clc;
clear;
close all;
warning off;
addpath(genpath(pwd));
if not(isfolder("figures"))
mkdir("figures")
end
%% (.p1) parameter sets for GFDM
gfdm = struct;
gfdm.K = 512; % Number of samples per sub-symbol
gfdm.M = 15; % Number of sub-symbols
gfdm.gfdmM = gfdm.M; % Accounts for OFDM simulation
gfdm.blockLength = gfdm.M*gfdm.K; % Block Length
gfdm.CP = 0.1; % Percentage of cyclic prefix
gfdm.pulse = 'rc'; % Pulse shaping filter (“rc” is raised cosine)
gfdm.a = 0.1; % Roll off factor of the pulse shaping filter
gfdm.mu = 4; % Modulation order of the QAM symbol
gfdm.subcarriers = 1:201; % Allocated subcarriers
gfdm.subsymbols = 2:15; % Allocated sub-symbols
gfdm.blocks = 10; % Number of blocks
%% (.p2) parameter sets for OFDM
ofdm = struct;
ofdm.K = 512;
ofdm.M = 1;
ofdm.gfdmM = gfdm.M;
ofdm.blockLength = ofdm.K;
ofdm.CP = 0.1;
ofdm.pulse = 'rc_td'; % (“rc_td” is a rectangular filter in frequency domain)
ofdm.a = 0.1;
ofdm.mu = 4;
ofdm.subcarriers = 1:201;
ofdm.subsymbols = 1;
ofdm.blocks = gfdm.blocks;
%% (.s1) Simulate GFDM and OFDM transmission
tic
[GFDMS, sym_gfdm_tx, cpx_gfdm_sym_tx, g1] = tx_simul(gfdm);
execution_tx_GFDM = toc;
tic
[OFDMS, sym_ofdm_tx, cpx_ofdm_sym_tx, g2] = tx_simul(ofdm);
execution_tx_OFDM = toc;
%% (.f1) Plot the PSD of the GFDM/OFDM signals
f = figure("Name", "PSD");
length = min(length(OFDMS), length(GFDMS));
t = linspace(-gfdm.K/2, gfdm.K/2, 2*length+1);
t = t(1:end-1)';
plot(t, mag2db(fftshift(abs(fft(OFDMS(1:length), 2*length))))/2, 'b');
hold on;
plot(t, mag2db(fftshift(abs(fft(GFDMS(1:length), 2*length))))/2, 'r');
hold off;
ylim([-40, 30]);
xlabel('f/F'); ylabel('PSD [dB]');
grid();
legend({'OFDM', 'GFDM'});
print(['figures' filesep 'PSD_gfdm_ofdm'], "-dpng");
set(f, 'visible', 'off');
%% (.s2) Simulate reception with different SNR values
snr = 1:21;
gfdm_ber = []; gfdm_ser = []; ofdm_ber = []; ofdm_ser = [];
execution_rx_GFDM = []; execution_rx_OFDM = [];
[theory_ber, theory_ser] = berawgn(snr, "qam", gfdm.mu^2);
for sii=snr
tic
[sym_rx1, cpx_sym_rx1, ber1, ser1] = rx_simul(gfdm, awgn(GFDMS, sii), sym_gfdm_tx, g1);
execution_rx_GFDM = [execution_rx_GFDM; toc];
tic
[sym_rx2, cpx_sym_rx2, ber2, ser2] = rx_simul(ofdm, awgn(OFDMS, sii), sym_ofdm_tx, g2);
execution_rx_OFDM = [execution_rx_OFDM; toc];
gfdm_ber = [gfdm_ber; ber1]; gfdm_ser = [gfdm_ser; ser1];
ofdm_ber = [ofdm_ber; ber2]; ofdm_ser = [ofdm_ser; ser2];
%% (.f2) Plot the Eye Diagrams/Scatter Plots for different SNR value
if mod(sii, 3) == 0
print_eye_scatter("gfdm", gfdm, cpx_sym_rx1, sii);
print_eye_scatter("ofdm", ofdm, cpx_sym_rx2, sii);
end
end
%% (.f3) Plot the BER/SER values of the GFDM/OFDM signals
print_ber_ser_q('SER', snr, theory_ser, gfdm_ser, ofdm_ser);
print_ber_ser_q('BER', snr, theory_ber, gfdm_ber, ofdm_ber);
print_ber_ser_q('QFactor', snr, [], 0.5*erfcinv(2*gfdm_ber), 0.5*erfcinv(2*ofdm_ber));
% (.es) Function that can print Eye Diagram, Scatter plot of different formats
function print_eye_scatter(name, opt, sym, sii)
f1 = eyediagram(sym(1:800), ceil(sqrt(opt.mu)));
print(strcat('figures', filesep, name, '_eye_snr_', string(sii)), "-dpng");
f2 = scatterplot(sym, 1, 0, 'r.');
hold on;
scatterplot(qammod(0:opt.mu^2-1, opt.mu^2, 'gray'), 1, 0, 'k*', f2);
title(strcat('Scatter plot,', {' '}, name, {', '}, 'SNR=', string(sii), 'dB'));
print(strcat('figures', filesep, name, '_scatter_snr_', string(sii)), "-dpng");
set(f1, 'visible', 'off');
set(f2, 'visible', 'off');
end
% (.bsq) Function that can print BER, SER, Q-VALUE of different formats
function print_ber_ser_q(name, snr, theory, gfdm, ofdm)
f = figure('Name', name);
if (size(theory) > 0)
semilogy(snr, theory,'-x');
hold on;
end
semilogy(snr, gfdm, 'o');
hold on;
semilogy(snr, ofdm, '*');
if (size(theory) > 0)
legend('theory', 'gfdm', 'ofdm');
else
legend('gfdm', 'ofdm');
end
xlabel('SNR, dB');
ylabel(name);
xlim([1, 20]);
print(['figures' filesep name], "-dpng");
set(f, 'visible', 'off');
end
% (.t) Simulation of transmitter GFDM/OFDM
function [signal, sym_tx, cpx_sym_tx, g] = tx_simul(opt)
signal = []; sym_tx = []; cpx_sym_tx = [];
% (.t1) Generate the transmitter pulse g
if strcmp(opt.pulse, 'rc')
t = linspace(-opt.M/2, opt.M/2, opt.M*opt.K + 1);
t = t(1:end-1);
t = t';
g = (sinc(t) .* cos(pi*opt.a*t) ./ (1-4*opt.a*opt.a*t.*t));
g = fftshift(g);
g(opt.K+1:opt.K:end) = 0;
g = g / sqrt(sum(g.*g));
elseif strcmp(opt.pulse, 'rc_td')
g = zeros(opt.M*opt.K, 1);
g(1:opt.K) = 1;
g = g / sqrt(sum(g.*g));
end
for b = 1:opt.blocks
gfdmM = 1;
% (.t2) OFDM case
if opt.M == 1
gfdmM = opt.gfdmM;
end
for m = 1 : gfdmM
% (.t3) Generate random data and map to QAM constellation
sym = randi(2 ^ opt.mu, length(opt.subsymbols) * length(opt.subcarriers), 1) - 1;
cpx_sym = qammod(sym, 2^opt.mu, 'gray')/ sqrt(2/3 * (2^opt.mu - 1));
sym_tx = [sym_tx; sym];
cpx_sym_tx = [cpx_sym_tx; cpx_sym];
% (.t4) Map data to correct dimensions or pad it with zeros
if length(opt.subcarriers)== opt.K && length(opt.subsymbols) == opt.M
D = reshape(cpx_sym, opt.K, opt.M);
else
Dm = reshape(cpx_sym, length(opt.subcarriers), length(opt.subsymbols));
res1 = zeros(opt.K, length(opt.subsymbols));
res1(opt.subcarriers, :) = Dm;
res = zeros(opt.K, opt.M);
res(:, opt.subsymbols) = res1;
D = res;
end
% (.t5) Modulate in time
DD = repmat(opt.K*ifft(D), opt.M, 1);
x = zeros(opt.K*opt.M, 1);
for m=1:opt.M
symbol = DD(:, m) .* g;
symbol = circshift(symbol, opt.K*(m-1));
x = x + symbol;
end
% (.t6) Add CP
cp = ceil(opt.CP*opt.blockLength);
xcp = [x(end-cp + (1:cp), :); x];
signal = [signal; xcp];
end
end
end
% (.r) Simulation of receiver GFDM/OFDM
function [sym_rx, cpx_sym_rx, ber, ser] = rx_simul(opt, signal, sym_tx, g)
sym_rx = []; cpx_sym_rx = []; ber = 0; ser = 0;
for b = 1:opt.blocks
cp = ceil(opt.CP*opt.blockLength);
gfdmM = 1;
dim1 = opt.blockLength + cp;
dim2 = dim1;
% (.r1) OFDM case
if opt.M == 1
gfdmM = opt.gfdmM;
dim1 = opt.gfdmM*opt.K + opt.gfdmM*cp;
dim2 = opt.K + cp;
end
for m = 1 : gfdmM
block = signal((b-1)*dim1 + (m-1)*dim2 + (1:dim2));
% (.r2) Remove CP
block = block(cp + 1:end);
% (.r3) Calculate the transmitter pulse g (Matched Filter)
g = g(round(1:opt.K/opt.K:end));
G = conj(fft(g));
% (.r4) Demodulate in frequency
L = length(G) / opt.M;
Xhat = fft(block);
Dhat = zeros(opt.K, opt.M);
for k=1:opt.K
carrier = circshift(Xhat, ceil(L*opt.M/2) - opt.M*(k-1));
carrier = fftshift(carrier(1:L*opt.M));
carrierMatched = carrier .* G;
dhat = ifft(sum(reshape(carrierMatched, opt.M, L), 2)/L);
Dhat(k,:) = dhat;
end
% (.r5) Unmap
if length(opt.subcarriers) == opt.K && length(subsymbols) == opt.M
dhat_mf = reshape(Dhat, numel(Dhat), 1);
else
Dm = Dhat(opt.subcarriers, opt.subsymbols);
dhat_mf = reshape(Dm, numel(Dm), 1);
end
% (.r6) Map constellation points to data
dhat_mf = dhat_mf * sqrt(2/3 * (2^opt.mu - 1));
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于MATLAB的gfdm和ofdm两种调制方式对比,包括功率谱,眼图,星座图,误码率等+含代码操作演示视频 运行注意事项:使用matlab2021a或者更高版本测试,运行里面的Runme.m文件,不要直接运行子函数文件。运行时注意matlab左侧的当前文件夹窗口必须是当前工程所在路径。 具体可观看提供的操作录像视频跟着操作。
资源推荐
资源详情
资源评论
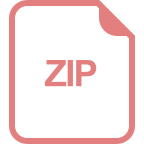
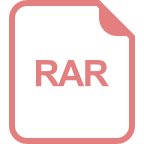
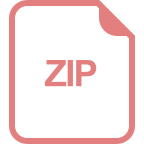
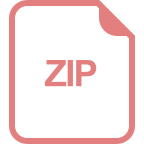
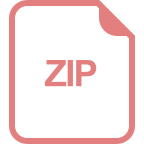
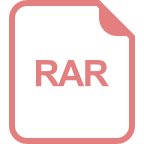
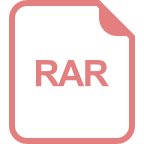
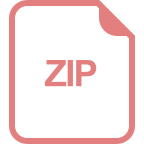
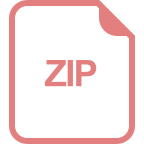
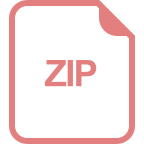
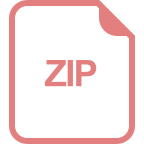
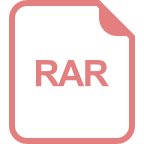
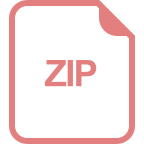
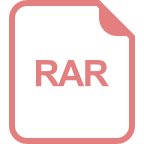
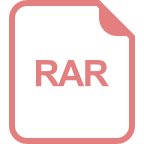
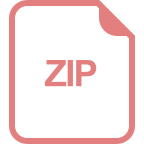
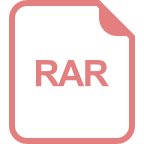
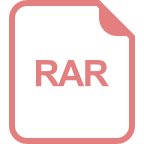
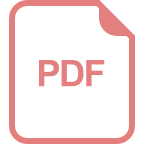
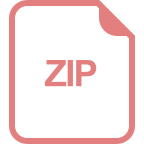
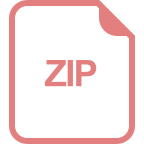
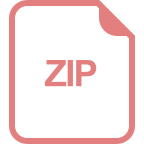
收起资源包目录



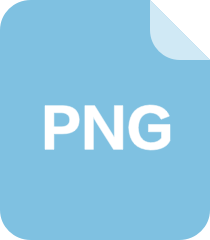
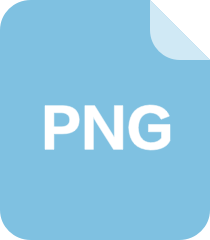
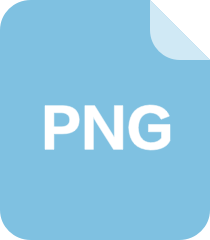
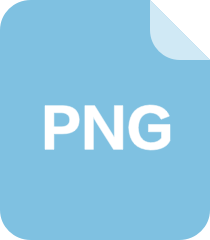
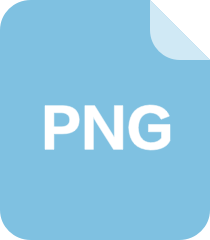
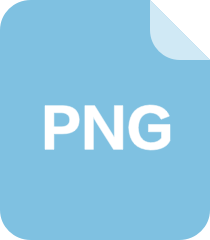
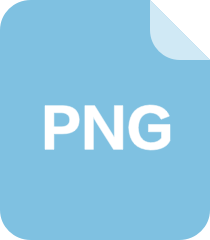
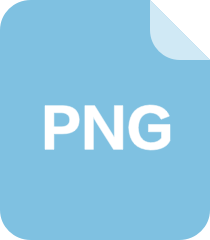
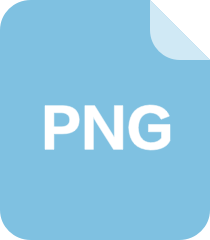
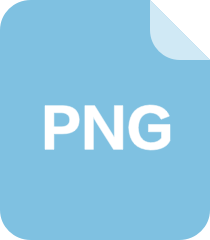
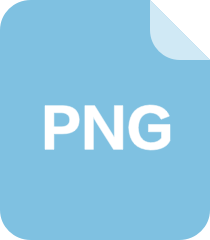
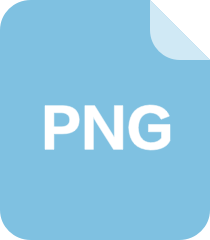
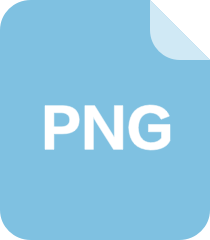
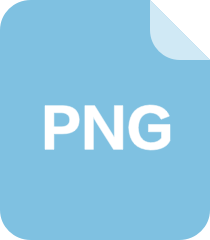
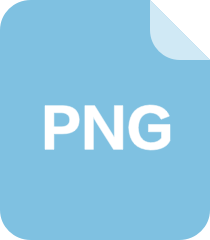
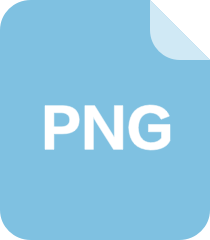
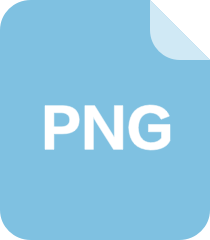
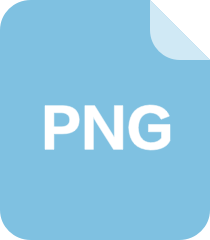
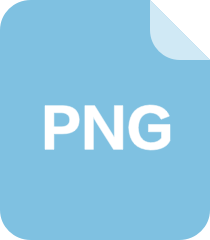
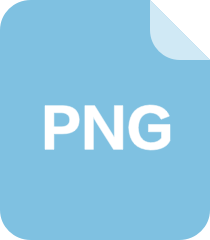
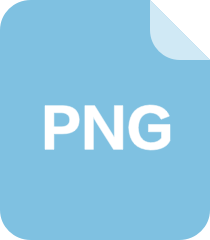
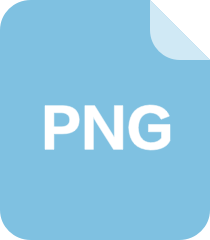
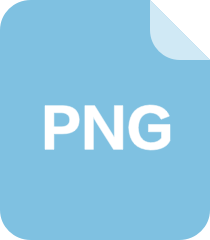
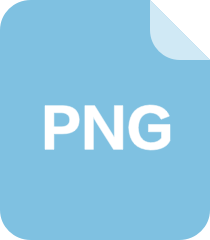
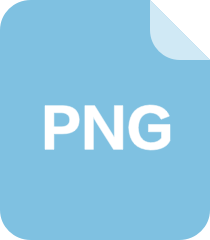
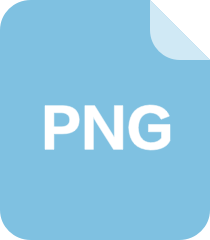
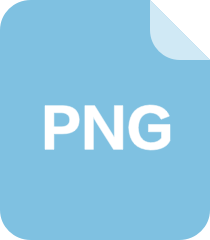
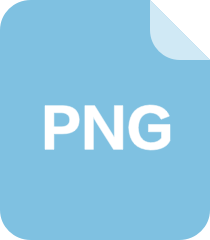
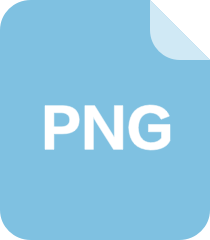
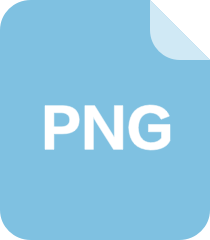
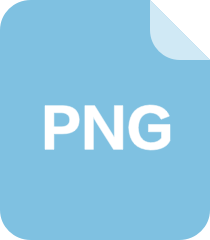
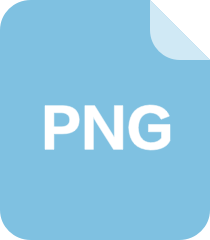
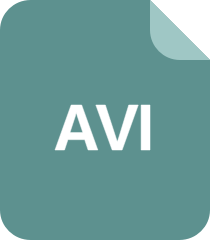
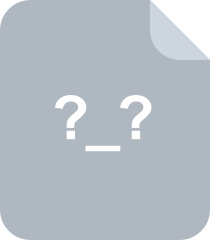
共 34 条
- 1


fpga和matlab
- 粉丝: 17w+
- 资源: 2628
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

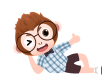
最新资源
- DirectX 12 编程第 1 卷示例.zip
- DirectX 12 离线安装程序适用于那些无法在其系统上运行在线安装程序的用户!.zip
- 计算机专业数据结构入门
- python《基于BERT的电商评论观点挖掘和情感分析》+项目源码+文档说明(高分作品)
- DirectX 12 示例实时体素化利用曲面细分进行原始处理和外推,以及利用深度剥离进行实体体素化 .zip
- AI指令合集-公众号推文
- 四川景区大数据可视化展示平台原型图
- Directx 12 玩具引擎.zip
- 51-MP3-语音识别分类垃圾桶
- 分类预测-python《基于Keras使用LSTM对电商评论进行情感分析》+项目源码+文档说明(高分作品)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


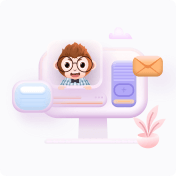
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页