在Java编程环境中,FTP(File Transfer Protocol)是一个广泛使用的协议,用于在本地计算机和远程服务器之间传输文件。本文将深入探讨如何使用Java实现一个FTP客户端,以根据服务器的目录结构在本地创建文件夹并下载文件。这个解决方案对于需要定期同步远程服务器数据或备份文件的应用非常有用。 我们需要引入一个支持FTP操作的Java库,如Apache Commons Net。这个库提供了丰富的FTP客户端功能,包括连接、上传、下载以及目录管理。在项目中,你可以通过Maven或Gradle添加依赖: ```xml <!-- Maven --> <dependency> <groupId>commons-net</groupId> <artifactId>commons-net</artifactId> <version>3.6</version> </dependency> // Gradle implementation 'commons-net:commons-net:3.6' ``` 接下来,我们创建一个`FtpClient`类来处理FTP操作。我们需要连接到FTP服务器: ```java import org.apache.commons.net.ftp.FTP; import org.apache.commons.net.ftp.FTPClient; public class FtpClient { private FTPClient ftpClient; public void connect(String host, int port, String username, String password) throws Exception { ftpClient = new FTPClient(); ftpClient.connect(host, port); if (ftpClient.login(username, password)) { ftpClient.setFileType(FTP.BINARY_FILE_TYPE); ftpClient.enterLocalPassiveMode(); } else { throw new Exception("Failed to login to FTP server"); } } // 其他FTP操作方法... } ``` 然后,我们可以实现一个`downloadDirectory`方法,遍历服务器目录结构,并在本地创建相应的文件夹,同时下载文件: ```java public void downloadDirectory(String remoteDirPath, String localRootPath) throws Exception { FTPClient.FTPReply reply = ftpClient.replyCode(); if (!FTPReply.isPositiveCompletion(reply)) { throw new Exception("Failed to change directory"); } FTPFile[] files = ftpClient.listFiles(remoteDirPath); for (FTPFile file : files) { String remoteFilePath = remoteDirPath + "/" + file.getName(); if (file.isDirectory()) { File localDir = new File(localRootPath + "/" + file.getName()); localDir.mkdirs(); // 递归下载子目录 downloadDirectory(remoteFilePath, localRootPath + "/" + file.getName()); } else { downloadFile(remoteFilePath, localRootPath); } } } private void downloadFile(String remoteFilePath, String localRootPath) throws Exception { File localFile = new File(localRootPath + "/" + remoteFilePath.substring(remoteFilePath.lastIndexOf("/") + 1)); FileOutputStream fos = new FileOutputStream(localFile); ftpClient.retrieveFile(remoteFilePath, fos); fos.close(); } ``` `downloadDirectory`方法首先改变FTP客户端的工作目录到指定的远程目录,然后获取该目录下的所有文件和子目录。对于每个文件,它将在本地创建一个对应路径的文件并下载;对于每个子目录,它会递归调用自身来处理子目录。 别忘了在完成操作后断开FTP连接: ```java public void disconnect() { try { if (ftpClient.isConnected()) { ftpClient.logout(); ftpClient.disconnect(); } } catch (IOException e) { e.printStackTrace(); } } ``` 现在,你可以创建一个`FtpTest`类来测试这个功能,提供FTP服务器的IP、端口、用户名、密码和根目录,以及本地保存文件的位置。通过调用`connect`、`downloadDirectory`和`disconnect`方法,即可实现标题中描述的功能。 Java中的FTP文件下载可以通过Apache Commons Net库轻松实现。通过建立FTP连接、遍历服务器目录、下载文件以及断开连接等步骤,可以创建一个功能完善的FTP客户端,将远程服务器的文件结构和文件复制到本地。这个解决方案对于开发者来说是一个有价值的工具,特别是在需要自动化文件同步或备份的场景中。
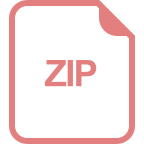
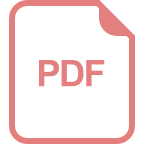
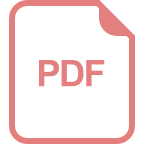
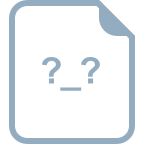
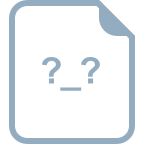
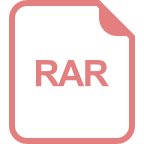
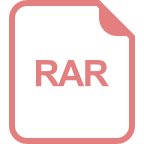
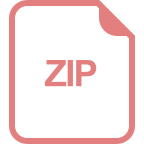
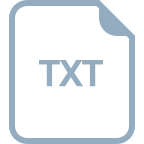
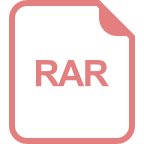
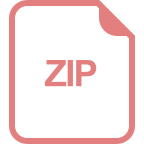
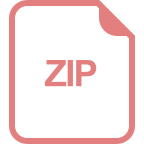
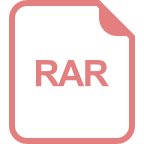
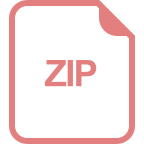



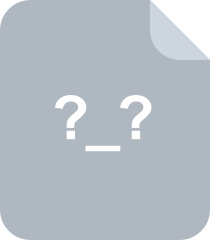
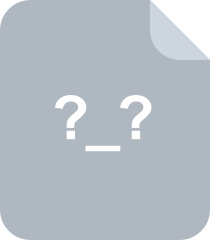
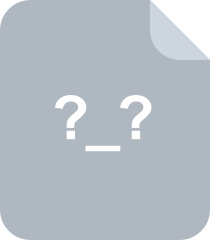

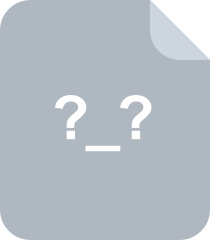
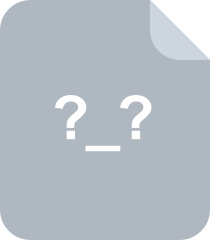
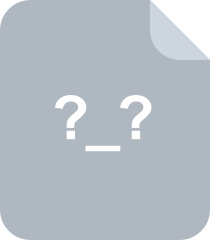
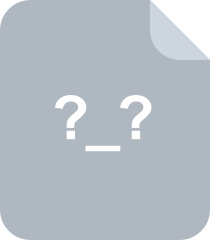
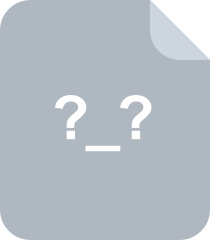

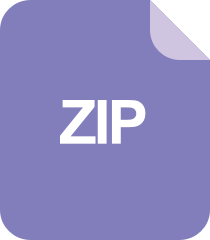
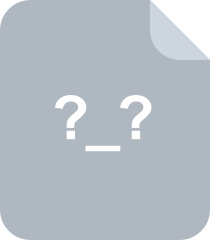
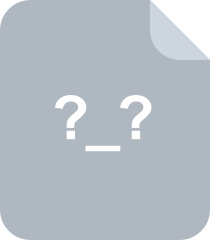
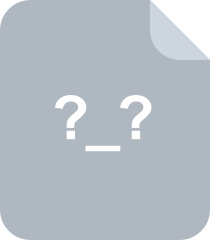

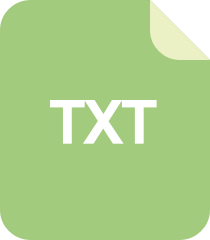
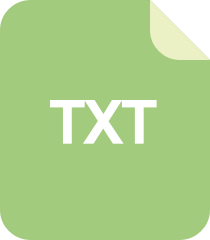

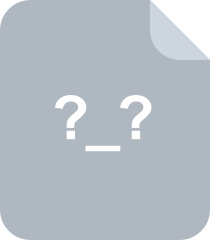


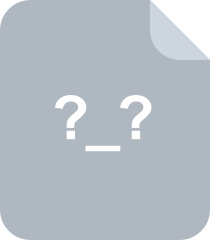
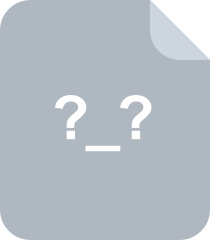


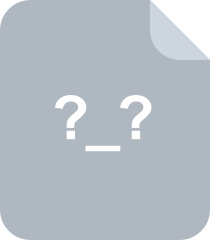

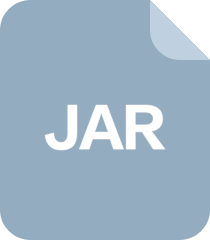
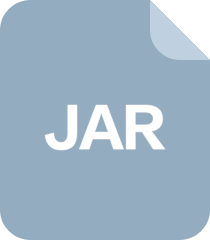
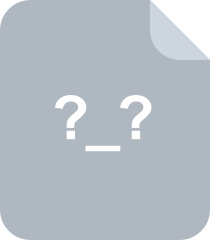
- 1
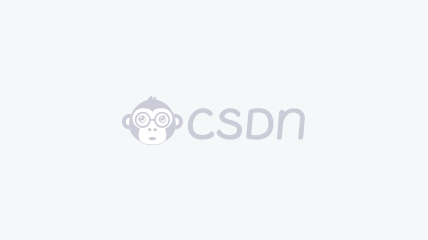
- vlongggg2018-11-06比较简单,没有生产应用价值,学习可以

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

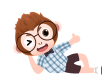
最新资源

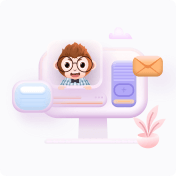
