package dao.hbn;
import java.util.Date;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.hibernate.LockMode;
import org.springframework.context.ApplicationContext;
import org.springframework.orm.hibernate3.support.HibernateDaoSupport;
import dao.PwdDao;
import domain.Password;
/**
* A data access object (DAO) providing persistence and search support for
* Password entities. Transaction control of the save(), update() and delete()
* operations can directly support Spring container-managed transactions or they
* can be augmented to handle user-managed Spring transactions. Each of these
* methods provides additional information for how to configure it for the
* desired type of transaction control.
*
* @see domain.Password
* @author MyEclipse Persistence Tools
*/
public class PwddDAOHbnImpl extends HibernateDaoSupport implements PwdDao{
private static final Log log = LogFactory.getLog(PwddDAOHbnImpl.class);
// property constants
public static final String NAME = "name";
public static final String USER_ID = "userId";
public static final String PASSWORD = "password";
public static final String NOTE = "note";
public static final String CREATE_BY = "createBy";
public static final String UPDATE_BY = "updateBy";
public static final String ALIAS = "alias";
protected void initDao() {
// do nothing
}
public void save(Password transientInstance) {
log.debug("saving Password instance");
try {
getHibernateTemplate().save(transientInstance);
log.debug("save successful");
} catch (RuntimeException re) {
log.error("save failed", re);
throw re;
}
}
public void delete(Password persistentInstance) {
log.debug("deleting Password instance");
try {
getHibernateTemplate().delete(persistentInstance);
log.debug("delete successful");
} catch (RuntimeException re) {
log.error("delete failed", re);
throw re;
}
}
public Password findById(java.lang.Integer id) {
log.debug("getting Password instance with id: " + id);
try {
Password instance = (Password) getHibernateTemplate().get(
"domain.Password", id);
return instance;
} catch (RuntimeException re) {
log.error("get failed", re);
throw re;
}
}
public List findByExample(Password instance) {
log.debug("finding Password instance by example");
try {
List results = getHibernateTemplate().findByExample(instance);
log.debug("find by example successful, result size: "
+ results.size());
return results;
} catch (RuntimeException re) {
log.error("find by example failed", re);
throw re;
}
}
public List findByProperty(String propertyName, Object value) {
log.debug("finding Password instance with property: " + propertyName
+ ", value: " + value);
try {
String queryString = "from Password as model where model."
+ propertyName + "= ?";
return getHibernateTemplate().find(queryString, value);
} catch (RuntimeException re) {
log.error("find by property name failed", re);
throw re;
}
}
public List findByName(Object name) {
return findByProperty(NAME, name);
}
public List findByUserId(Object userId) {
return findByProperty(USER_ID, userId);
}
public List findByPassword(Object password) {
return findByProperty(PASSWORD, password);
}
public List findByNote(Object note) {
return findByProperty(NOTE, note);
}
public List findByCreateBy(Object createBy) {
return findByProperty(CREATE_BY, createBy);
}
public List findByUpdateBy(Object updateBy) {
return findByProperty(UPDATE_BY, updateBy);
}
public List findByAlias(Object alias) {
return findByProperty(ALIAS, alias);
}
public List findAll() {
log.debug("finding all Password instances");
try {
String queryString = "from Password";
return getHibernateTemplate().find(queryString);
} catch (RuntimeException re) {
log.error("find all failed", re);
throw re;
}
}
public Password merge(Password detachedInstance) {
log.debug("merging Password instance");
try {
Password result = (Password) getHibernateTemplate().merge(
detachedInstance);
log.debug("merge successful");
return result;
} catch (RuntimeException re) {
log.error("merge failed", re);
throw re;
}
}
public void attachDirty(Password instance) {
log.debug("attaching dirty Password instance");
try {
getHibernateTemplate().saveOrUpdate(instance);
log.debug("attach successful");
} catch (RuntimeException re) {
log.error("attach failed", re);
throw re;
}
}
public void attachClean(Password instance) {
log.debug("attaching clean Password instance");
try {
getHibernateTemplate().lock(instance, LockMode.NONE);
log.debug("attach successful");
} catch (RuntimeException re) {
log.error("attach failed", re);
throw re;
}
}
public static PwddDAOHbnImpl getFromApplicationContext(ApplicationContext ctx) {
return (PwddDAOHbnImpl) ctx.getBean("PasswordDAO");
}
public Password getPwdByName(String name) {
return (Password) findByName(name);
}
@SuppressWarnings("unchecked")
public List<Password> getPwds() {
return findAll();
}
public Password getPwdById(Integer id) {
return findById(id);
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
JSF+Spring+Hibernate Hello学习程序
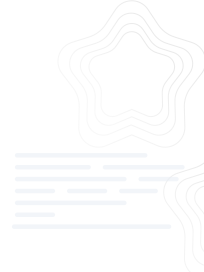
共219个文件
class:50个
jar:44个
java:39个


温馨提示
自己学习JSF, Spring, Hiebernate的 HelloWorld程序,开发环境MyEclipse,含有Tomahawk、RichFaces部分集成实例。具体包括登录、注册、树控件、日历等。可用作平时学习之用! 由于大小的限制,缺少的包请到相关官方网站下载添加进build path中
资源详情
资源评论
资源推荐
收起资源包目录

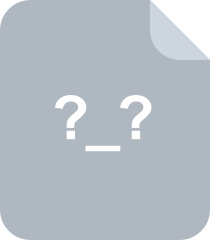
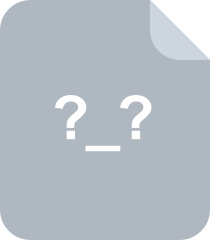
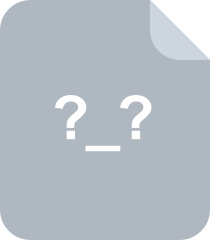
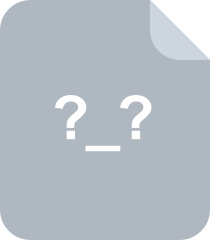
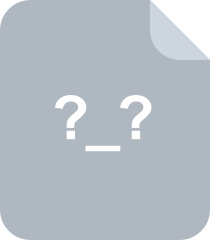
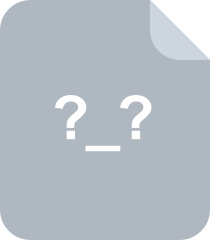
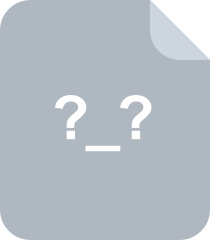
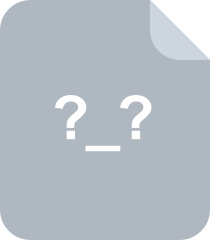
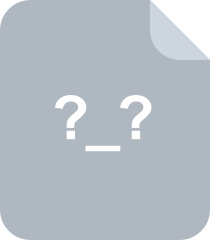
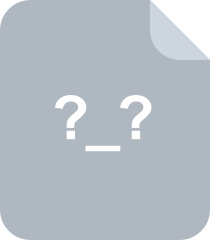
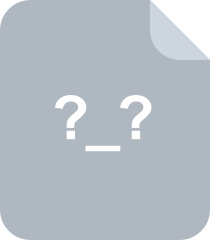
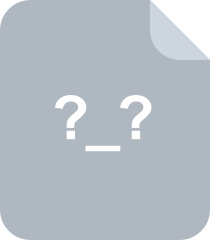
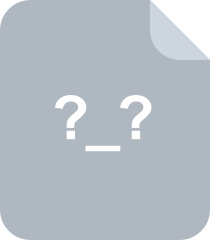
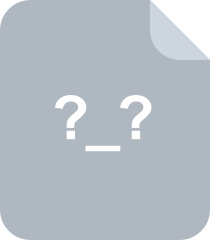
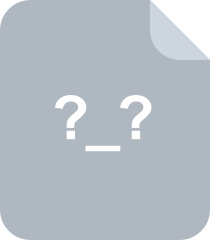
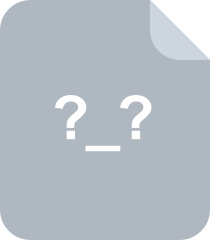
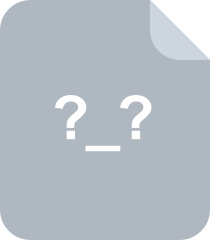
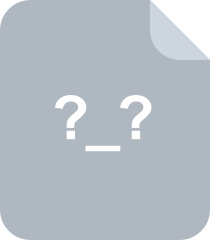
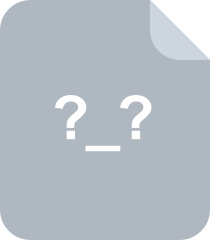
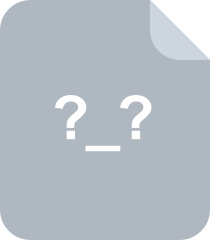
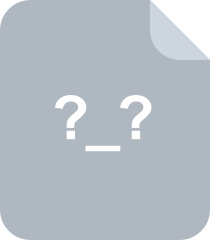
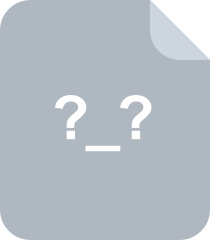
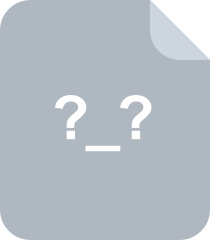
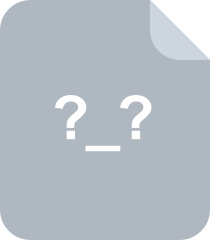
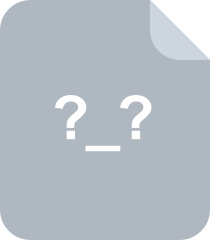
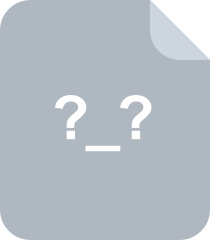
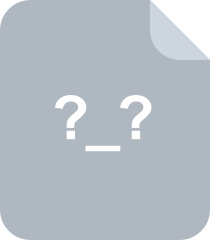
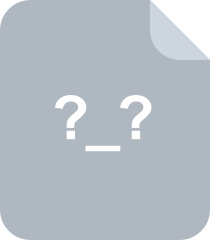
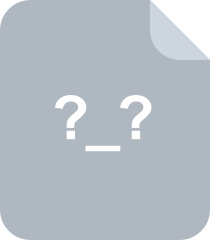
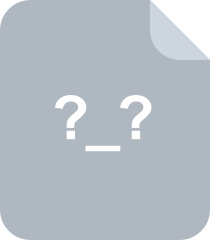
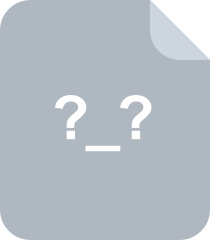
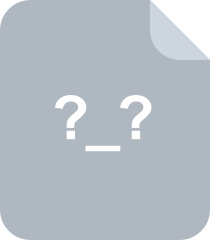
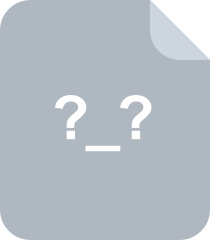
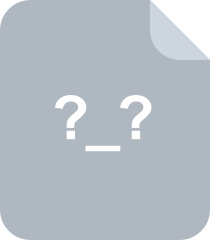
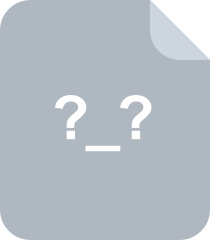
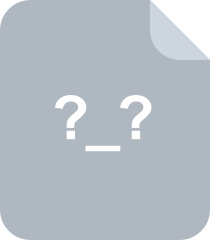
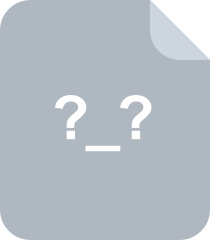
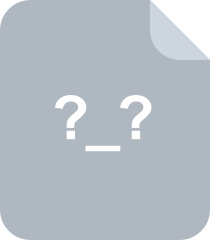
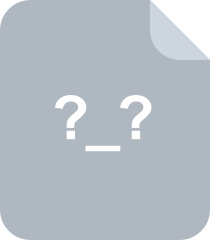
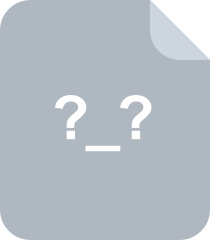
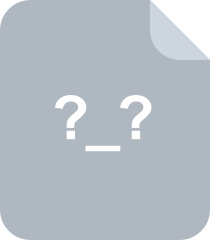
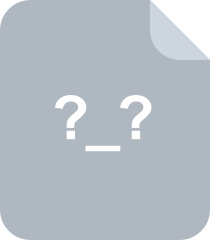
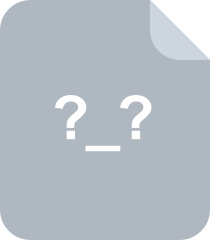
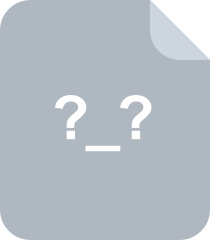
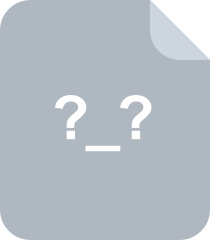
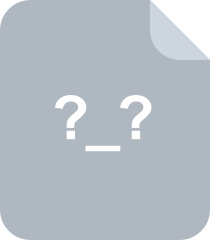
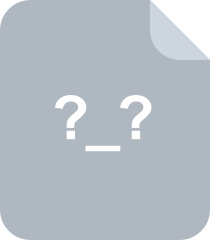
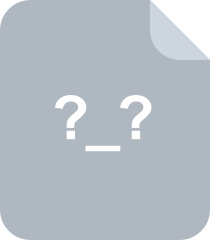
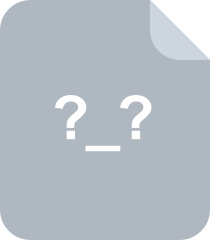
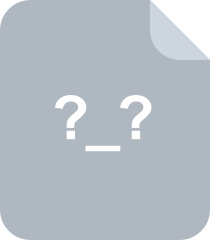
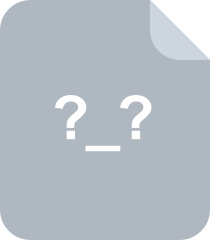
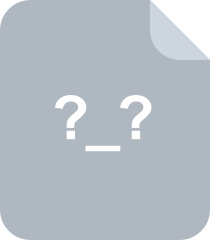
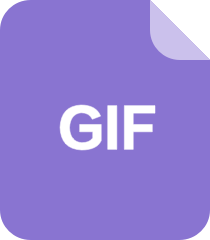
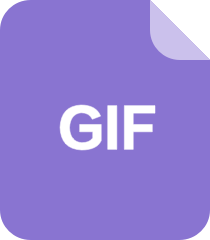
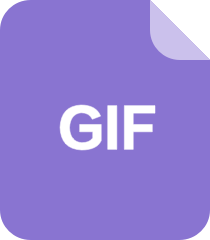
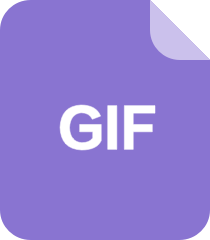
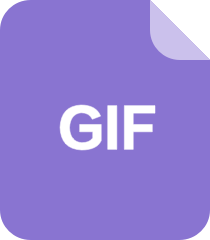
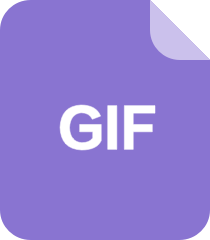
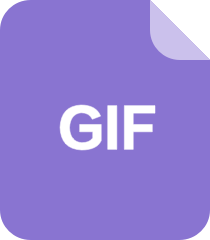
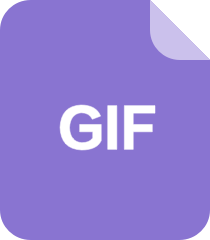
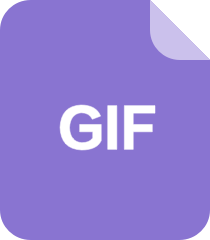
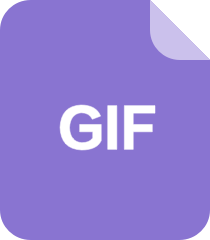
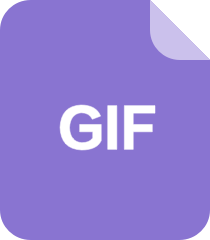
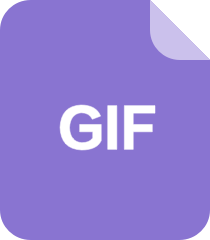
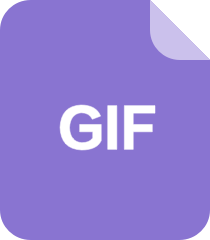
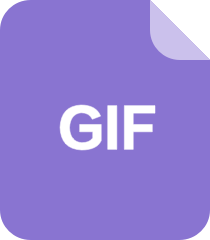
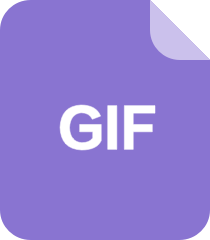
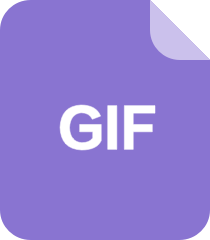
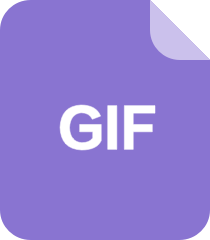
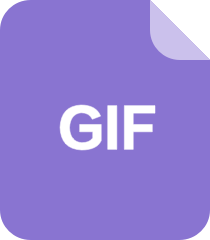
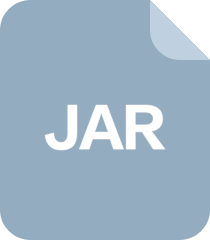
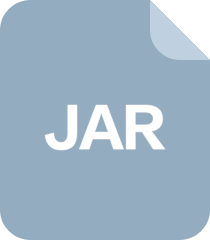
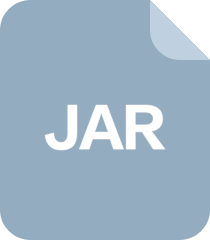
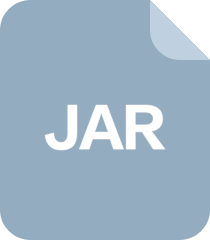
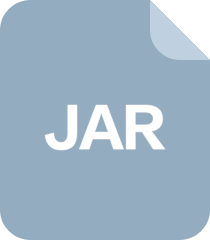
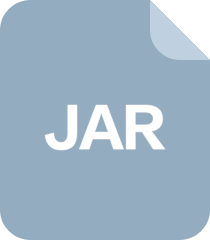
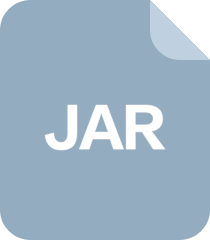
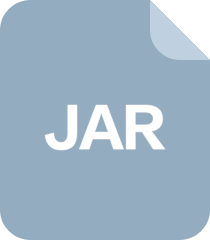
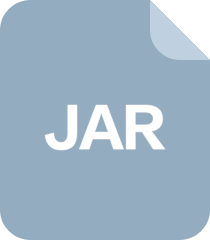
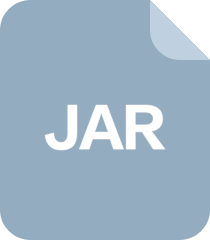
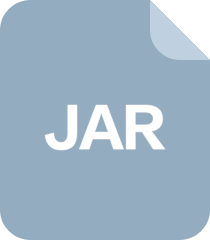
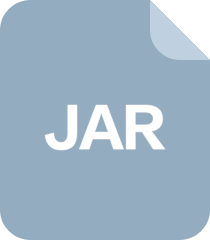
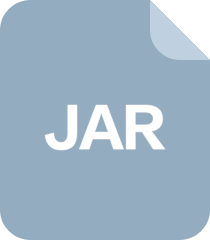
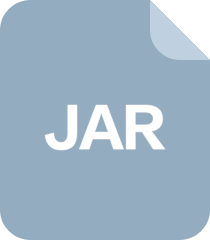
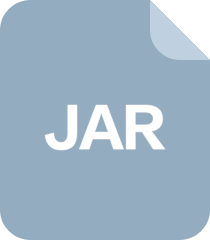
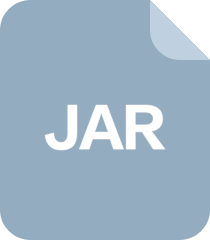
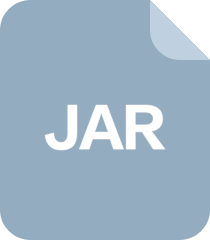
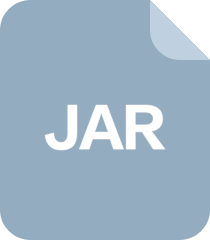
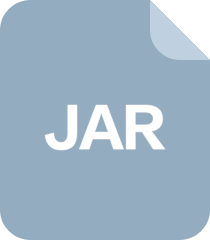
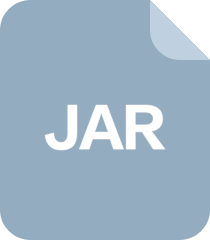
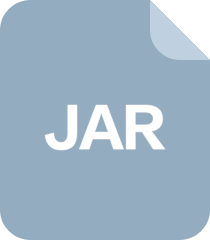
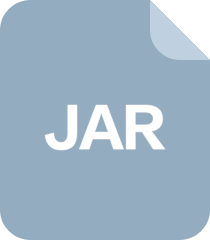
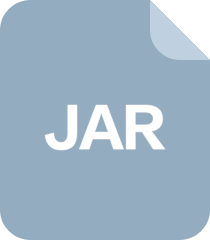
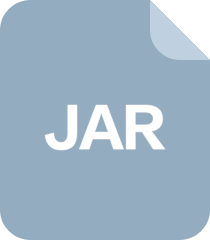
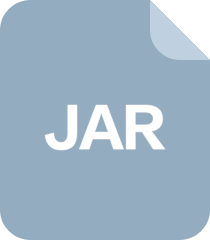
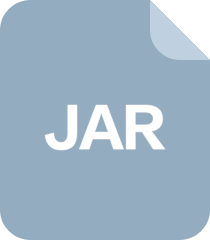
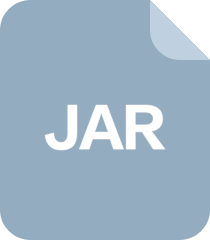
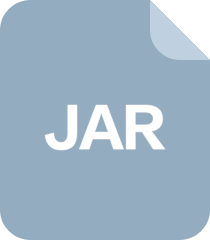
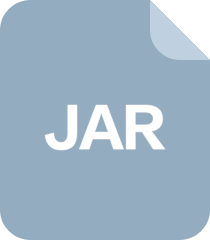
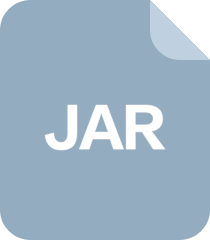
共 219 条
- 1
- 2
- 3
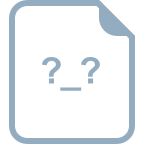
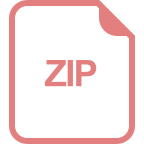
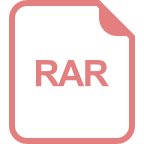
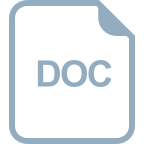
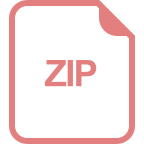
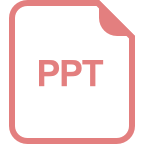
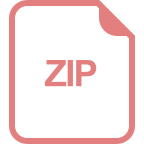
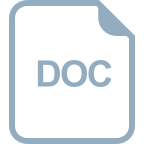
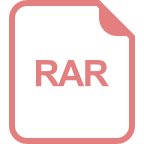
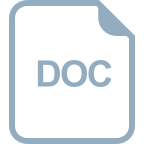
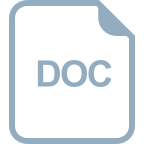
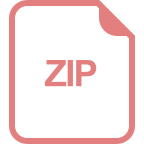
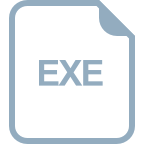
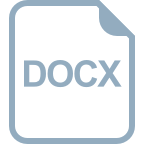
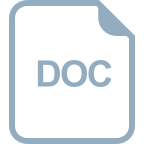

草色青青
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

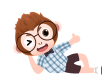
最新资源
- 知攻善防-应急响应靶机-web2.z32
- 还需要改的 只实现13数据
- 基于三菱PLC的温室大棚控制系统的设计塑料大棚温室控制 基于三菱PLC的智能农业温室大棚控制系统设计大棚电气控制组态画面
- Remote Ripple远程桌面允许用户从任何地方、任何设备上远程访问和控制其他计算机 软件的主要特点是其跨平台性,支持Windows、Mac、Linux等多种操作系统,以及iOS和Android
- 基于Unet网络实现对天文图像的降噪处理python源码+说明(高分项目)
- 知攻善防-应急响应靶机-web2.z35
- 知攻善防-应急响应靶机-web2.z36
- python基于Unet网络实现对天文图像的降噪处理源码+说明(高分项目)
- 知攻善防-应急响应靶机-web2.z01
- 知攻善防-应急响应靶机-web2.z02
- 知攻善防-应急响应靶机-web2.z03
- 知攻善防-应急响应靶机-web2.z04
- Oracle JDK1.8最后一个免费版本安装包及安装说明
- 基于STM32F051K8U6的光强传感器数据采集与风扇控制实现-含代码和注释
- 网上调查系统:性能优化与可扩展性分析
- 跨平台教务管理:教务信息平台的开发
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


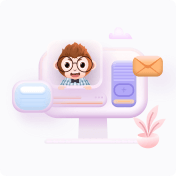
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1