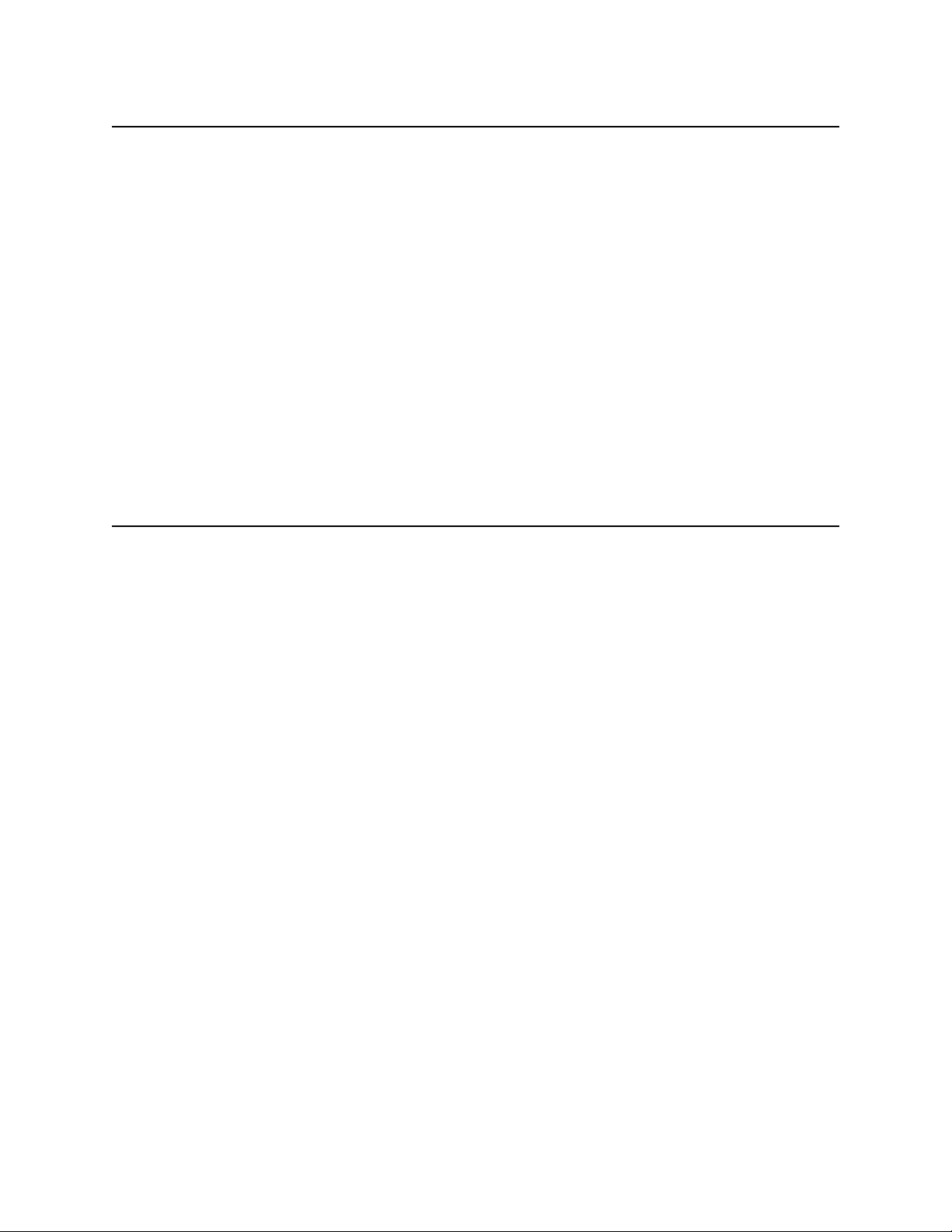
The Python Library Reference
Release 3.8.19
Guido van Rossum
and the Python development team
March 19, 2024
Python Software Foundation
Email: docs@python.org
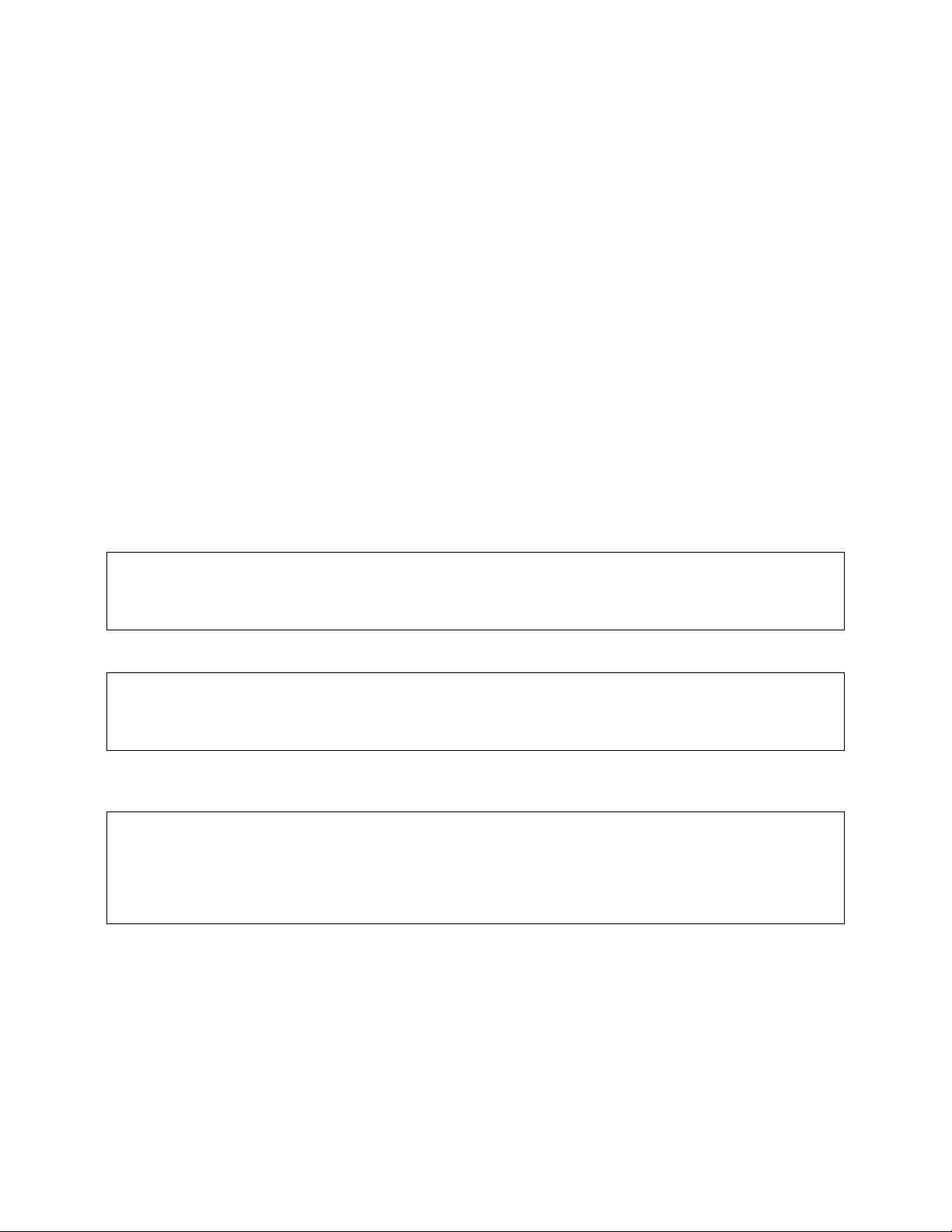
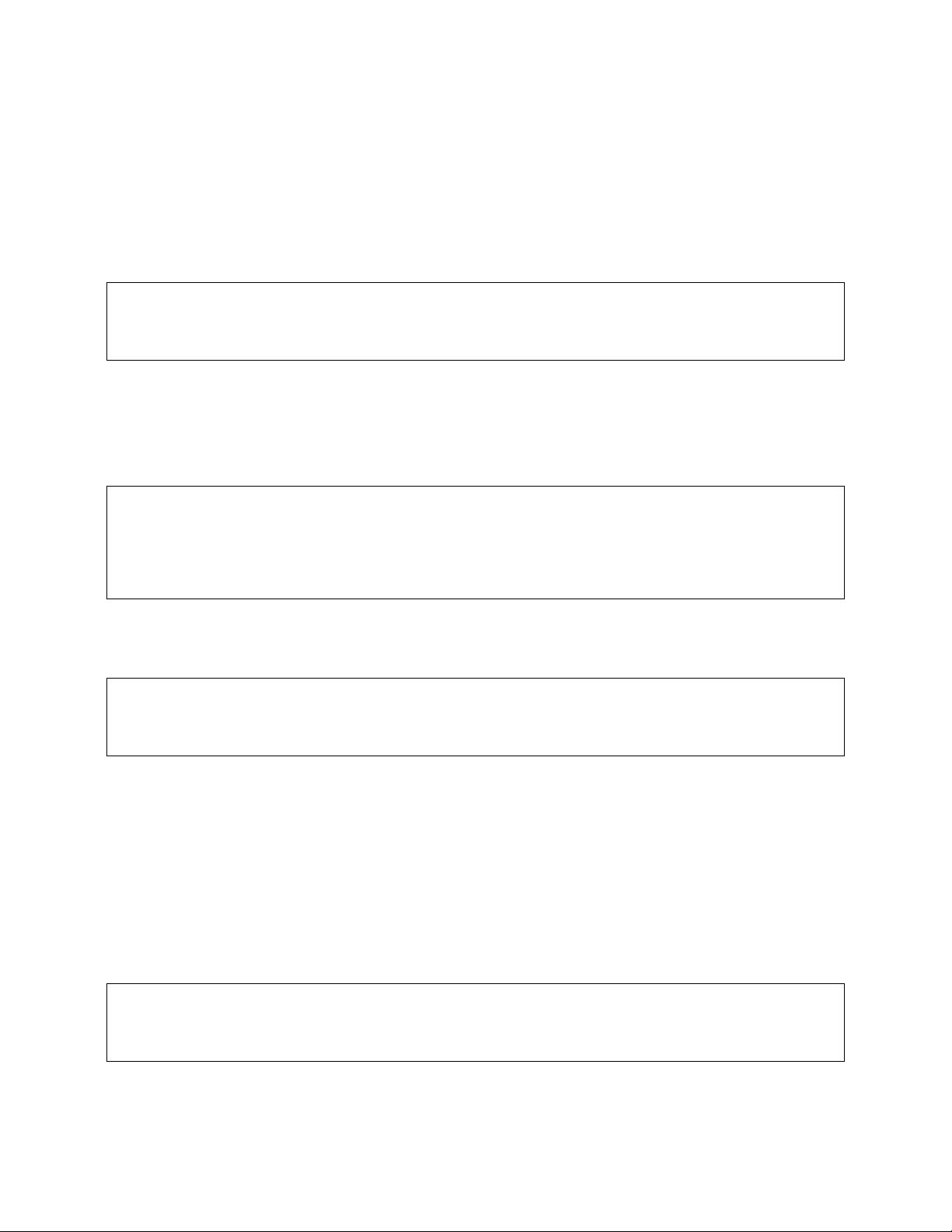
CONTENTS
1 Introduction 3
1.1 Notes on availability . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
2 Built-in Functions 5
3 Built-in Constants 27
3.1 Constants added by the site module . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 28
4 Built-in Types 29
4.1 Truth Value Testing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
4.2 Boolean Operations — and, or, not . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
4.3 Comparisons . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30
4.4 Numeric Types — int, float, complex . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30
4.5 Iterator Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 36
4.6 Sequence Types — list, tuple, range . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
4.7 Text Sequence Type — str . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 43
4.8 Binary Sequence Types — bytes, bytearray, memoryview . . . . . . . . . . . . . . . . . . . 53
4.9 Set Types — set, frozenset . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 75
4.10 Mapping Types — dict . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 77
4.11 Context Manager Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 82
4.12 Other Built-in Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
4.13 Special Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 85
4.14 Integer string conversion length limitation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 86
5 Built-in Exceptions 89
5.1 Base classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
5.2 Concrete exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
5.3 Warnings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 96
5.4 Exception hierarchy . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 97
6 Text Processing Services 99
6.1 string — Common string operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 99
6.2 re — Regular expression operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 110
6.3 difflib — Helpers for computing deltas . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 130
6.4 textwrap — Text wrapping and lling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
6.5 unicodedata — Unicode Database . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144
6.6 stringprep — Internet String Preparation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 146
6.7 readline — GNU readline interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 147
6.8 rlcompleter — Completion function for GNU readline . . . . . . . . . . . . . . . . . . . . . . . 152
7 Binary Data Services 153
i
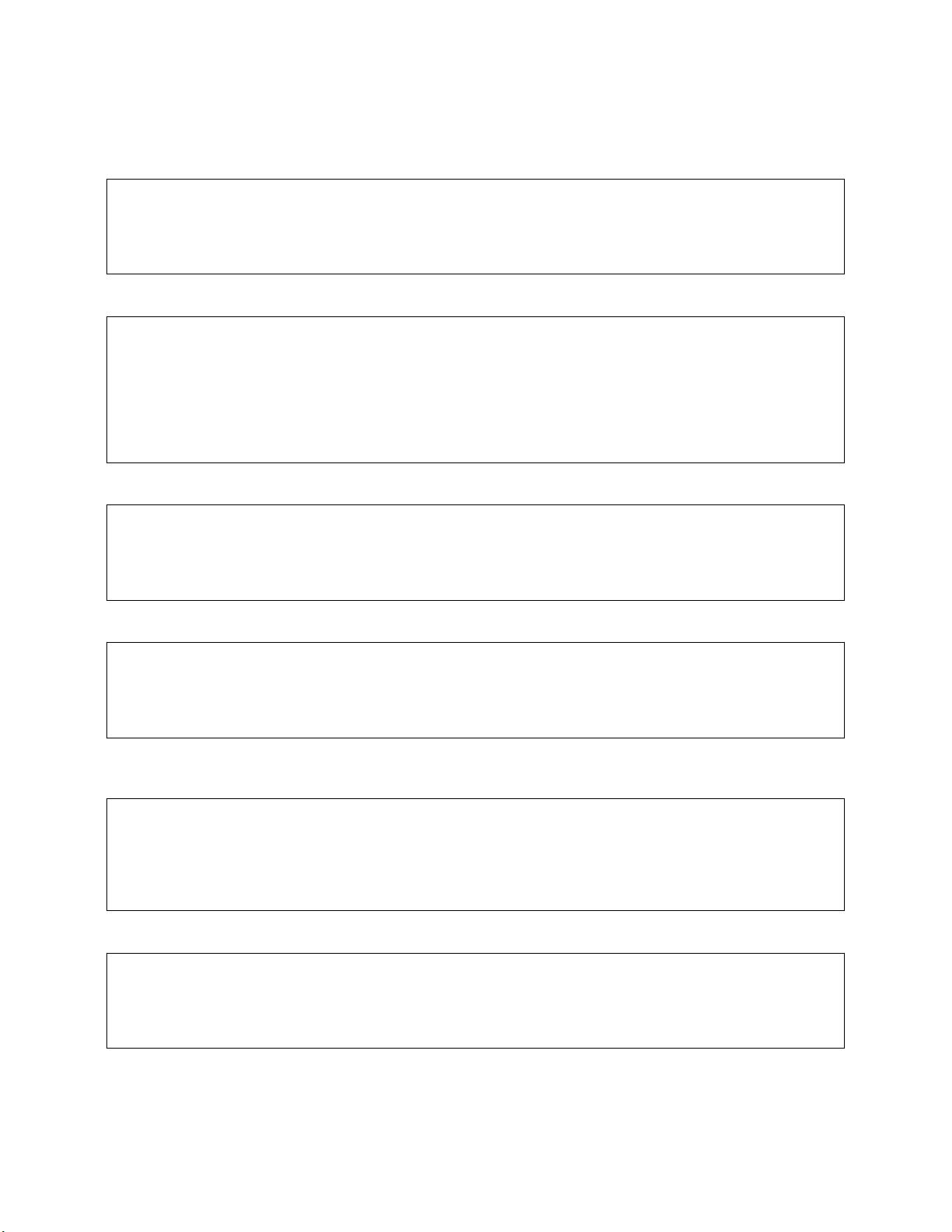
7.1 struct — Interpret bytes as packed binary data . . . . . . . . . . . . . . . . . . . . . . . . . . . . 153
7.2 codecs — Codec registry and base classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159
8 Data Types 177
8.1 datetime — Basic date and time types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 177
8.2 calendar — General calendar-related functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . 212
8.3 collections — Container datatypes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 217
8.4 collections.abc — Abstract Base Classes for Containers . . . . . . . . . . . . . . . . . . . . . 234
8.5 heapq — Heap queue algorithm . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 238
8.6 bisect — Array bisection algorithm . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 242
8.7 array — Ecient arrays of numeric values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 244
8.8 weakref — Weak references . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 247
8.9 types — Dynamic type creation and names for built-in types . . . . . . . . . . . . . . . . . . . . . 255
8.10 copy — Shallow and deep copy operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 260
8.11 pprint — Data pretty printer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 261
8.12 reprlib — Alternate repr() implementation . . . . . . . . . . . . . . . . . . . . . . . . . . . . 266
8.13 enum — Support for enumerations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 268
9 Numeric and Mathematical Modules 289
9.1 numbers — Numeric abstract base classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 289
9.2 math — Mathematical functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 292
9.3 cmath — Mathematical functions for complex numbers . . . . . . . . . . . . . . . . . . . . . . . . 298
9.4 decimal — Decimal xed point and oating point arithmetic . . . . . . . . . . . . . . . . . . . . . 302
9.5 fractions — Rational numbers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 329
9.6 random — Generate pseudo-random numbers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 331
9.7 statistics — Mathematical statistics functions . . . . . . . . . . . . . . . . . . . . . . . . . . . 338
10 Functional Programming Modules 349
10.1 itertools — Functions creating iterators for ecient looping . . . . . . . . . . . . . . . . . . . . 349
10.2 functools — Higher-order functions and operations on callable objects . . . . . . . . . . . . . . . 364
10.3 operator — Standard operators as functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 372
11 File and Directory Access 379
11.1 pathlib — Object-oriented lesystem paths . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 379
11.2 os.path — Common pathname manipulations . . . . . . . . . . . . . . . . . . . . . . . . . . . . 396
11.3 fileinput — Iterate over lines from multiple input streams . . . . . . . . . . . . . . . . . . . . . 401
11.4 stat — Interpreting stat() results . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 403
11.5 filecmp — File and Directory Comparisons . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 409
11.6 tempfile — Generate temporary les and directories . . . . . . . . . . . . . . . . . . . . . . . . . 411
11.7 glob — Unix style pathname pattern expansion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 415
11.8 fnmatch — Unix lename pattern matching . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 416
11.9 linecache — Random access to text lines . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 417
11.10 shutil — High-level le operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 418
12 Data Persistence 429
12.1 pickle — Python object serialization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 429
12.2 copyreg — Register pickle support functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . 446
12.3 shelve — Python object persistence . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 447
12.4 marshal — Internal Python object serialization . . . . . . . . . . . . . . . . . . . . . . . . . . . . 449
12.5 dbm — Interfaces to Unix “databases” . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 451
12.6 sqlite3 — DB-API 2.0 interface for SQLite databases . . . . . . . . . . . . . . . . . . . . . . . . 455
13 Data Compression and Archiving 477
13.1 zlib — Compression compatible with gzip . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 477
13.2 gzip — Support for gzip les . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 481
ii
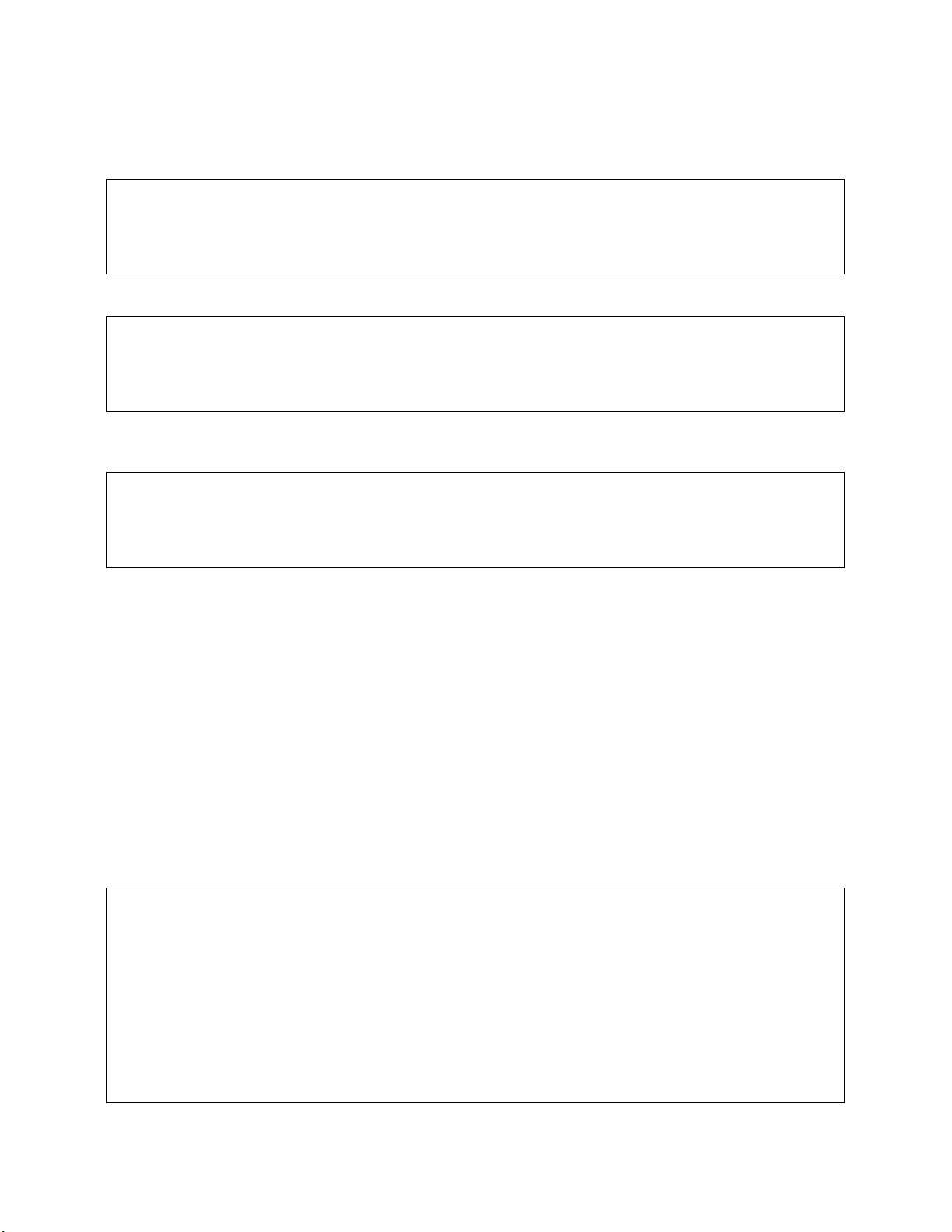
13.3 bz2 — Support for bzip2 compression . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 484
13.4 lzma — Compression using the LZMA algorithm . . . . . . . . . . . . . . . . . . . . . . . . . . . 488
13.5 zipfile — Work with ZIP archives . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 494
13.6 tarfile — Read and write tar archive les . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 503
14 File Formats 519
14.1 csv — CSV File Reading and Writing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 519
14.2 configparser — Conguration le parser . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 526
14.3 netrc — netrc le processing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 543
14.4 xdrlib — Encode and decode XDR data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 544
14.5 plistlib — Generate and parse Mac OS X .plist les . . . . . . . . . . . . . . . . . . . . . . 547
15 Cryptographic Services 551
15.1 hashlib — Secure hashes and message digests . . . . . . . . . . . . . . . . . . . . . . . . . . . . 551
15.2 hmac — Keyed-Hashing for Message Authentication . . . . . . . . . . . . . . . . . . . . . . . . . . 561
15.3 secrets — Generate secure random numbers for managing secrets . . . . . . . . . . . . . . . . . . 563
16 Generic Operating System Services 567
16.1 os — Miscellaneous operating system interfaces . . . . . . . . . . . . . . . . . . . . . . . . . . . . 567
16.2 io — Core tools for working with streams . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 619
16.3 time — Time access and conversions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 631
16.4 argparse — Parser for command-line options, arguments and sub-commands . . . . . . . . . . . . 641
16.5 getopt — C-style parser for command line options . . . . . . . . . . . . . . . . . . . . . . . . . . 674
16.6 logging — Logging facility for Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 676
16.7 logging.config — Logging conguration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 692
16.8 logging.handlers — Logging handlers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 703
16.9 getpass — Portable password input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 715
16.10 curses — Terminal handling for character-cell displays . . . . . . . . . . . . . . . . . . . . . . . . 716
16.11 curses.textpad — Text input widget for curses programs . . . . . . . . . . . . . . . . . . . . . 734
16.12 curses.ascii — Utilities for ASCII characters . . . . . . . . . . . . . . . . . . . . . . . . . . . 735
16.13 curses.panel — A panel stack extension for curses . . . . . . . . . . . . . . . . . . . . . . . . . 738
16.14 platform — Access to underlying platform’s identifying data . . . . . . . . . . . . . . . . . . . . . 739
16.15 errno — Standard errno system symbols . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 742
16.16 ctypes — A foreign function library for Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . 748
17 Concurrent Execution 783
17.1 threading — Thread-based parallelism . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 783
17.2 multiprocessing — Process-based parallelism . . . . . . . . . . . . . . . . . . . . . . . . . . . 796
17.3 multiprocessing.shared_memory — Provides shared memory for direct access across processes840
17.4 The concurrent package . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 844
17.5 concurrent.futures — Launching parallel tasks . . . . . . . . . . . . . . . . . . . . . . . . . 845
17.6 subprocess — Subprocess management . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 851
17.7 sched — Event scheduler . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 870
17.8 queue — A synchronized queue class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 871
17.9 contextvars — Context Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 875
17.10 _thread — Low-level threading API . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 879
17.11 _dummy_thread — Drop-in replacement for the _thread module . . . . . . . . . . . . . . . . . 881
17.12 dummy_threading — Drop-in replacement for the threading module . . . . . . . . . . . . . . 881
18 Networking and Interprocess Communication 883
18.1 asyncio — Asynchronous I/O . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 883
18.2 socket — Low-level networking interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 971
18.3 ssl — TLS/SSL wrapper for socket objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 995
18.4 select — Waiting for I/O completion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1031
18.5 selectors — High-level I/O multiplexing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1038
iii