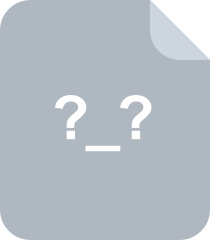
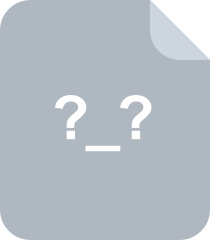
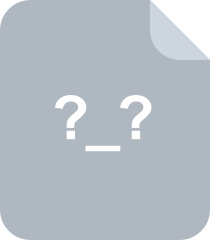
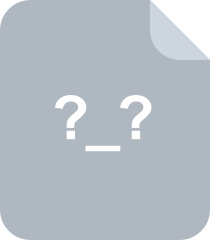
共 1224 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
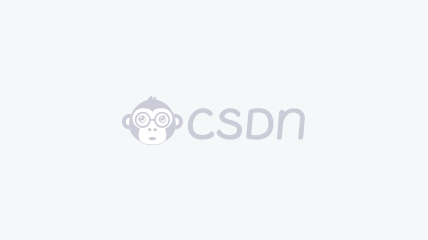

蔡陶军
- 粉丝: 4
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

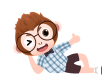
安全验证
文档复制为VIP权益,开通VIP直接复制
