# DO NOT EDIT THIS FILE!
#
# This file is generated from the CDP specification. If you need to make
# changes, edit the generator and regenerate all of the modules.
#
# CDP domain: Network
from __future__ import annotations
from .util import event_class, T_JSON_DICT
from dataclasses import dataclass
import enum
import typing
from . import debugger
from . import emulation
from . import io
from . import page
from . import runtime
from . import security
class ResourceType(enum.Enum):
'''
Resource type as it was perceived by the rendering engine.
'''
DOCUMENT = "Document"
STYLESHEET = "Stylesheet"
IMAGE = "Image"
MEDIA = "Media"
FONT = "Font"
SCRIPT = "Script"
TEXT_TRACK = "TextTrack"
XHR = "XHR"
FETCH = "Fetch"
PREFETCH = "Prefetch"
EVENT_SOURCE = "EventSource"
WEB_SOCKET = "WebSocket"
MANIFEST = "Manifest"
SIGNED_EXCHANGE = "SignedExchange"
PING = "Ping"
CSP_VIOLATION_REPORT = "CSPViolationReport"
PREFLIGHT = "Preflight"
OTHER = "Other"
def to_json(self):
return self.value
@classmethod
def from_json(cls, json):
return cls(json)
class LoaderId(str):
'''
Unique loader identifier.
'''
def to_json(self) -> str:
return self
@classmethod
def from_json(cls, json: str) -> LoaderId:
return cls(json)
def __repr__(self):
return 'LoaderId({})'.format(super().__repr__())
class RequestId(str):
'''
Unique request identifier.
'''
def to_json(self) -> str:
return self
@classmethod
def from_json(cls, json: str) -> RequestId:
return cls(json)
def __repr__(self):
return 'RequestId({})'.format(super().__repr__())
class InterceptionId(str):
'''
Unique intercepted request identifier.
'''
def to_json(self) -> str:
return self
@classmethod
def from_json(cls, json: str) -> InterceptionId:
return cls(json)
def __repr__(self):
return 'InterceptionId({})'.format(super().__repr__())
class ErrorReason(enum.Enum):
'''
Network level fetch failure reason.
'''
FAILED = "Failed"
ABORTED = "Aborted"
TIMED_OUT = "TimedOut"
ACCESS_DENIED = "AccessDenied"
CONNECTION_CLOSED = "ConnectionClosed"
CONNECTION_RESET = "ConnectionReset"
CONNECTION_REFUSED = "ConnectionRefused"
CONNECTION_ABORTED = "ConnectionAborted"
CONNECTION_FAILED = "ConnectionFailed"
NAME_NOT_RESOLVED = "NameNotResolved"
INTERNET_DISCONNECTED = "InternetDisconnected"
ADDRESS_UNREACHABLE = "AddressUnreachable"
BLOCKED_BY_CLIENT = "BlockedByClient"
BLOCKED_BY_RESPONSE = "BlockedByResponse"
def to_json(self):
return self.value
@classmethod
def from_json(cls, json):
return cls(json)
class TimeSinceEpoch(float):
'''
UTC time in seconds, counted from January 1, 1970.
'''
def to_json(self) -> float:
return self
@classmethod
def from_json(cls, json: float) -> TimeSinceEpoch:
return cls(json)
def __repr__(self):
return 'TimeSinceEpoch({})'.format(super().__repr__())
class MonotonicTime(float):
'''
Monotonically increasing time in seconds since an arbitrary point in the past.
'''
def to_json(self) -> float:
return self
@classmethod
def from_json(cls, json: float) -> MonotonicTime:
return cls(json)
def __repr__(self):
return 'MonotonicTime({})'.format(super().__repr__())
class Headers(dict):
'''
Request / response headers as keys / values of JSON object.
'''
def to_json(self) -> dict:
return self
@classmethod
def from_json(cls, json: dict) -> Headers:
return cls(json)
def __repr__(self):
return 'Headers({})'.format(super().__repr__())
class ConnectionType(enum.Enum):
'''
The underlying connection technology that the browser is supposedly using.
'''
NONE = "none"
CELLULAR2G = "cellular2g"
CELLULAR3G = "cellular3g"
CELLULAR4G = "cellular4g"
BLUETOOTH = "bluetooth"
ETHERNET = "ethernet"
WIFI = "wifi"
WIMAX = "wimax"
OTHER = "other"
def to_json(self):
return self.value
@classmethod
def from_json(cls, json):
return cls(json)
class CookieSameSite(enum.Enum):
'''
Represents the cookie's 'SameSite' status:
https://tools.ietf.org/html/draft-west-first-party-cookies
'''
STRICT = "Strict"
LAX = "Lax"
NONE = "None"
def to_json(self):
return self.value
@classmethod
def from_json(cls, json):
return cls(json)
class CookiePriority(enum.Enum):
'''
Represents the cookie's 'Priority' status:
https://tools.ietf.org/html/draft-west-cookie-priority-00
'''
LOW = "Low"
MEDIUM = "Medium"
HIGH = "High"
def to_json(self):
return self.value
@classmethod
def from_json(cls, json):
return cls(json)
class CookieSourceScheme(enum.Enum):
'''
Represents the source scheme of the origin that originally set the cookie.
A value of "Unset" allows protocol clients to emulate legacy cookie scope for the scheme.
This is a temporary ability and it will be removed in the future.
'''
UNSET = "Unset"
NON_SECURE = "NonSecure"
SECURE = "Secure"
def to_json(self):
return self.value
@classmethod
def from_json(cls, json):
return cls(json)
@dataclass
class ResourceTiming:
'''
Timing information for the request.
'''
#: Timing's requestTime is a baseline in seconds, while the other numbers are ticks in
#: milliseconds relatively to this requestTime.
request_time: float
#: Started resolving proxy.
proxy_start: float
#: Finished resolving proxy.
proxy_end: float
#: Started DNS address resolve.
dns_start: float
#: Finished DNS address resolve.
dns_end: float
#: Started connecting to the remote host.
connect_start: float
#: Connected to the remote host.
connect_end: float
#: Started SSL handshake.
ssl_start: float
#: Finished SSL handshake.
ssl_end: float
#: Started running ServiceWorker.
worker_start: float
#: Finished Starting ServiceWorker.
worker_ready: float
#: Started fetch event.
worker_fetch_start: float
#: Settled fetch event respondWith promise.
worker_respond_with_settled: float
#: Started sending request.
send_start: float
#: Finished sending request.
send_end: float
#: Time the server started pushing request.
push_start: float
#: Time the server finished pushing request.
push_end: float
#: Started receiving response headers.
receive_headers_start: float
#: Finished receiving response headers.
receive_headers_end: float
def to_json(self):
json = dict()
json['requestTime'] = self.request_time
json['proxyStart'] = self.proxy_start
json['proxyEnd'] = self.proxy_end
json['dnsStart'] = self.dns_start
json['dnsEnd'] = self.dns_end
json['connectStart'] = self.connect_start
json['connectEnd'] = self.connect_end
json['sslStart'] = self.ssl_start
json['sslEnd'] = self.ssl_end
json['workerStart'] = self.worker_start
json['workerReady'] = self.worker_ready
json['workerFetchStart'] = self.worker_fetch_start
json['workerRespondWithSettled'] = self.worker_respond_with_settled
json['sendStart'] = self.send_start
json['sendEnd'] = self.send_end
json['pushStart'] = self.push_start
json['pushEnd'] = self.push_end
json['receiveHeadersStart'] = self.receive_headers_start
json['receiveHeadersEnd'] = self.receive_headers_end
return json
@classmethod
def from_json(cls, json):
return cls(
request_time=float(json['requestTime

程序员Chino的日记
- 粉丝: 3774
- 资源: 5万+
最新资源
- gnutls-devel-3.3.29-9.el7-6.x64-86.rpm.tar.gz
- 二自由度整车模型:状态空间方程与微分方程建模,质心侧偏角与横摆角速度分析,参数自定义及说明文档,二自由度整车模型 资料包含状态空间方程、微分方程两种建模方式 输入为前轮转角,输出为质心侧偏角、横摆角速
- gnutls-utils-3.3.29-9.el7-6.x64-86.rpm.tar.gz
- gob2-2.0.19-4.el7.x64-86.rpm.tar.gz
- 2cd36c672d44edff9777d9256b1ca68c.part07
- gobject-introspection-1.56.1-1.el7.x64-86.rpm.tar.gz
- 蓄电池与超级电容混合储能系统的Simulink能量管理仿真模型研究,蓄电池超级电容混合储能系统simulink能量管理仿真模型 ,核心关键词:蓄电池; 超级电容; 混合储能系统; 能量管理; Sim
- gobject-introspection-devel-1.56.1-1.el7.x64-86.rpm.tar.gz
- 基于共享储能电站的工业用户经济调度优化方法与实证研究,基于共享储能电站的工业用户日前优化经济调度方法 文献复现 首先提出共享储能电站的概念,分析其商业运营模式 然后将共享储能电站应用到工业用户经济优
- gom-0.4-1.el7.x64-86.rpm.tar.gz
- gom-devel-0.4-1.el7.x64-86.rpm.tar.gz
- 基于三菱PLC与组态王技术的恒温加热炉温度控制系统设计:梯形图程序、接线图与组态画面详解,基于三菱PLC和组态王恒温控制系统的设计加热炉温度控制 带解释的梯形图程序,接线图原理图图纸,io分配,组态画
- gomtree-0.5.0-0.2.git16da0f8.el7.x64-86.rpm.tar.gz
- google-guice-3.1.3-9.el7.x64-86.rpm.tar.gz
- scratchMinecraft
- 光伏电池Matlab Simulink仿真模型:灵活模拟输出特性,自定义光照强度和温度下的U-P、U-I曲线,光伏电池模型 Matlab Simulink仿真模型(成品) 模拟了光伏电池的输出特性,可
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


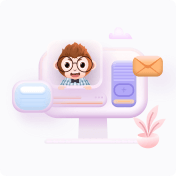