=================
Werkzeug Tutorial
=================
.. module:: werkzeug
Welcome to the Werkzeug 0.5 tutorial in which we will create a `TinyURL`_ clone
that stores URLs in a database. The libraries we will use for this
applications are `Jinja`_ 2 for the templates, `SQLAlchemy`_ for the database
layer and, of course, Werkzeug for the WSGI layer.
The reasons why we've decided on these libraries for the tutorial application
is that we want to stick to some of the design decisions `Django`_ took in the
past. One of them is using view functions instead of controller classes with
action methods, which is common in `Rails`_ and `Pylons`_, the other one is
designer-friendly templates.
The Werkzeug `example folder`_ contains a couple of applications that use other
template engines, too, so you may want to have a look at them. There is also
the source code of this application.
You can use `easy_install`_ to install the required libraries::
sudo easy_install Jinja2
sudo easy_install SQLAlchemy
If you're on Windows, omit the "sudo" (and make sure, `setuptools`_ is
installed); if you're on OS X, you can check if the libraries are also
available in port; or on Linux, you can check out your package manager for
packages called ``python-jinja2`` and ``python-sqlalchemy``.
If you're curious, check out the `online demo`_ of the application.
.. _TinyURL: http://tinyurl.com/
.. _Django: http://www.djangoproject.com/
.. _Jinja: http://jinja.pocoo.org/
.. _SQLAlchemy: http://sqlalchemy.org/
.. _Rails: http://www.rubyonrails.org/
.. _Pylons: http://pylonshq.com/
.. _example folder: http://dev.pocoo.org/projects/werkzeug/browser/examples
.. _easy_install: http://peak.telecommunity.com/DevCenter/EasyInstall
.. _setuptools: http://pypi.python.org/pypi/setuptools
.. _online demo: http://werkzeug.pocoo.org/e/shorty/
Part 0: The Folder Structure
============================
Before we can get started we have to create a Python package for our Werkzeug
application and the folders for the templates and static files.
This tutorial application is called `shorty` and the initial directory layout
we will use looks like this::
manage.py
shorty/
__init__.py
templates/
static/
The ``__init__.py`` and ``manage.py`` files should be empty for the time
being. The first one makes ``shorty`` a Python package, the second one will
hold our management utilities later.
Part 1: The WSGI Application
============================
Unlike Django or other frameworks, Werkzeug operates directly on the WSGI
layer. There is no fancy magic that implements the central WSGI application
for you. As a result of that the first thing you will do every time you write
a Werkzeug application is implementing this basic WSGI application object.
This can now either be a function or, even better, a callable class.
A callable class has huge advantages over a function. For one you can pass
it some configuration parameters and furthermore you can use inline WSGI
middlewares. Inline WSGI middlewares are basically middlewares applied
"inside" of our application object. This is a good idea for middlewares that
are essential for the application (session middlewares, serving of media
files etc.).
Here the initial code for our ``shorty/application.py`` file which implements
the WSGI application::
from sqlalchemy import create_engine
from werkzeug import Request, ClosingIterator
from werkzeug.exceptions import HTTPException
from shorty.utils import session, metadata, local, local_manager, url_map
from shorty import views
import shorty.models
class Shorty(object):
def __init__(self, db_uri):
local.application = self
self.database_engine = create_engine(db_uri, convert_unicode=True)
def init_database(self):
metadata.create_all(self.database_engine)
def __call__(self, environ, start_response):
local.application = self
request = Request(environ)
local.url_adapter = adapter = url_map.bind_to_environ(environ)
try:
endpoint, values = adapter.match()
handler = getattr(views, endpoint)
response = handler(request, **values)
except HTTPException, e:
response = e
return ClosingIterator(response(environ, start_response),
[session.remove, local_manager.cleanup])
That's a lot of code for the beginning! Let's go through it step by step.
First we have a couple of imports: From SQLAlchemy we import a factory
function that creates a new database engine for us. A database engine holds
a pool of connections for us and manages them. The next few imports pull some
objects into the namespace Werkzeug provides: a request object, a special
iterator class that helps us cleaning up stuff at the request end and finally
the base class for all HTTP exceptions.
The next five imports are not working because we don't have the utils module
written yet. However we should cover some of the objects already. The
`session` object pulled from there is not a PHP-like session object but a
SQLAlchemy database session object. Basically a database session object keeps
track of yet uncommited objects for the database. Unlike Django, an
instantiated SQLAlchemy model is already tracked by the session! The metadata
object is also an SQLAlchemy thing which is used to keep track of tables. We
can use the metadata object to easily create all tables for the database and
SQLAlchemy uses it to look up foreign keys and similar stuff.
The `local` object is basically a thread local object created in the utility
module for us. Every attribute on this object is bound to the current request
and we can use this to implicitly pass objects around in a thread-safe way.
The `local_manager` object ensures that all local objects it keeps track of
are properly deleted at the end of the request.
The last thing we import from there is the URL map which holds the URL routing
information. If you know Django you can compare that to the url patterns you
specify in the ``urls.py`` module, if you have used PHP so far it's comparable
with some sort of built-in "mod_rewrite".
We import our views module which holds the view functions and then we import
the models module which holds all of our models. Even if it looks like we
don't use that import it's there so that all the tables are registered on the
metadata properly.
So let's have a look at the application class. The constructor of this class
takes a database URI which is basically the type of the database and the login
credentials or location of the database. For SQLite this is for example
``'sqlite:////tmp/shorty.db'`` (note that these are **four** slashes).
In the constructor we create a database engine for that database URI and use
the `convert_unicode` parameter to tell SQLAlchemy that our strings are all
unicode objects.
Another thing we do here is binding the application to the local object. This
is not really required but useful if we want to play with the application in
a python shell. On application instanciation we have it bound to the current
thread and all the database functions will work as expected. If we don't do
that Werkzeug will complain that it's unable to find the database when it's
creating a session for SQLAlchemy.
The `init_database` function defined below can be used to create all the
tables we use.
And then comes the request dispatching function. In there we create a new
request object by passing the environment to the :class:`Request` constructor.
Once again we bind the application to the local object, this time, however,
we have to do this, otherwise things will break soon.
Then we create a new URL map adapter by binding the URL map to the current
WSGI environment. This basically looks at the environment of the incoming
request information and fetches the informatio

程序员Chino的日记
- 粉丝: 3752
- 资源: 5万+
最新资源
- ABAQUS高速铁路板式无砟轨道耦合动力学模型
- 短路电流计算 Matlab编程计算 针对常见的四种短路故障(单相接地短路,两相相间短路,两相接地短路,三相短路),可采取三种方法进行计算: 1.实用短路电流计算 2.对称分量法计算 3
- 优化算法改进 Matlab 麻雀搜索算法,粒子群优化算法,鲸鱼优化算法,灰狼优化算法,黏菌优化算法等优化算法,提供算法改进点 改进后的优化算法也可应用于支持向量机,最小二乘支持向量机,随机森林,核
- 遗传算法优化极限学习机做预测,运行直接出图,包含真实值,ELM,GA-ELM对比,方便比较 智能优化算法,粒子群算法,花授粉算法,麻雀算法,鲸鱼算法,灰狼算法等,优化BP神经网络,支持向量机,极限学
- FX3U,FX5U,控制IO卡 ,STM32F407ZET6工控板,包括pcb,原理图 , PLC STMF32F407ZET6 FX-3U PCB生产方案 板载资源介绍 1. 8路高速脉冲加方向
- 利用matlab和simulink搭建的纯跟踪控制器用于单移线轨迹跟踪,效果如图 版本各为2018b和2019 拿后内容包含: 1、simulink模型 2、纯跟踪算法的纯matlab代码,便于理解
- 三相光伏并网逆变器设计,原理图,PCB,以及源代码 主要包括以下板卡: 1)主控DSP板, 负责逆变器的逆变及保护控制 原理图为pdf. pcb为AD文件 2)接口板,负责信号采集、处理,以及
- 考虑气电联合需求响应的 气电综合能源配网系统协调优化运行 该文提出气电综合能源配网系统最优潮流的凸优化方法,即利用二阶锥规划方法对配电网潮流方 程约束进行处理,并提出运用增强二阶锥规划与泰勒级数展开相
- 光子晶体BIC,OAM激发 若需第二幅图中本征态以及三维Q等计算额外
- 基于共享储能电站的工业用户日前优化经济调度,通过协调各用户使用共享储能电站进行充放电,实现日运行最优 代码环境:matlab+yalmip+cplex gurobi ,注释详尽,结果正确 对学习储
- 三相PWM整流器simulink仿真模型,采用双闭关PI控制,SVPWM调制策略,可以实现很好的整流效果,交流侧谐波含量低,可以很好的应对负载突变等复杂工况
- 红外遥控器+红外一体化接收头部分的仿真 带程序 红外线编码是数据传输和家用电器遥控常用的一种通讯方法,其实质是一种脉宽调制的串行通讯 家电遥控中常用的红外线编码电路有μPD6121G型HT622型和
- 新能源系统低碳优化调度(用Matlab) 包含各类分布式电源消纳、热电联产、电锅炉、储能电池、天然气等新能源元素,实现系统中各种成本的优化,调度 若有需要,我也有matlab
- Matlab 遗传算法解决0-1背包问题(装包问题) 源码+详细注释 问题描述:已知不同物品质量与不同背包最大载重,求取最优值使得所有背包所装得的物品质量总和最大 可以改物品质量与背包载重数据
- 信捷plc控制3轴机械臂调试程序,只是调试程序,包含信捷plc程序,信捷触摸屏程序,手机组态软件程序,含手机组态软件 程序自己写的,后期还会增加相关项目 触摸屏示教程序写好,可以任意示教完成全部动
- ABS制动系统开发 PID控制 开关控制 matlab simulink carsim联合仿真,下面视频为pid控制效果和不带ABS的对比 滑移率控制目标20% 分离路面制动
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


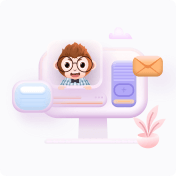