==================================================
Building and Distributing Packages with Setuptools
==================================================
``Setuptools`` is a collection of enhancements to the Python ``distutils``
that allow developers to more easily build and
distribute Python packages, especially ones that have dependencies on other
packages.
Packages built and distributed using ``setuptools`` look to the user like
ordinary Python packages based on the ``distutils``.
Feature Highlights:
* Create `Python Eggs <http://peak.telecommunity.com/DevCenter/PythonEggs>`_ -
a single-file importable distribution format
* Enhanced support for accessing data files hosted in zipped packages.
* Automatically include all packages in your source tree, without listing them
individually in setup.py
* Automatically include all relevant files in your source distributions,
without needing to create a ``MANIFEST.in`` file, and without having to force
regeneration of the ``MANIFEST`` file when your source tree changes.
* Automatically generate wrapper scripts or Windows (console and GUI) .exe
files for any number of "main" functions in your project. (Note: this is not
a py2exe replacement; the .exe files rely on the local Python installation.)
* Transparent Cython support, so that your setup.py can list ``.pyx`` files and
still work even when the end-user doesn't have Cython installed (as long as
you include the Cython-generated C in your source distribution)
* Command aliases - create project-specific, per-user, or site-wide shortcut
names for commonly used commands and options
* Deploy your project in "development mode", such that it's available on
``sys.path``, yet can still be edited directly from its source checkout.
* Easily extend the distutils with new commands or ``setup()`` arguments, and
distribute/reuse your extensions for multiple projects, without copying code.
* Create extensible applications and frameworks that automatically discover
extensions, using simple "entry points" declared in a project's setup script.
* Full support for PEP 420 via ``find_namespace_packages()``, which is also backwards
compatible to the existing ``find_packages()`` for Python >= 3.3.
.. contents:: **Table of Contents**
-----------------
Developer's Guide
-----------------
Installing ``setuptools``
=========================
.. _Installing Packages: https://packaging.python.org/tutorials/installing-packages/
To install the latest version of setuptools, use::
pip install -U setuptools
Refer to `Installing Packages`_ guide for more information.
Basic Use
=========
For basic use of setuptools, just import things from setuptools instead of
the distutils. Here's a minimal setup script using setuptools::
from setuptools import setup, find_packages
setup(
name="HelloWorld",
version="0.1",
packages=find_packages(),
)
As you can see, it doesn't take much to use setuptools in a project.
Run that script in your project folder, alongside the Python packages
you have developed.
Invoke that script to produce distributions and automatically include all
packages in the directory where the setup.py lives. See the `Command
Reference`_ section below to see what commands you can give to this setup
script. For example, to produce a source distribution, simply invoke::
python setup.py sdist
Of course, before you release your project to PyPI, you'll want to add a bit
more information to your setup script to help people find or learn about your
project. And maybe your project will have grown by then to include a few
dependencies, and perhaps some data files and scripts::
from setuptools import setup, find_packages
setup(
name="HelloWorld",
version="0.1",
packages=find_packages(),
scripts=['say_hello.py'],
# Project uses reStructuredText, so ensure that the docutils get
# installed or upgraded on the target machine
install_requires=['docutils>=0.3'],
package_data={
# If any package contains *.txt or *.rst files, include them:
'': ['*.txt', '*.rst'],
# And include any *.msg files found in the 'hello' package, too:
'hello': ['*.msg'],
},
# metadata to display on PyPI
author="Me",
author_email="me@example.com",
description="This is an Example Package",
keywords="hello world example examples",
url="http://example.com/HelloWorld/", # project home page, if any
project_urls={
"Bug Tracker": "https://bugs.example.com/HelloWorld/",
"Documentation": "https://docs.example.com/HelloWorld/",
"Source Code": "https://code.example.com/HelloWorld/",
},
classifiers=[
'License :: OSI Approved :: Python Software Foundation License'
]
# could also include long_description, download_url, etc.
)
In the sections that follow, we'll explain what most of these ``setup()``
arguments do (except for the metadata ones), and the various ways you might use
them in your own project(s).
Specifying Your Project's Version
---------------------------------
Setuptools can work well with most versioning schemes; there are, however, a
few special things to watch out for, in order to ensure that setuptools and
other tools can always tell what version of your package is newer than another
version. Knowing these things will also help you correctly specify what
versions of other projects your project depends on.
A version consists of an alternating series of release numbers and pre-release
or post-release tags. A release number is a series of digits punctuated by
dots, such as ``2.4`` or ``0.5``. Each series of digits is treated
numerically, so releases ``2.1`` and ``2.1.0`` are different ways to spell the
same release number, denoting the first subrelease of release 2. But ``2.10``
is the *tenth* subrelease of release 2, and so is a different and newer release
from ``2.1`` or ``2.1.0``. Leading zeros within a series of digits are also
ignored, so ``2.01`` is the same as ``2.1``, and different from ``2.0.1``.
Following a release number, you can have either a pre-release or post-release
tag. Pre-release tags make a version be considered *older* than the version
they are appended to. So, revision ``2.4`` is *newer* than revision ``2.4c1``,
which in turn is newer than ``2.4b1`` or ``2.4a1``. Postrelease tags make
a version be considered *newer* than the version they are appended to. So,
revisions like ``2.4-1`` and ``2.4pl3`` are newer than ``2.4``, but are *older*
than ``2.4.1`` (which has a higher release number).
A pre-release tag is a series of letters that are alphabetically before
"final". Some examples of prerelease tags would include ``alpha``, ``beta``,
``a``, ``c``, ``dev``, and so on. You do not have to place a dot or dash
before the prerelease tag if it's immediately after a number, but it's okay to
do so if you prefer. Thus, ``2.4c1`` and ``2.4.c1`` and ``2.4-c1`` all
represent release candidate 1 of version ``2.4``, and are treated as identical
by setuptools.
In addition, there are three special prerelease tags that are treated as if
they were the letter ``c``: ``pre``, ``preview``, and ``rc``. So, version
``2.4rc1``, ``2.4pre1`` and ``2.4preview1`` are all the exact same version as
``2.4c1``, and are treated as identical by setuptools.
A post-release tag is either a series of letters that are alphabetically
greater than or equal to "final", or a dash (``-``). Post-release tags are
generally used to separate patch numbers, port numbers, build numbers, revision
numbers, or date stamps from the release number. For example, the version
``2.4-r1263`` might denote Subversion revision 1263 of a post-release patch of
version ``2.4``. Or you might use ``2.4-20051127`` to denote a date-stamped
post-release.
Notice that after each pre or
没有合适的资源?快使用搜索试试~ 我知道了~
setuptools-42.0.0.zip
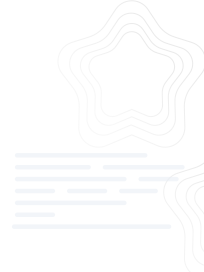
共196个文件
py:144个
txt:23个
exe:6个

0 下载量 28 浏览量
2024-05-13
23:25:23
上传
评论
收藏 832KB ZIP 举报
温馨提示
Python库是一组预先编写的代码模块,旨在帮助开发者实现特定的编程任务,无需从零开始编写代码。这些库可以包括各种功能,如数学运算、文件操作、数据分析和网络编程等。Python社区提供了大量的第三方库,如NumPy、Pandas和Requests,极大地丰富了Python的应用领域,从数据科学到Web开发。Python库的丰富性是Python成为最受欢迎的编程语言之一的关键原因之一。这些库不仅为初学者提供了快速入门的途径,而且为经验丰富的开发者提供了强大的工具,以高效率、高质量地完成复杂任务。例如,Matplotlib和Seaborn库在数据可视化领域内非常受欢迎,它们提供了广泛的工具和技术,可以创建高度定制化的图表和图形,帮助数据科学家和分析师在数据探索和结果展示中更有效地传达信息。
资源推荐
资源详情
资源评论
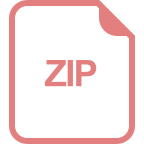
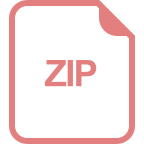
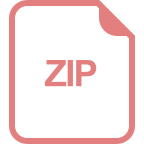
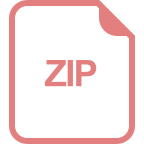
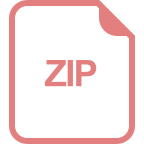
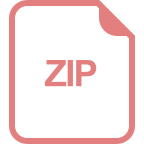
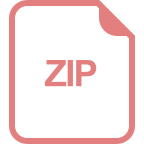
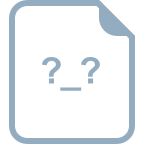
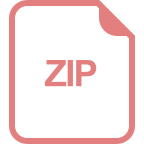
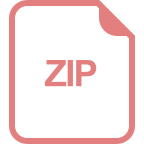
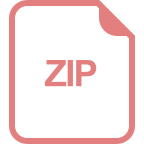
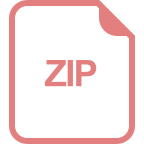
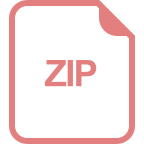
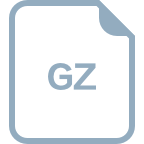
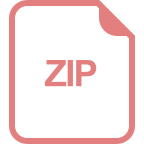
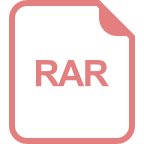
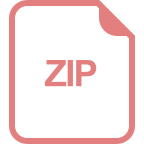
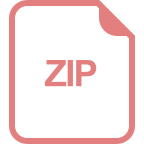
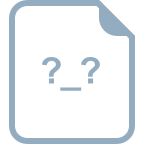
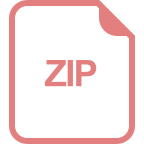
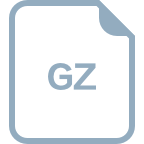
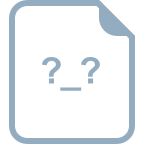
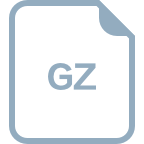
收起资源包目录

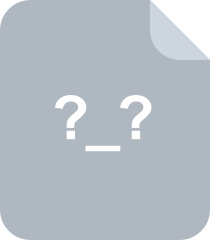
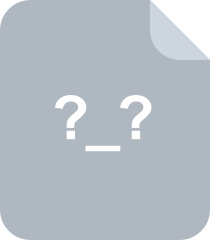
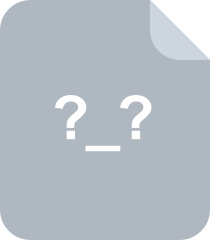
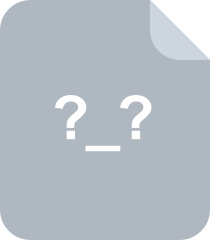
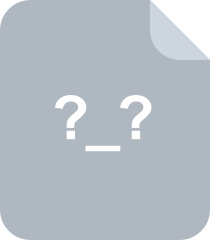
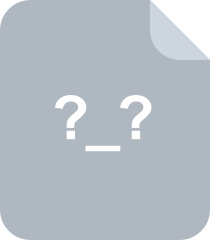
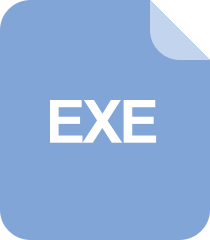
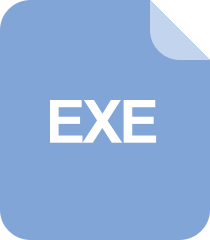
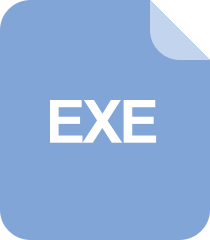
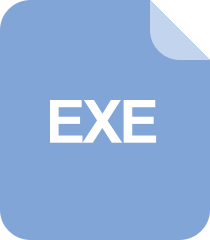
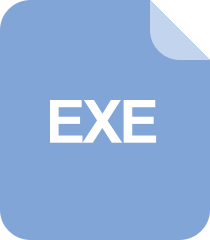
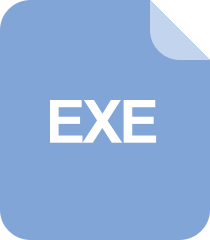
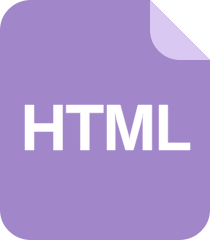
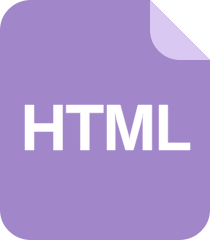
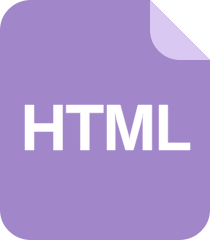
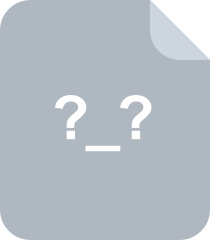
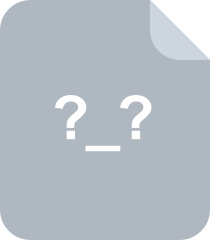
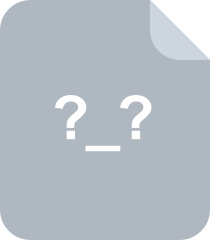
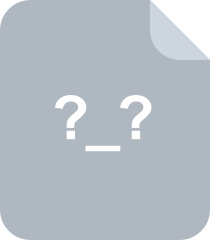
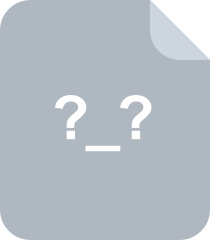
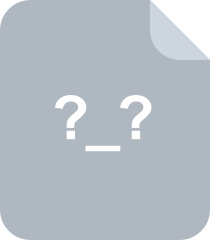
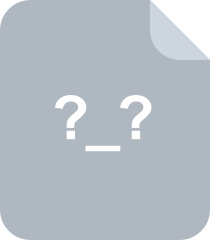
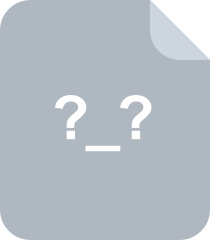
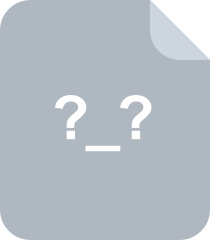
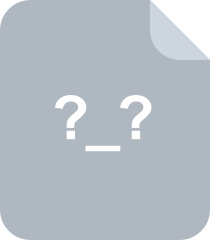
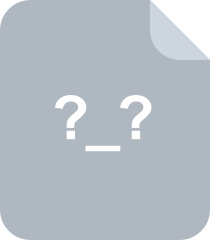
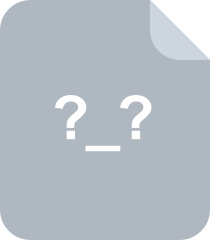
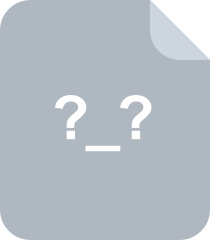
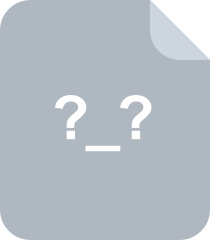
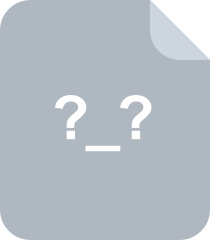
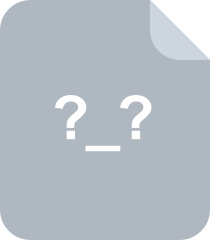
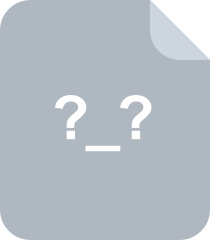
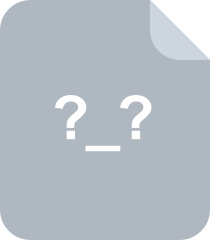
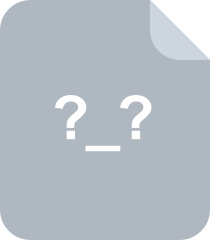
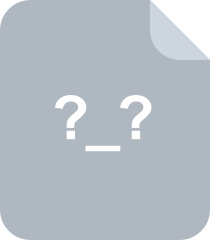
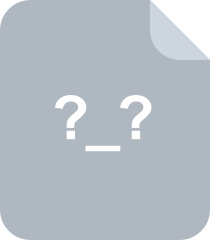
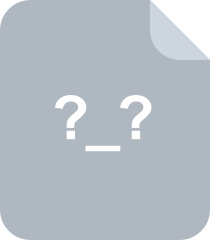
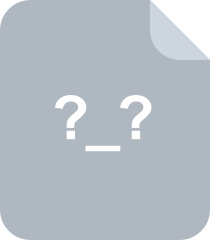
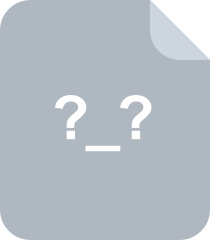
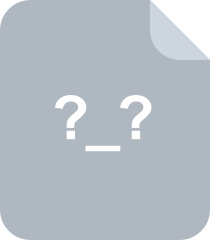
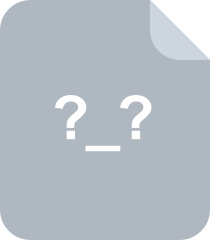
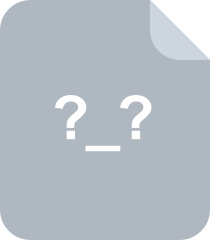
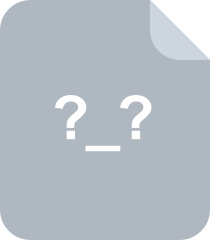
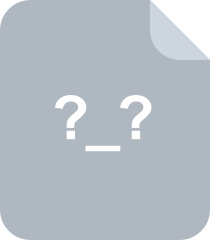
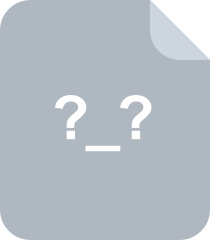
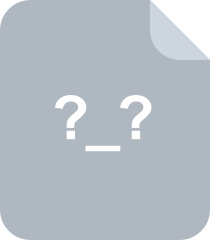
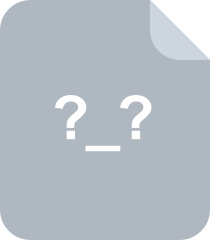
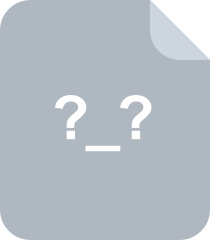
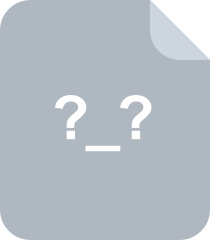
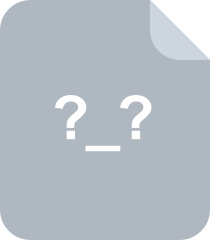
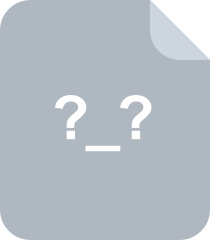
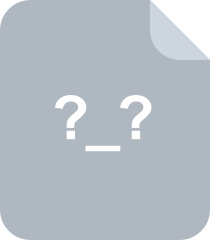
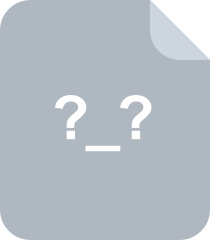
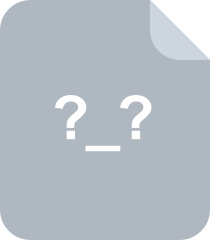
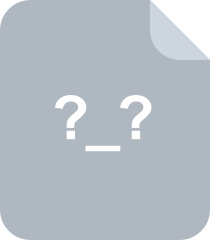
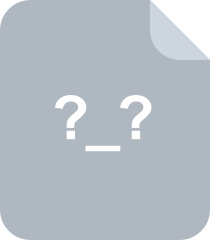
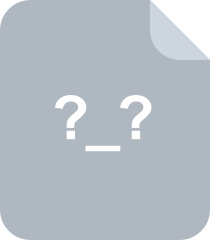
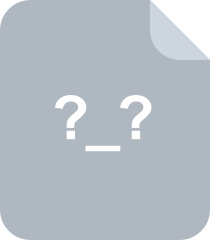
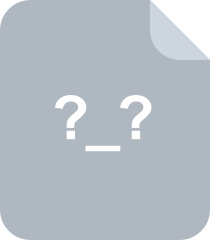
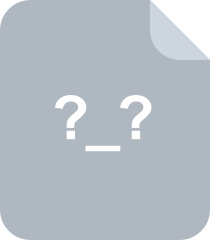
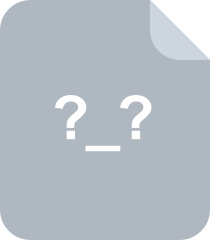
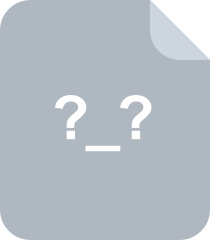
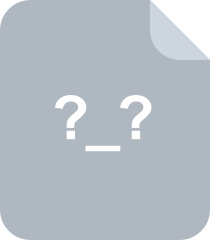
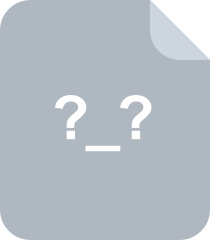
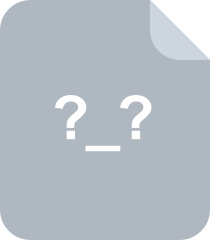
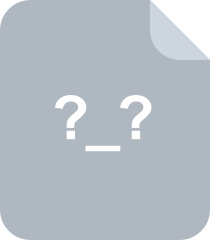
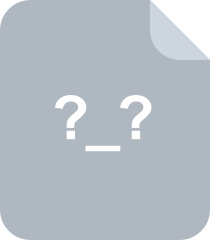
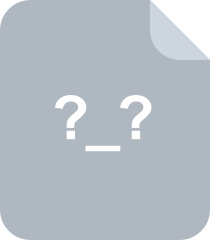
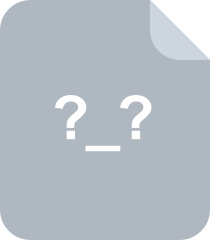
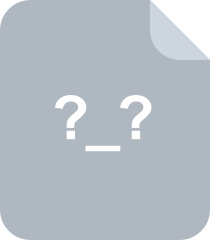
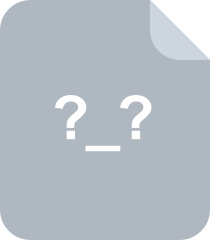
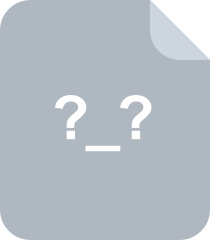
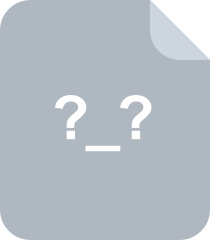
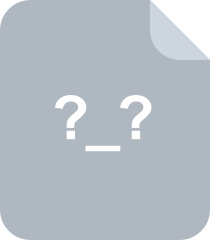
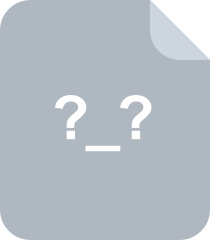
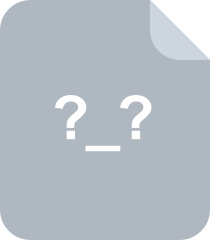
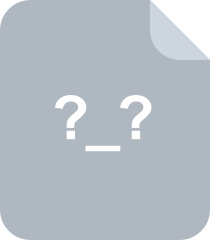
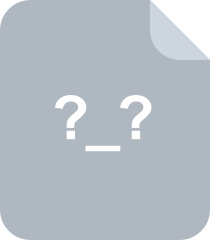
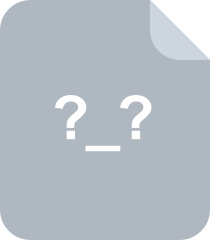
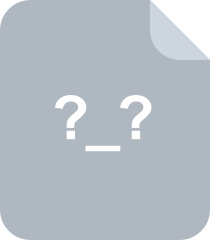
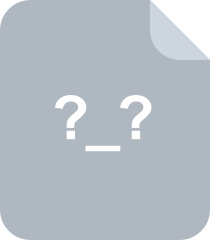
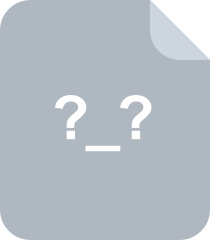
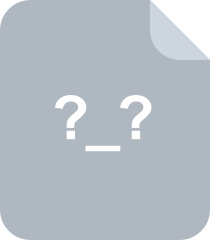
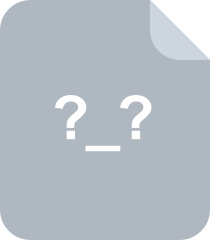
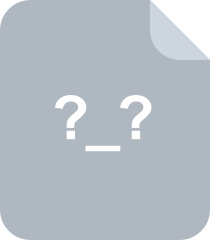
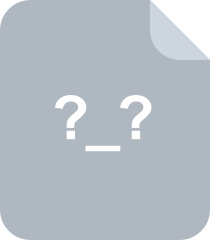
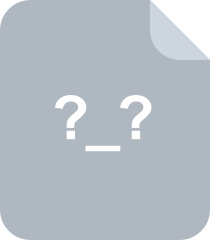
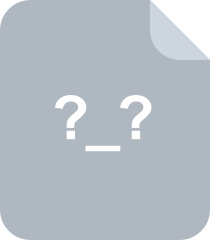
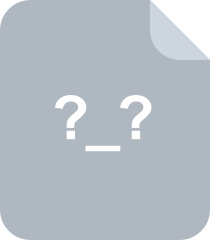
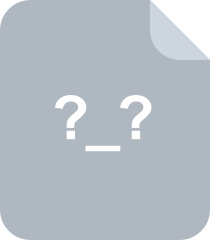
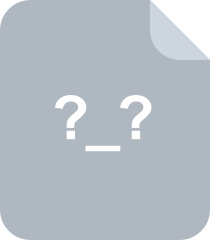
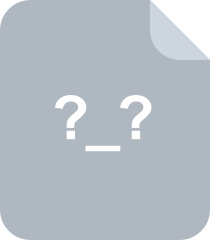
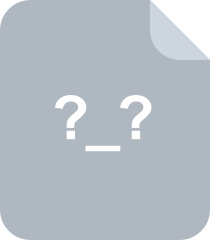
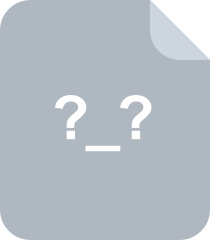
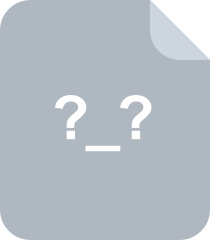
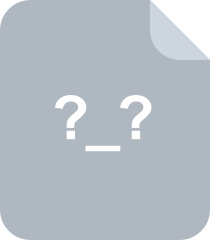
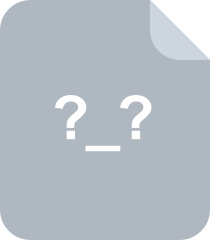
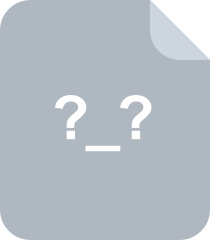
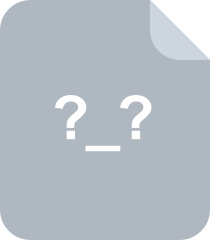
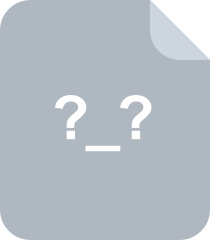
共 196 条
- 1
- 2
资源评论
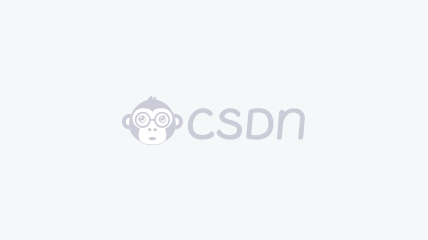

程序员Chino的日记
- 粉丝: 3665
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

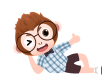
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


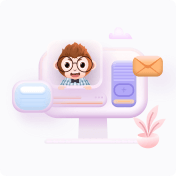
安全验证
文档复制为VIP权益,开通VIP直接复制
