<?php
/////////////////////////////////////////////////////////////////
/// getID3() by James Heinrich <info@getid3.org> //
// available at https://github.com/JamesHeinrich/getID3 //
// or https://www.getid3.org //
// or http://getid3.sourceforge.net //
// see readme.txt for more details //
/////////////////////////////////////////////////////////////////
// //
// module.audio-video.quicktime.php //
// module for analyzing Quicktime and MP3-in-MP4 files //
// dependencies: module.audio.mp3.php //
// dependencies: module.tag.id3v2.php //
// ///
/////////////////////////////////////////////////////////////////
if (!defined('GETID3_INCLUDEPATH')) { // prevent path-exposing attacks that access modules directly on public webservers
exit;
}
getid3_lib::IncludeDependency(GETID3_INCLUDEPATH.'module.audio.mp3.php', __FILE__, true);
getid3_lib::IncludeDependency(GETID3_INCLUDEPATH.'module.tag.id3v2.php', __FILE__, true); // needed for ISO 639-2 language code lookup
class getid3_quicktime extends getid3_handler
{
public $ReturnAtomData = true;
public $ParseAllPossibleAtoms = false;
/**
* @return bool
*/
public function Analyze() {
$info = &$this->getid3->info;
$info['fileformat'] = 'quicktime';
$info['quicktime']['hinting'] = false;
$info['quicktime']['controller'] = 'standard'; // may be overridden if 'ctyp' atom is present
$this->fseek($info['avdataoffset']);
$offset = 0;
$atomcounter = 0;
$atom_data_read_buffer_size = $info['php_memory_limit'] ? round($info['php_memory_limit'] / 4) : $this->getid3->option_fread_buffer_size * 1024; // set read buffer to 25% of PHP memory limit (if one is specified), otherwise use option_fread_buffer_size [default: 32MB]
while ($offset < $info['avdataend']) {
if (!getid3_lib::intValueSupported($offset)) {
$this->error('Unable to parse atom at offset '.$offset.' because beyond '.round(PHP_INT_MAX / 1073741824).'GB limit of PHP filesystem functions');
break;
}
$this->fseek($offset);
$AtomHeader = $this->fread(8);
$atomsize = getid3_lib::BigEndian2Int(substr($AtomHeader, 0, 4));
$atomname = substr($AtomHeader, 4, 4);
// 64-bit MOV patch by jlegateØktnc*com
if ($atomsize == 1) {
$atomsize = getid3_lib::BigEndian2Int($this->fread(8));
}
if (($offset + $atomsize) > $info['avdataend']) {
$info['quicktime'][$atomname]['name'] = $atomname;
$info['quicktime'][$atomname]['size'] = $atomsize;
$info['quicktime'][$atomname]['offset'] = $offset;
$this->error('Atom at offset '.$offset.' claims to go beyond end-of-file (length: '.$atomsize.' bytes)');
return false;
}
if ($atomsize == 0) {
// Furthermore, for historical reasons the list of atoms is optionally
// terminated by a 32-bit integer set to 0. If you are writing a program
// to read user data atoms, you should allow for the terminating 0.
$info['quicktime'][$atomname]['name'] = $atomname;
$info['quicktime'][$atomname]['size'] = $atomsize;
$info['quicktime'][$atomname]['offset'] = $offset;
break;
}
$atomHierarchy = array();
$parsedAtomData = $this->QuicktimeParseAtom($atomname, $atomsize, $this->fread(min($atomsize, $atom_data_read_buffer_size)), $offset, $atomHierarchy, $this->ParseAllPossibleAtoms);
$parsedAtomData['name'] = $atomname;
$parsedAtomData['size'] = $atomsize;
$parsedAtomData['offset'] = $offset;
if (in_array($atomname, array('uuid'))) {
@$info['quicktime'][$atomname][] = $parsedAtomData;
} else {
$info['quicktime'][$atomname] = $parsedAtomData;
}
$offset += $atomsize;
$atomcounter++;
}
if (!empty($info['avdataend_tmp'])) {
// this value is assigned to a temp value and then erased because
// otherwise any atoms beyond the 'mdat' atom would not get parsed
$info['avdataend'] = $info['avdataend_tmp'];
unset($info['avdataend_tmp']);
}
if (!empty($info['quicktime']['comments']['chapters']) && is_array($info['quicktime']['comments']['chapters']) && (count($info['quicktime']['comments']['chapters']) > 0)) {
$durations = $this->quicktime_time_to_sample_table($info);
for ($i = 0; $i < count($info['quicktime']['comments']['chapters']); $i++) {
$bookmark = array();
$bookmark['title'] = $info['quicktime']['comments']['chapters'][$i];
if (isset($durations[$i])) {
$bookmark['duration_sample'] = $durations[$i]['sample_duration'];
if ($i > 0) {
$bookmark['start_sample'] = $info['quicktime']['bookmarks'][($i - 1)]['start_sample'] + $info['quicktime']['bookmarks'][($i - 1)]['duration_sample'];
} else {
$bookmark['start_sample'] = 0;
}
if ($time_scale = $this->quicktime_bookmark_time_scale($info)) {
$bookmark['duration_seconds'] = $bookmark['duration_sample'] / $time_scale;
$bookmark['start_seconds'] = $bookmark['start_sample'] / $time_scale;
}
}
$info['quicktime']['bookmarks'][] = $bookmark;
}
}
if (isset($info['quicktime']['temp_meta_key_names'])) {
unset($info['quicktime']['temp_meta_key_names']);
}
if (!empty($info['quicktime']['comments']['location.ISO6709'])) {
// https://en.wikipedia.org/wiki/ISO_6709
foreach ($info['quicktime']['comments']['location.ISO6709'] as $ISO6709string) {
$ISO6709parsed = array('latitude'=>false, 'longitude'=>false, 'altitude'=>false);
if (preg_match('#^([\\+\\-])([0-9]{2}|[0-9]{4}|[0-9]{6})(\\.[0-9]+)?([\\+\\-])([0-9]{3}|[0-9]{5}|[0-9]{7})(\\.[0-9]+)?(([\\+\\-])([0-9]{3}|[0-9]{5}|[0-9]{7})(\\.[0-9]+)?)?/$#', $ISO6709string, $matches)) {
@list($dummy, $lat_sign, $lat_deg, $lat_deg_dec, $lon_sign, $lon_deg, $lon_deg_dec, $dummy, $alt_sign, $alt_deg, $alt_deg_dec) = $matches;
if (strlen($lat_deg) == 2) { // [+-]DD.D
$ISO6709parsed['latitude'] = (($lat_sign == '-') ? -1 : 1) * floatval(ltrim($lat_deg, '0').$lat_deg_dec);
} elseif (strlen($lat_deg) == 4) { // [+-]DDMM.M
$ISO6709parsed['latitude'] = (($lat_sign == '-') ? -1 : 1) * floatval(ltrim(substr($lat_deg, 0, 2), '0')) + floatval(ltrim(substr($lat_deg, 2, 2), '0').$lat_deg_dec / 60);
} elseif (strlen($lat_deg) == 6) { // [+-]DDMMSS.S
$ISO6709parsed['latitude'] = (($lat_sign == '-') ? -1 : 1) * floatval(ltrim(substr($lat_deg, 0, 2), '0')) + floatval(ltrim(substr($lat_deg, 2, 2), '0') / 60) + floatval(ltrim(substr($lat_deg, 4, 2), '0').$lat_deg_dec / 3600);
}
if (strlen($lon_deg) == 3) { // [+-]DDD.D
$ISO6709parsed['longitude'] = (($lon_sign == '-') ? -1 : 1) * floatval(ltrim($lon_deg, '0').$lon_deg_dec);
} elseif (strlen($lon_deg) == 5) { // [+-]DDDMM.M
$ISO6709parsed['longitude'] = (($lon_sign == '-') ? -1 : 1) * floatval(ltrim(substr($lon_deg, 0, 2), '0')) + floatval(ltrim(substr($lon_deg, 2, 2), '0').$lon_deg_dec / 60);
} elseif (strlen($lon_deg) == 7) { // [+-]DDDMMSS.S
$ISO6709parsed['longitude'] = (($lon_sign == '-') ? -1 : 1) * floatval(ltrim(substr($lon_deg, 0, 2), '0')) + floatval(ltrim(substr($lon_deg, 2, 2), '0') / 60) + floatval(ltrim(substr($lon_deg, 4, 2), '0').$lon_deg_dec / 3600);
}
if (strlen($alt_deg) == 3) { // [+-]DDD.D
$ISO6709parsed['altitude'] = (($alt_sign == '-') ? -1 : 1) * floatval(ltrim($alt_deg, '0').$alt_deg_dec);
} elseif (strlen($alt_deg) == 5) { // [+-]DDDMM.M
$ISO6709parsed['altitude'] = (($alt_sign == '-') ? -1 : 1) * floatval(ltrim(substr($alt_deg, 0, 2), '0')) + floatval(ltrim(substr($alt_deg, 2, 2), '0').$alt_deg_dec / 60);
} elseif (strlen($alt_deg) == 7) { // [+-]DDDMMSS.S
$ISO6709parsed['altitude'] = (($alt_sign == '-') ? -1 : 1) * floatval(ltrim(substr($alt_deg, 0, 2), '0')) + floa
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
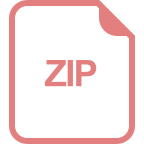
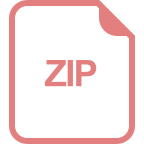
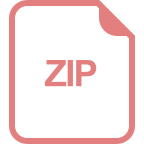
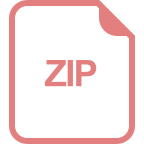
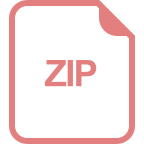
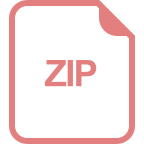
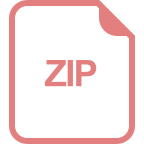
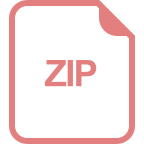
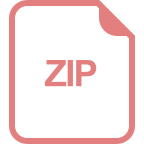
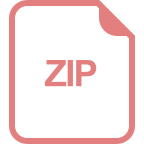
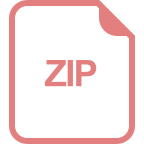
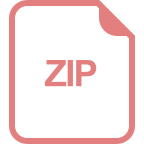
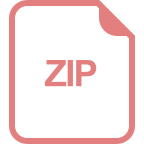
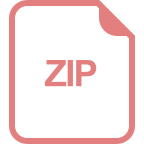
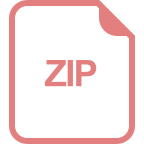
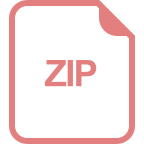
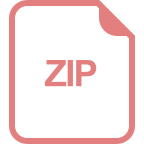
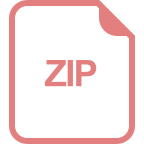
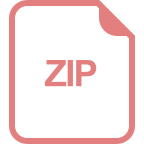
收起资源包目录

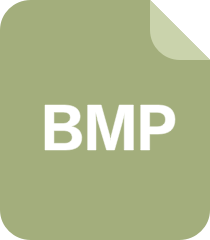
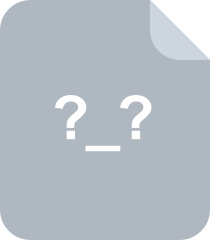
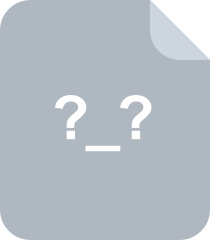
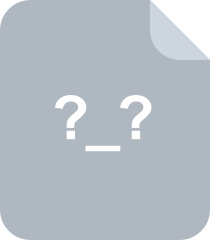
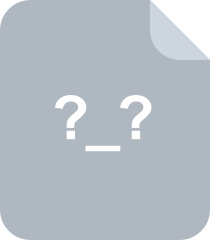
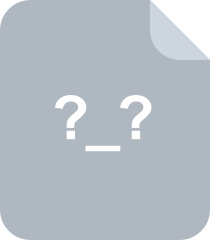
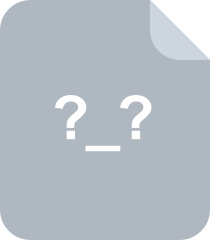
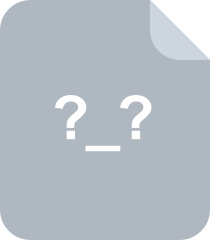
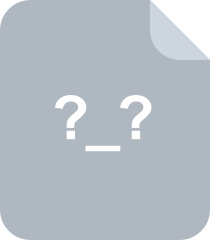
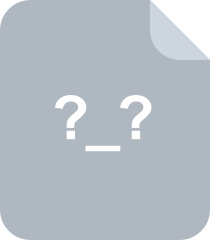
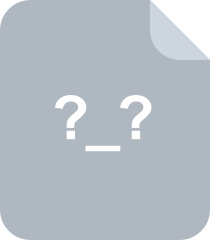
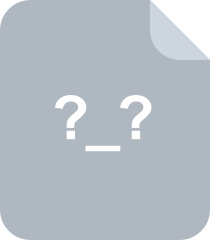
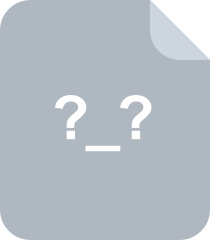
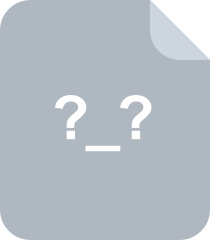
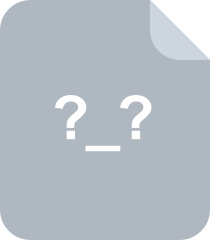
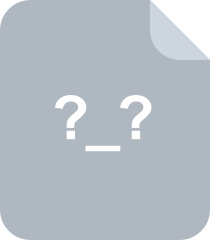
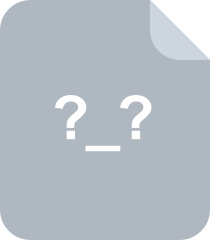
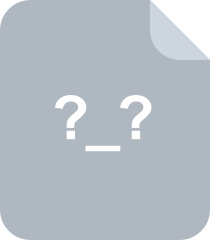
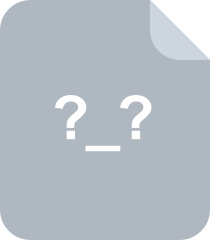
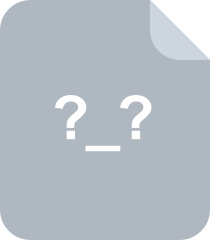
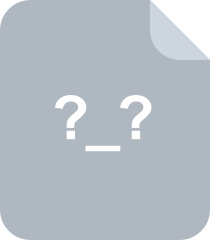
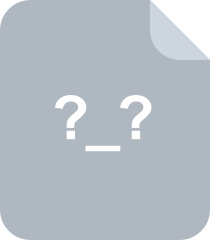
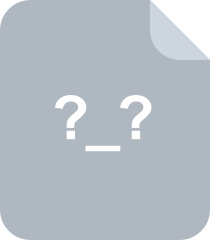
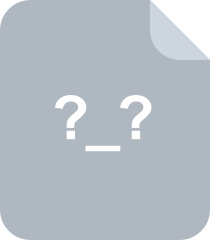
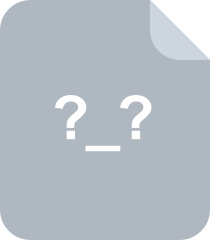
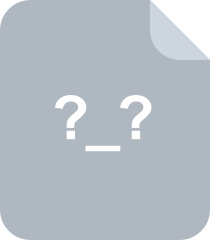
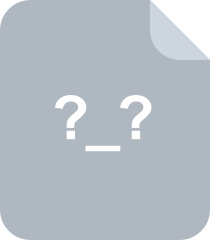
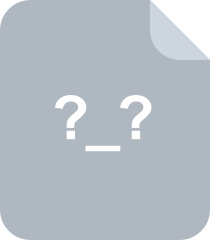
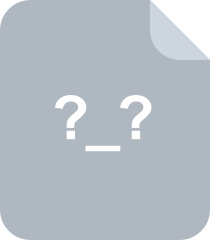
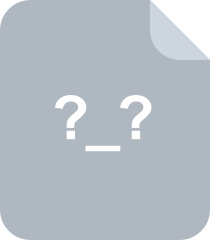
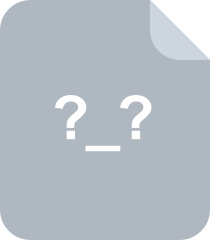
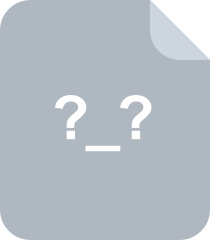
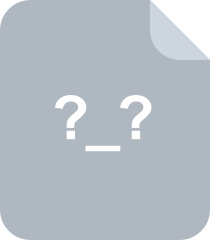
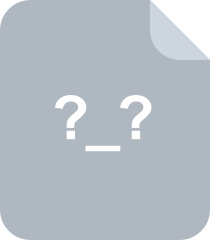
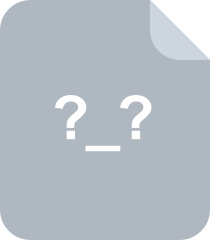
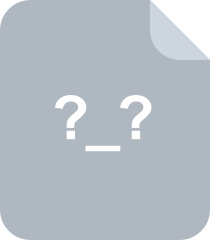
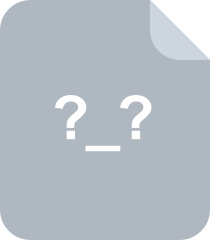
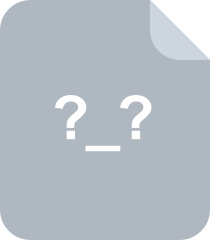
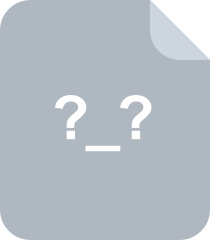
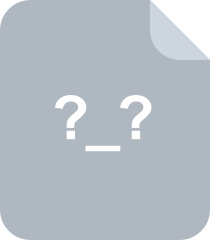
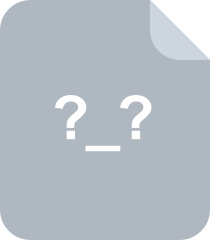
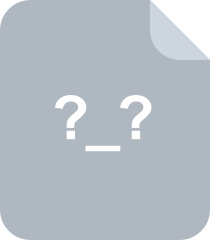
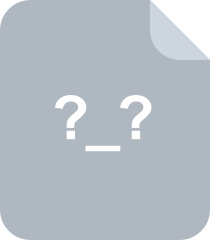
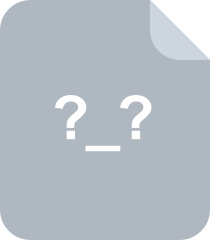
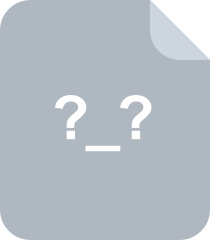
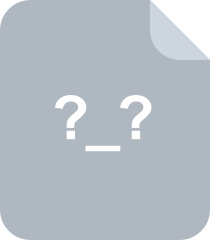
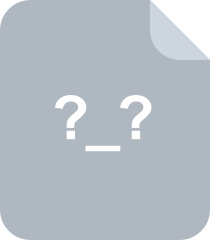
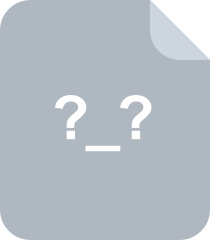
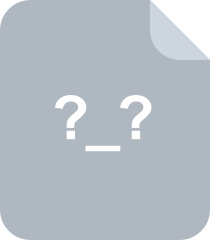
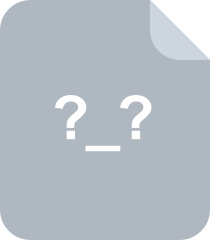
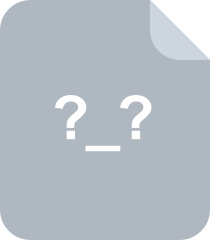
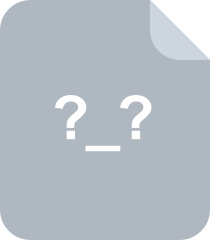
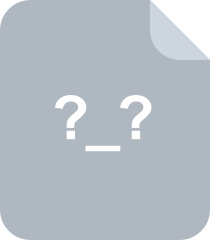
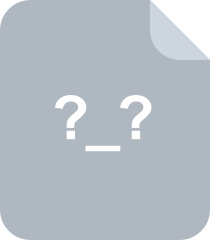
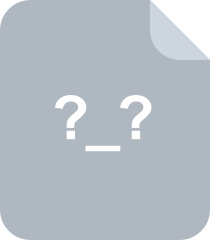
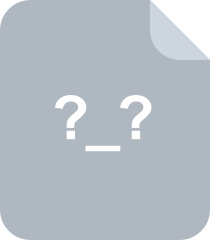
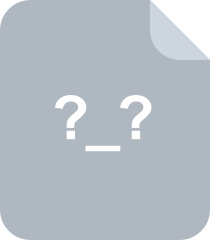
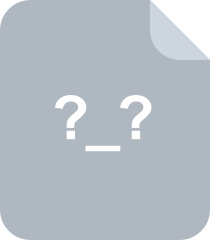
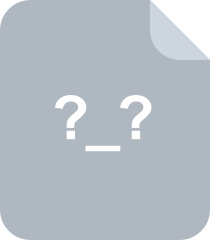
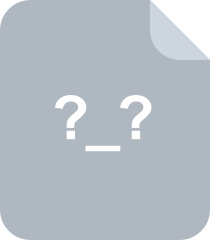
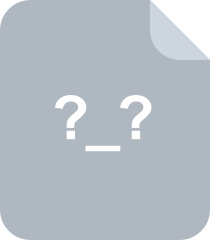
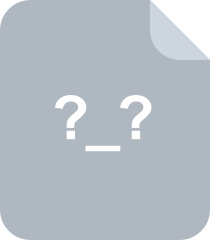
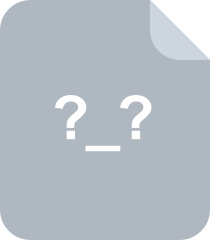
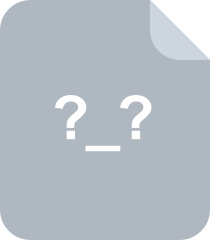
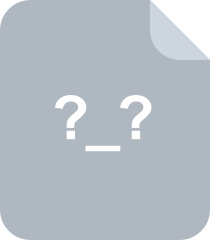
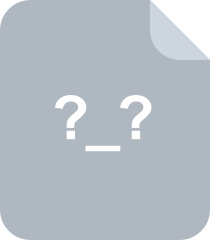
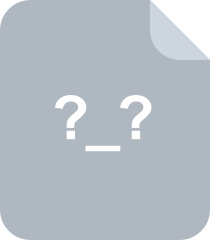
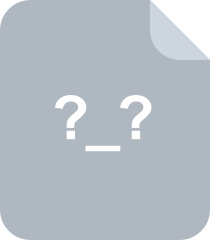
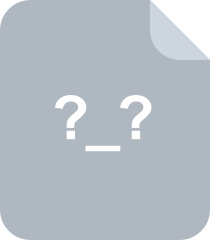
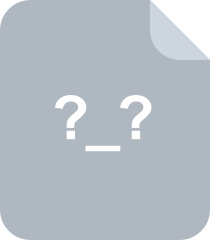
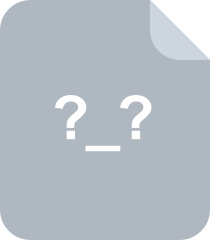
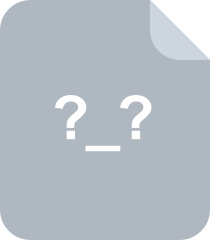
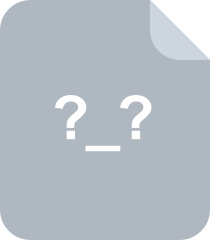
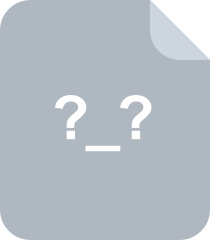
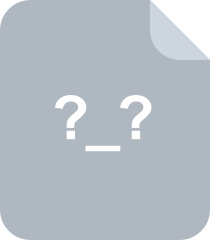
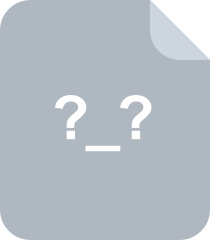
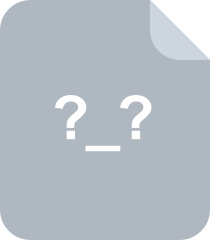
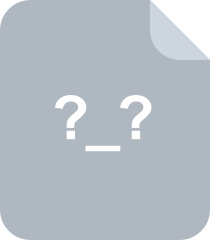
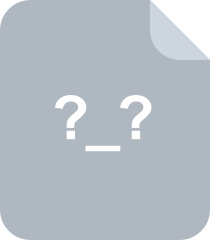
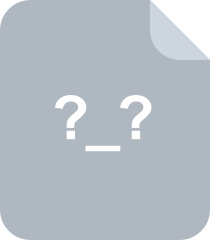
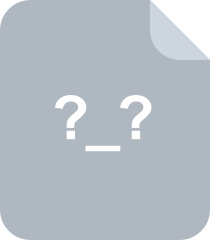
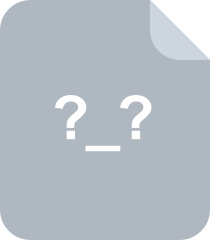
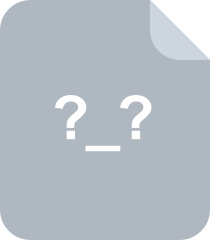
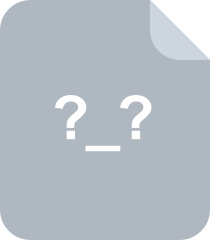
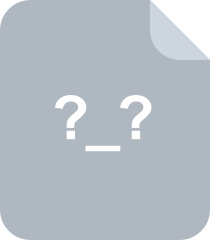
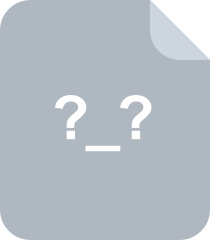
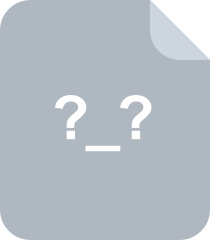
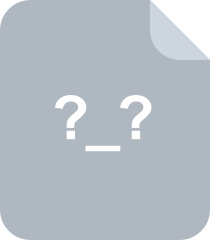
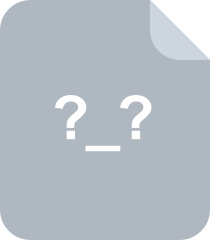
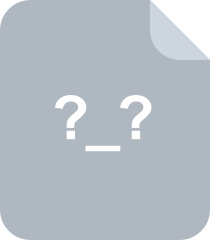
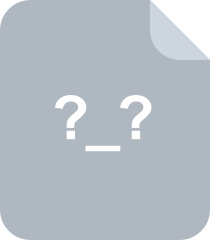
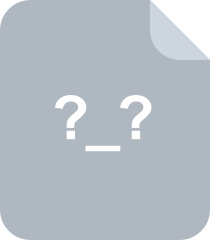
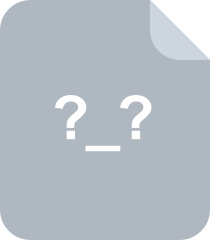
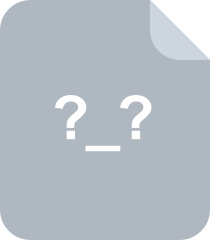
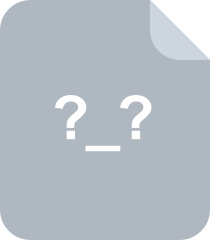
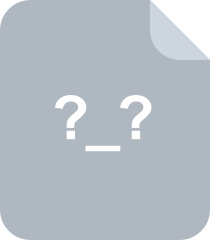
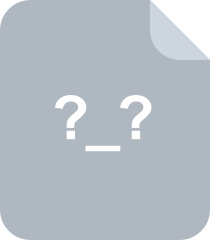
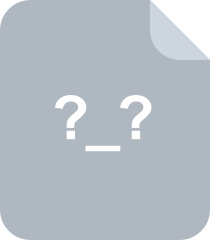
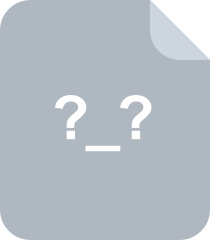
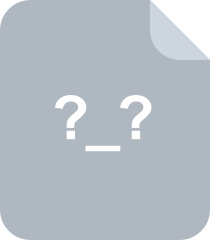
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
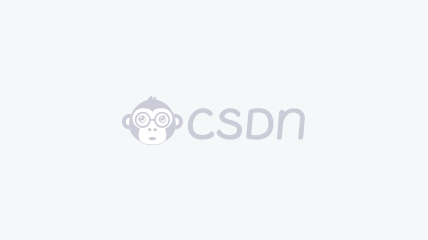

达达站长站
- 粉丝: 56
- 资源: 391
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

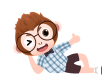
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


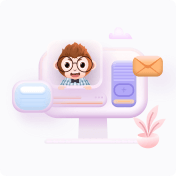
安全验证
文档复制为VIP权益,开通VIP直接复制
