# Node.js C++ codebase
Hi! ð Youâve found the C++ code backing Node.js. This README aims to help you
get started working on it and document some idioms you may encounter while
doing so.
## Coding style
Node.js has a document detailing its [C++ coding style][]
that can be helpful as a reference for stylistic issues.
## V8 API documentation
A lot of the Node.js codebase is around what the underlying JavaScript engine,
V8, provides through its API for embedders. Knowledge of this API can also be
useful when working with native addons for Node.js written in C++, although for
new projects [N-API][] is typically the better alternative.
V8 does not provide much public API documentation beyond what is
available in its C++ header files, most importantly `v8.h`, which can be
accessed online in the following locations:
* On GitHub: [`v8.h` in Node.js master][]
* On GitHub: [`v8.h` in V8 master][]
* On the Chromium projectâs Code Search application: [`v8.h` in Code Search][]
V8 also provides an [introduction for V8 embedders][],
which can be useful for understanding some of the concepts it uses in its
embedder API.
Important concepts when using V8 are the ones of [`Isolate`][]s and
[JavaScript value handles][].
## libuv API documentation
The other major dependency of Node.js is [libuv][], providing
the [event loop][] and other operation system abstractions to Node.js.
There is a [reference documentation for the libuv API][].
## File structure
The Node.js C++ files follow this structure:
The `.h` header files contain declarations, and sometimes definitions that donât
require including other headers (e.g. getters, setters, etc.). They should only
include other `.h` header files and nothing else.
The `-inl.h` header files contain definitions of inline functions from the
corresponding `.h` header file (e.g. functions marked `inline` in the
declaration or `template` functions). They always include the corresponding
`.h` header file, and can include other `.h` and `-inl.h` header files as
needed. It is not mandatory to split out the definitions from the `.h` file
into an `-inl.h` file, but it becomes necessary when there are multiple
definitions and contents of other `-inl.h` files start being used. Therefore, it
is recommended to split a `-inl.h` file when inline functions become longer than
a few lines to keep the corresponding `.h` file readable and clean. All visible
definitions from the `-inl.h` file should be declared in the corresponding `.h`
header file.
The `.cc` files contain definitions of non-inline functions from the
corresponding `.h` header file. They always include the corresponding `.h`
header file, and can include other `.h` and `-inl.h` header files as needed.
## Helpful concepts
A number of concepts are involved in putting together Node.js on top of V8 and
libuv. This section aims to explain some of them and how they work together.
<a id="isolate"></a>
### `Isolate`
The `v8::Isolate` class represents a single JavaScript engine instance, in
particular a set of JavaScript objects that can refer to each other
(the âheapâ).
The `v8::Isolate` is often passed to other V8 API functions, and provides some
APIs for managing the behaviour of the JavaScript engine or querying about its
current state or statistics such as memory usage.
V8 APIs are not thread-safe unless explicitly specified. In a typical Node.js
application, the main thread and any `Worker` threads each have one `Isolate`,
and JavaScript objects from one `Isolate` cannot refer to objects from
another `Isolate`.
Garbage collection, as well as other operations that affect the entire heap,
happen on a per-`Isolate` basis.
Typical ways of accessing the current `Isolate` in the Node.js code are:
* Given a `FunctionCallbackInfo` for a [binding function][],
using `args.GetIsolate()`.
* Given a [`Context`][], using `context->GetIsolate()`.
* Given a [`Environment`][], using `env->isolate()`.
### V8 JavaScript values
V8 provides classes that mostly correspond to JavaScript types; for example,
`v8::Value` is a class representing any kind of JavaScript type, with
subclasses such as `v8::Number` (which in turn has subclasses like `v8::Int32`),
`v8::Boolean` or `v8::Object`. Most types are represented by subclasses
of `v8::Object`, e.g. `v8::Uint8Array` or `v8::Date`.
<a id="internal-fields"></a>
### Internal fields
V8 provides the ability to store data in so-called âinternal fieldsâ inside
`v8::Object`s that were created as instances of C++-backed classes. The number
of fields needs to be defined when creating that class.
Both JavaScript values and `void*` pointers may be stored in such fields.
In most native Node.js objects, the first internal field is used to store a
pointer to a [`BaseObject`][] subclass, which then contains all relevant
information associated with the JavaScript object.
Typical ways of working with internal fields are:
* `obj->InternalFieldCount()` to look up the number of internal fields for an
object (`0` for regular JavaScript objects).
* `obj->GetInternalField(i)` to get a JavaScript value from an internal field.
* `obj->SetInternalField(i, v)` to store a JavaScript value in an
internal field.
* `obj->GetAlignedPointerFromInternalField(i)` to get a `void*` pointer from an
internal field.
* `obj->SetAlignedPointerInInternalField(i, p)` to store a `void*` pointer in an
internal field.
[`Context`][]s provide the same feature under the name âembedder dataâ.
<a id="js-handles"></a>
### JavaScript value handles
All JavaScript values are accessed through the V8 API through so-called handles,
of which there are two types: [`Local`][]s and [`Global`][]s.
<a id="local-handles"></a>
#### `Local` handles
A `v8::Local` handle is a temporary pointer to a JavaScript object, where
âtemporaryâ usually means that is no longer needed after the current function
is done executing. `Local` handles can only be allocated on the C++ stack.
Most of the V8 API uses `Local` handles to work with JavaScript values or return
them from functions.
Whenever a `Local` handle is created, a `v8::HandleScope` or
`v8::EscapableHandleScope` object must exist on the stack. The `Local` is then
added to that scope and deleted along with it.
When inside a [binding function][], a `HandleScope` already exists outside of
it, so there is no need to explicitly create one.
`EscapableHandleScope`s can be used to allow a single `Local` handle to be
passed to the outer scope. This is useful when a function returns a `Local`.
The following JavaScript and C++ functions are mostly equivalent:
```js
function getFoo(obj) {
return obj.foo;
}
```
```cpp
v8::Local<v8::Value> GetFoo(v8::Local<v8::Context> context,
v8::Local<v8::Object> obj) {
v8::Isolate* isolate = context->GetIsolate();
v8::EscapableHandleScope handle_scope(isolate);
// The 'foo_string' handle cannot be returned from this function because
// it is not âescapedâ with `.Escape()`.
v8::Local<v8::String> foo_string =
v8::String::NewFromUtf8(isolate, "foo").ToLocalChecked();
v8::Local<v8::Value> return_value;
if (obj->Get(context, foo_string).ToLocal(&return_value)) {
return handle_scope.Escape(return_value);
} else {
// There was a JS exception! Handle it somehow.
return v8::Local<v8::Value>();
}
}
```
See [exception handling][] for more information about the usage of `.To()`,
`.ToLocalChecked()`, `v8::Maybe` and `v8::MaybeLocal` usage.
##### Casting local handles
If it is known that a `Local<Value>` refers to a more specific type, it can
be cast to that type using `.As<...>()`:
```cpp
v8::Local<v8::Value> some_value;
// CHECK() is a Node.js utilitity that works similar to assert().
CHECK(some_value->IsUint8Array());
v8::Local<v8::Uint8Array> as_uint8 = some_value.As<v8::Uint8Array>();
```
Generally, using `val.As<v8::X>()` is only valid if `val->IsX()` is true, and
failing to follow that rule may lead to crashes.
#####
没有合适的资源?快使用搜索试试~ 我知道了~
node-v16.8.0.tar.gz
1 下载量 111 浏览量
2024-04-09
22:37:45
上传
评论
收藏 61.26MB GZ 举报
温馨提示
Node.js,简称Node,是一个开源且跨平台的JavaScript运行时环境,它允许在浏览器外运行JavaScript代码。Node.js于2009年由Ryan Dahl创立,旨在创建高性能的Web服务器和网络应用程序。它基于Google Chrome的V8 JavaScript引擎,可以在Windows、Linux、Unix、Mac OS X等操作系统上运行。 Node.js的特点之一是事件驱动和非阻塞I/O模型,这使得它非常适合处理大量并发连接,从而在构建实时应用程序如在线游戏、聊天应用以及实时通讯服务时表现卓越。此外,Node.js使用了模块化的架构,通过npm(Node package manager,Node包管理器),社区成员可以共享和复用代码,极大地促进了Node.js生态系统的发展和扩张。 Node.js不仅用于服务器端开发。随着技术的发展,它也被用于构建工具链、开发桌面应用程序、物联网设备等。Node.js能够处理文件系统、操作数据库、处理网络请求等,因此,开发者可以用JavaScript编写全栈应用程序,这一点大大提高了开发效率和便捷性。 在实践中,许多大型企业和组织已经采用Node.js作为其Web应用程序的开发平台,如Netflix、PayPal和Walmart等。它们利用Node.js提高了应用性能,简化了开发流程,并且能更快地响应市场需求。
资源推荐
资源详情
资源评论
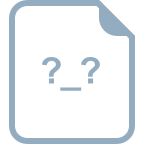
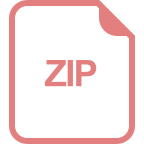
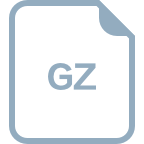
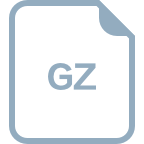
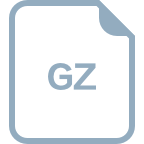
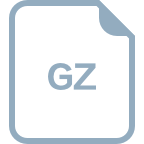
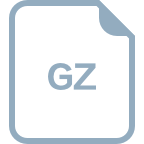
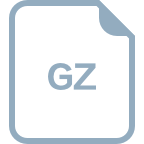
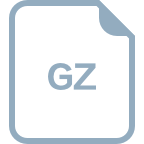
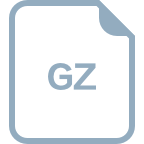
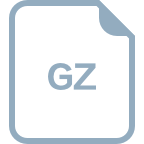
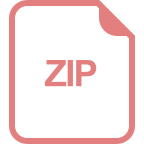
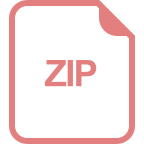
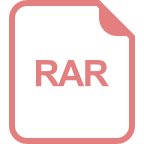
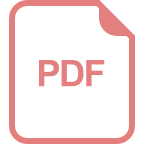
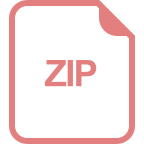
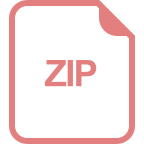
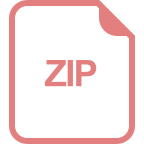
收起资源包目录

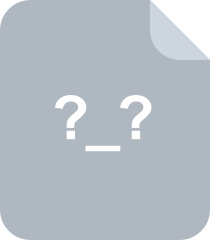
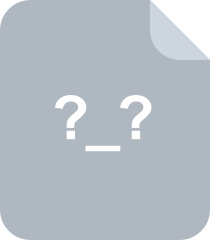
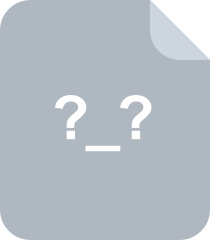
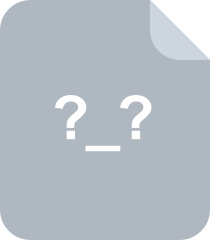
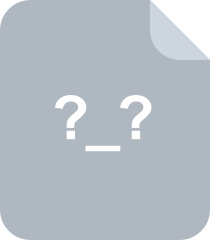
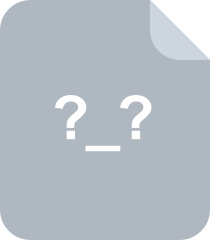
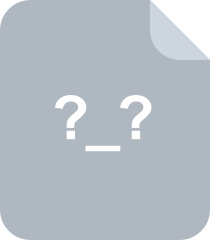
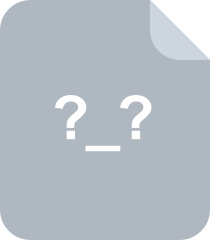
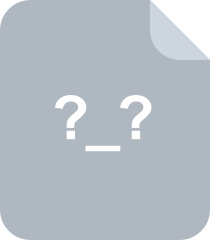
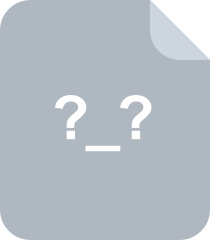
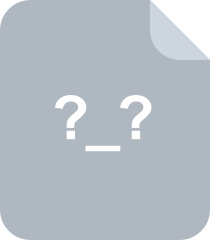
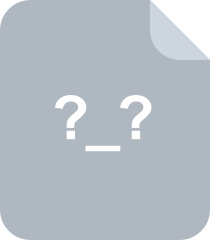
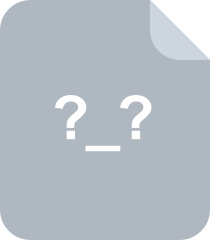
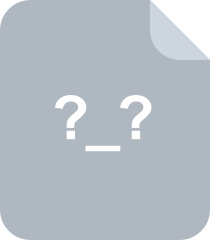
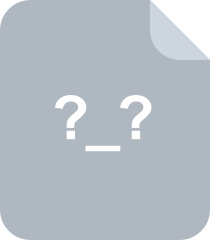
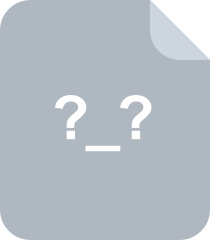
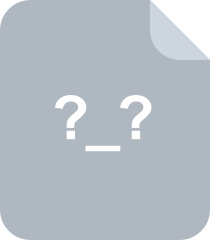
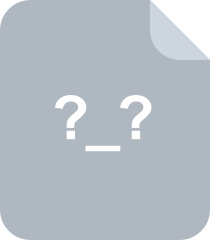
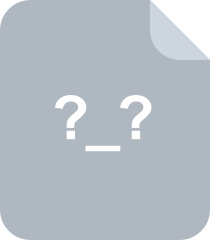
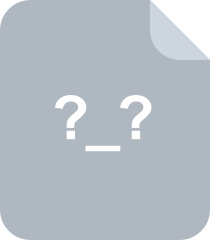
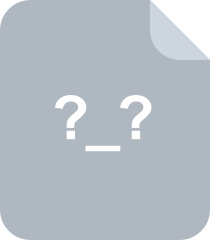
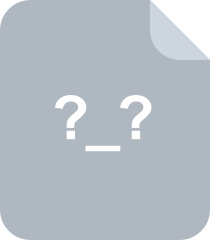
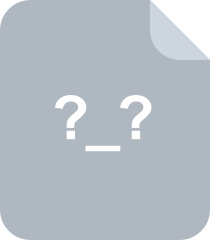
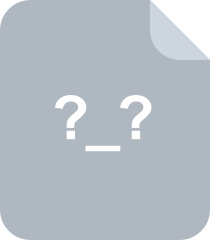
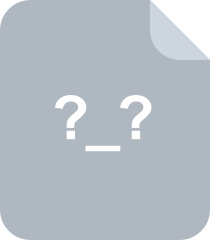
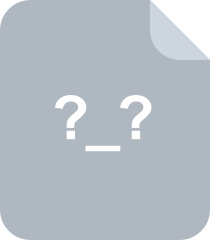
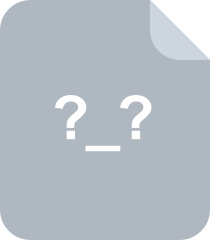
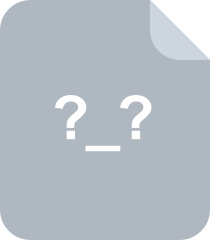
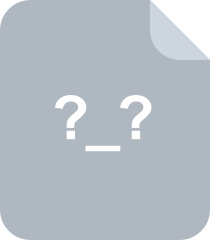
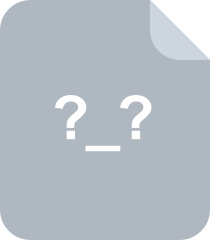
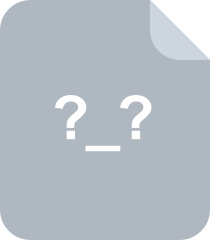
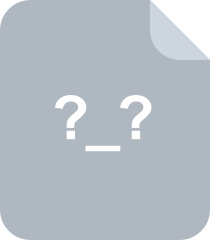
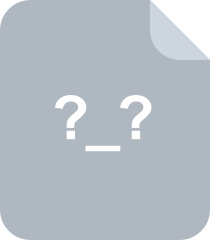
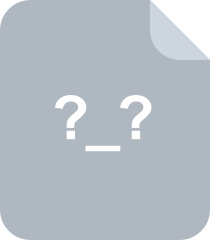
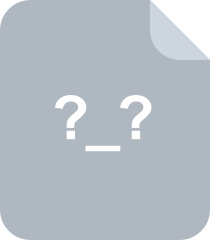
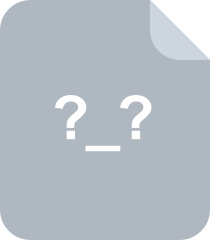
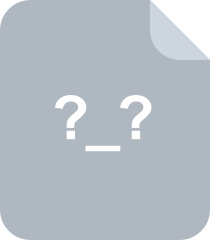
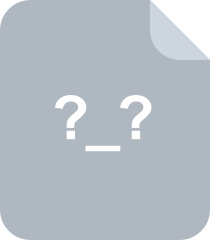
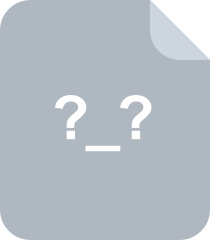
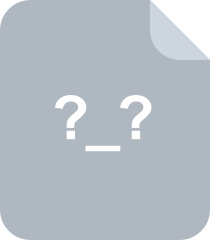
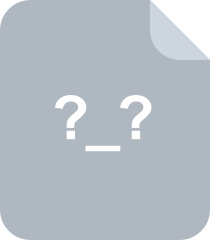
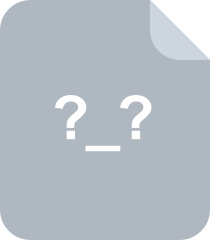
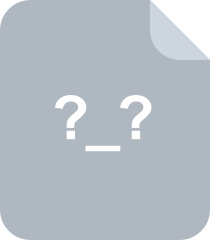
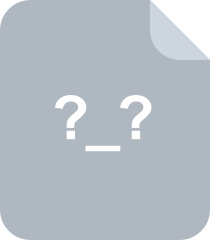
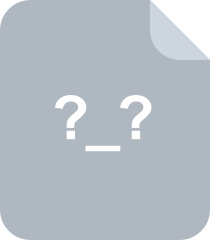
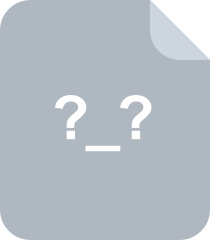
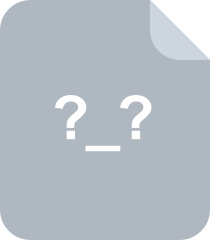
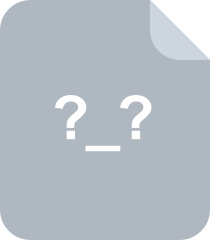
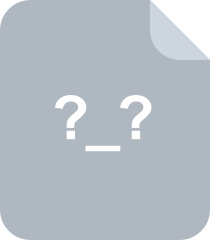
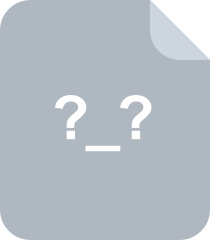
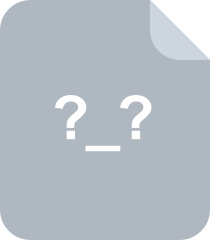
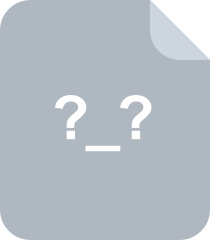
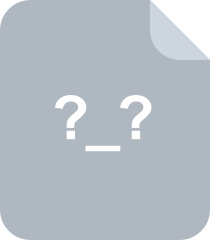
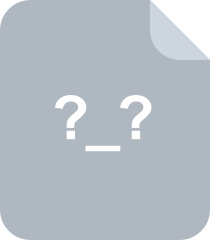
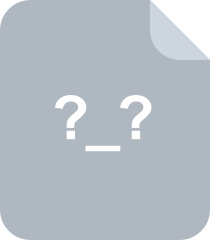
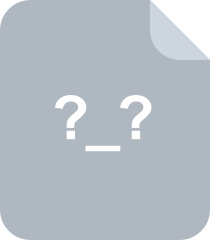
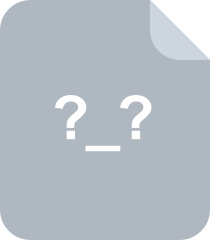
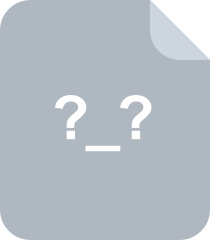
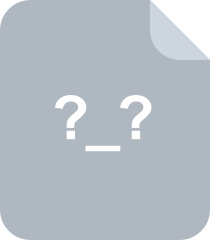
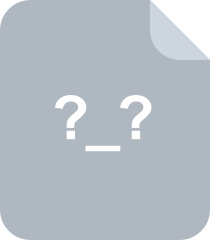
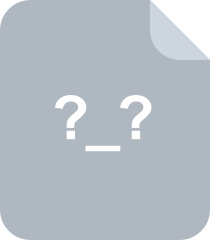
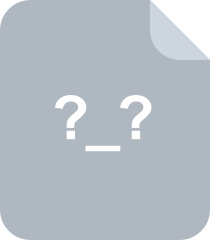
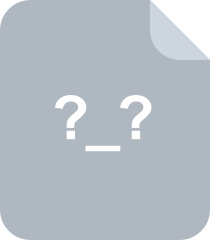
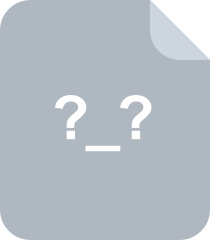
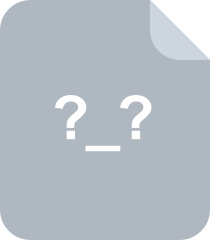
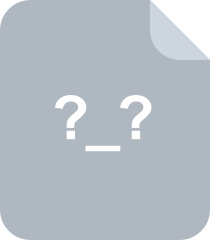
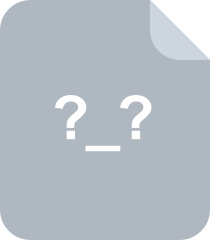
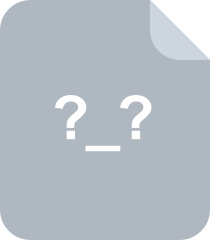
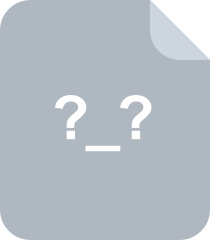
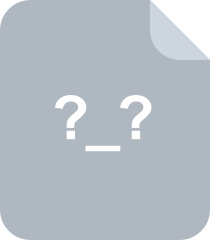
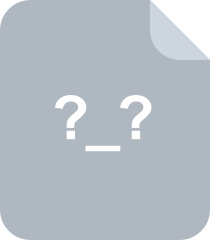
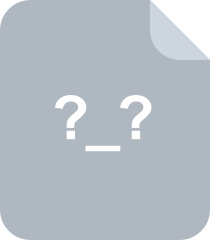
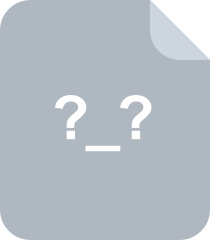
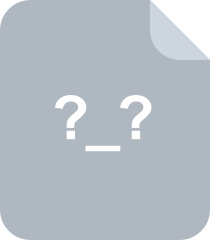
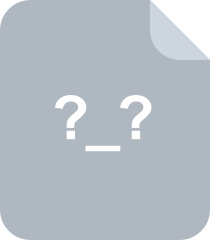
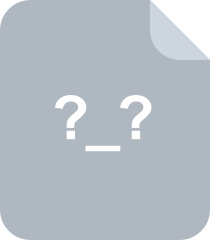
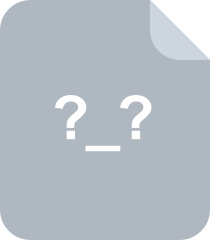
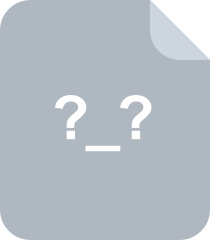
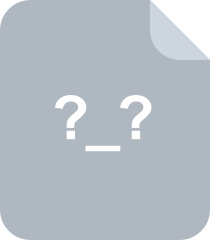
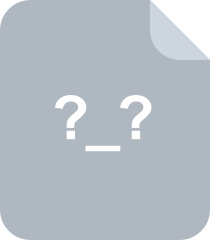
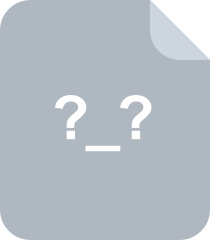
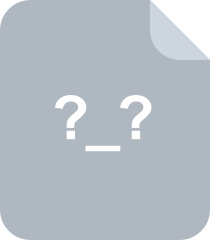
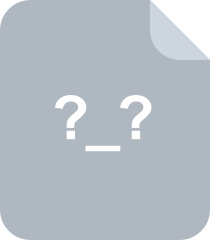
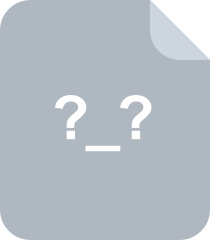
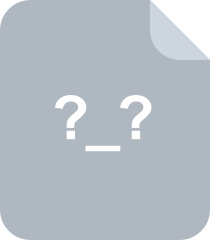
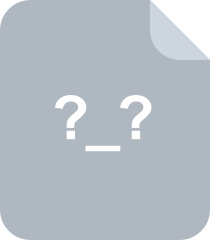
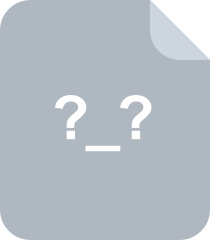
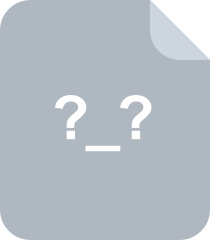
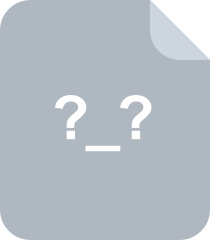
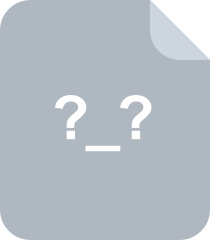
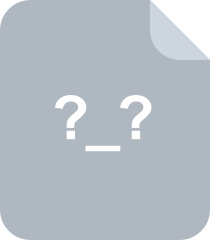
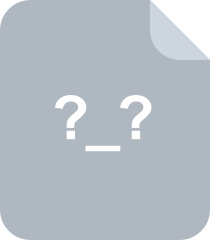
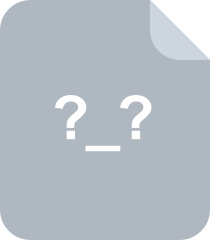
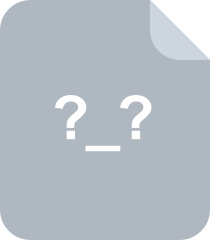
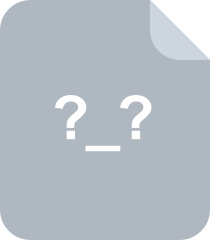
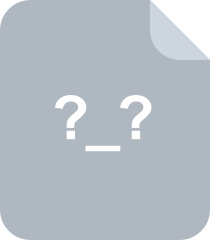
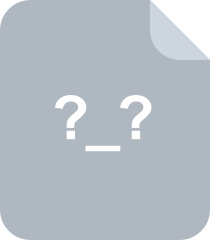
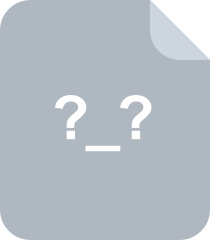
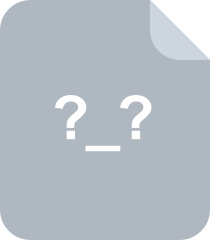
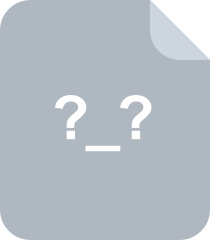
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
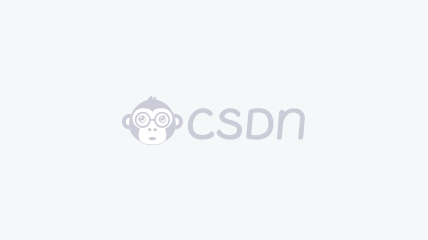

程序员Chino的日记
- 粉丝: 3693
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

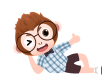
最新资源
- MATLAB实现EMD-iCHOA+GRU基于经验模态分解-改进黑猩猩算法优化门控循环单元的时间序列预测(含完整的程序和代码详解)
- christmasTree-圣诞树html网页代码
- LabVIEW-Version-Selector-labview
- awesome-ios-swift
- Servlet-servlet
- temperature-humidity-monitoring-system-labview
- javakeshe-java课程设计
- HormanyOs-notion鸿蒙版-鸿蒙
- Awesome-BUPT-Projects-自然语言处理课程设计
- JavaTest01-java课程设计
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


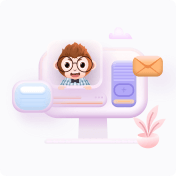
安全验证
文档复制为VIP权益,开通VIP直接复制
