"""High level plotting functions using matplotlib."""
# Except in strange circumstances, all functions in this module
# should take an ``ax`` keyword argument defaulting to None
# (which creates a new subplot) and an open-ended **kwargs to
# pass to the underlying matplotlib function being called.
# They should also return the ``ax`` object.
from __future__ import division
import colorsys
import itertools
import numpy as np
import pandas as pd
from scipy import stats, interpolate
import statsmodels.api as sm
import statsmodels.formula.api as sf
import matplotlib as mpl
import matplotlib.pyplot as plt
import moss
from seaborn.utils import (color_palette, ci_to_errsize,
husl_palette, desaturate)
def tsplot(x, data, err_style="ci_band", ci=68, interpolate=True,
estimator=np.mean, n_boot=10000, smooth=False,
err_palette=None, ax=None, err_kws=None, **kwargs):
"""Plot timeseries from a set of observations.
Parameters
----------
x : n_tp array
x values
data : n_obs x n_tp array
array of timeseries data where first axis is observations. other
objects (e.g. DataFrames) are converted to an array if possible
err_style : string or list of strings
names of ways to plot uncertainty across observations from set of
{ci_band, ci_bars, boot_traces, book_kde, obs_traces, obs_points}
ci : int or list of ints
confidence interaval size(s). if a list, it will stack the error
plots for each confidence interval
estimator : callable
function to determine centralt tendency and to pass to bootstrap
must take an ``axis`` argument
n_boot : int
number of bootstrap iterations
smooth : boolean
whether to perform a smooth bootstrap (resample from KDE)
ax : axis object, optional
plot in given axis; if None creates a new figure
err_kws : dict, optional
keyword argument dictionary passed through to matplotlib
function generating the error plot
kwargs : further keyword arguments for main call to plot()
Returns
-------
ax : matplotlib axis
axis with plot data
"""
if ax is None:
ax = plt.subplot(111)
if err_kws is None:
err_kws = {}
# Bootstrap the data for confidence intervals
data = np.asarray(data)
boot_data = moss.bootstrap(data, n_boot=n_boot, smooth=smooth,
axis=0, func=estimator)
ci_list = hasattr(ci, "__iter__")
if not ci_list:
ci = [ci]
ci_vals = [(50 - w / 2, 50 + w / 2) for w in ci]
cis = [moss.percentiles(boot_data, ci, axis=0) for ci in ci_vals]
central_data = estimator(data, axis=0)
# Plot the timeseries line to get its color
line, = ax.plot(x, central_data, **kwargs)
color = line.get_color()
line.remove()
kwargs.pop("color", None)
# Use subroutines to plot the uncertainty
if not hasattr(err_style, "__iter__"):
err_style = [err_style]
for style in err_style:
# Grab the function from the global environment
try:
plot_func = globals()["_plot_%s" % style]
except KeyError:
raise ValueError("%s is not a valid err_style" % style)
# Possibly set up to plot each observation in a different color
if err_palette is not None and "obs" in style:
orig_color = color
color = color_palette(err_palette, len(data), desat=.99)
plot_kwargs = dict(ax=ax, x=x, data=data,
boot_data=boot_data,
central_data=central_data,
color=color, err_kws=err_kws)
for ci_i in cis:
plot_kwargs["ci"] = ci_i
plot_func(**plot_kwargs)
if err_palette is not None and "obs" in style:
color = orig_color
# Replot the central trace so it is prominent
marker = kwargs.pop("marker", "" if interpolate else "o")
linestyle = kwargs.pop("linestyle", "-" if interpolate else "")
ax.plot(x, central_data, color=color,
marker=marker, linestyle=linestyle, **kwargs)
return ax
# Subroutines for tsplot errorbar plotting
# ----------------------------------------
def _plot_ci_band(ax, x, ci, color, err_kws, **kwargs):
"""Plot translucent error bands around the central tendancy."""
low, high = ci
ax.fill_between(x, low, high, color=color, alpha=0.2, **err_kws)
def _plot_ci_bars(ax, x, central_data, ci, color, err_kws, **kwargs):
"""Plot error bars at each data point."""
err = ci_to_errsize(ci, central_data)
ax.errorbar(x, central_data, yerr=err, fmt=None, ecolor=color,
label="_nolegend_", **err_kws)
def _plot_boot_traces(ax, x, boot_data, color, err_kws, **kwargs):
"""Plot 250 traces from bootstrap."""
ax.plot(x, boot_data[:250].T, color=color, alpha=0.25,
linewidth=0.25, label="_nolegend_", **err_kws)
def _plot_obs_traces(ax, x, data, ci, color, err_kws, **kwargs):
"""Plot a trace for each observation in the original data."""
if isinstance(color, list):
for i, obs in enumerate(data):
ax.plot(x, obs, color=color[i], alpha=0.5,
label="_nolegend_", **err_kws)
else:
ax.plot(x, data.T, color=color, alpha=0.2,
label="_nolegend_", **err_kws)
def _plot_obs_points(ax, x, data, color, err_kws, **kwargs):
"""Plot each original data point discretely."""
if isinstance(color, list):
for i, obs in enumerate(data):
ax.plot(x, obs, "o", color=color[i], alpha=0.8, markersize=4,
label="_nolegend_", **err_kws)
else:
ax.plot(x, data.T, "o", color=color, alpha=0.5, markersize=4,
label="_nolegend_", **err_kws)
def _plot_boot_kde(ax, x, boot_data, color, **kwargs):
"""Plot the kernal density estimate of the bootstrap distribution."""
kwargs.pop("data")
_ts_kde(ax, x, boot_data, color, **kwargs)
def _plot_obs_kde(ax, x, data, color, **kwargs):
"""Plot the kernal density estimate over the sample."""
_ts_kde(ax, x, data, color, **kwargs)
def _ts_kde(ax, x, data, color, **kwargs):
"""Upsample over time and plot a KDE of the bootstrap distribution."""
kde_data = []
y_min, y_max = moss.percentiles(data, [1, 99])
y_vals = np.linspace(y_min, y_max, 100)
upsampler = interpolate.interp1d(x, data)
data_upsample = upsampler(np.linspace(x.min(), x.max(), 100))
for pt_data in data_upsample.T:
pt_kde = stats.kde.gaussian_kde(pt_data)
kde_data.append(pt_kde(y_vals))
kde_data = np.transpose(kde_data)
rgb = mpl.colors.ColorConverter().to_rgb(color)
img = np.zeros((kde_data.shape[0], kde_data.shape[1], 4))
img[:, :, :3] = rgb
kde_data /= kde_data.max(axis=0)
kde_data[kde_data > 1] = 1
img[:, :, 3] = kde_data
ax.imshow(img, interpolation="spline16", zorder=1,
extent=(x.min(), x.max(), y_min, y_max),
aspect="auto", origin="lower")
def lmplot(x, y, data, color=None, row=None, col=None, col_wrap=None,
x_estimator=None, x_ci=95, n_boot=5000, fit_reg=True,
order=1, ci=95, logistic=False, truncate=False,
x_partial=None, y_partial=None, x_jitter=None, y_jitter=None,
sharex=True, sharey=True, palette="husl", size=None,
scatter_kws=None, line_kws=None, palette_kws=None):
"""Plot a linear model from a DataFrame.
Parameters
----------
x, y : strings
column names in `data` DataFrame for x and y variables
data : DataFrame
source of data for the model
color : string, optional
DataFrame column name to group the model by color
row, col : strings, optional
DataFrame column names to make separate plot facets
col_wrap : int, optional
wrap col variable at this width - cannot be used with row fa

程序员Chino的日记
- 粉丝: 3718
- 资源: 5万+
最新资源
- 【深度学习专栏】ch05配套资源
- LCD1602自留备用,侵权删
- 基于Python的申请信用评分卡模型分析项目源码 (高分项目)
- Multisim仿真可编程彩灯控制器电路设计及其实现-含详细步骤和代码
- 漂亮的收款打赏要饭网HTML页面源码.zip
- HTTP与HTTPS协议对比及其安全性分析
- 动力电极耳压边除毛刺机(sw17可编辑+工程图+BOM)全套技术资料100%好用.zip
- 中文学习系统:用户体验与界面设计
- Python绘制圣诞树:文本和图形实现
- 方型锂电池卷绕机sw14可编辑全套技术资料100%好用.zip
- 学生宿舍管理系统:集成技术与住宿服务优化
- 大一C语言项目实践-小游戏集成开发系统
- 使用HTML、CSS和JavaScript实现动态3D圣诞树效果
- 基于STM32单片机的激光雕刻机控制系统设计-含详细步骤和代码
- 工业机械手ABB CRB1100(step)全套技术资料100%好用.zip
- C++语言实现动态圣诞树绘制
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


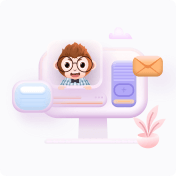