# pandas-stubs: Public type stubs for pandas
[](https://pypi.org/project/pandas-stubs/)
[](https://anaconda.org/conda-forge/pandas-stubs)
[](https://pypi.org/project/pandas-stubs/)
[](https://github.com/pandas-dev/pandas-stubs/blob/main/LICENSE)
[](https://pepy.tech/project/pandas-stubs)
[](https://gitter.im/pydata/pandas)
[](https://numfocus.org)
[](https://github.com/psf/black)
[](https://pycqa.github.io/isort/)
## What is it?
These are public type stubs for [**pandas**](http://pandas.pydata.org/), following the
convention of providing stubs in a separate package, as specified in [PEP 561](https://peps.python.org/pep-0561/#stub-only-packages). The stubs cover the most typical use cases of
pandas. In general, these stubs are narrower than what is possibly allowed by pandas,
but follow a convention of suggesting best recommended practices for using pandas.
The stubs are likely incomplete in terms of covering the published API of pandas.
The stubs are tested with [mypy](http://mypy-lang.org/) and [pyright](https://github.com/microsoft/pyright#readme) and are currently shipped with the Visual Studio Code extension
[pylance](https://github.com/microsoft/pylance-release#readme).
## Usage
Let’s take this example piece of code in file `round.py`
```python
import pandas as pd
decimals = pd.DataFrame({'TSLA': 2, 'AMZN': 1})
prices = pd.DataFrame(data={'date': ['2021-08-13', '2021-08-07', '2021-08-21'],
'TSLA': [720.13, 716.22, 731.22], 'AMZN': [3316.50, 3200.50, 3100.23]})
rounded_prices = prices.round(decimals=decimals)
```
Mypy won't see any issues with that, but after installing pandas-stubs and running it again:
```sh
mypy round.py
```
we get the following error message:
```text
round.py:6: error: Argument "decimals" to "round" of "DataFrame" has incompatible type "DataFrame"; expected "Union[int, Dict[Any, Any], Series[Any]]" [arg-type]
Found 1 error in 1 file (checked 1 source file)
```
And, if you use pyright:
```sh
pyright round.py
```
you get the following error message:
```text
round.py:6:40 - error: Argument of type "DataFrame" cannot be assigned to parameter "decimals" of type "int | Dict[Unknown, Unknown] | Series[Unknown]" in function "round"
Type "DataFrame" cannot be assigned to type "int | Dict[Unknown, Unknown] | Series[Unknown]"
"DataFrame" is incompatible with "int"
"DataFrame" is incompatible with "Dict[Unknown, Unknown]"
"DataFrame" is incompatible with "Series[Unknown]" (reportGeneralTypeIssues)
```
And after confirming with the [docs](https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.round.html)
we can fix the code:
```python
decimals = pd.Series({'TSLA': 2, 'AMZN': 1})
```
## Version Numbering Convention
The version number x.y.z.yymmdd corresponds to a test done with pandas version x.y.z, with the stubs released on the date mm/yy/dd.
It is anticipated that the stubs will be released more frequently than pandas as the stubs are expected to evolve due to more
public visibility.
## Where to get it
The source code is currently hosted on GitHub at: <https://github.com/pandas-dev/pandas-stubs>
Binary installers for the latest released version are available at the [Python
Package Index (PyPI)](https://pypi.org/project/pandas-stubs) and on [conda-forge](https://conda-forge.org/).
```sh
# conda
conda install pandas-stubs
```
```sh
# or PyPI
pip install pandas-stubs
```
## Dependencies
- [pandas: powerful Python data analysis toolkit](https://pandas.pydata.org/)
- [typing-extensions >= 4.2.0 - supporting the latest typing extensions](https://github.com/python/typing_extensions#readme)
## Installation from sources
- Make sure you have `python >= 3.8` installed.
- Install poetry
```sh
# conda
conda install poetry
```
```sh
# or PyPI
pip install poetry
```
- Install the project dependencies
```sh
poetry update -vvv
```
- Build and install the distribution
```sh
poetry run poe build_dist
poetry run poe install_dist
```
## License
[BSD 3](LICENSE)
## Documentation
Documentation is a work-in-progress.
## Background
These stubs are the result of a strategic effort lead by the core pandas team to integrate [Microsoft type stub repository](https://github.com/microsoft/python-type-stubs)
together with the [VirtusLabs pandas_stubs repository](https://github.com/VirtusLab/pandas-stubs).
These stubs were initially forked from the Microsoft project <https://github.com/microsoft/python-type-stubs> as of [this commit](https://github.com/microsoft/python-type-stubs/tree/6b800063bde687cd1846122431e2a729a9de625a).
We are indebted to Microsoft and that project for the initial set of public type stubs. We are also grateful for the original pandas-stubs project at <https://github.com/VirtusLab/pandas-stubs> that created the framework for testing the stubs.
## Differences between type declarations in pandas and pandas-stubs
The <https://github.com/pandas-dev/pandas/> project has type declarations for some parts of pandas, both for the internal and public API's. Those type declarations are used to make sure that the pandas code is _internally_ consistent.
The <https://github.com/pandas-dev/pandas-stubs/> project provides type declarations for the pandas _public_ API. The philosophy of these stubs can be found at <https://github.com/pandas-dev/pandas-stubs/blob/main/docs/philosophy.md/> While it would be ideal if the `pyi` files in this project would be part of the `pandas` distribution, this would require consistency between the internal type declarations and the public declarations, and the scope of a project to create that consistency is quite large. That is a long term goal. Finally, another goal is to do more frequent releases of the pandas-stubs than is done for pandas, in order to make the stubs more useful.
If issues are found with the public stubs, pull requests to correct those issues are welcome. In addition, pull requests on the pandas repository to fix the same issue are welcome there as well. However, since the goals of typing in the two projects are different (internal consistency vs. public usage), it may be a challenge to create consistent type declarations across both projects. See <https://pandas.pydata.org/docs/development/contributing_codebase.html#type-hints/> for a discussion of typing standards used within the pandas code.
## Getting help
Ask questions and report issues on the [pandas-stubs repository](https://github.com/pandas-dev/pandas-stubs/issues).
## Discussion and Development
Most development discussions take place on GitHub in the [pandas-stubs repository](https://github.com/pandas-dev/pandas-stubs/). Further, the [pandas-dev mailing list](https://mail.python.org/mailman/listinfo/pandas-dev) can also be used for specialized discussions or design issues, and a [Gitter channel](https://gitter.im/pydata/pandas) is available for quick development related questions.
## Contributing to pandas-stubs
All contributions, bu
没有合适的资源?快使用搜索试试~ 我知道了~
pandas_stubs-1.5.3.230304.tar.gz
需积分: 1 0 下载量 57 浏览量
2024-03-17
13:29:13
上传
评论
收藏 94KB GZ 举报
温馨提示
Python库是一组预先编写的代码模块,旨在帮助开发者实现特定的编程任务,无需从零开始编写代码。这些库可以包括各种功能,如数学运算、文件操作、数据分析和网络编程等。Python社区提供了大量的第三方库,如NumPy、Pandas和Requests,极大地丰富了Python的应用领域,从数据科学到Web开发。Python库的丰富性是Python成为最受欢迎的编程语言之一的关键原因之一。这些库不仅为初学者提供了快速入门的途径,而且为经验丰富的开发者提供了强大的工具,以高效率、高质量地完成复杂任务。例如,Matplotlib和Seaborn库在数据可视化领域内非常受欢迎,它们提供了广泛的工具和技术,可以创建高度定制化的图表和图形,帮助数据科学家和分析师在数据探索和结果展示中更有效地传达信息。
资源推荐
资源详情
资源评论
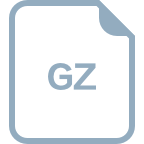
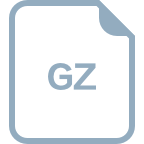
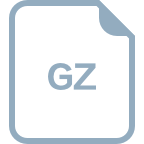
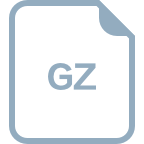
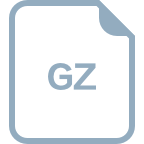
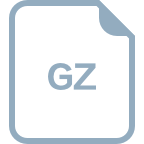
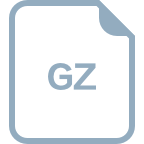
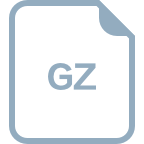
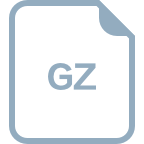
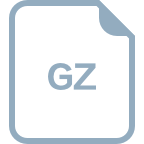
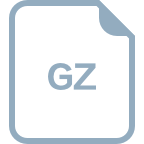
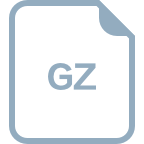
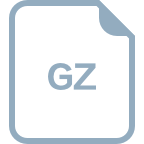
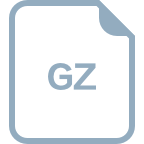
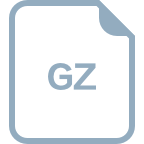
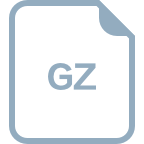
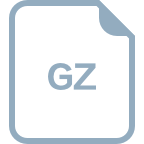
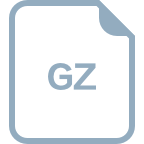
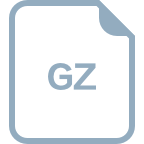
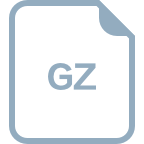
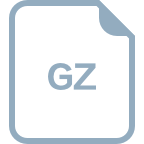
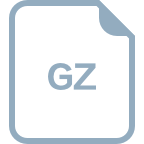
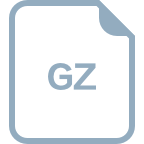
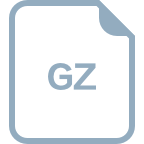
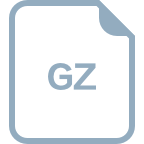
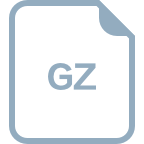
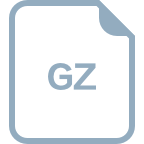
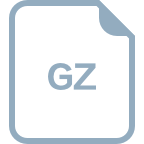
收起资源包目录

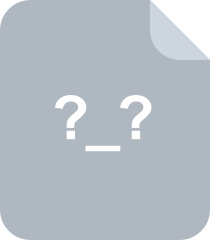
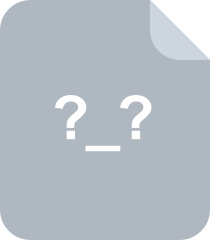
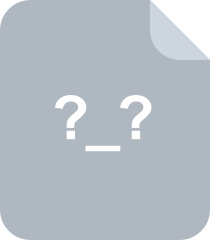
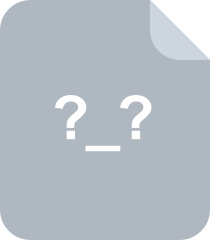
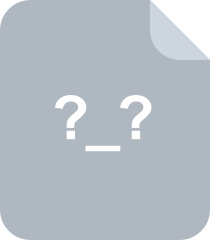
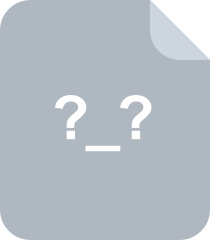
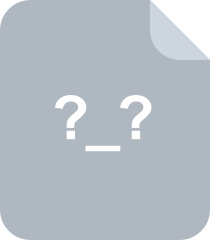
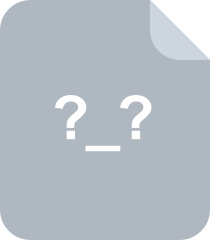
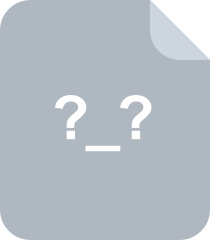
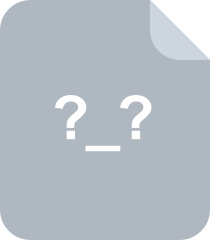
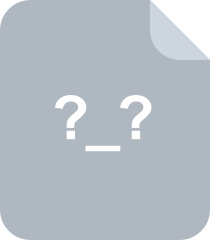
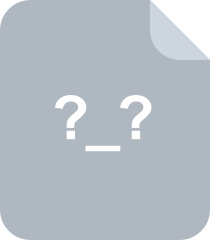
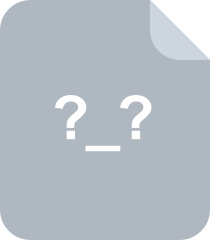
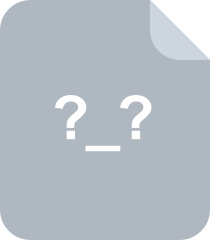
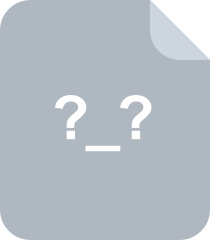
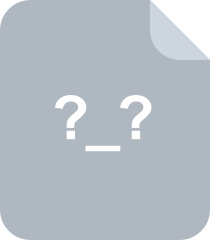
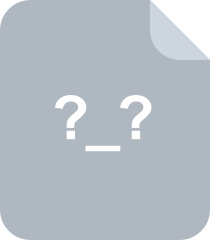
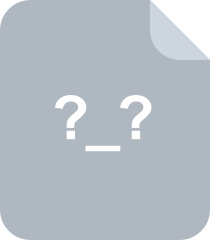
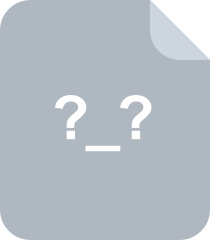
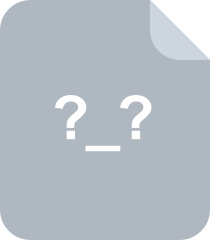
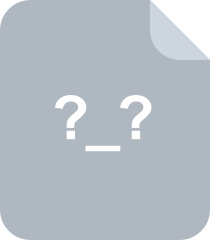
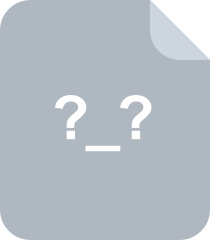
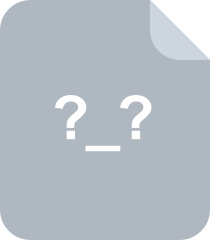
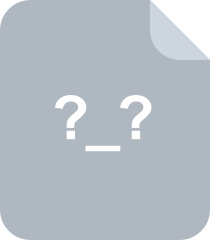
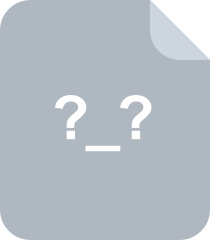
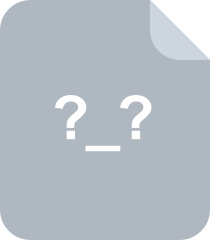
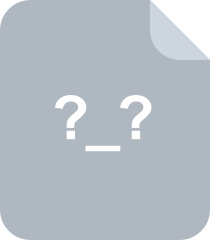
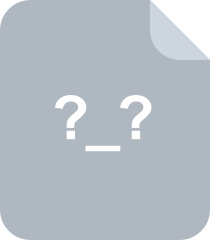
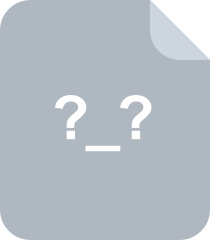
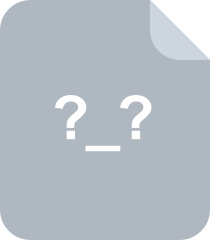
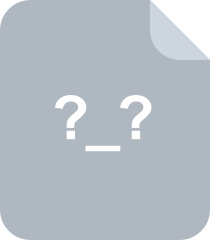
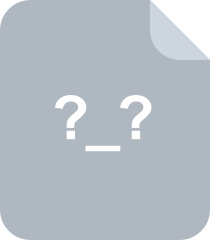
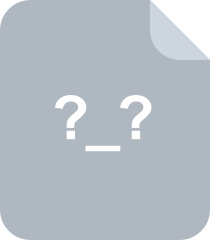
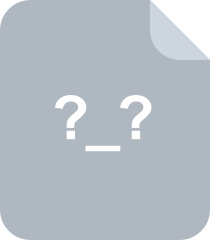
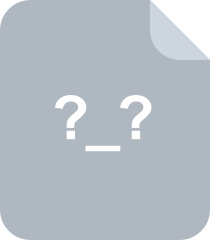
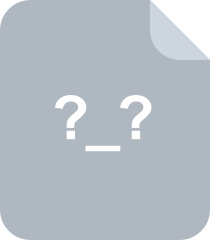
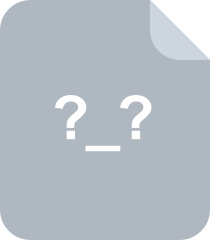
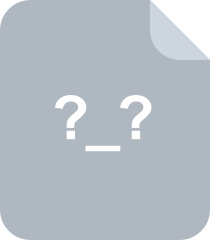
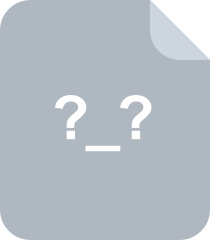
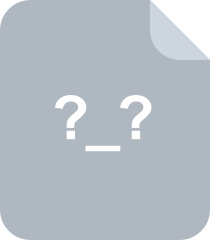
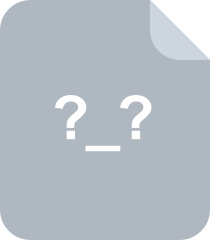
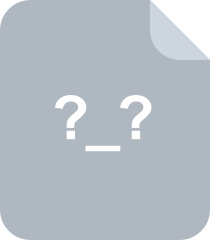
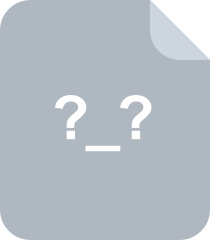
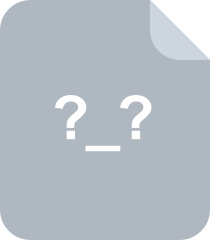
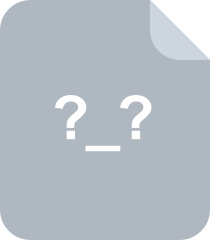
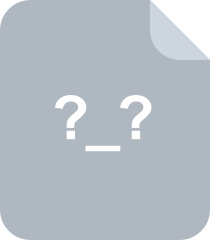
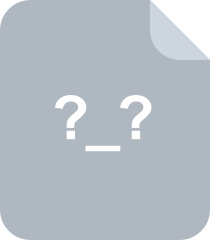
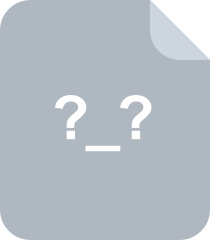
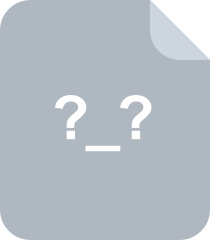
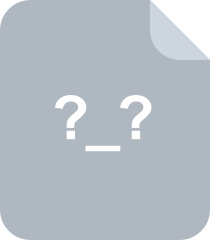
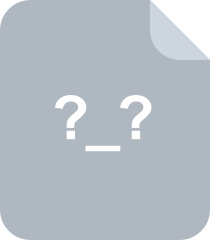
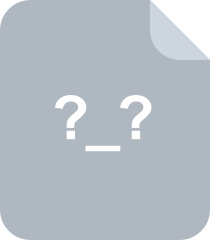
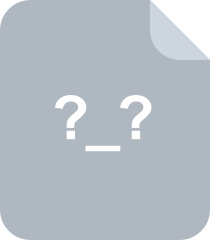
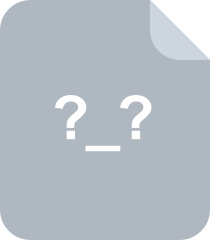
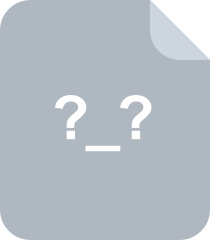
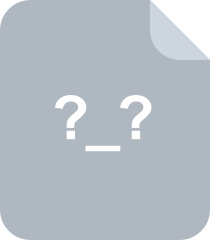
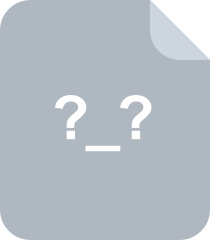
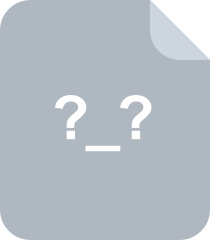
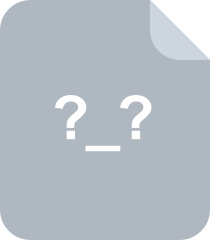
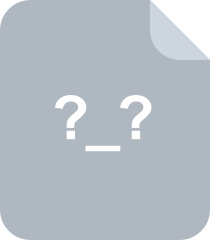
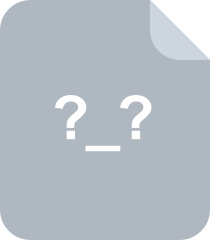
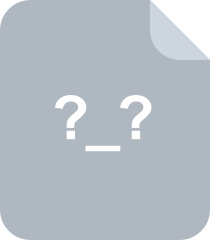
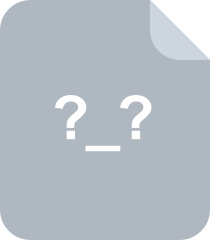
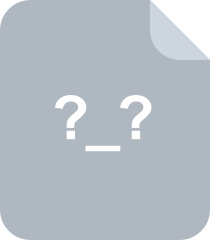
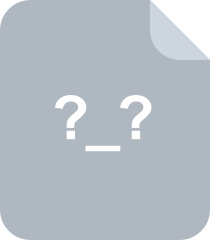
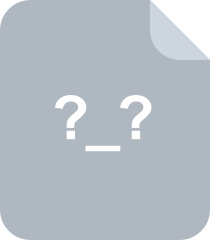
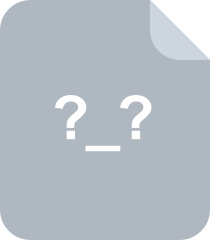
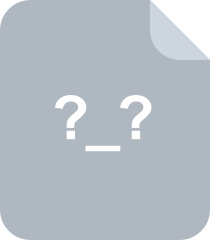
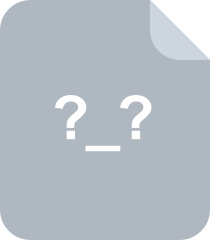
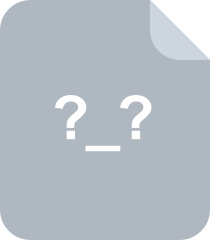
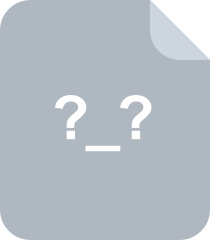
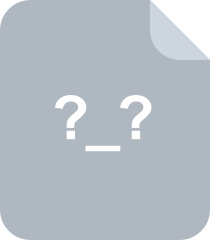
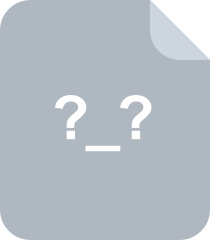
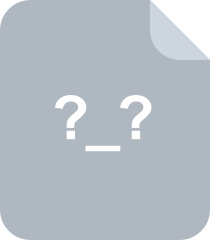
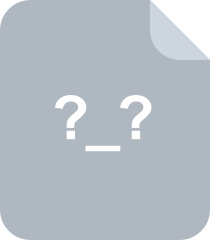
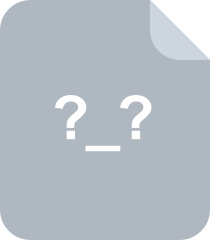
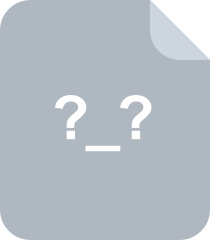
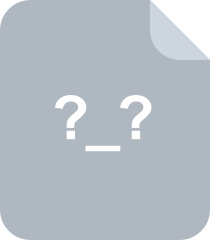
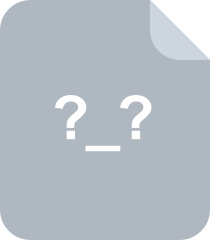
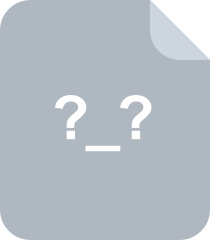
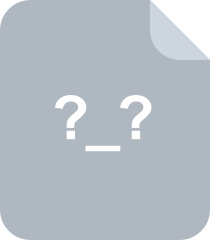
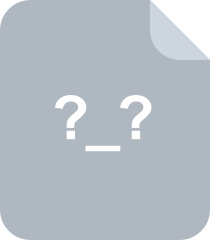
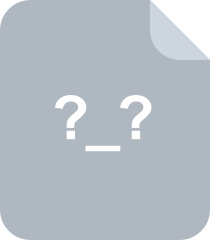
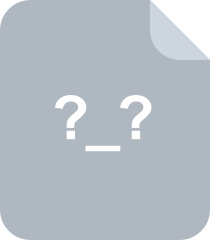
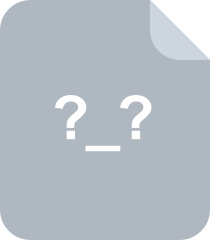
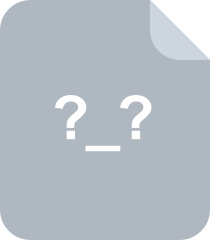
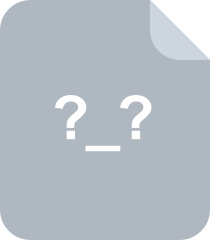
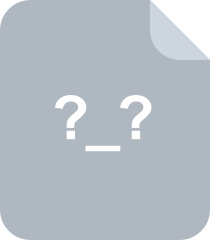
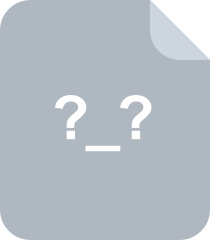
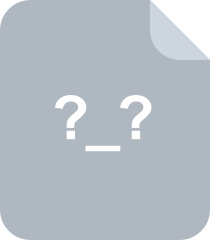
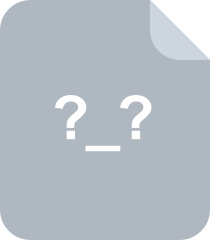
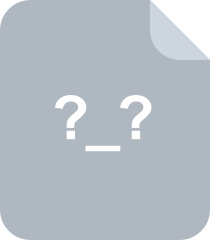
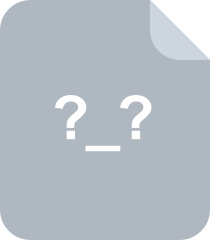
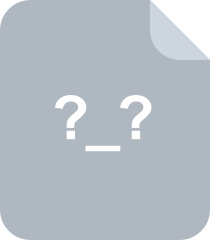
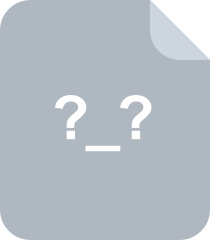
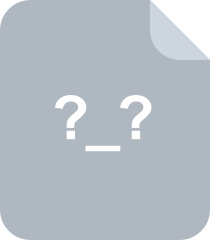
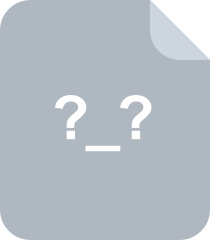
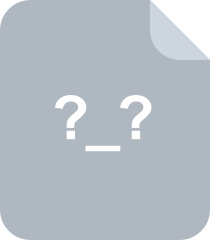
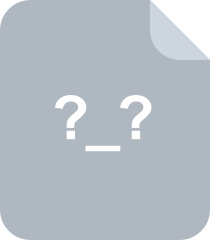
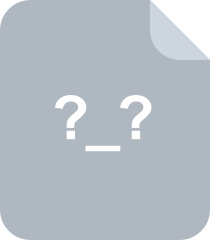
共 206 条
- 1
- 2
- 3
资源评论
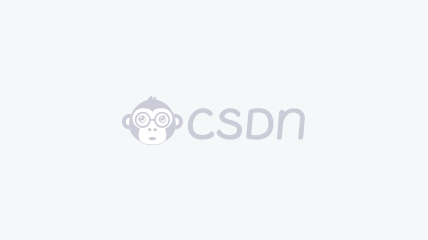

程序员Chino的日记
- 粉丝: 3719
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

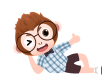
最新资源
- 白色大气风格的珠宝首饰电商模板下载.zip
- 白色大气风格的装饰装修模板下载.zip
- 白色大气风格的自行车运动模板下载.zip
- 白色大气风格的自由搏击俱乐部模板下载.zip
- 白色大气风格响应式app应用程序企业网站模板.zip
- 白色大气风格的足球俱乐部HTML5网站模板.zip
- 白色大气风格响应式IT技术在线企业网站模板.zip
- 白色大气风格响应式彩绘精品水果网站模板.zip
- 白色大气风格响应式大图幻灯博客网站模板.zip
- 白色大气风格响应式产品展示企业网页模板.zip
- 白色大气风格响应式个人主页博客网站模板.zip
- 白色大气风格响应式浪漫集体婚礼企业网站模板.zip
- 白色大气风格响应式果蔬类种植企业网站模板.zip
- 白色大气风格响应式通用后台管理网站模板.zip
- 白色大气风格响应式项目团队动态企业网站模板.zip
- 白色大气风格响应式旅游资讯企业网站模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


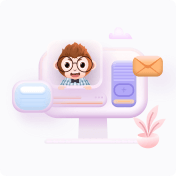
安全验证
文档复制为VIP权益,开通VIP直接复制
