# Glob
Match files using the patterns the shell uses.
The most correct and second fastest glob implementation in
JavaScript. (See **Comparison to Other JavaScript Glob
Implementations** at the bottom of this readme.)

## Usage
Install with npm
```
npm i glob
```
**Note** the npm package name is _not_ `node-glob` that's a
different thing that was abandoned years ago. Just `glob`.
```js
// load using import
import { glob, globSync, globStream, globStreamSync, Glob } from 'glob'
// or using commonjs, that's fine, too
const {
glob,
globSync,
globStream,
globStreamSync,
Glob,
} = require('glob')
// the main glob() and globSync() resolve/return array of filenames
// all js files, but don't look in node_modules
const jsfiles = await glob('**/*.js', { ignore: 'node_modules/**' })
// pass in a signal to cancel the glob walk
const stopAfter100ms = await glob('**/*.css', {
signal: AbortSignal.timeout(100),
})
// multiple patterns supported as well
const images = await glob(['css/*.{png,jpeg}', 'public/*.{png,jpeg}'])
// but of course you can do that with the glob pattern also
// the sync function is the same, just returns a string[] instead
// of Promise<string[]>
const imagesAlt = globSync('{css,public}/*.{png,jpeg}')
// you can also stream them, this is a Minipass stream
const filesStream = globStream(['**/*.dat', 'logs/**/*.log'])
// construct a Glob object if you wanna do it that way, which
// allows for much faster walks if you have to look in the same
// folder multiple times.
const g = new Glob('**/foo', {})
// glob objects are async iterators, can also do globIterate() or
// g.iterate(), same deal
for await (const file of g) {
console.log('found a foo file:', file)
}
// pass a glob as the glob options to reuse its settings and caches
const g2 = new Glob('**/bar', g)
// sync iteration works as well
for (const file of g2) {
console.log('found a bar file:', file)
}
// you can also pass withFileTypes: true to get Path objects
// these are like a Dirent, but with some more added powers
// check out http://npm.im/path-scurry for more info on their API
const g3 = new Glob('**/baz/**', { withFileTypes: true })
g3.stream().on('data', path => {
console.log(
'got a path object',
path.fullpath(),
path.isDirectory(),
path.readdirSync().map(e => e.name)
)
})
// if you use stat:true and withFileTypes, you can sort results
// by things like modified time, filter by permission mode, etc.
// All Stats fields will be available in that case. Slightly
// slower, though.
// For example:
const results = await glob('**', { stat: true, withFileTypes: true })
const timeSortedFiles = results
.sort((a, b) => a.mtimeMs - b.mtimeMs)
.map(path => path.fullpath())
const groupReadableFiles = results
.filter(path => path.mode & 0o040)
.map(path => path.fullpath())
// custom ignores can be done like this, for example by saying
// you'll ignore all markdown files, and all folders named 'docs'
const customIgnoreResults = await glob('**', {
ignore: {
ignored: p => /\.md$/.test(p.name),
childrenIgnored: p => p.isNamed('docs'),
},
})
// another fun use case, only return files with the same name as
// their parent folder, plus either `.ts` or `.js`
const folderNamedModules = await glob('**/*.{ts,js}', {
ignore: {
ignored: p => {
const pp = p.parent
return !(p.isNamed(pp.name + '.ts') || p.isNamed(pp.name + '.js'))
},
},
})
// find all files edited in the last hour, to do this, we ignore
// all of them that are more than an hour old
const newFiles = await glob('**', {
// need stat so we have mtime
stat: true,
// only want the files, not the dirs
nodir: true,
ignore: {
ignored: p => {
return new Date() - p.mtime > 60 * 60 * 1000
},
// could add similar childrenIgnored here as well, but
// directory mtime is inconsistent across platforms, so
// probably better not to, unless you know the system
// tracks this reliably.
},
})
```
**Note** Glob patterns should always use `/` as a path separator,
even on Windows systems, as `\` is used to escape glob
characters. If you wish to use `\` as a path separator _instead
of_ using it as an escape character on Windows platforms, you may
set `windowsPathsNoEscape:true` in the options. In this mode,
special glob characters cannot be escaped, making it impossible
to match a literal `*` `?` and so on in filenames.
## Command Line Interface
```
$ glob -h
Usage:
glob [options] [<pattern> [<pattern> ...]]
Expand the positional glob expression arguments into any matching file system
paths found.
-c<command> --cmd=<command>
Run the command provided, passing the glob expression
matches as arguments.
-A --all By default, the glob cli command will not expand any
arguments that are an exact match to a file on disk.
This prevents double-expanding, in case the shell
expands an argument whose filename is a glob
expression.
For example, if 'app/*.ts' would match 'app/[id].ts',
then on Windows powershell or cmd.exe, 'glob app/*.ts'
will expand to 'app/[id].ts', as expected. However, in
posix shells such as bash or zsh, the shell will first
expand 'app/*.ts' to a list of filenames. Then glob
will look for a file matching 'app/[id].ts' (ie,
'app/i.ts' or 'app/d.ts'), which is unexpected.
Setting '--all' prevents this behavior, causing glob to
treat ALL patterns as glob expressions to be expanded,
even if they are an exact match to a file on disk.
When setting this option, be sure to enquote arguments
so that the shell will not expand them prior to passing
them to the glob command process.
-a --absolute Expand to absolute paths
-d --dot-relative Prepend './' on relative matches
-m --mark Append a / on any directories matched
-x --posix Always resolve to posix style paths, using '/' as the
directory separator, even on Windows. Drive letter
absolute matches on Windows will be expanded to their
full resolved UNC maths, eg instead of 'C:\foo\bar', it
will expand to '//?/C:/foo/bar'.
-f --follow Follow symlinked directories when expanding '**'
-R --realpath Call 'fs.realpath' on all of the results. In the case
of an entry that cannot be resolved, the entry is
omitted. This incurs a slight performance penalty, of
course, because of the added system calls.
-s --stat Call 'fs.lstat' on all entries, whether required or not
to determine if it's a valid match.
-b --match-base Perform a basename-only match if the pattern does not
contain any slash characters. That is, '*.js' would be
treated as equivalent to '**/*.js', matching js files
in all directories.
--dot Allow patterns to match files/directories that start
with '.', even if the pattern does not start with '.'
--nobrace Do not expand {...} patterns
--nocase Perform a case-insensitive match. This defaults to
'true' on macOS and Windows platforms, and false on all
others.
Note:
没有合适的资源?快使用搜索试试~ 我知道了~
node-v20.12.1.tar.gz
1 下载量 12 浏览量
2024-04-09
22:59:40
上传
评论
收藏 84.18MB GZ 举报
温馨提示
Node.js,简称Node,是一个开源且跨平台的JavaScript运行时环境,它允许在浏览器外运行JavaScript代码。Node.js于2009年由Ryan Dahl创立,旨在创建高性能的Web服务器和网络应用程序。它基于Google Chrome的V8 JavaScript引擎,可以在Windows、Linux、Unix、Mac OS X等操作系统上运行。 Node.js的特点之一是事件驱动和非阻塞I/O模型,这使得它非常适合处理大量并发连接,从而在构建实时应用程序如在线游戏、聊天应用以及实时通讯服务时表现卓越。此外,Node.js使用了模块化的架构,通过npm(Node package manager,Node包管理器),社区成员可以共享和复用代码,极大地促进了Node.js生态系统的发展和扩张。 Node.js不仅用于服务器端开发。随着技术的发展,它也被用于构建工具链、开发桌面应用程序、物联网设备等。Node.js能够处理文件系统、操作数据库、处理网络请求等,因此,开发者可以用JavaScript编写全栈应用程序,这一点大大提高了开发效率和便捷性。 在实践中,许多大型企业和组织已经采用Node.js作为其Web应用程序的开发平台,如Netflix、PayPal和Walmart等。它们利用Node.js提高了应用性能,简化了开发流程,并且能更快地响应市场需求。
资源推荐
资源详情
资源评论
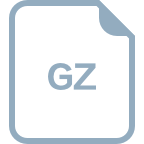
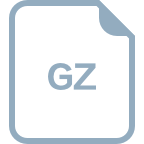
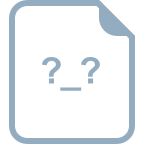
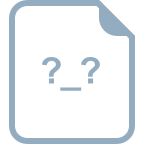
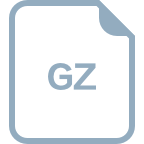
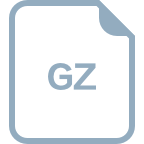
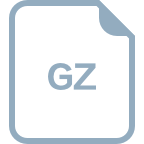
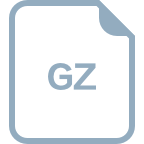
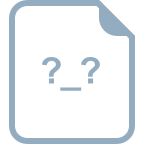
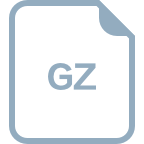
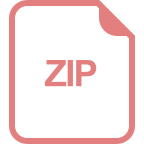
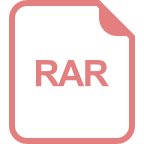
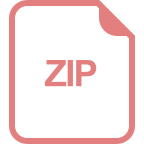
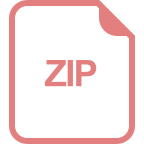
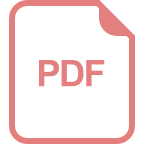
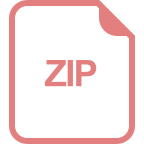
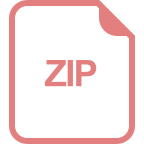
收起资源包目录

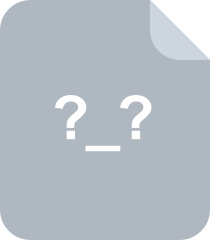
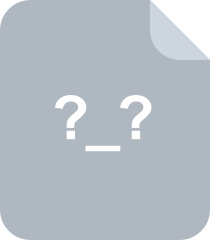
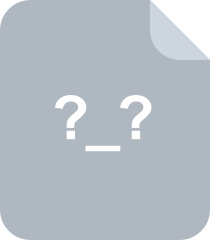
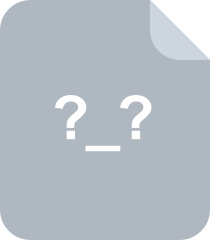
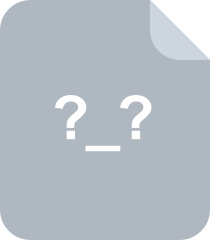
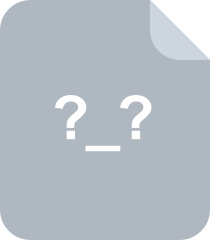
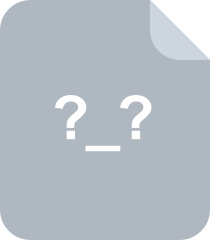
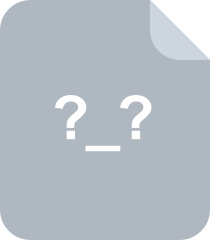
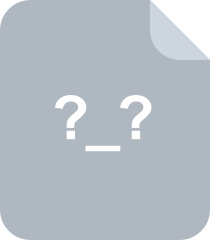
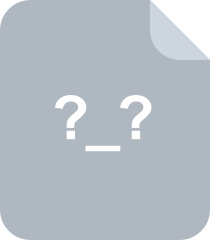
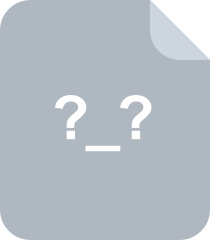
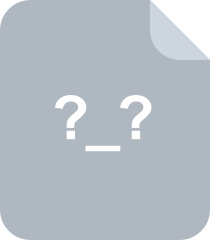
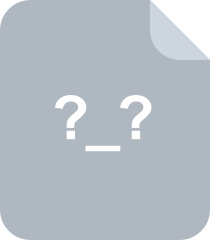
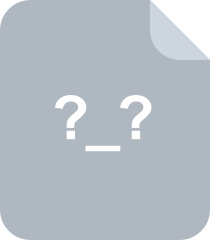
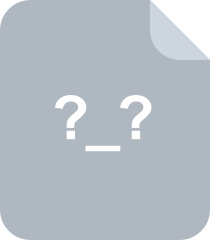
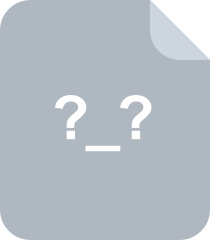
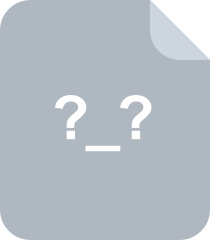
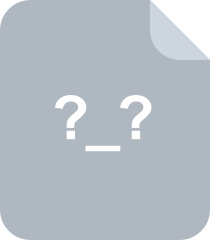
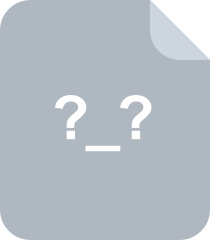
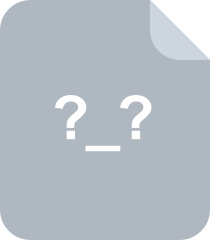
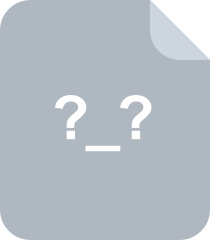
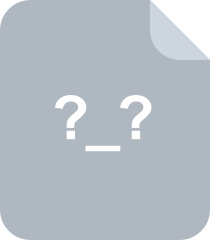
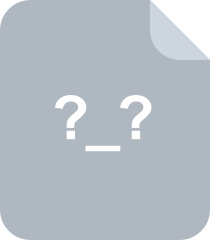
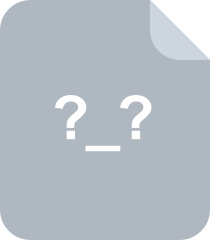
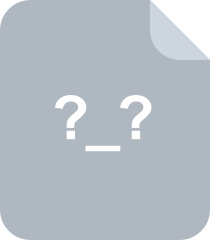
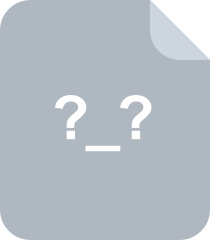
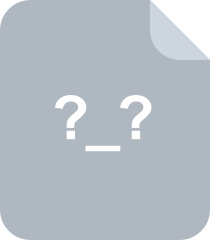
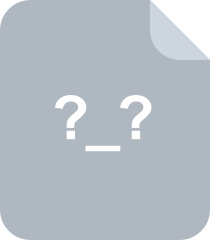
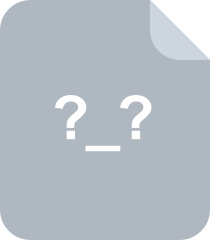
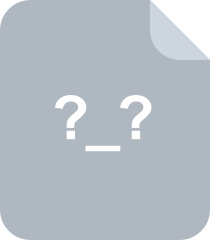
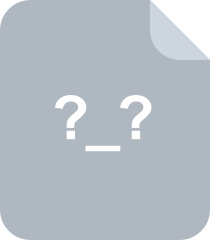
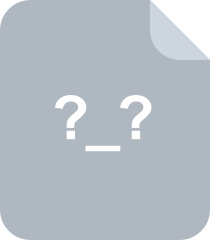
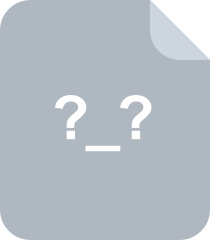
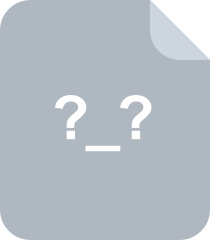
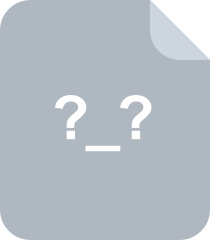
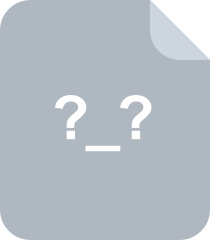
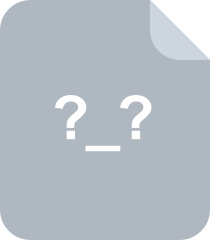
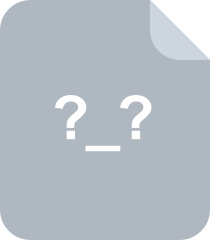
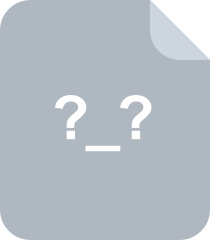
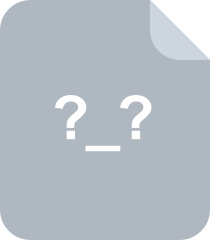
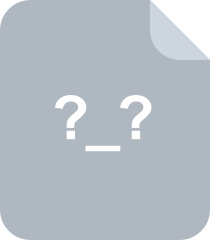
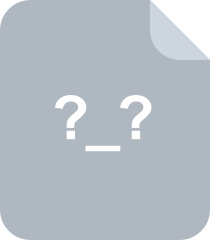
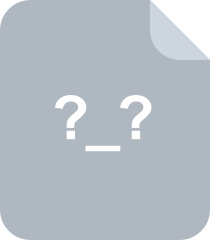
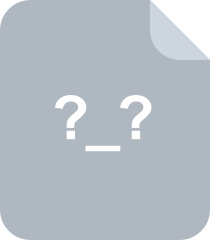
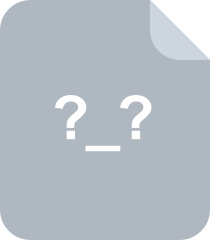
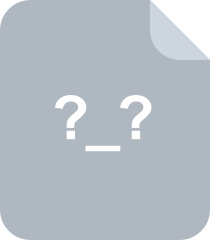
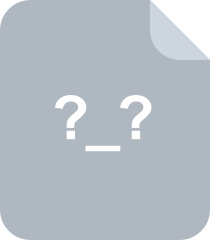
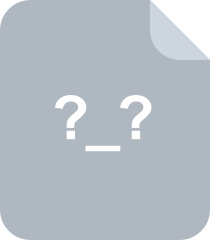
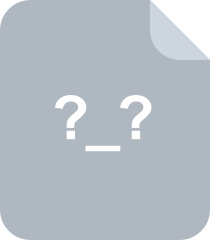
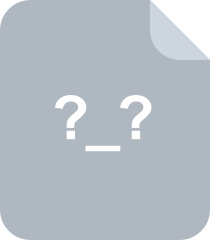
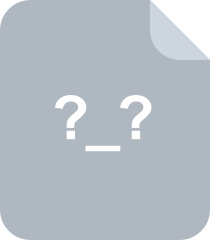
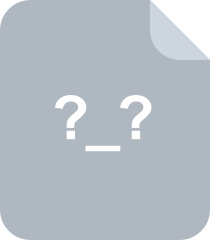
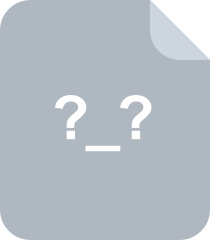
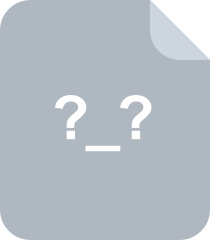
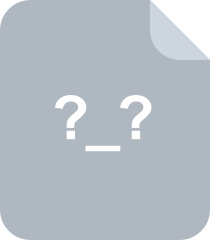
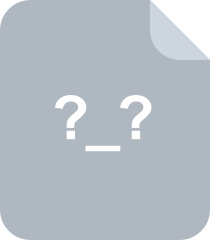
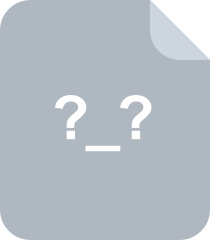
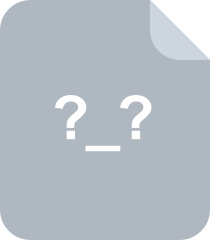
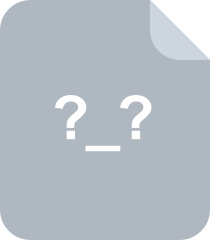
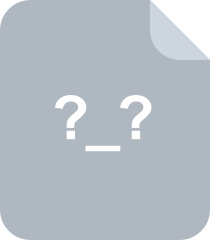
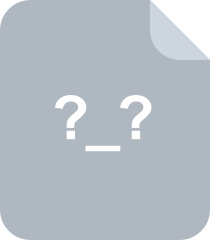
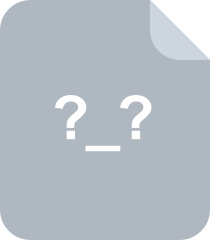
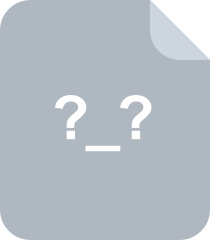
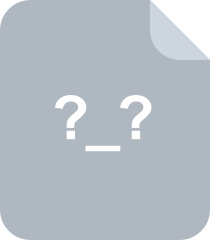
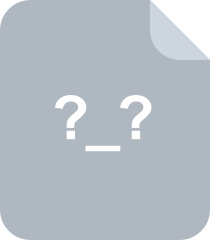
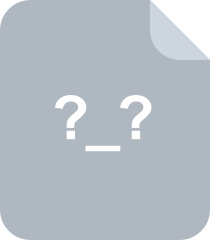
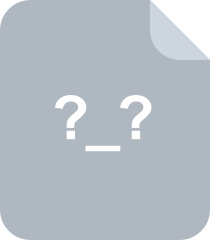
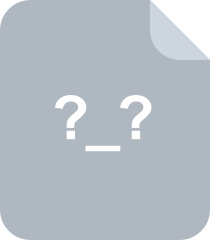
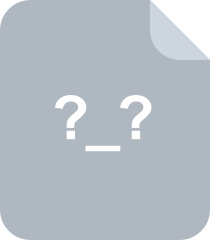
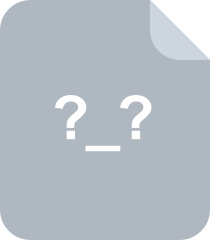
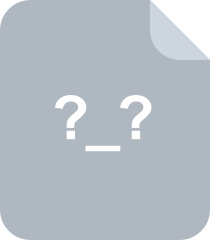
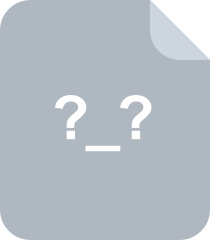
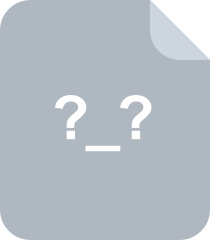
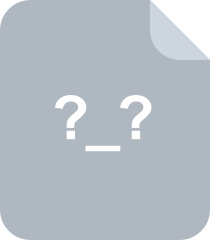
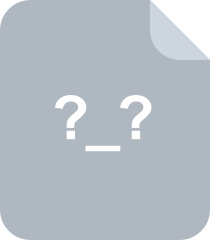
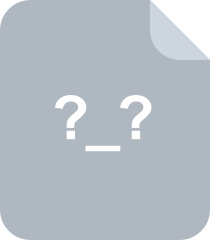
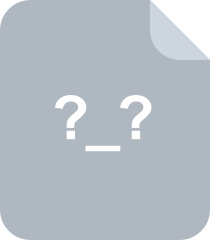
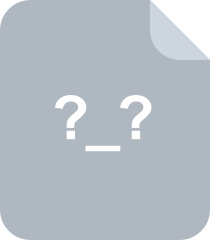
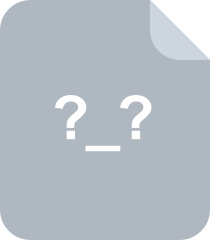
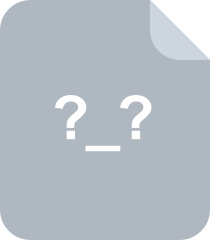
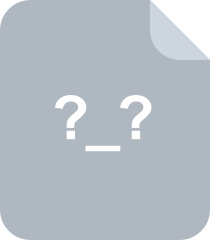
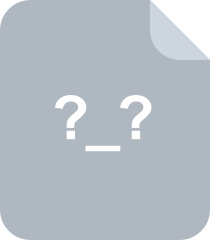
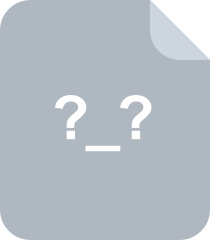
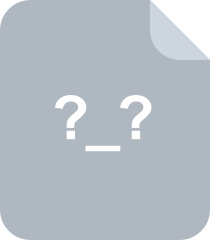
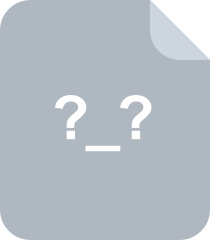
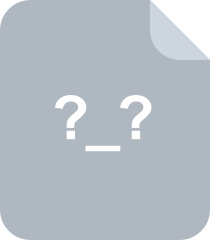
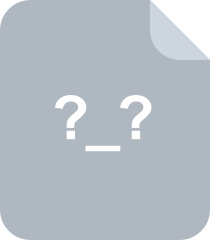
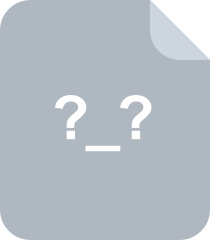
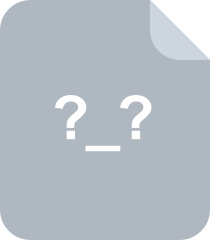
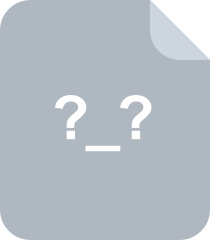
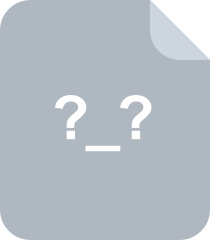
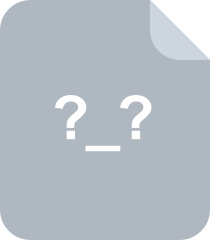
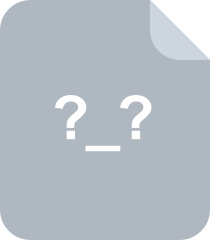
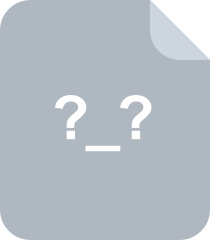
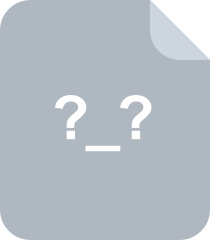
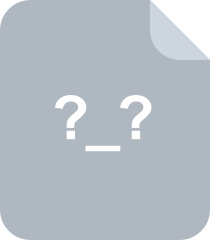
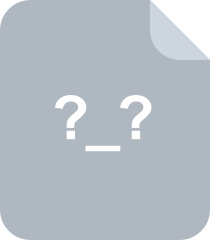
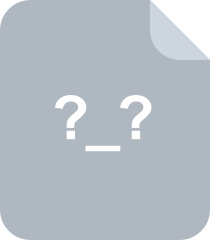
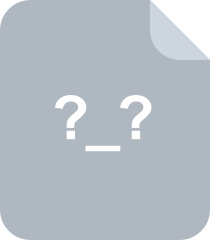
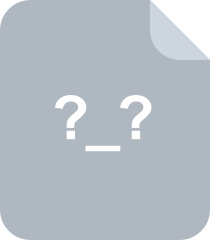
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
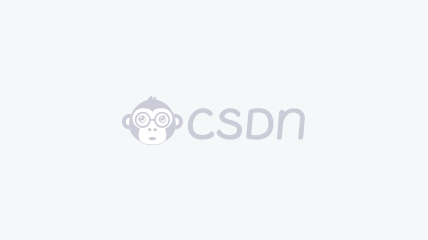

程序员Chino的日记
- 粉丝: 3664
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

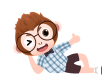
最新资源
- 星光暗区巨魔绘制自瞄V6.1.ipa
- HTML川剧网站源码.zip
- (源码)基于Maven + MyBatis的图书管理系统.zip
- 2024 年 10 月 26 日,第 19 届中国 Linux 内核开发者大会于湖北武汉成功举办 来自全国各地的近 400 名内核开发者相约华中科技大学,聆听讲座,共商 Linux 内核未来之发展12
- (源码)基于C++的仓储盘点系统.zip
- linux常用命令大全
- mongodb-compass-1.44.6-darwin-x64.dmg
- (源码)基于JavaFX和MyBatis的HSY寝室管理系统.zip
- 对AVEC2014视频进行Dlib或MTCNN人脸裁剪
- excel数据分析案例1数据
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


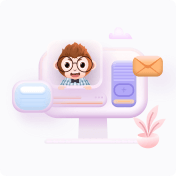
安全验证
文档复制为VIP权益,开通VIP直接复制
